Heygen AI is a powerful platform that enables users to create realistic AI-generated avatar videos programmatically. This comprehensive tutorial will guide you through the process of integrating and leveraging Heygen's API for a variety of use cases, from simple avatar videos to complex template-based content creation. Each section provides detailed step-by-step instructions to help you master Heygen's capabilities.
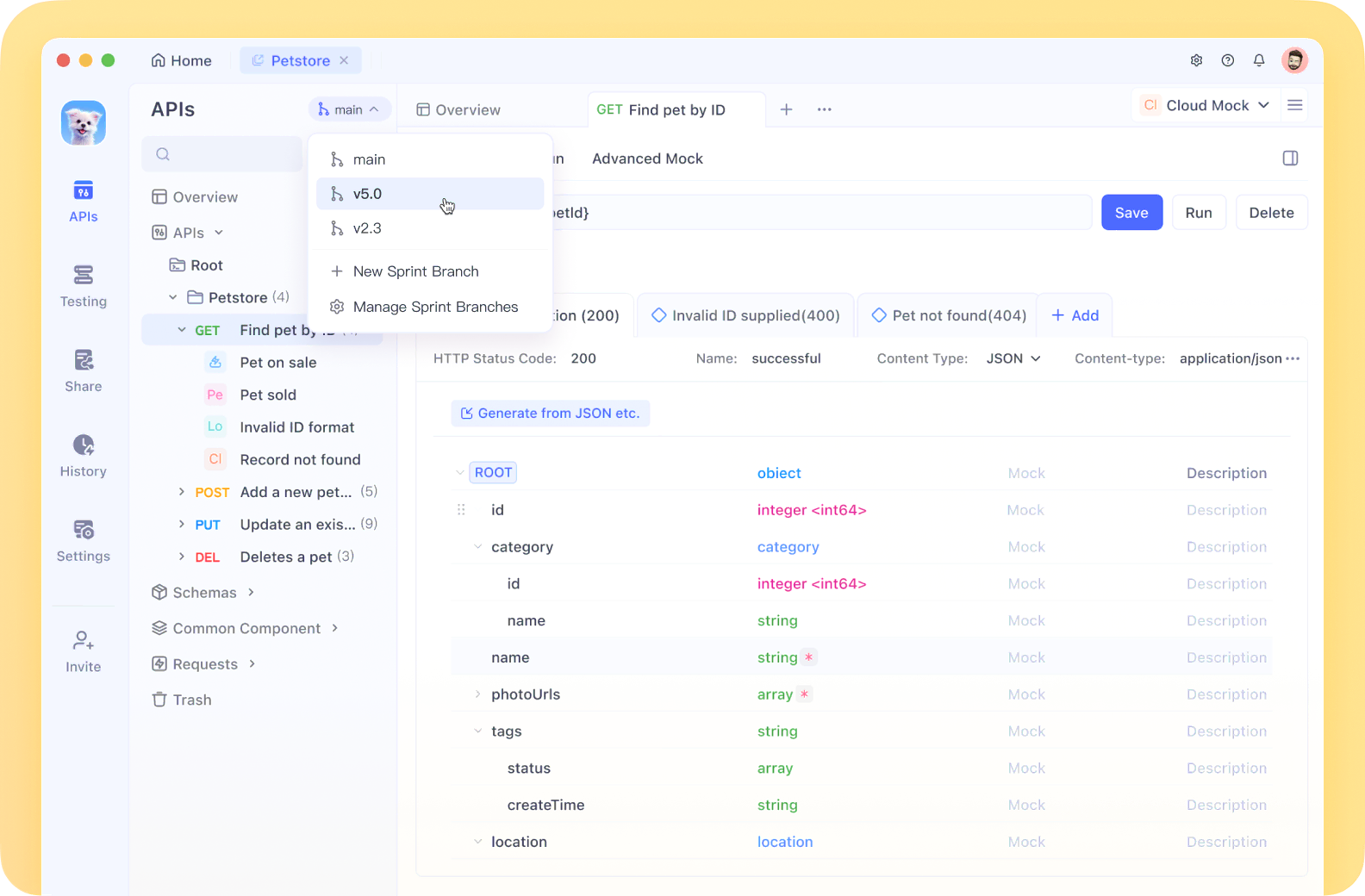
With its intuitive interface, collaborative features, and powerful automation capabilities, Apidog significantly reduces development time while improving API quality.
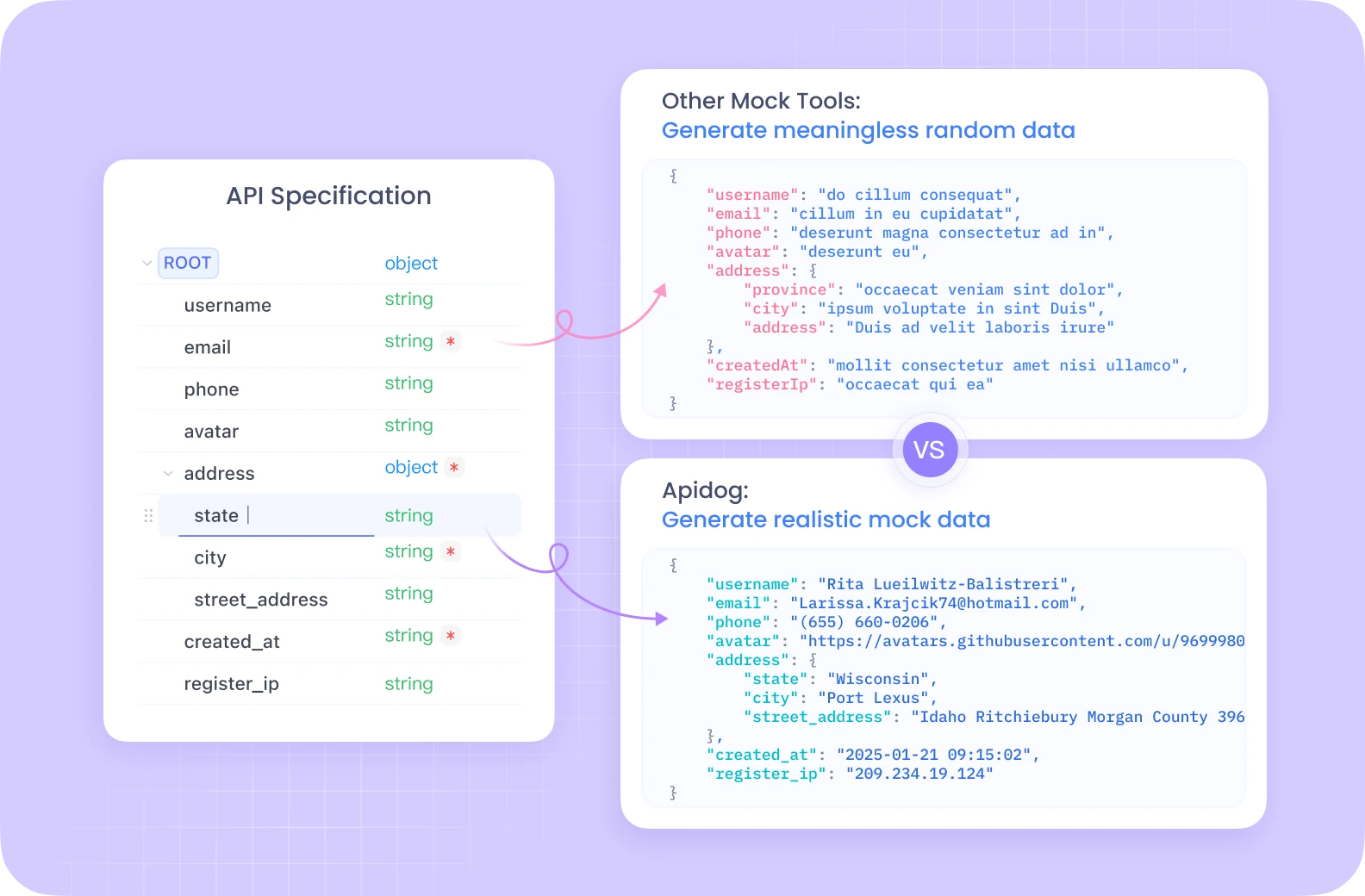
Whether you're building a complex microservice architecture or integrating with third-party APIs like Heygen, Apidog's mock servers, environment variables, and extensive testing options make it the preferred choice for developers who demand efficiency and reliability. Try Apidog today and experience the next evolution in API development tools.
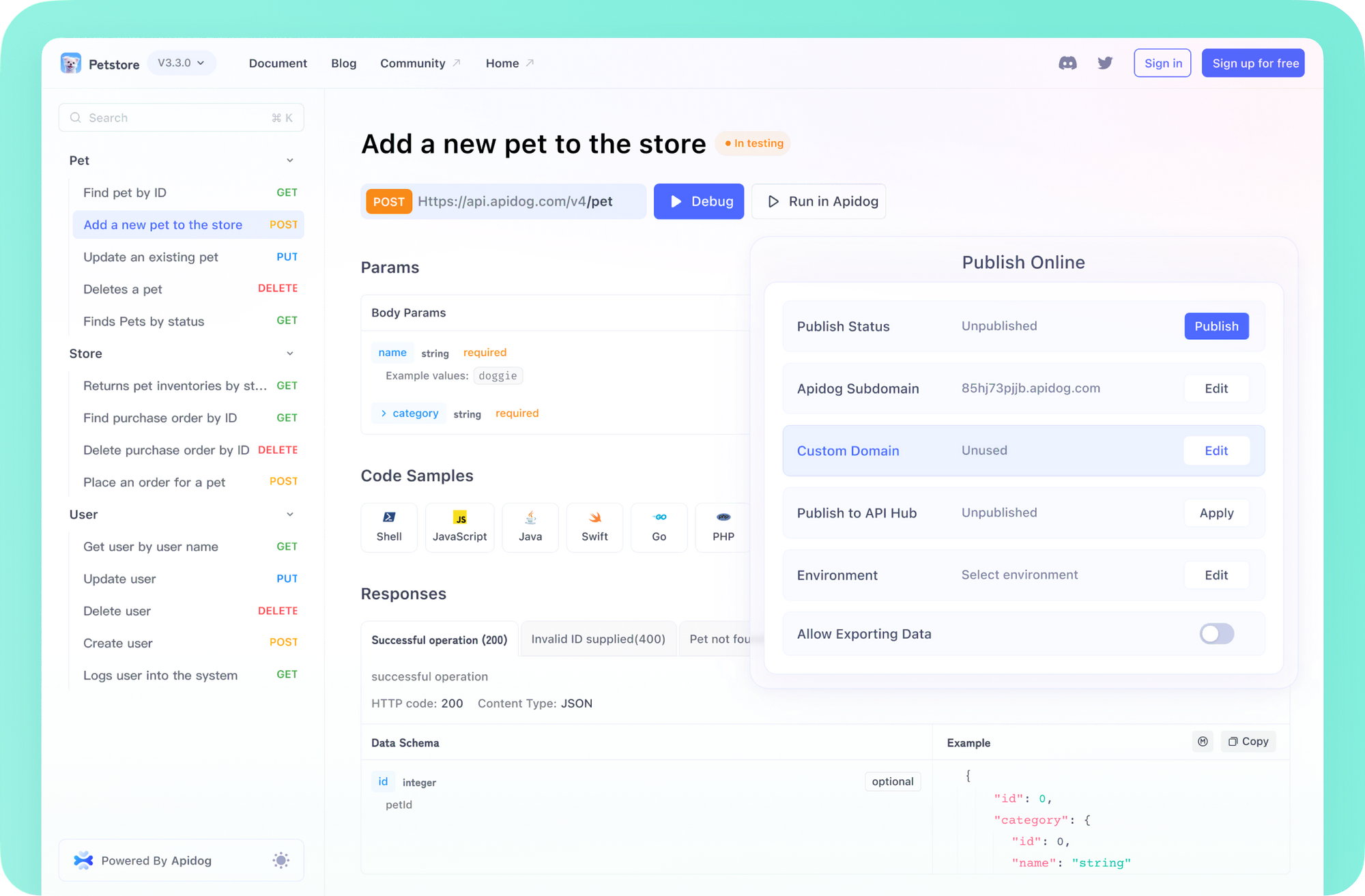
Introduction to Heygen API
Heygen API allows developers to create AI-powered avatar videos programmatically and integrate Heygen's capabilities into their applications. The API offers various features, including video generation, translation, and template-based content creation. By following this tutorial, you'll learn how to harness these capabilities to create engaging content for marketing, education, customer service, and more.
The API's core functionality revolves around generating videos with realistic AI avatars that can speak any text you provide or sync with audio files. Additionally, you can customize backgrounds, translate videos into multiple languages, and work with templates for more complex productions.
Beyond just creating basic videos, Heygen's API enables you to build sophisticated applications that can automatically generate personalized content for your users, create interactive experiences, or even translate existing videos into multiple languages. This makes it invaluable for global businesses, content creators, educators, and developers looking to incorporate AI-generated video into their products.
Getting Started with Heygen API
Creating an Account for Heygen API
Follow these steps to create your Heygen account:
- Visit Heygen's official website at heygen website.
- Click the "Sign Up" or "Get Started" button in the top-right corner
- Choose your preferred sign-up method (email, Google, etc.)
- Complete the registration form with your details
- Accept the terms and privacy policy
- Verify your email address if required
- Log in to your new account
After registration, you'll have access to both Heygen's web interface and API capabilities. New users typically receive a free trial with limited features. The free trial includes watermarked videos and limited usage quotas, but it's sufficient to test the API functionality before committing to a paid plan.
During the registration process, Heygen might ask about your intended use case. Providing this information can help Heygen tailor recommendations for your specific needs, though it's not strictly necessary for API access.
Obtaining Your API Key
To access the API, follow these detailed steps to get your API key:
- Log in to your Heygen account
- Click on your profile picture or avatar in the top-right corner
- Select "Settings" from the dropdown menu
- Navigate to the "Subscriptions" tab
- Scroll down to the "HeyGen API" section
- Click "Show" next to "API Token"
- Authenticate if prompted
- Copy your API token to use in your requests
Keep this token secure, as it provides full access to the API under your account. Never expose it in client-side code or public repositories. If you suspect your API key has been compromised, you can generate a new one from the same settings page.
Your API key will be used in all your requests to the Heygen API. Typically, you'll include it in the X-Api-Key
header of your HTTP requests. For example:
X-Api-Key: your-api-key-here
Different subscription tiers offer different API usage limits. The Free Trial provides basic access with watermarked videos, while paid plans (Pro, Scale, and Enterprise) offer increasing usage limits, features, and quality options. Check Heygen's pricing page for the most current information on plan features and limitations.
Basic Avatar Video Creation with Heygen API
Listing Available Avatars with Heygen API
To begin creating videos, you first need to know which avatars are available. Follow these steps:
- Open your terminal or API client
- Make the following request:
curl --request GET \\\\
--url <https://api.heygen.com/v2/avatars> \\\\
--header 'Accept: application/json' \\\\
--header 'X-Api-Key: <your-api-key>'
- Review the response, which will look like:
{
"error": null,
"data": {
"avatars": [
{
"avatar_id": "Angela-inblackskirt-20220820",
"avatar_name": "Angela in Black Dress",
"gender": "female",
"preview_image_url": "<preview_image_url>",
"preview_video_url": "<preview_video_url>"
},
// More avatars...
]
}
}
- Choose an avatar and note its
avatar_id
for later use
Each avatar has different appearances and styles. Some avatars are designed for specific contexts like business presentations, casual conversations, or educational content. Preview the avatars using the provided URLs to find one that matches your intended use case.
If you're using Python, you can use this code snippet instead:
import requests
headers = {
'Accept': 'application/json',
'X-Api-Key': 'your-api-key'
}
response = requests.get('<https://api.heygen.com/v2/avatars>', headers=headers)
avatars = response.json()['data']['avatars']
# Print all available avatars
for avatar in avatars:
print(f"ID: {avatar['avatar_id']}, Name: {avatar['avatar_name']}")
Avatars have different styles available, such as "normal" or "casual." The default style is "normal," but you can specify others when creating videos if the avatar supports multiple styles.
Listing Available Voices with Heygen API
Next, you need to select a voice for your avatar. Follow these steps:
- Make the following API request:
curl --request GET \\\\
--url <https://api.heygen.com/v2/voices> \\\\
--header 'Accept: application/json' \\\\
--header 'X-Api-Key: <your-api-key>'
- Review the response to find available voices:
{
"error": null,
"data": {
"voices": [
{
"voice_id": "26b2064088674c80b1e5fc5ab1a068ec",
"language": "English",
"gender": "male",
"name": "Rex",
"preview_audio": "<https://resource.heygen.ai/text_to_speech/bCo7SFtTvcJz6udLqHmUKf.mp3>",
"support_pause": false,
"emotion_support": true,
"support_interactive_avatar": true
},
// More voices...
]
}
}
- Choose a voice and note its
voice_id
for later use
Voices come in various languages, accents, and genders. Listen to the preview audio to find a voice that matches your project's tone and style. Some voices support additional features like emotional inflections or pauses, which can be useful for more dynamic presentations.
Pay attention to the support_interactive_avatar
property if you plan to use interactive avatars later, as not all voices support this feature.
Python equivalent:
response = requests.get('<https://api.heygen.com/v2/voices>', headers=headers)
voices = response.json()['data']['voices']
# Print all available voices
for voice in voices:
print(f"ID: {voice['voice_id']}, Name: {voice['name']}, Language: {voice['language']}")
# Filter for English voices only
english_voices = [v for v in voices if v['language'] == 'English']
print(f"Found {len(english_voices)} English voices")
Generating a Simple Avatar Video with Heygen API
Now that you have selected an avatar and voice, follow these steps to create a video:
- Prepare your request payload with the chosen avatar and voice:
{
"video_inputs": [
{
"character": {
"type": "avatar",
"avatar_id": "Angela-inTshirt-20220820",
"avatar_style": "normal"
},
"voice": {
"type": "text",
"input_text": "Welcome to the HeyGen API! This is a demonstration of how easy it is to create engaging avatar videos programmatically.",
"voice_id": "1bd001e7e50f421d891986aad5158bc8",
"speed": 1.1
},
"background": {
"type": "color",
"value": "#87CEEB"
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}
- Send the request to generate the video:
curl -X POST <https://api.heygen.com/v2/video/generate> \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-H 'Content-Type: application/json' \\\\
-d '<your-prepared-json>'
- Save the
video_id
from the response:
{
"error": null,
"data": {
"video_id": "abcdef123456"
}
}
Let's break down the request parameters:
video_inputs
: An array of scene configurationscharacter
: Specifies the avatar to usetype
: "avatar" for standard Heygen avatarsavatar_id
: The ID of your chosen avataravatar_style
: The visual style of the avatar (e.g., "normal", "casual")voice
: Configuration for speechtype
: "text" for text-to-speech conversioninput_text
: The script the avatar will speakvoice_id
: The ID of your chosen voicespeed
: Speaking speed (1.0 is normal, higher is faster)background
: Background configurationtype
: "color" for solid color backgroundsvalue
: Hex color codedimension
: Output video dimensionswidth
: Video width in pixelsheight
: Video height in pixels
You can adjust these parameters to customize your video. For example, changing the speed
value affects how quickly the avatar speaks, while dimension
controls the video resolution.
Python implementation:
import json
import requests
payload = {
"video_inputs": [
{
"character": {
"type": "avatar",
"avatar_id": "Angela-inTshirt-20220820",
"avatar_style": "normal"
},
"voice": {
"type": "text",
"input_text": "Welcome to the HeyGen API! This is a demonstration of how easy it is to create engaging avatar videos programmatically.",
"voice_id": "1bd001e7e50f421d891986aad5158bc8",
"speed": 1.1
},
"background": {
"type": "color",
"value": "#87CEEB"
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}
response = requests.post(
'<https://api.heygen.com/v2/video/generate>',
headers={
'X-Api-Key': 'your-api-key',
'Content-Type': 'application/json'
},
data=json.dumps(payload)
)
video_id = response.json()['data']['video_id']
print(f"Video generation initiated! Video ID: {video_id}")
Checking Video Generation Status with Heygen API
After initiating video generation, follow these steps to check its status:
- Use the
video_id
from the previous step to make a status check request:
curl --request GET \\\\
--url <https://api.heygen.com/v1/video_status.get?video_id=><video_id> \\\\
--header 'Accept: application/json' \\\\
--header 'X-Api-Key: <your-api-key>'
- Monitor the status in the response:
{
"code": 100,
"data": {
"id": "<id>",
"status": "processing",
"thumbnail_url": null,
"video_url": null,
"caption_url": null,
"gif_url": null,
"error": null,
"duration": null,
"callback_id": null,
"video_url_caption": null
},
"message": "Success"
}
- Repeat the check until the status changes to "completed" or "failed"
Video generation typically takes between 30 seconds and several minutes, depending on:
- Length of the input text
- Server load
- Video complexity
- Your subscription tier
Status values you might encounter:
pending
: Your request has been received but processing hasn't started yetprocessing
: Your video is currently being generatedcompleted
: Your video is ready for downloadfailed
: There was an error generating your video
When a video fails, the response will include an error message explaining the issue:
{
"code": 100,
"data": {
"id": "<id>",
"status": "failed",
"error": {
"code": 40119,
"message": "Video is too long",
"detail": "Video is too long (> 3600.0s). Please upgrade your plan to generate longer videos"
},
"thumbnail_url": null,
"video_url": null,
"caption_url": null,
"gif_url": null,
"duration": null,
"callback_id": null,
"video_url_caption": null
},
"message": "Success"
}
Python implementation with polling:
import time
import requests
def check_video_status(video_id, api_key):
headers = {
'Accept': 'application/json',
'X-Api-Key': api_key
}
max_attempts = 30 # Avoid infinite loops
attempt = 0
while attempt < max_attempts:
response = requests.get(
f'<https://api.heygen.com/v1/video_status.get?video_id={video_id}>',
headers=headers
)
data = response.json()['data']
status = data['status']
if status == 'completed':
print("Video generation complete!")
return {
'video_url': data['video_url'],
'thumbnail_url': data['thumbnail_url'],
'duration': data['duration']
}
elif status == 'failed':
error = data['error']
print(f"Video generation failed: {error}")
return None
else:
print(f"Video status: {status}. Checking again in 5 seconds...")
attempt += 1
time.sleep(5)
print("Maximum attempts reached. Video still not ready.")
return None
# Usage
result = check_video_status("abcdef123456", "your-api-key")
if result:
print(f"Video URL: {result['video_url']}")
print(f"Duration: {result['duration']} seconds")
Downloading Your Generated Video with Heygen API
Once the video status is "completed", follow these steps to download your video:
- Extract the
video_url
from the status response - Download the video using the URL:
curl <video_url> --output my_avatar_video.mp4
- Verify the downloaded file works correctly
Python implementation:
import requests
def download_video(video_url, output_filename="my_avatar_video.mp4"):
response = requests.get(video_url)
if response.status_code == 200:
with open(output_filename, 'wb') as file:
file.write(response.content)
print(f"Video downloaded successfully to {output_filename}")
print(f"File size: {len(response.content) / (1024 * 1024):.2f} MB")
return True
else:
print(f"Failed to download video. Status code: {response.status_code}")
return False
# Usage
success = download_video("<https://storage.googleapis.com/heygen-videos/>...")
Important Note: The video URL expires after 7 days. Make sure to download your video before it expires or implement a system to regenerate or re-retrieve the video URL as needed.
For production systems, consider:
- Setting up a scheduled job to download videos promptly after generation
- Storing videos in your own cloud storage for long-term access
- Implementing a database to track video IDs, generation dates, and expiration dates
Heygen API: Working with Templates
Templates offer more flexibility than simple avatar videos, allowing you to customize text, images, videos, audios, and avatars within a pre-designed layout.
Heygen API Templates vs. Simple Avatar Videos
Here's a comparison of the key differences:
Avatar Videos:
- Focus solely on an avatar speaking
- Limited to a single scene with one avatar
- Simple background options
- Best for straightforward talking-head videos
- Created entirely via API calls
Template Videos:
- Multiple scenes with various elements
- Customizable placeholders for text, images, videos, avatars, and audio
- Complex transitions and animations
- Support for multiple avatars in a single video
- Templates are created through the Heygen web interface
- API is used to generate videos based on templates
Templates are particularly useful for creating consistent series of videos or generating personalized videos at scale. For example, you could create a template for product demonstrations and then generate custom versions for different products by changing just the relevant text, images, and product details.
Heygen API Creating Templates with Placeholders
Templates are created through the Heygen web interface:
- Log in to your Heygen account
- Navigate to the Templates section
- Click "Create Template"
- Use the editor to design your template with these components:
- Text elements: Add texts that can be customized later
- Avatar scenes: Add avatars that will speak customizable content
- Image placeholders: Add spaces for custom images
- Video placeholders: Add spaces for custom video clips
- Audio elements: Add background music or sound effects
- For each element you want to make customizable, define a placeholder using double curly brackets:
- Text example:
{{product_name}}
- Avatar script example:
{{product_description}}
- Image placeholder: Select an image and mark it as a variable
- Arrange the elements as desired and preview the template
- Save your template with a descriptive name
For example, if creating a product announcement template, you might include:
- An opening text with
{{company_name}}
and{{product_name}}
- An avatar that says "We're excited to introduce {{product_name}}, which offers {{key_benefit}}"
- A placeholder image for
{{product_image}}
- Background music that can be replaced
Placeholders can be of different types:
- Text: For replacing written text or avatar scripts
- Image: For replacing image elements
- Video: For replacing video clips
- Avatar: For changing which avatar appears
- Voice: For changing the voice used by avatars
- Audio: For replacing background music or sound effects
Listing Your Templates with Heygen API
To retrieve all templates you've created:
curl --location '<https://api.heygen.com/v2/templates>' \\\\
--header 'accept: application/json' \\\\
--header 'x-api-key: <your-api-key>'
This returns information about all your templates, including their IDs and thumbnail URLs:
{
"error": null,
"data": {
"templates": [
{
"template_id": "abcdef123456",
"title": "Product Announcement",
"thumbnail_url": "<https://storage.googleapis.com/heygen-templates/thumbnails/>...",
"created_at": "2023-09-15T14:25:30Z"
},
{
"template_id": "ghijkl789012",
"title": "Weekly Update",
"thumbnail_url": "<https://storage.googleapis.com/heygen-templates/thumbnails/>...",
"created_at": "2023-09-18T09:12:45Z"
}
]
}
}
Python implementation:
import requests
headers = {
'accept': 'application/json',
'x-api-key': 'your-api-key'
}
response = requests.get('<https://api.heygen.com/v2/templates>', headers=headers)
templates = response.json()['data']['templates']
print(f"Found {len(templates)} templates:")
for template in templates:
print(f"ID: {template['template_id']}, Title: {template['title']}, Created: {template['created_at']}")
Retrieving Template Elements with Heygen API
To see the variables available in a specific template:
curl --location '<https://api.heygen.com/v2/template/><template_id>' \\\\
--header 'accept: application/json' \\\\
--header 'x-api-key: <your-api-key>'
This shows all customizable elements in your template:
{
"error": null,
"data": {
"template_id": "abcdef123456",
"title": "Product Announcement",
"variables": {
"company_name": {
"name": "company_name",
"type": "text",
"properties": {
"default_content": "Company Name"
}
},
"product_name": {
"name": "product_name",
"type": "text",
"properties": {
"default_content": "Product Name"
}
},
"key_benefit": {
"name": "key_benefit",
"type": "text",
"properties": {
"default_content": "amazing benefits to users"
}
},
"product_image": {
"name": "product_image",
"type": "image",
"properties": {
"default_url": "<https://example.com/default-image.jpg>"
}
}
}
}
}
Understanding the structure of your template's variables is crucial for generating videos correctly. Note the type of each variable (text, image, video, etc.) and any default values provided.
Python implementation:
def get_template_details(template_id, api_key):
headers = {
'accept': 'application/json',
'x-api-key': api_key
}
response = requests.get(
f'<https://api.heygen.com/v2/template/{template_id}>',
headers=headers
)
if response.status_code == 200:
template = response.json()['data']
variables = template['variables']
print(f"Template: {template['title']} (ID: {template['template_id']})")
print("\\\\nVariables:")
for var_name, var_details in variables.items():
var_type = var_details['type']
print(f"- {var_name} ({var_type})")
if var_type == 'text' and 'default_content' in var_details['properties']:
print(f" Default: \\\\"{var_details['properties']['default_content']}\\\\"")
elif var_type == 'image' and 'default_url' in var_details['properties']:
print(f" Default image: {var_details['properties']['default_url']}")
return variables
else:
print(f"Failed to get template details. Status code: {response.status_code}")
return None
# Usage
template_variables = get_template_details("abcdef123456", "your-api-key")
Generating Videos from Templates with Heygen API
To create a video using your template:
curl --location '<https://api.heygen.com/v2/template/><template_id>/generate' \\\\
--header 'X-Api-Key: <your-api-key>' \\\\
--header 'Content-Type: application/json' \\\\
--data '{
"caption": false,
"title": "Personalized Welcome",
"variables": {
"company_name": {
"name": "company_name",
"type": "text",
"properties": {
"content": "Acme Corporation"
}
},
"product_name": {
"name": "product_name",
"type": "text",
"properties": {
"content": "Super Widget Pro"
}
},
"key_benefit": {
"name": "key_benefit",
"type": "text",
"properties": {
"content": "30% faster productivity and seamless integration"
}
},
"product_image": {
"name": "product_image",
"type": "image",
"properties": {
"url": "<https://example.com/product-image.jpg>"
}
}
}
}'
This returns a video_id
that you can use to check status and download the video as in Steps 2.4 and 2.5.
{
"error": null,
"data": {
"video_id": "mnopqr345678"
}
}
Handling Template Variables with Heygen API
Different variable types require different property structures:
Text Variables:
"variable_name": {
"name": "variable_name",
"type": "text",
"properties": {
"content": "Your custom text here"
}
}
Image Variables:
"variable_name": {
"name": "variable_name",
"type": "image",
"properties": {
"url": "<https://example.com/your-image.jpg>"
}
}
Video Variables:
"variable_name": {
"name": "variable_name",
"type": "video",
"properties": {
"url": "<https://example.com/your-video.mp4>"
}
}
Avatar Variables:
"variable_name": {
"name": "variable_name",
"type": "avatar",
"properties": {
"avatar_id": "Angela-inTshirt-20220820"
}
}
Voice Variables:
"variable_name": {
"name": "variable_name",
"type": "voice",
"properties": {
"voice_id": "1bd001e7e50f421d891986aad5158bc8"
}
}
Audio Variables:
"variable_name": {
"name": "variable_name",
"type": "audio",
"properties": {
"url": "<https://example.com/your-audio.mp3>"
}
}
Python implementation for generating a template video:
import json
import requests
def generate_template_video(template_id, variables, api_key, title="Generated from Template"):
headers = {
'X-Api-Key': api_key,
'Content-Type': 'application/json'
}
# Format variables according to their types
formatted_variables = {}
for var_name, var_value in variables.items():
if isinstance(var_value, dict) and 'type' in var_value:
# Variable is already formatted
formatted_variables[var_name] = var_value
else:
# Assume text variable if not specified
formatted_variables[var_name] = {
"name": var_name,
"type": "text",
"properties": {
"content": var_value
}
}
payload = {
"caption": False,
"title": title,
"variables": formatted_variables
}
response = requests.post(
f'<https://api.heygen.com/v2/template/{template_id}/generate>',
headers=headers,
data=json.dumps(payload)
)
if response.status_code == 200:
video_id = response.json()['data']['video_id']
print(f"Template video generation initiated! Video ID: {video_id}")
return video_id
else:
print(f"Failed to generate template video. Status code: {response.status_code}")
print(f"Response: {response.text}")
return None
# Usage example
variables = {
"company_name": "Acme Corporation",
"product_name": "Super Widget Pro",
"key_benefit": "30% faster productivity and seamless integration",
"product_image": {
"name": "product_image",
"type": "image",
"properties": {
"url": "<https://example.com/product-image.jpg>"
}
}
}
video_id = generate_template_video("abcdef123456", variables, "your-api-key", "Product Launch Video")
Advanced Features of Heygen API
Customizing Video Backgrounds with Heygen API
You can use your own images or videos as backgrounds:
curl -X POST <https://api.heygen.com/v2/video/generate> \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-H 'Content-Type: application/json' \\\\
-d '{
"video_inputs": [
{
"character": {
"type": "avatar",
"avatar_id": "Daisy-inskirt-20220818",
"avatar_style": "normal"
},
"voice": {
"type": "text",
"input_text": "This video has a custom background image.",
"voice_id": "2d5b0e6cf36f460aa7fc47e3eee4ba54"
},
"background": {
"type": "image",
"url": "<https://example.com/your-background-image.jpg>"
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}'
Background types include:
Color Background: Use a simple solid color
"background": {
"type": "color",
"value": "#87CEEB"
}
Image Background: Use a static image
"background": {
"type": "image",
"url": "<https://example.com/your-background-image.jpg>"
}
Video Background: Use a video clip
"background": {
"type": "video",
"url": "<https://example.com/your-background-video.mp4>"
}
Transparent Background: Create a video with transparency
"background": {
"type": "transparent"
}
For image and video backgrounds, ensure your URLs are publicly accessible. Heygen needs to be able to download these files during video generation.
Using Audio Source as Voice with Heygen API
Instead of generating speech from text, you can use an existing audio file:
curl -X POST <https://api.heygen.com/v2/video/generate> \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-H 'Content-Type: application/json' \\\\
-d '{
"video_inputs": [
{
"character": {
"type": "avatar",
"avatar_id": "Daisy-inskirt-20220818",
"avatar_style": "normal"
},
"voice": {
"type": "audio",
"input_audio": "<https://example.com/your-audio-file.mp3>"
},
"background": {
"type": "color",
"value": "#008000"
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}'
This approach is useful when:
- You have pre-recorded narration
- You want to use a voice not available in Heygen
- You need precise control over the delivery, emphasis, and timing
- You're working with music or sound effects that should sync with the avatar's lip movements
The audio file should be in a common format like MP3, WAV, or M4A. The avatar will lip-sync to match the audio file. For best results:
- Use clear audio with minimal background noise
- Ensure the audio is of good quality (at least 128 kbps for MP3)
- Make sure the speech is at a natural pace
- Avoid background music in the speech audio itself
Python implementation:
import json
import requests
def create_avatar_video_with_audio(avatar_id, audio_url, api_key, background_color="#FFFFFF"):
headers = {
'X-Api-Key': api_key,
'Content-Type': 'application/json'
}
payload = {
"video_inputs": [
{
"character": {
"type": "avatar",
"avatar_id": avatar_id,
"avatar_style": "normal"
},
"voice": {
"type": "audio",
"input_audio": audio_url
},
"background": {
"type": "color",
"value": background_color
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}
response = requests.post(
'<https://api.heygen.com/v2/video/generate>',
headers=headers,
data=json.dumps(payload)
)
if response.status_code == 200:
video_id = response.json()['data']['video_id']
print(f"Video generation initiated with custom audio! Video ID: {video_id}")
return video_id
else:
print(f"Failed to generate video. Status code: {response.status_code}")
print(f"Response: {response.text}")
return None
# Usage example
video_id = create_avatar_video_with_audio(
"Daisy-inskirt-20220818",
"<https://example.com/narration.mp3>",
"your-api-key"
)
Creating Transparent Avatar Videos with Heygen API
Transparent avatar videos are useful for overlaying on other content, like websites, presentations, or other videos. Follow these steps to create a video with a transparent background:
curl -X POST <https://api.heygen.com/v2/video/generate> \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-H 'Content-Type: application/json' \\\\
-d '{
"video_inputs": [
{
"character": {
"type": "avatar",
"avatar_id": "Daisy-inskirt-20220818",
"avatar_style": "normal"
},
"voice": {
"type": "text",
"input_text": "This video has a transparent background, making it easy to overlay on other content.",
"voice_id": "2d5b0e6cf36f460aa7fc47e3eee4ba54"
},
"background": {
"type": "transparent"
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}'
Important notes about transparent videos:
- The output format will be WebM instead of MP4 to preserve transparency
- Not all media players support WebM with transparency
- File sizes may be larger than equivalent MP4 videos
- When embedding on websites, ensure your video player supports WebM with alpha channel
- For use in editing software, ensure your software supports importing WebM with transparency
Python implementation:
def create_transparent_avatar_video(avatar_id, text, voice_id, api_key):
headers = {
'X-Api-Key': api_key,
'Content-Type': 'application/json'
}
payload = {
"video_inputs": [
{
"character": {
"type": "avatar",
"avatar_id": avatar_id,
"avatar_style": "normal"
},
"voice": {
"type": "text",
"input_text": text,
"voice_id": voice_id
},
"background": {
"type": "transparent"
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}
response = requests.post(
'<https://api.heygen.com/v2/video/generate>',
headers=headers,
data=json.dumps(payload)
)
if response.status_code == 200:
return response.json()['data']['video_id']
else:
print(f"Error creating transparent video: {response.text}")
return None
Heygen API Working with Photo Avatars API
Photo Avatars allow you to create avatar videos using a still image of a person, bringing photos to life. This feature is especially useful when you want to create personalized videos with real people who aren't available to record video.
Step 1: Generate AI Avatar Photos for Heygen API
First, you need to generate avatar photos:
curl -X POST <https://api.heygen.com/v1/photo_avatar.generate> \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-H 'Content-Type: application/json' \\\\
-d '{
"name": "John Doe Avatar",
"gender": "male",
"image_url": "<https://example.com/john-photo.jpg>"
}'
Response:
{
"code": 100,
"data": {
"photo_avatar_id": "phav_abcdef123456",
"name": "John Doe Avatar",
"status": "pending"
},
"message": "Success"
}
Step 2: Check Photo Avatar Status for Heygen API
Poll the status until the avatar is ready:
curl -X GET <https://api.heygen.com/v1/photo_avatar.get?photo_avatar_id=phav_abcdef123456> \\\\
-H 'X-Api-Key: <your-api-key>'
Response when completed:
{
"code": 100,
"data": {
"photo_avatar_id": "phav_abcdef123456",
"name": "John Doe Avatar",
"status": "completed",
"preview_url": "<https://storage.googleapis.com/heygen-photo-avatars/preview/>..."
},
"message": "Success"
}
Step 3: Create Video with Photo Avatar for Heygen API
Once your photo avatar is ready, create a video:
curl -X POST <https://api.heygen.com/v2/video/generate> \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-H 'Content-Type: application/json' \\\\
-d '{
"video_inputs": [
{
"character": {
"type": "photo_avatar",
"photo_avatar_id": "phav_abcdef123456"
},
"voice": {
"type": "text",
"input_text": "This is a video created with a photo avatar. It brings still images to life!",
"voice_id": "2d5b0e6cf36f460aa7fc47e3eee4ba54"
},
"background": {
"type": "color",
"value": "#F5F5F5"
}
}
],
"dimension": {
"width": 1280,
"height": 720
}
}'
Best practices for photo avatars:
- Use high-quality front-facing photos with good lighting
- Ensure the subject's face is clearly visible
- Avoid photos with multiple faces
- The photo should show the subject from shoulders up
- Neutral expressions work best for versatile animations
Video Translation API Implementation for Heygen API
Heygen's Video Translation API allows you to translate existing videos into different languages, with the avatar speaking in the target language while maintaining lip synchronization.
Step 1: Submit a Translation Request for Heygen API
curl -X POST <https://api.heygen.com/v1/video_translate> \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-H 'Content-Type: application/json' \\\\
-d '{
"video_url": "<https://example.com/original-video.mp4>",
"target_language": "es",
"voice_id": "spanish_voice_id"
}'
Response:
{
"code": 100,
"data": {
"video_translate_id": "vt_abcdef123456"
},
"message": "Success"
}
Available target languages include:
- "en" (English)
- "es" (Spanish)
- "fr" (French)
- "de" (German)
- "it" (Italian)
- "pt" (Portuguese)
- "pl" (Polish)
- "zh" (Chinese)
- "ja" (Japanese)
- and many more
Step 2: Check Translation Status for Heygen API
Similar to checking video generation status:
curl -X GET <https://api.heygen.com/v1/video_translate_status.get?video_translate_id=vt_abcdef123456> \\\\
-H 'X-Api-Key: <your-api-key>'
Response when completed:
{
"code": 100,
"data": {
"id": "vt_abcdef123456",
"status": "completed",
"video_url": "<https://storage.googleapis.com/heygen-videos/translated/>...",
"error": null
},
"message": "Success"
}
Python implementation:
def translate_video(video_url, target_language, voice_id, api_key):
headers = {
'X-Api-Key': api_key,
'Content-Type': 'application/json'
}
payload = {
"video_url": video_url,
"target_language": target_language,
"voice_id": voice_id
}
response = requests.post(
'<https://api.heygen.com/v1/video_translate>',
headers=headers,
data=json.dumps(payload)
)
if response.status_code == 200:
translation_id = response.json()['data']['video_translate_id']
print(f"Translation job submitted. ID: {translation_id}")
return translation_id
else:
print(f"Failed to submit translation job: {response.text}")
return None
def check_translation_status(translation_id, api_key):
headers = {'X-Api-Key': api_key}
response = requests.get(
f'<https://api.heygen.com/v1/video_translate_status.get?video_translate_id={translation_id}>',
headers=headers
)
if response.status_code == 200:
return response.json()['data']
else:
print(f"Failed to check translation status: {response.text}")
return None
Implementing Webhooks for Heygen API
Webhooks allow Heygen to notify your application when events occur, such as video generation completion.
Available Webhook Events for Heygen API
Check the webhook events you can subscribe to:
curl --request GET \\\\
--url <https://api.heygen.com/v1/webhook/webhook.list> \\\\
--header 'accept: application/json' \\\\
--header 'x-api-key: <your-api-key>'
Response:
{
"code": 100,
"data": [
"avatar_video.success",
"avatar_video.fail",
"avatar_video_gif.success",
"avatar_video_gif.fail",
"video_translate.success",
"video_translate.fail",
"personalized_video"
],
"msg": null,
"message": null
}
Key webhook events include:
avatar_video.success
: When a video generation succeedsavatar_video.fail
: When a video generation failsvideo_translate.success
: When a video translation succeedsvideo_translate.fail
: When a video translation failspersonalized_video
: When a personalized video event occurs
Registering Webhook Endpoints for Heygen API
Register an endpoint to receive webhook notifications:
curl --location '<https://api.heygen.com/v1/webhook/endpoint.add>' \\\\
--header 'Content-Type: application/json' \\\\
--header 'X-Api-Key: <your-api-key>' \\\\
--data '{
"url": "<https://your-endpoint.com/webhook>",
"events": ["avatar_video.success", "avatar_video.fail"]
}'
Response:
{
"code": 100,
"data": {
"endpoint_id": "<endpoint_id>",
"username": "<username>",
"url": "<https://your-endpoint.com/webhook>",
"status": "enabled",
"events": ["avatar_video.success", "avatar_video.fail"],
"secret": "<secret>",
"created_at": "2023-06-28T13:34:51.293528",
"space_id": "<space_id>"
},
"msg": null,
"message": null
}
Save the endpoint_id
and secret
from the response, as you'll need them later.
Building a Simple Webhook Handler for Heygen API
Here's a full example of a webhook handler using Python and Flask:
from flask import Flask, request, jsonify
import hmac
import hashlib
import requests
import time
import os
app = Flask(__name__)
# Store this securely in environment variables or secure config
WEBHOOK_SECRET = os.environ.get('HEYGEN_WEBHOOK_SECRET')
API_KEY = os.environ.get('HEYGEN_API_KEY')
def verify_signature(payload, signature):
"""Verify the webhook signature"""
computed_signature = hmac.new(
WEBHOOK_SECRET.encode(),
payload.encode(),
hashlib.sha256
).hexdigest()
return hmac.compare_digest(computed_signature, signature)
@app.route('/webhook', methods=['POST', 'OPTIONS'])
def webhook():
if request.method == 'OPTIONS':
# Respond quickly to validation requests
return '', 200
# Get the signature from headers if your webhook implementation uses them
# signature = request.headers.get('X-Heygen-Signature')
# if not verify_signature(request.data.decode(), signature):
# return jsonify({"error": "Invalid signature"}), 401
data = request.json
event_type = data.get('event_type')
event_data = data.get('event_data')
print(f"Received webhook event: {event_type}")
if event_type == 'avatar_video.success':
process_successful_video(event_data)
elif event_type == 'avatar_video.fail':
process_failed_video(event_data)
elif event_type == 'video_translate.success':
process_successful_translation(event_data)
elif event_type == 'video_translate.fail':
process_failed_translation(event_data)
# Always respond quickly to webhook events
return jsonify({"status": "received"}), 200
def process_successful_video(data):
"""Process a successful video generation event"""
video_id = data.get('video_id')
video_url = data.get('url')
callback_id = data.get('callback_id')
print(f"Video {video_id} is ready!")
print(f"Video URL: {video_url}")
# Download the video
download_video(video_url, f"video_{video_id}.mp4")
# Here you could:
# 1. Send an email notification
# 2. Update a database record
# 3. Trigger the next step in your workflow
# 4. Push to your content management system
def process_failed_video(data):
"""Process a failed video generation event"""
video_id = data.get('video_id')
error_msg = data.get('msg')
callback_id = data.get('callback_id')
print(f"Video {video_id} generation failed!")
print(f"Error: {error_msg}")
# Here you could:
# 1. Log the error
# 2. Send an alert to administrators
# 3. Retry the generation with different parameters
def process_successful_translation(data):
"""Process a successful video translation event"""
translation_id = data.get('video_translate_id')
video_url = data.get('url')
callback_id = data.get('callback_id')
language = data.get('output_language')
print(f"Translation {translation_id} to {language} is ready!")
print(f"Video URL: {video_url}")
download_video(video_url, f"translation_{translation_id}_{language}.mp4")
def process_failed_translation(data):
"""Process a failed video translation event"""
translation_id = data.get('video_translate_id')
error_msg = data.get('message')
callback_id = data.get('callback_id')
language = data.get('output_language')
print(f"Translation {translation_id} to {language} failed!")
print(f"Error: {error_msg}")
def download_video(url, filename):
"""Download a video from the provided URL"""
try:
response = requests.get(url)
if response.status_code == 200:
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Downloaded video to {filename}")
return True
else:
print(f"Failed to download video. Status code: {response.status_code}")
return False
except Exception as e:
print(f"Exception during download: {e}")
return False
if __name__ == '__main__':
# For development only - use a production server in real applications
app.run(debug=True, port=5000)
Processing Webhook Events for Heygen API
Webhook payloads vary by event type:
avatar_video.success:
{
"event_type": "avatar_video.success",
"event_data": {
"video_id": "<video_id>",
"url": "<video-url>",
"callback_id": "<callback_id>"
}
}
avatar_video.fail:
{
"event_type": "avatar_video.fail",
"event_data": {
"video_id": "<video_id>",
"msg": "<failure-message>",
"callback_id": "<callback_id>"
}
}
video_translate.success:
{
"event_type": "video_translate.success",
"event_data": {
"video_translate_id": "<video_translate_id>",
"url": "<video-url>",
"callback_id": "<callback_id>",
"output_language": "<output-language>"
}
}
video_translate.fail:
{
"event_type": "video_translate.fail",
"event_data": {
"video_translate_id": "<video_translate_id>",
"message": "<failure-message>",
"callback_id": "<callback_id>",
"output_language": "<output-language>"
}
}
Managing Webhook Endpoints for Heygen API
Listing Registered Endpoints for Heygen API
To view all your registered webhook endpoints:
curl -XGET <https://api.heygen.com/v1/webhook/endpoint.list> \\\\
-H 'Accept: application/json' \\\\
-H 'X-Api-Key: <your-api-key>'
Updating an Endpoint for Heygen API
To modify an existing webhook endpoint:
curl -XPATCH <https://api.heygen.com/v1/webhook/endpoint.update> \\\\
-H 'Content-Type: application/json' \\\\
-H 'X-Api-Key: <your-api-key>' \\\\
-d '{
"endpoint_id": "<endpoint_id>",
"url": "<updated-url>",
"events": ["avatar_video.success", "avatar_video.fail", "video_translate.success"]
}'
Deleting an Endpoint for Heygen API
To remove a webhook endpoint:
curl --location --request DELETE '<https://api.heygen.com/v1/webhook/endpoint.delete?endpoint_id=><endpoint_id>' \\\\
--header 'accept: application/json' \\\\
--header 'X-Api-Key: <your-api-key>'
Heygen API OAuth 2.0 Integration
For applications that need to act on behalf of Heygen users, you can implement OAuth 2.0.
Setting Up OAuth for Heygen API
Prerequisites:
- Your Heygen Client ID (provided by the Heygen partnerships team)
- Your approved Redirect URI
Before you can implement OAuth, you need to submit an Integration Intake form to Heygen. Upon approval, you'll receive your Client ID and have your Redirect URI approved.
Implementing Authorization Flow for Heygen API
Heygen uses the Authorization Code Flow with PKCE (Proof Key for Code Exchange) for enhanced security. Here's how to implement it:
Step 1: Redirect Users to Authorization URL for Heygen API
Redirect your users to Heygen's authorization URL:
<https://app.heygen.com/oauth/authorize?client_id=YOUR_CLIENT_ID&state=RANDOM_STATE&redirect_uri=YOUR_REDIRECT_URI&response_type=code>
Replace:
YOUR_CLIENT_ID
: Your Heygen Client IDRANDOM_STATE
: A unique string to maintain state and prevent CSRF attacksYOUR_REDIRECT_URI
: Your approved redirect URI (must be URL-encoded)
Example implementation in a web application:
<a href="<https://app.heygen.com/oauth/authorize?client_id=abcdef123456&state=xyz789&redirect_uri=https%3A%2F%2Fyourapp.com%2Foauth%2Fcallback&response_type=code>">
Connect with Heygen
</a>
Step 2: Handle the Authorization Callback for Heygen API
After the user approves access, they will be redirected to your Redirect URI with a code parameter:
<https://yourapp.com/oauth/callback?code=AUTHORIZATION_CODE&state=RANDOM_STATE>
Here's how to handle this callback:
# Flask example
@app.route('/oauth/callback')
def oauth_callback():
# Get the code and state from the request
code = request.args.get('code')
state = request.args.get('state')
# Verify the state matches what you sent
if state != session.get('oauth_state'):
return "Invalid state parameter", 400
# Exchange the code for an access token
token_response = exchange_code_for_token(code)
if not token_response:
return "Failed to obtain access token", 500
# Store the tokens securely
session['access_token'] = token_response['access_token']
session['refresh_token'] = token_response['refresh_token']
session['token_expiry'] = time.time() + token_response['expires_in']
return redirect('/dashboard')
Step 3: Exchange Authorization Code for Access Token for Heygen API
Exchange the authorization code for an access token:
def exchange_code_for_token(code):
payload = {
"code": code,
"client_id": "YOUR_CLIENT_ID",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI",
"code_verifier": "YOUR_CODE_VERIFIER" # If using PKCE
}
headers = {
"Content-Type": "application/x-www-form-urlencoded"
}
response = requests.post(
"<https://api2.heygen.com/v1/oauth/token>",
data=payload,
headers=headers
)
if response.status_code == 200:
return response.json()
else:
print(f"Error exchanging code: {response.text}")
return None
The response contains:
{
"token_type": "Bearer",
"access_token": "YyzWfeiqmklLsvzNsallQgUgfKHaNSnpv60BNgLGsC",
"expires_in": 864000,
"refresh_token": "dfU22fh6iD4aCkpy9GI32ulJXVe5eC8u4rt4SXedJHHich6L"
}
Using Access Tokens for Heygen API
Make API requests with the access token:
def make_api_request(endpoint, access_token):
headers = {
"Authorization": f"Bearer {access_token}"
}
response = requests.get(
f"<https://api.heygen.com/v1/{endpoint}>",
headers=headers
)
if response.status_code == 200:
return response.json()
elif response.status_code == 401:
# Token might have expired
print("Authentication failed. Token might have expired.")
return None
else:
print(f"API request failed: {response.text}")
return None
For example, to get user account information:
user_info = make_api_request("pacific/account.get", access_token)
Refreshing Tokens for Heygen API
When an access token expires, use the refresh token to obtain a new one:
def refresh_access_token(refresh_token):
payload = {
"client_id": "YOUR_CLIENT_ID",
"grant_type": "refresh_token",
"refresh_token": refresh_token
}
headers = {
"Content-Type": "application/x-www-form-urlencoded"
}
response = requests.post(
"<https://api2.heygen.com/v1/oauth/refresh_token>",
data=payload,
headers=headers
)
if response.status_code == 200:
return response.json()
else:
print(f"Error refreshing token: {response.text}")
return None
Common Error Codes of Heygen API
Error Code | Description | Solution |
---|---|---|
40001 | Unauthorized | Check your API key or access token |
40004 | Resource not found | Verify IDs for avatars, voices, etc. |
40011 | Invalid parameters | Check your request payload for errors |
40119 | Video is too long | Shorten input text or upgrade your plan |
42902 | Rate limit exceeded | Implement backoff or upgrade your plan |
50001 | Internal server error | Retry or contact support |
When you encounter an error:
- Check the error message and code in the response
- Verify your inputs are valid
- Inspect your API key or token
- Look for typos in your request payload
- For persistent issues, check Heygen's status page or contact support
How to Optimize Heygen API for Better Performance
- Text Length: Keep input text concise (under 1500 characters for best performance)
- Resolution: Use lower resolutions for faster processing
- Background Type: Solid colors process faster than images or videos
- Parallel Requests: Limit concurrent requests to avoid rate limiting
- Error Handling: Implement exponential backoff for retries
- Webhook Processing: Keep webhook handlers fast and non-blocking
- Download Timing: Download videos promptly before URLs expire
Example of a robust error handling approach:
def make_api_request_with_retry(endpoint, payload, api_key, max_retries=3, base_delay=1):
headers = {
'X-Api-Key': api_key,
'Content-Type': 'application/json'
}
attempt = 0
while attempt < max_retries:
try:
response = requests.post(
f'<https://api.heygen.com/{endpoint}>',
headers=headers,
data=json.dumps(payload),
timeout=30
)
if response.status_code == 200:
return response.json()
if response.status_code == 429: # Rate limit
retry_after = int(response.headers.get('Retry-After', base_delay * (2 ** attempt)))
print(f"Rate limit hit. Waiting {retry_after} seconds.")
time.sleep(retry_after)
elif 500 <= response.status_code < 600: # Server errors
delay = base_delay * (2 ** attempt)
print(f"Server error. Retrying in {delay} seconds.")
time.sleep(delay)
else:
# Client error, don't retry
print(f"Client error: {response.status_code}: {response.text}")
return response.json()
except (requests.exceptions.RequestException, json.JSONDecodeError) as e:
delay = base_delay * (2 ** attempt)
print(f"Request failed: {e}. Retrying in {delay} seconds.")
time.sleep(delay)
attempt += 1
print(f"Failed after {max_retries} attempts")
return None
Conclusion
Throughout this comprehensive guide, you've learned how to harness the power of Heygen's API to create engaging AI-generated videos. We've covered everything from basic avatar videos to advanced features like templates, webhooks, and OAuth integration.
By following the step-by-step instructions in this tutorial, you now have the knowledge to:
- Create professional-looking avatar videos with customized scripts and voices
- Work with templates to produce consistent, scalable video content
- Implement webhooks to automate your workflow
- Integrate Heygen into your applications using OAuth
- Optimize your API usage for better performance and cost efficiency
The potential applications for Heygen's technology are vast:
- Marketing: Create personalized product demonstrations, testimonials, and promotional content
- Education: Develop engaging instructional videos with consistent presenters
- Customer Service: Build interactive help guides and FAQs
- Internal Communications: Standardize company announcements and training materials
- Content Creation: Scale your production of video content across languages and markets
As AI video technology continues to evolve, Heygen remains at the forefront with regular updates and new features. Keep an eye on their documentation for the latest capabilities and best practices.