FastAPI is a modern, high-performance web framework for building APIs with Python. One of its standout features is the handling of FastAPI query parameters, which allows developers to create flexible and user-friendly APIs. In this article, we will explore best practices and techniques for using FastAPI query parameters effectively in 2024.
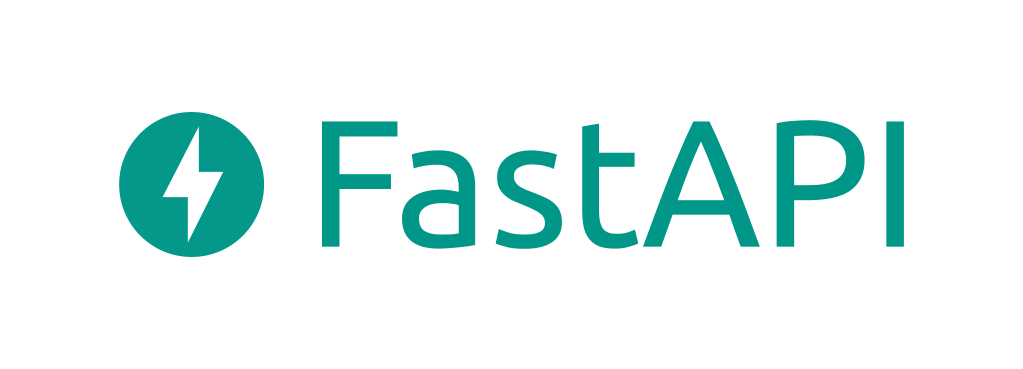
Why Use FastAPI Query Parameters?
- Flexibility: FastAPI query parameters allow clients to customize the results of their requests. For instance, users can filter search results based on specific criteria such as category, price, or rating.
- Usability: They simplify the interaction between clients and the API. Clients can easily append query parameters to the URL, while developers can quickly validate and process these parameters in their code.
- Performance: By using FastAPI query parameters, you can reduce the number of endpoints required for an API. Instead of creating separate endpoints for each filtering option, a single endpoint can handle multiple query parameters.
How to Use FastAPI Query Parameters
Defining FastAPI Query Parameters
To define query parameters in FastAPI, you simply include them as function parameters in your endpoint definition. Here’s a basic example:
from fastapi import FastAPI, Query
app = FastAPI()
@app.get("/items/")
async def read_items(
q: str | None = Query(default=None, max_length=50),
skip: int = Query(default=0, ge=0),
limit: int = Query(default=10, le=100)
):
return {"q": q, "skip": skip, "limit": limit}
In this example:
q
: An optional string parameter with a maximum length of 50 characters.skip
: An integer parameter that specifies how many items to skip, with a default value of 0 and a minimum value of 0.limit
: An integer parameter that specifies the maximum number of items to return, with a default value of 10 and a maximum value of 100.
Accessing FastAPI Query Parameters
When a client makes a request to the endpoint, FastAPI automatically extracts the query parameters from the URL and passes them to the function. For example, if a request is made to /items/?q=foo&skip=10&limit=5
, the function will receive:
q = "foo"
skip = 10
limit = 5
This automatic extraction makes it easy to work with FastAPI query parameters without additional parsing logic.
Optional and Required FastAPI Query Parameters
By default, FastAPI query parameters are optional. If a parameter is not provided in the request, FastAPI will use the default value specified. To make a query parameter required, simply omit the default value:
@app.get("/items/")
async def read_items(required_param: str):
return {"required_param": required_param}
If the required_param
is not included in the request, FastAPI will return a 422 Unprocessable Entity error, indicating that a required query parameter is missing.
Handling Multiple Values for FastAPI Query Parameters
FastAPI allows you to define a query parameter that can accept multiple values. You can do this by specifying the parameter type as a list:
from typing import List
@app.get("/items/")
async def read_items(q: List[str] = Query(default=[])):
return {"q": q}
In this case, the client can make a request like /items/?q=foo&q=bar&q=baz
, and FastAPI will parse the values into a list. This feature is particularly useful for scenarios where users want to filter results based on multiple criteria.
Validating FastAPI Query Parameters
FastAPI provides built-in validation for query parameters using the Query
function. You can specify constraints such as minimum and maximum values, length restrictions, and regex patterns. Here’s an example:
@app.get("/items/")
async def read_items(
q: str = Query(default=None, min_length=3, max_length=50),
item_id: int = Query(ge=1, le=100)
):
return {"q": q, "item_id": item_id}
In this example, q
must be a string with a minimum length of 3 and a maximum length of 50. The item_id
must be an integer between 1 and 100. FastAPI will automatically validate these constraints and return an error if the input does not meet the specified criteria.
Automatic Documentation Generation with FastAPI and OpenAPI
One of the standout advantages of using FastAPI is its seamless integration with OpenAPI, which allows for automatic generation of interactive API documentation. This feature enhances the usability of your API and provides a better experience for developers who consume your API. Here’s a closer look at how FastAPI achieves this and how you can enhance the documentation for your query parameters.
What is OpenAPI?
OpenAPI is a specification for building APIs that provides a standard way to describe the capabilities of your API. It allows both humans and machines to understand the capabilities of a service without accessing its source code or seeing any further documentation. FastAPI leverages this specification to automatically generate interactive documentation for your API endpoints.
How FastAPI Generates Documentation
When you define your FastAPI application and its endpoints, FastAPI automatically creates an OpenAPI schema based on the function signatures and the types of the parameters you define. This schema includes:
- Endpoint paths: The routes you define in your application.
- HTTP methods: The methods (GET, POST, PUT, DELETE, etc.) associated with each endpoint.
- Parameters: Both path and query parameters, including their types, default values, and constraints.
- Response models: The expected response format for each endpoint.
How to Interactive Documentation with FastAPI
FastAPI provides two interactive documentation interfaces out of the box:
- Swagger UI: Accessible at
/docs
, this interface allows users to explore your API's endpoints, view the request and response formats, and even test the endpoints directly from the browser. - ReDoc: Available at
/redoc
, this interface provides a more detailed and structured view of your API documentation, making it easy to navigate and understand the various endpoints and their parameters.
Both interfaces are automatically generated based on the OpenAPI schema created by FastAPI, ensuring that your documentation is always up-to-date with your code.
Enhancing Documentation for Query Parameters
While FastAPI generates basic documentation for your query parameters, you can enhance it further by providing detailed descriptions, examples, and additional metadata. Here’s how:
- Descriptive Text: Use the
description
parameter in theQuery
function to provide clear explanations of what each query parameter does. This is particularly helpful for users who may not be familiar with your API.
from fastapi import FastAPI, Query
app = FastAPI()
@app.get("/items/")
async def read_items(
q: str | None = Query(default=None, description="Search term for filtering items."),
skip: int = Query(default=0, ge=0, description="Number of items to skip."),
limit: int = Query(default=10, le=100, description="Maximum number of items to return.")
):
return {"q": q, "skip": skip, "limit": limit}
2. Examples: Provide examples for your query parameters to illustrate how they can be used. This is particularly useful for complex parameters or when there are specific formats that need to be followed.
@app.get("/items/")
async def read_items(
q: str | None = Query(default=None, description="Search term for filtering items.", example="apple"),
skip: int = Query(default=0, ge=0, description="Number of items to skip.", example=5),
limit: int = Query(default=10, le=100, description="Maximum number of items to return.", example=20)
):
return {"q": q, "skip": skip, "limit": limit}
3. Validation Constraints: Clearly state any validation constraints for your query parameters. This includes minimum and maximum values, length restrictions, and any specific formats. FastAPI handles these validations automatically, but documenting them helps users understand the limits.
4. Type Annotations: Use appropriate type annotations for your query parameters. FastAPI uses these annotations to automatically generate the expected data types in the documentation, which helps users understand what kind of data they should provide.
5. Grouping Related Parameters: If your API has several related query parameters, consider grouping them logically. This can help users understand how the parameters interact with each other and what combinations are valid.
Benefits of Enhanced Documentation
- Improved Usability: Well-documented query parameters make it easier for developers to understand how to interact with your API. This reduces the learning curve and increases adoption.
- Reduced Support Requests: Clear documentation can help answer common questions and reduce the number of support requests you receive from users trying to understand how to use your API.
- Faster Development: Developers can quickly reference the documentation while building applications, leading to faster integration and development cycles.
- Increased API Adoption: Comprehensive and user-friendly documentation can attract more developers to use your API, enhancing its overall success and reach.
- Consistency: Automatic documentation ensures that your API documentation is consistent with the actual implementation, reducing discrepancies that can lead to confusion.
Advanced Techniques for FastAPI Query Parameters
Using Enums for FastAPI Query Parameters
You can restrict FastAPI query parameters to a predefined set of values using Python Enums. This is useful for parameters that should only accept specific options:
from enum import Enum
class ItemType(str, Enum):
fruit = "fruit"
vegetable = "vegetable"
dairy = "dairy"
@app.get("/items/")
async def read_items(item_type: ItemType):
return {"item_type": item_type}
In this example, the item_type
query parameter can only be one of the values defined in the ItemType
Enum. If the client provides an invalid value, FastAPI will return a 422 error.
Query Parameter Dependencies
FastAPI allows you to create dependencies for query parameters, which can help you reuse logic across multiple endpoints. You can define a dependency function that returns a value based on the query parameters:
from fastapi import Depends
def query_param_dependency(q: str | None = None):
return q if q else "default"
@app.get("/items/")
async def read_items(q: str = Depends(query_param_dependency)):
return {"q": q}
In this example, the query_param_dependency
function checks if the query parameter q
is provided. If not, it returns a default value. This approach promotes code reusability and keeps your endpoint functions clean.
Error Handling for FastAPI Query Parameters
FastAPI automatically handles errors related to query parameters. If a required query parameter is missing or if a parameter fails validation, FastAPI returns a 422 Unprocessable Entity response with a detailed error message. You can customize error handling by using exception handlers:
from fastapi import HTTPException
@app.get("/items/")
async def read_items(q: str | None = Query(default=None)):
if q is None:
raise HTTPException(status_code=400, detail="Query parameter 'q' is required.")
return {"q": q}
This example demonstrates how to raise a custom HTTP exception when a required query parameter is not provided. This approach allows you to provide more informative error messages to clients.
Testing FastAPI Query Parameters
Testing your FastAPI application is crucial to ensure that your query parameters work as expected. You can use the httpx
library to perform tests on your FastAPI endpoints. Here’s an example:
import httpx
import pytest
@pytest.mark.asyncio
async def test_read_items():
async with httpx.AsyncClient() as client:
response = await client.get("http://localhost:8000/items/?q=test&skip=0&limit=10")
assert response.status_code == 200
assert response.json() == {"q": "test", "skip": 0, "limit": 10}
In this test, we make a GET request to the /items/
endpoint with query parameters and assert that the response is as expected. Testing is an essential part of the development process, ensuring that your API behaves correctly when handling FastAPI query parameters.
Conclusion
FastAPI query parameters are a powerful feature that allows you to pass additional data to your API endpoints through the URL. They provide flexibility, usability, and performance benefits when building APIs with FastAPI.In this guide, we explored how to define, access, validate, and document FastAPI query parameters using detailed examples. We covered topics such as optional and required parameters, multiple values, complex types, and automatic documentation generation. We also discussed advanced techniques, error handling, testing, and common pitfalls.By leveraging FastAPI query parameters, you can create more flexible and user-friendly APIs that can handle various filtering, sorting, and pagination requirements without the need for multiple dedicated endpoints. Remember to choose appropriate parameter types, validations, and descriptions to ensure your API is easy to use and well-documented for your clients. FastAPI's powerful query parameter capabilities can significantly enhance the functionality and usability of your API, making it an excellent choice for modern web applications.
Additional Resources About FastAPI
To further enhance your understanding of FastAPI query parameters, consider exploring the following resources:
- FastAPI Documentation: The official FastAPI documentation provides comprehensive information about query parameters and other features.
- FastAPI GitHub Repository: Check out the FastAPI GitHub repository for source code, examples, and community contributions.
- FastAPI Tutorials: Look for online tutorials and courses that focus on FastAPI to deepen your knowledge and skills.
By following these best practices and techniques for FastAPI query parameters, you can build robust and efficient APIs that cater to the needs of your users in 2024 and beyond.