The Quasar Alpha API is an exciting new tool in the AI landscape, offering developers a chance to interact with a prerelease long-context foundation model. Launched by OpenRouter , Quasar Alpha boasts a 1M token context length, making it ideal for coding tasks while also serving as a general-purpose model. Whether you're building applications, testing AI capabilities, or exploring innovative use cases, this API provides a robust platform to experiment with cutting-edge technology.
What Is the Quasar Alpha API?
The Quasar Alpha API is a prerelease model hosted by OpenRouter, designed for developers to test and provide feedback on an upcoming foundation model. According to OpenRouter’s announcement, Quasar Alpha offers a 1M token context length, is optimized for coding, and is available for free during its alpha phase. However, it comes with some limitations, such as heavy rate-limiting and logging of all prompts and completions for analysis by OpenRouter and its partner lab.
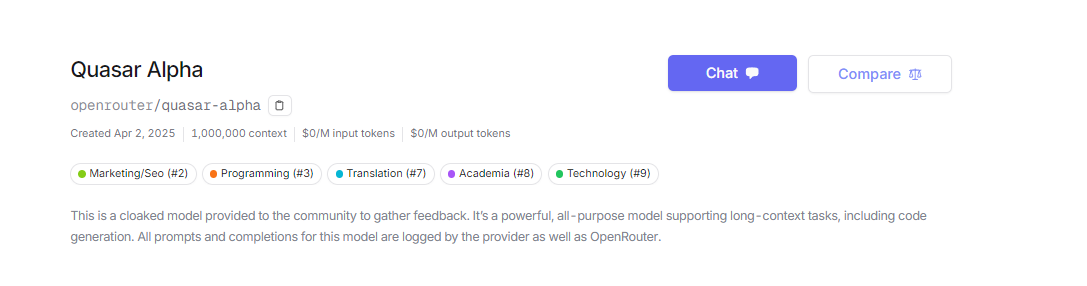
This API is compatible with OpenAI’s completion API, meaning you can use it directly or through the OpenAI SDK. This compatibility makes it accessible for developers familiar with OpenAI’s ecosystem, but it also opens the door for unique experimentation with Quasar Alpha’s long-context capabilities. Now, let’s set up your environment to start using the API.
Step 1: Setting Up Your Environment for Quasar Alpha API
To begin, ensure your development environment is ready to interact with the Quasar Alpha API. Follow these steps to get started.
1.1 Get Your API Key from OpenRouter
First, visit the OpenRouter website and sign up for an account if you don’t already have one.
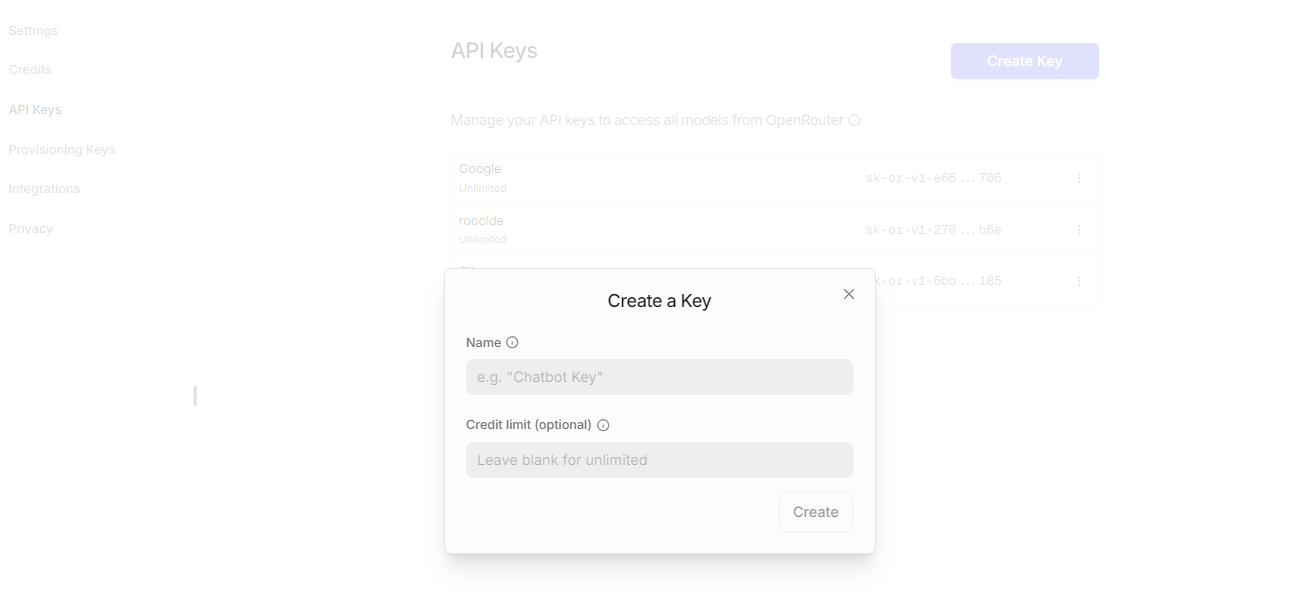
Once logged in, navigate to the API section to generate your API key. This key will authenticate your requests to the Quasar Alpha API. Keep it secure and avoid sharing it publicly.
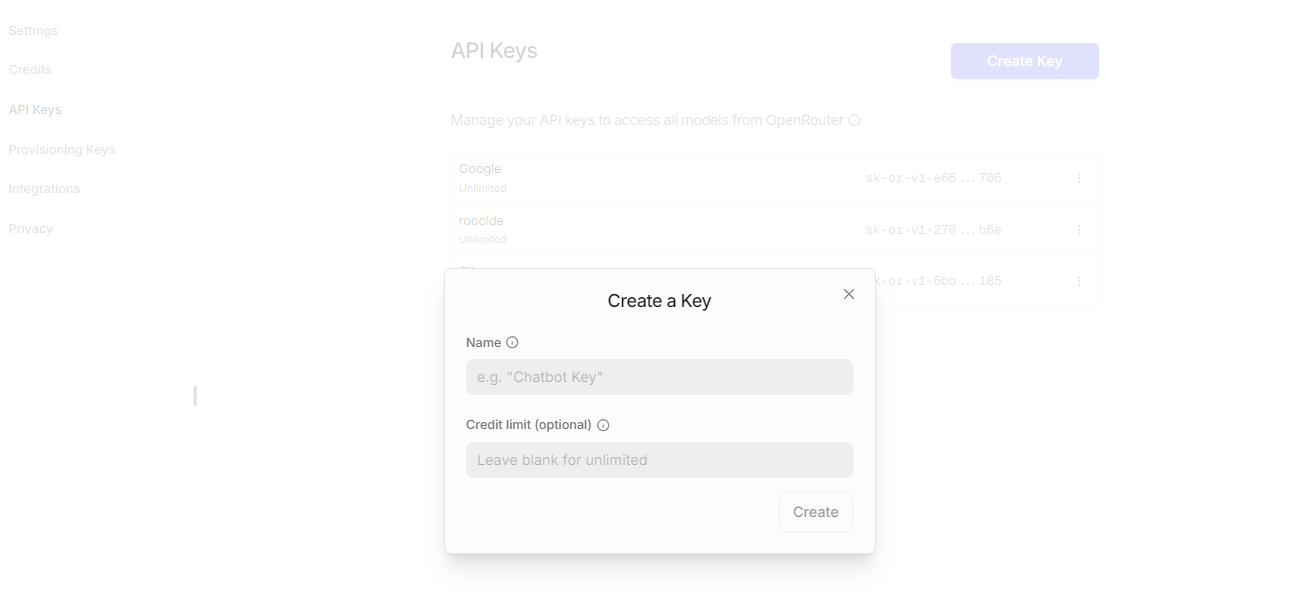
1.2 Install Required Tools
Next, install the necessary tools to make HTTP requests. You can use a programming language like Python with the requests
library or a tool like Apidog for a more streamlined experience. For Python, install the requests
library using pip:
pip install requests
1.3 Verify Your Setup
Finally, test your API key by making a simple request to the OpenRouter API. Use the base URL for Quasar Alpha: https://openrouter.ai/api/v1
. A quick way to verify is to check your account status with a GET request. Here’s an example using Python:
import requests
api_key = "your-api-key-here"
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json"
}
response = requests.get("https://openrouter.ai/api/v1/auth/status", headers=headers)
print(response.json())
If successful, you’ll receive a JSON response confirming your authentication. With your environment ready, let’s move on to making your first API call.
Step 2: Making Your First Quasar Alpha API Call
Now that your environment is set up, let’s make a basic API call to the Quasar Alpha API. The API follows an OpenAI-compatible structure, so you’ll use the /chat/completions
endpoint to interact with the model.
2.1 Construct the API Request
Start by crafting a POST request to the Quasar Alpha endpoint. The base URL is https://openrouter.ai/api/v1/chat/completions
, and you’ll need to specify the model as quasar-alpha
. Here’s a Python example:
import requests
api_key = "your-api-key-here"
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json",
"HTTP-Referer": "your-app-url", # Optional, for OpenRouter leaderboards
"X-Title": "Your App Name" # Optional, for OpenRouter leaderboards
}
data = {
"model": "quasar-alpha",
"messages": [
{"role": "user", "content": "Write a Python function to calculate the factorial of a number."}
],
"max_tokens": 500
}
response = requests.post("https://openrouter.ai/api/v1/chat/completions", json=data, headers=headers)
print(response.json())
2.2 Understand the Response
The API will return a JSON response containing the model’s output. For the above request, you might receive something like this:
{
"id": "chatcmpl-abc123",
"object": "chat.completion",
"created": 1698771234,
"model": "quasar-alpha",
"choices": [
{
"message": {
"role": "assistant",
"content": "Here's a Python function to calculate the factorial of a number:\n\n```python\ndef factorial(n):\n if n < 0:\n raise ValueError(\"Factorial is not defined for negative numbers\")\n if n == 0 or n == 1:\n return 1\n result = 1\n for i in range(2, n + 1):\n result *= i\n return result\n```\n\nThis function uses a loop to compute the factorial iteratively, which is more memory-efficient than a recursive approach for large numbers."
},
"finish_reason": "stop"
}
],
"usage": {
"prompt_tokens": 15,
"completion_tokens": 85,
"total_tokens": 100
}
}
The choices
field contains the model’s response, while usage
provides token usage details. Since Quasar Alpha logs all prompts and completions, be mindful of the data you send. Let’s now explore how to handle more complex requests.
Step 3: Advanced Usage of Quasar Alpha API for Coding Tasks
Given Quasar Alpha’s optimization for coding, let’s dive into a more advanced example: generating a full webpage. The 1M token context length allows you to provide extensive context, making it ideal for complex tasks.
3.1 Generate a Webpage with HTML, CSS, and JavaScript
Send a detailed prompt to create a webpage. Here’s an example request:
data = {
"model": "quasar-alpha",
"messages": [
{"role": "user", "content": "Create a webpage with HTML, CSS, and JavaScript that displays a counter with increment and decrement buttons."}
],
"max_tokens": 1000
}
response = requests.post("https://openrouter.ai/api/v1/chat/completions", json=data, headers=headers)
print(response.json()['choices'][0]['message']['content'])
The response might include a complete webpage code, such as:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Counter App</title>
<style>
body { font-family: Arial, sans-serif; text-align: center; padding: 50px; }
#counter { font-size: 2em; margin: 20px; }
button { padding: 10px 20px; font-size: 1em; margin: 5px; }
</style>
</head>
<body>
<h1>Counter App</h1>
<div id="counter">0</div>
<button onclick="increment()">Increment</button>
<button onclick="decrement()">Decrement</button>
<script>
let count = 0;
const counterElement = document.getElementById('counter');
function increment() {
count++;
counterElement.textContent = count;
}
function decrement() {
count--;
counterElement.textContent = count;
}
</script>
</body>
</html>
3.2 Handle Rate Limits
Quasar Alpha is heavily rate-limited during its alpha phase. If you encounter a 429 error (Too Many Requests), implement exponential backoff in your code to retry requests. Here’s a simple example:
import time
def make_request_with_backoff(data, headers, max_retries=5):
for attempt in range(max_retries):
response = requests.post("https://openrouter.ai/api/v1/chat/completions", json=data, headers=headers)
if response.status_code == 429:
wait_time = 2 ** attempt
print(f"Rate limit hit, waiting {wait_time} seconds...")
time.sleep(wait_time)
continue
return response
raise Exception("Max retries exceeded due to rate limiting")
response = make_request_with_backoff(data, headers)
print(response.json())
With these advanced techniques, you’re ready to test and debug your API interactions more effectively. Let’s now use Apidog to streamline this process.
Step 4: Testing Quasar Alpha API with Apidog
Apidog is a comprehensive API testing tool that simplifies the process of interacting with APIs like Quasar Alpha. Its features, such as environment management and scenario simulation, make it ideal for developers. Let’s see how to use Apidog to test the Quasar Alpha API.
4.1 Set Up Apidog
First, download and install Apidog from apidog.com. Once installed, create a new project and add the Quasar Alpha API endpoint: https://openrouter.ai/api/v1/chat/completions
.
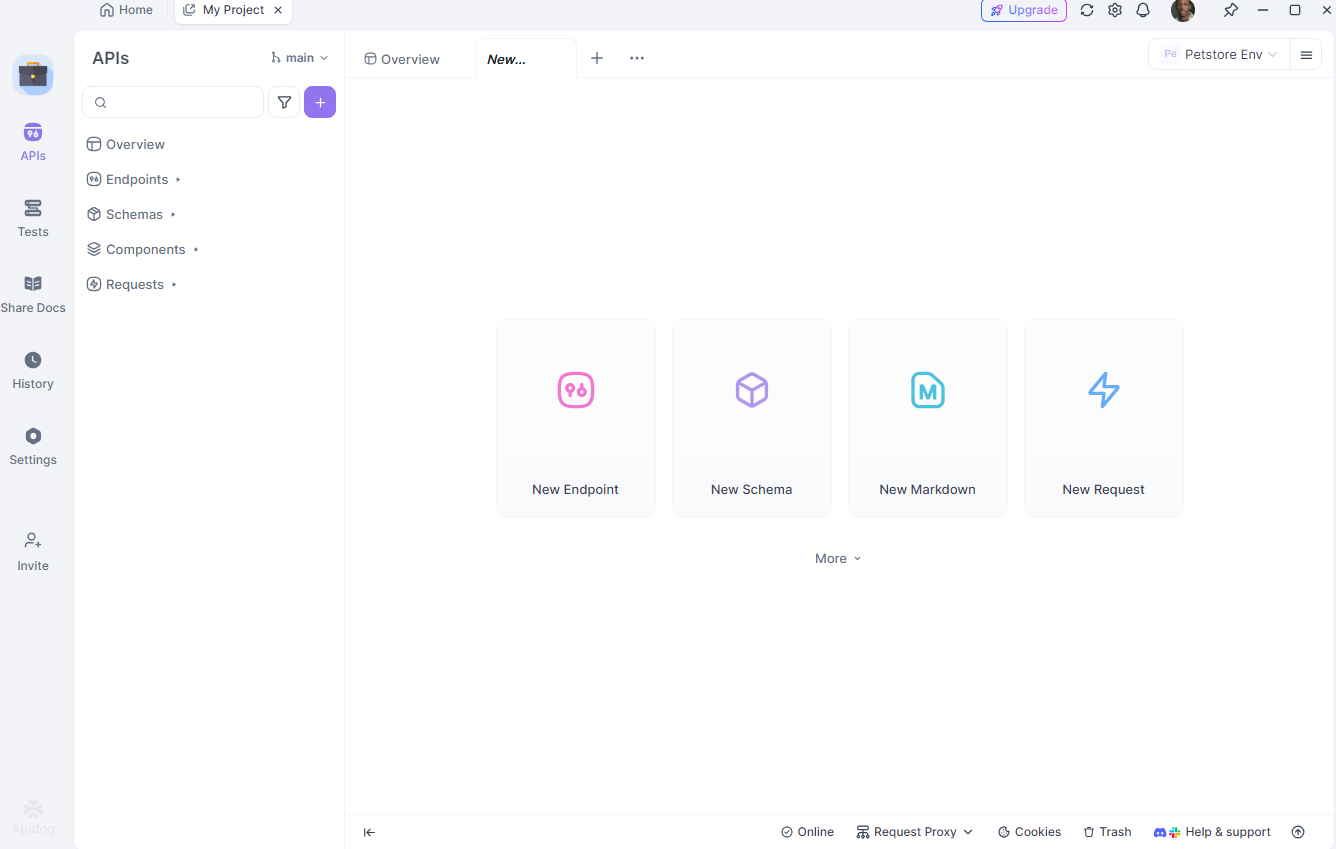
4.2 Configure Your Environment
Next, set up different environments (e.g., development and production) in Apidog. Define variables like your API key and base URL to easily switch between setups. In Apidog, go to the “Environments” tab and add:
api_key
: Your OpenRouter API keybase_url
:https://openrouter.ai/api/v1
4.3 Create a Test Request
Now, create a new POST request in Apidog.
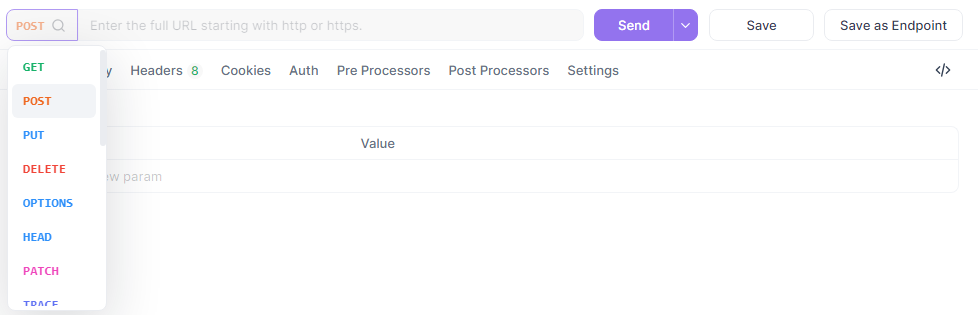
Set the URL to {{base_url}}/chat/completions
, add your headers, and input the JSON body:
{
"model": "quasar-alpha",
"messages": [
{"role": "user", "content": "Explain the difference between let and const in JavaScript."}
],
"max_tokens": 300
}
In the headers section, add:
Authorization
:Bearer {{api_key}}
Content-Type
:application/json
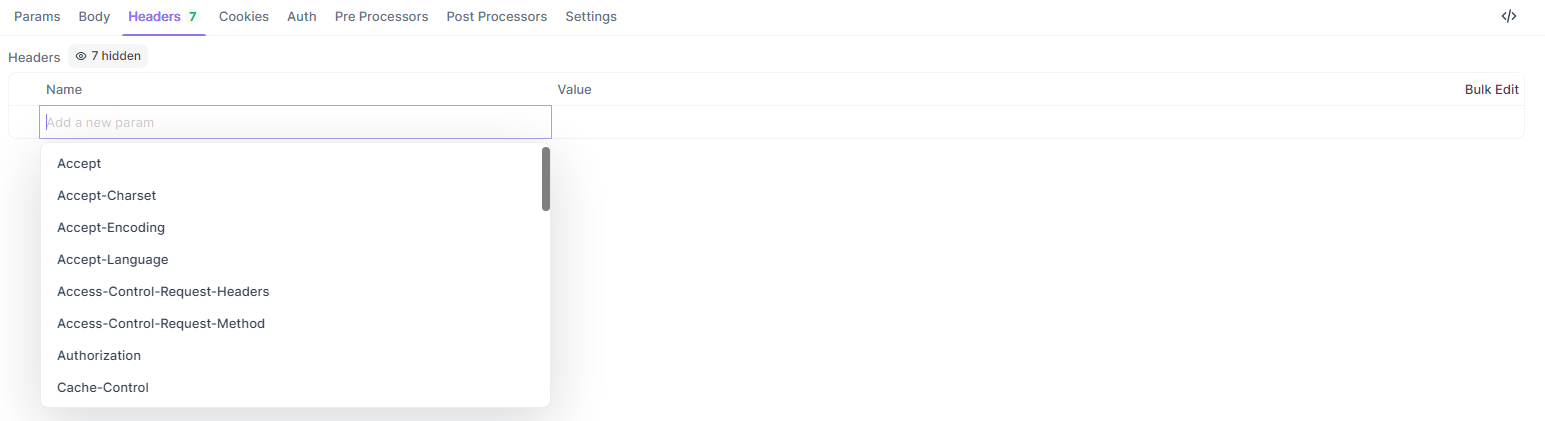
4.4 Run and Analyze the Test
Finally, send the request and analyze the response in Apidog’s visual interface. Apidog provides detailed reports, including response time, status code, and token usage. You can also save this request as a reusable scenario for future testing.

Apidog’s ability to simulate real-world scenarios and generate exportable reports makes it a powerful tool for debugging and optimizing your interactions with the Quasar Alpha API. Let’s wrap up with some best practices.
Conclusion
The Quasar Alpha API offers a unique opportunity to experiment with a prerelease long-context model, particularly for coding tasks. By setting up your environment, making API calls, leveraging advanced features, and testing with tools like Apidog, you can unlock the full potential of this API. Whether you’re a developer building applications or an AI enthusiast exploring new models, Quasar Alpha provides a powerful platform to innovate.
Start using the Quasar Alpha API today, and don’t forget to share your feedback with OpenRouter to help shape the future of this model. Happy coding!
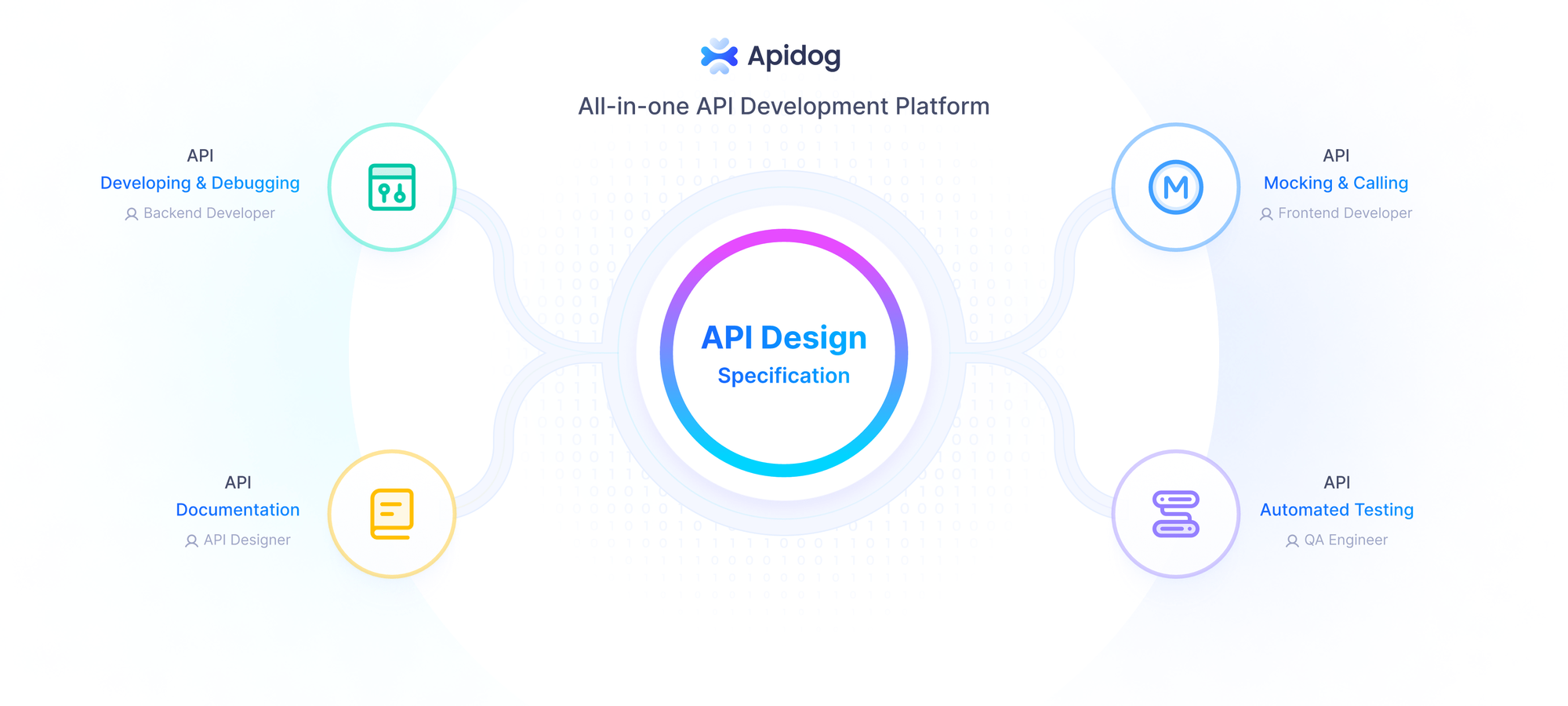