SOAP web services and REST APIs are two popular technologies used by web developers. However, with SOAP APIs slowly getting replaced by the REST architectural style, are there any ways to utilize the existing SOAP web services?
If flexibility and simplicity are what you are looking for in an API tool, consider trying Apidog. All you have to do is click the button below to get started! 👇 👇 👇
Before getting into the technicalities of converting a SOAP API into a REST API, an introduction regarding what SOAP APIs and REST APIs will be provided, and a clarification of the differences and strengths of both SOAP and REST APIs.
What are SOAP APIs?
SOAP (Simple Object Access Protocol) APIs follow a standardized protocol established by the World Wide Web Consortium (W3C). Similar to other APIs, SOAP APIs are implemented to exchange information between different applications and systems.
SOAP API Key Characteristics
- XML-based: SOAP APIs rely on XML (Extensible Markup Language) to structure data in requests and responses. This ensures a consistent and well-defined format for communication.
- Structured and formal: Compared to other API styles, SOAP APIs are more structured and formal. They clearly define every operation, its parameters, and the expected structure of responses using a document called a WSDL (Web Services Description Language) file.
- Reliable and secure: SOAP APIs are known for their reliability and security. They often utilize encryption and digital signatures to ensure secure data transmission.
Common Use Cases for SOAP APIs
Financial services: The financial sector heavily relies on secure and reliable data exchange. SOAP APIs, with their robust security features and structured data format, are well-suited for tasks like:
- Inter-bank communication
- Secure money transfers
- Credit card processing
Supply chain management: Effective supply chains depend on seamless information exchange between various companies. SOAP APIs can facilitate this by:
- Sharing inventory levels
- Communicating order statuses
- Tracking shipments
Legacy system integration: Modern applications often need to interact with older systems that lack modern API capabilities. SOAP APIs act as this bridge by:
- Exposing functionalities of legacy systems
- Integrating these functionalities into newer workflows
What are REST APIs?
REST (Representational State Transfer) APIs, occasionally called RESTful APIs, follow the REST architectural style.
REST API Key Characteristics
- Stateless: SOAP APIs are known to be stateless, meaning that each request sent from an application to the server includes all the necessary information for processing, and the server doesn't maintain any information about the state of previous interactions. This makes SOAP APIs scalable and intuitive for developers to understand.
- Resource-based: REST APIs interact with resources identified using Uniform Resource Identifiers (URIs). These resources could represent real-world entities like users, products, and actions.
- Standard HTTP methods: REST APIs leverage standard HTTP methods like GET, POST, PUT, and DELETE for different operations.
GET: Retrieves information about a resource.
POST: Creates a new resource.
PUT: Updates an existing resource.
DELETE: Deletes a resource. - Lightweight and flexible: Compared to SOAP APIs, REST APIs are generally considered lighter and more flexible. They are easier to set up, use, and integrate with different applications and platforms.
Common Use Cases for REST APIs
Mobile applications: Mobile applications rely on REST APIs to interact with backend servers. This interaction allows them to:
- Fetch data: Retrieve information like product listings, news updates, or user profiles. If the REST API is made with React, you might also use the Fetch API to help retrieve data.
- Perform actions: Add items to a shopping cart, post comments, or submit forms.
- Send user information: Share location data, user preferences, or login credentials.
Web applications: Many web applications, both single-page and traditional, utilize REST APIs to communicate with web servers and databases. This communication enables them to:
- Retrieve content: Fetch dynamic content like search results, personalized recommendations, or user-generated content.
- Authenticate users: Allow users to log in, manage their accounts, and manage user data.
- Manage data: Create, update, and delete data like articles, user profiles, or product information.
Social media platforms: At the heart of social media interaction lies the exchange of information facilitated by REST APIs. These APIs enable users to:
- Share content: Post updates, stories, and other media content.
- Interact with each other: Like, comment, and share other users' content.
- Access platform functionalities: Utilize features like messaging, creating groups, and managing account settings.
Why Convert APIs from SOAP to REST?
Aside from one of the main factors that SOAP APIs are a lot more complex to use and understand, there are other reasons why many developers are updating their SOAP APIs and turning them into REST APIs.
1. Simplicity and ease of use: REST APIs are generally considered simpler and easier to use than SOAP APIs. REST uses standard HTTP methods like GET, POST, PUT, and DELETE, which are familiar to most developers.
SOAP relies on XML for both requests and responses, which can be more complex when compared to JSON or other data formats commonly used in REST APIs.
2. Flexibility and scalability: REST APIs are more flexible and scalable than SOAP APIs as REST is resource-based, allowing for a more modular and adaptable design. New functionalities can be added by introducing new resources and URIs.
Meanwhile, SOAP is message-based, making it less flexible and potentially more complex to scale as the API grows.
3. Wider adoption and tooling: REST APIs are more widely adopted and supported than SOAP APIs.
- Many development tools, frameworks, and libraries are readily available for building and interacting with REST APIs.
- This wider ecosystem simplifies development and integration for both REST API providers and consumers.
4. Modern development practices: REST APIs better align with modern development practices and trends:
- REST APIs tend to be lighter-weight, leading to faster communication and improved application user experience.
- REST APIs' stateless nature facilitates easier caching and load-balancing strategies.
5. Improved developer experience: Overall, converting to a REST API can improve the developer experience in several ways:
- Simpler learning curve: Developers with experience interacting with web APIs will find REST APIs more intuitive and easier to learn.
- Increased productivity: The availability of tools and support for REST APIs can lead to faster development and easier maintenance.
- Wider community support: Developers can benefit from a broader online community and readily available resources for troubleshooting and learning.
SOAP and REST API Code Samples (A Comparison)
This section below will showcase SOAP and REST APIs using the Python language, providing the same functionality: retrieving product information based on ID. (Please note that copying the code sample provided below may not necessarily work on your device, so ensure that modifications are made.)
SOAP API (with zeep
library for communication):
from zeep import Client
# Replace with the actual WSDL URL of the SOAP API
wsdl_url = "https://example.com/soap/product?wsdl"
# Create a SOAP client
client = Client(wsdl_url)
# Product ID to retrieve information for
product_id = 123
# Define the operation name
operation_name = "GetProductDetails"
# Send the SOAP request with product ID as parameter
response = client.service[operation_name](productId=product_id)
# Extract product name and price from the response (assuming structure)
product_name = response["productName"]
product_price = response["price"]
print(f"Product Name: {product_name}")
print(f"Product Price: {product_price}")
SOAP API Explanation:
- Import the
zeep
library: This library facilitates communication with SOAP APIs in Python. - Define the WSDL URL: If you plan to use the code sample, you can replace
https://example.com/soap/product?wsdl
with the actual WSDL URL of the SOAP API you want to interact with. - Create a SOAP client: The
Client
objectzeep
is created with the WSDL URL. - Define operation name: The operation name for the SOAP API is
GetProductDetails
. - Send the request: The SOAP request is sent with the product ID as a parameter and extracts the desired data from the response.
REST API (using requests
library):
import requests
# Replace with the actual base URL of the REST API
base_url = "https://example.com/api/products"
# Product ID to retrieve information for
product_id = 123
# Construct the API endpoint URL with the specific product ID
url = f"{base_url}/{product_id}"
# Send GET request to the REST API endpoint
response = requests.get(url)
# Check for successful response (status code 200)
if response.status_code == 200:
# Parse the JSON response data (assuming JSON format)
data = response.json()
product_name = data["name"]
product_price = data["price"]
print(f"Product Name: {product_name}")
print(f"Product Price: {product_price}")
else:
print(f"Error retrieving product information: {response.status_code}")
REST API Explanation:
- Import
requests
: therequests
library is used to interact with the REST API. - Define base URL: The specific endpoint URL for the desired product is created by appending the product ID at the end of the URL.
- Send a request: The REST API sends a request to the endpoint, and checks if it receives a successful response.
- Parsing data: If successful, the data from the JSON response is parsed for extraction, else, it handles the unsuccessful response.
Notable Key Differences Between SOAP and REST Processes
From the code samples, it can be observed that there are differences in:
- Communication protocol: SOAP uses SOAP messages over HTTP, while REST uses standard HTTP methods like GET and utilizes data formats like JSON or XML.
- Data format: SOAP uses XML for both requests and responses, while REST commonly uses JSON or other formats.
- Structure: SOAP relies on WSDL for defining the API, while REST relies on well-defined URIs and standard HTTP methods.
How to Convert SOAP APIs into REST APIs?
Although as observed from the code samples both SOAP and REST APIs can run with the same client language, the composition, protocols, and structure differ so much that it would be a very complex method.
However, there are essential steps to converting SOAP APIs into REST APIs, no matter how simple or complex the SOAP API is.
1. Analyze the SOAP API:
- Understand the WSDL: Thoroughly examine the WSDL document. It defines operations, parameters, and expected response structures.
- Identify resources: Map the functionalities and data involved in the API to relevant "resources" in a RESTful sense. These resources represent entities like users, products, or actions.
- Map operations to HTTP methods: Determine the most appropriate HTTP method (GET, POST, PUT, DELETE) for each operation based on its purpose (retrieving, creating, updating, deleting resources).
2. Design the REST API:
- Define RESTful URIs: Create clear and descriptive URIs for accessing resources using standard syntax. These URIs should reflect the hierarchy and relationships between resources.
Example:/products/{id}
for retrieving a specific product by ID. - Define data formats: Choose a suitable data format for request and response payloads, commonly JSON or XML. Consider factors like compatibility with your application and the size and complexity of data.
- Document the API: Document the REST API using a standard format like OpenAPI (Swagger) or Postman Collection. This documentation will become a reference for developers who want to interact with your API.
3. Implementation:
- Develop the API server: Implement the logic for handling requests and responses based on the defined RESTful design. This typically involves building a server application using your chosen programming language and framework.
- Handle data conversion: Implement logic to translate between SOAP and REST data formats (if necessary). This might involve parsing SOAP messages and converting them to the chosen REST format (e.g., JSON) for responses.
4. Testing and deployment:
- Thoroughly test the REST API: Ensure it accurately reflects the functionality of the original SOAP API and adheres to RESTful principles.
- Deploy the REST API: Make the API available to developers by deploying it to a suitable platform or server environment.
Apidog - Convert SOAP to Rest with 1 Click
Apidog is an all-inclusive, design-first-oriented API tool for developers to build APIs. With Apidog, API developers can build, test, document, and mock APIs.
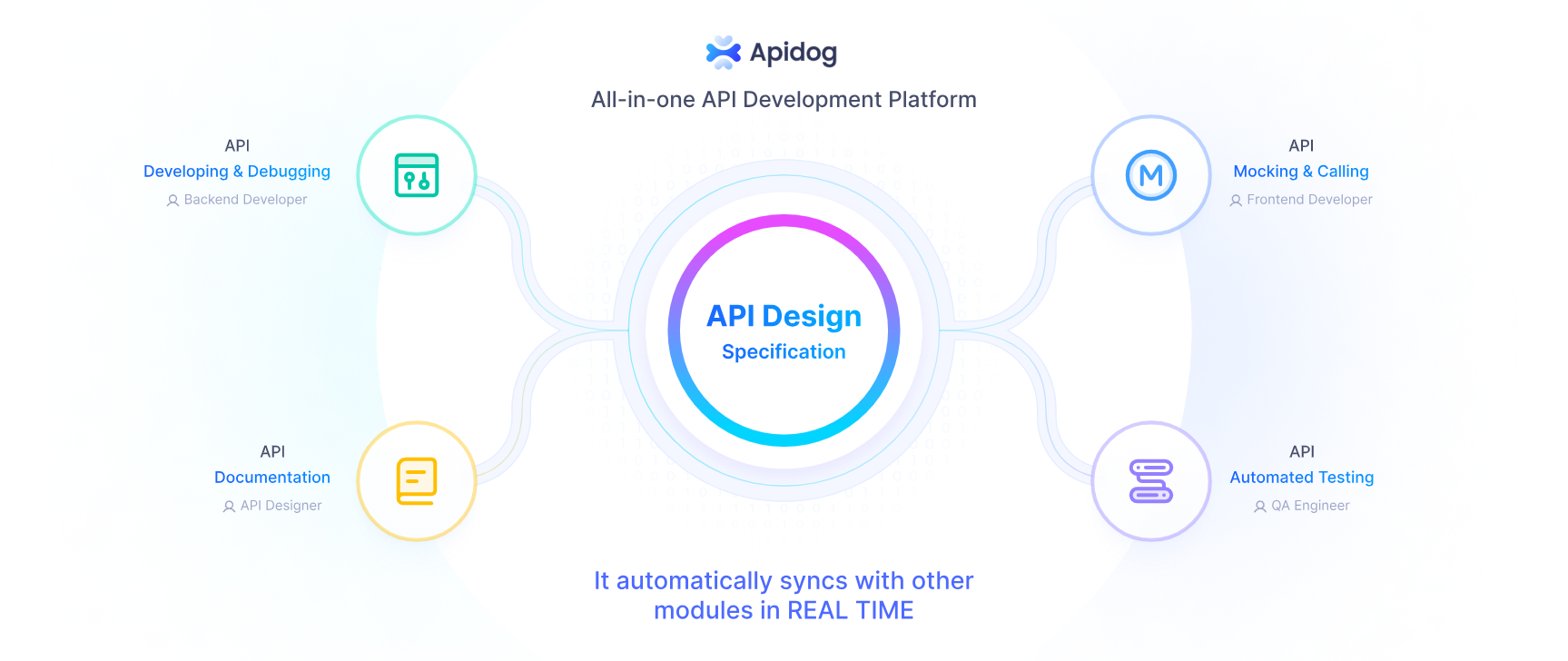
If you wish to view your SOAP APIs to convert them into REST APIs, Apidog has you covered. With facilitating modifications and specifications for the entire API lifecycle, Apidog can support every need an API developer seeks.
Importing SOAP-related WSDL File to Apidog
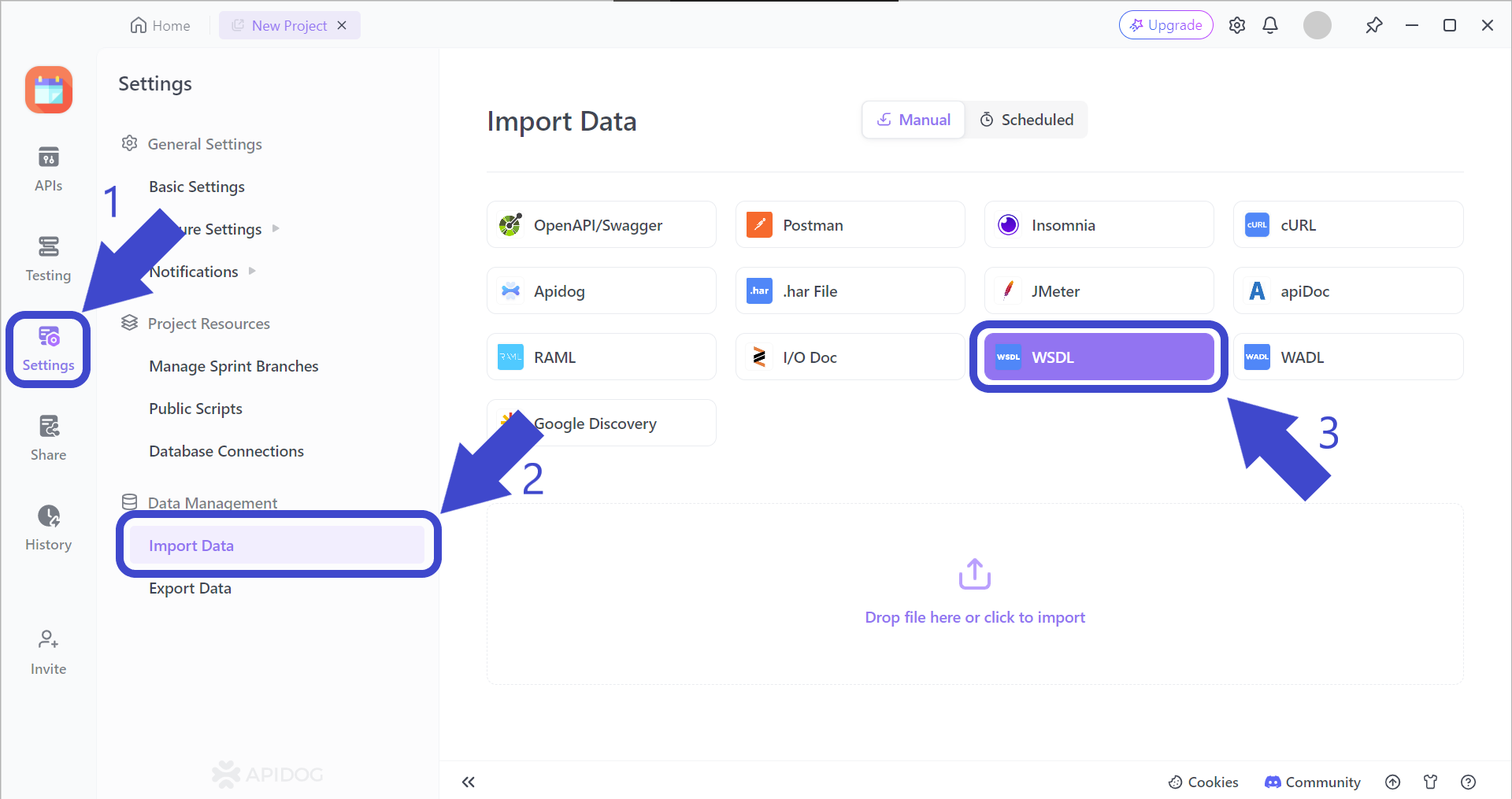
Under Settings
, you can import WSDL files by selecting the Import Data
section.
Exporting SOAP to REST With Apidog
After data is imported from WSDL file, you can see all the data of your SOAP API will be displayed in Apidog. You can modify them as you need.
With Apidog, you can convert your recently imported WSDL files into JSON files by choosing to export them. By exporting them, you can save the SOAP APIs as JSON files, which technically converts them into REST (As SOAP APIs are in XML markup).
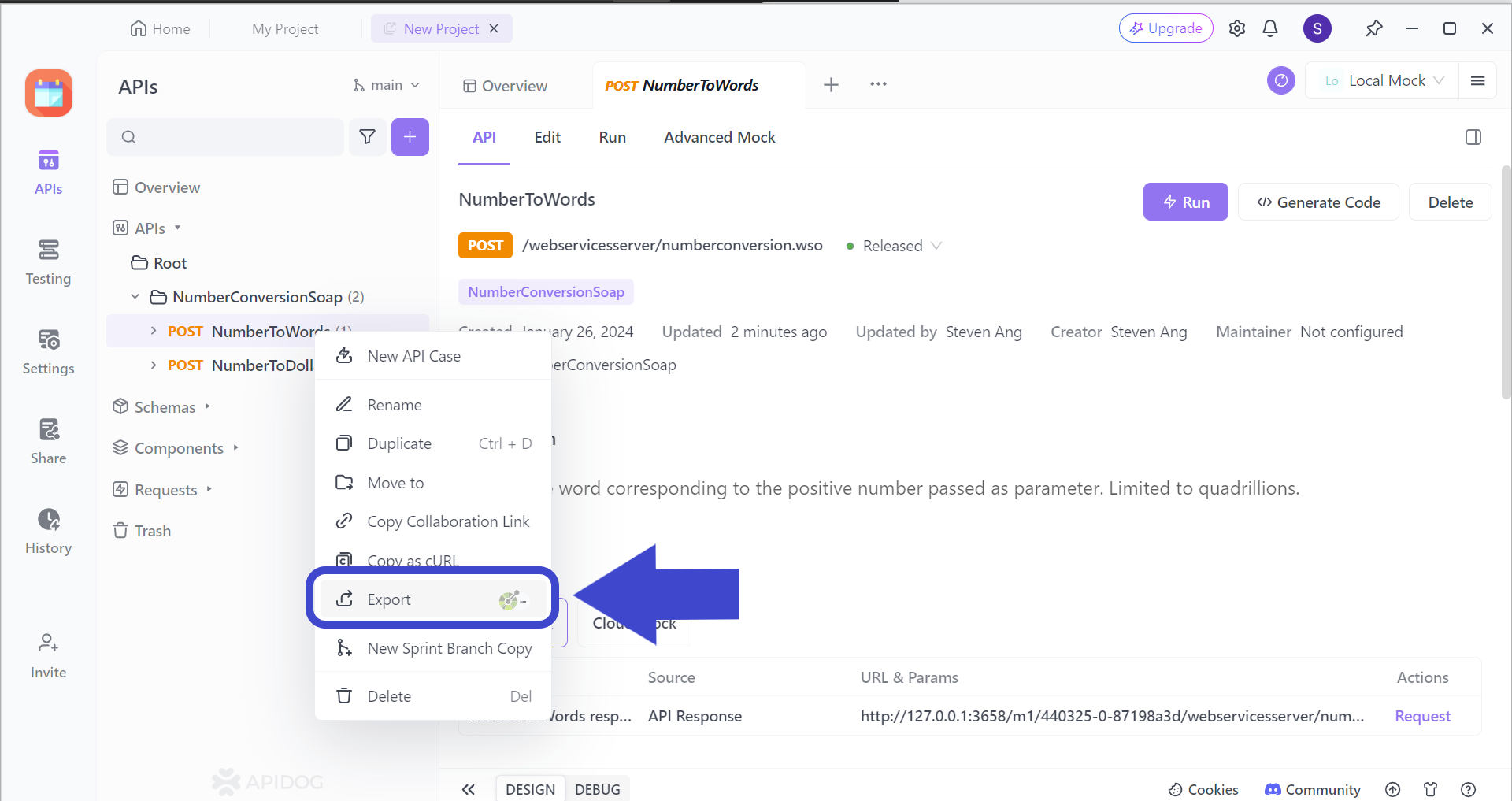
To begin file export, right-click on an API you would like to convert. Then, locate and press on Export
.
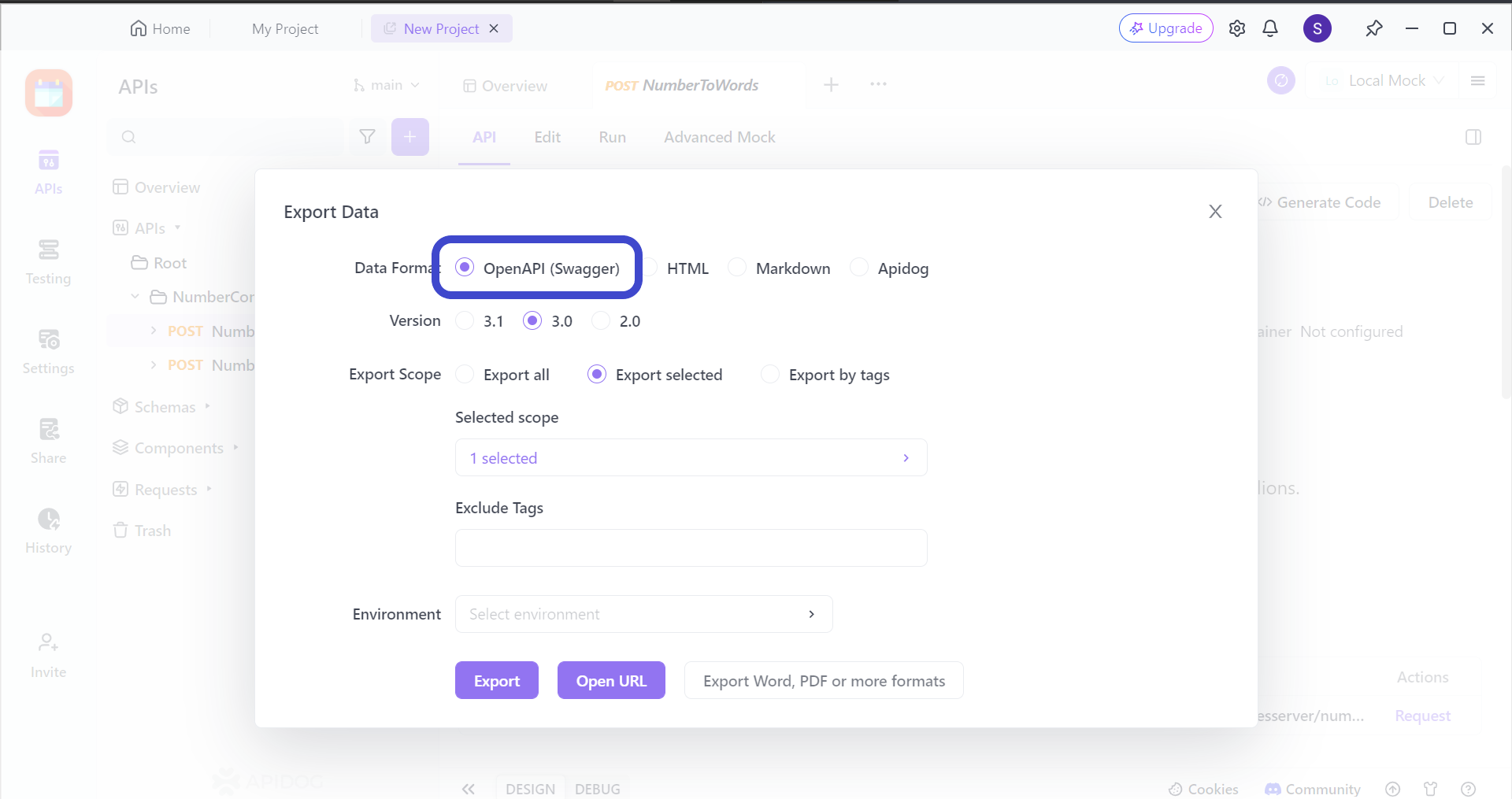
Lastly, select the OpenAPI (Swagger)
option to export the SOAP API as a JSON file.
Conclusion
Although converting SOAP APIs into REST APIs requires a lot of effort, it is becoming a necessity for a lot of API and web developers. SOAP APIs are slowly becoming overshadowed by REST APIs due ti how scalable and flexible REST APIs are. On top of that, REST APIs are a lot easier to understand and implement compared to SOAP APIs.
With Apidog, it may be possible to convert SOAP APIs into REST APIs. By importing WSDL files and exporting them as OpenAPI or Swagger files, you can obtain SOAP APIs in a JSON file type. If you want to learn more about the structure of your SOAP API, you can also view its details on Apidog, and conduct more testing and debugging with it.