Grok-3 is xAI's advanced large language model designed to compete with other state-of-the-art AI systems. As with most AI services, xAI implements rate limits on Grok-3 usage to ensure fair distribution of computational resources, maintain service stability, and manage infrastructure costs. This tutorial provides a comprehensive breakdown of Grok-3's rate limits and how to effectively work within these constraints.
Apidog isn’t just another testing tool—it’s designed to simplify and optimize your development process.
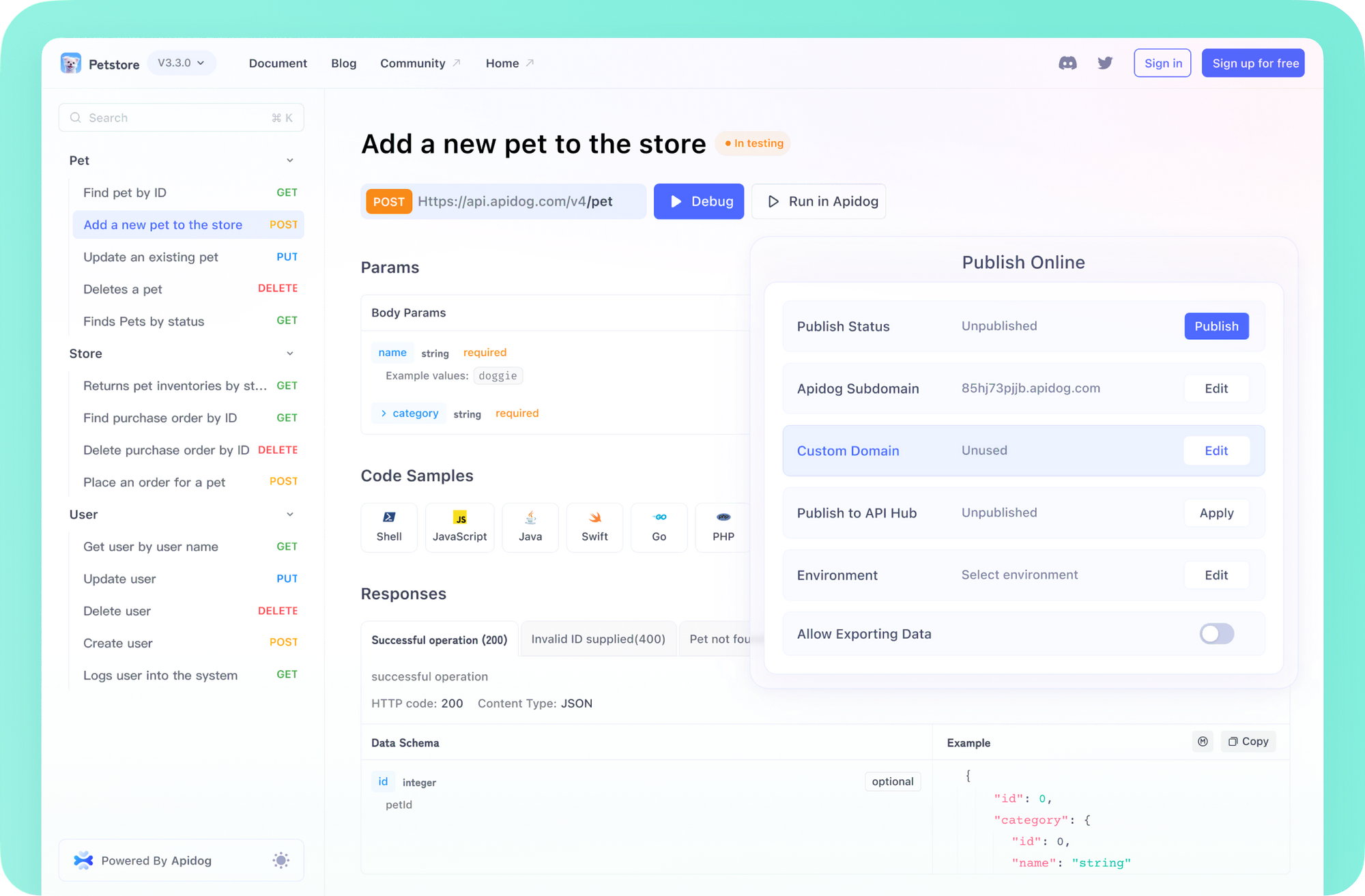
Grok-3 API Rate Limits: Current Structure
Based on available information, Grok-3 implements a tiered rate limiting system that varies depending on user account type and specific features being accessed. Let's examine the current known rate limits:
Grok-3 Access and Usage Limitations
Based on available information from verified sources, Grok-3 access is structured in a tiered system:
- X Premium+ Subscribers: Full access to Grok-3 is available to X Premium+ subscribers, which costs $40/month according to the eWeek article.
- Basic Access for X Users: According to the God of Prompt article, all X users have some level of access to Grok-3 with basic features including DeepSearch and Think Mode, but with unspecified daily limits.
- SuperGrok Subscription: Advanced features of Grok-3, including enhanced DeepSearch capabilities, Think Mode, and higher usage limits are available through a separate "SuperGrok" subscription, reportedly priced at 30/month or 300/year.
- Feature-Specific Limitations: While it's reasonable to assume that different features (standard chat, image generation, DeepSearch, etc.) have separate usage limits, no official documentation was found that specifies the exact numerical quotas or time windows for these limitations.
For the most accurate and current information about Grok-3's specific rate limits and usage quotas, users should consult xAI's official documentation or announcements directly from the company, as these details may change as the service evolves.
How Grok-3 API Rate Limits Are Enforced?
Grok-3's rate limits are enforced through a combination of:
- Per-User Tracking: xAI's systems track usage on a per-user basis (tied to account credentials)
- Feature-Specific Counters: Separate counters for different features (standard chat, image generation, DeepSearch, etc.)
- Rolling Window Implementation: Most limits use a rolling time window rather than fixed calendar-based resets
Grok-3 API Paid Plan (X Premium+) Benefits
Users with paid subscriptions receive higher rate limits and additional features:
- Higher interaction quotas across all categories
- Priority access during high-demand periods
- Full access to premium features like DeepSearch and Reason Mode
- Faster response times due to prioritized request handling
Ways to Handel Grok-3 API's Rate Limits
Strategies for Efficient Rate Limit Management
Request Batching: Combine multiple related queries into a single, well-structured prompt
# Instead of multiple requests:
response1 = grok3_client.complete("What is Python?")
response2 = grok3_client.complete("What are its key features?")
# Batch into one request:
response = grok3_client.complete("""
Please provide information about Python:
1. What is Python?
2. What are its key features?
""")
Implement Client-Side Caching: Store responses for common queries
import hashlib
import json
class Grok3CachingClient:
def __init__(self, api_key, cache_ttl=3600):
self.api_key = api_key
self.cache = {}
self.cache_ttl = cache_ttl
def complete(self, prompt):
# Generate cache key based on prompt
cache_key = hashlib.md5(prompt.encode()).hexdigest()
# Check if response is in cache
if cache_key in self.cache:
cached_response = self.cache[cache_key]
if time.time() - cached_response['timestamp'] < self.cache_ttl:
return cached_response['data']
# Make API call if not in cache
response = self._make_api_call(prompt)
# Cache the response
self.cache[cache_key] = {
'data': response,
'timestamp': time.time()
}
return response
Feature Usage Planning: Plan DeepSearch and Reason Mode usage strategically
def optimize_grok3_usage(queries):
prioritized_queries = []
deep_search_queries = []
reason_mode_queries = []
# Categorize and prioritize queries
for query in queries:
if requires_external_data(query):
deep_search_queries.append(query)
elif requires_complex_reasoning(query):
reason_mode_queries.append(query)
else:
prioritized_queries.append(query)
# Limit to available quotas
deep_search_queries = deep_search_queries[:10] # Limit to daily quota
reason_mode_queries = reason_mode_queries[:1] # Limit to available uses
return {
'standard': prioritized_queries,
'deep_search': deep_search_queries,
'reason_mode': reason_mode_queries
}
Rate Limit Awareness: Implement tracking for different limit categories
class Grok3RateLimitTracker:
def __init__(self):
self.limits = {
'standard': {'max': 20, 'remaining': 20, 'reset_time': None},
'image_gen': {'max': 10, 'remaining': 10, 'reset_time': None},
'deep_search': {'max': 10, 'remaining': 10, 'reset_time': None},
'reason': {'max': 1, 'remaining': 1, 'reset_time': None}
}
def update_from_headers(self, feature_type, headers):
if 'X-RateLimit-Remaining-Requests' in headers:
self.limits[feature_type]['remaining'] = int(headers['X-RateLimit-Remaining-Requests'])
if 'X-RateLimit-Reset-Requests' in headers:
self.limits[feature_type]['reset_time'] = parse_datetime(headers['X-RateLimit-Reset-Requests'])
def can_use_feature(self, feature_type):
return self.limits[feature_type]['remaining'] > 0
Handling Rate Limit Errors
When you encounter a rate limit error (HTTP 429), implement proper handling:
def handle_grok3_request(prompt, feature_type='standard'):
try:
response = grok3_client.complete(prompt, feature=feature_type)
return response
except RateLimitError as e:
reset_time = parse_reset_time(e.headers)
wait_time = (reset_time - datetime.now()).total_seconds()
logger.warning(f"Rate limit hit for {feature_type}. Reset in {wait_time} seconds")
# Implementation options:
# 1. Wait and retry
if wait_time < MAX_ACCEPTABLE_WAIT:
time.sleep(wait_time + 1)
return grok3_client.complete(prompt, feature=feature_type)
# 2. Queue for later processing
task_queue.add_task(prompt, feature_type, execute_after=reset_time)
# 3. Switch to alternative approach
if feature_type == 'deep_search':
return handle_grok3_request(prompt, feature_type='standard')
# 4. Inform user
return {"error": "Rate limit reached", "retry_after": format_datetime(reset_time)}
Multi-User Application Planning
For applications serving multiple users through a single Grok-3 API integration:
- User Quotas: Implement application-level quotas per user that are lower than the API's total quota
- Fair Scheduling: Use a queue system to ensure fair distribution of available API calls
- Priority Users: Consider implementing a tiered system where certain users have priority access
class Grok3ResourceManager:
def __init__(self, total_hourly_limit=100):
self.user_usage = defaultdict(int)
self.total_hourly_limit = total_hourly_limit
self.request_queue = PriorityQueue()
self.last_reset = time.time()
def request_access(self, user_id, priority=0):
# Reset counters if an hour has passed
if time.time() - self.last_reset > 3600:
self.user_usage.clear()
self.last_reset = time.time()
# Check if total API limit is approached
total_usage = sum(self.user_usage.values())
if total_usage >= self.total_hourly_limit:
return False
# Check individual user's fair share
fair_share = max(5, self.total_hourly_limit // len(self.user_usage))
if self.user_usage[user_id] >= fair_share:
# Queue the request for later
self.request_queue.put((priority, user_id))
return False
# Grant access
self.user_usage[user_id] += 1
return True
Conclusion
Understanding and properly managing Grok-3's rate limits is essential for building reliable applications with this powerful AI model. The current rate limit structure reflects xAI's balance between providing access and maintaining system performance:
- Free users: 20 standard interactions per 2 hours, with more limited access to specialized features
- Feature-specific limits: Separate quotas for DeepSearch (10/day) and Reason Mode (limited usage)
- Paid subscribers: Higher limits across all categories
By implementing the strategies outlined in this tutorial, developers can maximize their effective usage of Grok-3 while staying within these constraints. As xAI continues to evolve the Grok platform, these limits may change, so regularly checking the official documentation is recommended for the most up-to-date information.
For enterprise users with higher volume needs, xAI likely offers customized rate limit packages that can be negotiated based on specific use cases and requirements.