Git is one of the most widely used version control systems in software development. As you work on projects, you'll inevitably make commits that you later wish to undo, modify, or remove completely. This tutorial focuses specifically on how to cancel Git commits using the rebase command, which provides powerful options for manipulating your commit history.
Whether you've accidentally committed sensitive information, included broken code, or simply want to clean up your commit history, understanding how to cancel Git commits is an essential skill for every developer.
Streamline Your API Development with Apidog
While managing your Git commits effectively is crucial for version control, having the right tools for API development and testing is equally important. Consider switching to Apidog, the best Postman alternative on the market today.
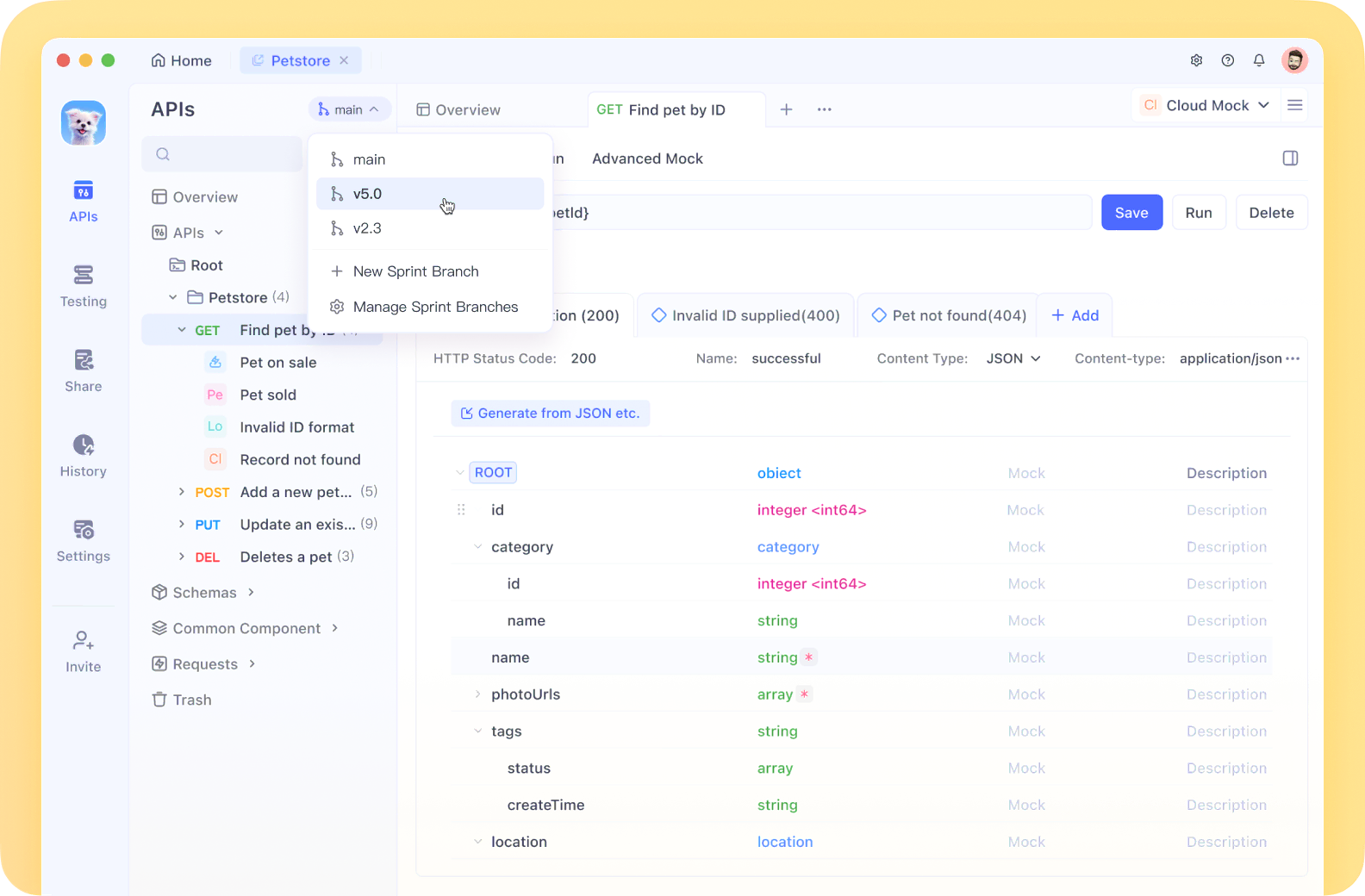
Apidog combines API documentation, design, mock, testing, and collaboration in a single platform, eliminating the need to switch between multiple tools.
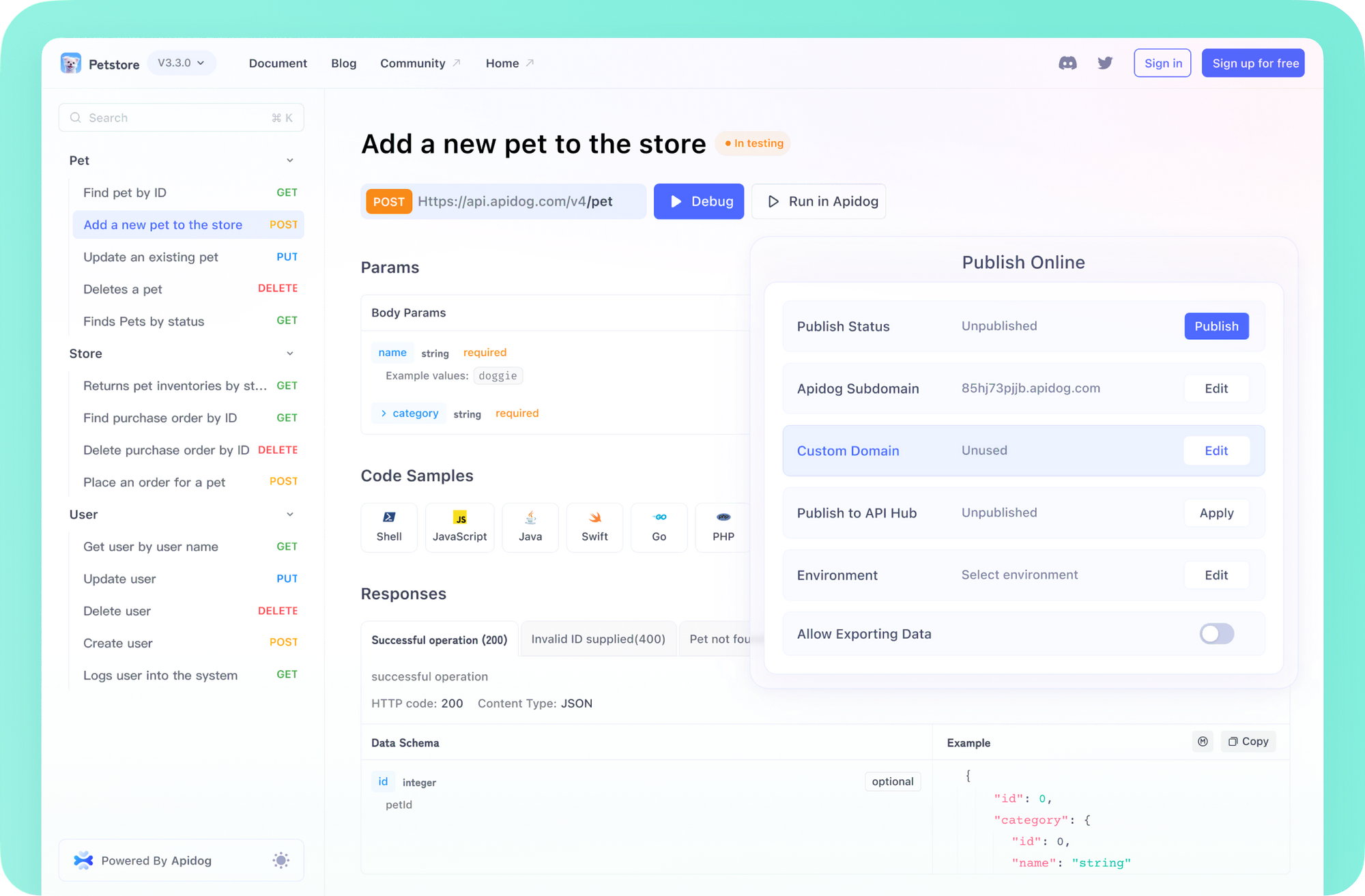
With its intuitive interface, automatic synchronization capabilities, and comprehensive features, Apidog enhances productivity and streamlines the entire API development lifecycle.
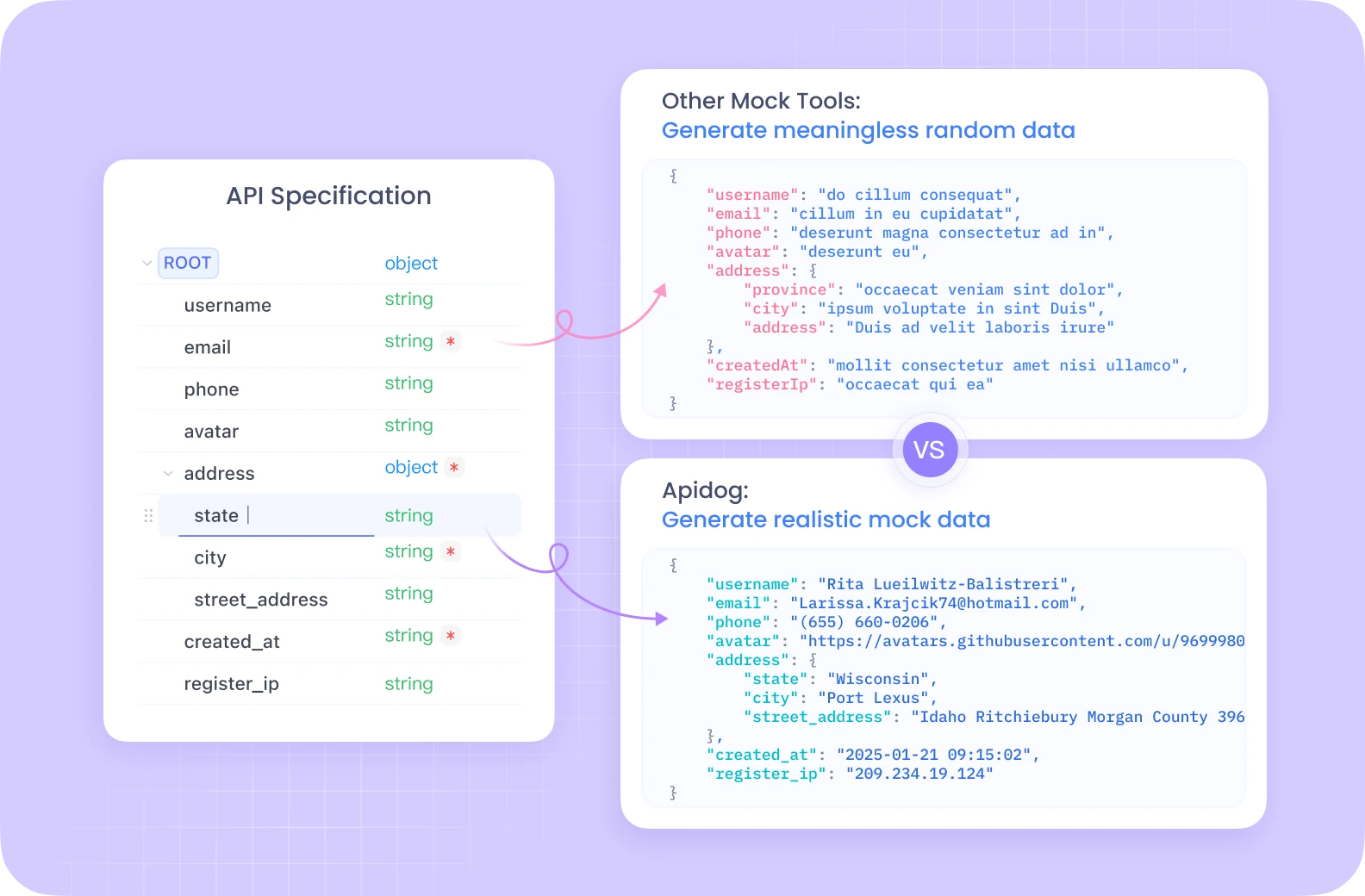
As we dive into Git rebase techniques, keep in mind that adopting efficient tools like Apidog can further optimize your development workflow.
Understanding Git Commit Structure
Before diving into cancellation techniques, let's review how Git stores commit information. Every Git commit:
- Has a unique hash identifier
- Points to its parent commit(s)
- Contains metadata (author, date, commit message)
- Stores a snapshot of your code at that point in time
When you need to cancel a Git commit, you're essentially wanting to alter this commit chain in some way. Depending on your specific needs, there are several approaches you can take.
Git Revert vs. Git Reset vs. Git Rebase: Choosing the Right Git Tool
There are three main ways to cancel a Git commit:
1. Git Revert
git revert HEAD
This creates a new commit that undoes changes from a previous commit. Your original commit remains in history, but its changes are reversed.
Best for: When you want to keep a record that the commit happened but undo its effects. This is the safest option when working with shared repositories.
2. Git Reset
git reset --soft HEAD^
This moves your branch pointer to a previous commit. The --soft
flag keeps your changes in staging, while --hard
would discard them completely.
Best for: When you want to completely remove recent commits from history and haven't shared your work yet.
3. Git Rebase
git rebase -i HEAD~n # where n is the number of commits to show
This allows you to manipulate commit history in various ways, including deleting commits entirely.
Best for: Advanced Git users who want precise control over their commit history.
The Git Rebase Command: A Powerful History Manipulation Tool
The rebase command is one of Git's most powerful features. Unlike merge, which creates a new commit to integrate changes, rebase rewrites commit history by applying commits on top of another base commit.
The interactive rebase (-i
flag) is particularly useful for canceling commits, as it allows you to:
- Delete commits
- Squash multiple commits
- Reorder commits
- Edit commit messages
- Split commits
While powerful, rebase should be used with caution since it rewrites Git history, which can create problems in collaborative environments.
How to Cancel a Git Commit Using Git Rebase
Let's walk through the process of canceling a Git commit using the rebase command:
Step 1: Start an Interactive Git Rebase Session
First, determine how far back in history you need to go. If you want to cancel the last three commits, you'd use:
git rebase -i HEAD~3
This will open your default text editor with a list of commits, most recent at the bottom:
pick f2a9770 Add feature X
pick c69a283 Fix bug in feature X
pick 7c6b236 Update documentation
Step 2: Delete the Git Commit
To cancel a commit, change the word "pick" to "d" (or "drop") for the commit you want to remove:
pick f2a9770 Add feature X
d c69a283 Fix bug in feature X
pick 7c6b236 Update documentation
In this example, we're removing the "Fix bug in feature X" commit while keeping the other two.
Step 3: Save and Exit
Save the file and close the editor. Git will process your instructions and apply the changes.
Step 4: Resolve Any Git Conflicts
If there are conflicts between commits after removing a commit, Git will pause the rebase process and ask you to resolve them. After resolving conflicts:
git add .
git rebase --continue
Step 5: Force Push If Necessary
If you've already pushed the commits you've now canceled, you'll need to force push to update the remote branch:
git push --force-with-lease
WARNING: Force pushing rewrites history on the remote repository, which can cause problems for other developers who have pulled the old history. Only use force push when you're certain it won't disrupt others' work.
Advanced Git Rebase Techniques for Commit Management
Beyond simply canceling commits, interactive rebase offers several other options:
Squashing Git Commits
You can combine multiple commits into one:
pick f2a9770 Add feature X
squash c69a283 Fix bug in feature X
pick 7c6b236 Update documentation
This will combine the "Fix bug" commit into the "Add feature" commit.
Reordering Git Commits
Simply change the order of lines in the rebase editor:
pick 7c6b236 Update documentation
pick f2a9770 Add feature X
Editing Git Commits
Use edit
instead of pick
to pause the rebase at a specific commit:
pick f2a9770 Add feature X
edit c69a283 Fix bug in feature X
pick 7c6b236 Update documentation
When the rebase reaches this commit, Git stops and allows you to amend the commit before continuing.
Git Rebase Risks and Best Practices
Risks of Using Git Rebase
- Irreversible Changes: Unlike merge, rebase rewrites commit history, making it difficult to recover from mistakes.
- Force Push Required: After rebasing pushed commits, you'll need to force push, which can overwrite others' changes.
- Commit Alteration: Rebase changes commit hashes, which means the original commits are replaced with new ones.
- Conflicts: Complex rebases can lead to multiple conflict resolution steps.
Git Rebase Best Practices
Create Backup Branches: Before attempting complex rebase operations, create a backup branch.
git branch backup-before-rebase
Only Rebase Unpushed Commits: As a general rule, avoid rebasing commits that have been pushed to a shared repository.
Use -force-with-lease
Instead of -force
: This provides a safety check to prevent overwriting others' changes.
git push --force-with-lease
Practice in a Sandbox Repository: If you're new to rebasing, practice in a test repository before using it on important projects.
Only Rebase Your Own Feature Branches: Avoid rebasing branches that multiple developers are working on.
When to Use Each Git Cancellation Method
- Use Git Revert when:
- You need to undo changes but keep a record of the original commit
- You're working in a shared branch like main/master
- You want the safest option
- Use Git Reset when:
- You want to completely remove recent commits
- You haven't shared your commits with others
- You're working on a local feature branch
- Use Git Rebase when:
- You want fine-grained control over your commit history
- You need to clean up your commit history before sharing
- You're comfortable with advanced Git operations
Git Rebase vs. Git Merge: Understanding the Difference
While not directly related to canceling commits, understanding the difference between rebase and merge helps clarify why rebase is powerful for history manipulation:
- Merge creates a new commit that combines changes from different branches, preserving the complete history of both branches.
- Rebase rewrites history by moving commits from one branch onto another, creating a linear history instead of a branched one.
This difference is key to why rebase can be used to cancel commits—it doesn't just add new commits; it rewrites history.
Conclusion
The Git rebase command is a powerful tool for canceling commits and maintaining a clean, meaningful commit history. While it requires care and attention to use properly, mastering rebase gives you precise control over your Git history.
Remember that the safest approach depends on your specific situation:
- If you've shared your work, consider using
git revert
- If you're working locally,
git reset
orgit rebase
may be appropriate - Always create backup branches before attempting complex Git operations
By understanding the various approaches to canceling Git commits, you'll be better equipped to handle mistakes and maintain a clean repository history. Practice these techniques in a safe environment until you're comfortable applying them to your actual projects.