The cURL project, formally known as "Client for URLs," offers developers a comprehensive suite of functionalities for managing data transfers. It operates in two primary modes: a user-oriented command-line interface for basic interactions and a robust library designed for seamless integration within software development projects.
To learn what functionalities Apidog provides, click the button below!
Formal Definition of curl_init()
Based on the official PHP website, the curl_init function initializes a cURL session and returns a cURL handle for use with the curl_setopt(), curl_exec(), and curl_close() functions.
Parameters Involved
url
If you provide a URL, the CURLOPT_URL
option will be set to its value. You can also manually set this option using the curl_setopt() function.
However, do note that the file
protocol is disabled by cURL itself if the open_basedir
has been set.
Return Values
The curl_init() function returns a cURL handle on success, and false
on errors.
What is a DELETE Request?
In the context of the web, a DELETE request is a specific instruction sent using the Hypertext Transfer Protocol (HTTP) to a server. It instructs the server to remove or delete a designated resource. This resource could be a file, a database entry, or any other piece of data that the server manages.
How do DELETE Requests Work?
Here's a breakdown of how DELETE requests work:
Step 1 - Client Initiates
A client, such as a web browser or a software application, sends the DELETE request to the server.
Step 2 - Resource Specified
The request includes a Uniform Resource Identifier (URI) that pinpoints the exact resource on the server that should be deleted.
Step 3 - Server Action
Upon receiving the DELETE request, the server processes it and, if successful, removes the specified resource.
Step 4 - Response Sent
The server then sends a response code back to the client indicating the outcome of the deletion attempt. Common success codes include 200 (OK), 202 (Accepted), and 204 (No Content).
Notable Points About DELETE Requests
Idempotent
Sending the same DELETE request multiple times should have the same effect (i.e., deleting the resource once).
Not Guaranteed
Even with a successful response code, the actual deletion might not happen immediately due to server implementation or policies.
Permanent Deletion
Unlike some editing operations, DELETE requests typically result in the permanent removal of the resource.
Code Examples of Making DELETE Requests with curl_init() Function
Example 1 - Basic DELETE Request
<?php
$url = "https://api.example.com/resources/123"; // Replace with the actual URL
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "DELETE"); // Set request method to DELETE
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Capture response
$response = curl_exec($ch);
$http_code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
if ($http_code == 200) {
echo "Resource deleted successfully!";
} else {
echo "Error deleting resource: " . $http_code;
}
?>
Code explanation:
- We define the target URL for the DELETE request.
curl_init($url)
initializes the cURL handle.curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "DELETE")
sets the request method to "DELETE".curl_setopt($ch, CURLOPT_RETURNTRANSFER, true)
ensures the response is captured as a string.$response = curl_exec($ch)
executes the DELETE request and stores the response.$http_code = curl_getinfo($ch, CURLINFO_HTTP_CODE)
retrieves the HTTP response code.- We close the cURL handle with
curl_close($ch)
. - The code checks the response code. A 200 indicates successful deletion.
Example 2 - DELETE Request with Authentication
<?php
$url = "https://api.example.com/protected/resources/456"; // Replace with URL
$username = "your_username";
$password = "your_password";
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "DELETE");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, $username . ":" . $password); // Set credentials
$response = curl_exec($ch);
$http_code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
// ... (check response code and handle accordingly)
?>
Code explanation:
- We define the URL and authentication credentials.
- The code structure follows the previous example with additional lines for authentication.
curl_setopt($ch, CURLOPT_USERPWD, $username . ":" . $password)
sets the username and password for basic authentication.
Example 3 - DELETE Request with JSON Payload (Python)
import json
import curl
url = "https://api.example.com/data"
data = {"id": 789} # Replace with your data
headers = {"Content-Type": "application/json"}
response = curl.easy.request(
url,
custom_request="DELETE",
data=json.dumps(data).encode("utf-8"), # Encode data as JSON bytes
headers=headers
)
if response.status_code == 200:
print("Resource deleted successfully!")
else:
print(f"Error deleting resource: {response.status_code}")
Code explanation:
1. We import the necessary libraries and define the URL and data object.
2. We set the Content-Type
header to indicate JSON data.
3. curl.easy.request
performs the request with arguments:
url
: Target URLcustom_request
: Set to "DELETE"data
: JSON data encoded as bytesheaders
: Dictionary containing the Content-Type header
4. We check the response status code for success (200).
Note to Remember
Please ensure that the code samples above are not copied and pasted into your IDEs, as they are simplified and will require further modifications to fit your application's needs.
For the official documentation, you can check it out at: https://www.php.net/manual/en/book.curl.php
Apidog - Design cURL API to Perfection
If you are seeking a refined solution to efficient API development, you should consider trying Apidog - it is an API development platform that equips developers with all the necessary tools for the entire API lifecycle.
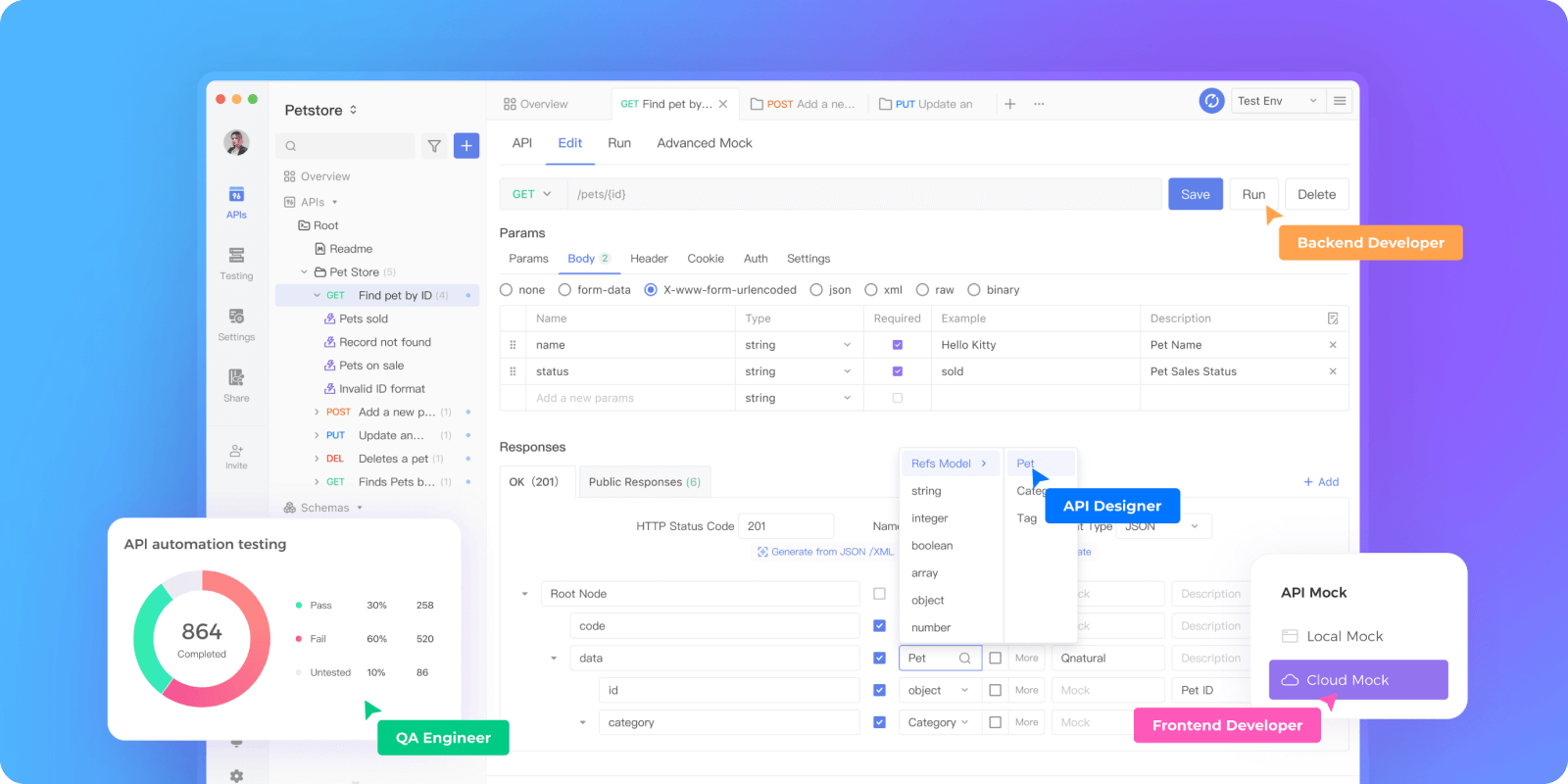
Import cURL APIs to Apidog
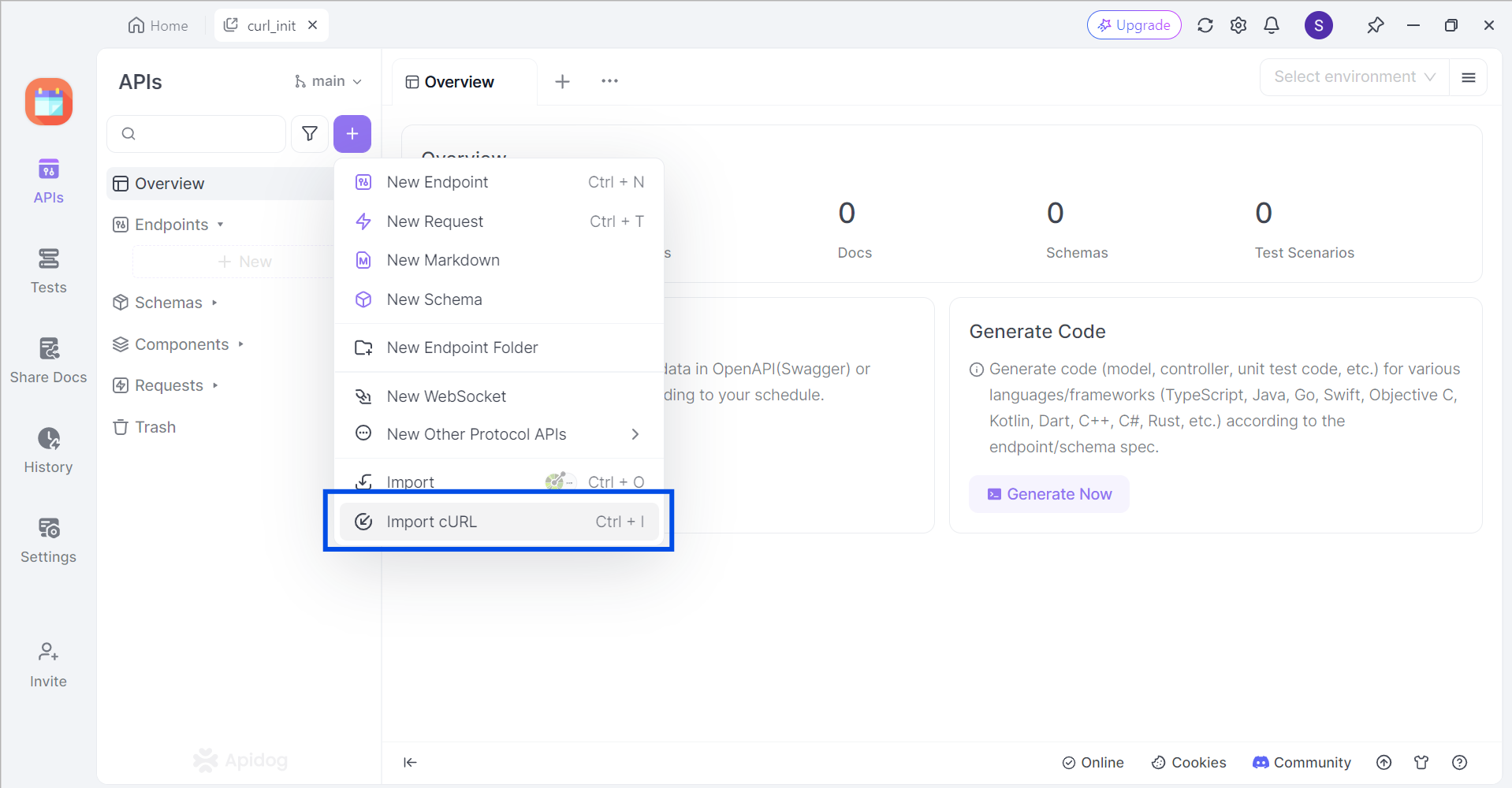
Apidog supports users who wish to import cURL commands to Apidog. In an empty project, click the purple +
button around the top left portion of the Apidog window, and select Import cURL
.
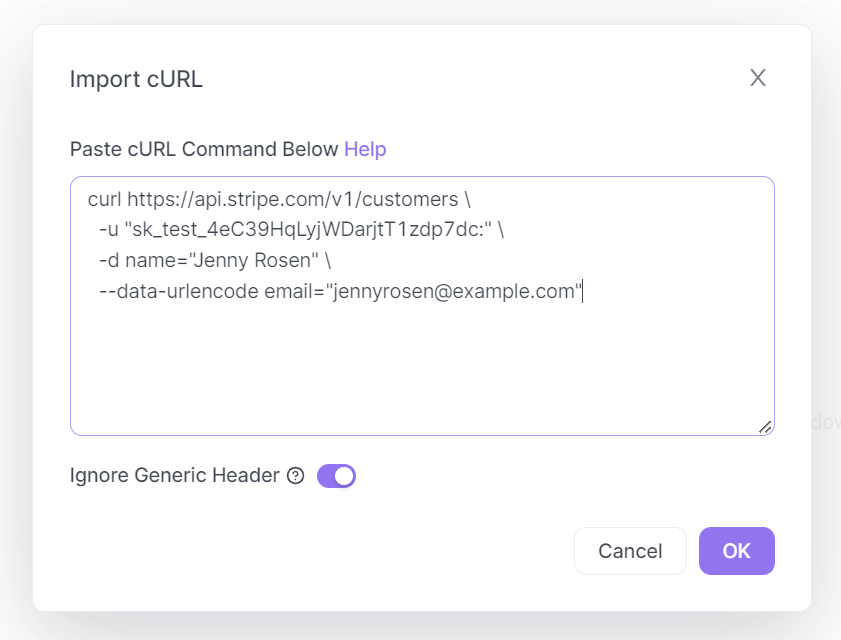
Copy and paste the cURL command into the box displayed on your screen.
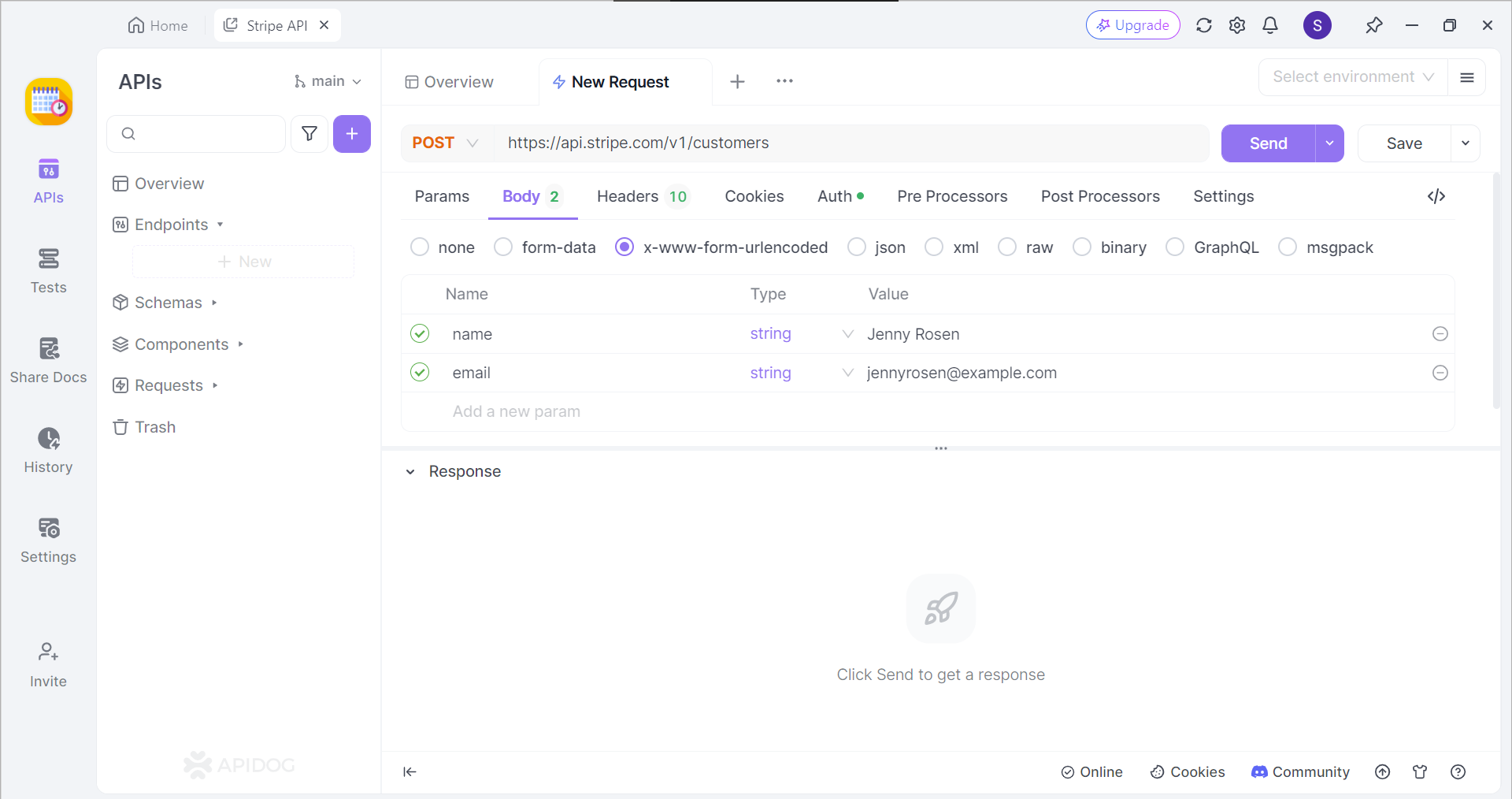
If successful, you should now be able to view the cURL command in the form of an API request.
Generate PHP Code for cURL API with Apidog
If you do not have prior experience coding in the PHP programming language, fear not! Apidog has a code generation feature that you can rely on, providing you with code frameworks for multiple other programming languages.
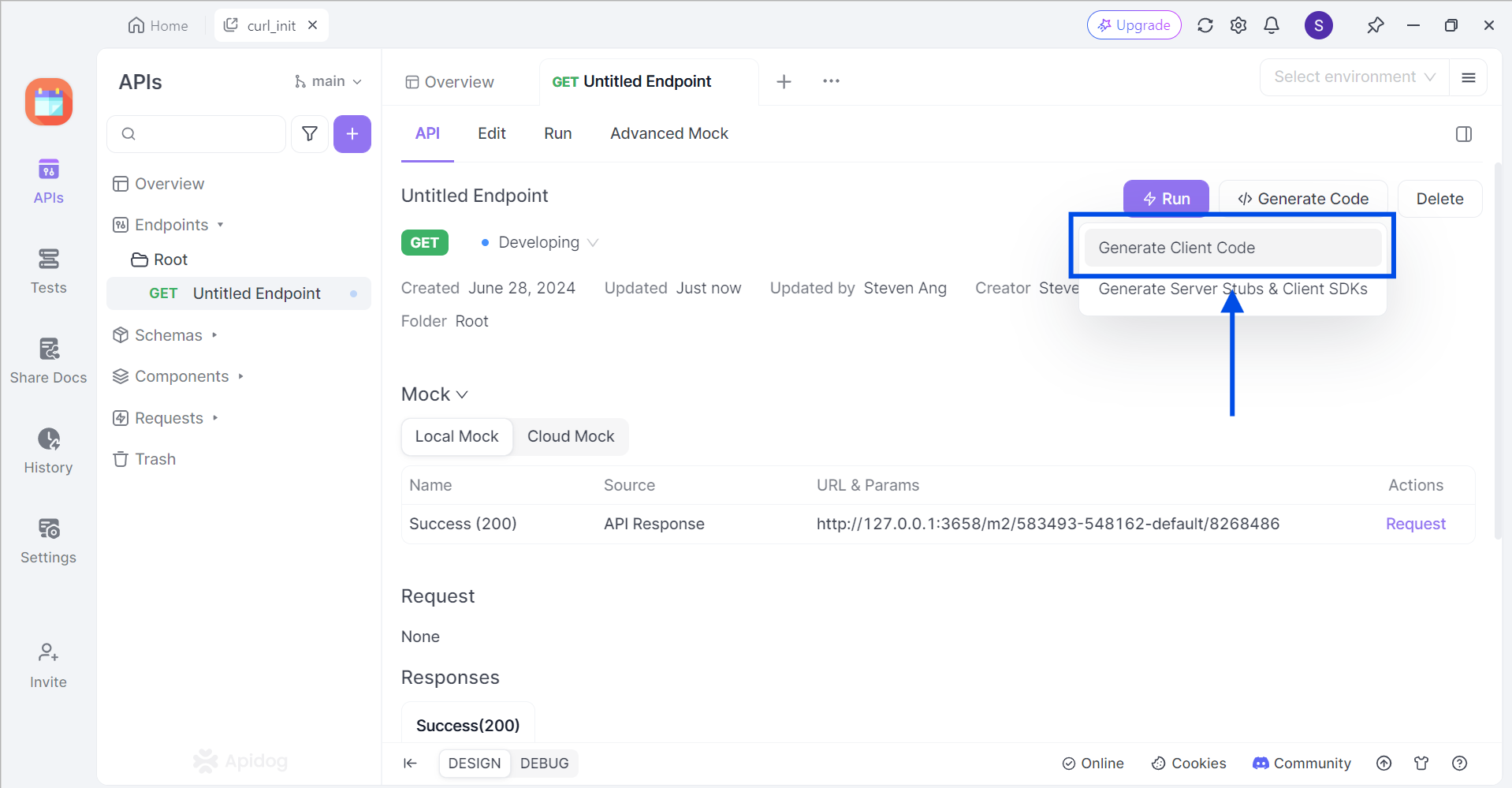
First, locate the </> Generate Code
button on any API or request, and select Generate Client Code
on the drop-down list.
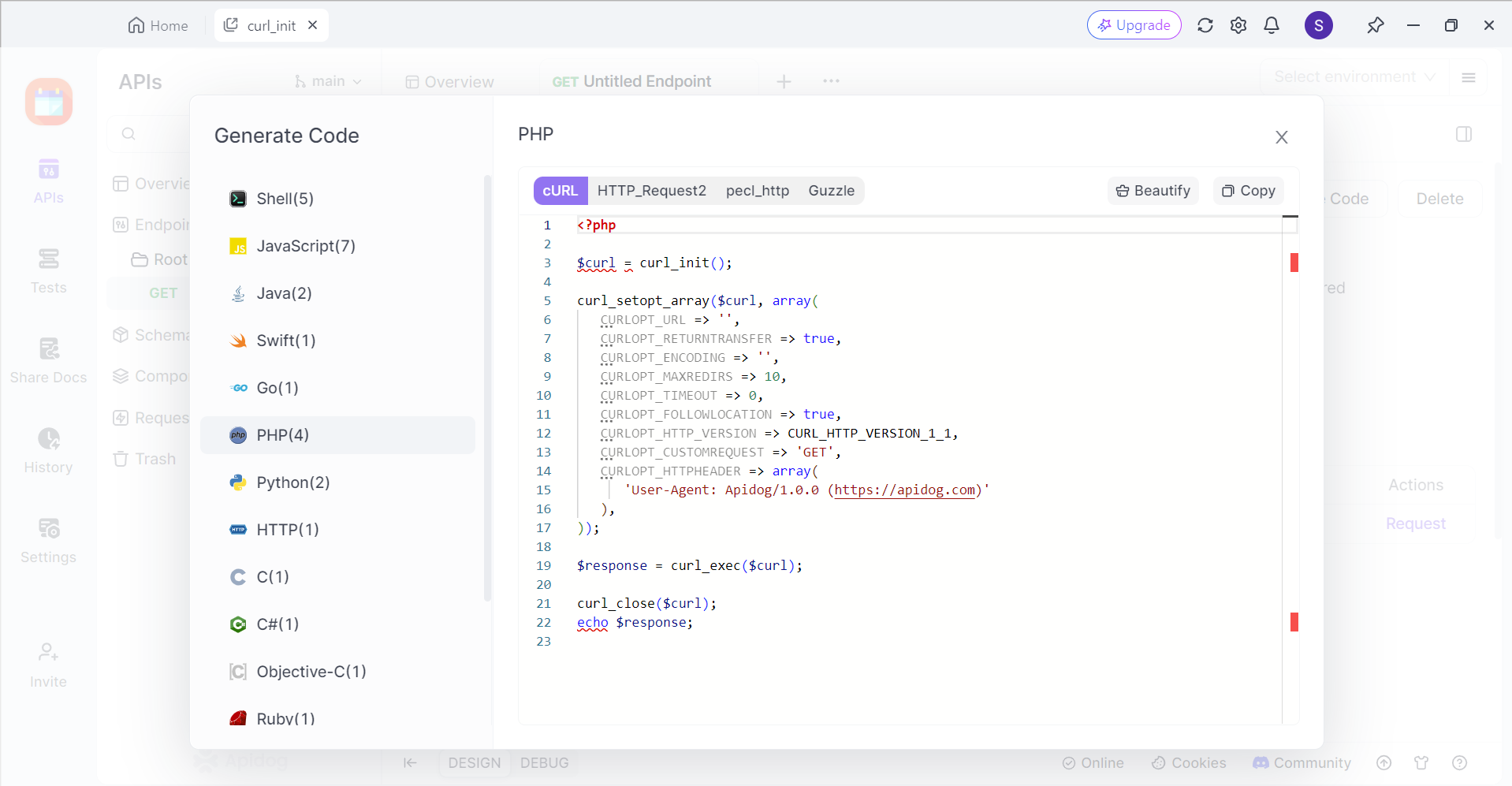
Next, select PHP, and find the cURL section. You should now see the generated code for cURL. All you have to do is copy and paste it to your IDE (Integrated Development Environment) and continue developing your application.
Conclusion
The curl_init() function within cURL empowers developers with a programmatic approach to data deletion. By crafting DELETE requests, resources on remote servers can be eliminated directly from within applications. This functionality proves invaluable for managing data lifecycles, synchronizing information across systems, and ensuring adherence to data governance regulations.
Furthermore, cURL offers versatility in crafting DELETE requests. From basic operations to those requiring authentication or complex data payloads, the library provides the necessary tools. By leveraging cURL's capabilities, developers can achieve precise control over data deletion within their software solutions.