In the world of JavaScript testing, Jest has emerged as a powerhouse, offering a robust and feature-rich environment for developers. However, one question that frequently pops up in developer circles is: "Is Jest really running tests concurrently?" Let's embark on a comprehensive journey to unravel this mystery and explore the intricacies of Jest's test execution model.
Diving Deep into Jest's Execution Model: Is the Test Really Running Concurrently?
Jest, at its core, is designed to optimize test execution by leveraging parallel processing. However, the term "concurrent" can be a bit misleading when it comes to how Jest actually runs tests. Let's break it down:
- File-level parallelism: Jest runs different test files concurrently across multiple worker processes.
- In-file sequentiality: Tests within a single file are executed sequentially.
This hybrid approach allows Jest to balance speed with predictability. Here's a more detailed look:
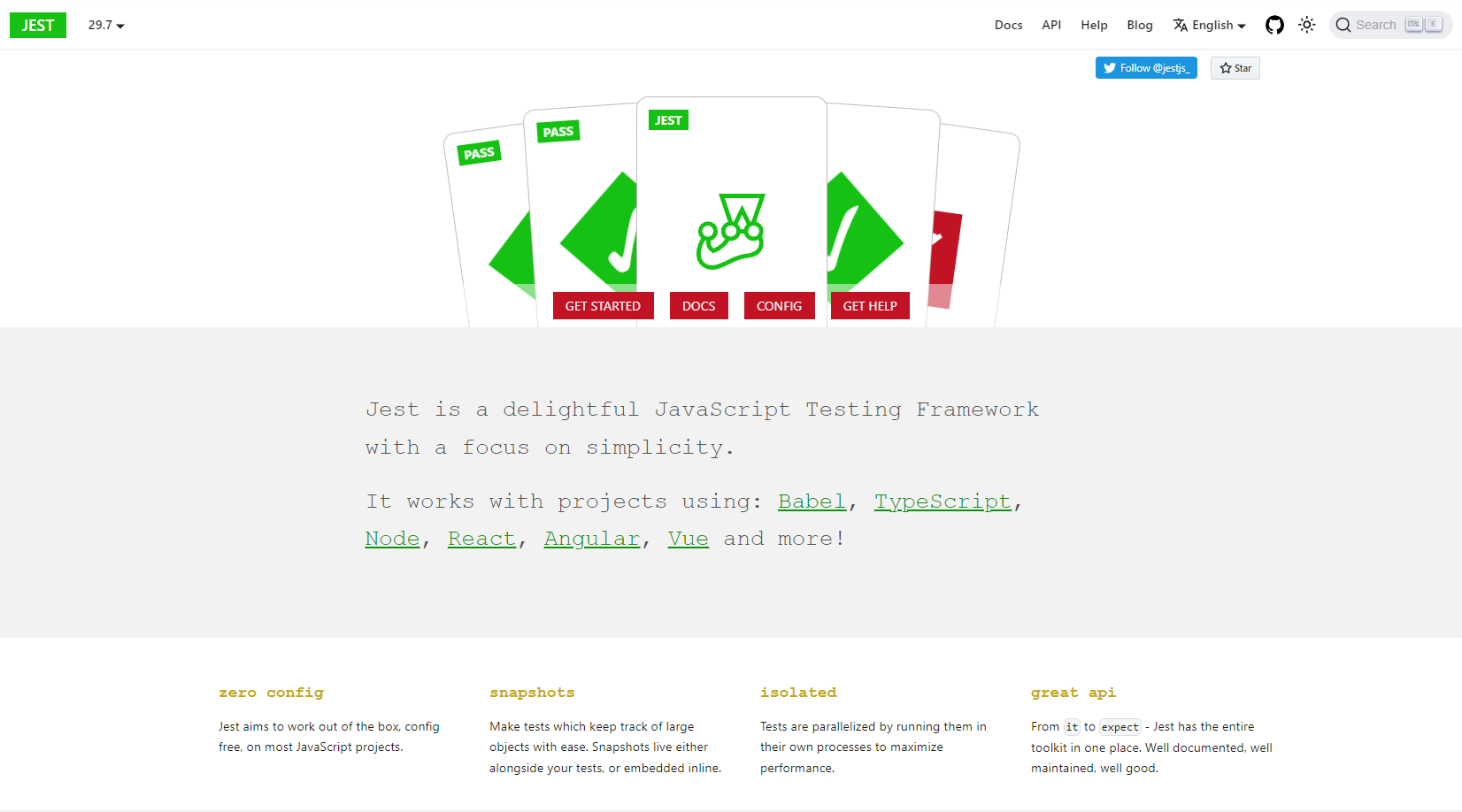
File-level Parallelism
- Jest spawns multiple worker processes (the number depends on your configuration and system resources).
- Each worker process is assigned one or more test files to execute.
- These files run independently of each other, truly concurrently.
In-file Sequentiality
- Within each file, tests are run in the order they're defined.
- This ensures predictable setup and teardown within a test suite.
- It also prevents potential race conditions between closely related tests.
Advanced Configuration for Concurrent Execution: Is the Test Really Running Concurrently?
To truly harness Jest's concurrent capabilities, you need to understand and tweak its configuration. Let's explore some advanced options:
Adjusting Worker Count
The --maxWorkers
option is your primary tool for controlling concurrency. Here are some ways to use it:
{
"scripts": {
"test": "jest --maxWorkers=4",
"test:half": "jest --maxWorkers=50%",
"test:auto": "jest --maxWorkers=auto"
}
}
--maxWorkers=4
: Uses exactly 4 worker processes.--maxWorkers=50%
: Uses half of the available CPU cores.--maxWorkers=auto
: Lets Jest decide based on system resources (default behavior).
Controlling Test Order
While Jest runs files in parallel, you might want to control the order of test execution within files:
describe.order.sequence('Critical Path', () => {
test('Step 1', () => { /* ... */ });
test('Step 2', () => { /* ... */ });
test('Step 3', () => { /* ... */ });
});
This ensures these tests run in the specified order, even if other tests in the file are shuffled.
Isolating Test Environments
For true concurrency, each test should be isolated. Jest provides the --isolatedModules
flag:
{
"jest": {
"isolatedModules": true
}
}
This option runs each test file in a separate VM, ensuring complete isolation but potentially increasing overhead.
Practical Verification: Is the Test Really Running Concurrently?
To truly understand Jest's concurrency model, let's set up a practical experiment:
- Create multiple test files:
// test1.js
test('Long running test in file 1', async () => {
console.log('Test 1 started at:', new Date().toISOString());
await new Promise(resolve => setTimeout(resolve, 3000));
console.log('Test 1 ended at:', new Date().toISOString());
});
// test2.js
test('Long running test in file 2', async () => {
console.log('Test 2 started at:', new Date().toISOString());
await new Promise(resolve => setTimeout(resolve, 3000));
console.log('Test 2 ended at:', new Date().toISOString());
});
// test3.js
describe('Multiple tests in file 3', () => {
test('Quick test 1', () => {
console.log('Quick test 1 at:', new Date().toISOString());
});
test('Quick test 2', () => {
console.log('Quick test 2 at:', new Date().toISOString());
});
});
- Run Jest with verbose logging:
jest --verbose --runInBand
The --runInBand
flag forces Jest to run all tests in a single process, useful for comparison.
- Now run without
--runInBand
:
jest --verbose
Compare the timestamps. You'll likely see that test1.js
and test2.js
run concurrently, while the tests within test3.js
run sequentially.
Leveraging Jest's Concurrency for Different Test Types: Is the Test Really Running Concurrently?
Jest's concurrency model can be particularly beneficial for certain types of tests:
Unit Tests
- Typically fast and isolated
- Benefit greatly from file-level parallelism
- Example:
// math.test.js
import { add, subtract } from './math';
test('add function', () => {
expect(add(2, 3)).toBe(5);
});
test('subtract function', () => {
expect(subtract(5, 3)).toBe(2);
});
Integration Tests
- May involve setup/teardown of resources
- Can run concurrently if properly isolated
- Example using a test database:
// user.integration.test.js
import { createUser, deleteUser } from './userService';
import { connectDB, disconnectDB } from './database';
beforeAll(async () => {
await connectDB();
});
afterAll(async () => {
await disconnectDB();
});
test('create and delete user', async () => {
const user = await createUser({ name: 'John Doe' });
expect(user.id).toBeDefined();
await deleteUser(user.id);
// Verify user is deleted
});
E2E Tests
- Often longer-running
- May need to run sequentially to avoid conflicts
- Can use Jest's
describe.serial
for enforced order:
// checkout.e2e.test.js
import { launchBrowser, closeBrowser } from './testUtils';
describe.serial('Checkout Process', () => {
let browser;
beforeAll(async () => {
browser = await launchBrowser();
});
afterAll(async () => {
await closeBrowser(browser);
});
test('Add item to cart', async () => {
// Implementation
});
test('Proceed to checkout', async () => {
// Implementation
});
test('Complete payment', async () => {
// Implementation
});
});
Advanced Techniques for Concurrent Testing with Jest: Is the Test Really Running Concurrently?
To truly master concurrent testing with Jest, consider these advanced techniques:
Custom Test Runners
Jest allows you to create custom test runners, giving you fine-grained control over test execution:
// customRunner.js
class CustomRunner {
constructor(globalConfig, context) {
this.globalConfig = globalConfig;
this.context = context;
}
async runTests(tests, watcher, onStart, onResult, onFailure) {
// Custom logic for running tests
// You can implement your own parallelization strategy here
}
}
module.exports = CustomRunner;
Configure Jest to use your custom runner:
{
"jest": {
"runner": "<rootDir>/customRunner.js"
}
}
Test Sharding
For very large test suites, you can implement test sharding:
jest --shard=1/3
This runs only the first third of your test files, allowing you to distribute tests across multiple machines or CI jobs.
Dynamic Test Generation
Leverage Jest's dynamic test generation to create tests that adapt to your data or environment:
const testCases = [
{ input: 1, expected: 2 },
{ input: 2, expected: 4 },
{ input: 3, expected: 6 },
];
testCases.forEach(({ input, expected }) => {
test(`doubleNumber(${input}) should return ${expected}`, () => {
expect(doubleNumber(input)).toBe(expected);
});
});
This approach allows you to easily scale your test suite without duplicating code.
Integrating APIdog with Jest for Comprehensive API Testing: Is the Test Really Running Concurrently?
Apidog can significantly enhance your API testing workflow when used in conjunction with Jest.
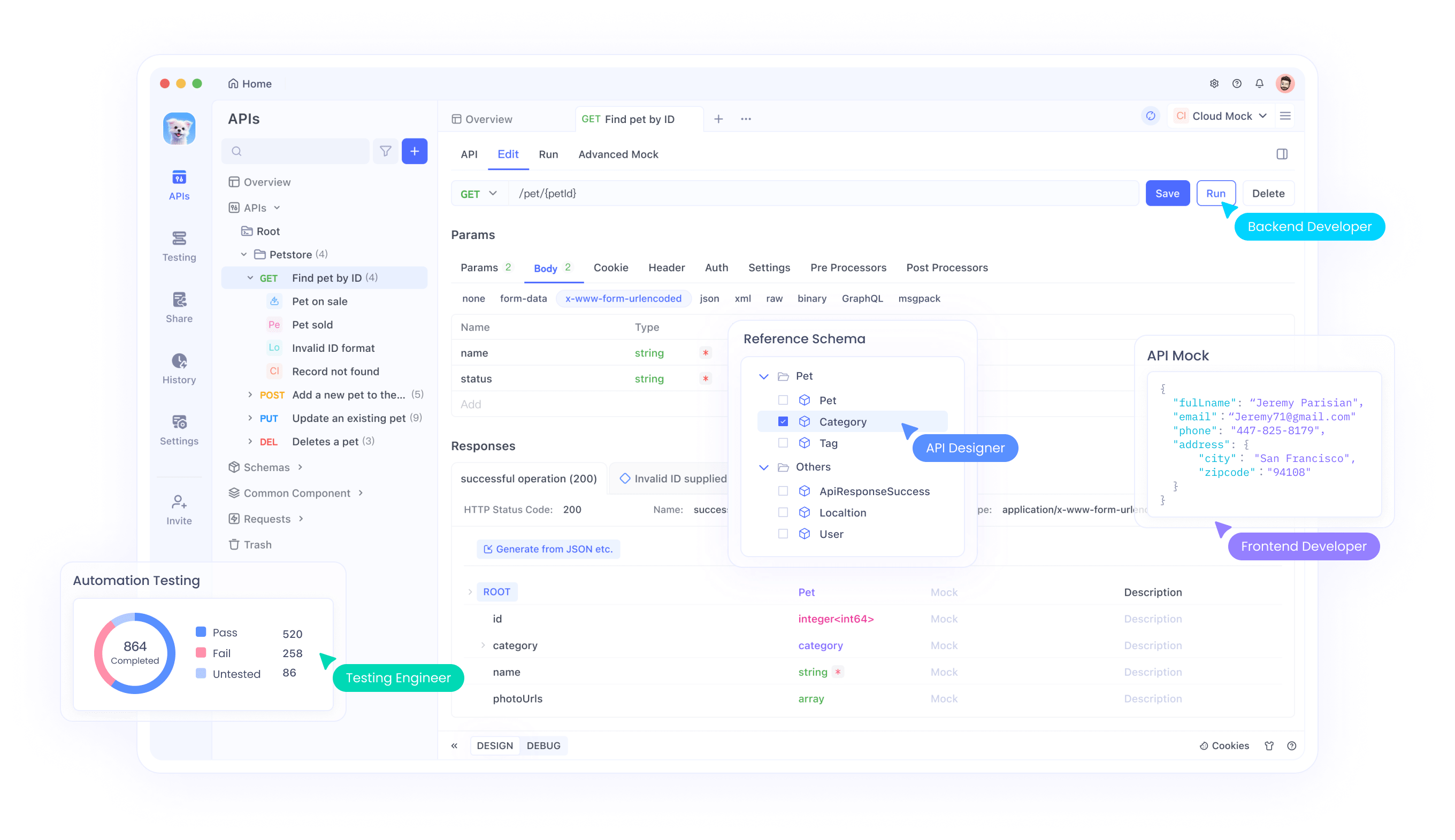
Debugging with Apidog is easy. Once enter the details of your API, including the endpoint and request parameters, you can easily inspect the response and debug your API with the debug mode.
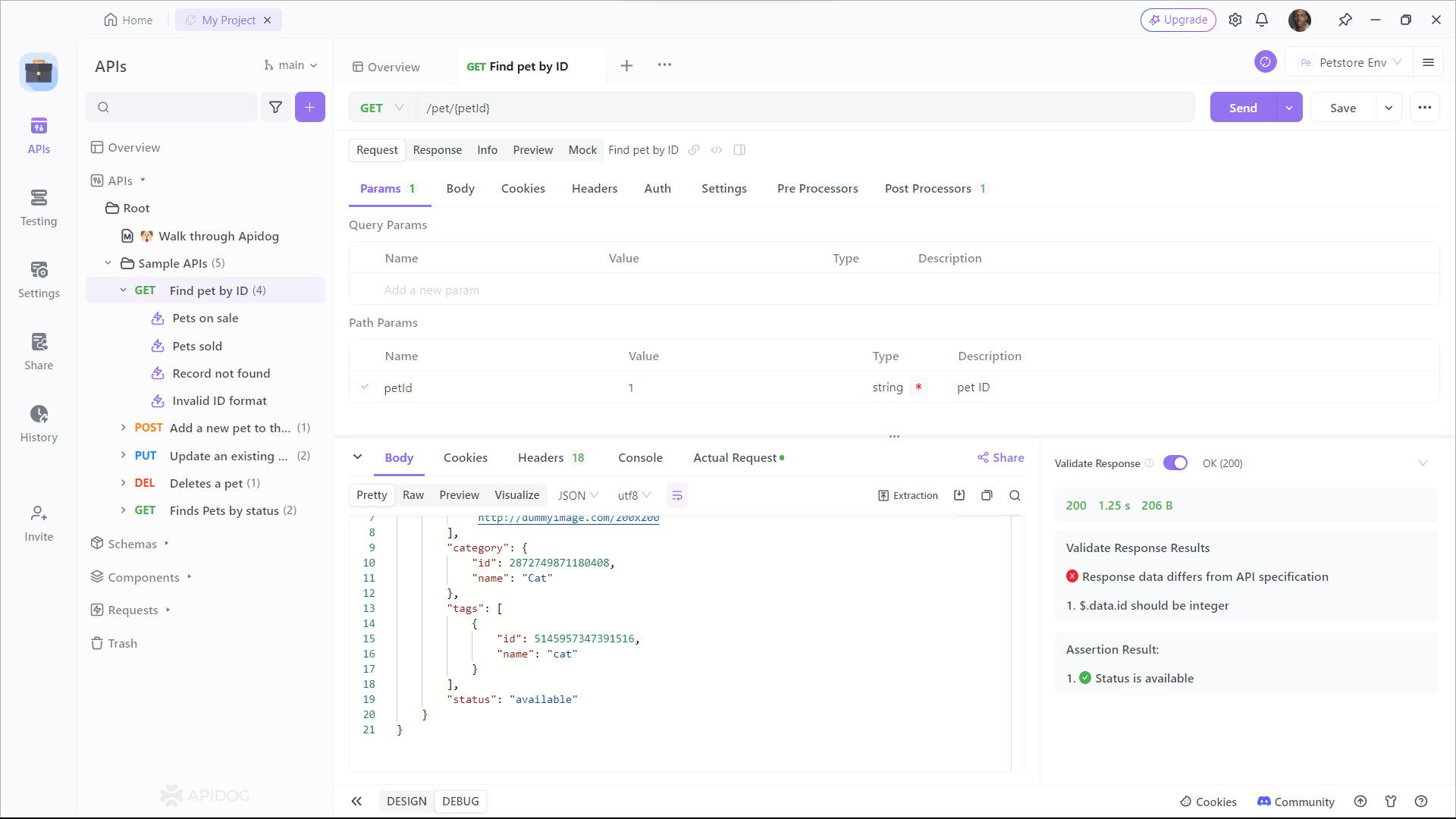
FAQs: Is the Test Really Running Concurrently?
Let's dive deeper into some frequently asked questions about Jest and concurrency:
Are Jest tests run sequentially?
It depends on the context:
- Tests in different files can run concurrently.
- Tests within the same file run sequentially by default.
You can enforce sequential execution across all tests using the --runInBand
flag, which is useful for debugging or when dealing with shared resources that can't be accessed concurrently.
How does Jest run tests?
Jest follows these steps:
- Collects all test files based on your configuration.
- Divides these files among available worker processes.
- Each worker process:
- Loads the test file
- Executes the tests in that file sequentially
- Reports results back to the main Jest process
- The main process collects all results and generates a report.
This approach allows for parallelism at the file level while maintaining predictable execution within each file.
Is Jest used for parallelization of tasks?
While Jest is primarily a testing framework, its parallel execution model can be leveraged for task parallelization in certain scenarios:
- Running multiple independent scripts or checks as part of a CI/CD pipeline.
- Performing data processing tasks that can be split across multiple files.
- Executing multiple independent API calls or database queries.
However, for general-purpose task parallelization, dedicated tools like GNU Parallel or Node.js's worker_threads
module might be more appropriate.
What are the cons of Jest testing?
While Jest is powerful, it's important to be aware of potential drawbacks:
Resource Intensity: Running many tests in parallel can be memory and CPU intensive, especially on CI servers.
Complexity in Debugging: Parallel execution can make it harder to reproduce and debug failing tests.
Potential for Flaky Tests: Concurrent execution can sometimes lead to race conditions or timing-related issues.
Learning Curve: Jest's extensive feature set and configuration options can be overwhelming for beginners.
Overhead for Small Projects: For very small projects, Jest's setup and runtime might be overkill.
Mocking Complexity: While powerful, Jest's mocking capabilities can lead to overly complex test setups if not used judiciously.
Conclusion: Is the Test Really Running Concurrently?
Jest's approach to test execution offers a nuanced form of concurrency. While it doesn't run every single test simultaneously, its file-level parallelism combined with in-file sequentiality provides a balanced approach to test execution.
By understanding and leveraging Jest's concurrency model, you can:
- Significantly reduce overall test execution time
- Maintain test reliability and predictability
- Scale your test suite effectively as your project grows
Remember, the key to effective testing with Jest isn't just about running tests concurrently, but about writing well-structured, isolated tests that can take full advantage of Jest's execution model. Whether you're using Jest standalone or integrating it with tools like APIdog, the goal is to create a robust, efficient testing strategy that supports your development process and ensures the quality of your software.
As you continue to work with Jest, experiment with different configurations, explore advanced features, and always keep an eye on test performance and reliability. With practice and careful consideration of your specific needs, you can harness the full power of Jest's concurrent capabilities to create a fast, reliable, and maintainable test suite.