When writing tests for code that makes API calls, it's important to mock out those calls to ensure your tests are reliable, fast, and not dependent on an external service. Jest, a popular JavaScript testing framework, provides several ways to easily mock API calls in your tests. Let's dive into the different approaches you can use.
Apidog is a comprehensive low-code API platform that equips developers with high-quality functionalities for the entire API lifecycle. With additional tools like automated code generation, CI/CD integration, and customizable scripts, experience professional API development within a single platform!
To learn more about Apidog, make sure to click the button below!
Using jest.mock()
One way to mock API calls in Jest is by using the jest.mock()
function to mock the entire module that makes the API request. Here's an example:
// api.js
import axios from 'axios';
export const getUsers = () => {
return axios.get('/users');
};
// api.test.js
import axios from 'axios';
import { getUsers } from './api';
jest.mock('axios');
test('getUsers returns data from API', async () => {
const users = [{ id: 1, name: 'John' }];
axios.get.mockResolvedValueOnce({ data: users });
const result = await getUsers();
expect(axios.get).toHaveBeenCalledWith('/users');
expect(result.data).toEqual(users);
});
In this example, we use jest.mock('axios')
to automatically mock the entire axios module. Then we use axios.get.mockResolvedValueOnce()
to mock the response for the next axios.get
call. Our test can then verify the API was called correctly and returns the mocked data1.
Using Manual Mocks
Another approach is to manually mock the module that makes the API call by creating a __mocks__
folder and putting a mock implementation file inside:
// __mocks__/axios.js
export default {
get: jest.fn(() => Promise.resolve({ data: {} })),
post: jest.fn(() => Promise.resolve({ data: {} })),
// ...
};
Now in your test, you can mock different responses for each test:
// api.test.js
import axios from 'axios';
import { getUsers } from './api';
jest.mock('axios');
test('getUsers returns data from API', async () => {
const users = [{ id: 1, name: 'John' }];
axios.get.mockResolvedValueOnce({ data: users });
const result = await getUsers();
expect(axios.get).toHaveBeenCalledWith('/users');
expect(result.data).toEqual(users);
});
With this manual mock, you have full control to mock the different Axios methods like get
, post
, etc. with your own implementations2.
Using Axios-mock-adapter
You can use the library to mock Axios requests more advancedly. First, install it:
npm install axios-mock-adapter --save-dev
Then in your tests:
// api.test.js
import axios from 'axios';
import MockAdapter from 'axios-mock-adapter';
import { getUsers } from './api';
describe('getUsers', () => {
let mock;
beforeAll(() => {
mock = new MockAdapter(axios);
});
afterEach(() => {
mock.reset();
});
test('returns users data', async () => {
const users = [{ id: 1, name: 'John' }];
mock.onGet('/users').reply(200, users);
const result = await getUsers();
expect(result.data).toEqual(users);
});
});
With the axios-mock-adapter, you can mock requests based on URLs, parameters, headers, and more. You can also simulate errors and timeouts.
Injecting a Mocked Axios Instance
If your code uses axios directly, another option is to inject a mocked axios instance into your code during tests:
// api.js
import axios from 'axios';
export const getUsers = () => {
return axios.get('/users');
};
// api.test.js
import axios from 'axios';
import { getUsers } from './api';
jest.mock('axios', () => ({
get: jest.fn(),
}));
test('getUsers returns data from API', async () => {
const users = [{ id: 1, name: 'John' }];
axios.get.mockResolvedValueOnce({ data: users });
const result = await getUsers();
expect(axios.get).toHaveBeenCalledWith('/users');
expect(result.data).toEqual(users);
});
Here we mock axios itself, not the entire module, and provide our own mocked get
function.
Tips for Mocking API Calls
Here are a few tips to keep in mind when mocking API calls in Jest:
- Reset your mocks between tests to ensure independent tests by using
beforeEach
andafterEach
- Don't mock too much - only mock the functions and modules your code actually uses
- Test for failure cases too by mocking errors and unexpected responses
- Consider creating reusable mock fixtures for common API responses
Mock APIs With Apidog
If you wish to try a different API development experience, you can consider using Apidog!
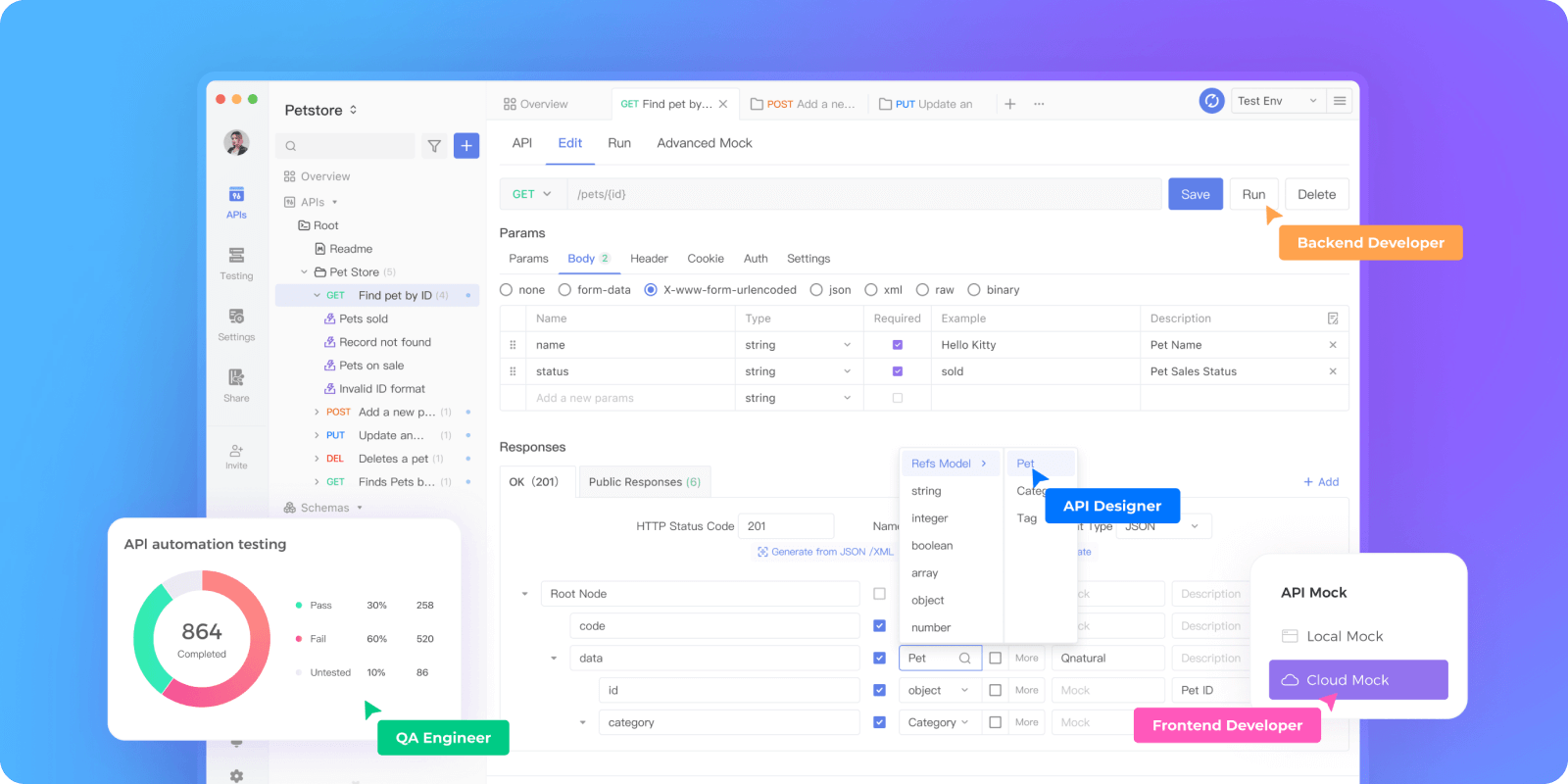
Apidog is a low-code API platform that provides developers with a simple and intuitive user interface for developing APIs. With Apidog, you can build, test, mock, and document APIs. Let us take a closer look at Apidog!
Apidog Smart Mock
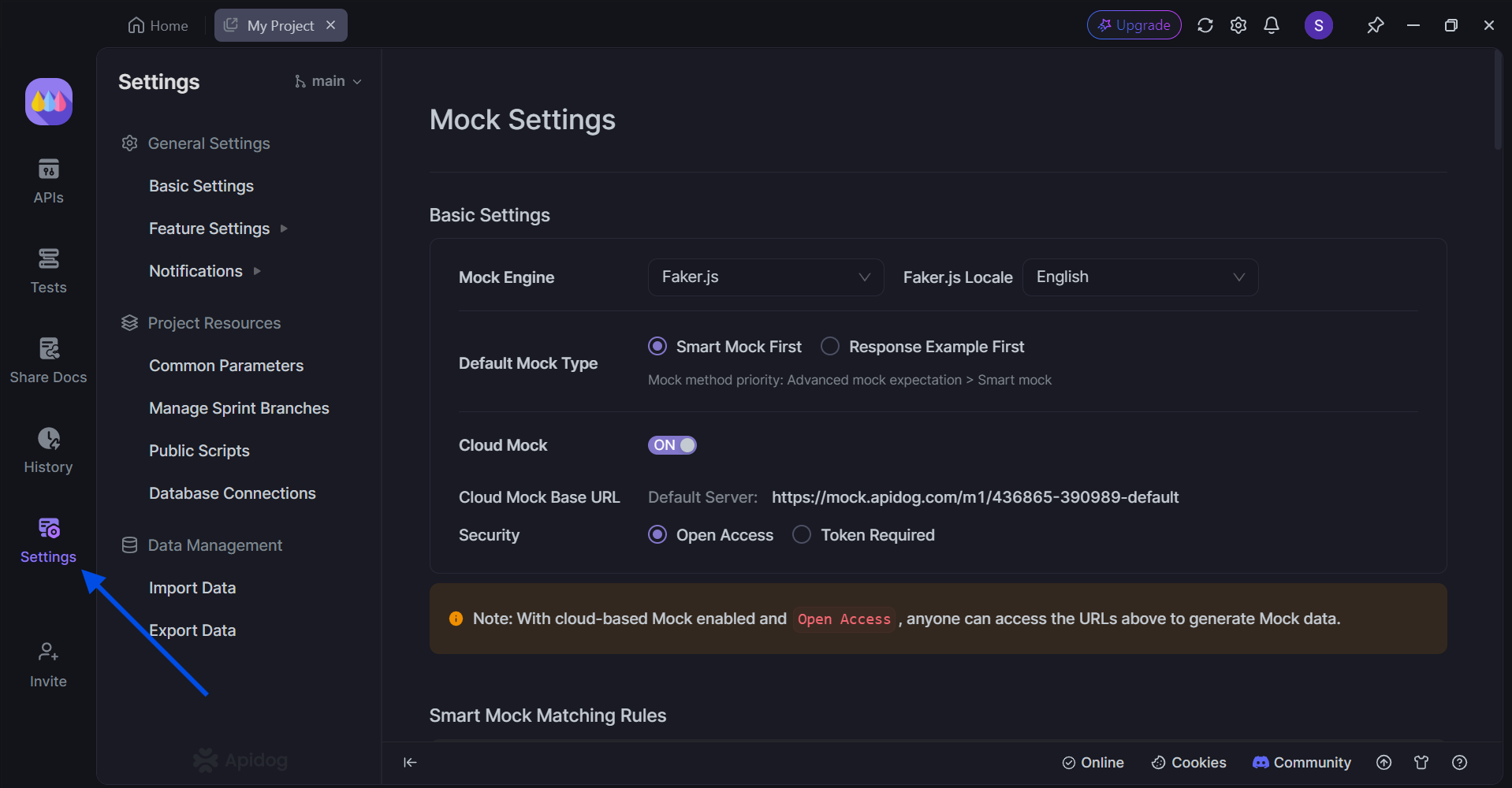
Let Apidog automatically generate realistic mock data without manual configuration if you have no definitive mock rules.
Apidog Advanced Mock
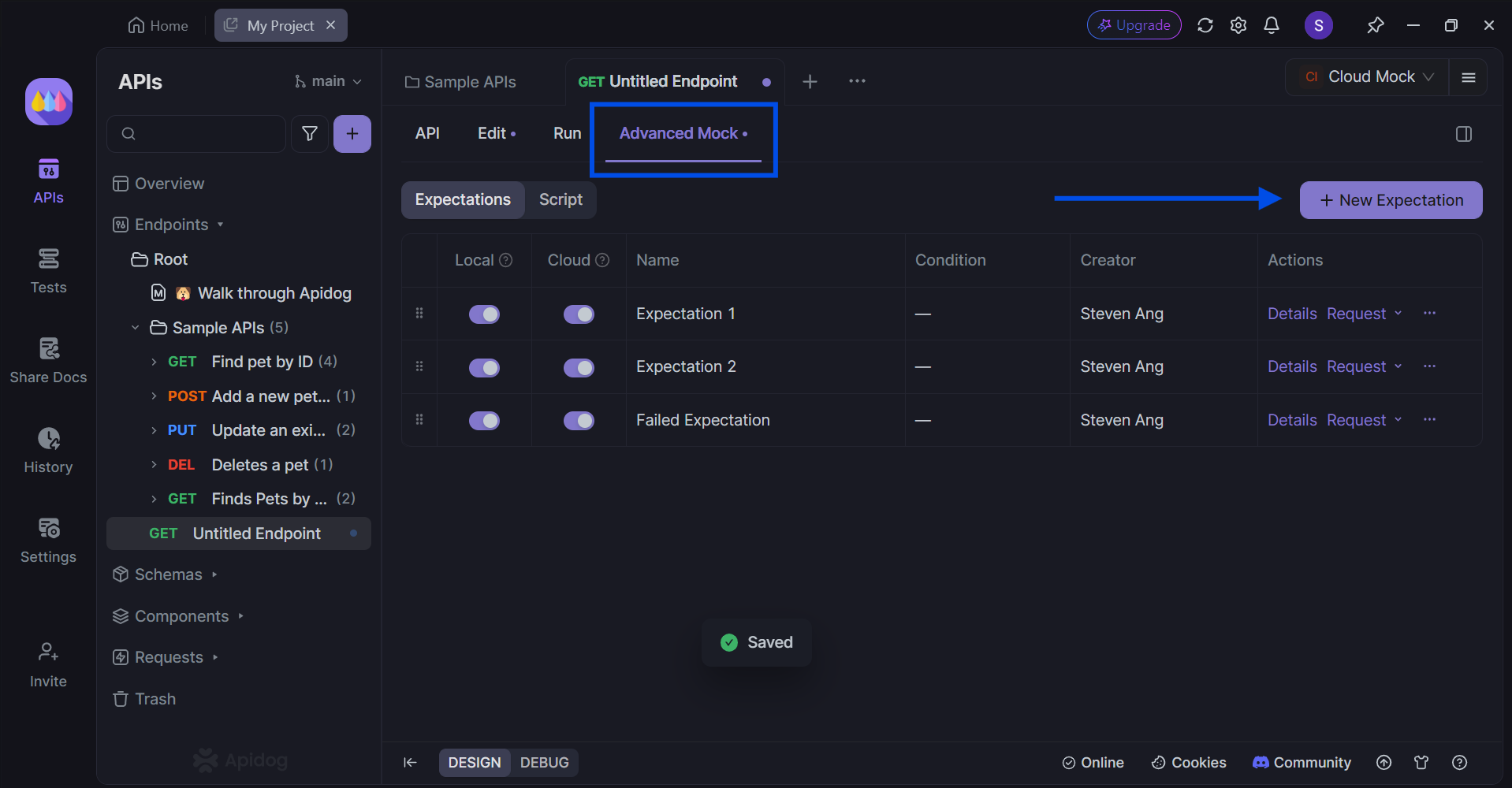
Modify API responses with custom scripts to simulate real-life interactions between the client and server side.
Apidog Cloud Mock
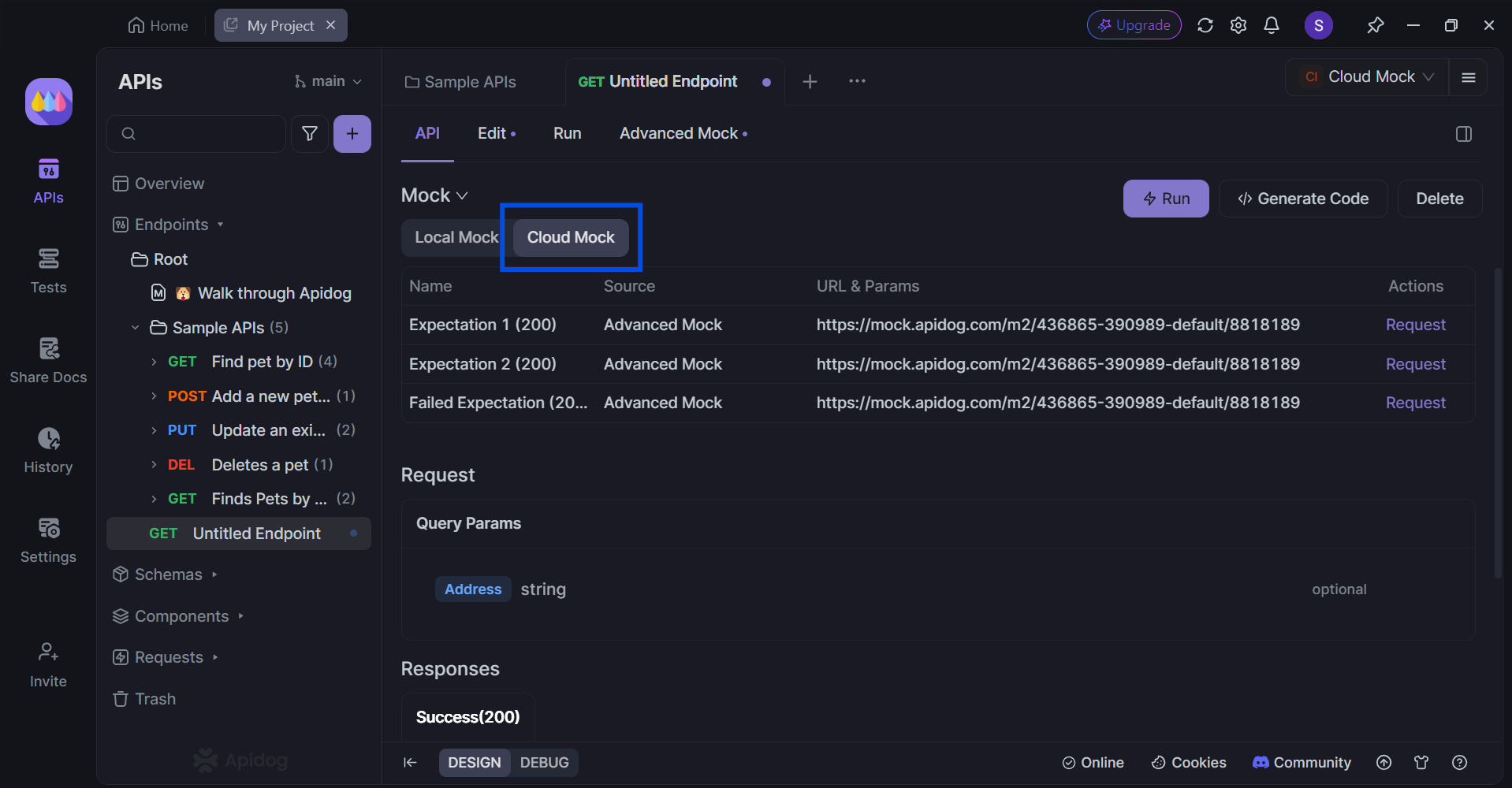
Collaborate with your team with the cloud mock feature through a fixed address, accessible on the cloud mock server.
Conclusion
Mocking is a fundamental skill for writing good tests, especially when dealing with external dependencies like API calls. Jest provides many ways to mock API calls in your tests, from mocking entire modules with jest.mock()
, to manually mocking modules, to using libraries like axios-mock-adapter
for more advanced cases. The key is to choose the right approach for your needs while keeping your tests independent and focused on the code being tested, not the implementation details of the APIs. With these mocking techniques in your toolbelt, you can write resilient tests for code that depends on APIs.