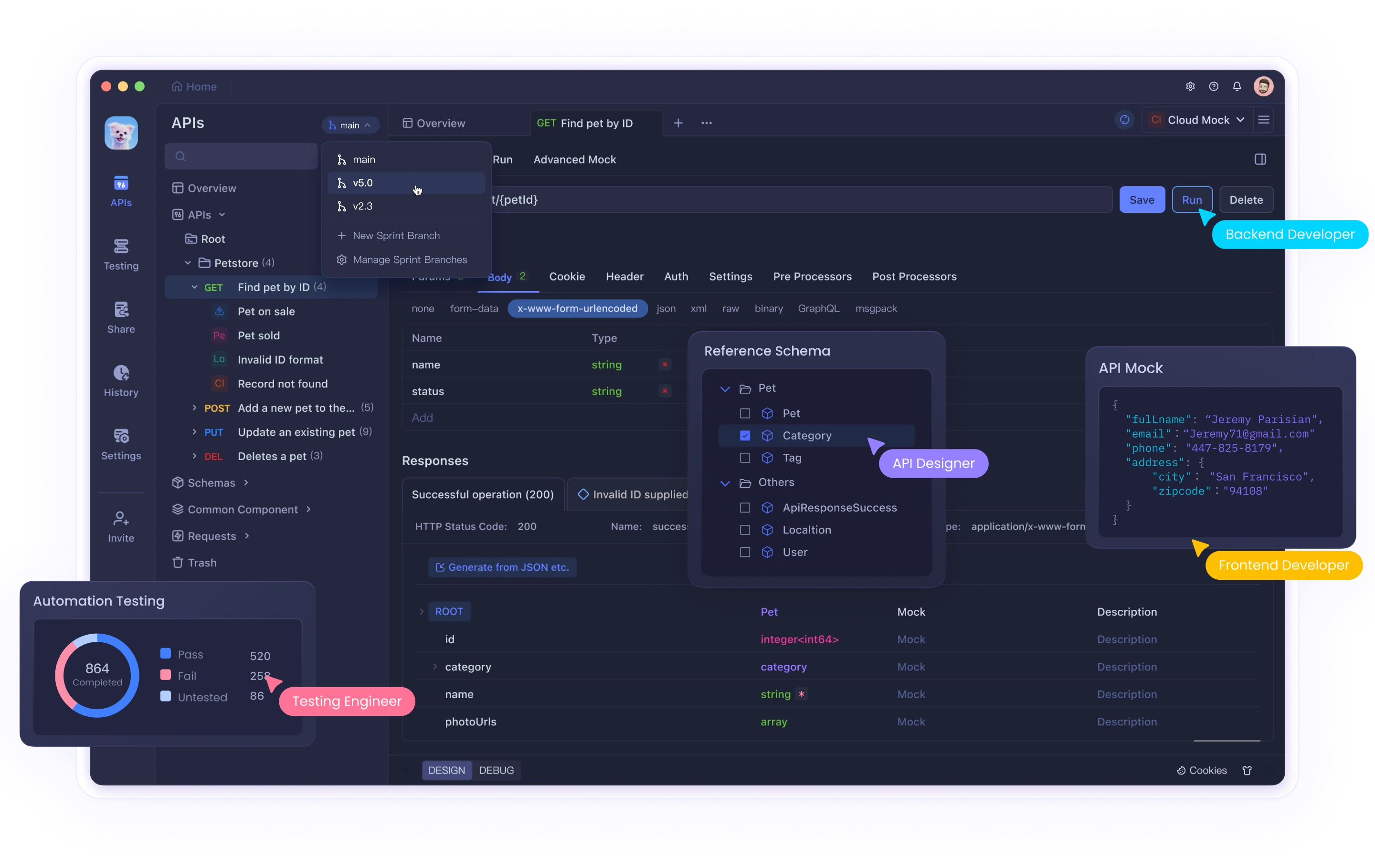
In today's fast-evolving digital landscape, artificial intelligence and advanced language models have become indispensable tools for developers and businesses alike. Anthropic’s Claude API is one of these avant-garde solutions that offer the ability to integrate conversational AI into applications, enabling dynamic chatbots, smart assistants, and automated customer support systems. For developers looking to experiment or integrate Claude into their projects without incurring additional costs in 2025, there are several ways to gain free access to an API key. This guide will walk you through three practical options to get started for free, complete with step-by-step instructions and sample code for each.
Introduction
Claude’s conversational abilities make it a powerful tool for developing innovative applications. Whether you are a seasoned developer or a curious newcomer, learning how to integrate and utilize Claude can open up opportunities to leverage state-of-the-art AI technology. While many platforms come with subscription fees or pay-as-you-go models, there are methods to use the Claude API key without spending a dime—at least in the initial stages. This article will help you navigate three different approaches:
- Using the Official Free Trial Access
- Leveraging a Community SDK for Claude
- Deploying a Serverless Reverse Proxy for API Requests
Each option comes with detailed steps and sample code to prove that free exploration is possible in 2025. Remember, these methods are part of a developer’s toolkit to experiment and build prototypes. As you grow your application or require higher usage volumes, transitioning to a paid plan may become necessary.
Prerequisites
Before diving into the available options, ensure that you have the following prerequisites installed and ready:
- A basic understanding of RESTful API calls.
- Familiarity with a programming language such as Python or JavaScript.
- An active internet connection and access to a code editor.
- (Optional) Access to a cloud platform that supports serverless functions if you choose Option 3.
Option 1: Official Free Trial Access
Overview
Many API providers, including those offering access to Claude, often provide new users with a free trial. These trials are intended to let developers explore the capabilities of the API without an immediate financial commitment. The free trial typically includes a set amount of free credits or a limited-time access period during which you can try out various functionalities.
Step-by-Step Process
Sign Up for a Developer Account:
- Start by visiting the official Claude API website.
- Navigate to the registration page and create a developer account.
- Complete the registration process by providing your email, selecting a password, and verifying your email address.
Access the API Dashboard:
- Once your account is set up and verified, log in to the dashboard.
- Locate the section where API keys are provided.
- Request your free API key, which should now be available due to the promotional free trial offer.
Review Documentation and Test Endpoints:
- Take time to browse through the available documentation.
- Familiarize yourself with the endpoints, request/response formats, and available parameters.
- Use the testing tool provided on the dashboard (if available) to send a test request and ensure your API key is active.
Make Your First API Call:
- Use the API key in your application to issue a basic API call.
- Below is a sample code snippet in Python demonstrating a simple API call to Claude:
import requests
import json
# Replace with your actual API key
API_KEY = 'YOUR_API_KEY_HERE'
# Define headers including the API key
headers = {
'Authorization': f'Bearer {API_KEY}',
'Content-Type': 'application/json'
}
# Prepare the data payload, including the prompt and other parameters
data = {
'prompt': 'Hello Claude! How can you assist me today?',
'max_tokens': 50
}
# Make the POST request to the Claude API endpoint
response = requests.post('https://api.anthropic.com/v1/complete', headers=headers, json=data)
# Parse and print the API response
result = response.json()
print(result)
Experiment with Different Prompts:
- Change the prompt and adjust parameters such as
max_tokens
to see how the API responds. - Use this phase to understand rate limits and manage your free trial credits wisely.
Things to Note
- The free trial may have usage limits and the API key might expire after a set number of requests or days. Make sure to review your dashboard for any notifications.
- This option is ideal for those who prefer a straightforward approach with official support and documentation.
Option 2: Leveraging a Community SDK for Claude
Overview
The open-source community is extremely resourceful, and many developers build SDKs (Software Development Kits) that simplify the process of interacting with APIs like Claude. These SDKs are designed to abstract some of the complexities of direct HTTP requests and provide a more seamless integration process. Many of these solutions are free and garner community support through forums, GitHub, or other channels.
Step-by-Step Process
- Locate a Trusted Community SDK:
- Search for popular community-driven libraries that provide a wrapper around the Claude API.
- Platforms like GitHub are ideal for finding an SDK that suits your project’s needs.
- Install the SDK:
- Install the SDK using your package manager of choice.
- For a Node.js environment, you might install the SDK via npm. For example:
npm install anthropic-sdk
Set Up Your Environment:
- After installation, configure your local environment by setting the acquired API key as an environment variable.
- This approach helps keep your credentials safe from hardcoding directly into your source code.
Use the SDK to Make API Requests:
- The SDK will offer built-in methods to interact with the Claude API.
- Below is a sample code snippet in Node.js using a community SDK:
// Import the Claude client from the community SDK
const { ClaudeClient } = require('anthropic-sdk');
// Initialize the client with your API key from the environment variable
const client = new ClaudeClient(process.env.CLAUDE_API_KEY);
(async () => {
try {
// Use the 'complete' method provided by the SDK
const response = await client.complete({
prompt: "Hello Claude, what's the weather like today?",
max_tokens: 50
});
console.log("API Response:", response);
} catch (error) {
console.error("Error communicating with Claude API:", error);
}
})();
- Debug and Customize:
- Use the debugging tools provided by the SDK to troubleshoot any issues.
- Customize the request parameters as needed to fit your application’s requirements.
Things to Note
- Community SDKs may come with additional features such as streamlined error handling, logging, and pre-built utilities to help you manage responses.
- Always check the SDK's repository for updates and best practices to ensure security and performance.
Option 3: Deploying a Serverless Reverse Proxy
Overview
Another innovative approach to using the Claude API key for free is by deploying a serverless function that acts as a reverse proxy. This method essentially allows you to manage your API key on the backend securely while exposing a limited public endpoint that your front-end application can access. This setup is especially useful for prototype applications where you need to hide your API key from the client side.
Step-by-Step Process
Set Up a Serverless Platform:
- Choose a serverless platform such as AWS Lambda, Google Cloud Functions, or Vercel.
- Create a new function or project within your chosen environment.
Configure Environment Variables:
- Securely store your API key by setting it as an environment variable in your serverless environment.
- This ensures that the key is never exposed in your client-side code.
Develop the Reverse Proxy Function:
- Create a function that accepts incoming HTTP requests from your application.
- The function should then forward the request to the Claude API, including the necessary headers with your API key.
- Below is an example of an AWS Lambda function written in Python:
import os
import json
import requests
def lambda_handler(event, context):
# Retrieve the API key from environment variables
api_key = os.getenv('CLAUDE_API_KEY')
# Extract the prompt parameter from the incoming event
prompt = event.get('queryStringParameters', {}).get('prompt', 'Hello, Claude!')
# Set up headers for the Claude API request
headers = {
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json'
}
# Prepare the payload data
payload = {
'prompt': prompt,
'max_tokens': 50
}
# Make the POST request to the Claude API
response = requests.post('https://api.anthropic.com/v1/complete', headers=headers, json=payload)
# Return the API response back to the client
return {
'statusCode': 200,
'headers': { 'Content-Type': 'application/json' },
'body': response.text
}
Deploy and Test the Function:
- Deploy your function on the serverless platform.
- Test the endpoint using tools like Postman or simply by accessing the URL in your web application.
- Verify that your API key is securely managed and that the function returns the desired Claude API responses.
Integrate the Endpoint with Your Application:
- Modify your client-side code to call your serverless endpoint instead of directly invoking the Claude API.
- This abstraction helps minimize security risks while still offering a free route to test the Claude API functionalities.
Things to Note
- Reverse proxy setups not only secure your API keys by keeping them hidden from the client-side but also allow you to enforce additional security measures like rate limiting.
- Be mindful of the free tier limits on your chosen serverless platform. Many providers offer generous free usage quotas, but understanding the limits will help prevent unexpected downtimes.
Best Practices When Using Free API Keys
While exploring these free methods for using the Claude API key, it is essential to maintain good security hygiene. Here are some best practices to consider:
- Secure Storage: Always store your API key in environment variables or secure vaults rather than embedding them directly in your code.
- Monitor Usage: Keep track of the number of requests made using your API key. Free trial accounts may have rate limits or daily quotas, so it’s crucial to monitor your usage to avoid abrupt interruptions.
- Error Handling: Implement robust error handling in your application. Whether you’re making direct API calls or using a reverse proxy, handle responses gracefully to provide fallback options if the service is unavailable.
- Regular Updates: Stay updated with the API provider’s announcements regarding usage policies. Even free access models may change over time, and you must ensure that your integration is compliant with the latest guidelines.
- Transition Plans: While free access is ideal for testing and prototyping, have a strategy in place should your application scale up. Transitioning to a paid plan or exploring enterprise-level options early on can save you headaches later.
Conclusion
Accessing powerful conversational AI like Claude for free in 2025 is entirely feasible with the right approach. Whether you choose to sign up for an official free trial, leverage a community SDK, or deploy a reverse proxy using serverless functions, each method provides a unique set of advantages tailored to different development needs.
- Option 1 is perfect for those who prefer official support and ready-made documentation, making it easy to get started without extensive setups.
- Option 2 leverages the ingenuity of the developer community, with SDKs that simplify integration and provide additional features. This can make building applications with Claude more intuitive, especially for developers already comfortable with Node.js or similar environments.
- Option 3 offers a robust way to secure your API key and control access to the API through a backend proxy. This method is ideal when security is a paramount concern and can also help centralize logging and monitoring for all API interactions.
Each option comes with its own practical code samples and easy-to-follow instructions. By following these steps, you can integrate and experiment with the Claude API without upfront costs, enabling you to innovate, test new ideas, and build applications that can later be adapted to larger-scale production environments.
In the versatile realm of modern application development, free access gateways such as these empower developers to explore the latest advances in AI-driven technology without the burden of initial costs. Your journey into integrating Claude begins with understanding these tools and best practices, and as you progress, you will find that your early prototyping efforts lay a strong foundation for future innovation.
With this guide at your disposal, you now have three viable paths to leverage the Claude API key for free in 2025. Experiment, test, and customize these methods as per your project requirements, and embrace the exciting prospects that AI-driven conversations bring to your applications.
Happy coding!