JavaScript HTTP PUT methods are one of the four famous HTTP methods used in JavaScript: GET, PUT, POST, and DELETE. Each HTTP method has its specialty, therefore they are not interchangeable and replaceable with one another.
Apidog stands out for its intuitive visual design interface, making it easy for users to adapt quickly. For those in search of an effective API solution, Apidog presents itself as a compelling option. Click the button below to start exploring its features! 👇 👇 👇
What is JavaScript HTTP PUT?
Basically, the term "JavaScript HTTP PUT" refers to a method used for updating an existing resource found on a server. Therefore, you can also refer to JavaScript HTTP PUT as an HTTP method, along with the other GET, POST, and DELETE counterparts.
Key Characteristics (and Components) of a JavaScript HTTP PUT Method
Modern technologies such as the Fetch API work together with JavaScript HTTP PUT methods to create interesting applications. However, a JavaScript HTTP PUT method may have distinct features on its own, such as:
- Method: The JavaScript HTTP PUT method is used to update an existing resource on the server. It's one of the standard HTTP methods alongside GET, POST, DELETE, etc.
- Data Payload: Similar to POST requests, PUT requests typically include a payload in the request body. This payload contains the data that will be used to update the resource on the server.
- Headers: PUT requests may include headers that provide additional information about the request, such as the content type (
Content-Type
) specifying the format of the data being sent (e.g., JSON, XML, etc.). - Endpoint: The URL of the server endpoint to which the PUT request is sent. This URL identifies the resource that will be updated on the server.
- Idempotent: PUT requests are idempotent, meaning that making the same request multiple times should have the same effect as making it once. This ensures that repeated requests do not cause unintended side effects.
- Asynchronous: JavaScript PUT requests are usually made asynchronously to prevent blocking the execution of other scripts or the rendering of the webpage. This is typically achieved using functions like
fetch
orXMLHttpRequest
. - Response Handling: After sending a PUT request, JavaScript code typically handles the response from the server. This may involve parsing the response data (e.g., JSON or XML) and updating the webpage accordingly.
Why Use JavaScript HTTP PUT Methods?
Certain characteristics that the JavaScript HTTP PUT method possesses allow it to be a prime method for API communication. Here are some reasons that you may be interested to know!
- Idempotency: JavaScript HTTP PUT requests are idempotent, meaning that making the same request multiple times should have the same effect as making it once. This ensures that repeated requests do not cause unintended side effects or data inconsistencies.
- Resource Update: PUT requests are specifically designed for updating resources on the server. They are commonly used when you need to modify the state or attributes of an existing resource, such as updating user profiles, editing documents, or changing settings.
- RESTful APIs: PUT requests are a fundamental part of the REST (Representational State Transfer) architectural style, which emphasizes a stateless client-server communication model and the manipulation of resources through standard HTTP methods. If you are building or interacting with RESTful APIs, using PUT requests is standard practice for updating resources.
- Asynchronous Operations: JavaScript HTTP PUT methods allow you to update server-side resources asynchronously, without blocking the execution of other scripts or the rendering of the webpage. This enables a smoother and more responsive user experience in web applications.
- Data Integrity: PUT requests are often used in scenarios where data integrity is critical, as they allow you to update specific fields or attributes of a resource without affecting other parts of the data. This helps maintain consistency and accuracy in the application's data model.
- Efficiency: PUT requests can be more efficient than alternatives like PATCH requests (which are used for partial updates) when you need to update an entire resource or a significant portion of it. They provide a straightforward way to send the updated data to the server in a single request.
JavaScript HTTP PUT Method Code Example (With Fetch API)
// Define the data you want to update
const updateData = {
username: 'newUsername',
email: 'newemail@example.com'
};
// Convert the data to JSON format
const jsonData = JSON.stringify(updateData);
// Define the URL of the server endpoint
const url = 'https://example.com/api/resource/123'; // Replace with your endpoint URL
// Configure the fetch options
const options = {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: jsonData
};
// Make the PUT request using the fetch API
fetch(url, options)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON response
})
.then(data => {
console.log('Resource updated successfully:', data);
})
.catch(error => {
console.error('There was a problem with your fetch operation:', error);
});
Code Explanation (In order):
- Defined the data we want to update as a JavaScript object (
updateData
). - Converted the data to JSON format using
JSON.stringify()
and store it in thejsonData
variable. - Defined the URL of the server endpoint (
url
), specifying the resource to update (e.g.,resource/123
). - Configured the fetch options object (
options
) with the method set to'PUT'
, headers indicating JSON content type, and the JSON data in the request body. - Used the
fetch
function to make the PUT request with the specified URL and options. - Handled the response asynchronously using Promises, parsing the JSON response and logging it to the console.
- Handled errors using the
catch
method to log any encountered errors.
Common Use Cases for JavaScript HTTP PUT Methods
There are a lot of situations that require an application to update its current data based on changes made by clients! Here are a few common cases that might be hidden in plain sight.
- User Profile Updates: When users update their profiles on a website or application, JavaScript HTTP PUT requests can be used to send the updated user data to the server. This could include changes to user information such as username, email, password, profile picture, etc.
- Document Editing: In collaborative document editing applications, JavaScript HTTP PUT requests can be used to save changes made by users to documents stored on the server. This allows multiple users to work on the same document simultaneously and see each other's changes in real time.
- Settings Configuration: Applications often allow users to customize their preferences and settings. JavaScript HTTP PUT requests can be used to update these settings on the server, ensuring that the user's preferences are saved and applied across different sessions.
- Product Updates in E-commerce: In e-commerce applications, JavaScript HTTP PUT requests are commonly used to update product information such as price, quantity, description, and availability. This allows administrators to manage their product catalog efficiently and keep it up-to-date.
- Task Management: Task management applications often allow users to update task details such as title, description, due date, priority, etc. JavaScript HTTP PUT requests can be used to send these updates to the server and ensure that the task information is synchronized across devices.
- Content Management: Content management systems (CMS) use JavaScript HTTP PUT requests to update content such as articles, blog posts, images, videos, etc. This allows content creators and administrators to make changes to the website or application content dynamically.
- Inventory Management: In inventory management systems, JavaScript HTTP PUT requests are used to update inventory levels, track product movements, and manage stock quantities. This ensures that inventory data remains accurate and up-to-date.
- Form Data Editing: When users submit forms containing data that needs to be edited or updated, JavaScript HTTP PUT requests can be used to send the updated form data to the server for processing. This allows for seamless data editing workflows in web applications.
Update Your API Development Tool to Apidog Today!
Apidog is a powerful, all-in-one API development tool that encourages API developers to design visually. With a stunningly beautiful user interface that is easy to learn, new Apidog users can easily adapt to the new API tool environment.
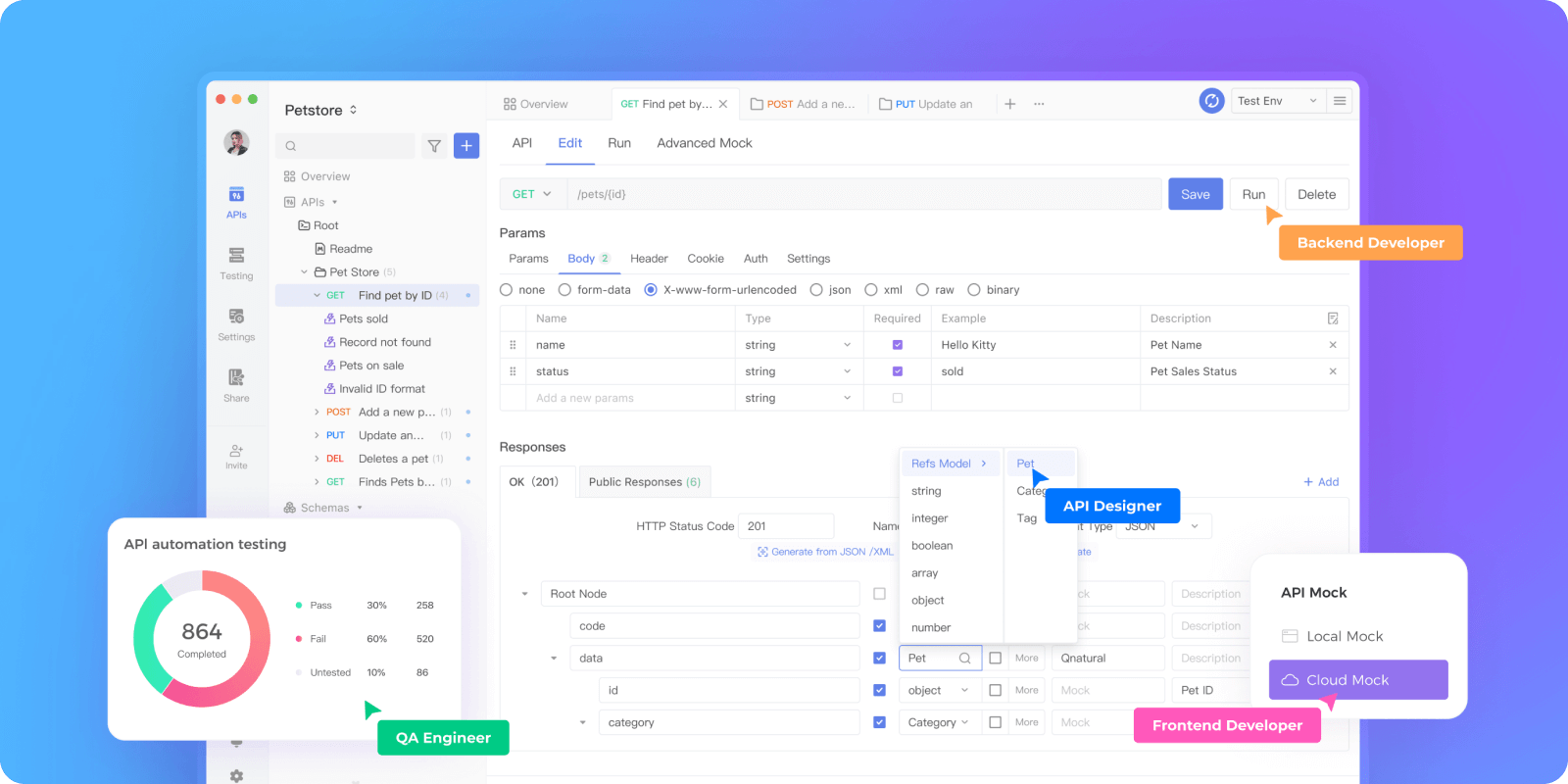
Building a New JavaScript HTTP PUT Request with Apidog
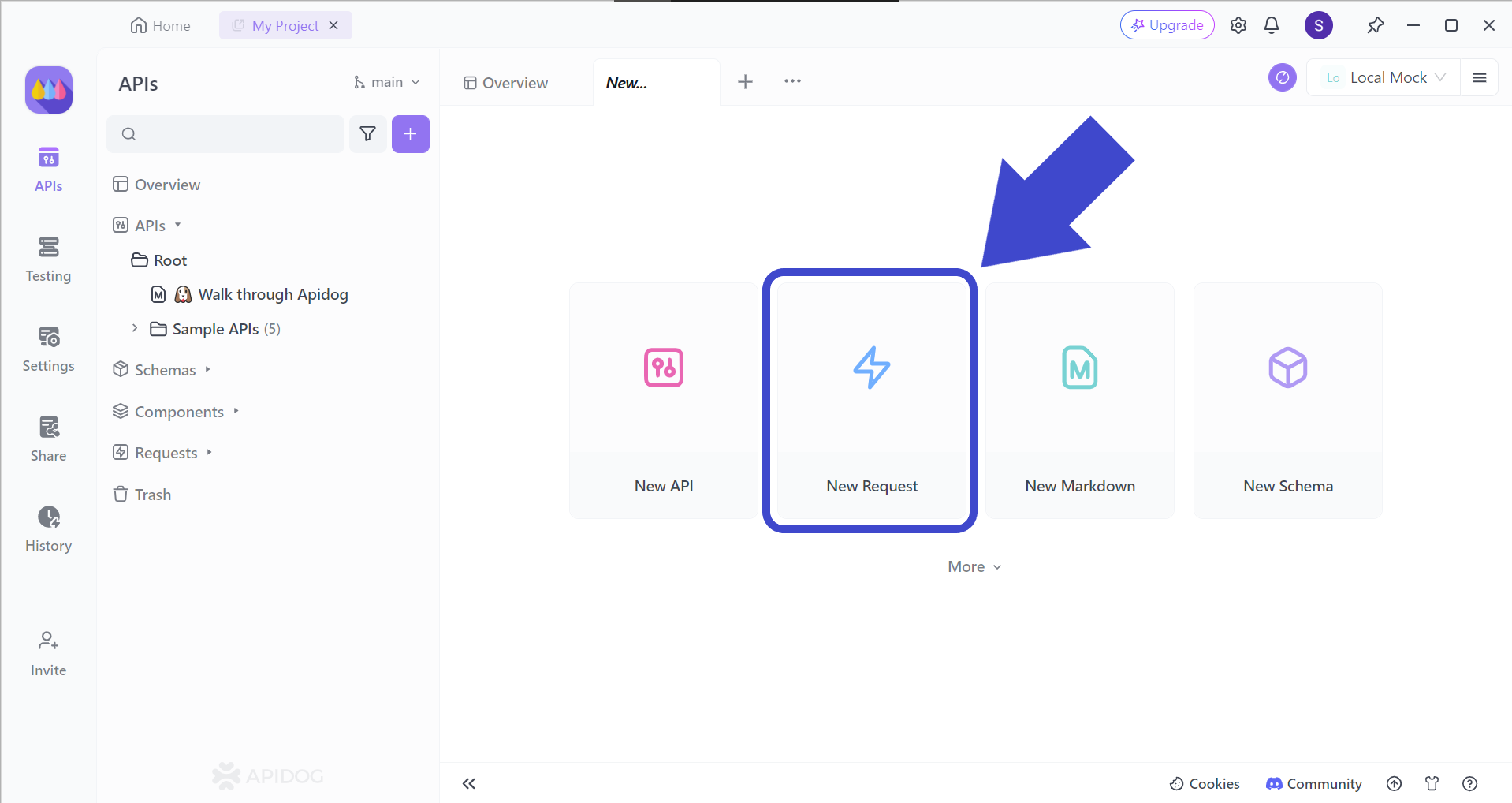
Begin by pressing the New Request
button as pointed out by the arrow in the picture above.
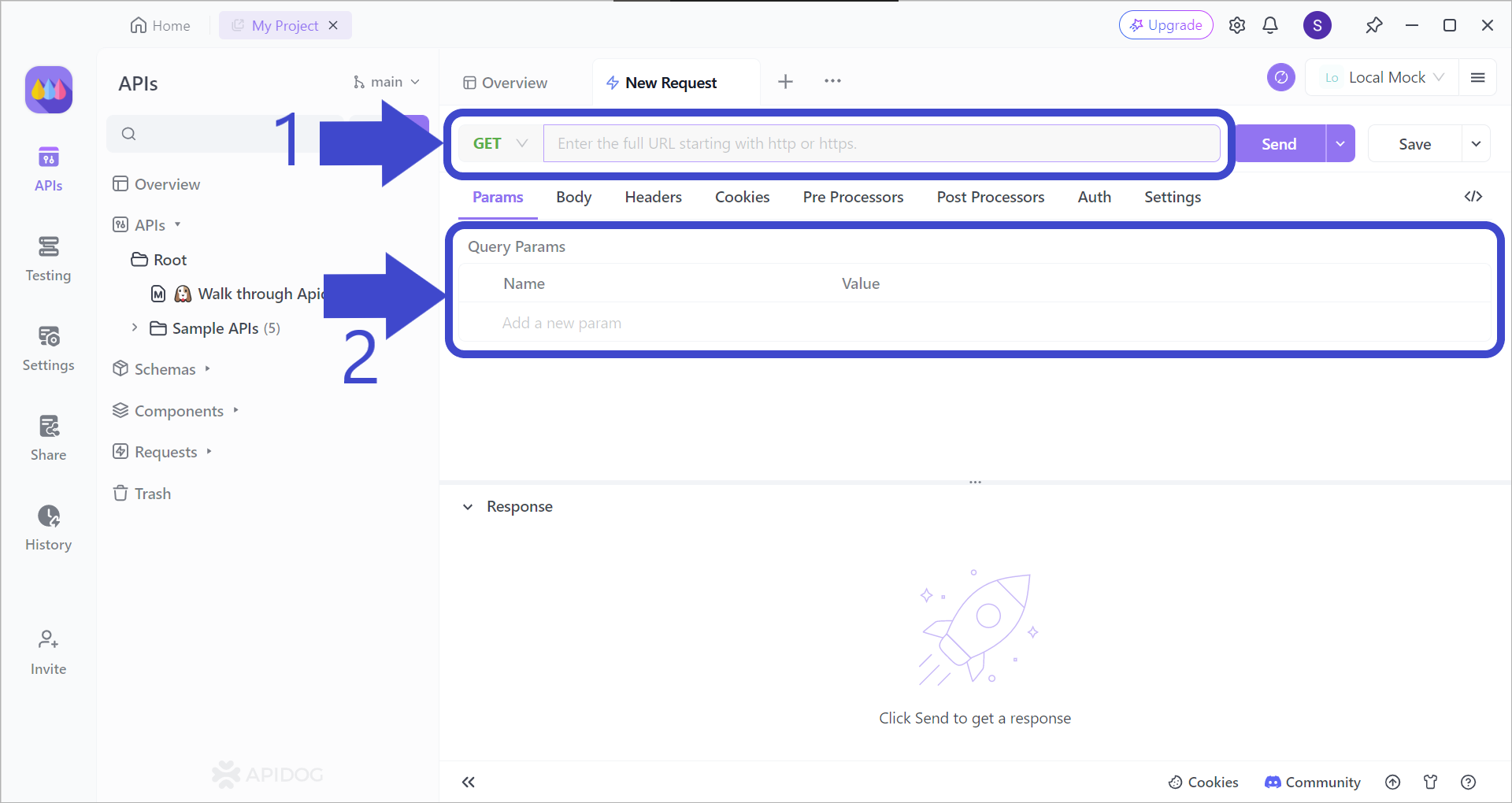
To create a JavaScript HTTP PUT request, make sure to select the PUT
method, and create a relevant URL.
Observing the Response Obtained from JavaScript HTTP PUT Method Using Apidog
You can utilize Apidog's simple and intuitive user interface to analyze the response returned after the request has been sent.
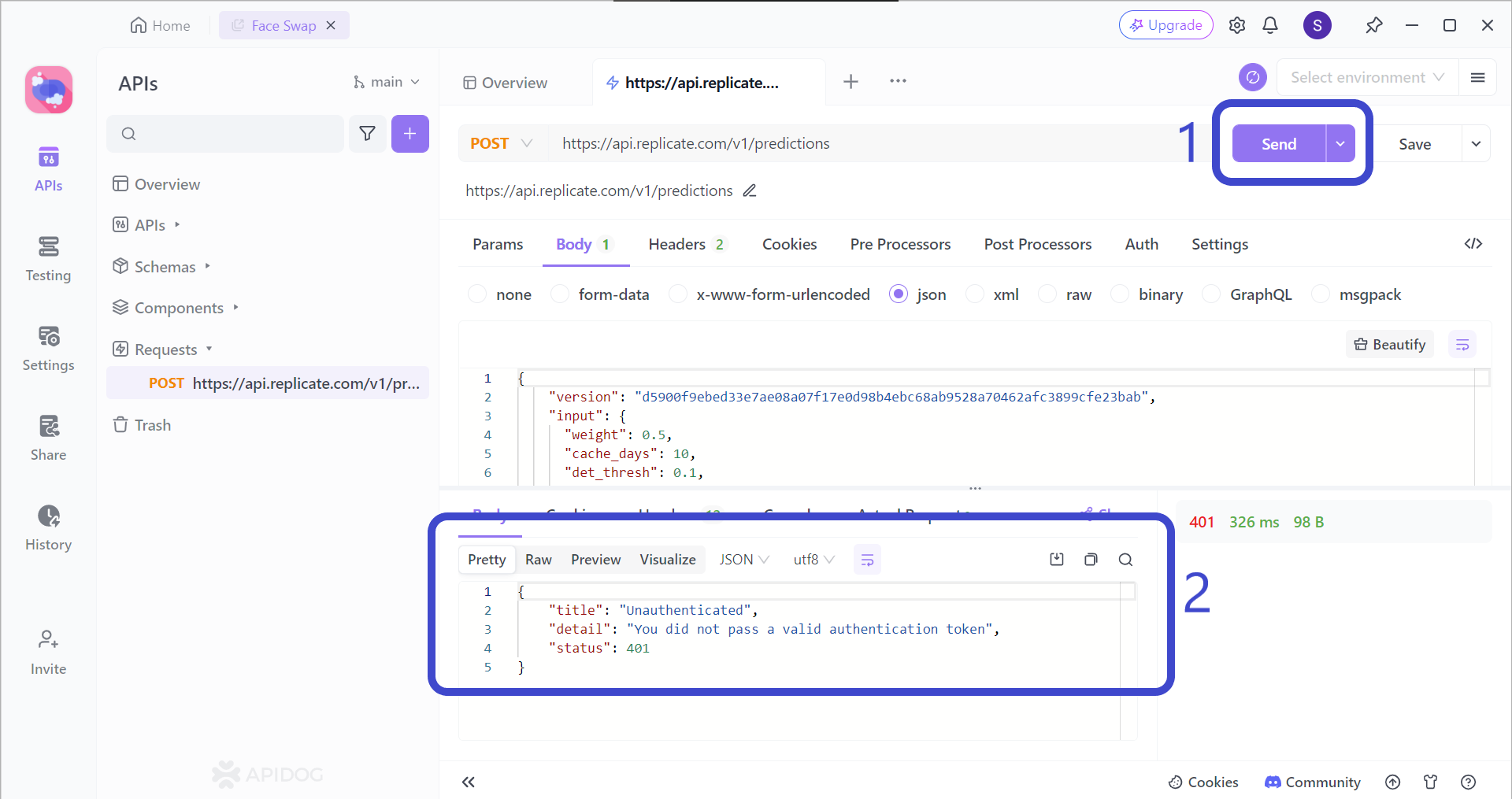
Make the JavaScript HTTP PUT method request by pressing the Send
button found in the right corner of the Apidog window. Then, you should be able to view the response on the bottom portion of the screen.
Setting API Responses for Your API Using Apidog
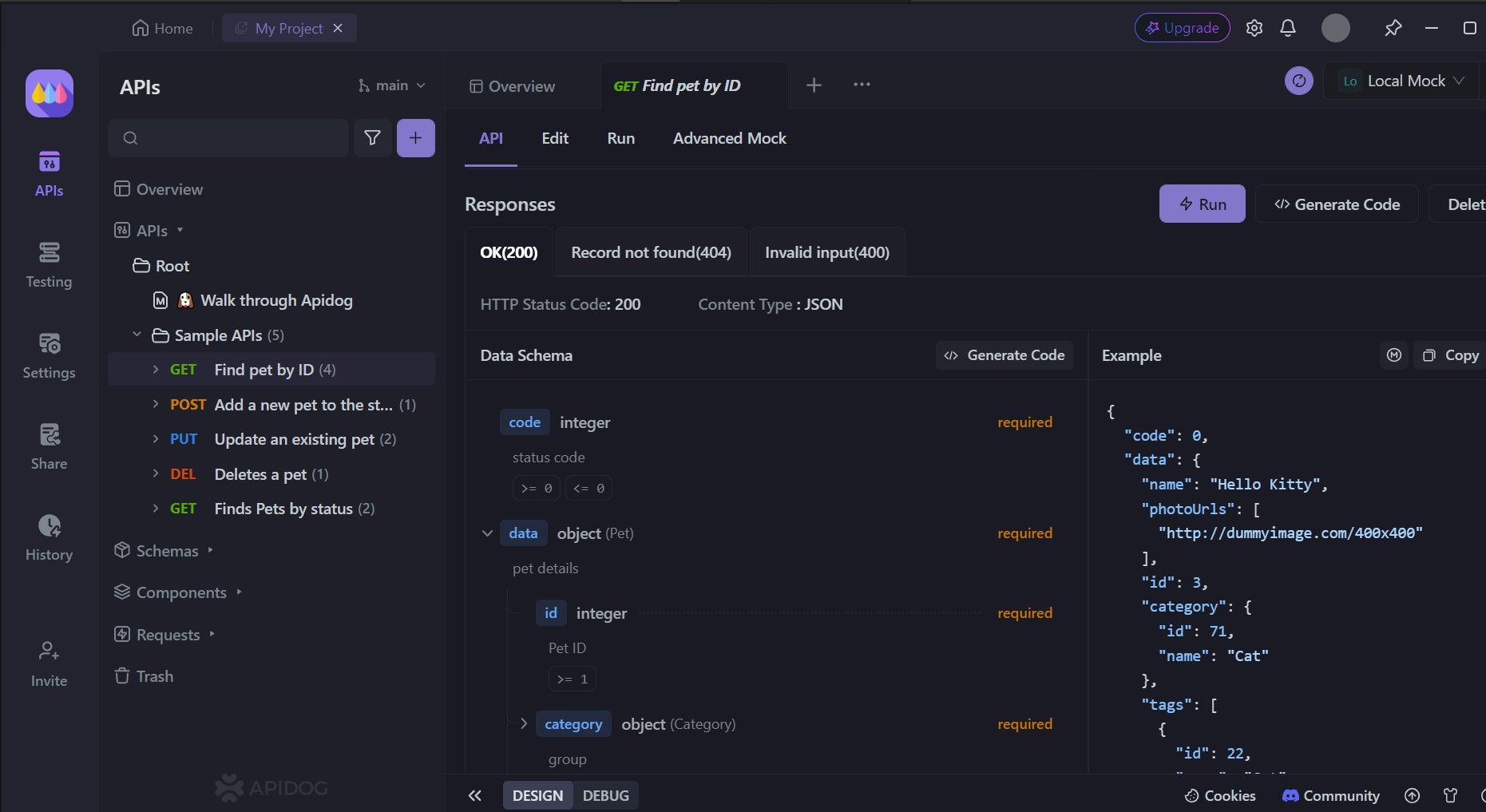
If you wish to create your API of your own, you can also initialize your very own status codes and responses. Don't worry if you feel like you are having a coder's "Writer's Block" - Apidog has its unique feature: API Hub.
Using Apidog's API Hub For Inspiration and Help
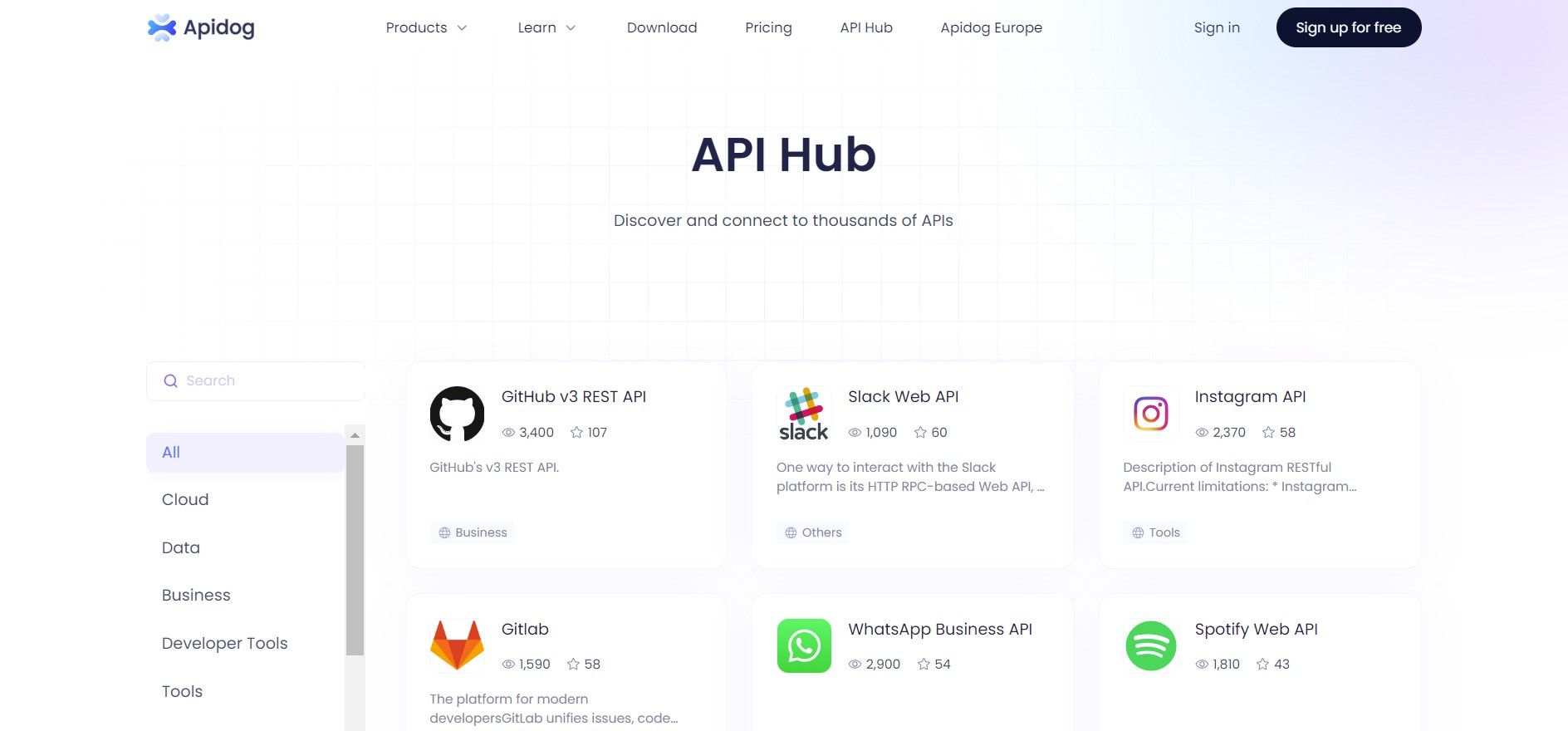
With API Hub, you can find thousands of third-party APIs that are waiting to collaborate with your very own API. So, don't let the sky be the limit of your imagination - get creative and start building APIs with Apidog today!
Conclusion
JavaScript HTTP PUT methods are a crucial tool in web development for updating existing resources on a server. They offer a reliable and standardized approach to modifying data, ensuring that applications can maintain data integrity and accuracy over time.
Key characteristics of JavaScript HTTP PUT methods include their idempotent nature, which ensures that repeated requests have the same effect as making them once, and their suitability for updating entire resources or significant portions of data in a single operation.
To ensure that JavaSCript HTTP PUT methods are returning usable responses, make sure to utilize a trustable API platform like Apidog to view and analyze the API responses. With Apidog, you can also test and debug APIs, so why don't you consider Apidog today?