For years, creating high-quality video content has been a complex, time-consuming, and often expensive endeavor, requiring specialized skills in cinematography, editing, sound design, and animation. Generative AI, particularly in video, is set to lower these barriers significantly. Imagine generating compelling b-roll footage, crafting dynamic social media animations, or even producing short cinematic sequences, all from textual descriptions or still images. This is the promise of models like Veo 3.
Google has been a significant contributor to AI research and development, and its commitment to generative media is evident in the continuous evolution of models available through Vertex AI. Vertex AI serves as a unified machine learning platform, providing access to Google's cutting-edge AI models, including those from DeepMind, and enabling users to build, deploy, and scale ML applications with ease. The introduction of Veo 3, Imagen 4, and Lyria 2 further solidifies Vertex AI as a powerhouse for creative AI.
Want an integrated, All-in-One platform for your Developer Team to work together with maximum productivity?
Apidog delivers all your demans, and replaces Postman at a much more affordable price!
Introducing Veo 3: The Next Leap in AI Video Generation
Veo 3, developed by Google DeepMind, represents the latest advancement in Google's video generation technology. It aims to provide users with the ability to generate high-quality videos that are not only visually impressive but also rich in auditory detail. Key enhancements and features announced for Veo 3 include:
- Improved Video Quality: Veo 3 is engineered to produce videos of superior quality when generated from both text and image prompts. This means more realistic textures, better motion coherence, and more faithful adherence to complex prompt details. The model is capable of handling intricate prompt details, translating nuanced textual descriptions into compelling visual narratives.
- Integrated Speech Generation: A significant step forward is Veo 3's ability to incorporate speech, such as dialogue and voice-overs, directly into the generated videos. This feature opens up vast possibilities for storytelling, marketing content, and educational materials, allowing creators to add another layer of narrative depth without needing separate audio production workflows for basic speech.
Google Veo 3 realism just broke the Internet yesterday.
— Min Choi (@minchoi) May 22, 2025
This is 100% AI
10 wild examples:
1. Street interview that never happened pic.twitter.com/qdxZVhOO3G
The potential impact of these features is already being recognized by early adopters. Klarna, a leader in digital payments, has been leveraging Veo (and Imagen) on Vertex AI to boost content creation efficiency. They've noted significant reductions in production timelines for assets ranging from b-roll to YouTube bumpers. Justin Thomas, Head of Digital Experience & Growth at Klarna, remarked on the transformation: "With Veo and Imagen, we’ve transformed what used to be time-intensive production processes into quick, efficient tasks that allow us to scale content creation rapidly... What once took us eight weeks is now only taking eight hours, resulting in substantial cost savings.”
How to Use Google Veo API with Vertex AI
Google's Veo models are accessible on Vertex AI, allowing you to generate videos from text or image prompts. You can interact with Veo through the Google Cloud console or by making requests to the Vertex AI API. This guide focuses on using the API, with examples primarily using the Gen AI SDK for Python and REST calls.
Method 1: Use Google Veo 3 with Google Flow
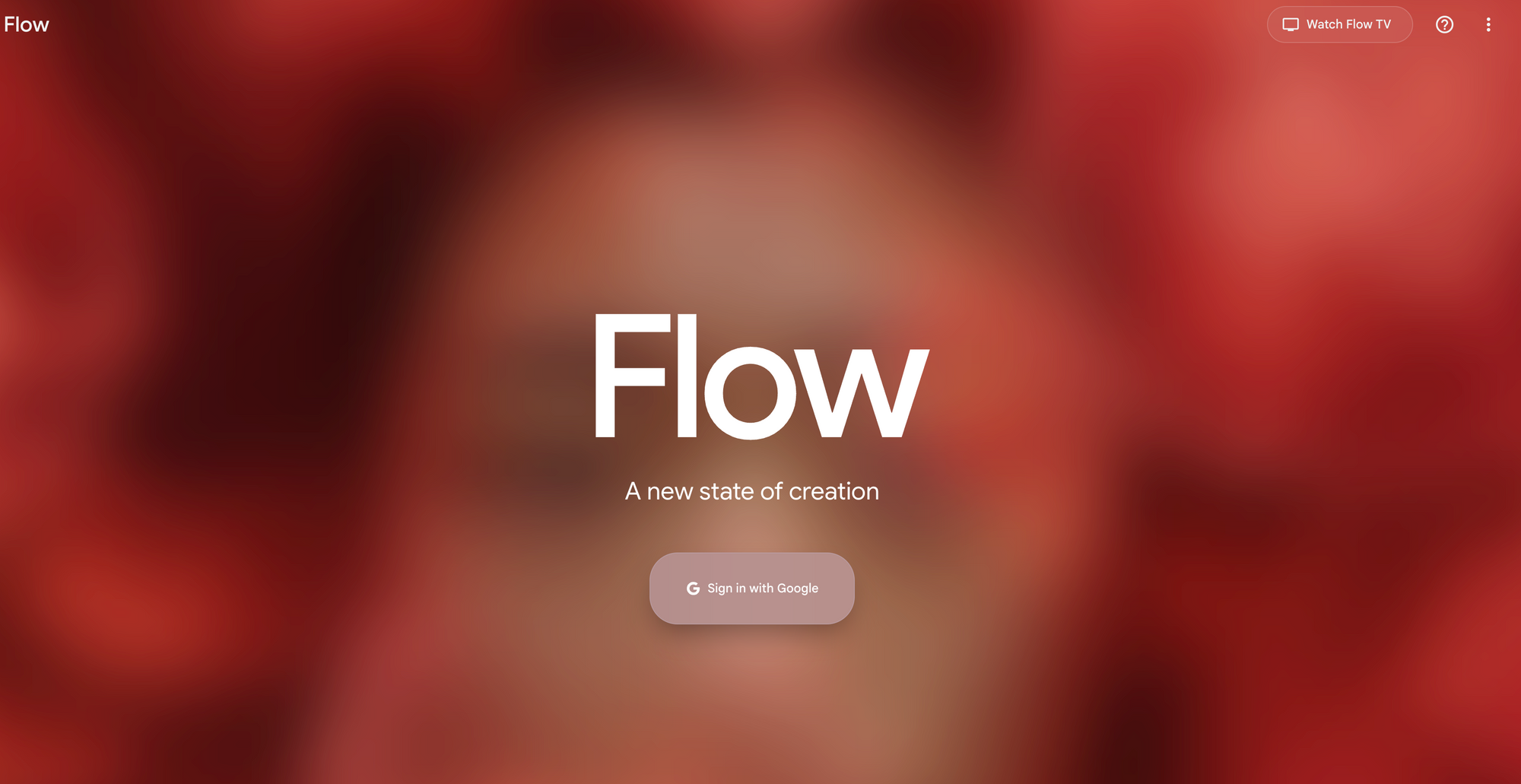
Google has introduced Flow, a revolutionary AI filmmaking tool custom-designed for Google's most advanced models—Veo, Imagen, and Gemini. Flow represents the evolution of VideoFX and is specifically built by and for creatives.
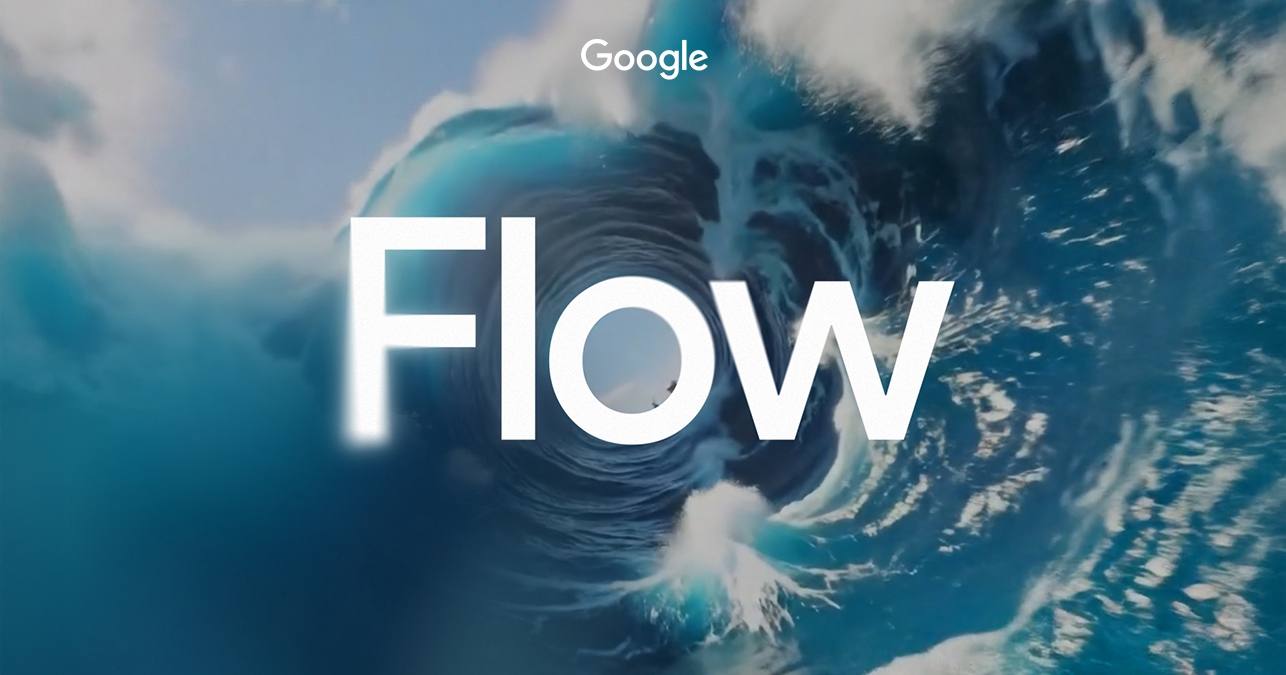
What Flow Offers:
- Intuitive Interface: Describe your vision in everyday language with Gemini-powered prompting
- Camera Controls: Direct control over camera motion, angles, and perspectives
- Scenebuilder: Seamlessly edit and extend existing shots with continuous motion and consistent characters
- Asset Management: Organize ingredients and prompts efficiently
- Flow TV: Browse an ever-growing showcase of clips with visible prompts and techniques
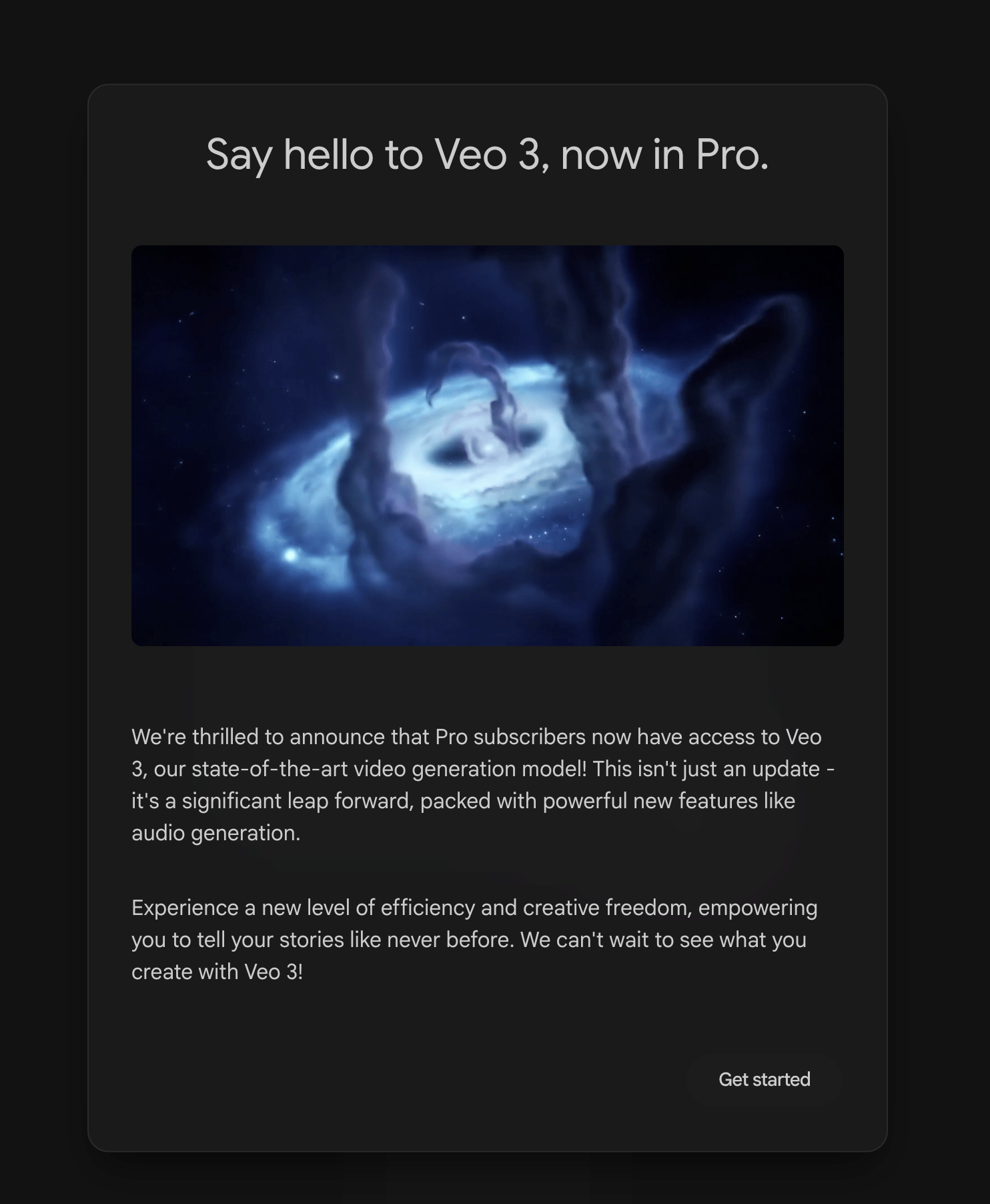
Pricing and Access:
- Google AI Pro Plan: Provides key Flow features and 100 generations per month
- Google AI Ultra Plan: Offers the highest usage limits and early access to Veo 3 with native audio generation, including environmental sounds and character dialogue
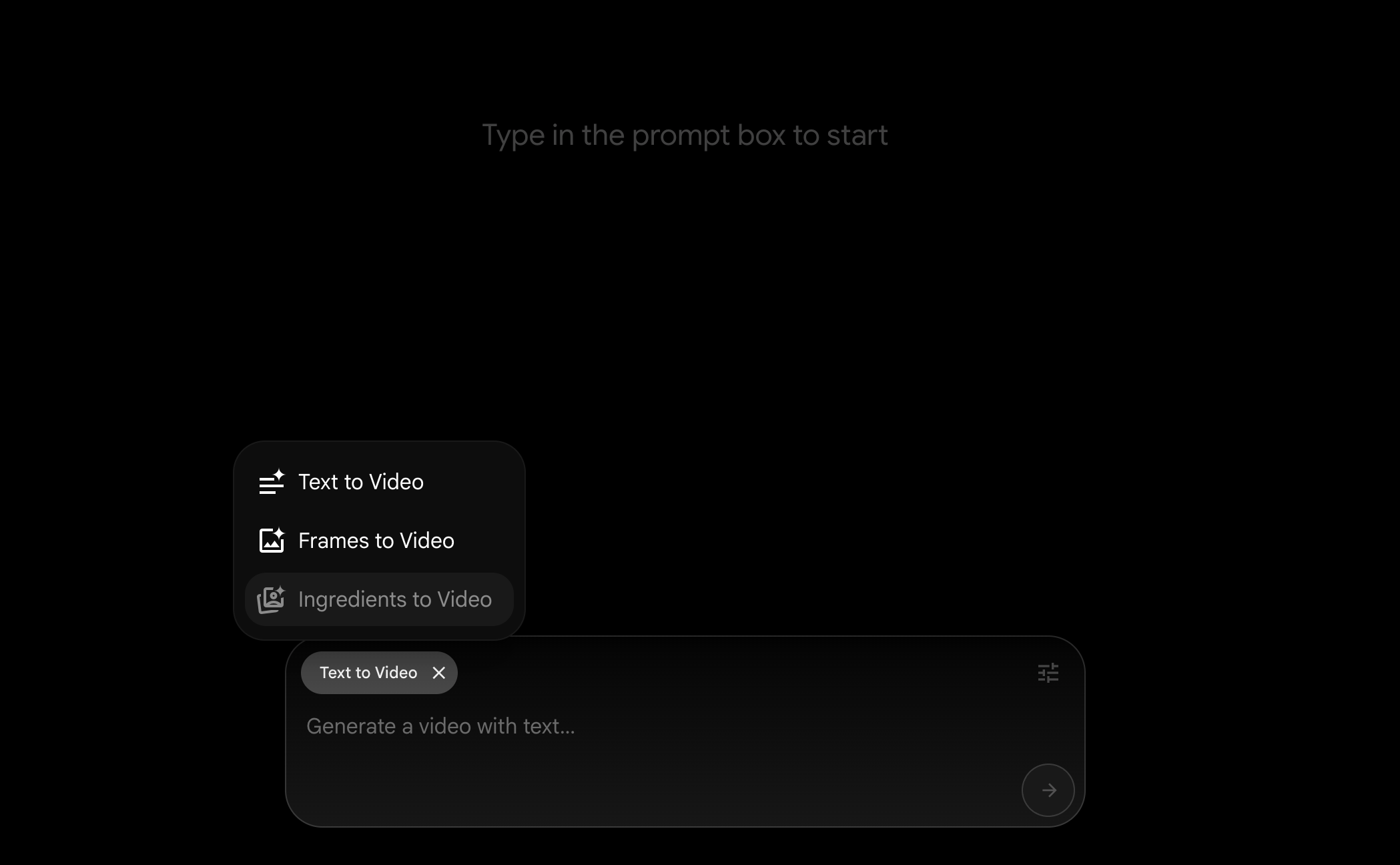
Flow is currently available to subscribers in the U.S., with more countries coming soon. This method is ideal for creators who want a user-friendly interface without dealing with complex API calls or technical setup.
Method 2: Use Google Cloud's $300 Free Credits on Sign Up
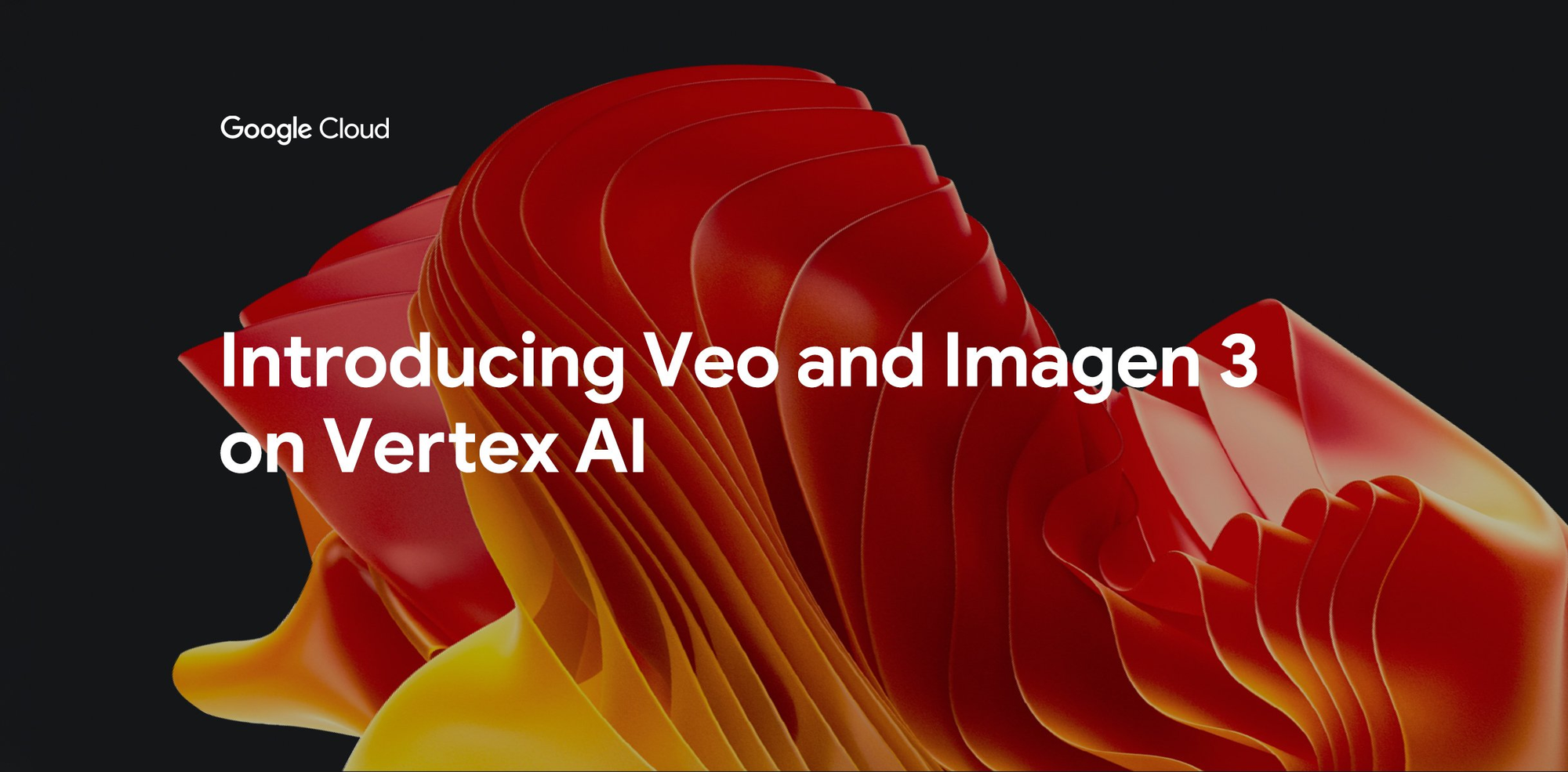

For instance, as highlighted on the Google Cloud website, new users can receive $300 in free credits and free usage of over 20 products. These credits can be applied towards services on Vertex AI, including experimentation with Google Veo 3 models.
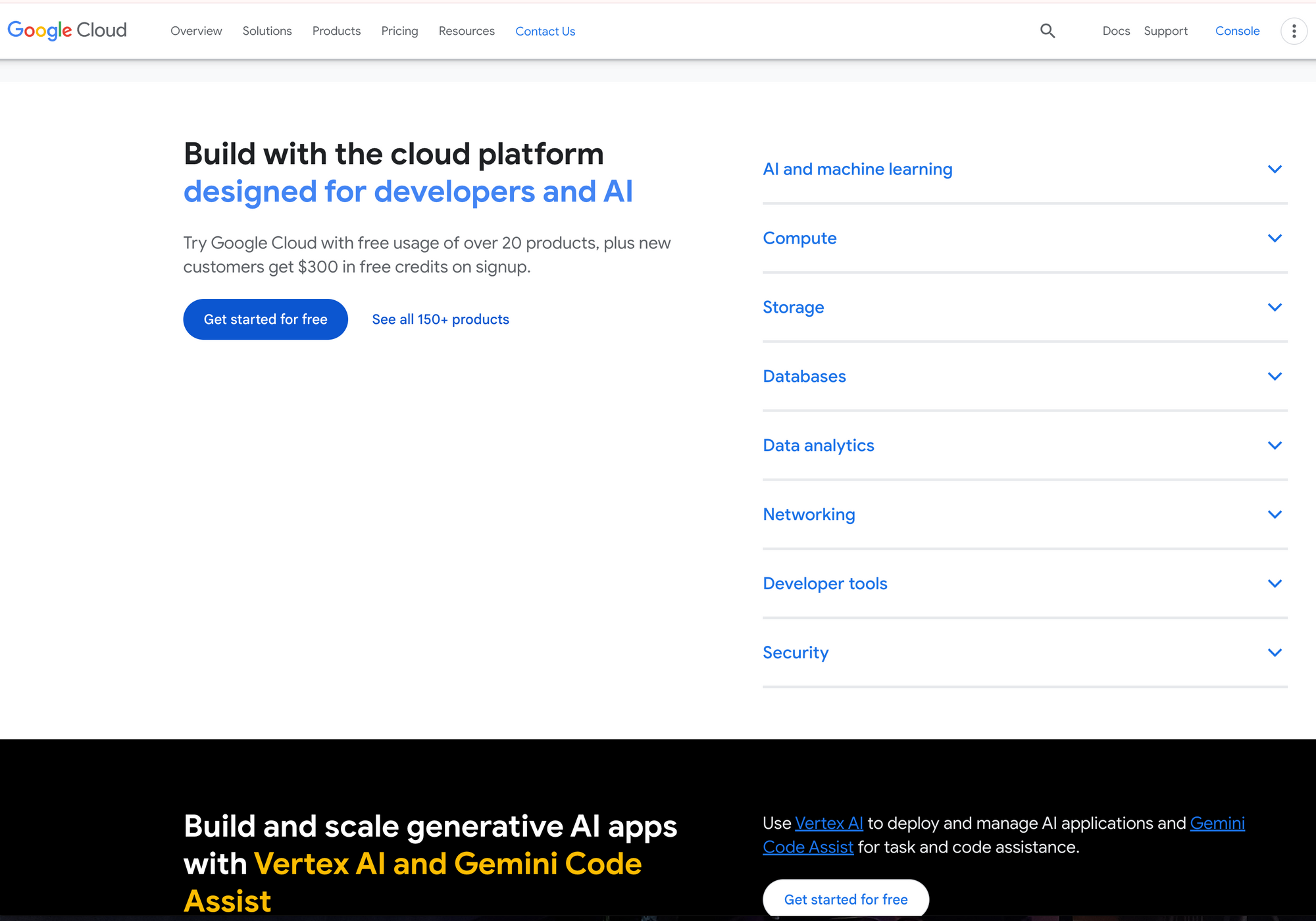
You need to walkthrough the signing up process, which requires verification:
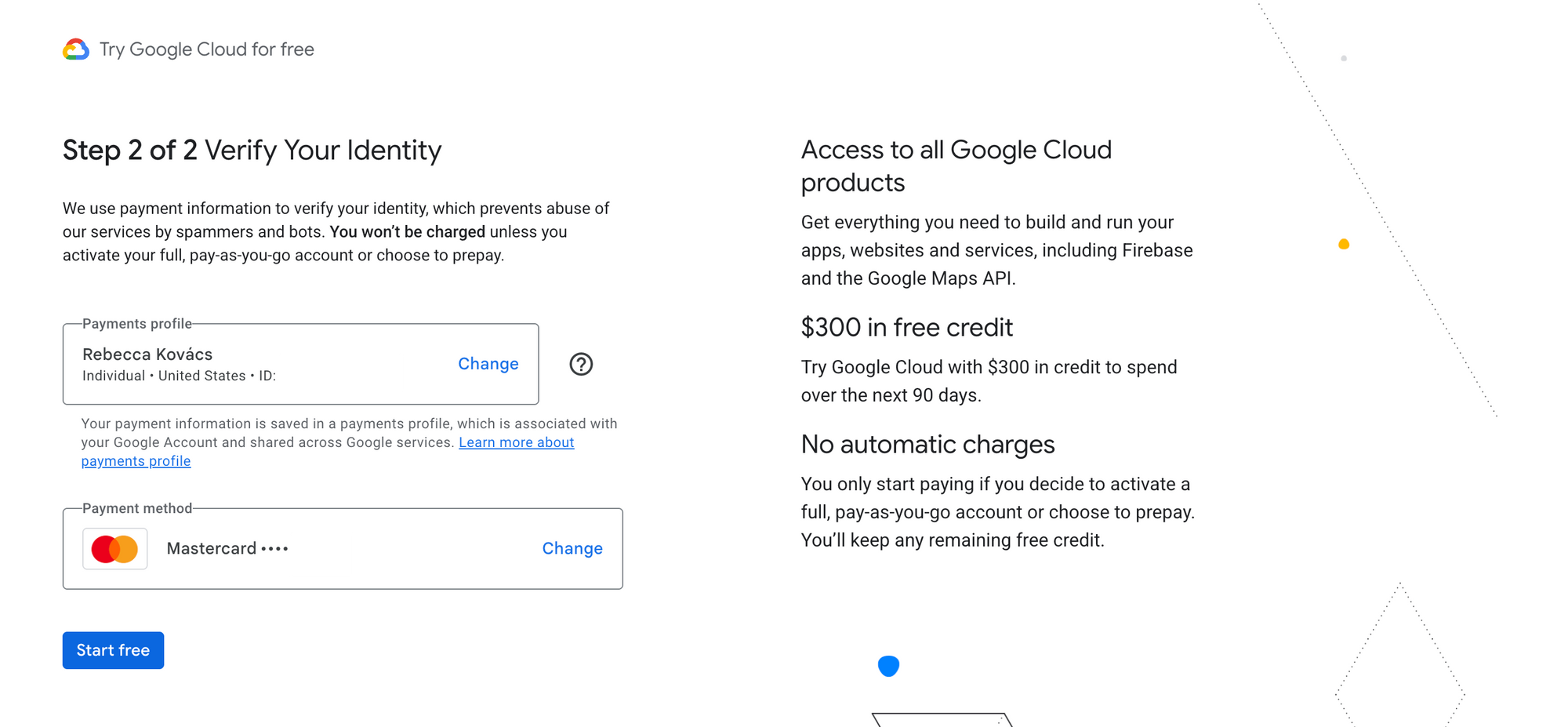
This effectively allows you to try Veo 3 for free, up to the limit of your credits or the platform's free tier allowances. To get started, you'll need to create a Google Cloud account, set up a project, and ensure the Vertex AI API is enabled.
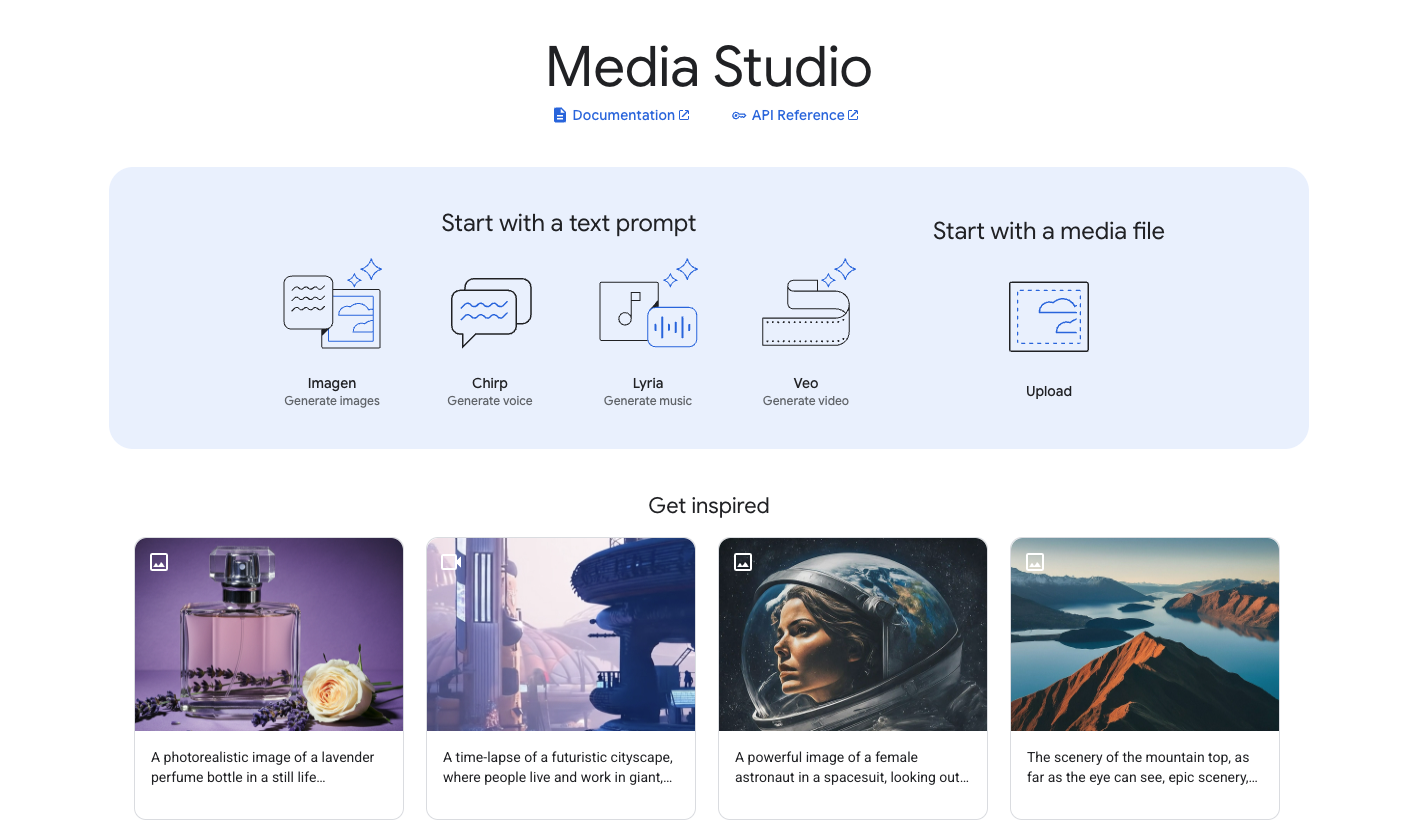
Accessing Veo 3 via API
Currently, Veo 3 (veo-3.0-generate-preview) is available through the Vertex AI API but requires allowlist access. According to the official documentation, this model is in Preview status with controlled access.
Read more about the Official Docs from Google Here:

API Access Requirements:
- Join the waitlist for veo-3.0-generate-preview access
- The model supports text-to-video and image-to-video generation
- Current limitations: 16:9 aspect ratio, 720p resolution, 24 FPS, 8-second maximum length
- Maximum 10 API requests per minute per project.
Method 3: Student Discount for Google AI Pro
Google offers educational discounts and programs that can make accessing Veo 3 more affordable for students and educators.How It Works:
- Students may be eligible for discounted or free access to Google AI Pro
- Educational institutions often have special pricing arrangements
- Some regions offer specific student programs with enhanced credits
Method 3: Student Discount for Google AI Pro
Google offers educational discounts and programs that can make accessing Veo 3 more affordable for students and educators.
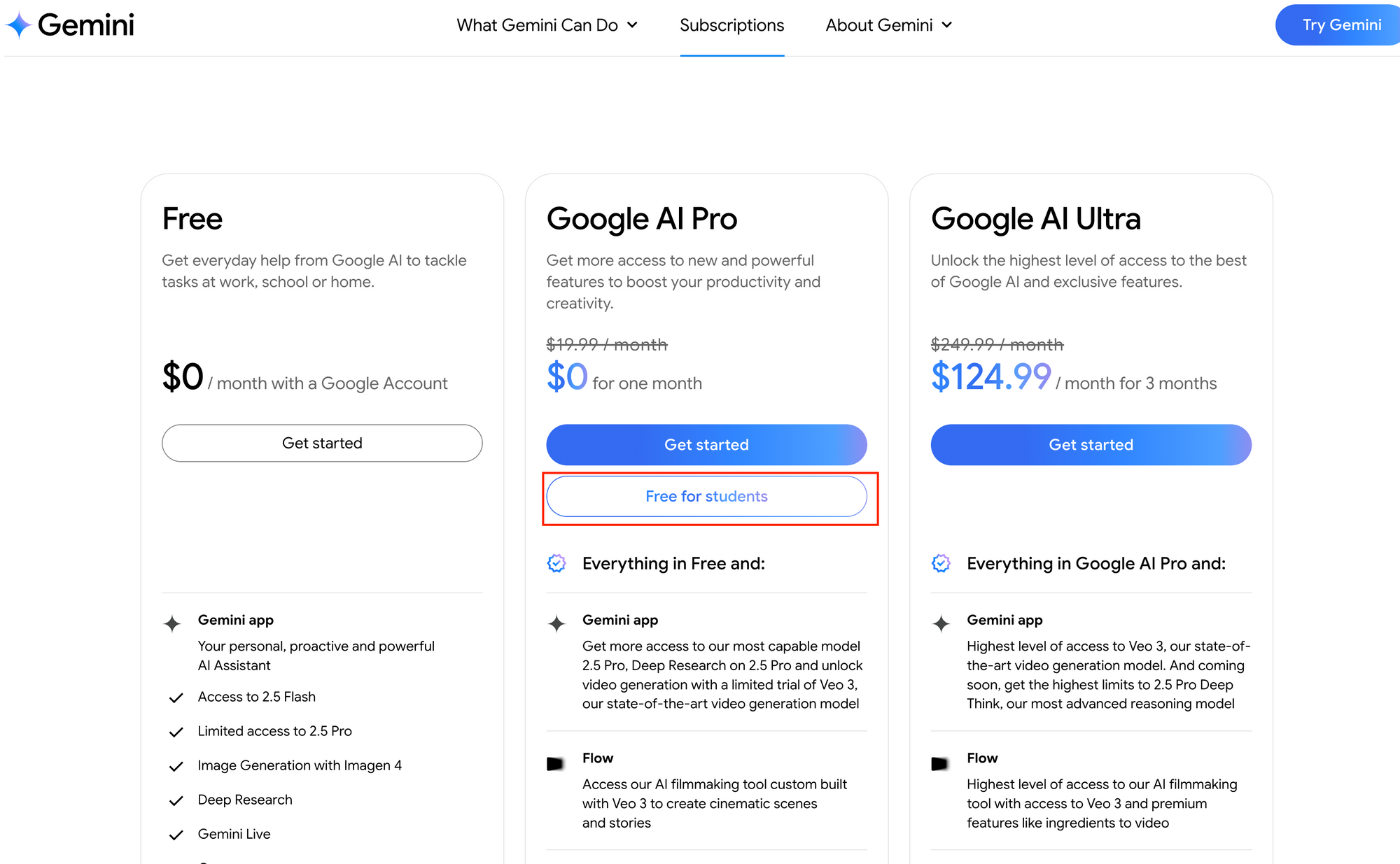
How It Works:
- Students may be eligible for discounted or free access to Google AI Pro
- Educational institutions often have special pricing arrangements
- Some regions offer specific student programs with enhanced credits
To get Student Access, you need to:
Step 1. Sign up the plan at Google One
Keep in mind that some users might be suffering from this error:
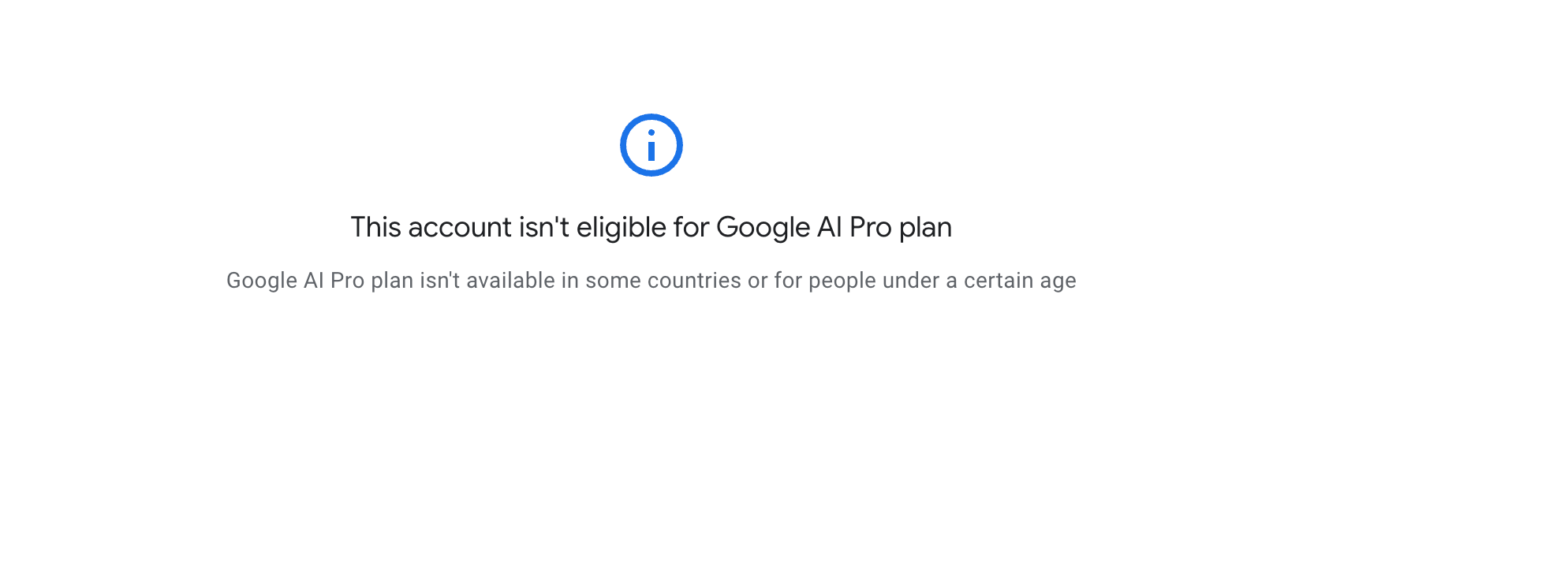
Step 2. Scroll down and choose the 15 Month free option for University Students
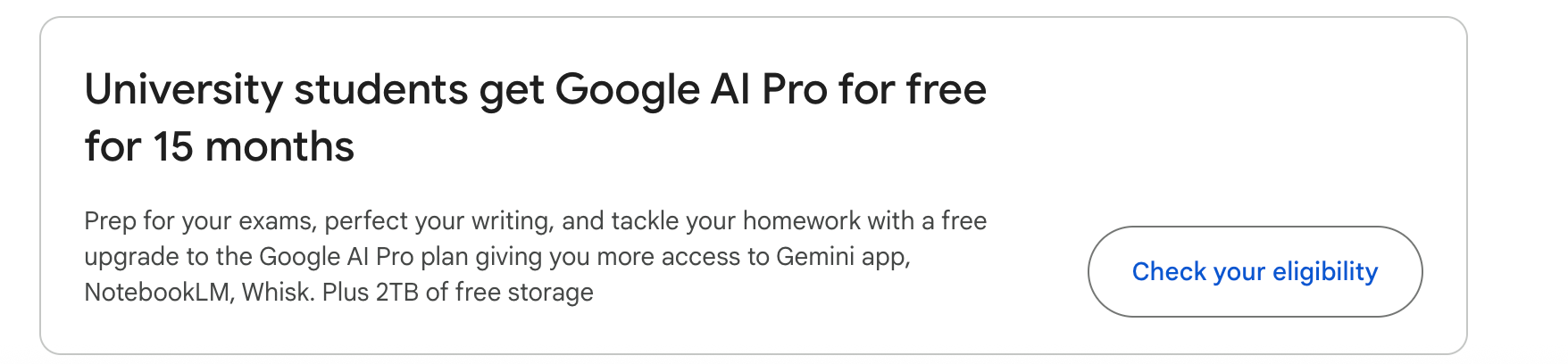
Step 3. Verify Student Status
- Use your educational email address (.edu domain)
- Provide student ID or enrollment verification
- Check if your institution has existing Google for Education partnerships
Important Notes:
- Eligibility varies by region and institution
- Some accounts may show "This account isn't eligible for Google AI Pro plan" if they don't meet current criteria
- Educational pricing and availability change frequently, so check official Google education pages for current offers
Step 4. Once your request is approved, go to Google Gemini and select the Video Option, where you can try Veo 3 Now! (with some limitations, of course)
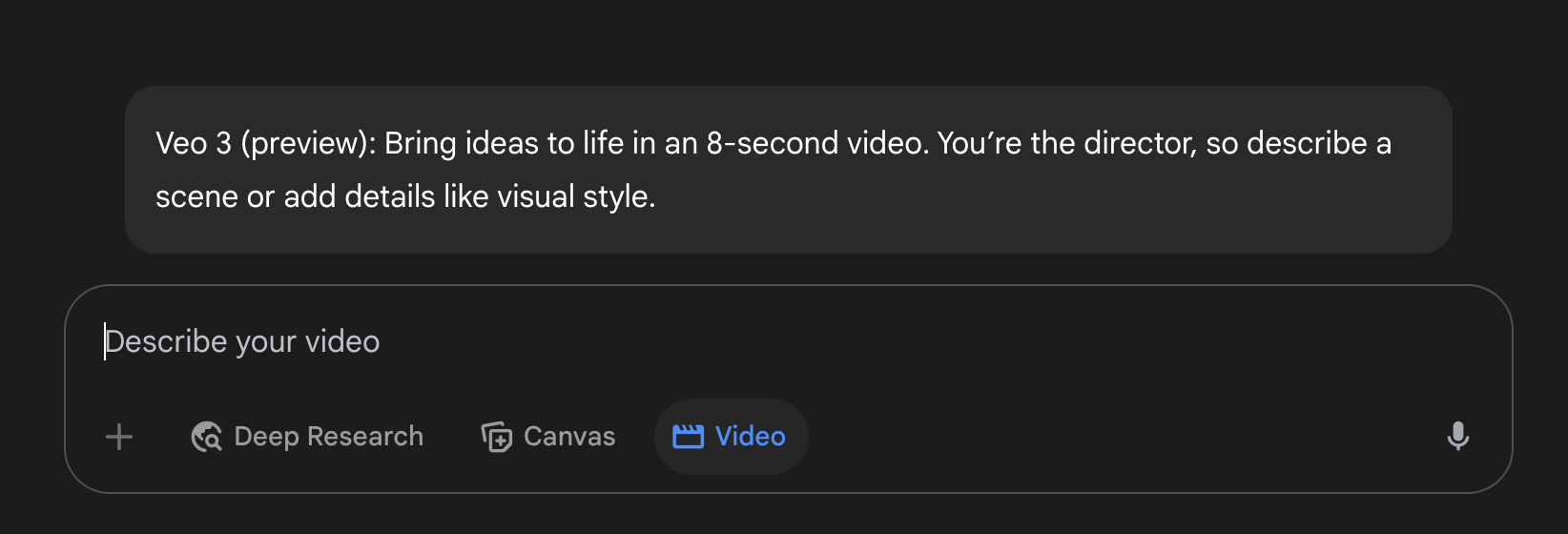
How to Write Better Prompts for Veo 3
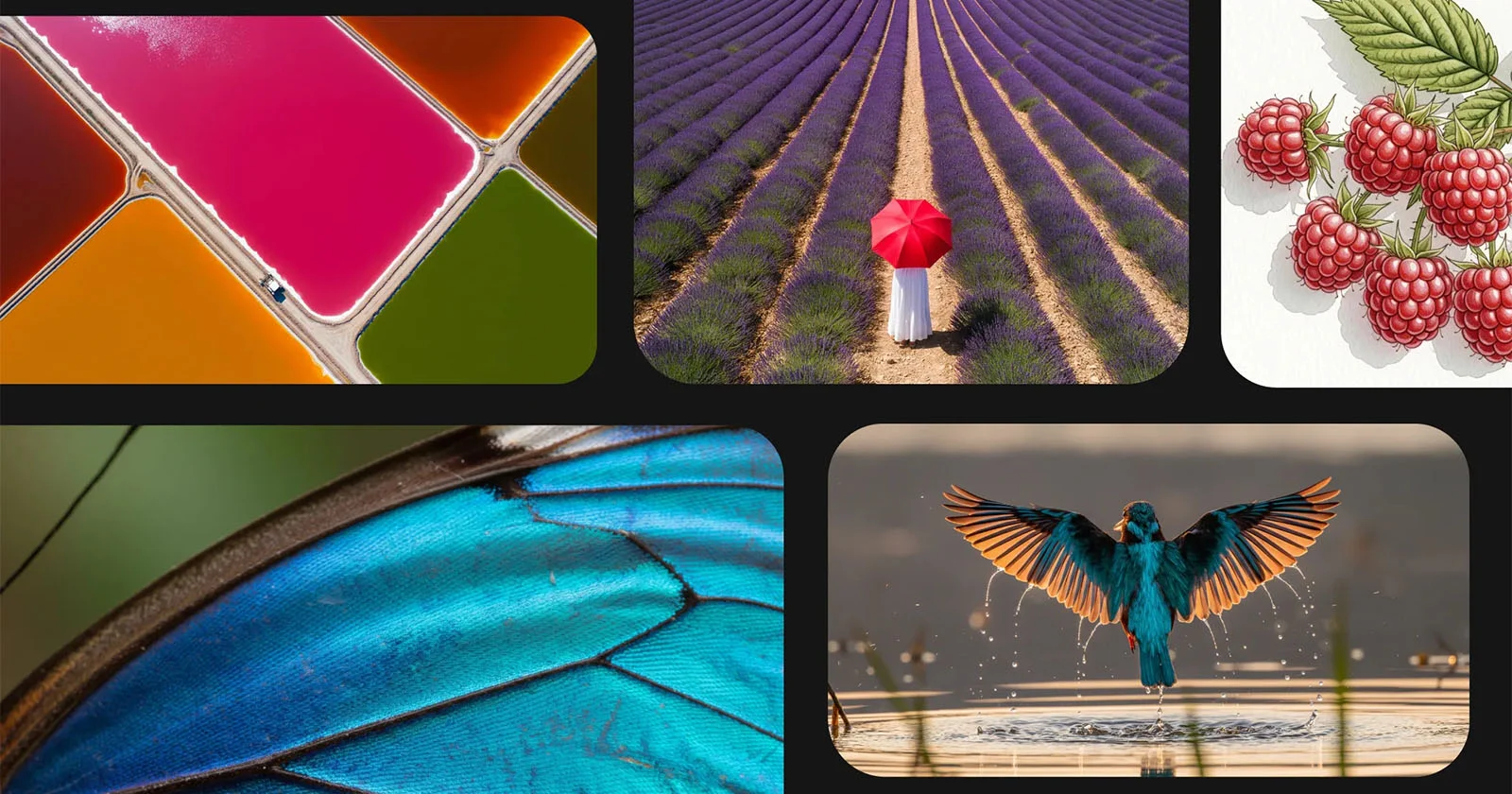
Google Veo Models generates videos based on your textual descriptions. More detailed prompts generally result in higher quality and more relevant videos. Consider describing:
- Subjects and actions.
- Setting and environment.
- Cinematic styles, camera motions.
- Mood and tone.
For models supporting audio (like veo-3.0-generate-preview
), you can include descriptions for transcription (dialogue) and sound effects.
- Prompt Rewriter (Prompt Enhancement):
Veo includes an LLM-based prompt enhancement tool. This feature can rewrite your prompts to add more descriptive details, camera motions, transcriptions, and sound effects, aiming for higher quality video output. - Enabled by Default: This feature is enabled by default for models like
veo-2.0-generate-001
andveo-3.0-generate-preview
. - Disabling: You can turn prompt enhancement off by setting the
enhancePrompt
parameter toFalse
in your REST API call (or a similar parameter in the SDK if available). - Important for
veo-3.0-generate-preview
: You cannot disable the prompt rewriter when using theveo-3.0-generate-preview
model. - Rewritten Prompt in Response: If the original prompt is fewer than 30 words long, the rewritten prompt used by the model is delivered in the API response.
Want an integrated, All-in-One platform for your Developer Team to work together with maximum productivity?
Apidog delivers all your demans, and replaces Postman at a much more affordable price!
Conclusion
Google's Veo models on Vertex AI represent a significant advancement in generative AI, particularly for video creation. By providing intuitive API access through both the Gen AI SDK for Python and direct REST endpoints, Google empowers developers and creators to integrate powerful text-to-video and image-to-video capabilities into their workflows and applications.