What is Flutter: Understanding the Flutter Framework
Flutter is an open-source application framework developed by Google that has revolutionized cross-platform app development. It allows developers to create natively compiled applications for multiple platforms from a single codebase. This means you can write your code once and deploy it across iOS, Android, web, Windows, macOS, and Linux.
Flutter's key characteristics include:
- Cross-platform development capability
- Use of Dart programming language
- Consistent UI across different platforms with its material design components
- Hot reload functionality for real-time code updates
- High-performance rendering
APIDog integrates perfectly into the Flutter development workflow, allowing you to test your backend endpoints, mock responses, and collaborate with team members—all while maintaining the same efficiency you expect from your Flutter development experience.
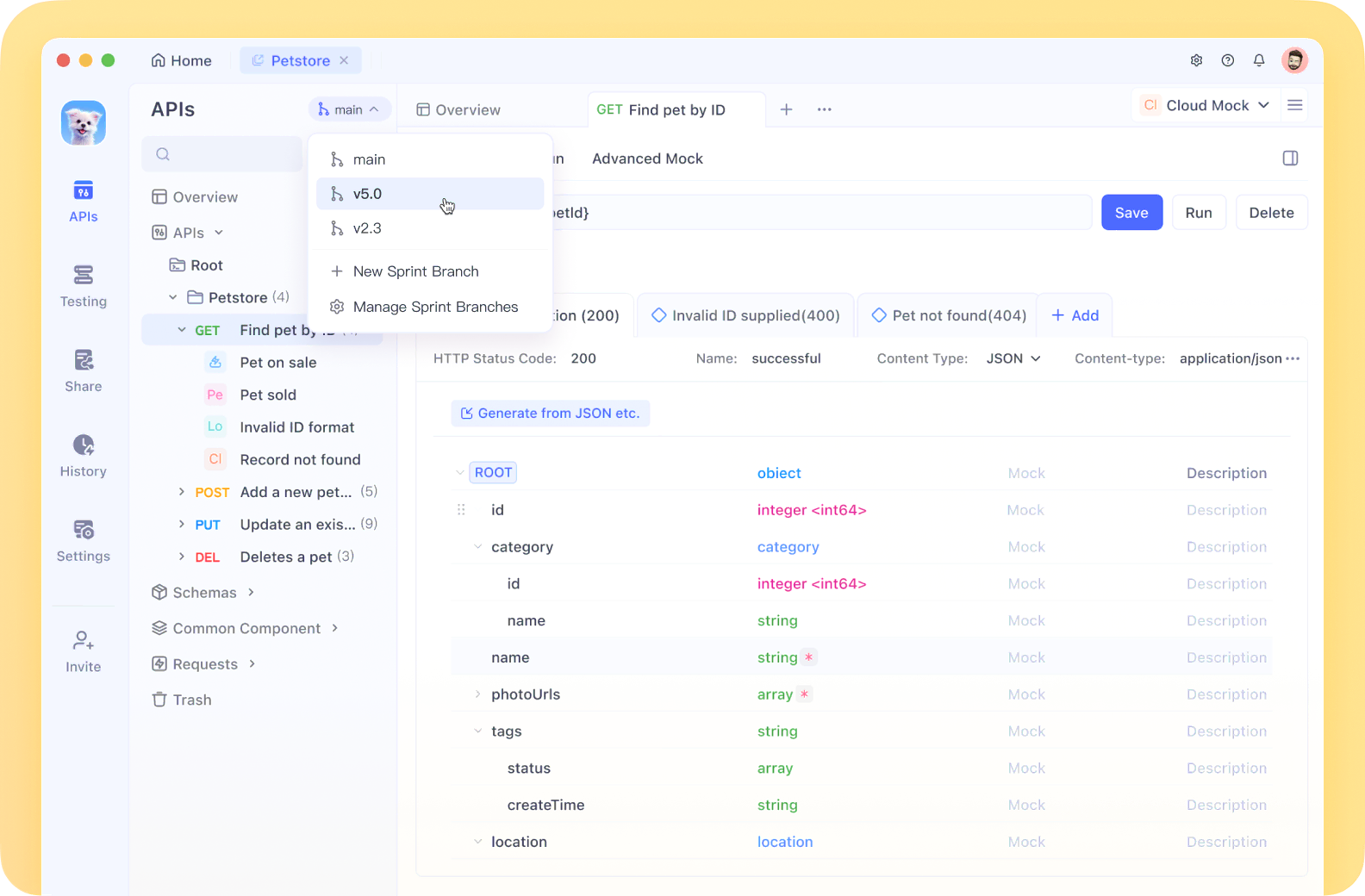
Whether you're building RESTful services or working with GraphQL, APIDog's powerful yet lightweight approach makes it an ideal companion for Flutter developers focused on creating exceptional cross-platform applications.
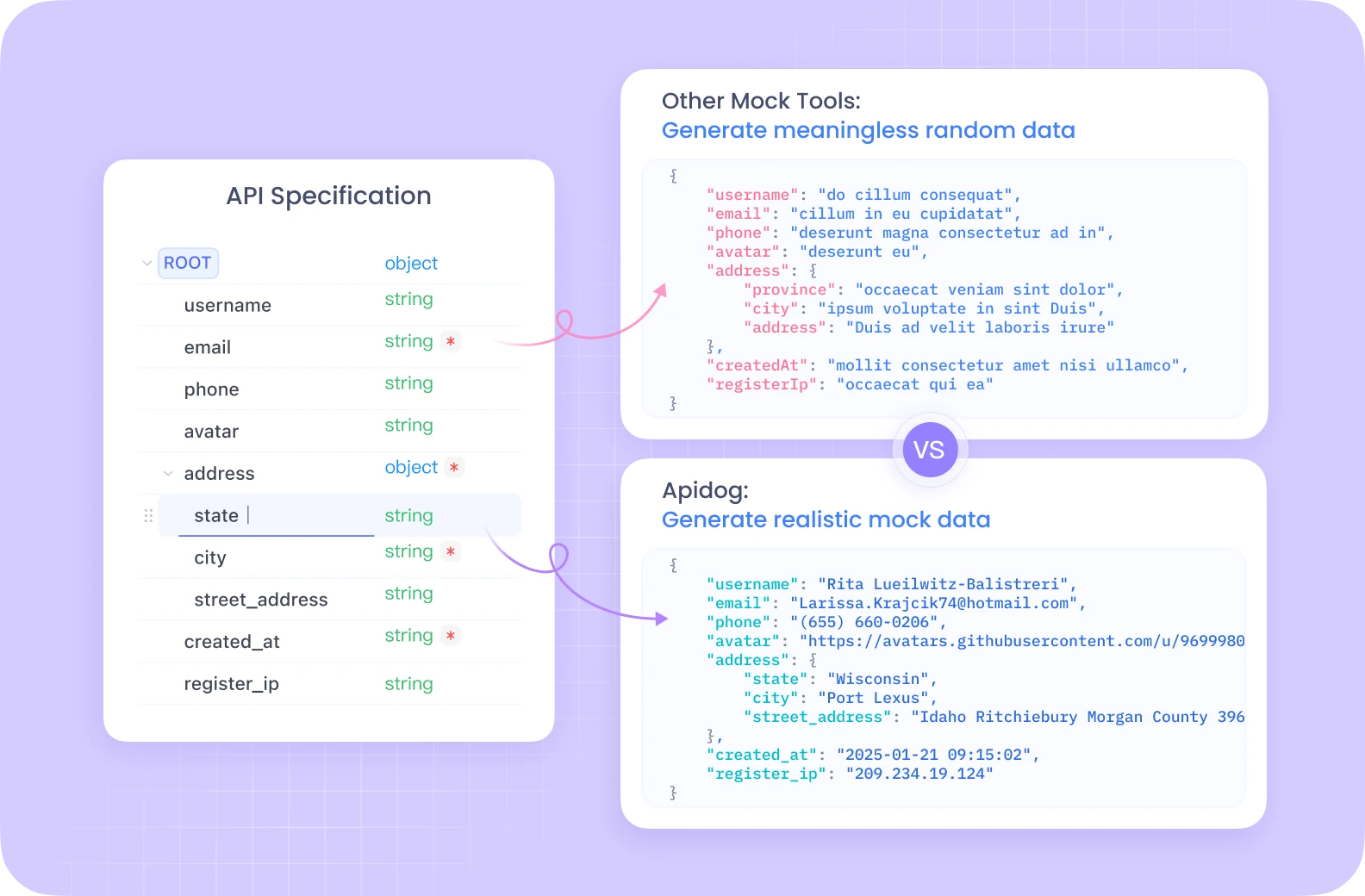
Why Choose Flutter: Flutter Advantages for Developers
Flutter has gained immense popularity in the developer community, with its GitHub repository garnering more stars than many established frameworks. There are several reasons why developers are gravitating toward Flutter:
- Single Codebase: Unlike traditional development where you need separate teams for iOS and Android, Flutter allows you to maintain just one codebase.
- Beautiful UI: Flutter comes with a rich set of customizable widgets that follow Material Design principles, making your apps visually appealing across platforms.
- Hot Reload: This feature allows developers to see changes in real-time without losing the current application state, significantly speeding up the development process.
- Native Performance: Flutter compiles to native ARM code, ensuring high performance that rivals natively developed apps.
- Simple Learning Curve: Compared to other frameworks, Flutter's architecture is relatively straightforward and easier to grasp for beginners.
Flutter Setup: Installing the Flutter Development Environment
Let's get started by setting up the Flutter development environment on macOS. The process is similar for Windows and Linux with slight variations.
Step 1: Download the Flutter SDK
Download the latest stable Flutter SDK from the official website (flutter.dev). For this tutorial, we'll use Flutter 2.0.2:
- Go to Flutter installation page
- Download the zip file (flutter_macos_2.0.2-stable.zip)
- Extract it to a location of your choice (e.g.,
~/development
)
mkdir -p ~/development
unzip ~/Downloads/flutter_macos_2.0.2-stable.zip -d ~/development
Step 2: Add Flutter to your PATH
To use Flutter commands from any terminal window, add Flutter to your PATH:
- Open or create your bash profile:
open -e ~/.bash_profile
- Add the following line to your profile:
export PATH="$PATH:~/development/flutter/bin"
- Save the file and apply the changes:
source ~/.bash_profile
Step 3: Pre-cache Flutter tools
Run the following command to download essential Flutter tools:
flutter precache
Step 4: Check Flutter installation
Verify your installation by running:
flutter doctor
This command checks your environment and displays a report of the status of your Flutter installation. It will tell you if there are any dependencies you need to install to complete the setup.
Flutter Requirements: Setting Up Platform-Specific Tools for Flutter Development
To develop Flutter apps for different platforms, you'll need to set up platform-specific tools.
For iOS Development:
- Install Xcode from the Mac App Store
- Open Xcode and accept the license agreement
- Install the iOS simulator:
open -a Simulator
For Android Development:
- Download and install Android Studio from developer.android.com/studio
- Accept Android SDK licenses:
flutter doctor --android-licenses
- Create an Android Virtual Device (AVD):
- Open Android Studio
- Go to Tools > AVD Manager
- Click "Create Virtual Device" and select a device definition (e.g., Pixel 4)
- Select a system image (preferably the latest stable Android version)
- Name your AVD and click "Finish"
- Install Flutter plugin for Android Studio:
- Go to Android Studio > Preferences > Plugins
- Search for "Flutter" and click "Install"
- Also install the "Dart" plugin when prompted
Creating Your First Flutter App: Flutter Project Setup
Now let's create a simple Flutter application to understand the basics.
Step 1: Create a new Flutter project
flutter create my_first_flutter_app
cd my_first_flutter_app
This command creates a new Flutter project with all the necessary files and folders.
Step 2: Exploring the Flutter project structure
A Flutter project contains several important directories:
- lib/: Contains the main Dart code for your application
- android/: Contains Android-specific files
- ios/: Contains iOS-specific files
- web/: Contains files for web deployment
- pubspec.yaml: Contains project dependencies and configurations
The most important file is lib/main.dart
, which is the entry point of your Flutter application.
Step 3: Run your Flutter app
You can run your Flutter application on different platforms:
For iOS simulator:
flutter run
For Android emulator:
flutter run
(Make sure your emulator is running)
For web:
flutter run -d chrome
Understanding Flutter Basics: Flutter Architecture and Widgets
Flutter's architecture is based on the concept of widgets. Everything in Flutter is a widget!
Widget Types in Flutter
Flutter has two main types of widgets:
- StatelessWidget: Used for UI parts that don't change (immutable)
- StatefulWidget: Used for UI parts that can change based on user interaction or data changes
Let's understand the default app created by Flutter:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('You have pushed the button this many times:'),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
Hot Reload: Flutter's Powerful Development Feature
One of Flutter's most powerful features is Hot Reload, which allows you to see changes in your code almost instantly without losing the current state of your app.
To use Hot Reload:
- Make changes to your code
- Save the file (Ctrl+S or Cmd+S)
- Flutter automatically applies your changes while the app is running
You can also trigger Hot Reload manually by pressing "r" in the terminal where your Flutter app is running.
Customizing Your Flutter App: Modifying Flutter UI
Let's modify our counter app to understand how Flutter UI works:
- Open
lib/main.dart
- Change the theme color from blue to green:
theme: ThemeData(
primarySwatch: Colors.green,
),
- Change the counter text:
Text('You clicked the button this many times:'),
- Save the file and watch the changes apply instantly via Hot Reload
Flutter Resources: Where to Learn More About Flutter Development
Flutter has excellent documentation and a supportive community. Here are some resources to help you continue your Flutter journey:
- Flutter Official Documentation: Comprehensive guides and API references
- Flutter Cookbook: Recipes for common Flutter patterns
- Flutter Tutorial: Step-by-step guide for beginners
- Dart Language Tour: Learn the Dart programming language
- Flutter YouTube Channel: Video tutorials and updates
Flutter Community: Engaging with the Flutter Ecosystem
Getting involved with the Flutter community can greatly accelerate your learning:
- Join the Flutter Community on GitHub
- Participate in Flutter's Discord server
- Follow Flutter on Twitter
- Browse Flutter packages on pub.dev
Flutter Next Steps: Advancing Your Flutter Development Skills
Now that you have set up your development environment and created your first Flutter app, here are some next steps to further improve your skills:
- Learn Dart in depth: Understanding Dart will help you write better Flutter code
- Explore state management: Learn about providers, Bloc, Redux, or GetX
- Work with Flutter packages: Integrate third-party packages to add functionality
- Practice UI development: Try recreating complex UIs from popular apps
- Build a complete project: Apply your knowledge by building a real-world app
Conclusion: Your Flutter Journey Begins
Flutter is a powerful framework that's changing how mobile, web, and desktop apps are developed. With its hot reload feature, expressive UI, and native performance, it offers an excellent developer experience.
As you continue your Flutter journey, remember that practice is key. Start with small projects and gradually increase complexity as you become more comfortable with the framework.
Flutter's documentation is comprehensive and regularly updated, making it an excellent reference as you progress from beginner to advanced levels. The vibrant community also means that help is always available when you encounter challenges.
Happy Flutter development!