Building RESTful APIs is a fundamental skill for modern web development, enabling seamless communication between clients and servers. Flask-RESTX, an extension of the popular Flask framework, simplifies this process by providing tools to create robust APIs efficiently. In this tutorial, we'll explore how to build a RESTful API using Flask-RESTX and demonstrate how to test it with Apidog, an integrated platform for API design, debugging, documentation, mocking, and testing.
1. Introduction to RESTful APIs
REST (Representational State Transfer) is an architectural style that uses standard HTTP methods to interact with resources. APIs adhering to REST principles are termed RESTful APIs. They provide a predictable and uniform interface for client-server interactions, making web services more accessible and maintainable.
2. Setting Up Your Development Environment
Before we start building our API, let's set up our development environment.
Prerequisites
Python 3.7 or higher: Ensure Python is installed on your system. Verify by running:
python --version
Virtual Environment: It's good practice to create a virtual environment for your project to manage dependencies.
python -m venv venv
source venv/bin/activate # On Windows: venv\Scripts\activate
Installing Flask and Flask-RESTX
With the virtual environment activated, install Flask and Flask-RESTX using pip:
pip install flask flask-restx
This command installs both Flask and Flask-RESTX, allowing us to build and manage our API endpoints effectively.
3. Installing and Configuring Flask-RESTX
Flask-RESTX is an extension that adds support for quickly building REST APIs with Flask. It encourages best practices with minimal setup.
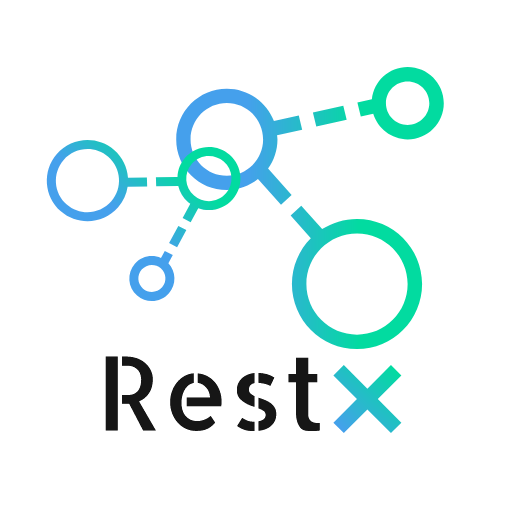
Installation
If you haven't installed Flask-RESTX yet, you can do so using pip:
pip install flask-restx
Configuration
Create a new Python file, e.g., app.py
, and set up the basic configuration:
from flask import Flask
from flask_restx import Api
app = Flask(__name__)
api = Api(app, version='1.0', title='Sample API',
description='A sample API using Flask-RESTX')
if __name__ == '__main__':
app.run(debug=True)
This script initializes a Flask application and wraps it with a Flask-RESTX API instance, providing a foundation for building endpoints.
4. Creating Your First API Endpoint
Let's create a simple endpoint to understand how Flask-RESTX works.
Defining a Namespace
Namespaces in Flask-RESTX help organize your API and prevent endpoint name collisions.
from flask_restx import Namespace, Resource
ns = Namespace('hello', description='Hello World operations')
Creating a Resource
Resources in Flask-RESTX correspond to endpoints and define how HTTP methods are handled.
@ns.route('/')
class HelloWorld(Resource):
def get(self):
return {'message': 'Hello, World!'}
Registering the Namespace
Finally, register the namespace with the API:
api.add_namespace(ns)
Now, when you run your application and navigate to http://localhost:5000/hello/
, you should see:
{
"message": "Hello, World!"
}
5. Structuring Your Flask-RESTX Application
As your application grows, it's essential to maintain a clean and organized structure.
Recommended Project Layout
project/
├── app/
│ ├── __init__.py
│ ├── controllers/
│ │ ├── __init__.py
│ │ └── hello_controller.py
│ ├── models/
│ │ ├── __init__.py
│ │ └── hello_model.py
│ └── services/
│ ├── __init__.py
│ └── hello_service.py
├── run.py
└── requirements.txt
Initializing the Application
In app/__init__.py
:
from flask import Flask
from flask_restx import Api
def create_app():
app = Flask(__name__)
api = Api(app, version='1.0', title='Sample API',
description='A sample API using Flask-RESTX')
from .controllers.hello_controller import ns as hello_namespace
api.add_namespace(hello_namespace)
return app
In run.py
:
from app import create_app
app = create_app()
if __name__ == '__main__':
app.run(debug=True)
This modular approach separates concerns, making the application more maintainable and scalable.
6. Implementing CRUD Operations
CRUD operations—Create, Read, Update, Delete—are fundamental for API development. Let's implement these for a simple Item
resource.
Defining the Model
In app/models/item_model.py
:
from flask_restx import fields
def get_item_model(api):
return api.model('Item', {
'id': fields.Integer(readOnly=True, description='The unique identifier of an item'),
'name': fields.String(required=True, description='Item name'),
'price': fields.Float(required=True, description='Item price')
})
Implementing the Resource
In app/controllers/item_controller.py
:
from flask_restx import Namespace, Resource, reqparse
from app.models.item_model import get_item_model
ns = Namespace('items', description='Item operations')
item_model = get_item_model(ns)
items = []
item_id_counter = 1
parser = reqparse.RequestParser()
parser.add_argument('name', type=str, required=True, help='Name of the item')
parser.add_argument('price', type=float, required=True, help='Price of the item')
@ns.route('/')
class ItemList(Resource):
@ns.marshal_list_with(item_model)
def get(self):
return items
@ns.expect(item_model)
@ns.marshal_with(item_model, code=201)
def post(self):
global item_id_counter
args = parser.parse_args()
item = {
'id': item_id_counter,
'name': args['name'],
'price': args['price']
}
items.append(item)
item_id_counter += 1
return item, 201
@ns.route('/<int:id>')
@ns.response(404, 'Item not found')
@ns.param('id', 'The item identifier')
class Item(Resource):
@ns.marshal_with(item_model)
def get(self, id):
item = next((item for item in items if item['id'] == id), None)
if item is not None:
return item
ns.abort(404, message="Item not found")
@ns.expect(item_model)
@ns.marshal_with(item_model)
def put(self, id):
item = next((item for item in items if item['id'] == id), None)
if item is not None:
args = parser.parse_args()
item.update({
'name': args['name'],
'price': args['price']
})
return item
ns.abort(404, message="Item not found")
@ns.response(204, 'Item deleted')
def delete(self, id):
global items
item = next((item for item in items if item['id'] == id), None)
if item is not None:
items = [item for item in items if item['id'] != id]
return '', 204
ns.abort(404, message="Item not found")
In this implementation:
- GET /items/: Retrieves the list of items.
- POST /items/: Adds a new item.
- GET /items/{id}: Retrieves a specific item by ID.
- PUT /items/{id}: Updates an existing item by ID.
- DELETE /items/{id}: Deletes an item by ID.
This setup provides a complete CRUD interface for managing items in your API.
7. Validating and Serializing Data with Flask-RESTX
Data validation and serialization are crucial for ensuring the integrity and consistency of the data your API processes.Flask-RESTX offers decorators and fields to facilitate this.
Using Models for Validation
Define a model to specify the expected input and output formats.
from flask_restx import fields
item_model = api.model('Item', {
'id': fields.Integer(readOnly=True, description='The unique identifier of an item'),
'name': fields.String(required=True, description='Item name'),
'price': fields.Float(required=True, description='Item price')
})
By decorating your resource methods with @ns.expect(item_model)
, Flask-RESTX will automatically validate incoming JSON data against this model.
Serializing Responses
Use the @ns.marshal_with
decorator to format the output according to the model.
@ns.marshal_with(item_model)
def get(self, id):
# Retrieve and return the item
This ensures that the response data adheres to the specified structure, promoting consistency across your API.
8. Testing RESTful APIs with Apidog
Testing is a vital part of API development, ensuring that your endpoints function as intended. Apidog is a comprehensive tool that streamlines API testing, design, and documentation.
Setting Up Apidog
Download and Install: Download Apidog for free and follow the installation instructions for your operating system.
Create a New Project: Launch Apidog and create a new project for your API.
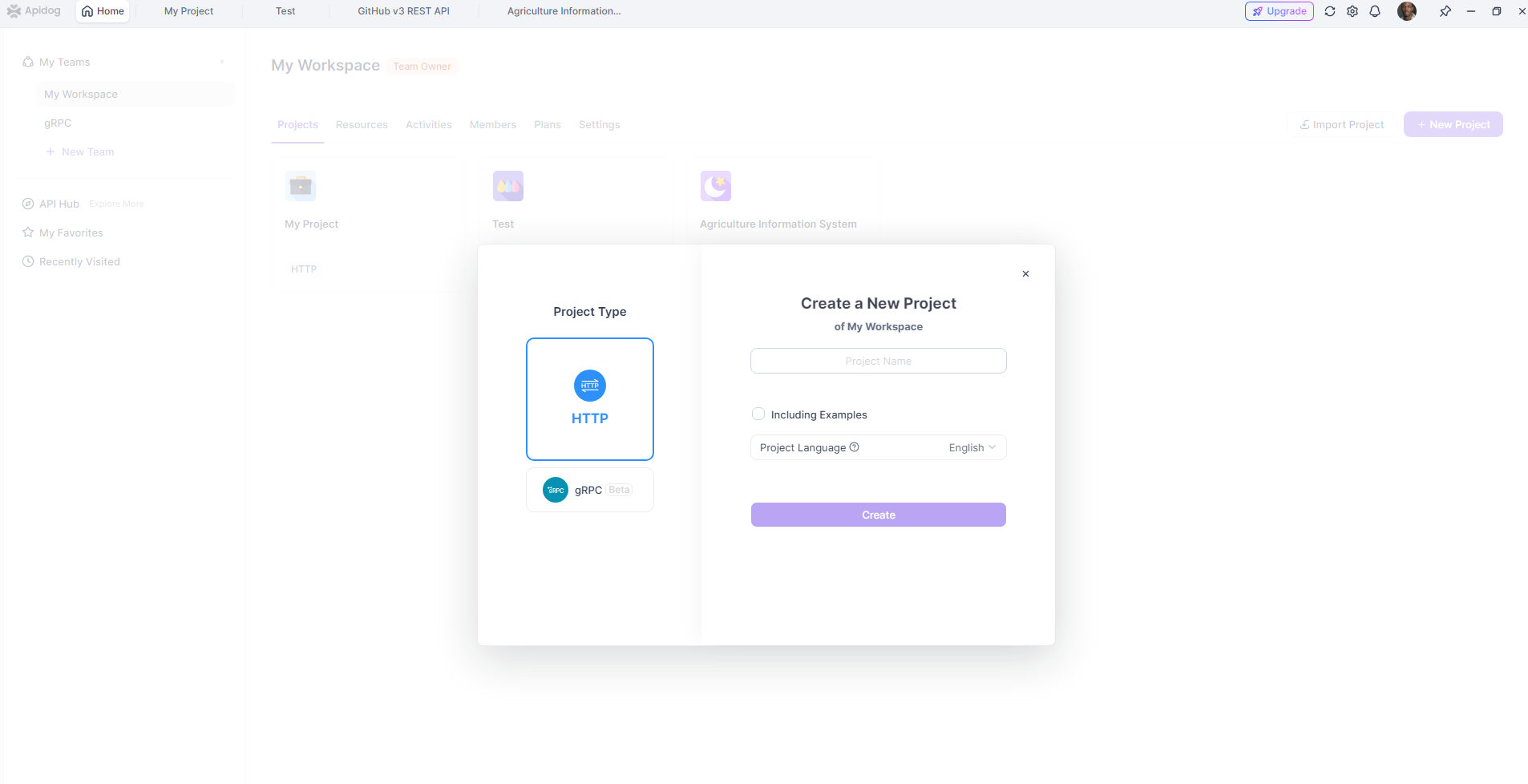
Importing Your API Specification
If you've documented your API using OpenAPI/Swagger, you can import the specification into Apidog:
Import Specification: In your Apidog project, select the option to import an API specification and upload your OpenAPI file.
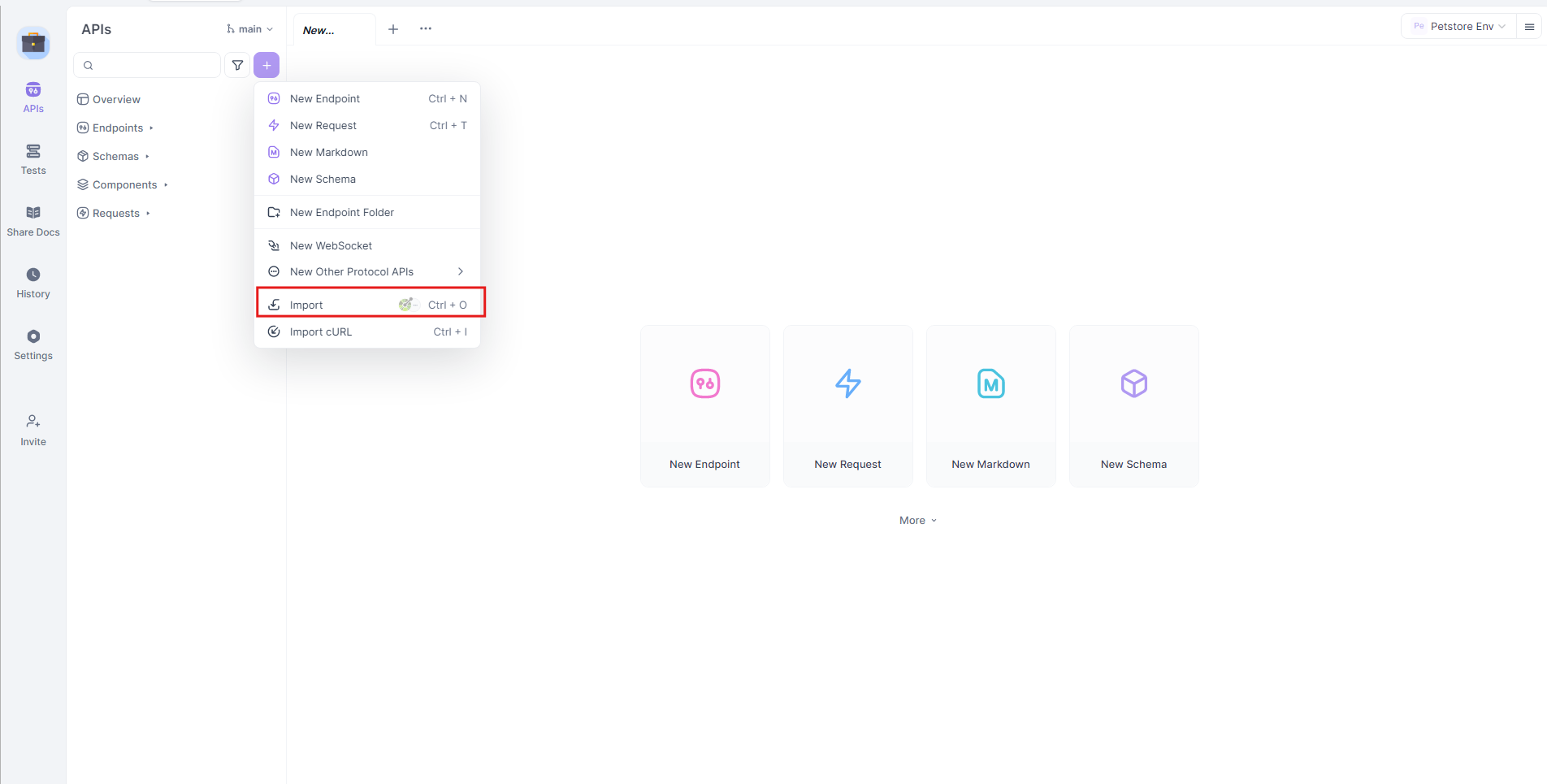
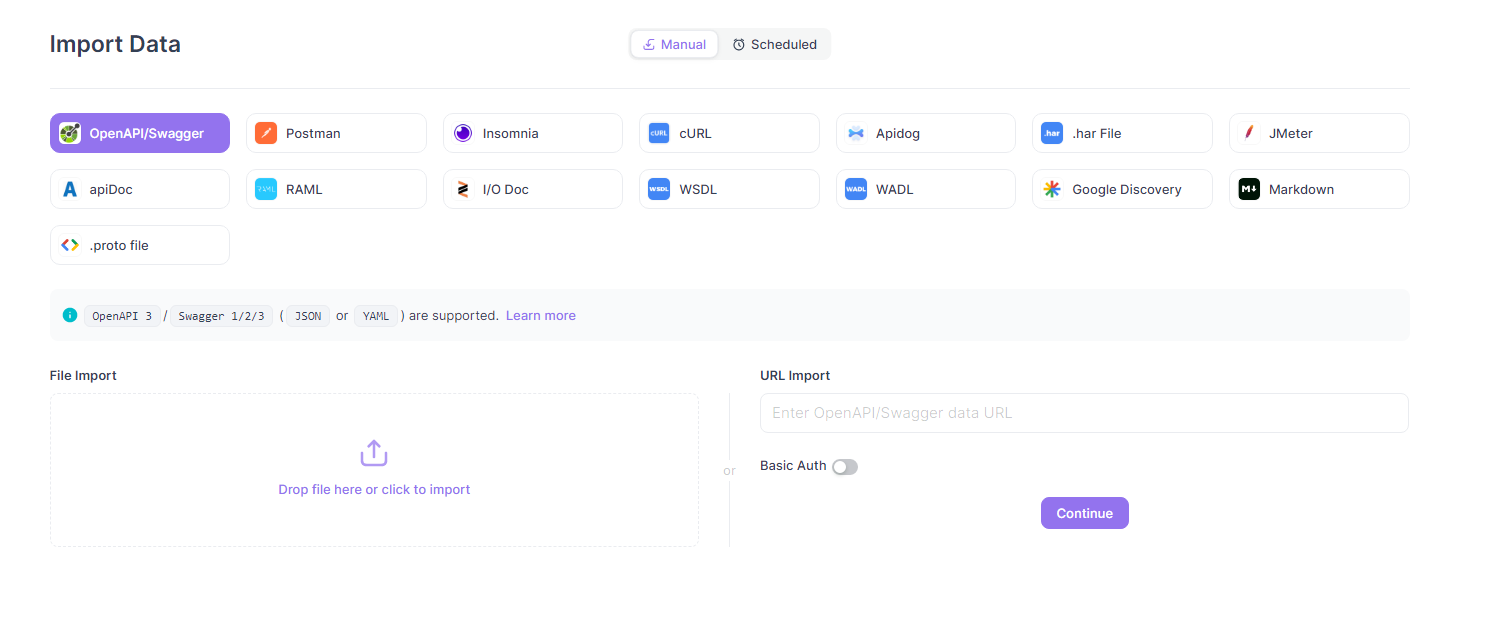
Explore Endpoints: Apidog will parse the file and display your API endpoints, parameters, and models.
You can also design APIs from scratch in Apidog.
Testing Endpoints
Select an Endpoint: Choose the endpoint you wish to test from the list.
Configure the Request:
- Method: Ensure the correct HTTP method (GET, POST, PUT, DELETE) is selected.
- Parameters: Input any required query parameters or path variables.
- Headers: Set necessary headers, such as
Content-Type: application/json
. - Body: For POST and PUT requests, provide the JSON payload.
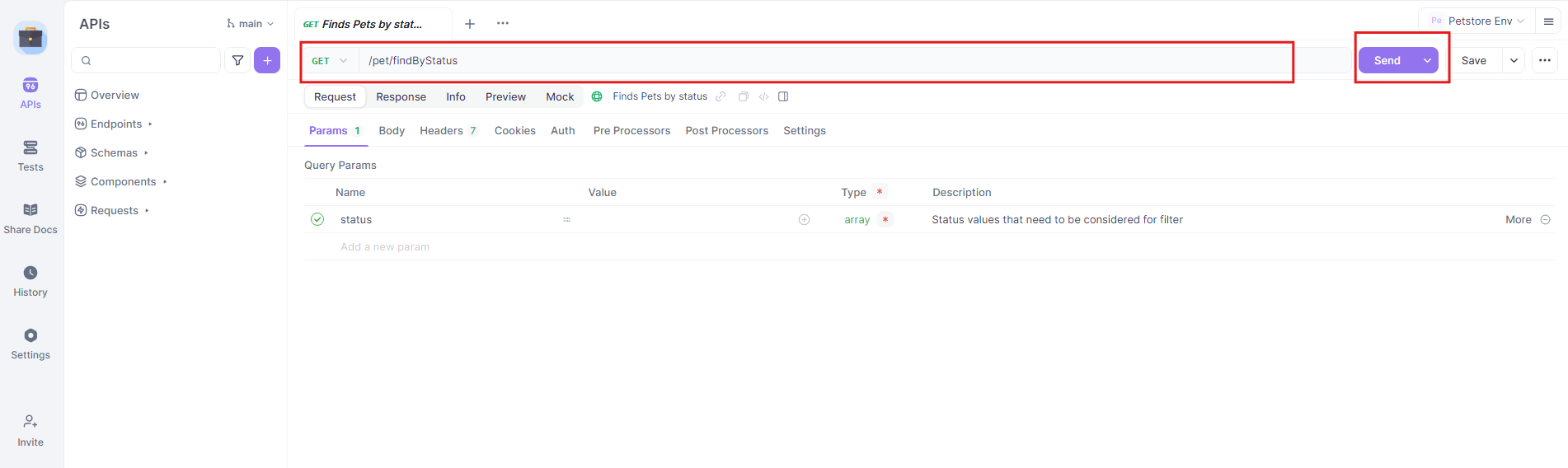
Send the Request: Click the Send
button to execute the request.
Review the Response:
(Pro tip: Apidog validates the API response automatically.)
- Status Code: Verify that the response status code matches expectations (e.g., 200 for success, 201 for creation).
- Response Body: Check that the returned data is correct and properly formatted.
- Headers: Inspect response headers for additional information.
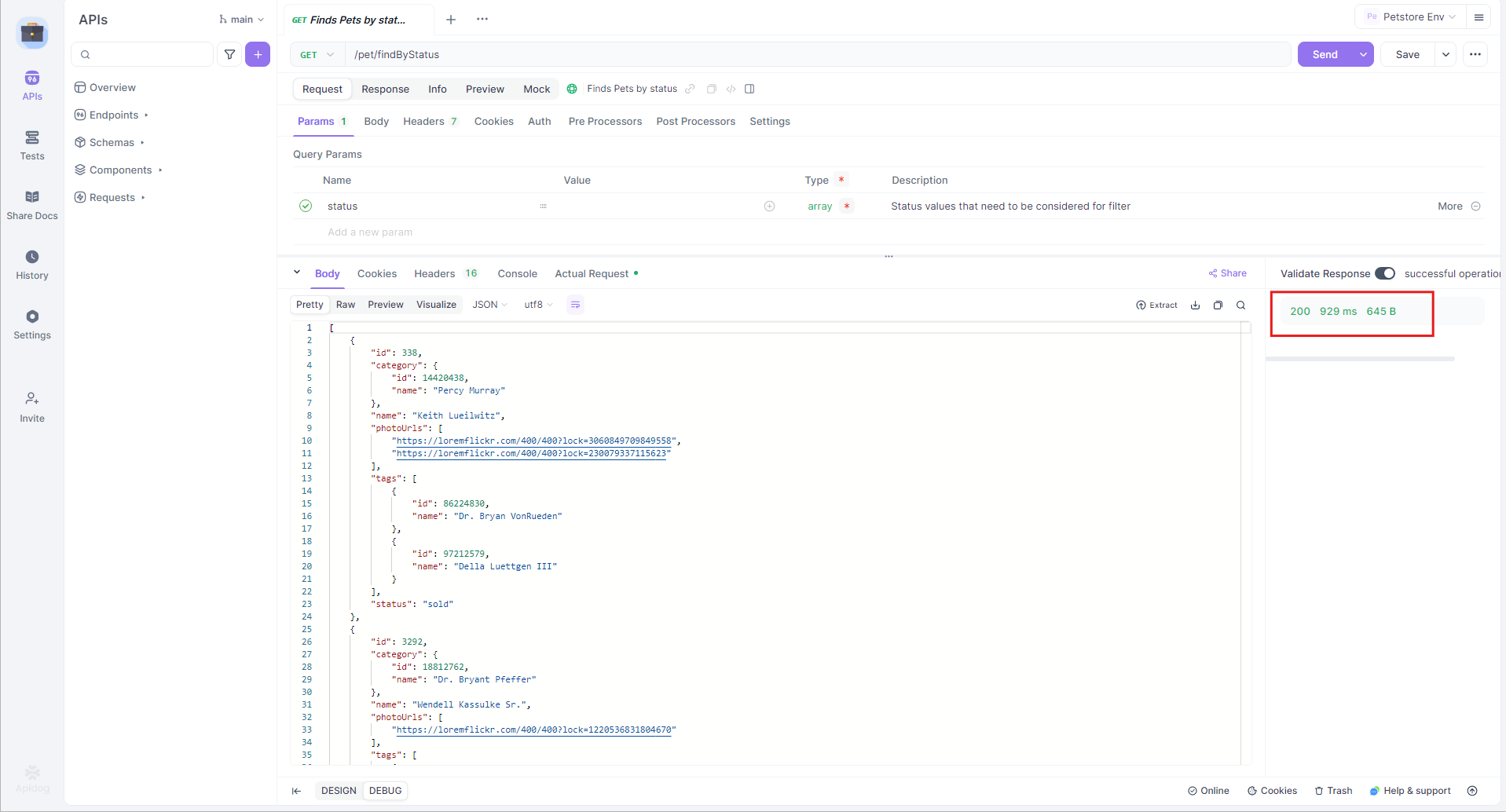
9. Generating API Documentation with Flask-RESTX
Comprehensive documentation is essential for any API, facilitating ease of use and integration for developers. Flask-RESTX provides built-in support for generating interactive API documentation.
Generating comprehensive and user-friendly API documentation is crucial for developers and users interacting with your API. Flask-RESTX simplifies this process by automatically generating Swagger UI documentation, providing an interactive interface to explore and test your API endpoints.
Automatic Swagger Documentation
By default, Flask-RESTX generates Swagger documentation accessible at your API's root URL. This feature offers an immediate overview of your API's structure and available endpoints without additional configuration.
Customizing Documentation with Decorators
Flask-RESTX provides several decorators to enhance and customize your API documentation:
@api.doc()
: Adds additional information to your resources or methods. For example, to document parameters:
@api.route('/my-resource/<id>')
@api.doc(params={'id': 'An ID'})
class MyResource(Resource):
def get(self, id):
return {}
@api.response()
: Documents the expected responses, including status codes and descriptions:
@api.route('/my-resource/<id>')
class MyResource(Resource):
@api.response(403, 'Not Authorized')
def post(self, id):
api.abort(403)
@api.marshal_with()
: Specifies the output model for a response, ensuring consistent data formatting:
@api.route('/item/<int:id>')
class ItemResource(Resource):
@api.marshal_with(item_model)
def get(self, id):
# Retrieve and return the item
@api.expect()
: Defines the expected input model for requests, facilitating validation and documentation:
@api.route('/item')
class ItemResource(Resource):
@api.expect(item_model)
def post(self):
# Handle the post request
Organizing with Namespaces
Namespaces in Flask-RESTX help group-related resources, enhancing the organization of your API and its documentation.
from flask_restx import Namespace, Resource
ns = Namespace('items', description='Item operations')
@ns.route('/<int:id>')
class ItemResource(Resource):
def get(self, id):
# Retrieve and return the item
By registering the namespace with the API, all associated routes and documentation are appropriately grouped:
api.add_namespace(ns)
Accessing the Swagger UI
Once your Flask-RESTX application is running, you can access the automatically generated Swagger UI by navigating to the root URL of your API (e.g., http://localhost:5000/
).This interface provides an interactive exploration of your API, displaying available endpoints, expected inputs, and potential responses.
By leveraging these features, you ensure that your API is well-documented, user-friendly, and easily understandable for developers and consumers alike..
10. Enhancing API Documentation with Apidog
While Flask-RESTX provides basic documentation, Apidog offers advanced features for API documentation, including customization, automated code generation, and real-time testing.
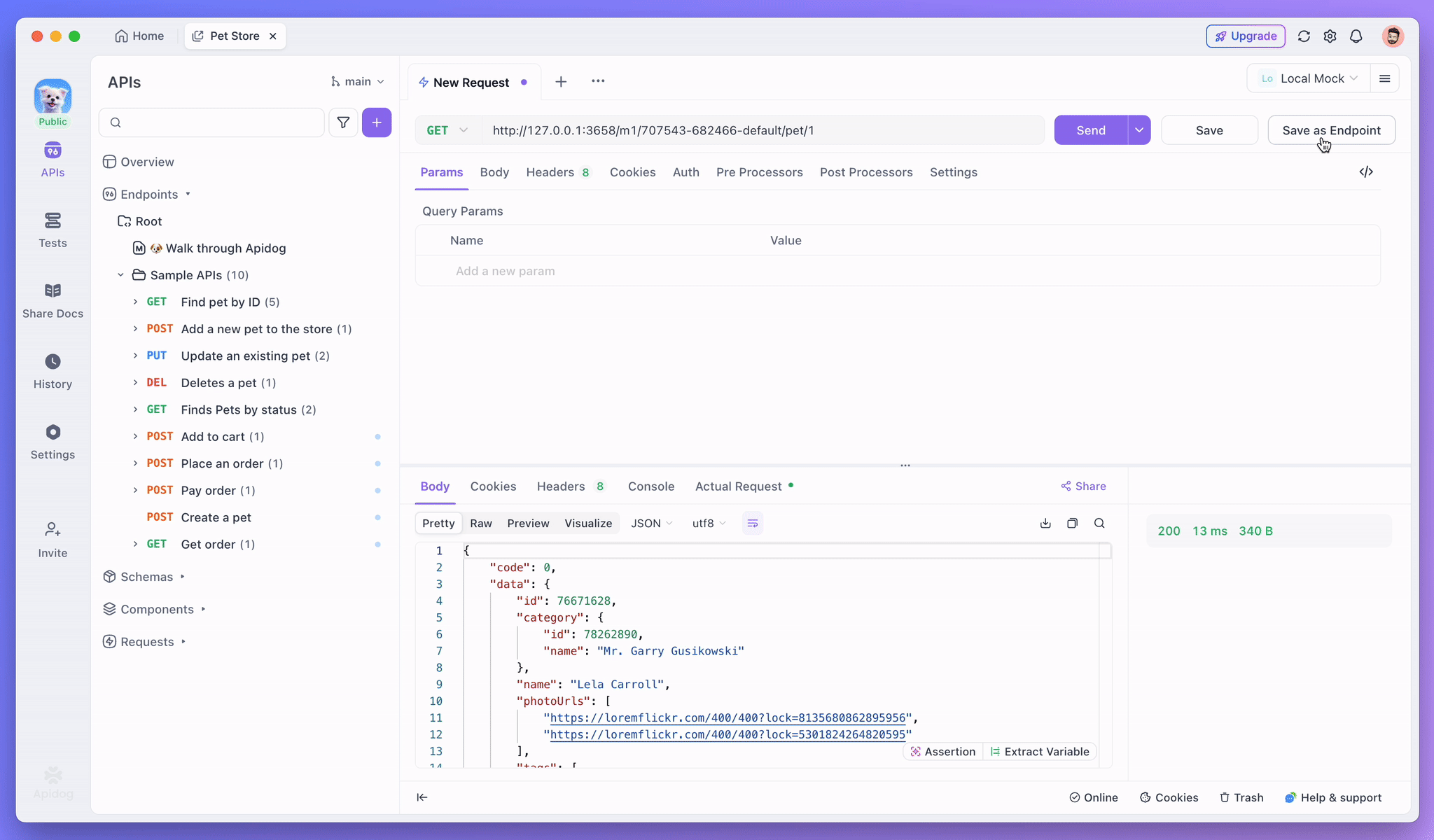
Use Apidog's visual editor to define your API endpoints, request parameters, and response schemas. With a single click, generate comprehensive and interactive API documentation.
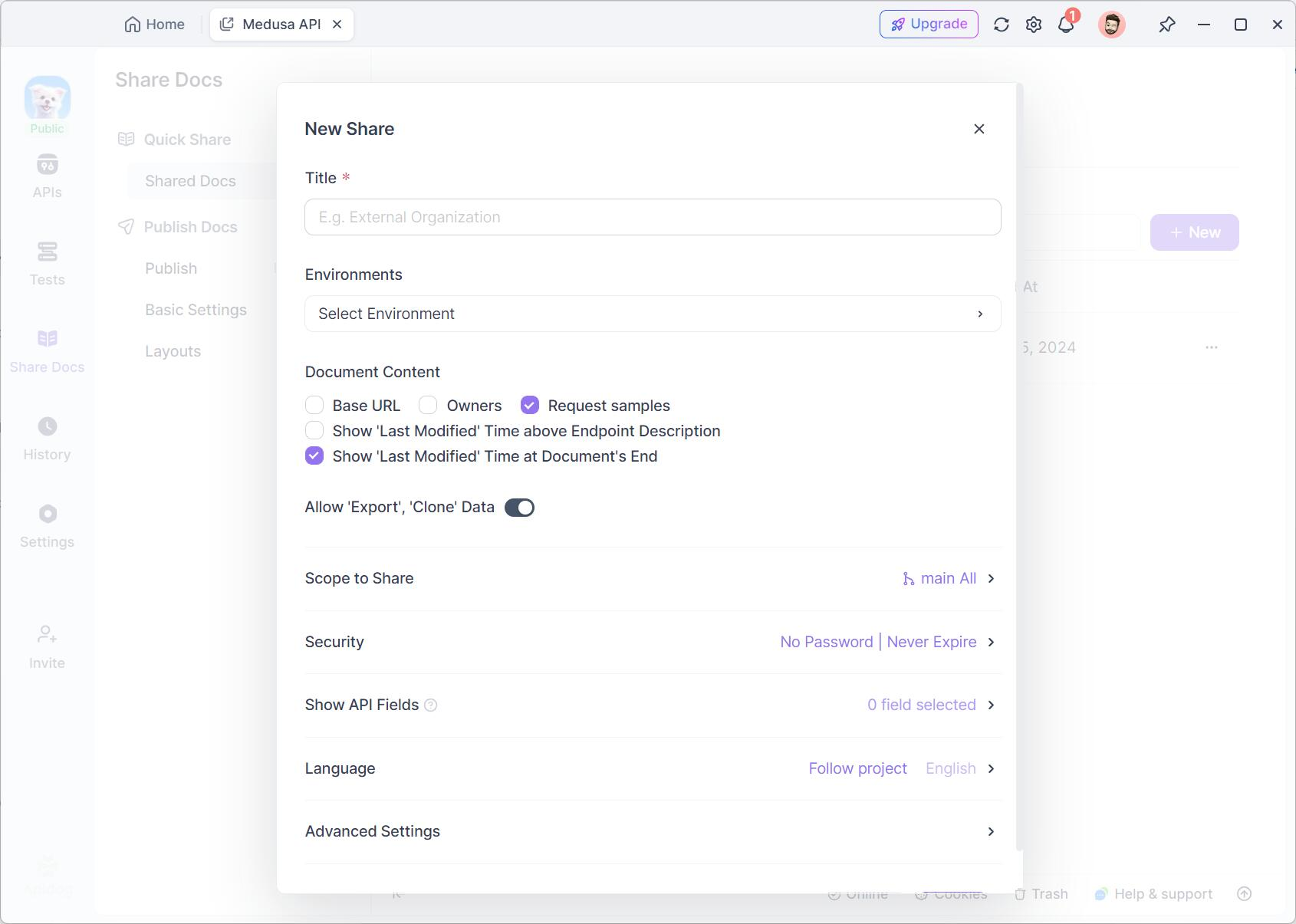
Share your documentation with team members or external partners, allowing them to test API endpoints directly within the documentation.
By integrating Flask-RESTX with Apidog, you can create robust APIs with professional-grade documentation, enhancing both development efficiency and user experience.
Conclusion
In this comprehensive guide, we've explored the process of building RESTful APIs using Flask-RESTX, a powerful extension for Flask that streamlines API development. We've covered essential topics such as setting up your development environment, creating and managing API endpoints, validating and serializing data, and generating interactive API documentation.
Additionally, we've introduced Apidog, a versatile tool that enhances your API development workflow by offering features like automated testing, performance testing, and collaborative documentation. By integrating Apidog into your development process, you can ensure your APIs are robust, efficient, and well-documented.