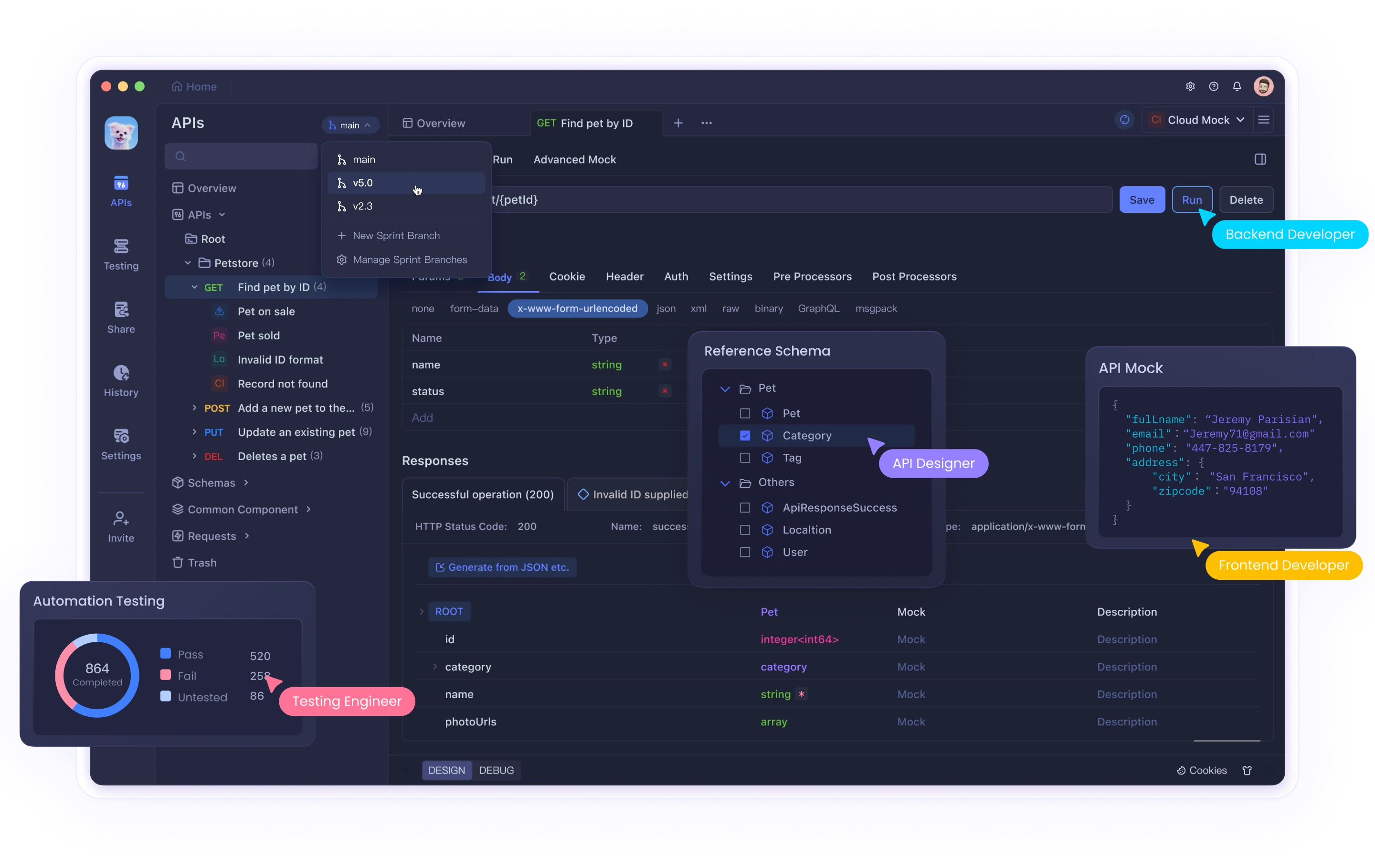
As the tech landscape continues to evolve, Google remains at the forefront of innovation with its powerful suite of APIs. In 2025, one of the most talked-about offerings is the Google Gemini API—a tool that allows developers to integrate advanced machine learning, natural language processing, and data analytics into their applications. Whether you’re building cutting-edge chatbots, data analysis platforms, or creative AI-powered tools, getting started with Google Gemini is an exciting venture.
This guide will walk you through three different options to obtain and use your Google Gemini API key for free in 2025. Each option comes complete with step-by-step instructions and sample code in popular programming languages, making it easy to integrate the API into your own projects. Let’s dive in!
Option 1: Using the Official Google Cloud Platform Free Tier
Overview
Google Cloud Platform (GCP) has long been known for its generous free tier and trial credits for new users. With Google Gemini, GCP continues this tradition by offering a free usage tier that developers can use to explore and integrate the API without incurring costs. This option is ideal for those who want direct access to the API via an official channel, complete with robust documentation and security measures.
Step-by-Step Instructions
Create a Google Cloud Account
- Visit the Google Cloud Console and sign up for a new account if you don’t already have one. Google typically offers a free trial with credits for new users, so take advantage of this offer.
Create a New Project
- In the GCP dashboard, click on “Select a project” and then “New Project.” Give your project a descriptive name (e.g., “GeminiDemoProject”) and select your billing information, if prompted, even if you are using the free tier.
Enable the Google Gemini API
- Navigate to “APIs & Services” in the dashboard.
- Click “Enable APIs and Services” and search for “Google Gemini API.”
- Select the API from the list and click the “Enable” button.
Generate the API Key
- Once the API is enabled, go to the “Credentials” section.
- Click on “Create Credentials” and select “API Key.” A new key will be generated.
- Store this key securely. It is your gateway to using the Gemini API.
Testing the API with Sample Code (Python)
- Before you start integrating with your main application, test the API using a simple Python script. Ensure that you have the
requests
library installed. - Here’s a sample script to help you get started:
import requests
# Replace 'YOUR_API_KEY' with your actual Google Gemini API key
api_key = 'YOUR_API_KEY'
endpoint = 'https://gemini.googleapis.com/v1/query'
headers = {
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json'
}
data = {
"prompt": "What is Google Gemini and how can it revolutionize API integrations in 2025?",
"max_tokens": 100
}
try:
response = requests.post(endpoint, json=data, headers=headers)
response.raise_for_status()
print("API Response:", response.json())
except requests.exceptions.HTTPError as http_err:
print("HTTP error occurred:", http_err)
except Exception as err:
print("An error occurred:", err)
This script sends a query to the Gemini API and prints the JSON response. You can modify the prompt and other parameters according to your project’s needs.
Benefits and Considerations
- Direct Access: Using GCP ensures you are directly interacting with a Google-managed service.
- Security: Manage and control the API key using Google’s built-in tools.
- Scalability: Easily scale your usage by upgrading your account if necessary.
- Monitoring: Access detailed logs and analytics through the GCP console.
However, remember to safeguard your API key and restrict its usage to trusted sources only, as misuse can lead to unexpected charges or security vulnerabilities.
Option 2: Utilizing the Google Gemini Playground
Overview
The Google Gemini Playground is designed specifically for developers who want to experiment with the power of Google’s latest API offerings without setting up a full production environment. The playground provides a controlled, interactive environment where you can quickly generate and test a temporary API key. This option is perfect for rapid prototyping, educational purposes, and small-scale testing.
Step-by-Step Instructions
Access the Google Gemini Playground
- Navigate to the Google Gemini Playground website in your browser. The playground is user-friendly and provides an interactive interface for testing API requests.
Sign In with Your Google Account
- Log in using your Google credentials. If you don’t have an account, you can create one quickly.
- Once signed in, you will see options to experiment with various API endpoints provided by Gemini.
Request a Temporary API Key
- Look for the “Request API Key” or “Get Started for Free” button on the playground homepage.
- Click the button and follow the on-screen instructions. In most cases, you will receive a temporary API key that is valid for a limited period or limited number of requests.
- Note down your temporary key—it’s essential for authentication in subsequent steps.
Test Your API Requests in the Playground Console
- Start by entering a simple query in the console. The interactive console will display the API response, helping you understand the kind of data you can expect.
- Use the provided interface to tweak parameters like
prompt
,max_tokens
, and others.
Testing the API with Sample Code (Node.js)
- To integrate the API into your own Node.js projects, use the following code snippet. Make sure you install the
axios
package using npm if you haven’t already.
const axios = require('axios');
// Replace 'YOUR_TEMPORARY_KEY' with your temporary API key from the Gemini Playground
const apiKey = 'YOUR_TEMPORARY_KEY';
const url = 'https://gemini.googleapis.com/v1/query';
const requestData = {
prompt: 'Describe the benefits of using the Google Gemini API in modern web development.',
max_tokens: 150
};
axios.post(url, requestData, {
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
})
.then(response => {
console.log("API Response:", response.data);
})
.catch(error => {
console.error("Error accessing the API:", error);
});
This Node.js script demonstrates how to send a POST request to the Gemini API and handle the response. It serves as a useful starting point for integrating the API into your backend services.
Benefits and Considerations
- Rapid Testing: The Playground allows you to test and debug your queries in real-time.
- No Initial Setup: There’s no need to create a full Google Cloud project, making this approach faster and more straightforward for prototyping.
- Safe Environment: The temporary nature of the API key reduces the risk of long-term exposure.
- Interactive Learning: The playground’s interface is designed to help you understand the API’s capabilities quickly.
Keep in mind that temporary API keys provided in the playground are meant for development and testing purposes only. For any long-term or production usage, consider moving to a permanent solution like Option 1.
Option 3: Leveraging Third-Party Platforms for Free Access
Overview
For developers who prefer not to directly interact with Google Cloud’s infrastructure or the Gemini Playground, third-party platforms can provide an excellent alternative. Many SaaS providers and developer platforms have partnered with Google to offer free access to the Google Gemini API as part of their developer packages. These platforms not only simplify API key management but also offer additional tools like analytics dashboards, SDKs, and community support.
Step-by-Step Instructions
Choose a Third-Party Platform
- Research and select a reputable third-party provider that offers free access to the Google Gemini API. Look for features like ease of use, security, and a user-friendly dashboard. Popular platforms often have intuitive UIs and clear documentation to help you get started quickly.
Register on the Platform
- Create an account on the chosen platform. Most providers offer a free tier that includes a limited number of API requests per month, perfect for small projects or development purposes.
- Complete any required verification steps such as email confirmation or CAPTCHA challenges.
Link Your Google Account (If Required)
- Some platforms may require you to connect your Google account to verify your identity or to sync your API usage with your Google profile. Follow the platform’s instructions to grant the necessary permissions.
Generate Your API Key
- Once your account is set up, navigate to the API settings or dashboard section.
- Request a Google Gemini API key. In many cases, the platform will automatically generate a key for you and provide clear instructions on how to authenticate your requests.
- Securely store this API key.
Testing the API in a Sandbox Environment
- Most third-party platforms offer a sandbox or testing environment. Use this to experiment with different API calls and see live responses without affecting your production environment.
Testing the API with Sample Code (PHP)
- To get you started with integrating the API into your web applications, here is a PHP sample code snippet that demonstrates how to make a request to the Gemini API using your third-party generated API key.
<?php
// Replace 'YOUR_THIRD_PARTY_API_KEY' with your actual API key provided by the third-party platform
$apiKey = 'YOUR_THIRD_PARTY_API_KEY';
$url = 'https://gemini.googleapis.com/v1/query';
$data = array(
"prompt" => "What innovative features does the Google Gemini API offer for developers in 2025?",
"max_tokens" => 100
);
$headers = array(
"Authorization: Bearer " . $apiKey,
"Content-Type: application/json"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if(curl_errno($ch)){
echo 'Request Error:' . curl_error($ch);
}
curl_close($ch);
echo "API Response: " . $response;
?>
This PHP script leverages cURL to send a POST request to the Google Gemini API endpoint. It’s a convenient example for developers building web applications where PHP is the server-side language.
Benefits and Considerations
- Simplified Onboarding: Third-party platforms often streamline the process of accessing APIs, minimizing setup time.
- Enhanced Features: Many providers offer additional tools such as SDKs, analytics, and community forums to help you get the most out of the API.
- Flexible Integration: These platforms can be particularly useful if you want to integrate multiple APIs from different providers under one roof.
- Free Tiers and Trials: While most platforms offer a free tier, verify the usage limits to ensure they meet your project requirements.
Third-party integrations are an excellent option for developers who prefer not to deal directly with Google Cloud’s administrative interfaces, yet they still want full access to the powerful Gemini API features.
Final Thoughts
As we step into a new era of API integration in 2025, Google Gemini stands out as a frontrunner in delivering advanced AI and machine learning capabilities in an easily accessible format. The three options covered in this guide—using the official Google Cloud Platform free tier, the interactive Gemini Playground, and third-party platforms—offer distinct advantages depending on your development needs and technical preferences.
Key Takeaways
Option 1 (Google Cloud Platform Free Tier):
- Best for developers looking for direct access, enhanced security, and scalable production-level integration.
- Offers robust management of API keys through GCP and detailed monitoring tools.
- Sample code in Python provides a straightforward implementation guide.
Option 2 (Google Gemini Playground):
- Perfect for rapid prototyping, experiments, and learning.
- Provides a safe, interactive environment with temporary API keys to help developers maintain focus on testing and development.
- Sample code in Node.js demonstrates how to integrate the API quickly.
Option 3 (Third-Party Platforms):
- Ideal for those who prefer a simplified onboarding process and additional developer support tools.
- Many platforms simplify API key management and often bundle value-added features such as analytics and SDK support.
- Sample code in PHP gives an example of integrating the API in a web development environment.
Additional Tips for Successful Integration
API Key Security:
- Always protect your API key. Whether you opt for a direct GCP setup or a third-party platform, ensure that the key is stored securely and never exposed publicly.
- Apply restrictions (IP addresses, referrer restrictions) as allowed by the API key management settings.
Handle API Limits Wisely:
- Free tiers often come with usage limits. Monitor your API usage regularly through the provided dashboards.
- Consider implementing exponential backoff and retries in your code to handle rate limiting gracefully.
Stay Updated:
- Technology evolves rapidly. Keep an eye on official announcements from Google regarding updates to the Gemini API, as new features and enhancements might be introduced throughout 2025.
- Regularly review the documentation and developer forums to stay abreast of best practices and common pitfalls.
Experiment and Iterate:
- Use the playground and sandbox environments to experiment without risk. Iterative testing can lead to better understanding and more efficient integration.
- Customize sample codes to fit your project’s needs and share your findings with your developer community.
Wrapping Up
Google Gemini's free offerings in 2025 provide an unparalleled opportunity for developers to build innovative applications using the latest in AI technology. Whether you’re a hobbyist, a startup founder, or an enterprise developer, the options outlined in this guide give you the flexibility to choose the integration approach that best suits your workflow. By following the detailed steps and using the provided sample codes, you can quickly begin to harness the potential of the Gemini API.
Embrace the power of advanced API integrations with Google Gemini and drive your projects to new levels of sophistication. With a robust ecosystem of tools, flexible access options, and a growing developer community, the future of AI-powered development is brighter than ever.
Happy coding, and enjoy the journey into the world of Google Gemini in 2025!