Imagine having the ability to extract data from any website and gather insights at scale—all with just a few lines of code. Sounds like magic, right? Well, Firecrawl makes this possible.
In this beginner’s guide, I’ll walk you through everything you need to know about Firecrawl, from installation to advanced data extraction techniques. Whether you’re a developer, data analyst, or just curious about web scraping, this tutorial will help you get started with Firecrawl and integrate it into your workflows.

What is Firecrawl?
Firecrawl is an innovative web scraping and crawling engine that converts website content into formats like markdown, HTML, and structured data. This makes It ideal for Large Language Models (LLMs) and AI applications. With Firecrawl, you can efficiently gather both structured and unstructured data from websites, simplifying your data analysis workflow.
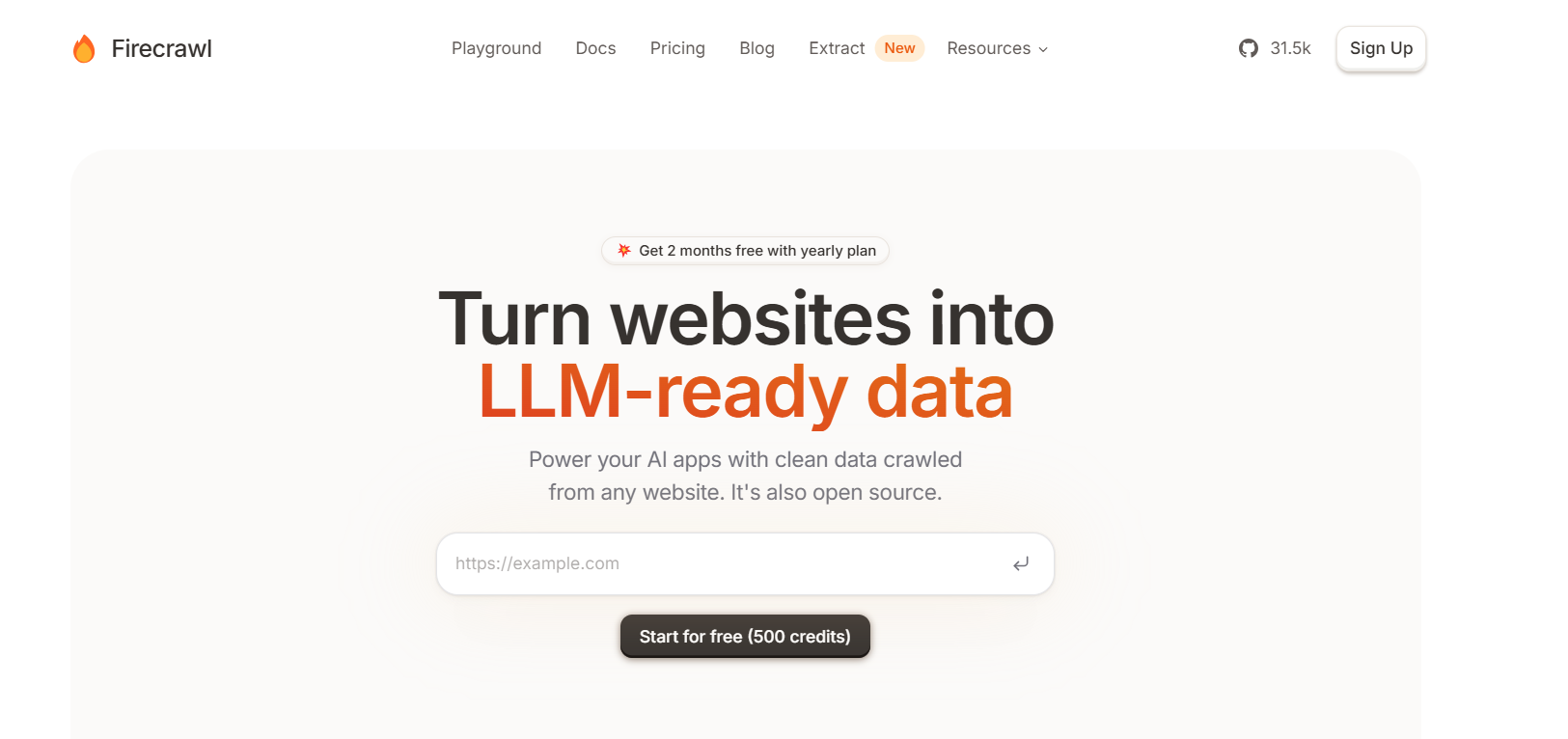
Key Features of Firecrawl
Crawl: Comprehensive Web Crawling
Firecrawl's /crawl
endpoint allows you to recursively traverse a website, extracting content from all sub-pages. This feature is perfect for discovering and organizing large amounts of web data, converting it into LLM-ready formats.
Scrape: Targeted Data Extraction
Use the Scrape feature to extract specific data from a single URL. Firecrawl can deliver content in various formats, including markdown, structured data, screenshots, and HTML. This is particularly useful for extracting specific information from known URLs.
Map: Rapid Site Mapping
The Map feature quickly retrieves all URLs associated with a given website, providing a comprehensive overview of its structure. This is invaluable for content discovery and organization.
Extract: Transforming Unstructured Data into Structured Format
The /extract
endpoint is Firecrawl’s AI-powered feature that simplifies the process of collecting structured data from websites. It handles the heavy lifting of crawling, parsing, and organizing the data into a structured format.
Getting Started with Firecrawl
Step 1: Sign Up and Get Your API Key
Visit Firecrawl's oficial website and sign up for an account. Once logged in, navigate to your dashboard to find your API key.
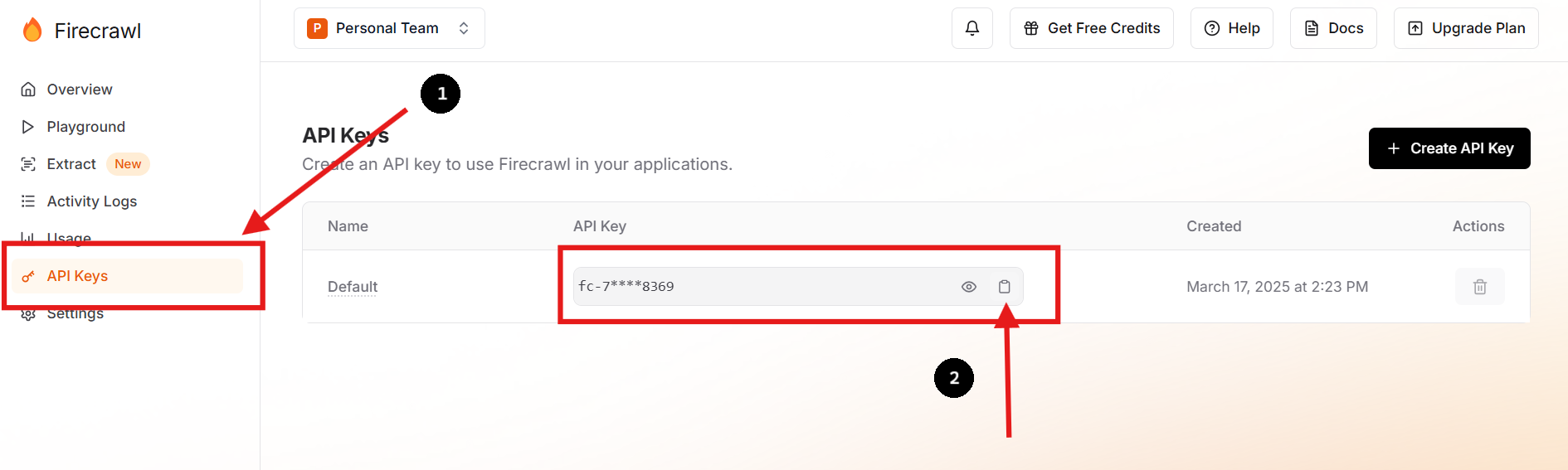
You can also create a new API key and delete the previous one if you prefer or need to do so.

Step 2: Set Up Your Environment
In your project's directory, create a .env
file to securely store your API key as an environment variable. You can do this by running the following commands in your terminal:
touch .env
echo "FIRECRAWL_API_KEY='fc-YOUR-KEY-HERE'" >> .env
This approach keeps sensitive information out of your main codebase, enhancing security and simplifying configuration management.
Step 3: Install the Firecrawl SDK
For Python users, install the Firecrawl SDK using pip:
pip install firecrawl
Step 4: Use Firecrawl's "Scrape
" Function
Here’s a simple example of how to scrape a website using the Python SDK:
from firecrawl import FirecrawlApp
from dotenv import load_dotenv
import os
# Load environment variables from .env file
load_dotenv()
# Initialize FirecrawlApp with the API key from .env
app = FirecrawlApp(api_key=os.getenv("FIRECRAWL_API_KEY"))
# Define the URL to scrape
url = "https://www.python-unlimited.com/webscraping/hotels.php?page=1"
# Scrape the website
response = app.scrape_url(url)
# Print the response
print(response)
Sample Output:
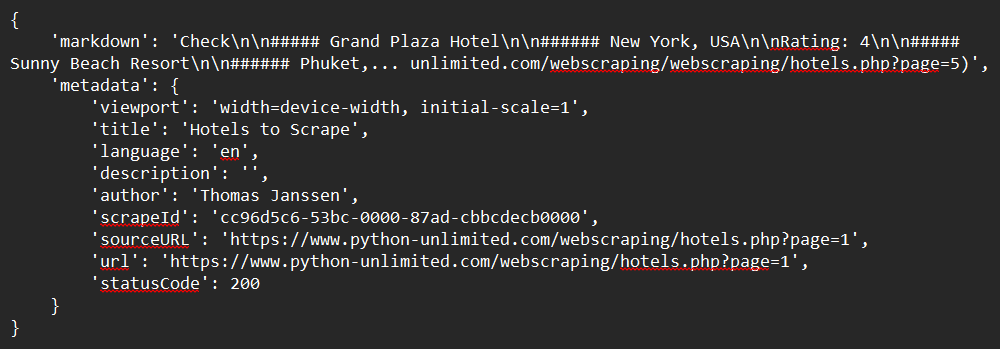
Step 5: Use Firecrawl's "Crawl
" Function
Here we will see a simple example of how to crawl a website using the Python SDK:
from firecrawl import FirecrawlApp
from dotenv import load_dotenv
import os
# Load environment variables from .env file
load_dotenv()
# Initialize FirecrawlApp with the API key from .env
app = FirecrawlApp(api_key=os.getenv("FIRECRAWL_API_KEY"))
# Crawl a website and capture the response:
crawl_status = app.crawl_url(
'https://www.python-unlimited.com/webscraping/hotels.php?page=1',
params={
'limit': 100,
'scrapeOptions': {'formats': ['markdown', 'html']}
},
poll_interval=30
)
print(crawl_status)
Sample Output:
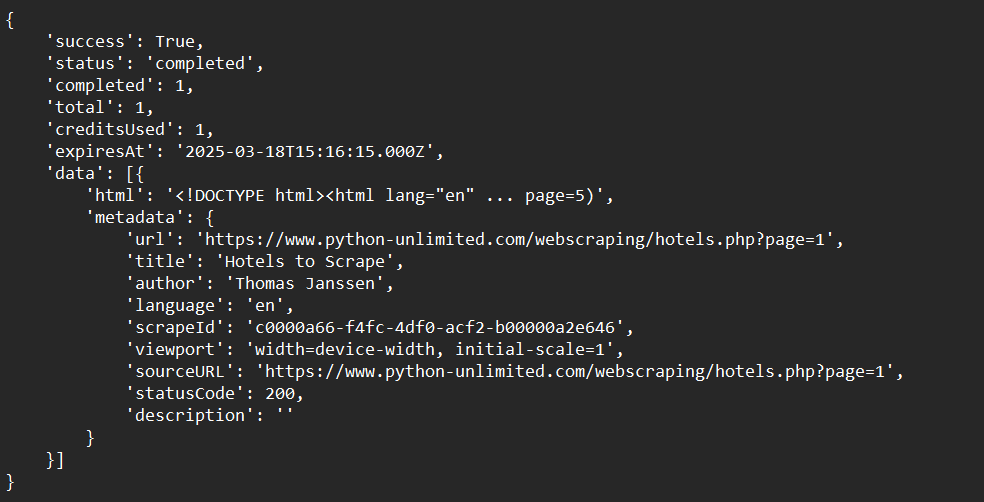
Step 6: Use Firecrawl's "Map
" Function
Here’s a simple example of how to Map website data using the Python SDK:
from firecrawl import FirecrawlApp
from dotenv import load_dotenv
import os
# Load environment variables from .env file
load_dotenv()
# Initialize FirecrawlApp with the API key from .env
app = FirecrawlApp(api_key=os.getenv("FIRECRAWL_API_KEY"))
# Map a website:
map_result = app.map_url('https://www.python-unlimited.com/webscraping/hotels.php?page=1')
print(map_result)
Sample Output:

Step 7: Use Firecrawl's "Extract
" Function (Open Beta)
Below is a simple example of how to extract website data using the Python SDK:
from firecrawl import FirecrawlApp
from pydantic import BaseModel, Field
from dotenv import load_dotenv
import os
# Load environment variables from .env file
load_dotenv()
# Initialize FirecrawlApp with the API key from .env
app = FirecrawlApp(api_key=os.getenv("FIRECRAWL_API_KEY"))
# Define schema to extract contents into
class ExtractSchema(BaseModel):
company_mission: str
supports_sso: bool
is_open_source: bool
is_in_yc: bool
# Call the extract function and capture the response
response = app.extract([
'https://docs.firecrawl.dev/*',
'https://firecrawl.dev/',
'https://www.ycombinator.com/companies/'
], {
'prompt': "Extract the data provided in the schema.",
'schema': ExtractSchema.model_json_schema()
})
# Print the response
print(response)
Sample Output:
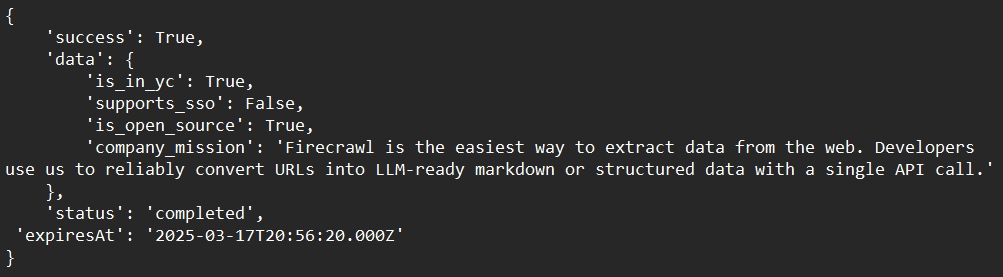
Advanced Techniques with Firecrawl
Handling Dynamic Content
Firecrawl can handle dynamic JavaScript-based content by using headless browsers to render pages before scraping. This ensures you capture all the content, even if it’s loaded dynamically.
Bypassing Web Scraping Blockers
Use Firecrawl’s built-in features to bypass common web scraping blockers, such as CAPTCHAs or rate limits. This involves rotating user agents and IP addresses to mimic natural traffic.
Integrating with LLMs
Combine Firecrawl with LLMs like LangChain to build powerful AI workflows. For example, you can use Firecrawl to gather data and then feed it into an LLM for analysis or generation tasks.
Troubleshooting Common Issues
Issue: "API Key Not Recognized"
Solution: Ensure your API key is correctly stored as an environment variable or in a .env
file.
Issue: "Crawling Too Slow"
Solution: Use asynchronous crawling to speed up the process. Firecrawl supports concurrent requests to improve efficiency.
Issue: "Content Not Extracted Correctly"
Solution: Check if the website uses dynamic content. If so, ensure Firecrawl is configured to handle JavaScript rendering.
Conclusion
Congratulations on completing this comprehensive beginner's guide on Firecrawl! We have covered everything you need to get started—from what Firecrawl is, to detailed installation instructions, usage examples, and advanced customization options. By now, you should have a clear understanding of how to:
- Set up and install Firecrawl in your development environment.
- Configure and run Firecrawl to scrape, crawl, map and extract data efficiently.
- Troubleshoot your crawling processes to meet your specific needs.
Firecrawl is an incredibly powerful tool that can significantly streamline your data extraction workflows. it's flexibility, efficiency, and ease of integration make it an ideal choice for modern web crawling challenges.
Now it's time to put your new skills into practice. Start experimenting with different websites, tweak your parsers, and integrate with additional tools to create a truly customized solution that meets your unique requirements.
Ready to 10x your web scraping workflow? Download Apidog for free today and discover how it can enhance your Firecrawl integration!