JSON (JavaScript Object Notation) has become a ubiquitous format, prized for its simplicity and readability. Among the various data types supported by JSON, the boolean type plays a crucial role in representing binary states. This comprehensive guide will delve into the intricacies of JSON boolean values, exploring their implementation, best practices, and common pitfalls. We'll also discuss how tools like Apidog can streamline your JSON-related workflows.
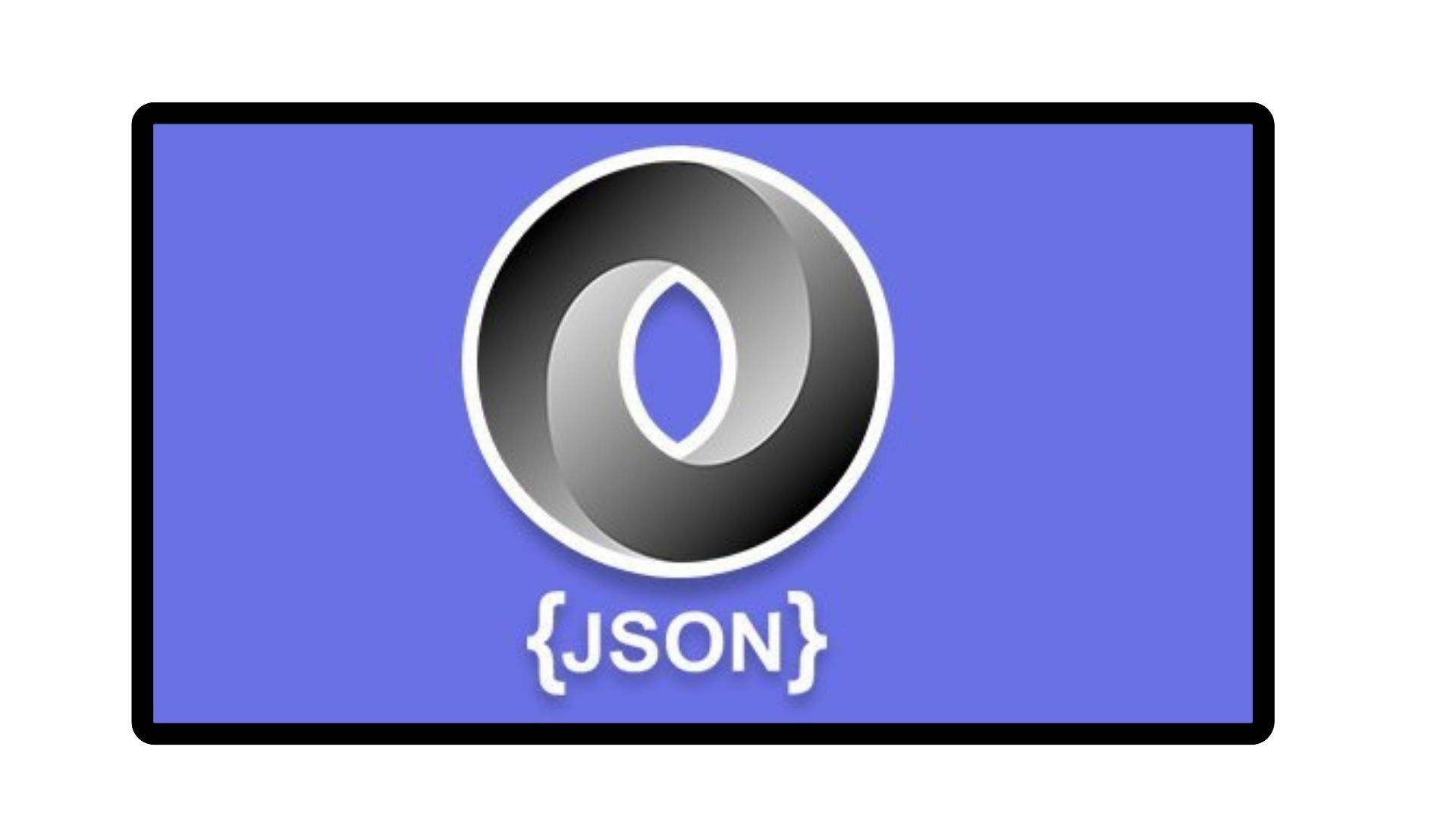
What is a JSON Boolean?
JSON boolean is a data type that represents one of two values: true or false. These values are essential for expressing binary states, such as on/off, yes/no, or enabled/disabled in JSON data structures. Unlike some programming languages that may use 1 and 0 or other representations, JSON strictly uses the lowercase keywords "true" and "false" for boolean values.
The Importance of Boolean Values in JSON
Boolean values are fundamental in JSON for several reasons:
- Simplicity: They provide a clear, unambiguous way to represent binary states.
- Efficiency: Boolean values are compact, using minimal data to convey information.
- Logic Operations: They are crucial for conditional logic in applications consuming JSON data.
- Data Validation: Boolean fields can be used to validate or flag certain conditions in data structures.
JSON Boolean Syntax
In JSON, boolean values are represented as follows:
{
"isActive": true,
"isDeleted": false
}
It's important to note that JSON boolean values are always lowercase. Using uppercase (TRUE or FALSE) or quotation marks ("true" or "false") will result in invalid JSON or incorrect data types.
Common Use Cases for JSON Boolean
Boolean values in JSON find applications in various scenarios:
- User Settings: Representing user preferences or feature toggles.
- State Management: Indicating the state of objects or processes.
- Conditional Rendering: Determining whether to display certain UI elements.
- Data Flags: Marking records for specific processing or attention.
- API Responses: Indicating success or failure of operations.
Best Practices for Using JSON Boolean
To ensure the effective use of boolean values in your JSON data, consider the following best practices:
- Use Descriptive Names: Choose clear, self-explanatory names for boolean fields.
- Avoid Double Negatives: Prefer positive naming conventions to improve readability.
- Consistency: Maintain consistent naming conventions across your JSON structures.
- Default Values: Consider providing default boolean values to handle missing data gracefully.
- Documentation: Clearly document the meaning and implications of boolean fields in your API or data model documentation.
JSON Boolean vs. Other Data Types
While boolean values are straightforward, it's essential to understand how they differ from other data types in JSON:
- Strings: Avoid using string values like "true" or "false" when a boolean is more appropriate.
- Numbers: While some systems use 0 and 1 to represent false and true, JSON booleans are distinct from numeric values.
- Null: The null value in JSON is different from false. Use null to represent the absence of a value, not a false state.
Handling JSON Boolean in Different Programming Languages
Different programming languages may handle JSON boolean values slightly differently. Let's explore how to work with JSON booleans in some popular languages:
JavaScript
JavaScript, being the native language of JSON, handles boolean values seamlessly:
const data = { isActive: true };
console.log(data.isActive); // Output: true
console.log(typeof data.isActive); // Output: boolean
Python
Python's json module automatically converts JSON boolean values to Python's True and False:
import json
data = json.loads('{"isActive": true}')
print(data['isActive']) # Output: True
print(type(data['isActive'])) # Output: <class 'bool'>
Java
In Java, you can use libraries like Jackson or Gson to parse JSON. These libraries typically map JSON booleans to Java's boolean primitive or Boolean object:
import com.fasterxml.jackson.databind.ObjectMapper;
ObjectMapper mapper = new ObjectMapper();
JsonNode node = mapper.readTree("{\\\\"isActive\\\\": true}");
boolean isActive = node.get("isActive").asBoolean();
System.out.println(isActive); // Output: true
C#
C# uses the System.Text.Json namespace for JSON operations, which maps JSON booleans to C#'s bool type:
using System.Text.Json;
string jsonString = "{\\\\"isActive\\\\": true}";
JsonDocument doc = JsonDocument.Parse(jsonString);
bool isActive = doc.RootElement.GetProperty("isActive").GetBoolean();
Console.WriteLine(isActive); // Output: True
Common Pitfalls and How to Avoid Them
When working with JSON boolean values, be aware of these common pitfalls:
- Case Sensitivity: Remember that JSON boolean values are always lowercase. Using "True" or "FALSE" will result in invalid JSON.
- Type Confusion: Avoid mixing boolean and string representations. Use true instead of "true" for boolean values.
- Implicit Type Conversion: Be cautious when working with languages or systems that may implicitly convert boolean values to other types.
- Missing Values: Handle cases where a boolean field might be missing from the JSON structure to prevent errors.
- Serialization Issues: Ensure your serialization libraries correctly handle boolean values, especially when working with custom objects.
JSON Boolean in API Design
When designing APIs that use JSON, consider the following tips for boolean fields:
- Clear Naming: Use unambiguous names for boolean fields (e.g., "isEnabled" instead of "enabled").
- Documentation: Clearly document the expected values and behavior of boolean fields in your API documentation.
- Consistency: Maintain consistent naming and usage patterns across your API endpoints.
- Validation: Implement proper validation to ensure that boolean fields receive correct values.
- Default Values: Consider providing default values for optional boolean fields to improve API usability.
Tools for Working with JSON Boolean
Several tools can help you work more efficiently with JSON data, including boolean values:
- JSON Validators: Online tools like JSONLint can help you validate your JSON and ensure correct boolean syntax.
- JSON Editors: Visual editors like JSON Editor Online provide a user-friendly interface for editing JSON data.
- API Testing Tools: Platforms like Postman allow you to test APIs and work with JSON responses, including boolean fields.
- Apidog: A comprehensive API development platform that simplifies working with JSON data, including boolean values.
Introducing Apidog for JSON and API Development
Apidog is an all-in-one platform for API design, debugging, documentation, and testing. It offers several features that can streamline your work with JSON data, including boolean values:
- Visual API Design: Design your APIs visually, including JSON schemas with boolean fields.
- API Testing: Test your APIs and validate JSON responses, ensuring correct handling of boolean values.
- Mock Servers: Create mock servers that return JSON data with boolean fields for frontend development and testing.
- Documentation Generation: Automatically generate API documentation that clearly describes boolean fields and their usage.
- Code Generation: Generate client SDKs that correctly handle JSON boolean values in various programming languages.
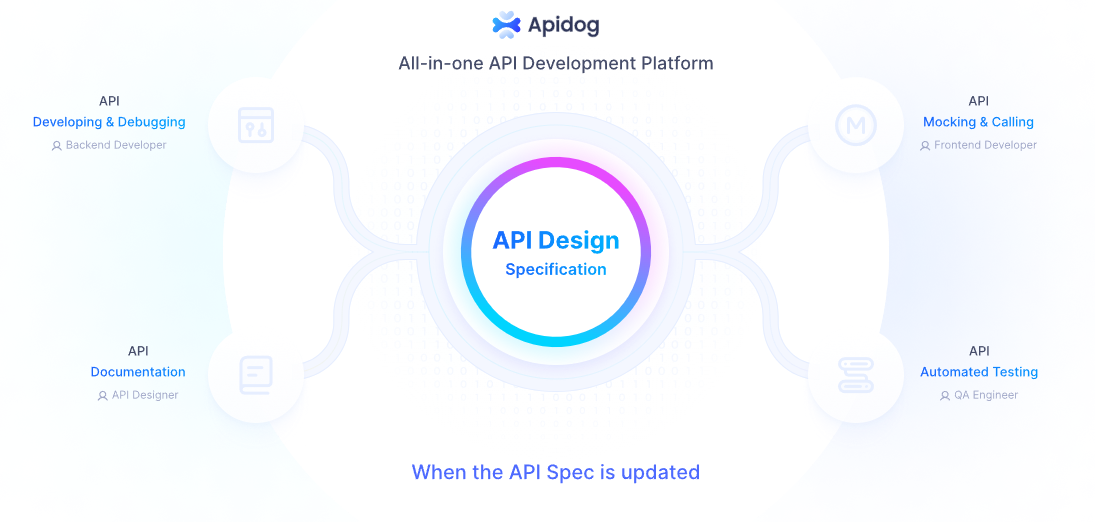
By using Apidog, you can ensure consistent and correct usage of JSON boolean values throughout your API development lifecycle.
Future Trends in JSON and Boolean Data
As data interchange continues to evolve, we can expect some trends related to JSON and boolean data:
- Schema Validation: Increased use of JSON Schema for validating boolean fields and other data types.
- GraphQL Adoption: Growing popularity of GraphQL, which provides more fine-grained control over data types, including booleans.
- JSON-LD: Expanded use of JSON for Linked Data (JSON-LD), which may introduce new ways of representing boolean concepts.
- Performance Optimizations: Development of more efficient parsing and serialization methods for JSON data, including boolean values.
- AI and Machine Learning: Increased use of boolean flags in JSON data for machine learning model features and configurations.
Conclusion
JSON boolean values, while simple in concept, play a crucial role in data representation and application logic. By understanding their proper usage, avoiding common pitfalls, and leveraging tools like Apidog, developers can ensure more robust and efficient data interchange in their applications.
As you continue to work with JSON in your projects, remember the importance of clear, consistent, and well-documented boolean fields. Whether you're designing APIs, building frontend applications, or working on data processing systems, mastering the nuances of JSON boolean values will contribute to cleaner, more maintainable code and better overall system design.