The Kraken API provides developers with a powerful interface to one of the world's leading cryptocurrency exchanges. Whether you're building trading applications, monitoring markets, or managing cryptocurrency portfolios, this guide will help you navigate the essentials of Kraken's API capabilities and implementation approaches.
What is the Kraken API?
Kraken's API allows developers to programmatically access the exchange's functionality through a RESTful interface. With this API, you can:
- Access real-time market data (prices, order books, trades)
- Place and manage trading orders
- Monitor account balances and positions
- Process deposits and withdrawals
- Access historical trading data
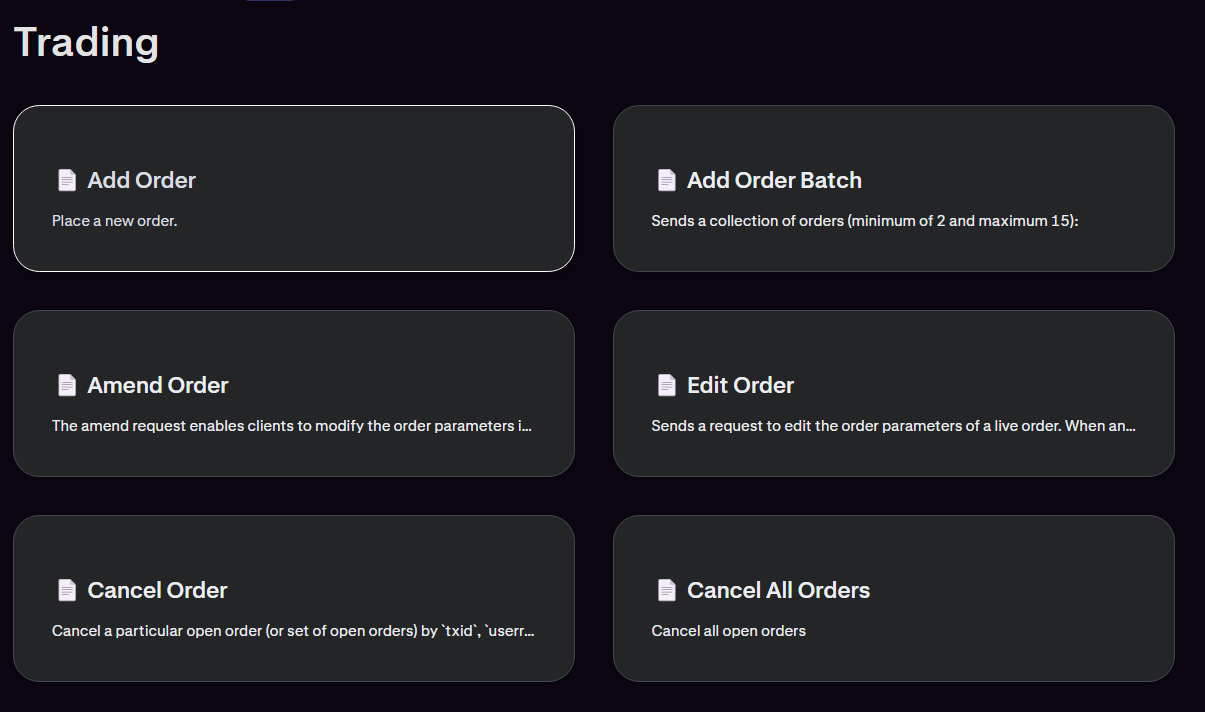
Setting Up for API Access
Creating Your Kraken Account
Before using the API, you'll need to:
- Sign up for a Kraken account at kraken.com
- Complete verification requirements based on your usage needs
- Enable two-factor authentication (2FA) for enhanced security
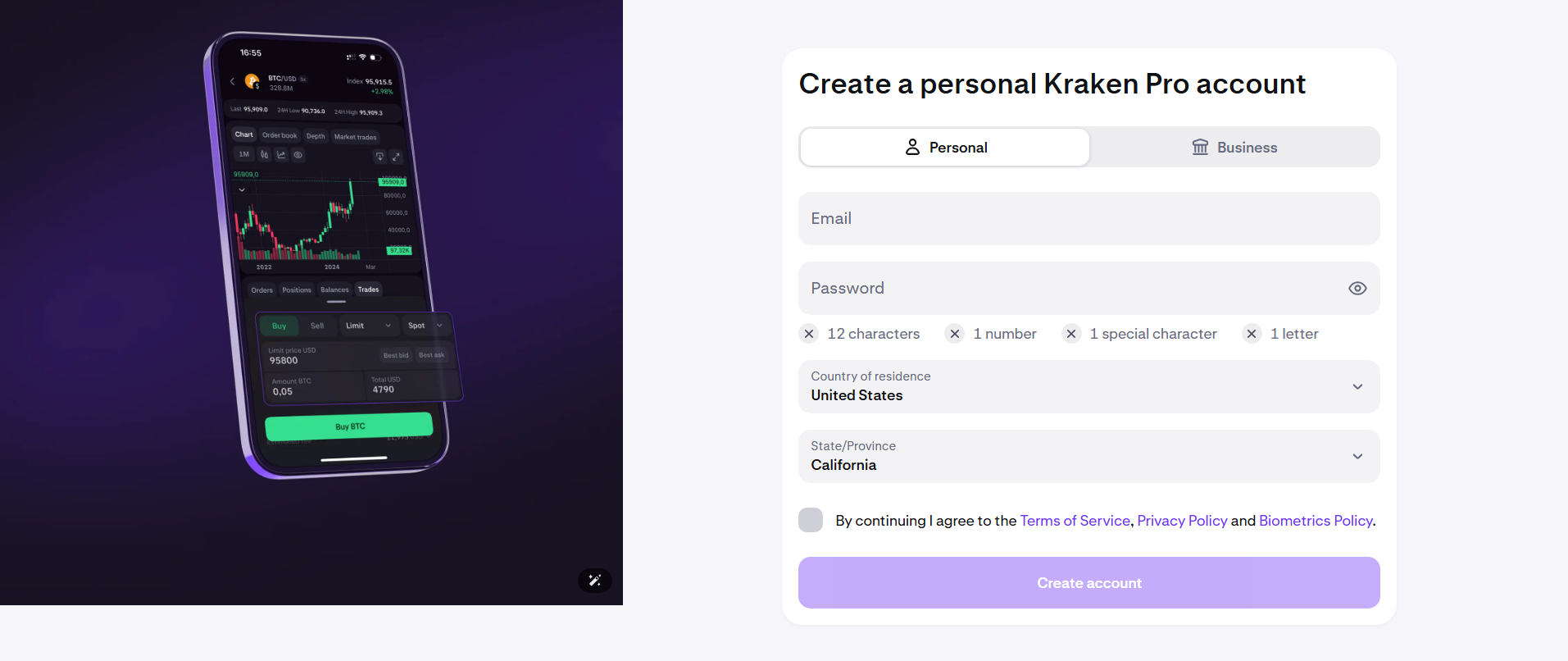
Generating API Keys
To interact with the API, create API keys with appropriate permissions:
- Log in to your Kraken account
- Navigate to Connection & API
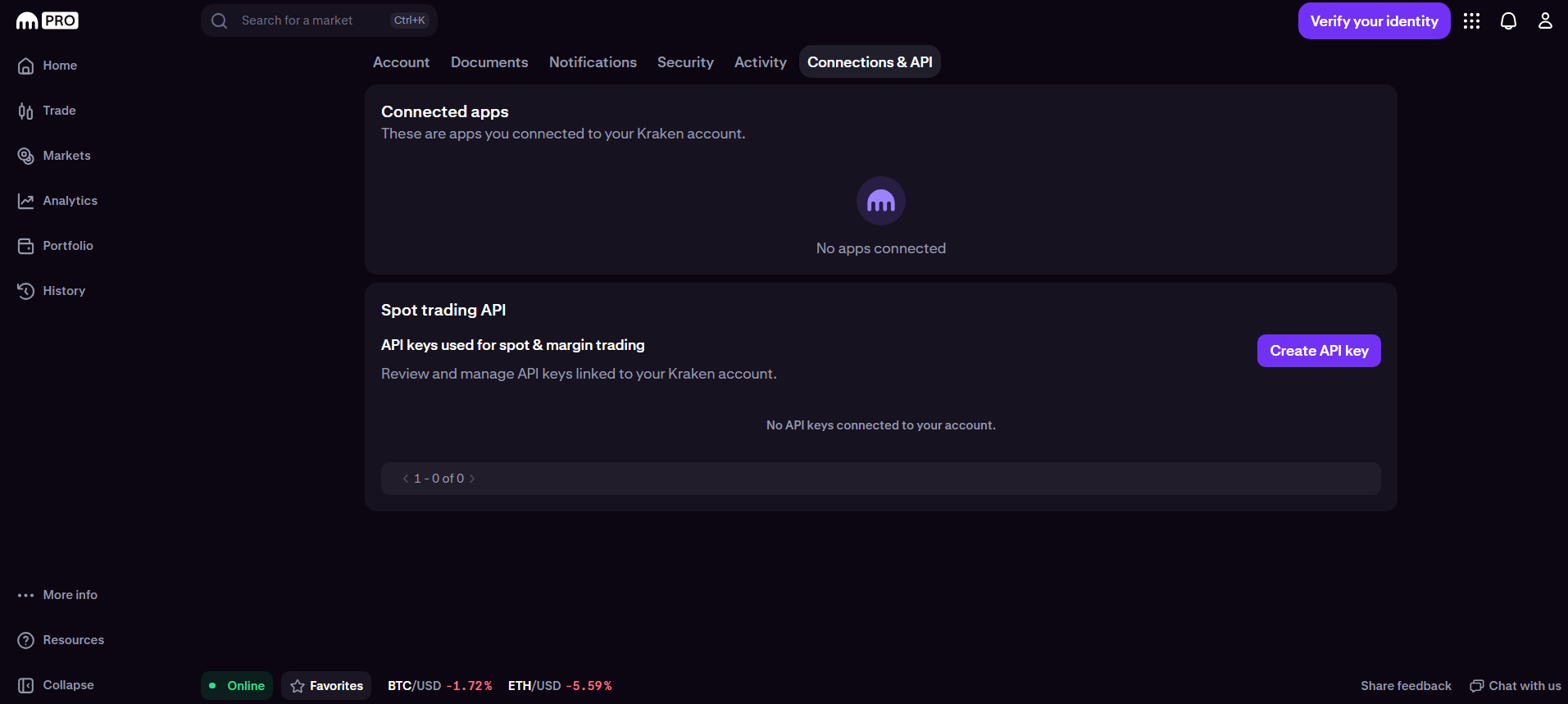
3. Click Generate New Key
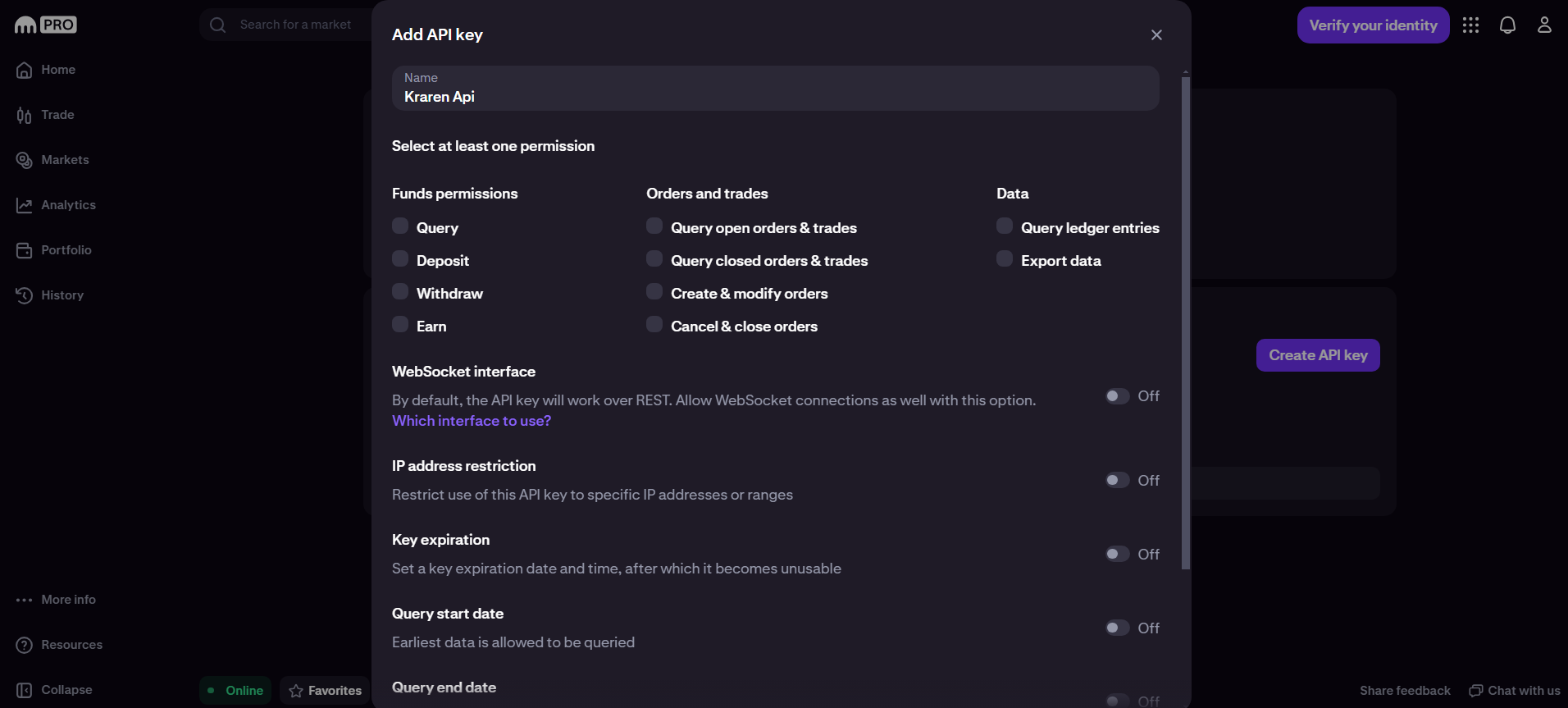
4. Set appropriate permissions:
- Query: Read-only access to account information
- Trade: Ability to place and cancel orders
- Deposit: Generate deposit addresses
- Withdraw: Create withdrawal requests
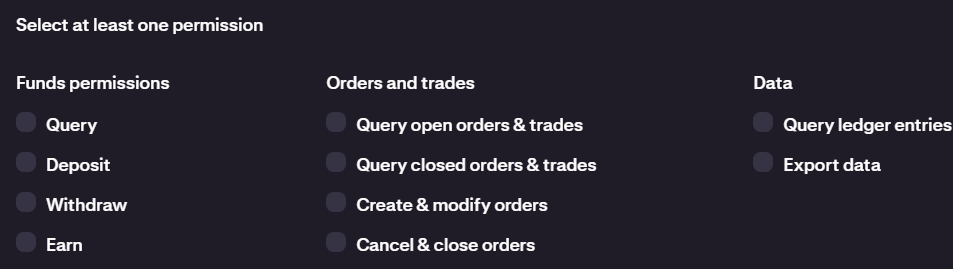
⚠️ SECURITY WARNING
- Store API keys securely and never share your private key
- Restrict API key permissions to only what's necessary
- Consider implementing IP address restrictions
Understanding the API Structure
The Kraken API is organized into a logical structure:
API Base URL and Endpoints
All API requests start with the base URL: https://api.kraken.com
The current API version is designated by /0/
in the URL path, followed by either:
- /public/ - Endpoints that don't require authentication
- /private/ - Endpoints that require authentication with your API key
Response Format
All API responses follow a standard JSON format:
{
"error": [],
"result": { /* Response data */ }
}
Making Your First API Requests
Public API Requests
Public endpoints provide market data without requiring authentication.
Example: Getting Ticker Information
import requests
# Get ticker information for Bitcoin in USD
response = requests.get('https://api.kraken.com/0/public/Ticker?pair=XBTUSD')
ticker_data = response.json()
if not ticker_data['error']:
btc_data = ticker_data['result']['XXBTZUSD']
print(f"BTC/USD Last Trade Price: {btc_data['c'][0]}")
print(f"BTC/USD 24h Volume: {btc_data['v'][1]}")
else:
print(f"Error: {ticker_data['error']}")
Example: Order Book Data
fetch('https://api.kraken.com/0/public/Depth?pair=ETHUSD&count=5')
.then(response => response.json())
.then(data => {
if (data.error.length === 0) {
const orderbook = data.result.XETHZUSD;
console.log("ETH/USD Order Book Top 5 Bids:", orderbook.bids);
console.log("ETH/USD Order Book Top 5 Asks:", orderbook.asks);
}
});
Private API Authentication
Private endpoints require authentication with your API keys through a process involving:
- Generating a nonce (increasing number)
- Creating a request signature using HMAC-SHA512
- Sending the request with your API key and signature
Authentication Implementation
import time
import base64
import hashlib
import hmac
import urllib.parse
import requests
def kraken_request(api_key, api_sec, endpoint, data=None):
"""Make an authenticated request to Kraken API"""
if data is None:
data = {}
api_url = "https://api.kraken.com"
# Add nonce to data
data['nonce'] = str(int(time.time() * 1000))
# Encode data for signature
encoded_data = urllib.parse.urlencode(data)
# Create signature
signature_data = (data['nonce'] + encoded_data).encode()
message = endpoint.encode() + hashlib.sha256(signature_data).digest()
signature = hmac.new(base64.b64decode(api_sec), message, hashlib.sha512)
signature_digest = base64.b64encode(signature.digest()).decode()
# Set headers
headers = {
'API-Key': api_key,
'API-Sign': signature_digest
}
# Send request
response = requests.post(api_url + endpoint, headers=headers, data=data)
return response.json()
Core API Functionality
Account Information
Checking Account Balance
balance = kraken_request(api_key, api_secret, "/0/private/Balance")
if not balance['error']:
for asset, amount in balance['result'].items():
print(f"{asset}: {amount}")
Order Management
Placing a Market Order
# Parameters for buying 0.01 BTC at market price
order_params = {
'pair': 'XBTUSD',
'type': 'buy',
'ordertype': 'market',
'volume': '0.01'
}
order_result = kraken_request(api_key, api_secret, "/0/private/AddOrder", order_params)
if not order_result['error']:
print(f"Order placed! Transaction ID: {order_result['result']['txid'][0]}")
Placing a Limit Order
# Parameters for selling 0.01 BTC at $50,000
limit_params = {
'pair': 'XBTUSD',
'type': 'sell',
'ordertype': 'limit',
'price': '50000',
'volume': '0.01'
}
limit_result = kraken_request(api_key, api_secret, "/0/private/AddOrder", limit_params)
Querying Open Orders
open_orders = kraken_request(api_key, api_secret, "/0/private/OpenOrders")
if not open_orders['error']:
orders = open_orders['result']['open']
for order_id, details in orders.items():
print(f"ID: {order_id}")
print(f"Type: {details['descr']['type']} {details['descr']['ordertype']}")
print(f"Pair: {details['descr']['pair']}")
Using APIdog with Kraken API
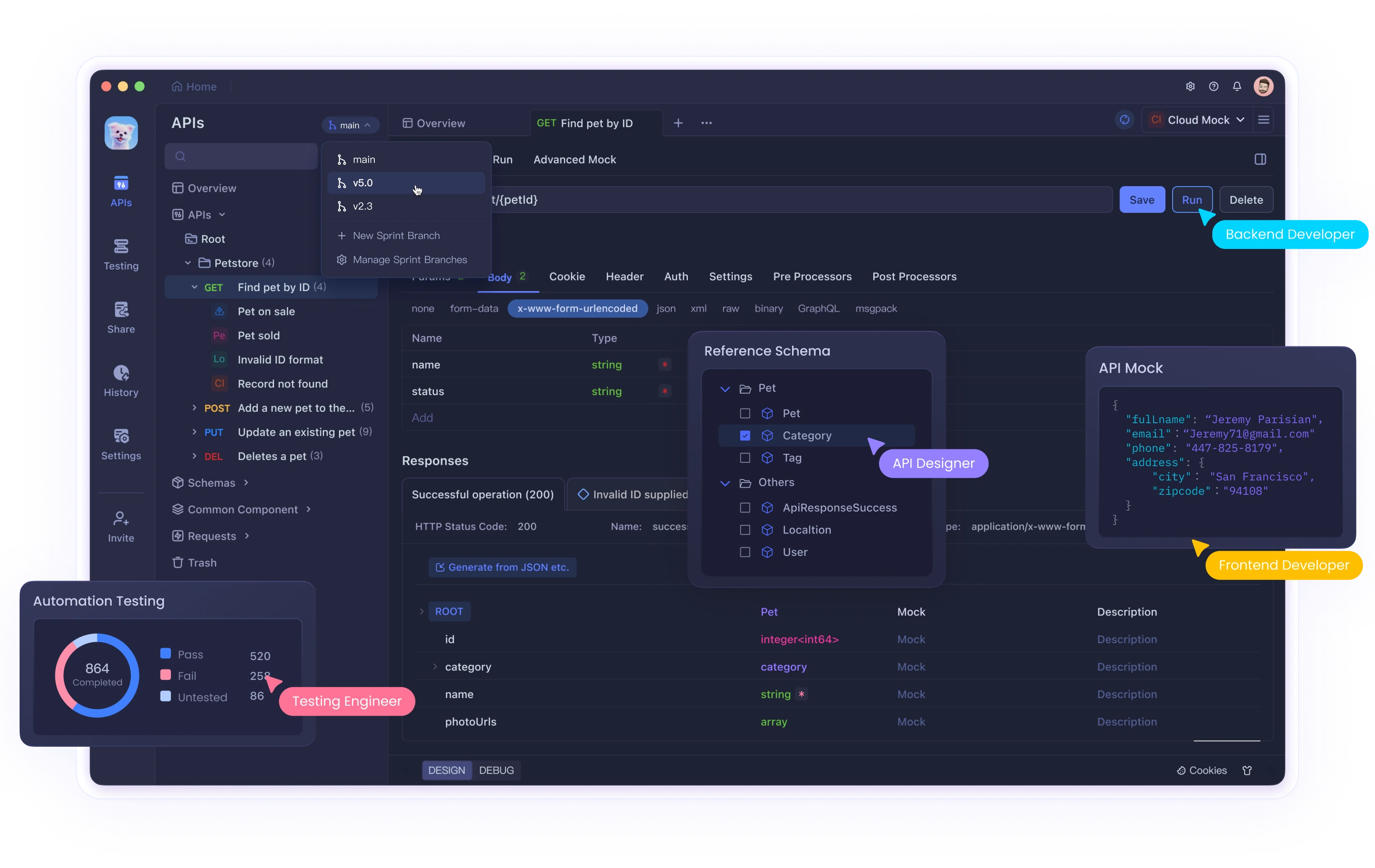
APIdog offers a powerful platform for developing, testing, and documenting your Kraken API integrations. Here's how to leverage APIdog for streamlined development:
Setting Up Kraken API in APIdog
Create a New Project
- Launch APIdog and create a new project
- Set up an environment for Kraken API testing
Configure Environment Variables
- Create variables for
API_KEY
,API_SECRET
, andAPI_URL
- Set the API_URL to
https://api.kraken.com
Import or Create Endpoints
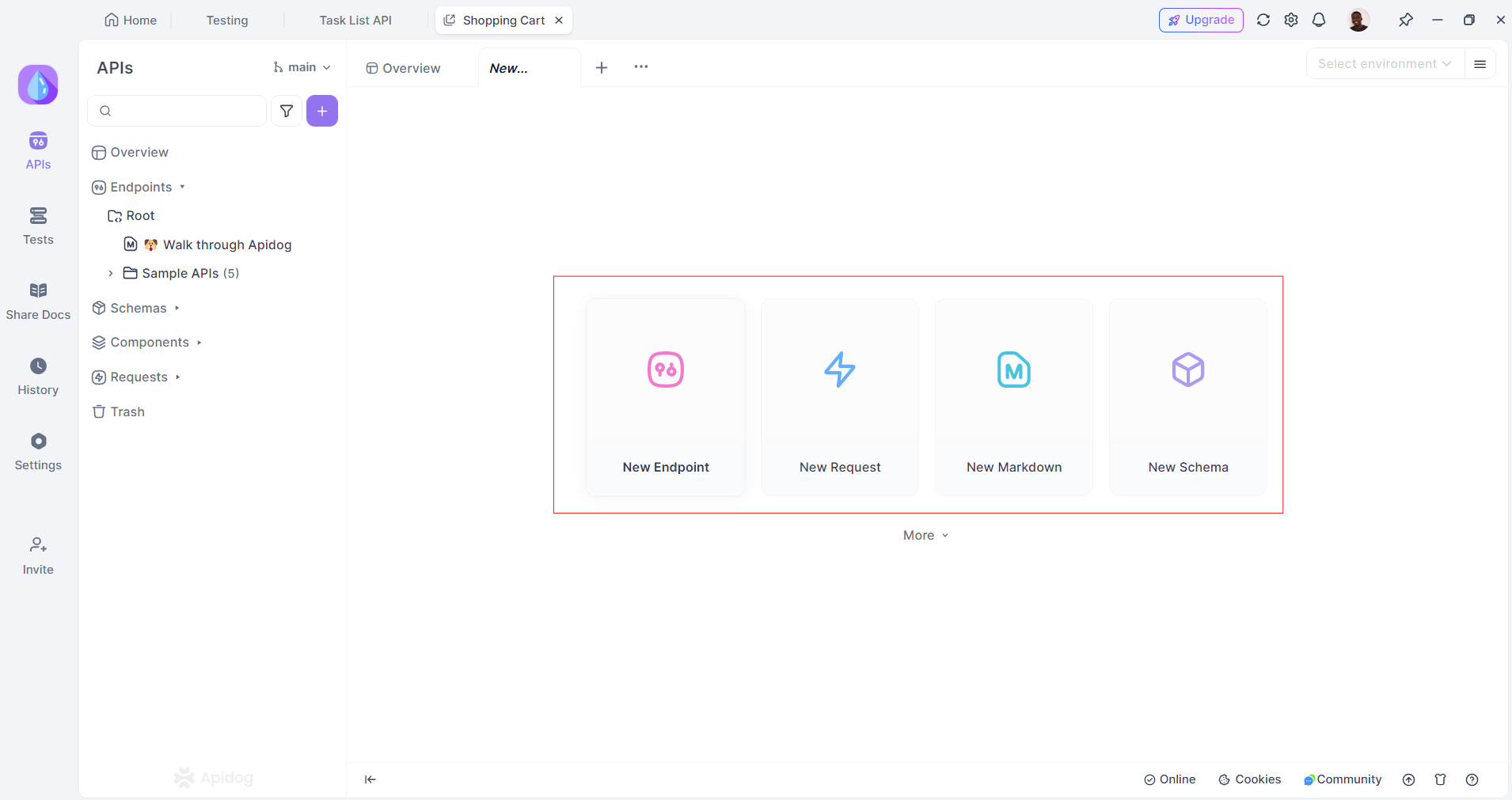
- Either import the Kraken API specification or create collections manually
- Organize endpoints into logical folders (Market Data, Trading, Account, etc.)
Implementing Kraken Authentication in APIdog
APIdog makes it easy to set up Kraken's custom authentication:
- Create a Pre-request Script for generating the necessary authentication:
// Pre-request script for Kraken authentication
const crypto = require('crypto-js');
// Get environment variables
const apiKey = pm.environment.get("API_KEY");
const apiSecret = pm.environment.get("API_SECRET");
// Add nonce to request body
const nonce = Date.now() * 1000;
pm.request.body.update({
mode: 'urlencoded',
urlencoded: [
...pm.request.body.urlencoded.all(),
{key: 'nonce', value: nonce.toString()}
]
});
// Get the request path
const path = pm.request.url.getPathWithQuery().replace(/\?.*/, '');
// Create signature
const postData = pm.request.body.urlencoded.toString();
const message = path + crypto.SHA256(nonce.toString() + postData).toString();
const signature = crypto.enc.Base64.stringify(
crypto.HmacSHA512(message, crypto.enc.Base64.parse(apiSecret))
);
// Set headers
pm.request.headers.add({key: 'API-Key', value: apiKey});
pm.request.headers.add({key: 'API-Sign', value: signature});
Testing and Documenting with APIdog
Create Test Requests
- Set up requests for common operations like getting ticker data, placing orders, etc.
- Use the request builder to configure proper parameters
Add Test Scripts
- Validate API responses with test scripts
- Check for errors and expected data structures
// Example test script for balance endpoint
pm.test("Response status is 200", () => {
pm.response.to.have.status(200);
});
pm.test("No errors returned", () => {
const response = pm.response.json();
pm.expect(response.error).to.be.an('array').that.is.empty;
});
pm.test("Balance data exists", () => {
const response = pm.response.json();
pm.expect(response.result).to.exist.and.to.be.an('object');
});
Generate Documentation
- Use APIdog's documentation tools to create comprehensive API guides
- Include examples, descriptions, and response samples
Share and Collaborate
- Share your Kraken API collection with team members
- Use APIdog's collaboration features for team development
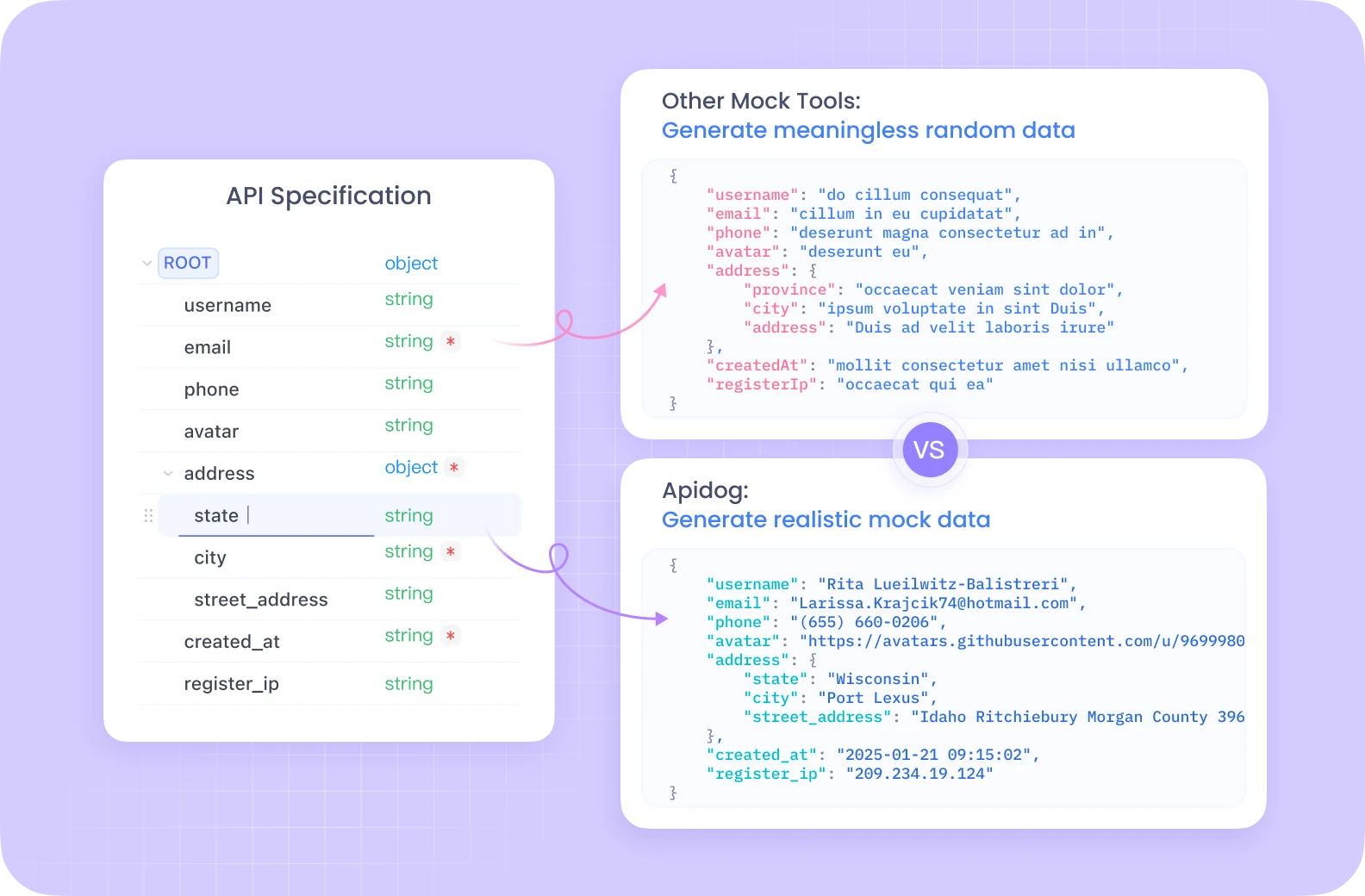
APIdog Mock Servers for Development
While developing, you can use APIdog's mock servers to simulate Kraken API responses:
- Create example responses for each endpoint
- Configure response rules based on request parameters
- Test your application against mock responses before going live
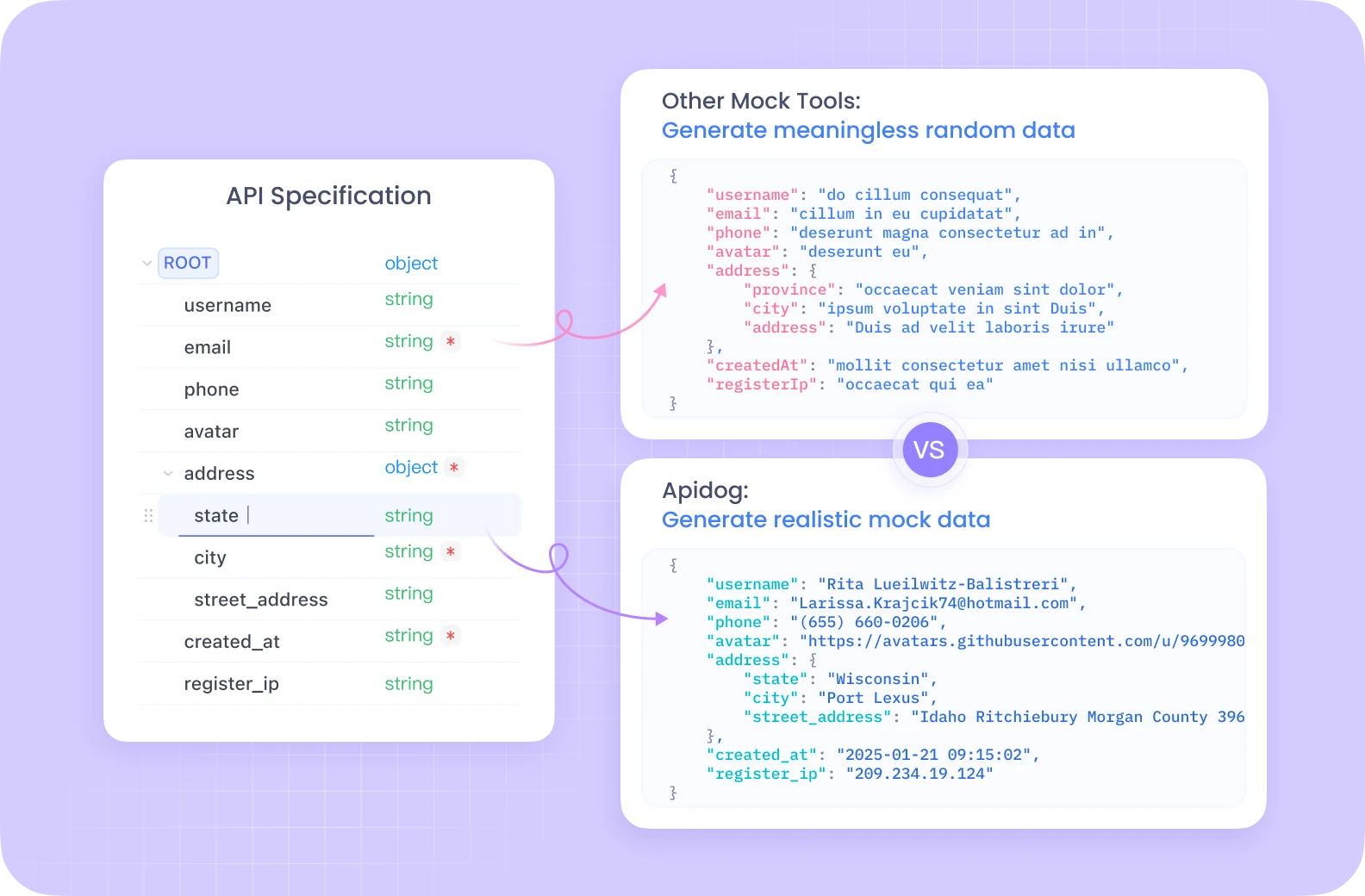
This approach allows for faster development cycles and protects you from hitting API rate limits during development.
Best Practices for Kraken API Integration
Rate Limiting and Optimization
Kraken implements specific rate limits:
- Public endpoints: 1 request per second
- Private endpoints: 15-20 requests per minute
To handle these effectively:
- Implement throttling in your code
- Use WebSockets for real-time data instead of frequent REST API calls
- Batch queries when possible
Error Handling
Implement robust error handling for API interactions:
def safe_api_call(api_function, max_retries=3):
"""Wrapper for API calls with retry logic"""
retries = 0
while retries < max_retries:
try:
response = api_function()
if 'error' in response and response['error']:
error = response['error'][0]
# Handle rate limiting
if 'EAPI:Rate limit' in error:
sleep_time = (2 ** retries) # Exponential backoff
time.sleep(sleep_time)
retries += 1
continue
else:
return None
return response['result']
except Exception as e:
time.sleep(2 ** retries)
retries += 1
return None
Security Considerations
- Store API credentials securely - Never hardcode them in your applications
- Implement IP restrictions in your Kraken API key settings
- Use the minimum required permissions for each API key
- Monitor API usage for unauthorized access
- Rotate API keys periodically
Common Use Cases
Market Data Monitoring
def monitor_price(pair, interval=60):
"""Monitor price at regular intervals"""
while True:
response = requests.get(f'https://api.kraken.com/0/public/Ticker?pair={pair}')
data = response.json()
if not data['error']:
for p, info in data['result'].items():
price = info['c'][0]
print(f"{pair} current price: {price}")
time.sleep(interval)
Simple Trading Bot
def simple_trading_strategy(pair, api_key, api_secret):
"""Example of a simple trading strategy"""
# Get account balance
balance = kraken_request(api_key, api_secret, "/0/private/Balance")
# Get current price
ticker = requests.get(f'https://api.kraken.com/0/public/Ticker?pair={pair}')
ticker_data = ticker.json()
if not ticker_data['error']:
current_price = float(ticker_data['result'][pair]['c'][0])
# Simple strategy: buy if price dropped 5% from yesterday
yesterday_close = float(ticker_data['result'][pair]['o'])
if current_price < yesterday_close * 0.95:
print(f"Price dropped more than 5% - buying opportunity")
# Implement buy logic
Conclusion
The Kraken API provides powerful tools for cryptocurrency trading and portfolio management. This guide has covered the essentials of API access, authentication, and common operations. By leveraging tools like APIdog for development and testing, you can build robust applications that interact seamlessly with the Kraken exchange.
Remember to always refer to the official Kraken API documentation for the most up-to-date information and detailed endpoint references. As you become more comfortable with the basics, you can explore advanced features like WebSocket connections for real-time data and more sophisticated trading strategies.
Whether you're building a personal portfolio tracker, implementing algorithmic trading, or creating enterprise-grade cryptocurrency solutions, mastering the Kraken API opens up significant possibilities in the digital asset ecosystem.