If you are looking for a way to create fast, scalable, and reliable APIs, you might want to check out gRPC. gRPC is a modern, open-source framework that lets you communicate between different services using protocol buffers. Protocol buffers are a binary serialization format that can encode structured data efficiently and consistently. In this blog post, I will show you what gRPC client is, how it works, and how you can use it to build awesome APIs with apidog.
What is gRPC Client?
gRPCclient is a software component that can send requests and receive responses from a gRPC server. A gRPC server is a service that can handle gRPC calls and provide the requested data or functionality. A gRPC call is a remote procedure call (RPC) that uses protocol buffers as the message format.
gRPC client can be written in any of the supported languages, such as C#, Java, Python, Ruby, Go, etc. You can also use gRPC client libraries to simplify the development process and take advantage of the features provided by gRPC, such as authentication, encryption, load balancing, etc.
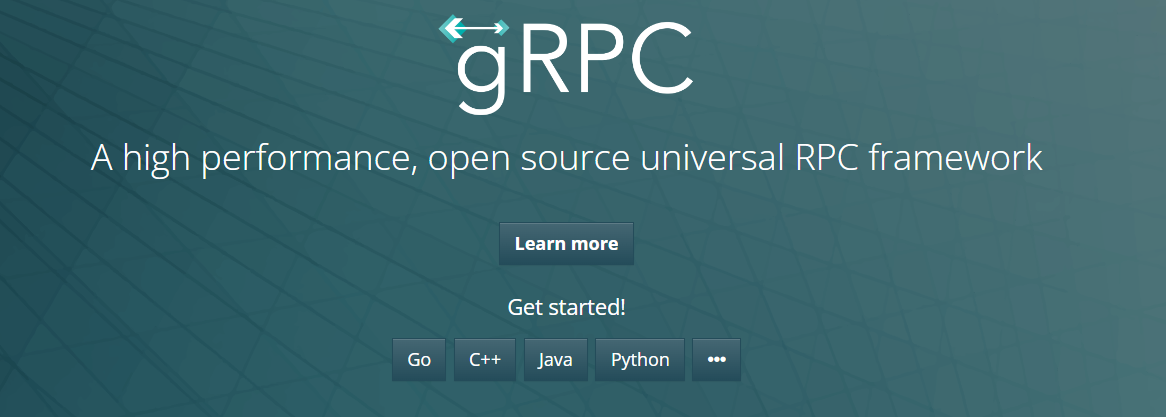
How Does gRPC Client Work?
gRPC client works by following these steps:
- Define the service interface and the message types using protocol buffers. This is a common step for both the client and the server. You can use the
.proto
file extension to write the service definition and the message schema. For example, here is a simple service definition that has one method calledSayHello
that takes aHelloRequest
message and returns aHelloReply
message:
syntax = "proto3";
package helloworld;
service Greeter {
rpc SayHello (HelloRequest) returns (HelloReply) {}
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
- Generate the client code from the
.proto
file using the appropriate compiler plugin for your language. This will create the classes and methods that you can use to interact with the gRPC server. For example, if you are using C#, you can use theprotoc
tool with thegrpc_csharp_plugin
to generate the client code:
protoc -I=. --csharp_out=. --grpc_out=. --plugin=protoc-gen-grpc=grpc_csharp_plugin helloworld.proto
This will create two files: Helloworld.cs
and HelloworldGrpc.cs
. The first file contains the message types, and the second file contains the service interface and the client class.
- Create an instance of the client class and use it to call the server methods. You need to specify the address and the port of the server when creating the client. You can also configure other options, such as credentials, timeouts, etc. For example, here is how you can create a gRPC client and call the
SayHello
method in C#:
using Grpc.Core;
using Helloworld;
class Program
{
static void Main(string[] args)
{
// Create a channel to communicate with the server
var channel = new Channel("localhost:50051", ChannelCredentials.Insecure);
// Create a client instance from the channel
var client = new Greeter.GreeterClient(channel);
// Create a request message
var request = new HelloRequest { Name = "Alice" };
// Call the server method and get the response
var response = client.SayHello(request);
// Print the response message
Console.WriteLine(response.Message);
// Shutdown the channel
channel.ShutdownAsync().Wait();
}
}
How to Use gRPC Client with apidog?
Apidog is a tool that helps you design, document, and test your APIs. You can use apidog to create interactive documentation for your gRPC APIs and share it with your team or clients. You can also use apidog to generate mock servers and clients for your gRPC APIs and test them in various scenarios.
In this mode, the client can continuously send multiple request messages to the server without waiting for immediate responses. After processing all the requests, the server sends a single response message back to the client. This approach is well-suited for efficiently transmitting large amounts of data in a streaming manner, which helps reduce latency and optimize data exchange.
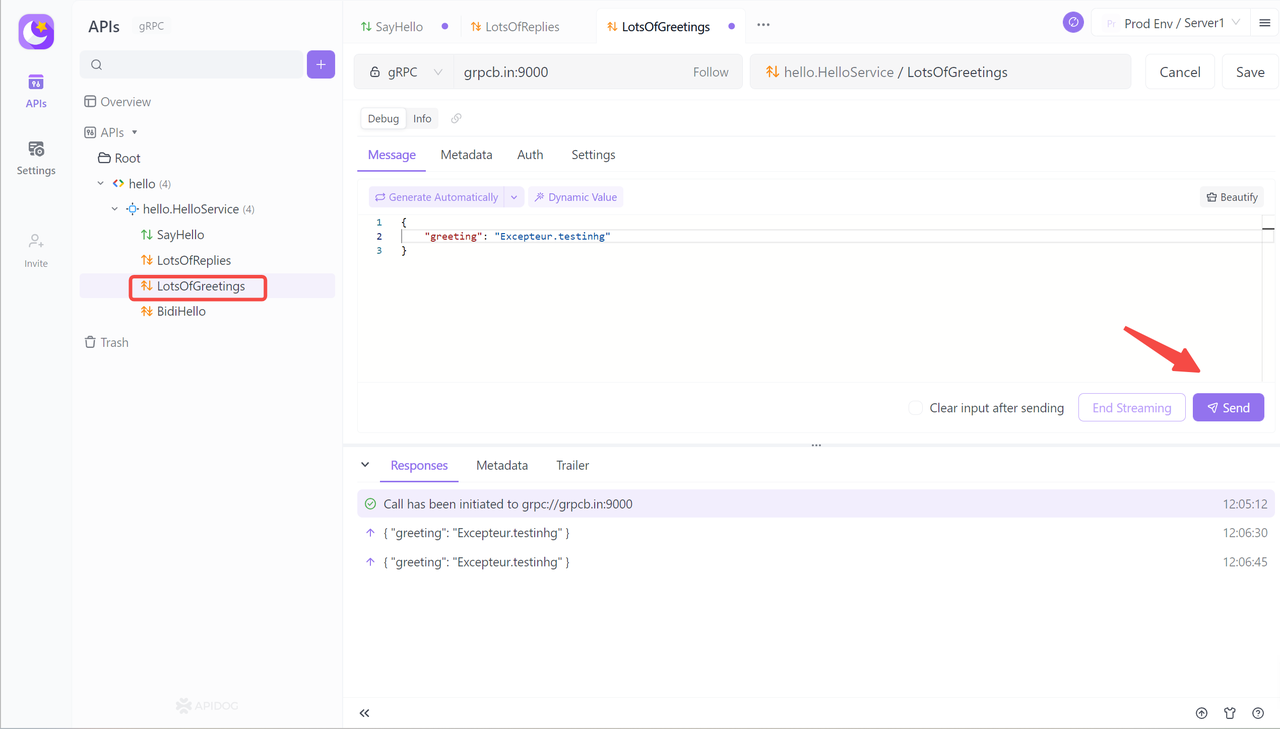
Go to the test tab and create test cases and scenarios for your gRPC API. You can use the mock server and client or the real server and client to test your API. You can also use assertions, variables, hooks, etc. to enhance your tests. You can run your tests and see the results and reports in the test panel.
Why Should You Use gRPC Client?
gRPC client has many benefits that make it a great choice for building APIs. Here are some of the reasons why you should use gRPC client:
- gRPC client is fast and efficient. gRPC uses HTTP/2 as the underlying transport protocol, which supports multiplexing, streaming, compression, etc. gRPC also uses protocol buffers as the message format, which are binary and compact. These features make gRPC client faster and more efficient than other alternatives, such as REST or SOAP.
- gRPC client is simple and consistent. gRPC uses a simple and consistent service definition language, which makes it easy to write and maintain. gRPC also generates the client code from the service definition, which ensures that the client and the server are always in sync and compatible. gRPC also handles the low-level details, such as serialization, deserialization, network errors, etc. for you, so you can focus on the business logic.
- gRPC client is cross-platform and cross-language. gRPC supports many languages and platforms, such as C#, Java, Python, Ruby, Go, etc. You can use gRPC client to communicate with gRPC servers written in any of these languages and running on any of these platforms. You can also use gRPC client to communicate with other gRPC clients, creating a network of microservices that can work together seamlessly.
- gRPC client is extensible and customizable. gRPC allows you to extend and customize the client behavior using interceptors, which are functions that can modify the requests and responses. You can use interceptors to implement features such as logging, tracing, authentication, encryption, etc. You can also use gRPC plugins to integrate with other tools and frameworks, such as apidog, which can help you design, document, and test your gRPC APIs.
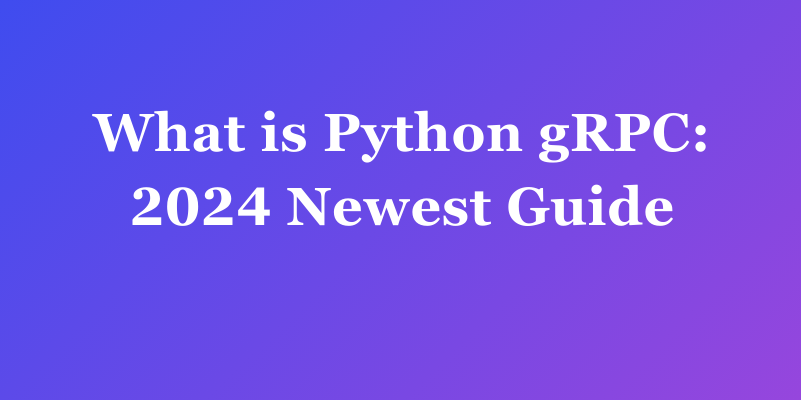
What are the Challenges of Using gRPC Client?
gRPC client is not without its challenges. Here are some of the difficulties that you might face when using gRPC client:
- gRPC client is not widely supported by browsers and proxies. gRPC uses HTTP/2 and protocol buffers, which are not natively supported by most browsers and proxies. This means that you might need to use additional libraries or tools, such as grpc-web or Envoy, to enable gRPC communication between the browser and the server. You might also need to configure your proxies to allow HTTP/2 and binary traffic, which might not be trivial or possible in some cases.
- gRPC client is not very human-readable or interoperable. gRPC uses protocol buffers as the message format, which are binary and not easy to read or modify by humans. This makes it harder to debug or inspect the requests and responses. gRPC also uses a specific service definition language, which might not be compatible with other standards or tools, such as OpenAPI or Postman. You might need to use converters or adapters, such as protoc-gen-openapi or grpcurl, to enable interoperability between gRPC and other formats or tools.
What are the Best Practices for gRPC Client?
To make the most out of gRPC client, you should follow some best practices, such as:
- Use meaningful and consistent names: You should use clear and descriptive names for your services, methods, and messages, and follow the naming conventions for your language and platform. This will make your gRPC client easier to understand and maintain.
- Use appropriate types and formats: You should use the types and formats that best suit your data and your use case, and avoid using unnecessary or complex types and formats. This will make your gRPC client more efficient and reliable.
- Use proper error handling: You should use the error codes and messages that gRPC provides, and handle the errors gracefully on the client side. This will make your gRPC client more robust and user-friendly.
- Use logging and monitoring: You should use the logging and monitoring tools that gRPC offers, and keep track of the performance and the health of your gRPC client. This will make your gRPC client more transparent and secure.
Conclusion
gRPC client is a powerful and versatile tool that allows you to create and consume fast, scalable, and reliable APIs. gRPC client uses HTTP/2 and protocol buffers to exchange data between services, and supports multiple languages and platforms. gRPC client also offers many benefits, such as performance, scalability, reliability, compatibility, and productivity.
To get started with gRPC client, you can use the gRPC tools or Apidog tool. You can also follow the best practices and overcome the challenges and limitations of gRPC client. I hope you enjoyed this blog post, and learned something new and useful about gRPC client.