How to Make a curl_init GET Request?
PHP's curl_init() function can be used for initiating communication with a web server and retrieving data via the GET method. By specifying the desired URL, developers can facilitate tasks like data acquisition and API interaction!
cURL, short for "Client for URLs," is a software powerhouse that empowers developers with a versatile toolkit for transferring data. It offers two functionalities: a user-friendly command-line tool and a powerful library that can be integrated into programs.
If you need to edit or test your PHP cURL commands, check out Apidog's website by clicking the button below.
Formal Definition of curl_init()
Based on the official PHP website, the curl_init function initializes a cURL session and returns a cURL handle for use with the curl_setopt(), curl_exec(), and curl_close() functions.
Parameters Involved
url
If you provide a URL, the CURLOPT_URL
option will be set to its value. You can also manually set this option using the curl_setopt() function.
However, do note that the file
protocol is disabled by cURL itself if the open_basedir
has been set.
Return Values
The curl_init() function returns a cURL handle on success, and false
on errors.
What is a GET Request?
In the context of Hypertext Transfer Protocol (HTTP), a GET request is a specific method used to retrieve data from a designated resource on a server. It is classified as a safe method, meaning it does not alter the state of the resource itself. Here's a breakdown of the formal definition:
Function
GET requests are a representation of the specified resource.
Expected Outcome
A successful GET request should return a response containing the requested data, typically in the form of HTML, text, or other data formats.
Data Modification
GET requests are intended to retrieve existing information and should not be used to modify data on the server.
Caching
Responses to GET requests are often cachable by the client (web browser) and intermediary servers, which can improve performance for subsequent requests for the same resource.
GET Request Key Points
- GET requests are fundamental to how web browsers interact with web servers to retrieve web pages and other resources.
- They are considered "safe" because they do not modify the server-side data.
- They are often used for fetching static content like HTML pages, images, and stylesheets.
Code Examples of curl_init() GET Requests
To have a headstart on understanding how to make GET requests with the curl_init()
function involved, continue reading this section!
Basic GET Request
<?php
$url = "https://www.example.com";
// Initialize curl
$ch = curl_init($url);
// Set option to return the transfer as a string
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if(curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Process the response (e.g., echo, decode JSON)
echo $response;
}
// Close the curl session
curl_close($ch);
?>
GET Request with Additional Option
<?php
$url = "https://www.example.com";
// Initialize curl
$ch = curl_init($url);
// Set user agent
curl_setopt($ch, CURLOPT_USERAGENT, "My PHP Script");
// Set option to follow redirects
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1);
// Set option to return the transfer as a string
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if(curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Process the response
echo $response;
}
// Close the curl session
curl_close($ch);
?>
Complex GET Request Example
This is a slightly complicated GET request that involves an API that permits filtering products based on category price range, and sorting options.
<?php
$url = "https://api.example.com/products";
// Define filter parameters
$filters = array(
"category" => "electronics",
"price_min" => 100,
"price_max" => 500,
"sort" => "price_asc"
);
// Build query string with encoded parameters
$queryString = http_build_query($filters);
$url .= "?" . $queryString;
// Initialize curl
$ch = curl_init($url);
// Set option to return the transfer as a string
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// Set headers to indicate JSON data is expected (optional)
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json'
));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if(curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response (assuming response is JSON)
$data = json_decode($response, true);
// Process the data (e.g., loop through products)
if (isset($data['products'])) {
foreach ($data['products'] as $product) {
echo "Product Name: " . $product['name'] . ", Price: $" . $product['price'] . PHP_EOL;
}
}
}
// Close the curl session
curl_close($ch);
?>
Code Explanation:
- We define an API endpoint URL and filter parameters (category, price range, and sort).
- We use
http_build_query
to create a query string with encoded parameter values and append it to the URL. curl_setopt
sets options for the request, including capturing the response and potentially setting headers if the API expects a specific data format (like JSON).- After error checking, the response is decoded as JSON (assuming the API returns JSON data).
- We iterate through the decoded data (products array) and display product details.
Must-Read Note
Do note that these are only code examples that are simplified to explain how it is possible to make GET requests using the curl_init()
function, so ensure that the required modifications are made in your respective IDE!
For the official documentation, you can check it out at: https://www.php.net/manual/en/book.curl.php
Apidog - Work With cURL Files Effortlessly
Apidog is a sophisticated API development platform that provides users with all the necessary tools for the entire API lifecycle. With functionalities from popular tools like Postman and Insomnia, you no longer have to download any other applications!
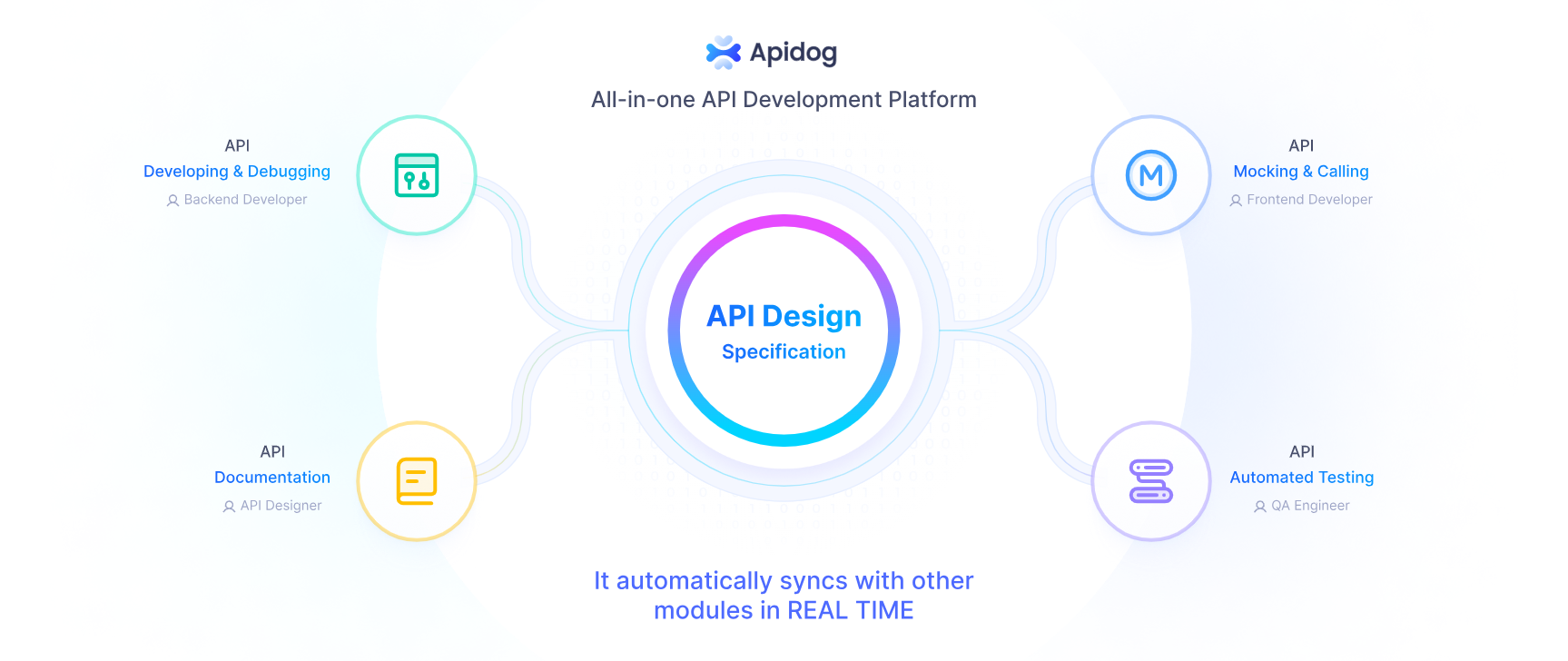
Swiftly Import cURL Commands into Apidog
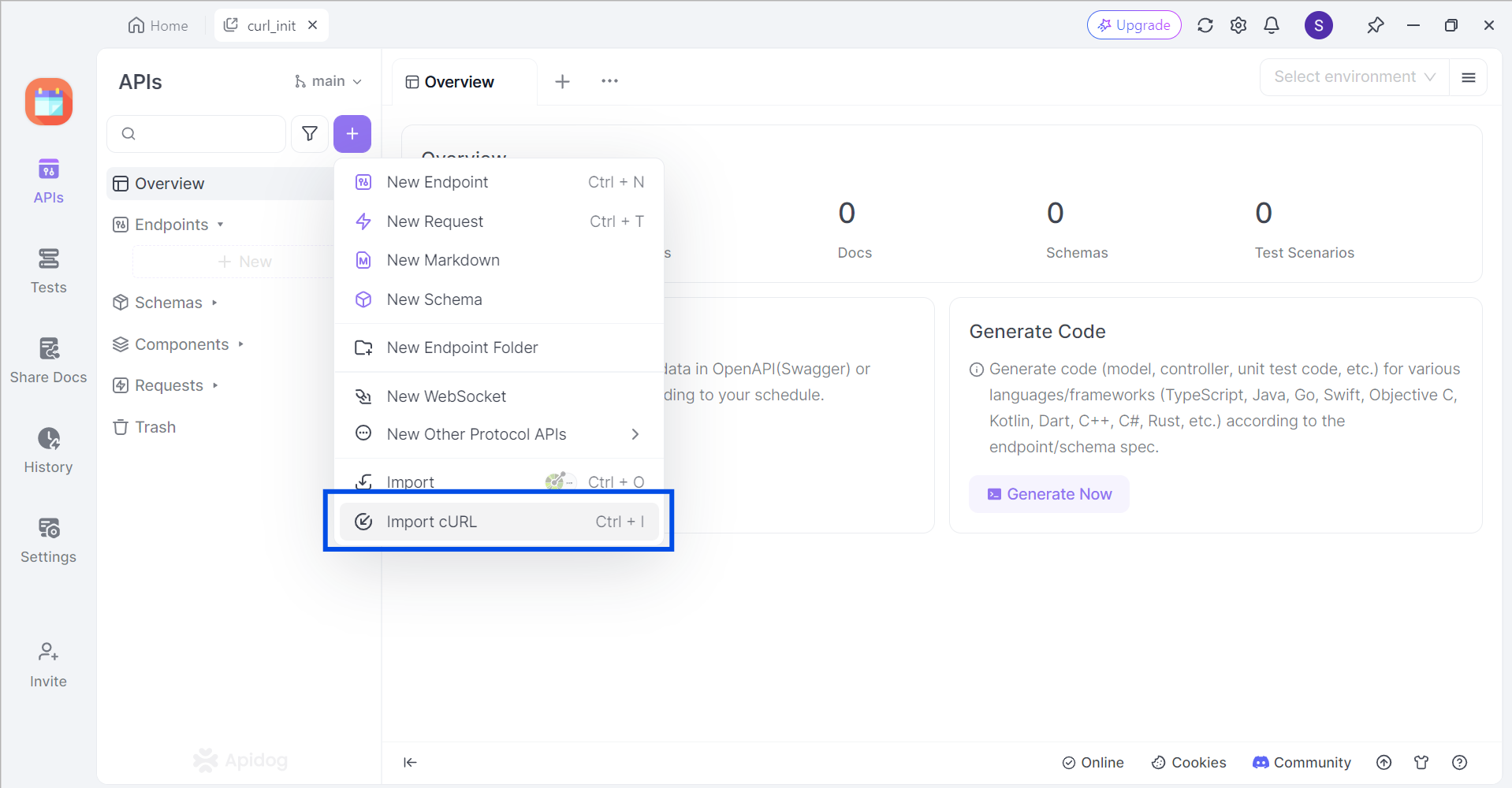
Apidog supports users who wish to import cURL commands to Apidog. In an empty project, click the purple +
button around the top left portion of the Apidog window, and select Import cURL
.
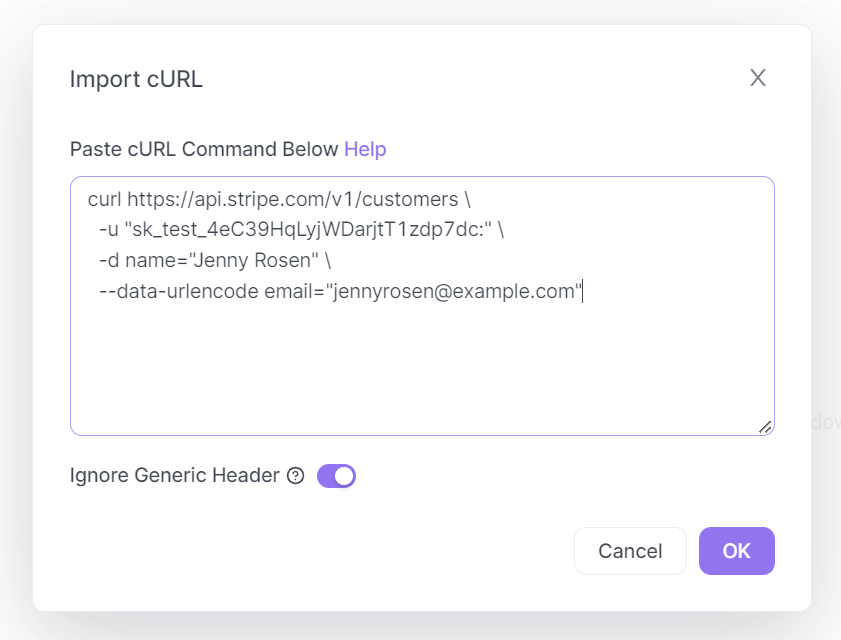
Copy and paste the cURL command into the box displayed on your screen.
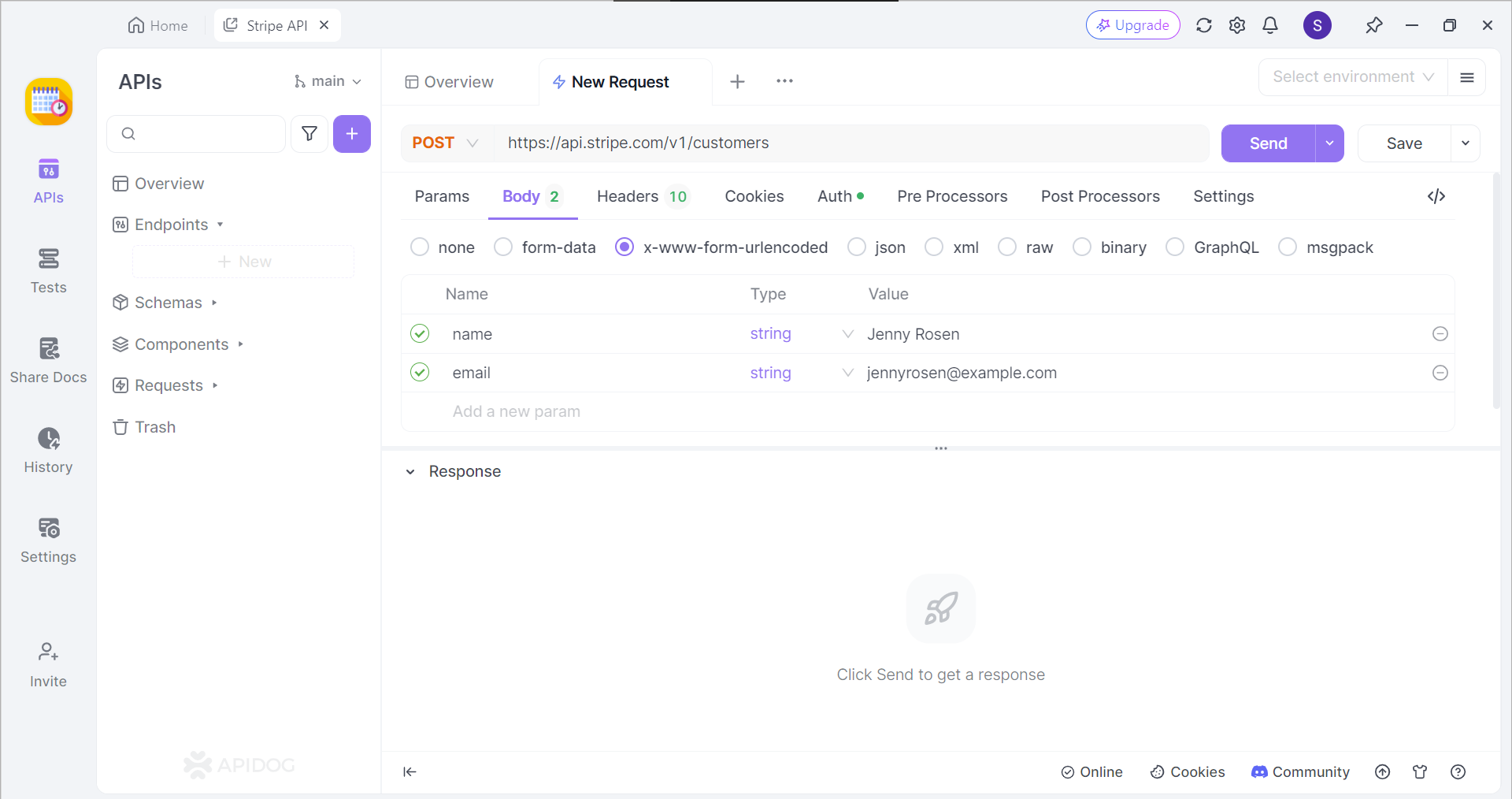
If successful, you should now be able to view the cURL command in the form of an API request.
Generate PHP Code with Apidog
If you require assistance with PHP coding, Apidog has a code generation feature that can help you with that.
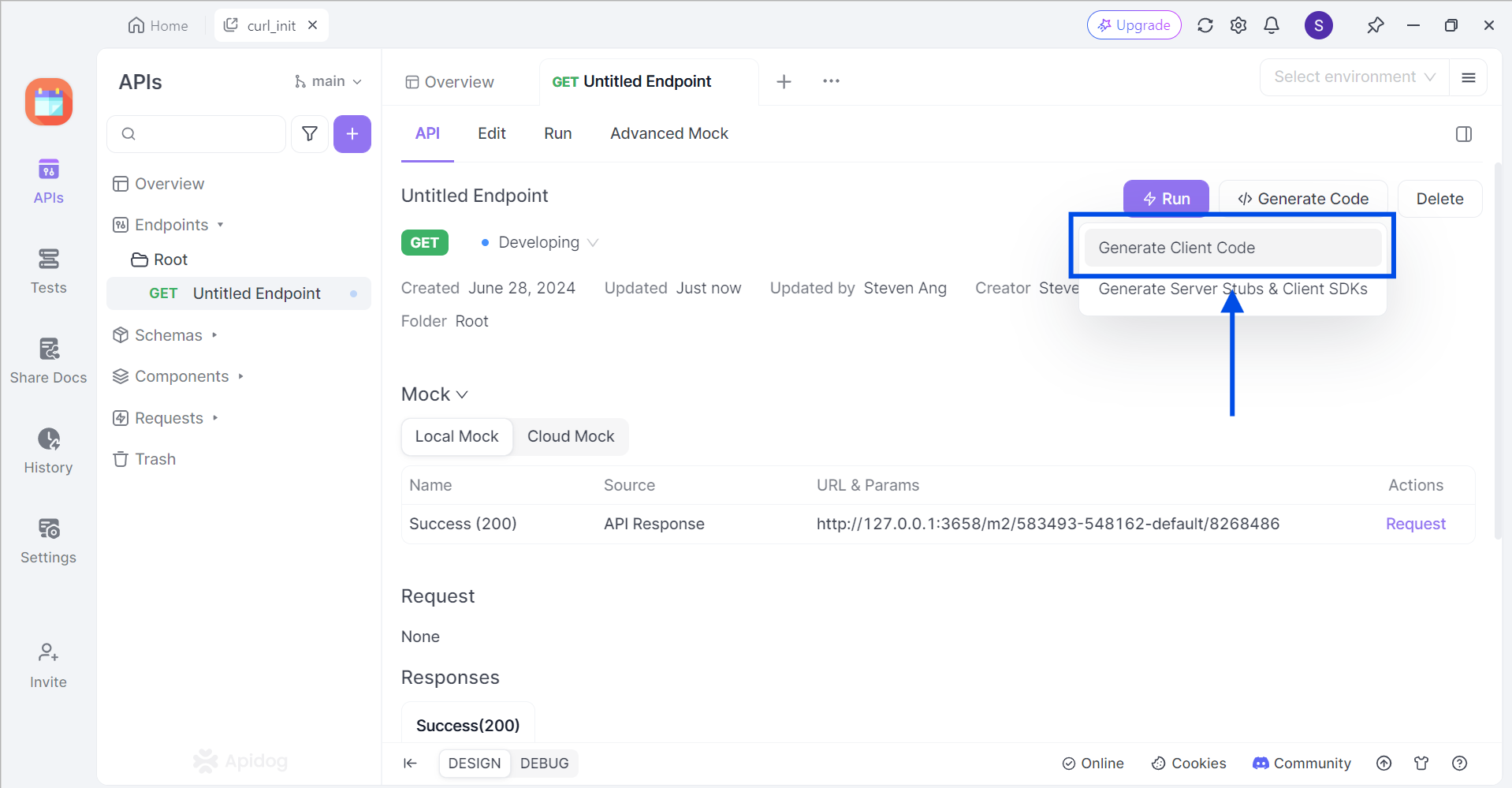
First, locate the </> Generate Code
button on any API or request, and select Generate Client Code
on the drop-down list.
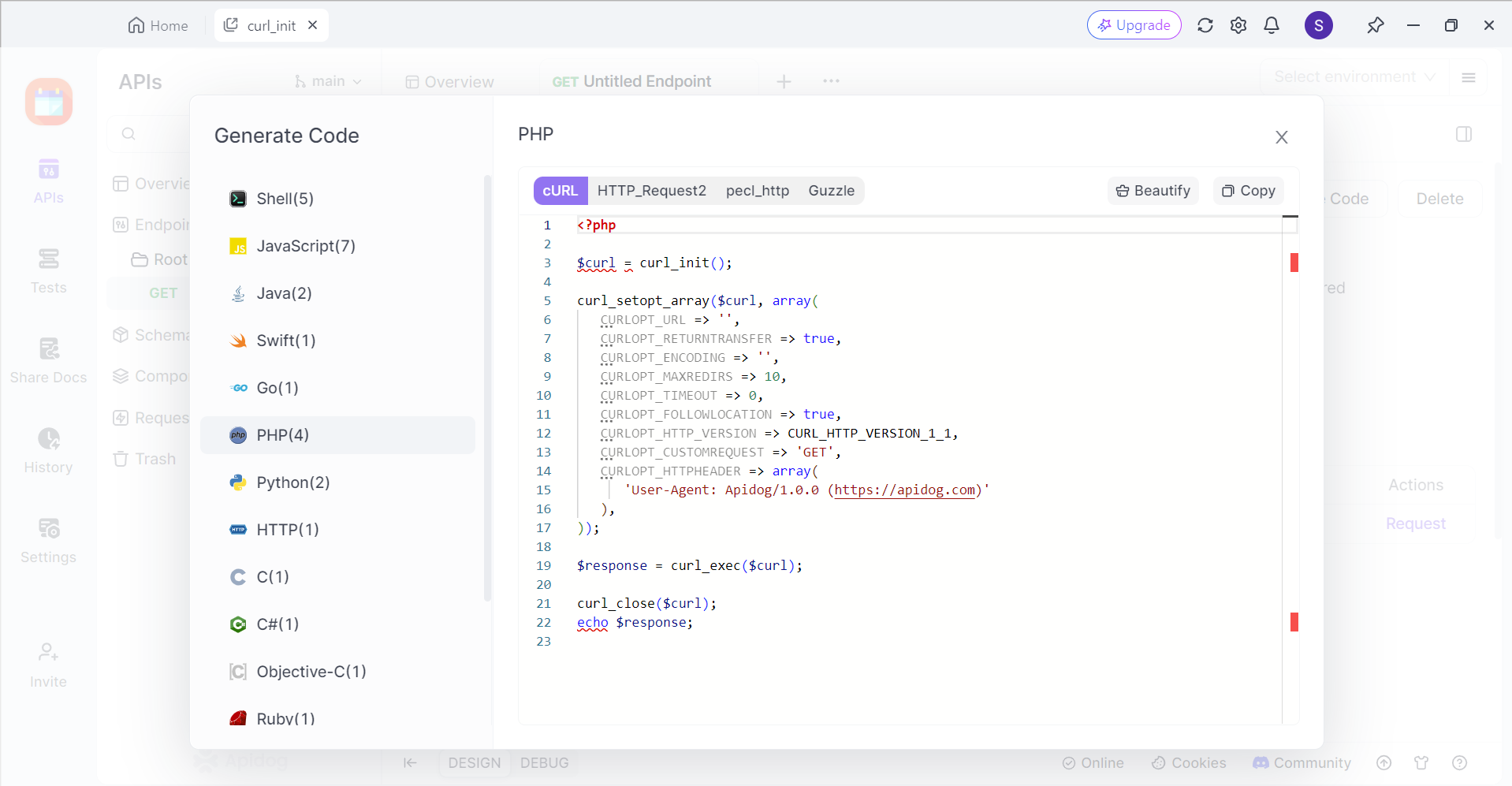
Next, select PHP, and find the cURL section. You should now see the generated code for cURL. All you have to do is copy and paste it to your IDE (Integrated Development Environment) and continue developing your application.
Conclusion
the curl_init()
function in PHP empowers you to retrieve data from web servers using GET requests. This fundamental building block allows you to interact with APIs, download files, and scrape web content. By mastering curl_init()
, you can automate tasks, gather information programmatically, and extend the functionality of your PHP applications.
Remember to explore the extensive options offered by cURL to fine-tune your requests, handle errors gracefully, and ensure secure communication. With its versatility and power,curl_init()
unlocks a world of possibilities for your web development endeavors.