Flutter Version Management (FVM) is an essential tool for Flutter developers that streamlines the process of managing multiple Flutter SDK versions across different projects. As Flutter evolves rapidly with frequent updates, having a robust version management system becomes crucial for maintaining project stability. This comprehensive guide will walk you through everything you need to know about FVM, from installation to advanced usage scenarios.
While developing Flutter applications, you'll often need to test and interact with APIs. Apidog is an excellent alternative to Postman that offers a comprehensive set of features for API development and testing.
Why Consider Apidog?
Apidog provides a streamlined experience with features that make API testing more efficient:
- All-in-one Platform: API documentation, design, debugging, automated testing, and mocking in a single tool
- Intuitive Interface: User-friendly design with powerful capabilities
- Built-in Mock Server: Create mock APIs without writing any code
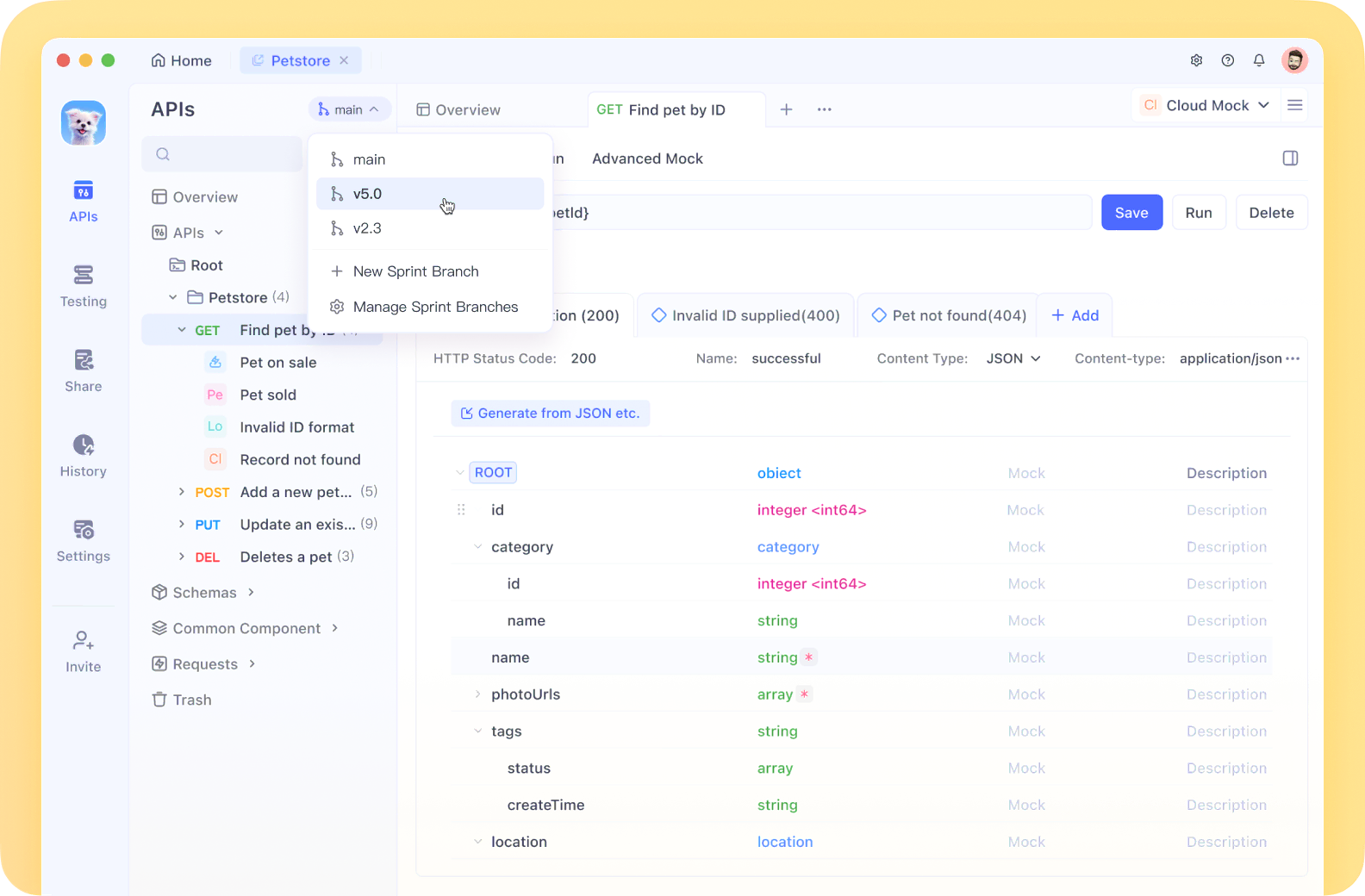
4. Collaboration Features: Real-time team collaboration with synchronized updates
5. OpenAPI Support: Import and export OpenAPI specifications seamlessly
6. Auto-generated Documentation: Create beautiful, interactive API docs automatically
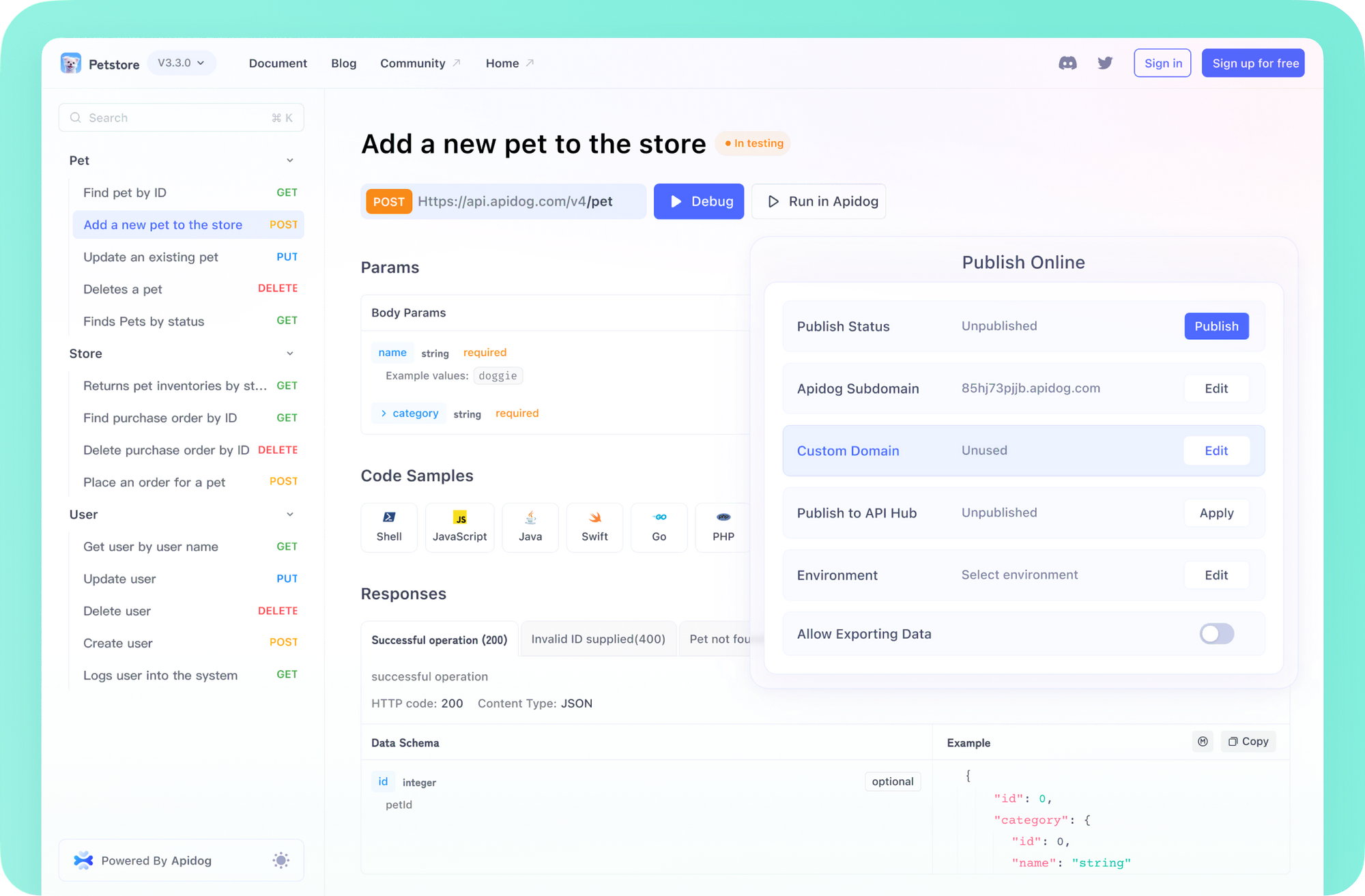
7. Advanced Testing Capability: Create complex test scenarios with powerful scripting
Why You Need Flutter Version Management
Before diving into the technical details, let's understand why FVM is important for Flutter developers:
- Project-Specific Flutter Versions: Different projects may require different Flutter SDK versions based on package compatibility or client requirements.
- Team Consistency: Ensures all team members use the same Flutter version, eliminating "works on my machine" issues.
- Easy SDK Switching: Quickly switch between stable, beta, or dev channels without time-consuming reinstallations.
- Multiple Project Support: Work on various projects simultaneously without version conflicts.
- Testing New Releases: Safely experiment with new Flutter versions without affecting your stable production projects.
Installation
Let's start by installing FVM on your system. The installation process differs slightly depending on your operating system:
For macOS:
Using Homebrew:
brew tap leoafarias/fvm
brew install fvm
Using install script:
curl -fsSL https://fvm.app/install.sh | bash
For Windows:
Using Chocolatey:
choco install fvm
Or download the standalone package from the FVM GitHub repository.
For Linux:
Using the install script:
curl -fsSL https://fvm.app/install.sh | bash
Global Activation
After installing FVM, activate it globally:
dart pub global activate fvm
Basic FVM Commands and Usage
Now that you have FVM installed, let's explore the essential commands for managing Flutter SDK versions.
Installing Flutter Versions
To install a specific Flutter version:
fvm install 3.16.0
You can also install a specific channel:
fvm install stable
fvm install beta
fvm install dev
For advanced users, you can even install a specific commit by using the git commit hash:
fvm install fa345b1 # Short hash
fvm install 476ad8a917e64e345f05e4147e573e2a42b379f9 # Long hash
Setting a Flutter Version for Your Project
To set a specific Flutter version for your project, navigate to your project directory and run:
fvm use 3.16.0
This command creates a .fvm
folder in your project and sets up a symbolic link to the specified Flutter SDK.
To use the latest version from a specific channel:
fvm use stable
If you want to pin the channel to its current version (preventing automatic updates):
fvm use stable --pin
Listing Installed Versions
To see all the Flutter versions you've installed via FVM:
fvm list
Alternatively, you can use the shorthand:
fvm ls
Viewing Available Flutter Releases
To view all available Flutter SDK releases:
fvm releases
To filter releases by channel:
fvm releases --channel beta
Removing a Flutter Version
When you no longer need a specific Flutter version:
fvm remove 3.16.0
Setting a Global Flutter Version
To set a global Flutter version to use across your system:
fvm global 3.16.0
To unlink the global version:
fvm global --unlink
Project Configuration
After you've set up FVM for your project, you'll need to configure your development environment to use the Flutter SDK managed by FVM.
Updating .gitignore
FVM creates a symbolic link at .fvm/flutter_sdk
that points to the cache of your selected Flutter version. Add this to your .gitignore
:
.fvm/flutter_sdk
IDE Configurations
VS Code
Create or update .vscode/settings.json
in your project with the following:
{
"dart.flutterSdkPath": ".fvm/flutter_sdk",
// Remove .fvm files from search
"search.exclude": {
"**/.fvm": true
},
// Remove from file watching
"files.watcherExclude": {
"**/.fvm": true
}
}
Android Studio
- Go to Languages & Frameworks > Flutter
- Change the Flutter SDK path to the absolute path of your FVM symbolic link (e.g.,
/absolute-project-path/.fvm/flutter_sdk
) - Apply the changes and restart Android Studio
To ignore the Flutter SDK root directory within Android Studio, add this to .idea/workspace.xml
:
<component name="VcsManagerConfiguration">
<ignored-roots>
<path value="$PROJECT_DIR$/.fvm/flutter_sdk" />
</ignored-roots>
</component>
XCode
If you're working on iOS, open Xcode, go to Build phases, and add this in Run script:
export FLUTTER_ROOT="$PROJECT_DIR/../.fvm/flutter_sdk"
Advanced FVM Features
Project Flavors
FVM supports project flavors, allowing different parts of your application to use different Flutter SDK versions:
fvm use 3.16.0 --flavor production
fvm use 3.10.0 --flavor development
To switch between flavors:
fvm use production
fvm use development
To run a Flutter command with a specific flavor:
fvm flavor production flutter build
Executing Flutter Commands with Specific Versions
The spawn
command allows you to run Flutter commands with a specific SDK version:
fvm spawn 3.16.0 flutter build
fvm spawn 3.10.0 flutter test
Running Commands with Project's Flutter Version
The exec
command executes commands or scripts using the project's configured Flutter version:
fvm exec melos bootstrap
fvm exec path/to/script.sh
Working with FVM in CI/CD Environments
For continuous integration, add these steps to your workflow:
- Install FVM
- Run
fvm install
- Use
fvm flutter <command>
for running Flutter commands
Example for GitHub Actions:
steps:
- uses: actions/checkout@v3
- name: Install FVM
run: |
dart pub global activate fvm
fvm install
- name: Build and Test
run: |
fvm flutter pub get
fvm flutter test
Best Practices for Using FVM
- Always use FVM for Flutter commands - Run Flutter commands through FVM (e.g.,
fvm flutter pub get
) to ensure consistency. - Include FVM version in project documentation - Document the required Flutter version in your README to help new team members.
- Lock Flutter version in CI/CD - Ensure your continuous integration pipeline uses the same Flutter version as your development environment.
- Consider using
--pin
for stability - When using a channel like 'stable', consider pinning it to avoid automatic updates. - Regular cache cleanup - Periodically remove unused Flutter versions with
fvm remove
to save disk space.
Troubleshooting Common Issues
FVM Version Not Recognized
If your IDE isn't recognizing the Flutter version set by FVM:
- Ensure the Flutter SDK path is correctly set in your IDE settings
- Restart your IDE after configuration changes
- Verify the symbolic link is correctly created with
ls -la .fvm/flutter_sdk
Permission Issues
If you encounter permission errors:
- On Unix-based systems, try
sudo fvm <command>
- Check folder permissions for the FVM cache directory
Path Issues
If Flutter commands aren't found after using FVM:
- Ensure the Flutter SDK path is in your system PATH
- Use absolute paths to the Flutter SDK in your scripts
Conclusion
Flutter Version Management (FVM) is an indispensable tool for modern Flutter development. By allowing precise control over Flutter SDK versions across different projects, it eliminates compatibility issues and ensures consistency across development teams.
Starting with the basics of installation and progressing to advanced features like project flavors, this guide has covered the essential aspects of using FVM effectively. By incorporating FVM into your Flutter development workflow, you can enhance productivity, maintain project stability, and seamlessly collaborate with team members.
Remember to always run Flutter commands through FVM to ensure version consistency and avoid unexpected behaviors. With proper FVM usage, you can confidently work on multiple Flutter projects with different SDK requirements and easily experiment with new Flutter versions without affecting your production code.
Happy Flutter coding with FVM!