Fetching data from APIs is a daily task for many developers. But, fetching API with a cookie? That can sometimes be a bit tricky. In this guide, we'll walk you through the steps to fetch API data using cookies. And for those looking for a robust API testing tool, don’t forget to download Apidog for free to simplify your workflow!
Understanding the Basics: What is an API?
Before diving into the specifics of fetching an API with a cookie, let's cover some basics. An API (Application Programming Interface) allows different software systems to communicate with each other. APIs enable the exchange of data and functionalities between different systems.
What Are Cookies?
Cookies are small pieces of data stored on the user's device by the web browser while browsing a website. They are used to remember information about the user, such as login status or preferences, and are crucial for session management, user tracking, and storing stateful information.
Why Use Cookies with API Fetching?
Cookies are often used in API requests for authentication purposes. When an API requires user authentication, a session cookie may be necessary to maintain the session state between the client and the server. This is particularly common in scenarios where the API calls are made on behalf of an authenticated user.
Tools for Fetching APIs
Before jumping into code, let’s talk about the tools you’ll need. While you can write all the code yourself, tools like Apidog can simplify the process. Apidog provides a suite of tools for API development, including testing, documentation, and mocking.
Setting Up Your Environment
To fetch an API with a cookie, you’ll need:
- A code editor: Visual Studio Code, Sublime Text, or any other editor you prefer.
- A development environment: Node.js for server-side JavaScript or any environment that supports HTTP requests.
- A tool like Apidog: This will streamline your API development process.
Basic API Fetch with JavaScript
Let’s start with a simple example of fetching an API using JavaScript. We’ll use the fetch
function, which is native to modern browsers.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
This basic example shows how to fetch data from an API. But what if the API requires a cookie for authentication?
Fetch API with Cookie
Fetching an API with a cookie involves a few more steps. You need to include the cookie in the headers of your request. Here’s how you can do it:
Step-by-Step Guide
Obtain the Cookie:
First, you need to obtain the cookie from the server. This usually happens when you log in or perform some initial authentication step.
Include the Cookie in Your Fetch Request:
Once you have the cookie, include it in the headers of your fetch
request.
// Example of obtaining a cookie (typically from a login endpoint)
fetch('https://api.example.com/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ username: 'user', password: 'pass' })
})
.then(response => {
// Assuming the cookie is in the 'Set-Cookie' header
const cookie = response.headers.get('Set-Cookie');
// Use the cookie in the next request
return fetch('https://api.example.com/data', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Cookie': cookie // Include the cookie in the headers
});
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
Common Pitfalls and How to Avoid Them
- CORS Issues: Cross-Origin Resource Sharing (CORS) can prevent your requests from succeeding if the server isn’t configured to allow requests from your origin. Ensure the server includes appropriate CORS headers.
- Cookie Expiration: Cookies often have an expiration date. Ensure your code handles cases where the cookie has expired and needs to be refreshed.
Using Apidog to Simplify API Fetching
What is Apidog?
Apidog is an API development tool that makes it easier to create, test, and manage APIs. With Apidog, you can automate many aspects of API development, reducing the amount of manual work required.
Setup Your Apidog Environment
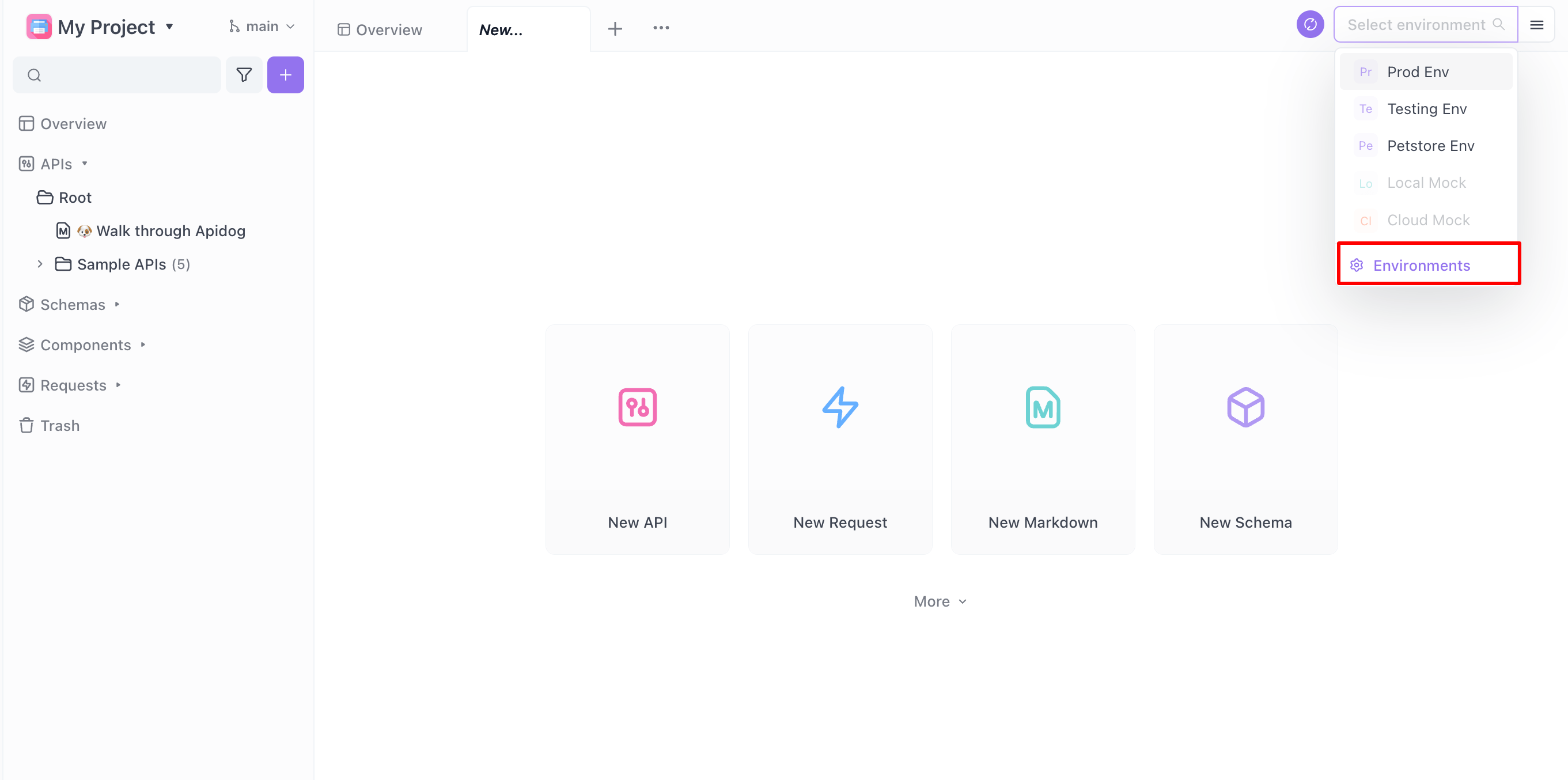
To initiate API testing using Axios and cookies with Apidog, setting up your testing environment is the first critical step. This setup will define the foundational parameters for your API interactions.
Initialize Apidog: Begin by launching Apidog and creating a new environment tailored to your project. This is your workspace for configuring all API testing settings.
Configure API Details: Input the base URL of your API in this environment. It serves as the root for all endpoint requests. Also, set any necessary headers or authentication tokens required by your API.
Creating a New Request
Creating a new request in Apidog involves specifying the type of request and the target API endpoint for testing.
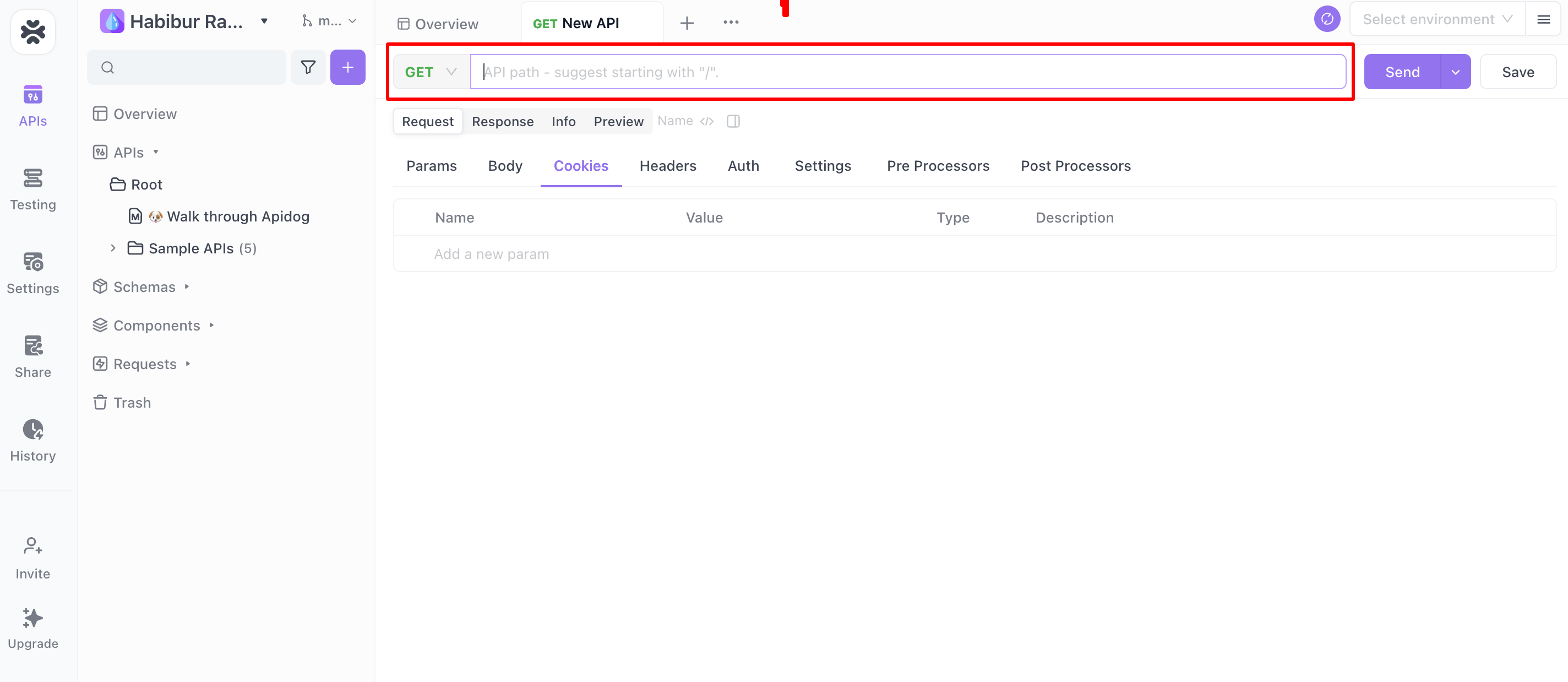
Create Request: In Apidog, establish a new API request, selecting 'GET' as the type if you’re starting with basic requests.
Input Endpoint: Enter the complete URL for the API endpoint you intend to test. This URL directs Apidog where to send your request.
Adding Cookies
Accurate cookie management is essential for effective API testing. In Apidog, the approach to handling cookies varies based on your testing environment.
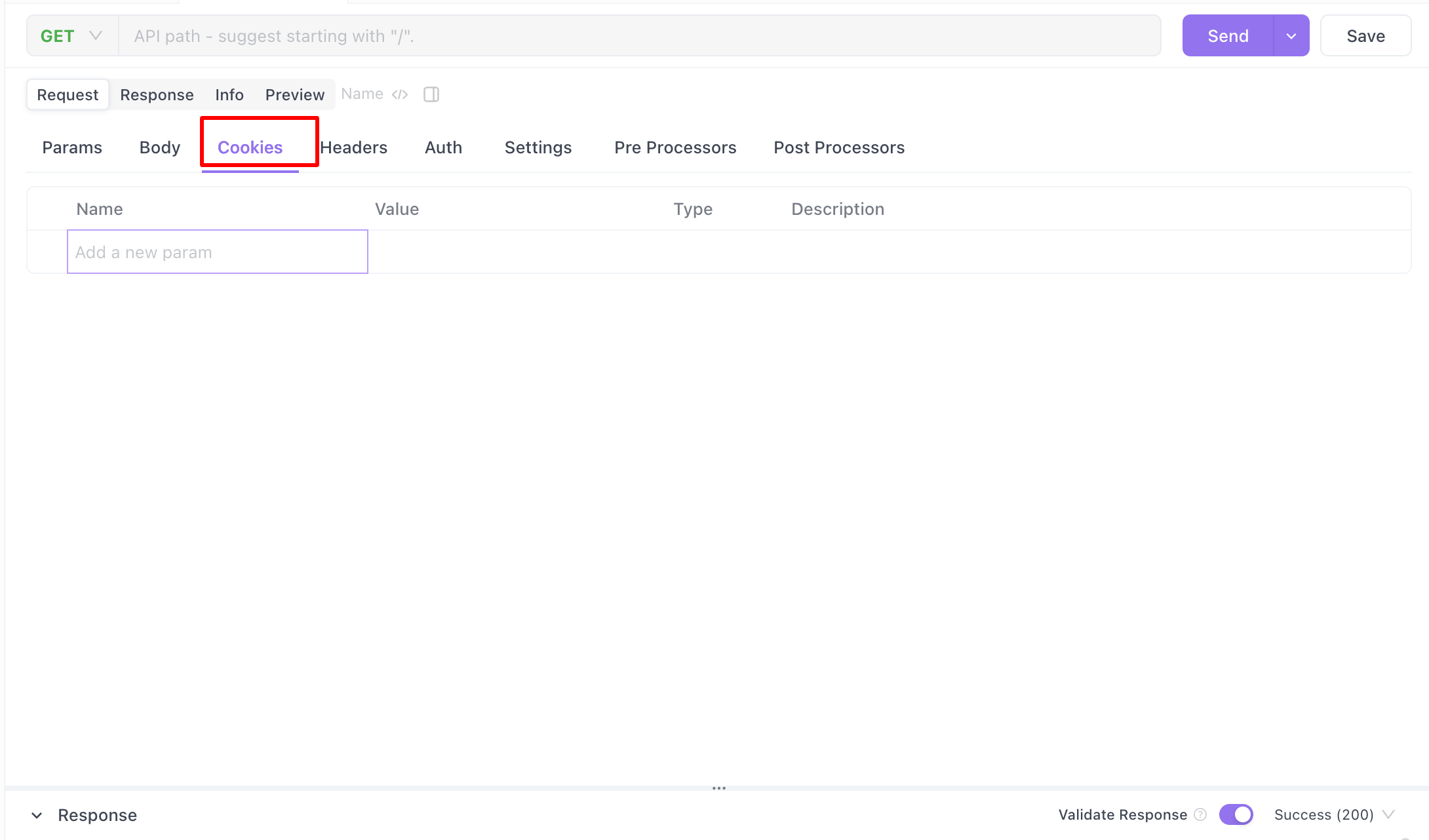
Browser Testing: When testing within a browser, Apidog automatically manages cookies, attaching them as necessary to your requests.
Real-World Example
Let’s look at a real-world example where fetching an API with a cookie is essential. Imagine you’re building a dashboard that displays user-specific data from a third-party service. To access this data, the service requires user authentication via cookies.
Step-by-Step Implementation
User Logs In:
When the user logs in, your application sends a request to the third-party service and stores the session cookie.
Fetch User Data:
Use the stored session cookie to fetch the user-specific data.
// Example: User logs in
function login(username, password) {
return fetch('https://api.thirdparty.com/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ username, password })
})
.then(response => response.headers.get('Set-Cookie'));
}
// Example: Fetch user data using the session cookie
function fetchUserData(cookie) {
return fetch('https://api.thirdparty.com/userdata', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Cookie': cookie
})
.then(response => response.json());
}
// Usage
login('user', 'pass')
.then(cookie => fetchUserData(cookie))
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
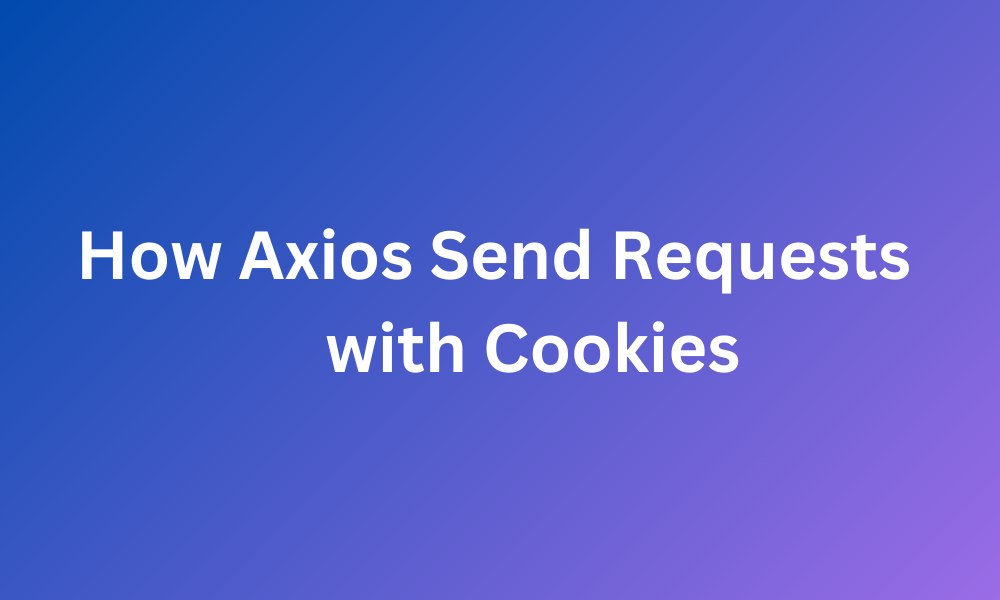
Benefits of Using Cookies with API Requests
Security
Using cookies for API requests enhances security by ensuring that only authenticated users can access certain endpoints. This prevents unauthorized access and helps protect sensitive data.
Session Management
Cookies help manage user sessions, allowing users to stay logged in and maintain their session state across different pages and requests.
Simplified User Experience
By using cookies, you can provide a seamless user experience. Users don’t have to repeatedly log in, and their preferences and state are preserved across different interactions with your application.
Common Challenges and How to Overcome Them
Cookie Handling in Different Environments
Handling cookies can vary across different environments, such as browsers and server-side applications. Ensure you understand the specific requirements and limitations of the environment you’re working in.
Security Concerns
While cookies are useful, they also introduce security concerns, such as cross-site scripting (XSS) and cross-site request forgery (CSRF). Implement proper security measures, such as setting the HttpOnly
and Secure
flags on cookies, to mitigate these risks.
Debugging Issues
Debugging issues related to cookies can be challenging. Use browser developer tools to inspect cookies and monitor API requests. Tools like Apidog can also help by providing detailed logs and insights into your API calls.
Conclusion
Fetching an API with a cookie might seem daunting at first, but with the right approach and tools, it becomes manageable. Remember to handle cookies securely and be mindful of common pitfalls. Tools like Apidog can simplify the process, allowing you to focus on building great applications.
Whether you’re a seasoned developer or just starting, mastering the art of fetching APIs with cookies will enhance your skills and enable you to create more secure and user-friendly applications. Happy coding!