Google BigQuery has revolutionized the way organizations handle large-scale data analytics. Its serverless architecture, scalability, and familiar SQL interface make it a powerful tool for deriving insights from massive datasets. While interacting with BigQuery through the Google Cloud Console or the bq
command-line tool is common, the true power of automation, integration, and custom application development is unlocked through its comprehensive set of Application Programming Interfaces (APIs).
This guide provides a step-by-step exploration of how to use BigQuery APIs, enabling you to programmatically interact with your data warehouse, build data pipelines, integrate BigQuery into your applications, and manage resources effectively. We'll cover the different types of APIs available, how to set up your environment, practical examples using the Python client library, and introduce specialized APIs for advanced use cases.
Want an integrated, All-in-One platform for your Developer Team to work together with maximum productivity?
Apidog delivers all your demans, and replaces Postman at a much more affordable price!
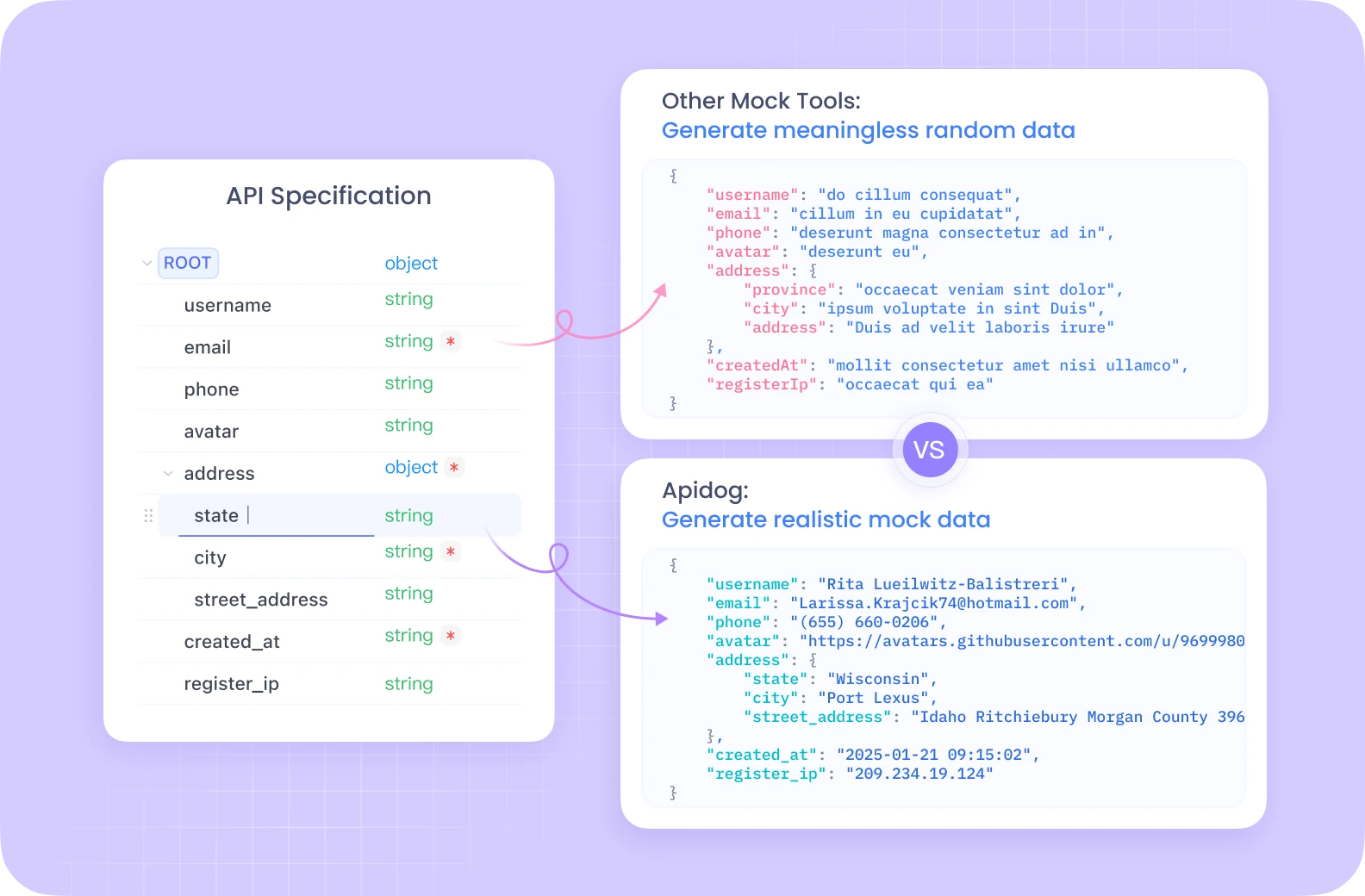
Understanding BigQuery APIs
Before diving into code, it's crucial to understand the core concepts and the different ways you can interact with BigQuery programmatically.
Core BigQuery Concepts:
- Project: The top-level container in Google Cloud, holding all your resources, including BigQuery datasets, tables, and jobs. Billing and permissions are typically managed at the project level.
- Dataset: A container for tables and views within a specific project. Datasets help organize your data and control access. Think of them like schemas or databases in traditional systems.
- Table: Stores your structured data in rows and columns with a defined schema. BigQuery supports various data types and nested/repeated fields.
- Job: Represents asynchronous actions performed in BigQuery, such as running queries, loading data, exporting data, or copying tables. APIs allow you to start, monitor, and manage these jobs.
Types of BigQuery APIs:
BigQuery offers several ways to interact with its services programmatically:
REST API: This is the foundational API built on HTTP and JSON. It provides direct access to BigQuery resources and operations. You can interact with it using standard HTTP requests (GET, POST, PUT, DELETE) targeting specific endpoints (e.g., https://bigquery.googleapis.com/bigquery/v2/projects/{projectId}/datasets
). While powerful and offering fine-grained control, using the REST API directly requires handling authentication, request formatting, response parsing, and error handling manually. Authentication typically involves OAuth 2.0 access tokens.
Client Libraries: Google provides high-level client libraries for various popular programming languages (including Python, Java, Go, Node.js, C#, PHP, Ruby). These libraries wrap the underlying REST API, offering a more idiomatic, developer-friendly experience. They simplify common tasks, handle authentication (often automatically via Application Default Credentials), manage retries, and reduce the amount of boilerplate code you need to write. This is the recommended approach for most application development.
Specialized APIs: For specific high-performance or specialized tasks, BigQuery offers dedicated APIs:
- BigQuery Storage Read API: Designed for high-throughput data reading directly from BigQuery managed storage. It's significantly faster than standard query results export, especially for large datasets, and integrates well with data processing frameworks like Apache Spark, Apache Beam, and Pandas. It allows for parallel reading via multiple streams.
- BigQuery Connection API: Enables you to create and manage connections to external data sources, such as Cloud SQL, Spanner, or Cloud Storage. This allows you to query data in these external systems directly from BigQuery using the
EXTERNAL_QUERY
function, without needing to load the data into BigQuery first. - Analytics Hub API: Facilitates secure and scalable data sharing across different organizations or business units. It allows you to create "Listings" – shareable references to datasets – within "Data Exchanges". Consumers can subscribe to these listings to access the shared data within their own projects.
- BigLake API: Used to manage BigLake tables. BigLake allows you to create tables in BigQuery that operate over data stored in open formats (like Parquet, ORC, Avro) in data lakes (primarily Google Cloud Storage). This provides a unified interface for querying both managed BigQuery storage and data lake storage with consistent security and governance.
Setting Up Your Environment
Before you can start making API calls, you need to configure your local or server environment.
Prerequisites:
- Google Cloud Account: You need an active Google Cloud account.
- Google Cloud Project: Create a new project or select an existing one in the Google Cloud Console. Note your Project ID.
- Enable BigQuery API: Ensure the BigQuery API is enabled for your project. You can do this via the Cloud Console (APIs & Services > Library > Search for "BigQuery API" > Enable). You might also need to enable other APIs like the BigQuery Storage Read API or BigQuery Connection API depending on your use case.
- Billing: Ensure billing is enabled for your project. BigQuery operations incur costs based on data storage, analysis processed, and streaming inserts.
Authentication:
Your application needs to authenticate to Google Cloud to prove its identity and authorization to access BigQuery resources. The recommended method for most scenarios is Application Default Credentials (ADC).
- Application Default Credentials (ADC): This strategy allows your application to find credentials automatically based on the environment it's running in, without needing to embed keys directly in code.
- Local Development: Install the Google Cloud CLI (
gcloud
). Run the commandgcloud auth application-default login
. This will open a browser window for you to log in with your Google account, granting the SDK access to your credentials. Client libraries will automatically detect these credentials. - Compute Engine, Cloud Functions, App Engine, etc.: ADC automatically uses the service account associated with the resource where your code is running.
- Service Accounts: For applications running outside Google Cloud or requiring specific permissions, you can use service accounts.
- Create a service account in the Cloud Console (IAM & Admin > Service Accounts).
- Grant the necessary BigQuery roles (e.g.,
BigQuery Data Editor
,BigQuery Job User
,BigQuery User
) to the service account. - Download the service account key file (JSON format).
- Set the environment variable
GOOGLE_APPLICATION_CREDENTIALS
to the absolute path of the downloaded JSON key file. Client libraries will automatically use this key file for authentication if the environment variable is set.
Installing Client Libraries (Python Example):
We'll focus on Python for our examples. You can install the necessary libraries using pip
:
pip install google-cloud-bigquery
# Optional: Install storage API library for faster reads
pip install google-cloud-bigquery-storage
# Optional: Install pandas integration and db-dtypes for better type handling
pip install pandas db-dtypes pyarrow
Ensure you have Python installed (version 3.7+ recommended for the latest library features).
Using the BigQuery Client Library (Python Examples)
Now, let's explore common BigQuery operations using the google-cloud-bigquery
Python library.
1. Importing and Initializing the Client:
First, import the library. Then, create a client instance. If ADC is configured correctly, the client will authenticate automatically.
from google.cloud import bigquery
import pandas as pd
# Construct a BigQuery client object.
# If GOOGLE_APPLICATION_CREDENTIALS is set, it uses the service account.
# If gcloud auth application-default login was run, it uses those credentials.
# If running on GCP infra, it uses the instance's service account.
client = bigquery.Client()
# You can explicitly specify the project ID if needed,
# otherwise it often infers from the environment/ADC credentials.
# client = bigquery.Client(project='your-project-id')
print("Client created successfully.")
2. Running Queries:
The most common operation is running SQL queries.
- Simple Synchronous Query: For queries expected to complete quickly.
query_and_wait()
sends the query and waits for results.
# Define your SQL query
query = """
SELECT name, SUM(number) as total_people
FROM `bigquery-public-data.usa_names.usa_1910_2013`
WHERE state = 'TX'
GROUP BY name, state
ORDER BY total_people DESC
LIMIT 10
"""
# Make an API request and wait for the job to complete.
query_job = client.query(query) # API request
print(f"Started job: {query_job.job_id}")
# Wait for the job to complete and get results.
rows = query_job.result() # Waits for job to complete.
print("\nTop 10 names in TX (1910-2013):")
for row in rows:
# Row values can be accessed by field name or index.
print(f"Name: {row.name}, Count: {row['total_people']}") # Access by attribute or key
# Convert results to a Pandas DataFrame
df = rows.to_dataframe()
print("\nResults as Pandas DataFrame:")
print(df.head())
- Asynchronous Queries: For long-running queries, start the job and check its status later.
query = """
SELECT corpus, COUNT(word) as distinct_words
FROM `bigquery-public-data.samples.shakespeare`
GROUP BY corpus
ORDER BY distinct_words DESC;
"""
job_config = bigquery.QueryJobConfig(
# Use standard SQL syntax for queries.
use_legacy_sql=False
)
# Start the query, passing in the extra configuration.
query_job = client.query(query, job_config=job_config) # Does not wait
print(f"Started asynchronous job: {query_job.job_id}")
# --- Later in your application ---
# Check job status (optional)
# from google.cloud.exceptions import NotFound
# try:
# job = client.get_job(query_job.job_id, location=query_job.location)
# print(f"Job {job.job_id} status: {job.state}")
# if job.state == "DONE":
# if job.error_result:
# print(f"Job failed: {job.errors}")
# else:
# results = job.result() # Get results
# print("Results fetched.")
# # Process results...
# except NotFound:
# print(f"Job {query_job.job_id} not found.")
# Or simply wait for completion when needed
results = query_job.result() # This will block until the job is done
print("Asynchronous job completed.")
for row in results:
print(f"Corpus: {row.corpus}, Distinct Words: {row.distinct_words}")
- Parameterized Queries: Essential for security (preventing SQL injection) when incorporating user input into queries.
from google.cloud.bigquery import ScalarQueryParameter, ArrayQueryParameter, StructQueryParameter, QueryJobConfig
# Example: Find names starting with a specific prefix in a given state
state_param = "NY"
prefix_param = "Ma"
min_count_param = 1000
query = """
SELECT name, SUM(number) as total_people
FROM `bigquery-public-data.usa_names.usa_1910_2013`
WHERE state = @state_abbr AND name LIKE @name_prefix
GROUP BY name
HAVING total_people >= @min_count
ORDER BY total_people DESC;
"""
job_config = QueryJobConfig(
query_parameters=[
ScalarQueryParameter("state_abbr", "STRING", state_param),
# Use 'val%' for LIKE operator
ScalarQueryParameter("name_prefix", "STRING", f"{prefix_param}%"),
ScalarQueryParameter("min_count", "INT64", min_count_param),
]
)
query_job = client.query(query, job_config=job_config)
print(f"Started parameterized query job: {query_job.job_id}")
rows = query_job.result()
print(f"\nNames starting with '{prefix_param}' in {state_param} with >= {min_count_param} people:")
for row in rows:
print(f"Name: {row.name}, Count: {row.total_people}")
3. Managing Datasets:
You can create, list, get details of, and delete datasets.
# Define dataset ID and location
project_id = client.project
dataset_id = f"{project_id}.my_new_dataset"
dataset_location = "US" # e.g., "US", "EU", "asia-northeast1"
# Construct a full Dataset object to send to the API.
dataset = bigquery.Dataset(dataset_id)
dataset.location = dataset_location
dataset.description = "Dataset created via Python client library"
try:
# Make an API request to create the dataset.
dataset = client.create_dataset(dataset, timeout=30) # Make an API request.
print(f"Created dataset {client.project}.{dataset.dataset_id}")
# List datasets in the project
print("\nDatasets in project:")
datasets = list(client.list_datasets()) # API request
if datasets:
for ds in datasets:
print(f"\t{ds.dataset_id}")
else:
print(f"\t{client.project} project does not contain any datasets.")
# Get dataset info
retrieved_dataset = client.get_dataset(dataset_id) # API request
print(f"\nRetrieved dataset info for {dataset_id}:")
print(f"\tDescription: {retrieved_dataset.description}")
print(f"\tLocation: {retrieved_dataset.location}")
except Exception as e:
print(f"Error during dataset operations: {e}")
finally:
# Clean up: Delete the dataset
try:
client.delete_dataset(
dataset_id, delete_contents=True, not_found_ok=True
) # API request
print(f"\nSuccessfully deleted dataset '{dataset_id}'.")
except Exception as e:
print(f"Error deleting dataset {dataset_id}: {e}")
4. Managing Tables:
Similar operations exist for tables: creating tables (defining schema), loading data, getting metadata, and deleting tables.
# Using the previously created dataset ID (ensure it exists or remove deletion step above)
dataset_id_for_table = "my_new_dataset" # Use a valid dataset ID
table_id = f"{client.project}.{dataset_id_for_table}.my_new_table"
# Define the schema
schema = [
bigquery.SchemaField("full_name", "STRING", mode="REQUIRED"),
bigquery.SchemaField("age", "INTEGER", mode="REQUIRED"),
bigquery.SchemaField("email", "STRING", mode="NULLABLE"),
]
# Create the table
table = bigquery.Table(table_id, schema=schema)
try:
# Ensure dataset exists first
client.create_dataset(dataset_id_for_table, exists_ok=True)
table = client.create_table(table) # API request
print(
f"Created table {table.project}.{table.dataset_id}.{table.table_id}"
)
# --- Loading Data (Example: from Pandas DataFrame) ---
data = {'full_name': ['Alice Smith', 'Bob Johnson'],
'age': [30, 45],
'email': ['alice@example.com', None]}
dataframe = pd.DataFrame(data)
job_config = bigquery.LoadJobConfig(
# Specify schema is recommended, ensures proper types
schema=schema,
# Optional: overwrite table data
write_disposition="WRITE_TRUNCATE",
# Or append: write_disposition="WRITE_APPEND",
)
load_job = client.load_table_from_dataframe(
dataframe, table_id, job_config=job_config
) # API request
print(f"Starting job {load_job.job_id} to load data from DataFrame")
load_job.result() # Waits for the job to complete.
print("DataFrame load job finished.")
destination_table = client.get_table(table_id) # API request
print(f"Loaded {destination_table.num_rows} rows into table {table_id}")
# --- Loading Data (Example: from Google Cloud Storage URI) ---
# Assume a CSV file gs://your-bucket/data.csv exists with compatible data
# uri = "gs://your-bucket/data.csv"
# job_config_gcs = bigquery.LoadJobConfig(
# schema=schema,
# skip_leading_rows=1, # Skip header row
# source_format=bigquery.SourceFormat.CSV,
# write_disposition="WRITE_APPEND", # Append to existing data
# )
# load_job_gcs = client.load_table_from_uri(
# uri, table_id, job_config=job_config_gcs
# )
# print(f"Starting job {load_job_gcs.job_id} to load data from GCS")
# load_job_gcs.result()
# print("GCS load job finished.")
# destination_table = client.get_table(table_id)
# print(f"Total rows after GCS load: {destination_table.num_rows}")
except Exception as e:
print(f"Error during table operations: {e}")
finally:
# Clean up: Delete the table
try:
client.delete_table(table_id, not_found_ok=True) # API request
print(f"Successfully deleted table '{table_id}'.")
# Optionally delete the dataset again if it was just for this example
# client.delete_dataset(dataset_id_for_table, delete_contents=True, not_found_ok=True)
# print(f"Successfully deleted dataset '{dataset_id_for_table}'.")
except Exception as e:
print(f"Error deleting table {table_id}: {e}")
5. Working with Jobs:
All asynchronous operations (query, load, export, copy) create Job resources. You can list and manage these jobs.
# List recent jobs
print("\nRecent BigQuery Jobs:")
for job in client.list_jobs(max_results=10): # API request
print(f"Job ID: {job.job_id}, Type: {job.job_type}, State: {job.state}, Created: {job.created}")
# Get a specific job (replace with a valid job ID from previous runs)
# try:
# job_id_to_get = "..." # Replace with a real job ID
# location = "US" # Replace with the job's location if not default
# retrieved_job = client.get_job(job_id_to_get, location=location) # API request
# print(f"\nDetails for job {retrieved_job.job_id}:")
# print(f"\tState: {retrieved_job.state}")
# if retrieved_job.error_result:
# print(f"\tError: {retrieved_job.errors}")
# except NotFound:
# print(f"Job {job_id_to_get} not found.")
# except Exception as e:
# print(f"Error retrieving job: {e}")
Leveraging Specialized APIs (Concepts and Use Cases)
While the core client library covers many use cases, specialized APIs offer enhanced performance or functionality for specific tasks.
1. BigQuery Storage Read API (Python):
- Purpose: Extremely fast data retrieval from BigQuery tables, bypassing the standard query execution engine. Ideal for exporting large datasets or feeding data into ML training pipelines or data processing frameworks.
- How it works: You create a
ReadSession
specifying the table, columns, and row filters. The API returns one or moreStreams
. You read data (typically in Apache Arrow or Avro format) from these streams, often in parallel. - Client Library:
google-cloud-bigquery-storage
- Conceptual Python Usage (with Pandas integration):
# Requires: pip install google-cloud-bigquery-storage pyarrow pandas db-dtypes
from google.cloud import bigquery_storage_v1
from google.cloud.bigquery_storage_v1 import types, GetDataStreamRequest
# --- Using Pandas read_gbq (Simplest integration) ---
# This automatically uses the Storage API if installed and beneficial
# table_id_read = "bigquery-public-data.usa_names.usa_1910_2013"
# cols_to_read = ["name", "number", "state"]
# row_filter = "state = 'CA' AND number > 5000"
#
# try:
# df_storage = pd.read_gbq(
# table_id_read,
# project_id=client.project,
# columns=cols_to_read,
# row_filter=row_filter,
# use_bqstorage_api=True, # Explicitly request Storage API
# progress_bar_type='tqdm' # Optional progress bar
# )
# print("\nRead data using Storage API via pandas.read_gbq:")
# print(df_storage.head())
# print(f"Read {len(df_storage)} rows.")
# except Exception as e:
# print(f"Error reading with Storage API via read_gbq: {e}")
# --- Manual Storage API Usage (More Control) ---
# bqstorageclient = bigquery_storage_v1.BigQueryReadClient()
# table = f"projects/{project_id}/datasets/{dataset_id}/tables/{table_name}" # Replace with your table details
# requested_session = types.ReadSession(
# table=table,
# data_format=types.DataFormat.ARROW,
# read_options=types.ReadSession.TableReadOptions(
# selected_fields=["col1", "col2"], # Specify columns
# row_restriction="col1 > 100" # Specify filter
# ),
# )
# parent = f"projects/{project_id}"
# read_session = bqstorageclient.create_read_session(
# parent=parent,
# read_session=requested_session,
# max_stream_count=1, # Request number of parallel streams
# )
# stream = read_session.streams[0]
# reader = bqstorageclient.read_rows(stream.name)
# frames = [message.arrow_record_batch for message in reader.messages()]
# if frames:
# arrow_table = pa.Table.from_batches(frames)
# df_manual = arrow_table.to_pandas()
# print("\nRead data manually using Storage API:")
# print(df_manual.head())
# else:
# print("No data read using manual Storage API.")
2. BigQuery Connection API:
- Purpose: Query external data sources (Cloud SQL, Spanner, Cloud Storage) directly from BigQuery without data movement.
- How it works:
- Use the API (or Cloud Console/
bq
tool) to create aConnection
resource, specifying the external source type and details. - Grant the connection's service account appropriate permissions on the external resource (e.g., Cloud SQL User role).
- Use the
EXTERNAL_QUERY("connection_id", "external_sql_query")
function within your BigQuery SQL.
- Client Library:
google-cloud-bigquery-connection
provides methods likecreate_connection
,get_connection
,list_connections
,delete_connection
.
3. Analytics Hub API:
- Purpose: Securely share curated datasets across organizations or teams.
- Key Concepts:
- Data Exchange: A container for sharing data. Can be public or private.
- Listing: A reference to a specific BigQuery dataset (or potentially view/table in the future) that is shared within an exchange. Publishers create listings; subscribers access them.
- APIs: Available via REST and client libraries (e.g.,
google-cloud-analyticshub
) for managing exchanges, listings, and subscriptions programmatically.
4. BigLake API:
- Purpose: Manage BigLake tables, which allow querying data in Cloud Storage using BigQuery interface and governance.
- How it works: Define a table in BigQuery that references data files (Parquet, ORC, etc.) in a GCS bucket. The BigLake API (primarily REST at present) is used to create, update, and manage the metadata for these tables. Querying happens through standard BigQuery SQL.
Using the REST API Directly
While client libraries are generally preferred, you might use the REST API directly if:
- You are using a language without an official Google client library.
- You need access to bleeding-edge features not yet exposed in the libraries.
- You require absolute control over the HTTP request/response cycle.
Making Requests:
You'll typically use an HTTP client (like curl
or Python's requests
library). You need to:
- Obtain an OAuth 2.0 Access Token (e.g., using
gcloud auth print-access-token
). - Construct the correct API endpoint URL.
- Create the JSON request body according to the API method's specification.
- Include the access token in the
Authorization: Bearer <token>
header. - Handle the HTTP response (status codes, JSON parsing, error messages).
Example: Running a Query via REST (jobs.query
)
# 1. Get Access Token
TOKEN=$(gcloud auth print-access-token)
# 2. Define Project ID and Request Body
PROJECT_ID="your-project-id" # Replace with your project ID
REQUEST_BODY=$(cat <<EOF
{
"query": "SELECT name, SUM(number) as total_people FROM \`bigquery-public-data.usa_names.usa_1910_2013\` WHERE state = 'CA' GROUP BY name ORDER BY total_people DESC LIMIT 5;",
"useLegacySql": false
}
EOF
)
# 3. Make the API Call using curl
curl -X POST \
"https://bigquery.googleapis.com/bigquery/v2/projects/${PROJECT_ID}/jobs" \
-H "Authorization: Bearer ${TOKEN}" \
-H "Content-Type: application/json; charset=utf-8" \
-d "${REQUEST_BODY}"
# The response will contain job information, including the job ID.
# You would then need to make subsequent calls to jobs.getQueryResults
# using the job ID to retrieve the actual data rows once the job completes.
This example only initiates the query job. Retrieving results requires polling the job status and then calling the jobs.getQueryResults
endpoint. This highlights the extra steps involved compared to client libraries.
Best Practices and Tips
- Prefer Client Libraries: Use Google Cloud client libraries whenever possible for simplicity, robustness, and easier maintenance.
- Use ADC: Rely on Application Default Credentials for authentication simplifies deployment across different environments. Avoid embedding credentials in code.
- Parameterized Queries: Always use parameterized queries when incorporating external input to prevent SQL injection vulnerabilities.
- Optimize Queries: Write efficient SQL. Avoid
SELECT *
, useWHERE
clauses effectively, understand partitioning and clustering for performance on large tables. Use the query plan explanation feature. - Error Handling: Implement robust error handling, especially for network issues or API-specific errors (e.g., quota exceeded, not found). Client libraries often include built-in retry mechanisms for transient errors.
- Resource Management: Explicitly delete datasets or tables created during testing or temporary processing to avoid unnecessary storage costs.
- Monitor Costs and Quotas: Be mindful of BigQuery pricing (bytes processed for queries, storage costs). Monitor your usage and be aware of API quotas (e.g., concurrent query limits, API request rate limits). Use features like maximum bytes billed (
maximum_bytes_billed
inJobConfig
) to control query costs. - Use Specialized APIs: Leverage the Storage Read API for large data exports/reads and the Connection API for querying external sources efficiently.
Conclusion
The BigQuery APIs provide a powerful and flexible way to interact with your data warehouse programmatically. Whether you need to automate data loading, run complex analytical queries as part of an application, integrate BigQuery insights into dashboards, or manage resources dynamically, the APIs offer the necessary tools.
By understanding the different API types, setting up your environment correctly, and leveraging the convenience of client libraries (especially in Python), you can unlock the full potential of BigQuery beyond the interactive console. Start with the core API for common tasks, explore specialized APIs like the Storage Read API for performance-critical operations, and remember the REST API is always available for ultimate control. As you build applications on BigQuery, these APIs will be essential components of your data architecture.