The artificial intelligence landscape has witnessed a groundbreaking advancement with Anthropic's release of Claude 4 model family. Consequently, developers worldwide are eager to integrate these powerful language models into their applications. This comprehensive guide will walk you through the technical process of accessing both Claude Opus 4 and Claude Sonnet 4 APIs, enabling you to harness their advanced capabilities in your projects.
Understanding the Claude 4 Model Family Architecture
The Claude 4 family consists of two distinct models, each engineered for different performance requirements and computational needs.
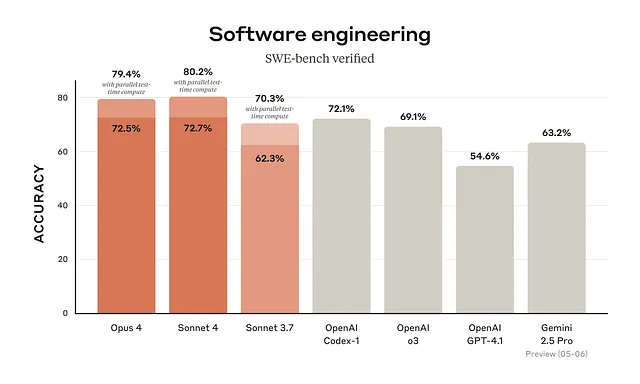
Claude Opus 4 represents the flagship model of the family, delivering unparalleled performance in complex reasoning tasks, advanced coding challenges, and sophisticated analysis. This model excels in scenarios requiring deep understanding, creative problem-solving, and nuanced decision-making processes. Furthermore, Claude Opus 4 demonstrates exceptional capabilities in multi-step reasoning, making it ideal for enterprise-level applications demanding high accuracy and comprehensive responses.
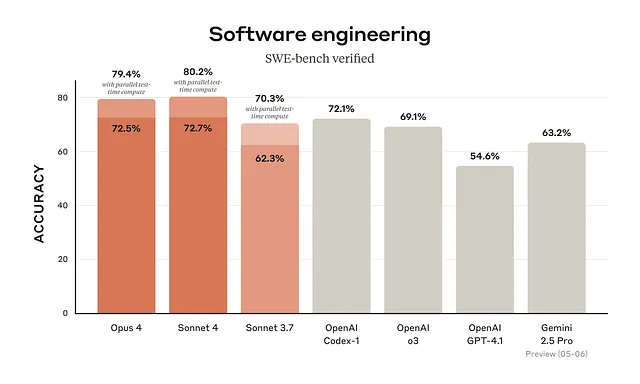
Claude Sonnet 4, on the other hand, focuses on efficiency without compromising quality. This model provides an optimal balance between performance and speed, making it perfect for everyday applications, rapid prototyping, and high-volume processing scenarios. Additionally, Claude Sonnet 4 offers cost-effective solutions for businesses requiring consistent AI assistance across multiple touchpoints.
Prerequisites and Account Setup Requirements
Successfully accessing Claude 4 APIs requires proper preparation and account configuration. Initially, you must establish an Anthropic developer account through the official console platform. This process involves several verification steps designed to ensure secure and authorized access to the API endpoints.
Creating Your Anthropic Developer Account
Navigate to the Anthropic Console and complete the registration process. The platform requires standard information including email verification, contact details, and intended use case descriptions. Moreover, Anthropic implements additional security measures for API access, including phone number verification and terms of service acceptance.
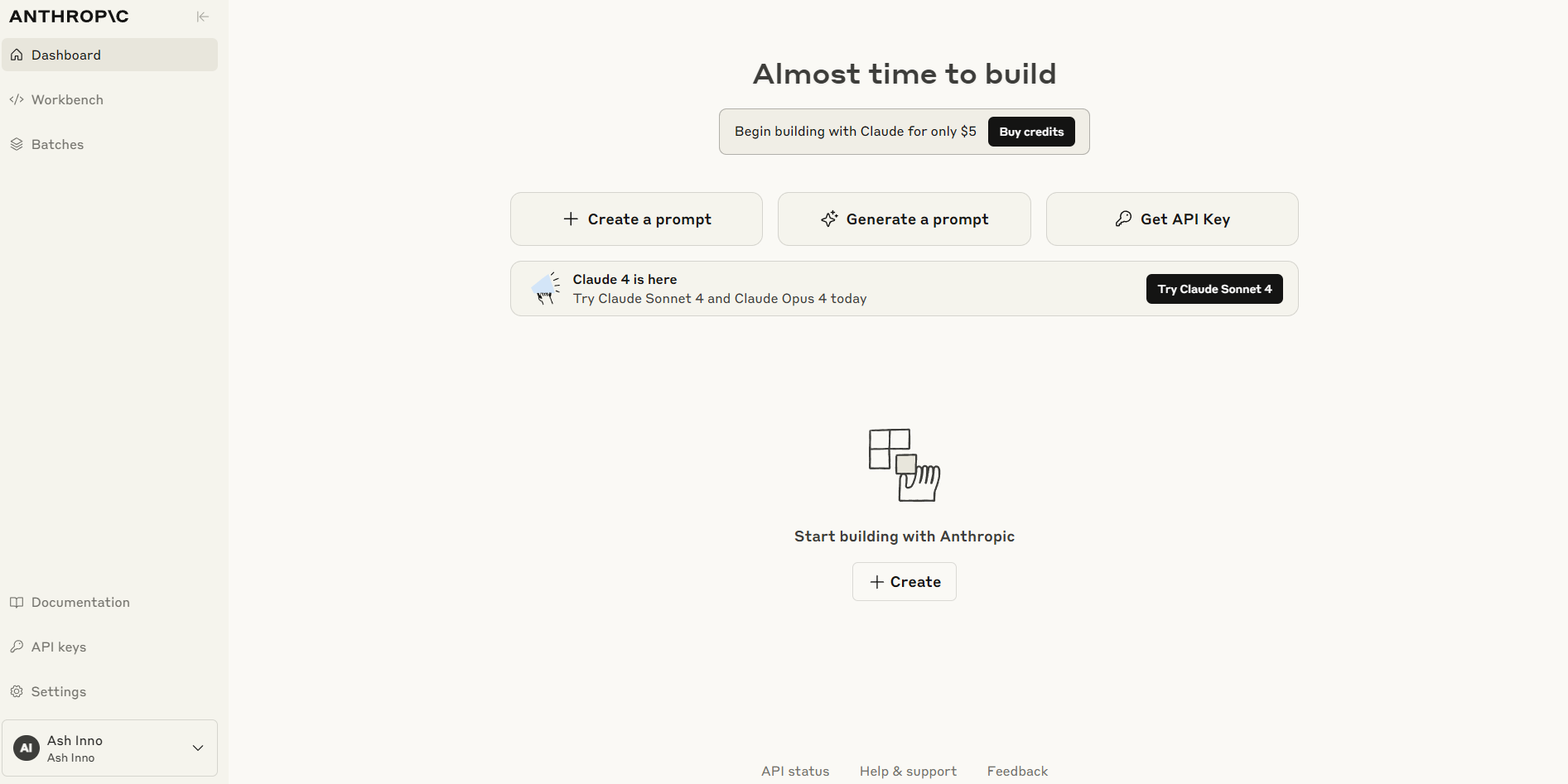
Once your account receives approval, you gain access to the developer dashboard where API key generation and usage monitoring occur. The dashboard provides comprehensive analytics, billing information, and model-specific configuration options essential for effective API management.
API Key Generation and Management
After successful account creation, generating your API keys becomes the next critical step. Navigate to the API section within your developer console and create new credentials specifically for Claude 4 access. Importantly, Anthropic provides different key types for various environments, including development, staging, and production deployments.
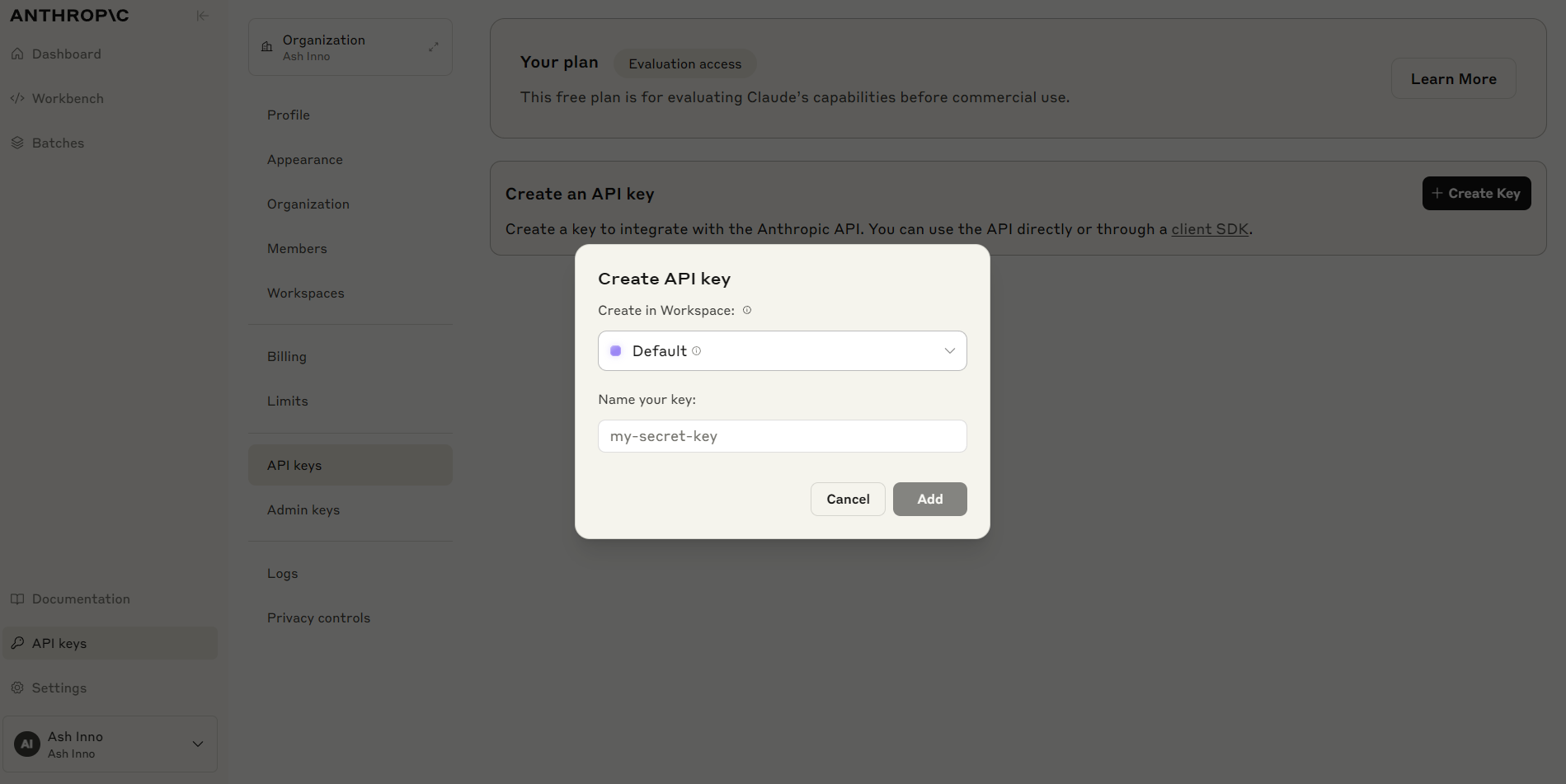
Security best practices demand careful handling of these credentials. Store your API keys securely using environment variables or dedicated secret management systems. Never hardcode API keys directly into your source code, as this practice exposes sensitive information and violates security protocols.
Claude Opus 4 API Integration Guide
Integrating Claude Opus 4 into your applications requires understanding its specific model string and configuration parameters. The model identifier for Claude Opus 4 is claude-opus-4-20250514
, which you must specify in your API requests to ensure proper model routing.
Basic API Request Structure
The fundamental structure of Claude Opus 4 API requests follows RESTful principles with specific headers and payload requirements. Each request must include authentication headers, content-type specifications, and properly formatted JSON payloads containing your prompts and configuration parameters.
import requests
import json
def call_claude_opus_4(prompt, max_tokens=1000):
url = "https://api.anthropic.com/v1/messages"
headers = {
"Content-Type": "application/json",
"X-API-Key": "your-api-key-here",
"anthropic-version": "2023-06-01"
}
payload = {
"model": "claude-opus-4-20250514",
"max_tokens": max_tokens,
"messages": [
{
"role": "user",
"content": prompt
}
]
}
response = requests.post(url, headers=headers, json=payload)
return response.json()
Advanced Configuration Parameters
Claude Opus 4 supports numerous configuration parameters that fine-tune model behavior according to your specific requirements. Temperature settings control response creativity, while top-p parameters influence token selection probability. Additionally, system messages enable you to establish consistent behavior patterns and role definitions for your AI assistant.
The temperature
parameter accepts values between 0 and 1, where lower values produce more deterministic outputs and higher values increase creativity and variability. Similarly, the top_p
parameter implements nucleus sampling, allowing precise control over response diversity and coherence.
Claude Sonnet 4 API Implementation
Claude Sonnet 4 integration follows similar patterns to Claude Opus 4 but utilizes the model string claude-sonnet-4-20250514
. This model optimizes for speed and efficiency while maintaining high-quality outputs, making it suitable for applications requiring rapid response times and cost-effective processing.
Optimizing for Performance and Cost
When implementing Claude Sonnet 4, consider request batching and connection pooling to maximize throughput and minimize latency. The model's architecture allows for efficient parallel processing, enabling applications to handle multiple concurrent requests without significant performance degradation.
Furthermore, implementing proper error handling and retry mechanisms ensures robust application behavior under various network conditions. Exponential backoff strategies prove particularly effective for managing rate limits and temporary service unavailability.
Real-time Integration Patterns
Claude Sonnet 4 excels in real-time applications such as chatbots, content generation systems, and interactive assistants. Implementing WebSocket connections or Server-Sent Events enables seamless streaming responses, providing users with immediate feedback and improved user experience.
async function streamClaudeSonnet4(prompt) {
const response = await fetch('https://api.anthropic.com/v1/messages', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-API-Key': process.env.ANTHROPIC_API_KEY,
'anthropic-version': '2023-06-01'
},
body: JSON.stringify({
model: 'claude-sonnet-4-20250514',
max_tokens: 1000,
stream: true,
messages: [{
role: 'user',
content: prompt
}]
})
});
return response.body;
}
Authentication and Security Best Practices
Securing your Claude 4 API integration requires implementing multiple layers of protection and following industry-standard security protocols. Authentication extends beyond simple API key usage to include request signing, rate limiting, and comprehensive access logging.
Implementing Secure Authentication Patterns
Deploy OAuth 2.0 flows for user-facing applications that require individual user authentication. This approach ensures proper attribution of API usage while maintaining user privacy and security. Additionally, implement JWT tokens for session management and API access control in distributed systems.
Rate limiting proves crucial for preventing abuse and managing costs effectively. Implement client-side rate limiting to respect API quotas while providing users with clear feedback about request status and remaining capacity.
Monitoring and Logging Strategies
Comprehensive logging enables effective debugging, performance optimization, and security monitoring. Log all API requests and responses while ensuring sensitive information remains protected. Furthermore, implement alerting systems for unusual usage patterns, error spikes, and security-related events.
Testing Claude 4 APIs with Apidog
Apidog emerges as an invaluable tool for testing and debugging Claude 4 API integrations. This comprehensive API testing platform provides intuitive interfaces for crafting requests, analyzing responses, and automating testing workflows. Moreover, Apidog supports collaborative development environments where teams can share API configurations and testing scenarios.
Setting Up Apidog for Claude Testing
Configure Apidog environments for different Claude 4 models by creating separate workspace configurations. This approach enables seamless switching between Claude Opus 4 and Claude Sonnet 4 testing scenarios while maintaining organized project structure.
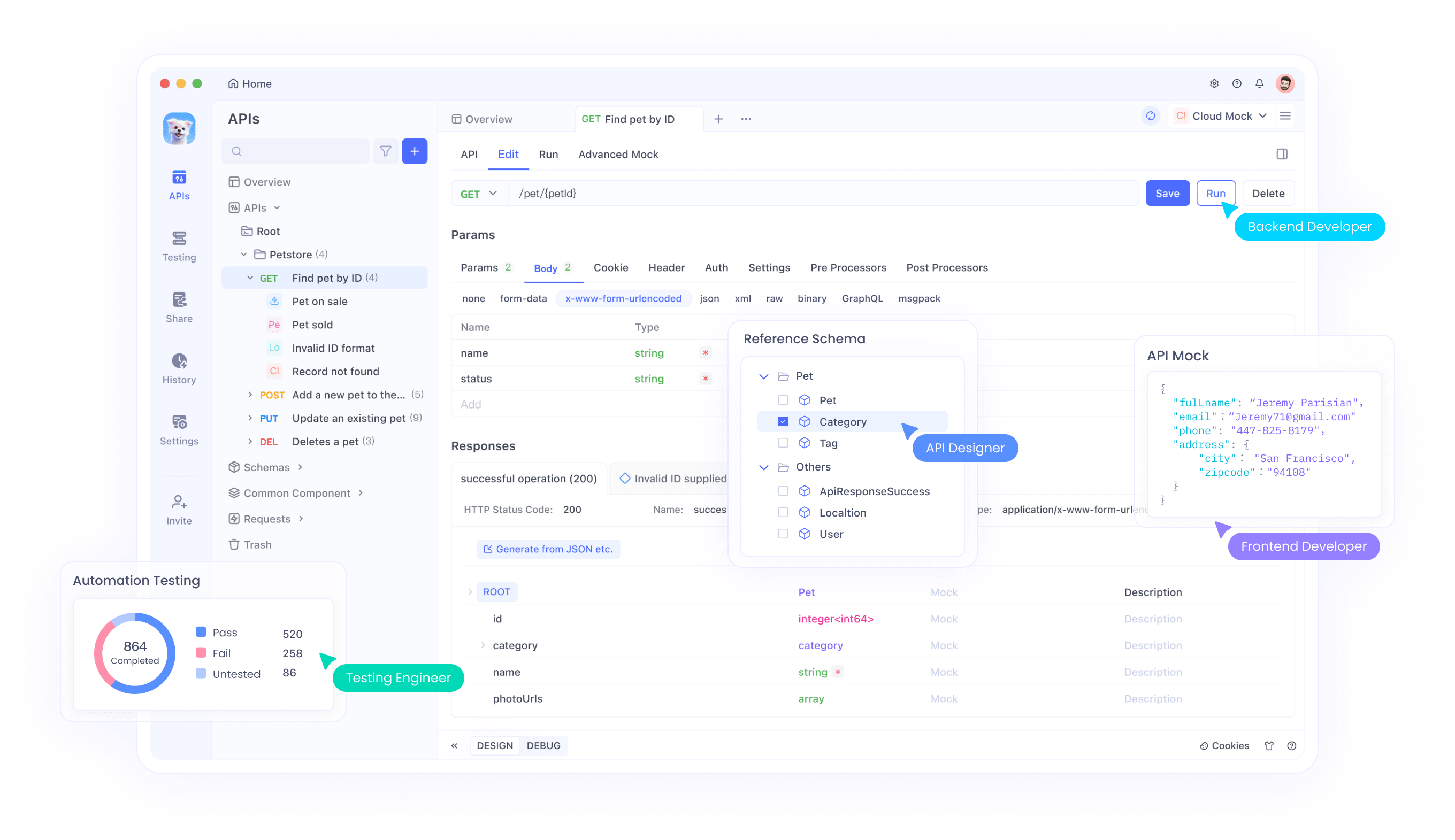
Import your API specifications into Apidog to generate automatic documentation and testing templates. The platform's intelligent parsing capabilities recognize Claude API patterns and suggest optimal testing configurations.
Advanced Testing Scenarios
Develop comprehensive test suites covering various prompt types, parameter combinations, and edge cases. Apidog's scripting capabilities enable automated validation of response quality, performance metrics, and error handling behaviors.
Furthermore, implement load testing scenarios to evaluate API performance under different traffic conditions. This testing approach helps identify optimal configuration parameters and scaling requirements for production deployments.
Cost Management and Billing Considerations
Understanding Claude 4 pricing structures enables effective cost management and budget planning. Both models utilize token-based pricing with different rates reflecting their respective capabilities and computational requirements.
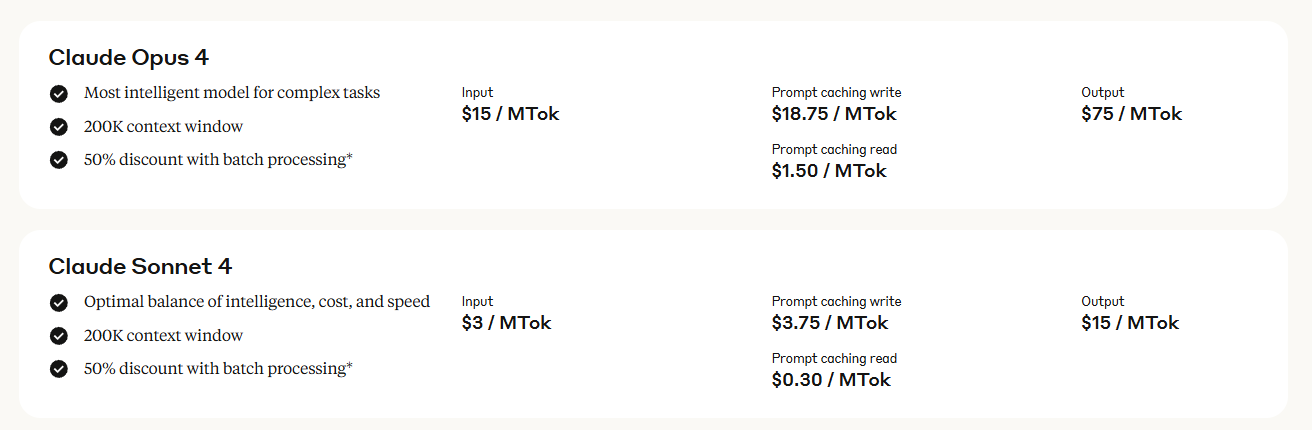
Token Usage Optimization
Monitor token consumption patterns to identify optimization opportunities and cost reduction strategies. Implement token counting mechanisms in your application to provide users with real-time usage feedback and prevent unexpected charges.
Furthermore, analyze prompt efficiency to minimize unnecessary token usage while maintaining response quality. This analysis often reveals opportunities for significant cost savings through prompt restructuring and optimization.
Budget Monitoring and Alerts
Establish comprehensive billing alerts and usage monitoring systems to prevent unexpected costs and ensure sustainable API usage. Implement automated scaling and throttling mechanisms that respond to usage patterns and budget constraints.
Production Deployment Considerations
Deploying Claude 4 integrations to production environments requires careful planning and comprehensive testing. Consider scalability requirements, availability targets, and disaster recovery procedures during the deployment planning phase.
Scalability and Load Management
Design your integration architecture to handle varying load patterns and traffic spikes effectively. Implement auto-scaling mechanisms that respond to demand fluctuations while maintaining cost efficiency.
Furthermore, consider geographic distribution and CDN integration to minimize latency for global user bases. This approach ensures consistent performance regardless of user location.
Monitoring and Maintenance
Establish comprehensive monitoring systems that track API performance, error rates, and user satisfaction metrics. Implement automated health checks and alerting systems to ensure rapid response to service disruptions.
Additionally, develop maintenance procedures for handling API updates, model changes, and security patches. Regular maintenance ensures continued reliability and optimal performance.
Future Considerations and Roadmap
The Claude 4 model family represents the current state-of-the-art in language model capabilities, but continued evolution remains certain. Stay informed about upcoming releases, feature additions, and deprecation schedules to maintain optimal integration performance.
Staying Updated with API Changes
Monitor Anthropic's official communication channels for announcements regarding API updates, new features, and breaking changes. Implement versioning strategies that enable smooth transitions between API versions.
Furthermore, participate in developer communities and forums to share experiences and learn from other Claude 4 integrators. This collaboration often reveals optimization techniques and best practices not covered in official documentation.
Conclusion
Successfully integrating Claude Opus 4 and Claude Sonnet 4 APIs requires understanding their unique characteristics, implementing proper security measures, and following optimization best practices. Both models offer powerful capabilities that can transform your applications and provide exceptional user experiences.
Remember that effective API integration extends beyond initial implementation to include ongoing monitoring, optimization, and maintenance. By following the guidelines outlined in this comprehensive guide, you can build robust, scalable, and cost-effective Claude 4 integrations that deliver consistent value to your users.
The combination of proper planning, thorough testing with tools like Apidog, and continuous optimization ensures successful Claude 4 API integration that scales with your application's growth and evolving requirements.
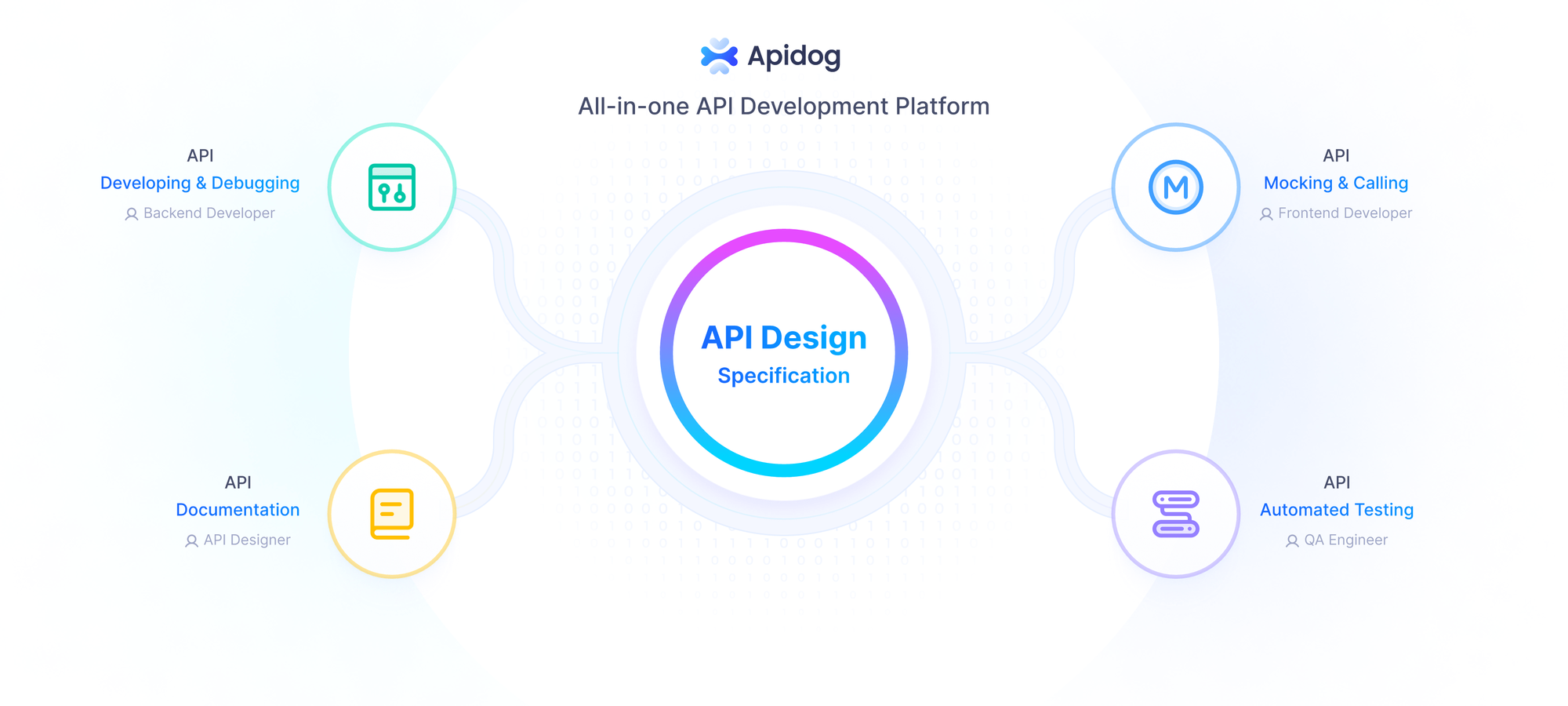