In the ever-evolving landscape of application development, efficient data retrieval remains paramount. Traditional RESTful APIs have long served this purpose, but they can become cumbersome when dealing with complex data dependencies. This article delves into GraphQL endpoints, a query language that offers a powerful alternative for developers.
To learn what other functionalities Apidog can provide, make sure to click the button below.
By providing a mechanism to request specific data fields, GraphQL streamlines data fetching and simplifies intricate application logic. We will explore the core functionalities of GraphQL endpoints and their advantages from a developer's perspective.
What is GraphQL?
GraphQL is a query language that allows developers to interact with APIs by precisely requesting the data needed by eliminating unnecessary information.
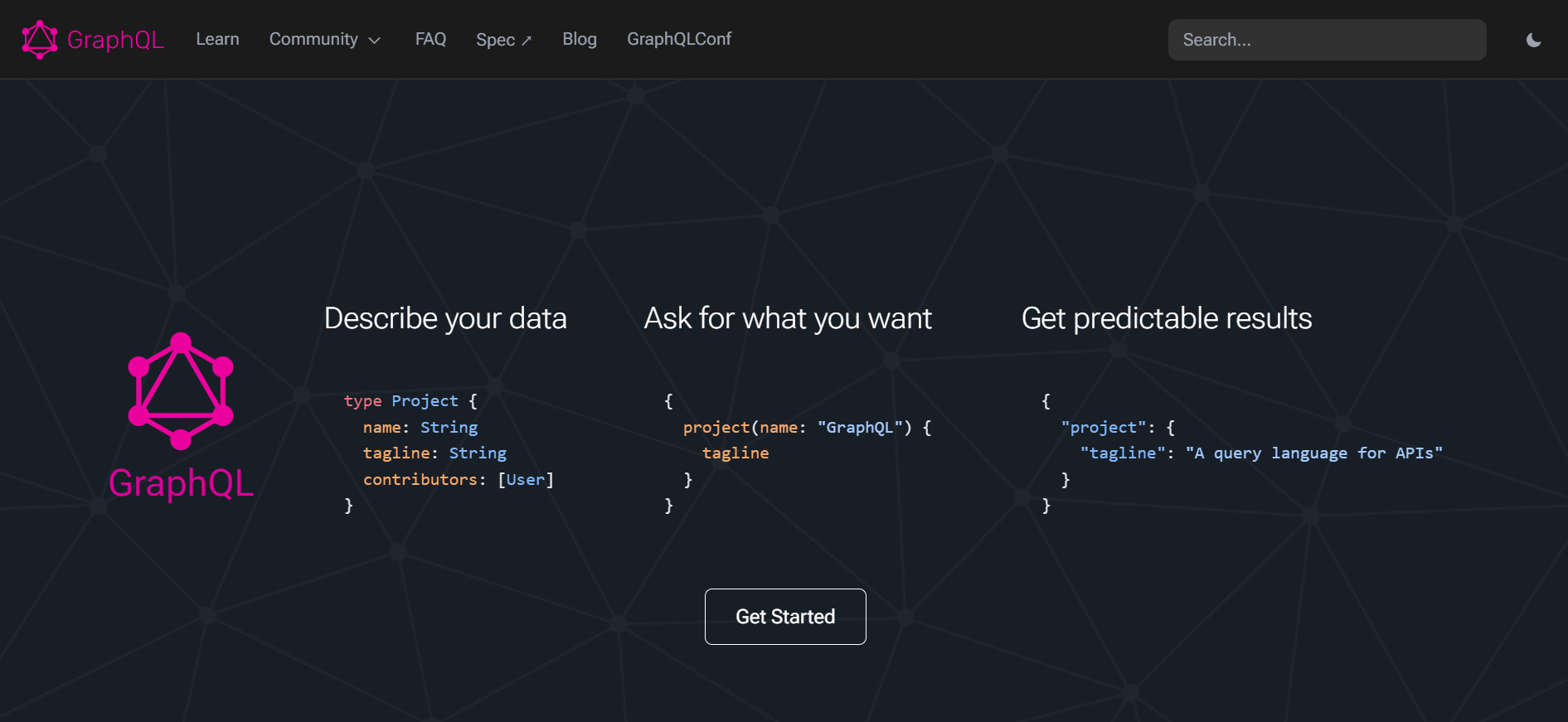
GraphQL also provides developers with a platform to view complete and understandable descriptions of API data.
Core Concept of GraphQL
Unlike traditional RESTful APIs that return predefined data structures, GraphQL empowers developers to request specific data fields within their API. Imagine a bookshelf analogy. With REST APIs, you might get the entire bookshelf delivered when you only need a specific book.
However, GraphQL allows you to directly request the book by title, author, or even just the cover image. This targeted approach reduces unnecessary data transfer and simplifies complex requests that require data from multiple resources.
GraphQL Schema
The foundation of any GraphQL endpoint is the schema. This schema acts as a blueprint, defining the available data types, fields within those types, and the relationships between them. It's essentially a contract between the client (your application) and the server (the data source) that specifies what data is accessible and how it's structured. Think of it as a detailed library catalog that tells you exactly what books are available and what information is associated with each one.
Examples of GraphQL Schema
Example 1 - Simple Blog Schema
type Author {
id: ID!
name: String!
posts: [Post!]!
}
type Post {
id: ID!
title: String!
content: String!
author: Author!
}
type Query {
post(id: ID!): Post
posts: [Post!]!
}
This schema defines three types:
- Author: Represents an author with ID, name, and a list of posts they wrote (represented by the
[Post!]!
syntax - non-null list of non-null Post objects). - Post: Represents a blog post with ID, title, content, and the author who wrote it.
- Query: Defines the available entry points for fetching data. Here, we can query for a specific post by ID (
post
) or retrieve all posts (posts
).
Example 2 - Simplified E-commerce Schema
type Product {
id: ID!
name: String!
price: Float!
category: Category!
reviews: [Review!]!
}
type Category {
id: ID!
name: String!
}
type Review {
id: ID!
content: String!
rating: Int!
}
type Query {
product(id: ID!): Product
products(category: String): [Product!]!
}
This schema showcases more complex relationships:
- Product: Represents a product with details like ID, name, price, category, and a list of reviews.
- Category: Represents a product category with ID and name.
- Review: Represents a product review with ID, content, and rating.
- Query: Similar to the previous example, it allows fetching a specific product (
product
) or filtering products by category (products
).
GraphQL Extensions
Mutations: These operations allow developers to modify data on the server side. Imagine adding a new book to your library catalog. Mutations provide a structured way to create, update, or delete data within your API.
Subscriptions: This feature enables real-time data updates. Think of getting notified when a new book is added to the library. Subscriptions allow applications to react to changes in the underlying data without constant polling.
Advantages of GraphQL Endpoints for Developers
Reduced Complexity and Improved Efficiency
Fetch Only What You Need: Unlike REST APIs that often return entire data structures, GraphQL allows developers to request specific fields within those structures. This targeted approach eliminates unnecessary data transfer, reducing network traffic and improving overall application performance. Imagine fetching just the title and author of a blog post instead of the entire post content and comments when that's all your application needs.
Simplify Complex Data Requests: Traditional REST APIs might require multiple requests to fetch data from different endpoints, leading to complex code and potential data inconsistency. GraphQL allows combining data from various sources into a single request with nested queries. This simplifies complex data retrieval and streamlines application logic.
Increased Flexibility and Developer Productivity
Precise Data Control: Developers have granular control over the data they request, allowing for dynamic and adaptable applications. This flexibility is particularly beneficial for building single-page applications (SPAs) where data needs can vary based on user interaction.
Evolution-Friendly Schemas: GraphQL schemas clearly define the data structure and relationships. This clarity makes it easier to evolve the API over time by adding new fields or modifying existing ones without breaking existing client applications. As long as backward compatibility rules are followed, changes to the schema won't disrupt applications that utilize older versions.
Enhanced Maintainability and Code Clarity
Self-Documenting Schemas: The GraphQL schema acts as a self-documenting contract, clearly outlining the available data and its structure. This eliminates the need for separate documentation, improving code clarity and reducing the maintenance burden for developers.
Type Safety and Error Reduction: GraphQL employs a strong typing system that enforces data types for both queries and responses. Type safety helps catch errors early in the development process, leading to more robust and maintainable applications.
Powerful Developer Tools and Ecosystem
Improved Development Experience: A rich ecosystem of GraphQL tools exists to streamline development. These tools include interactive query editors, schema validation tools, and code generation utilities. This robust ecosystem fosters a more efficient and enjoyable development experience.
Real-time Data with Subscriptions: GraphQL subscriptions enable applications to receive real-time updates whenever the underlying data changes. This eliminates the need for constant polling, simplifying development, and improving application responsiveness.
Public GraphQL Endpoints
There are a few resources where you can test your knowledge of GraphQL endpoints.
Public APIs
GitHub: https://docs.github.com/en/graphql
Explore the vast amount of data available on GitHub, including user information, repositories, and issues.
Facebook: https://developers.facebook.com/docs/graph-api/
Interact with Facebook data like user profiles, posts, and events (requires developer approval).
Spotify: https://developer.spotify.com/documentation/web-api
Access information about music, artists, playlists, and more.
SWAPI (Star Wars API): https://github.com/graphql/swapi-graphql
Explore data from the Star Wars universe in a fun and familiar way.
PokeAPI: https://pokeapi.co/docs/graphql
Dive into the world of Pokemon with detailed information about creatures, moves, and abilities.
Open-source Projects
Hasura: https://hasura.io/graphql/
A popular GraphQL engine that simplifies building backends for applications.
Apollo GraphQL https://www.apollographql.com/
A comprehensive suite of tools for client-side development with GraphQL.
Incorporate GraphQL Seamlessly With Apidog
When trying to implement the GraphQL query language in your app, you should consider searching for a tool that properly supports GraphQL. This is where Apidog, a comprehensive API tool, can definitely be handy for developers.
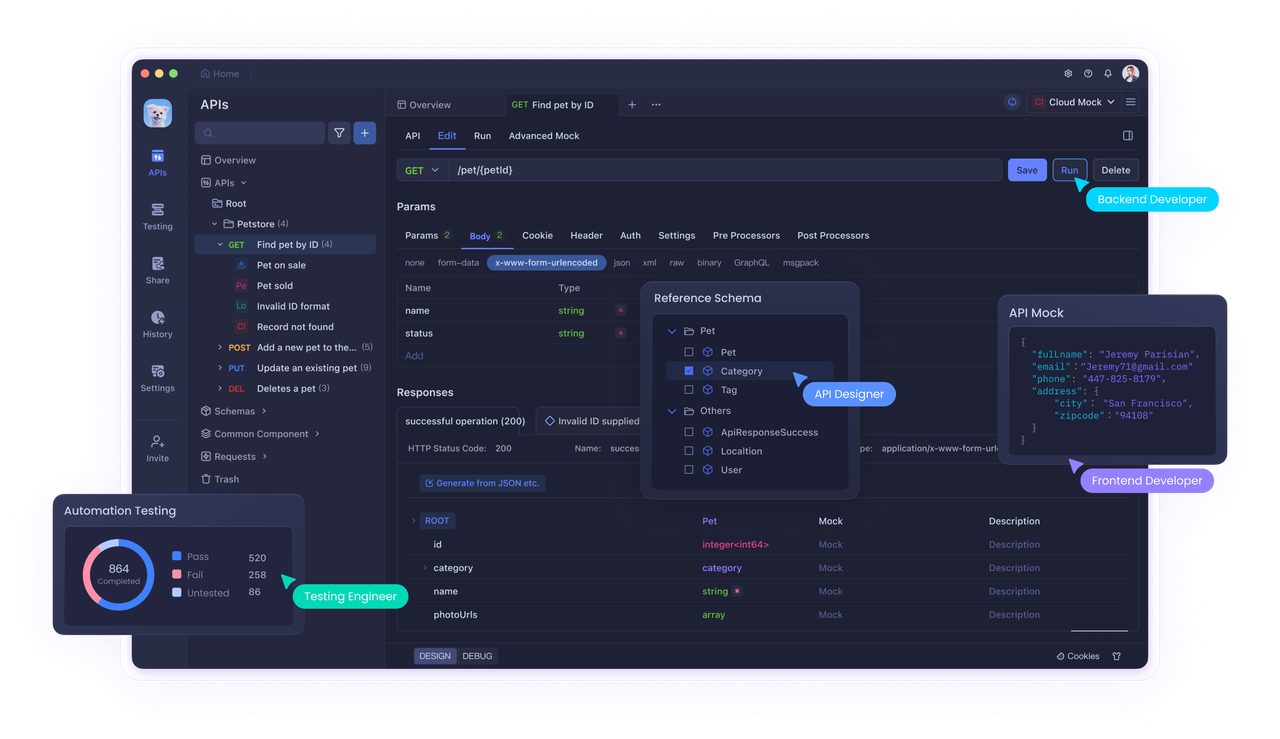
Create a New GraphQL Request With Apidog
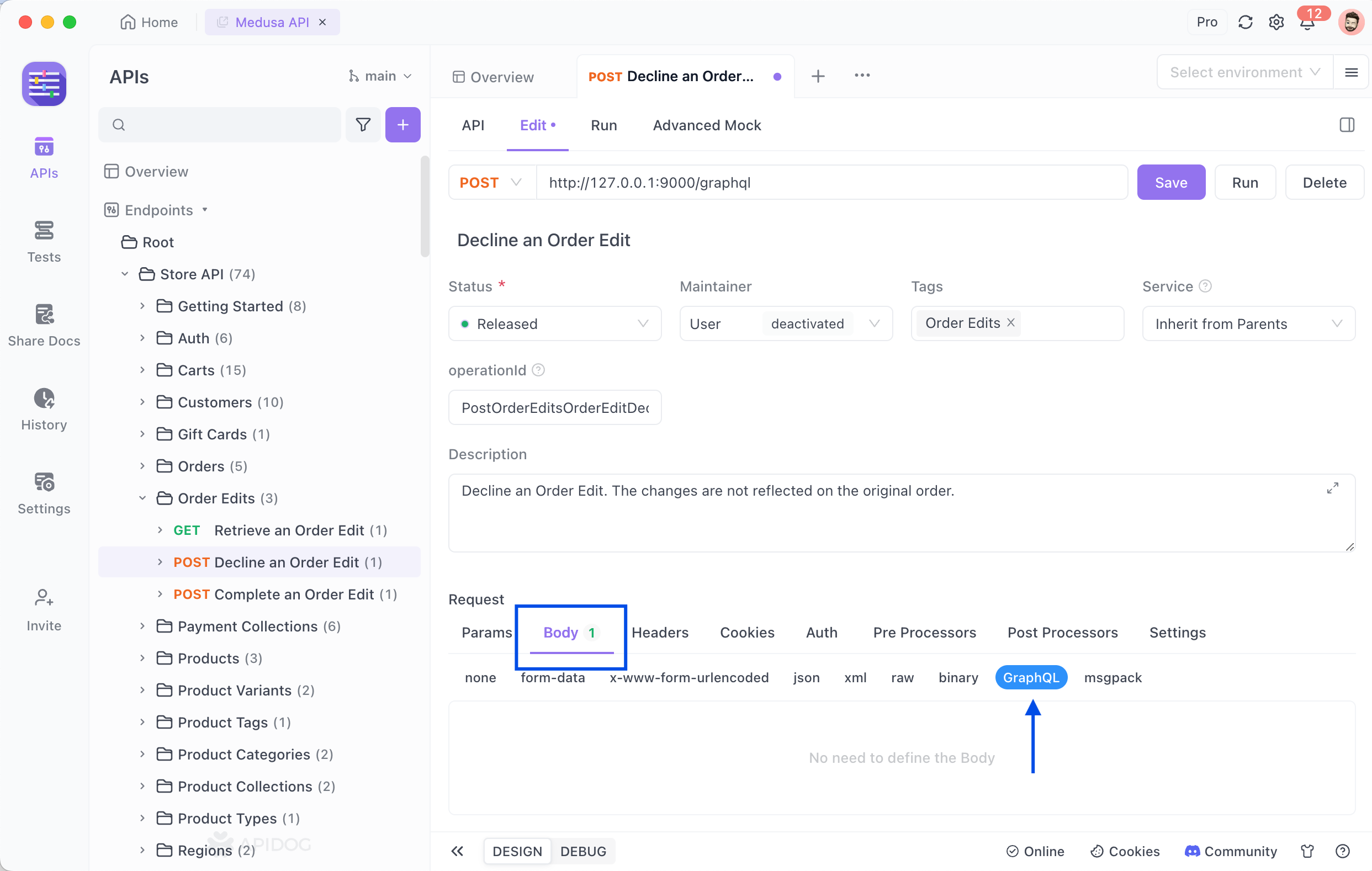
To create a new GraphQL request in a project, click on "Body" → "GraphQL" in that order.
Requesting GraphQL
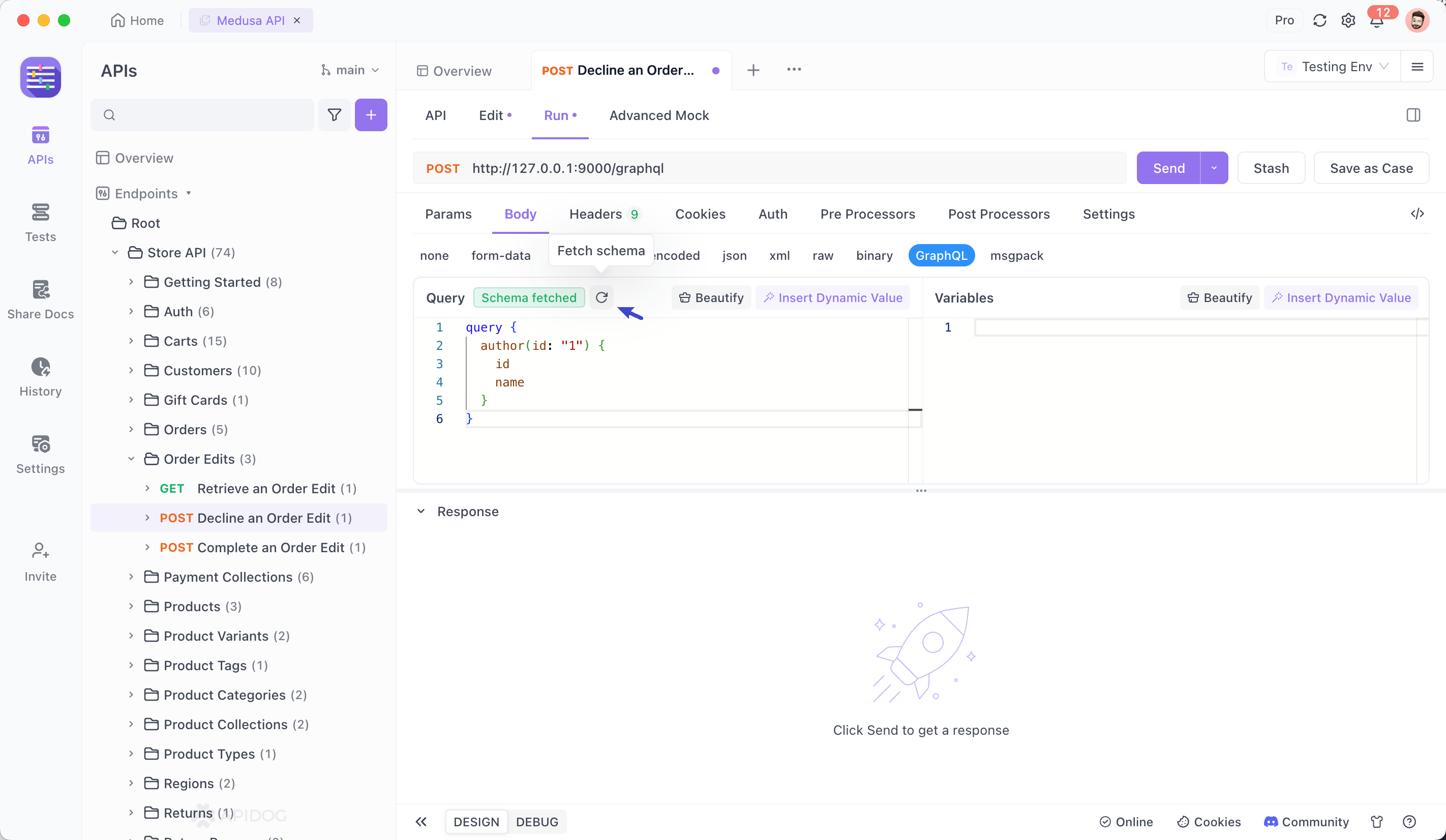
Enter your query in the Query box on the "Run" tab. You can also click the manual Fetch Schema
button in the input box to enable the "code completion" feature for Query expressions, assisting in entering Query statements.
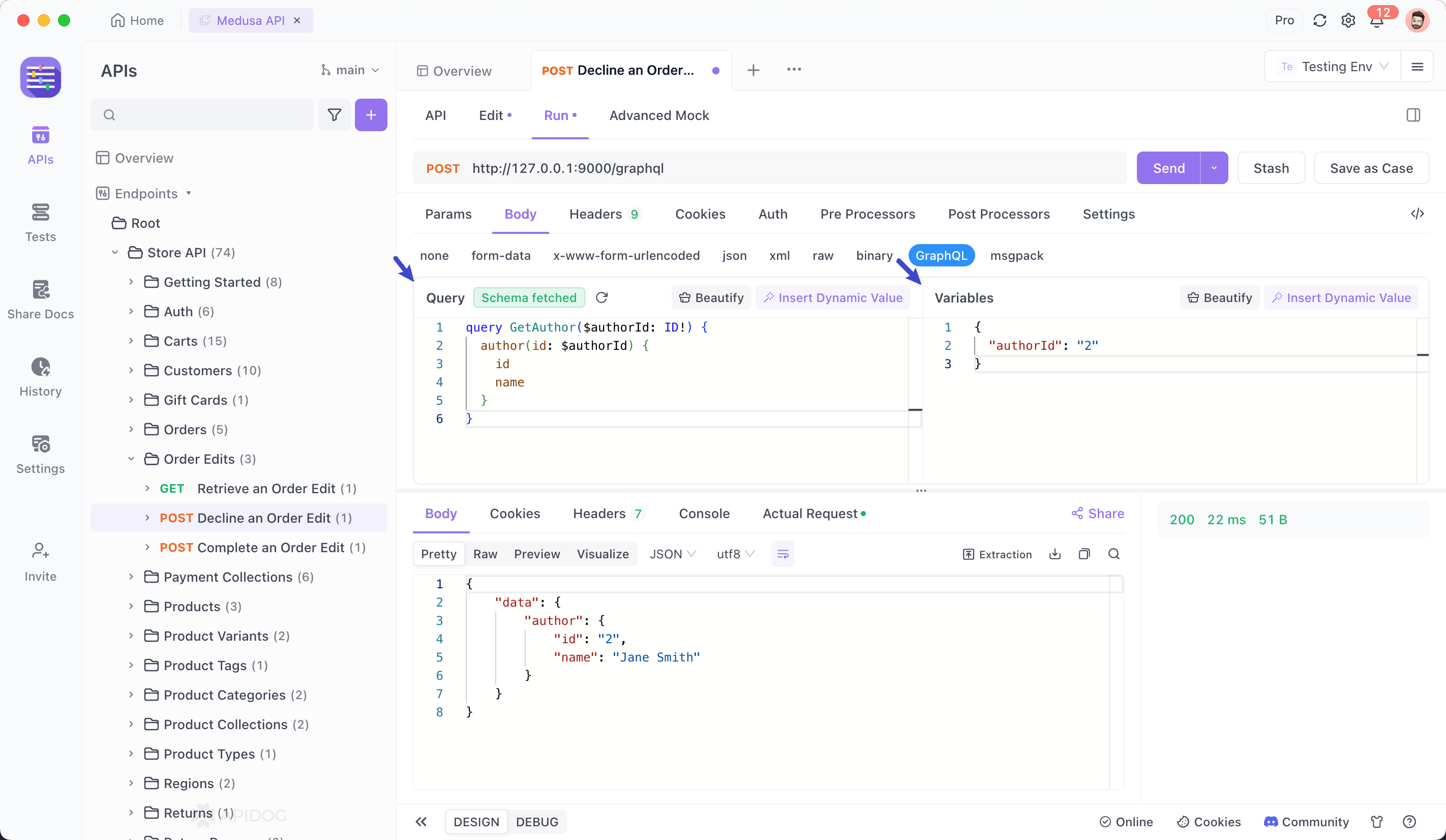
Query statements support using GraphQL variables for requesting. However, for specific usage, please refer to the GraphQL syntax.
Conclusion
GraphQL endpoints offer a compelling alternative to traditional RESTful APIs for developers. By empowering developers to request specific data fields and fostering a more efficient data retrieval process, GraphQL streamlines application development and reduces complexity. Additionally, the clarity and flexibility provided by GraphQL schemas contribute to enhanced code maintainability and a more enjoyable development experience.
As the adoption of GraphQL continues to grow, its potential to revolutionize the way developers interact with APIs becomes increasingly evident. Whether you're building a complex web application or a mobile app with dynamic data needs, GraphQL endpoints are worth considering for their efficiency, flexibility, and developer-friendly approach.