Imagine giving your Cursor IDE superpowers - like automatically searching the web or analyzing your documents without leaving your editor. In this tutorial, we'll walk through creating a custom MCP (Model Context Protocol) server that adds these exact capabilities to Cursor.
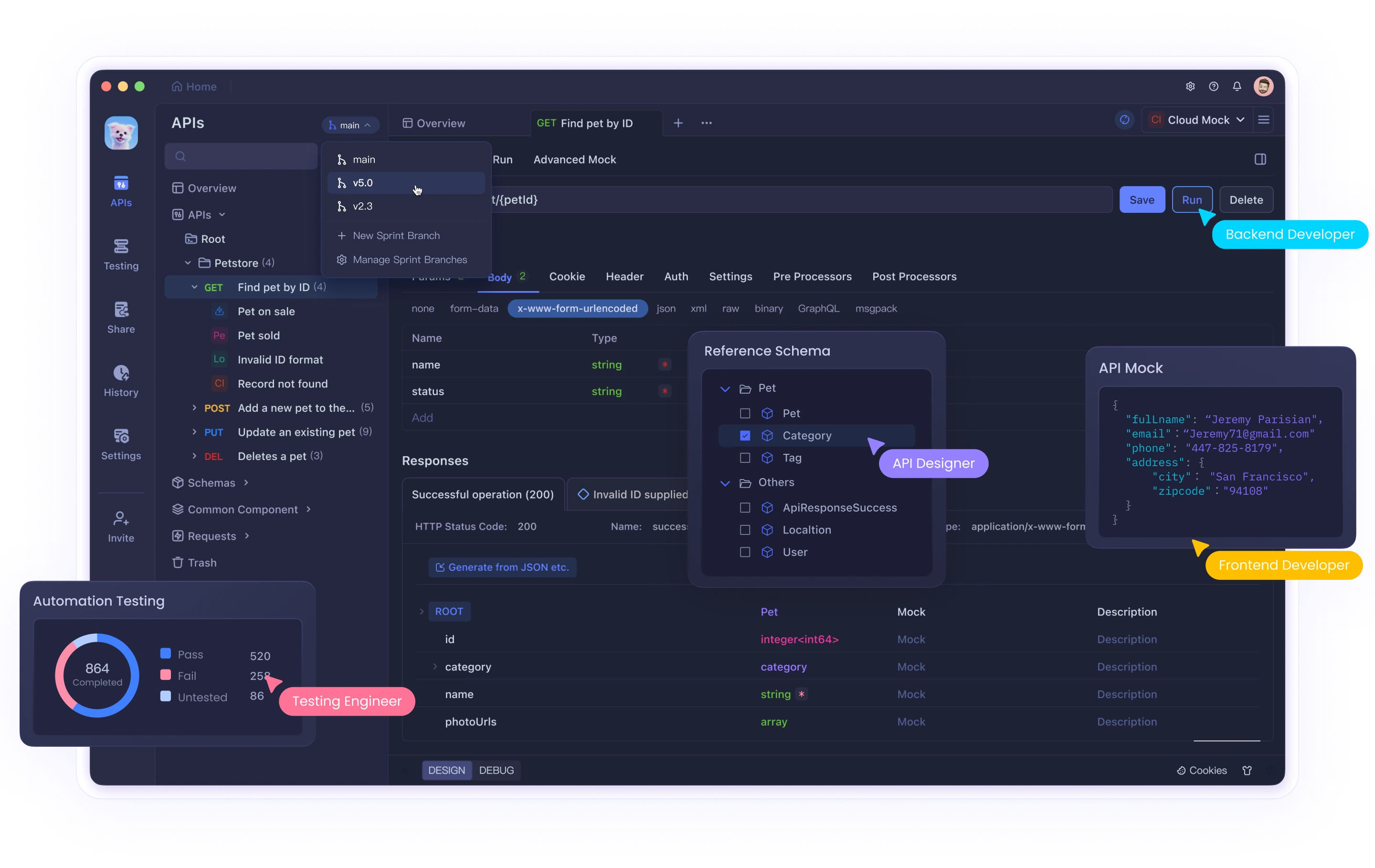
Why Build a Custom MCP Server?
MCP servers let you extend Cursor's functionality beyond its built-in features. With your own MCP server, you can:
- Add web search capabilities
- Create tools for analyzing your documents (RAG)
- Connect to specialized APIs
- Build custom AI workflows
Recent updates make MCP server development easier than ever - perfect for beginners!
Step 1: Setting Up Your Development Environment
Prerequisites
Before we begin, make sure you have:
- Cursor IDE (latest version)
- Python 3.8+ installed
- UV package manager (we'll install this below)
- Basic familiarity with terminal commands
Getting the Starter Template
We'll use a ready-made template to get started quickly:
- Clone the repository:
git clone https://github.com/patchy631/ai-engineering-hub/tree/main/cursor_linkup_mcp
- Open the folder in Cursor IDE
Step 2: Setting Up the MCP Server in Cursor
In Cursor, go to:
Settings > Cursor Settings > MCP > Add New MCP Server
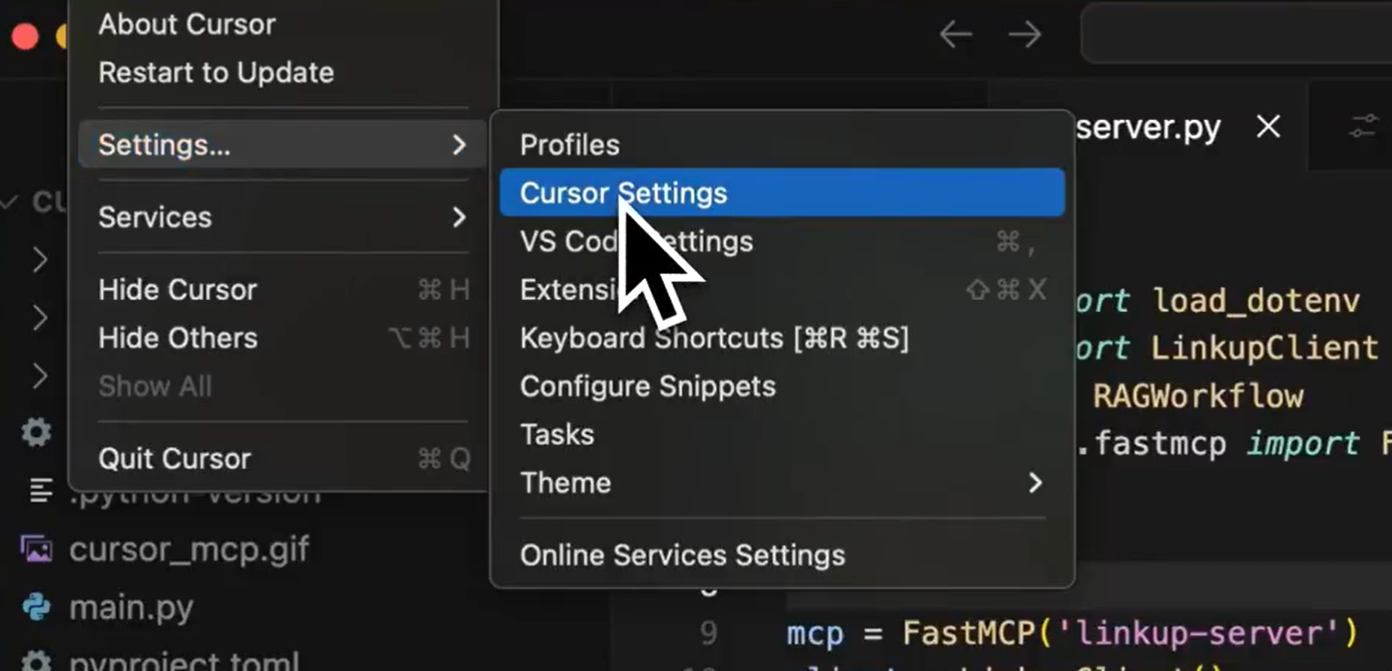
Configure your server:
- Name: Give it a descriptive name (e.g., "twitter-demo")
- Command: We'll use the UV package manager
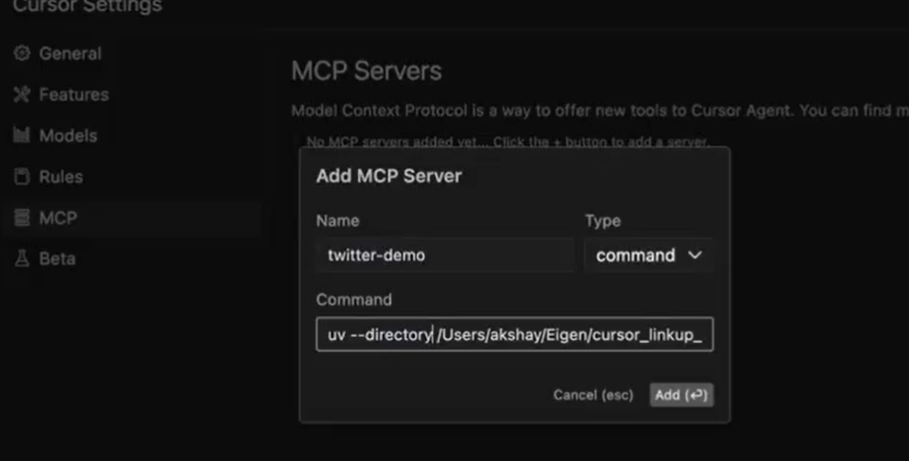
If you don't have UV installed:
pip install uv
Set the command to run your server:
uv --directory /path/to/cursor_linkup_mcp run server.py
(Replace /path/to/
with the actual location where you cloned the repository)
Click "Add" to save your configuration
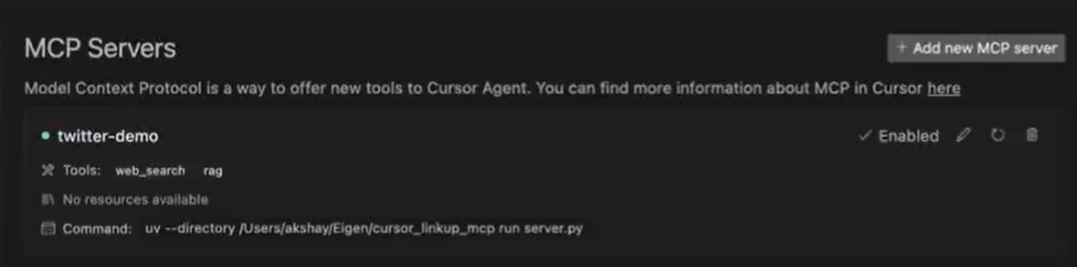
Step 3: Testing Your New Tools
Now that your server is set up, let's test its capabilities:
1. Web Search Tool
This allows Cursor to search the web for answers to your questions.
How to use:
- Open a new chat in "Agent" mode
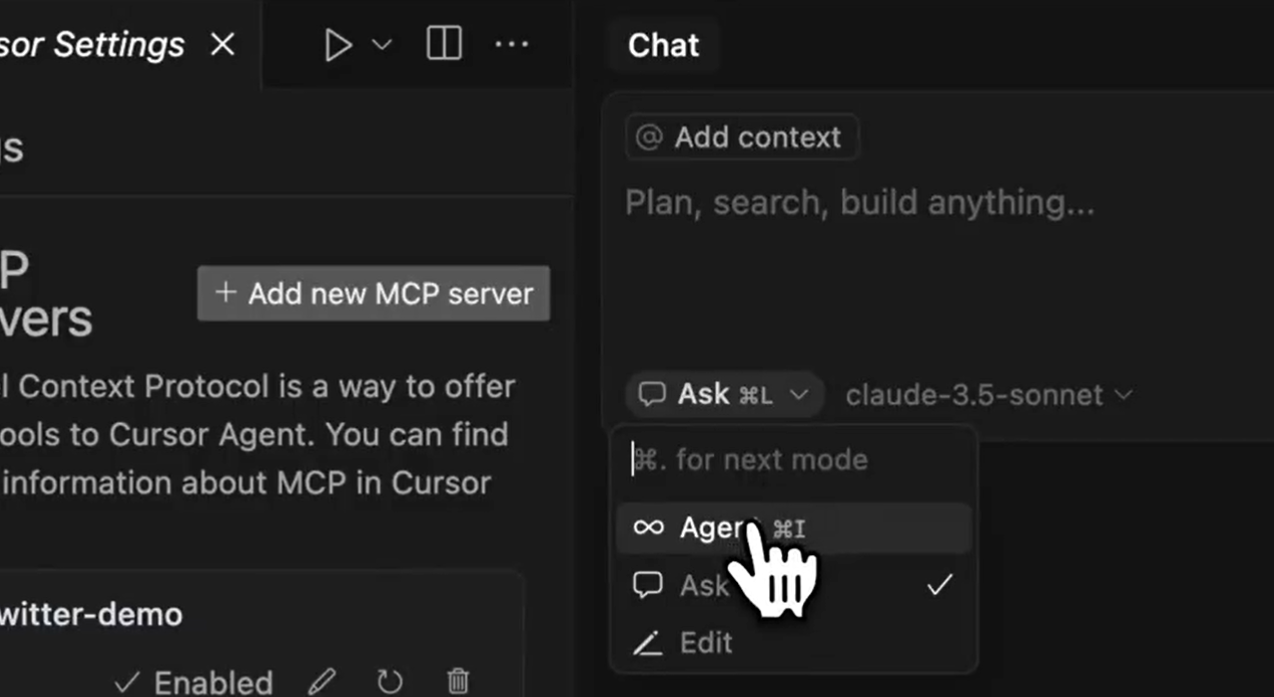
2. Ask a question that requires web lookup, like:
>> Who won the latest cricket match between India and Australia?
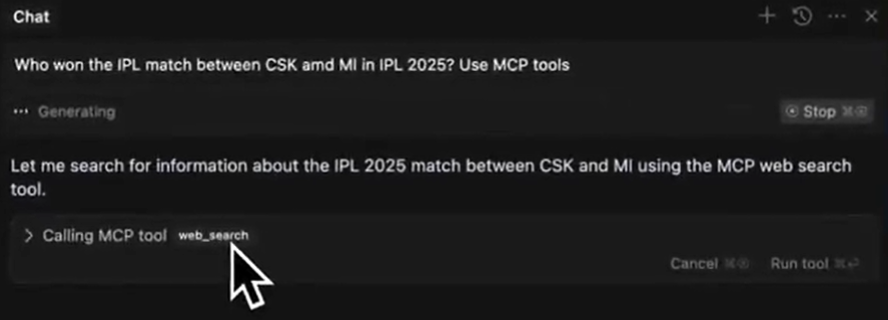
3. Cursor will use your MCP server to find and display the answer
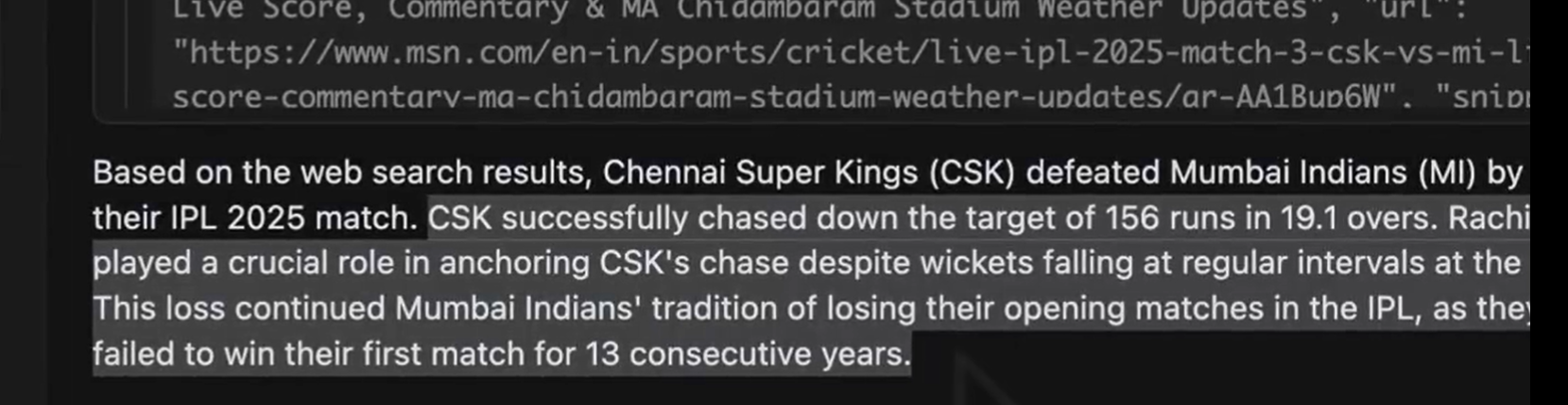
2. RAG (Document Analysis) Tool
This lets Cursor analyze your personal documents.
How to set up:
- In the cloned repository, find the
data
folder
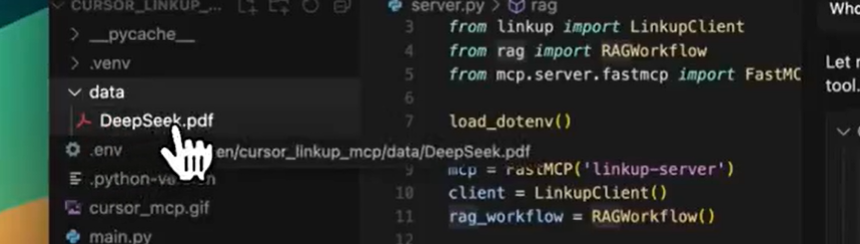
2. Add any documents you want to analyze (PDFs, Word files, etc.)
3. In chat, ask questions about your documents:
>> Summarize the key points from my file about how DeepSeek R1 is trained.
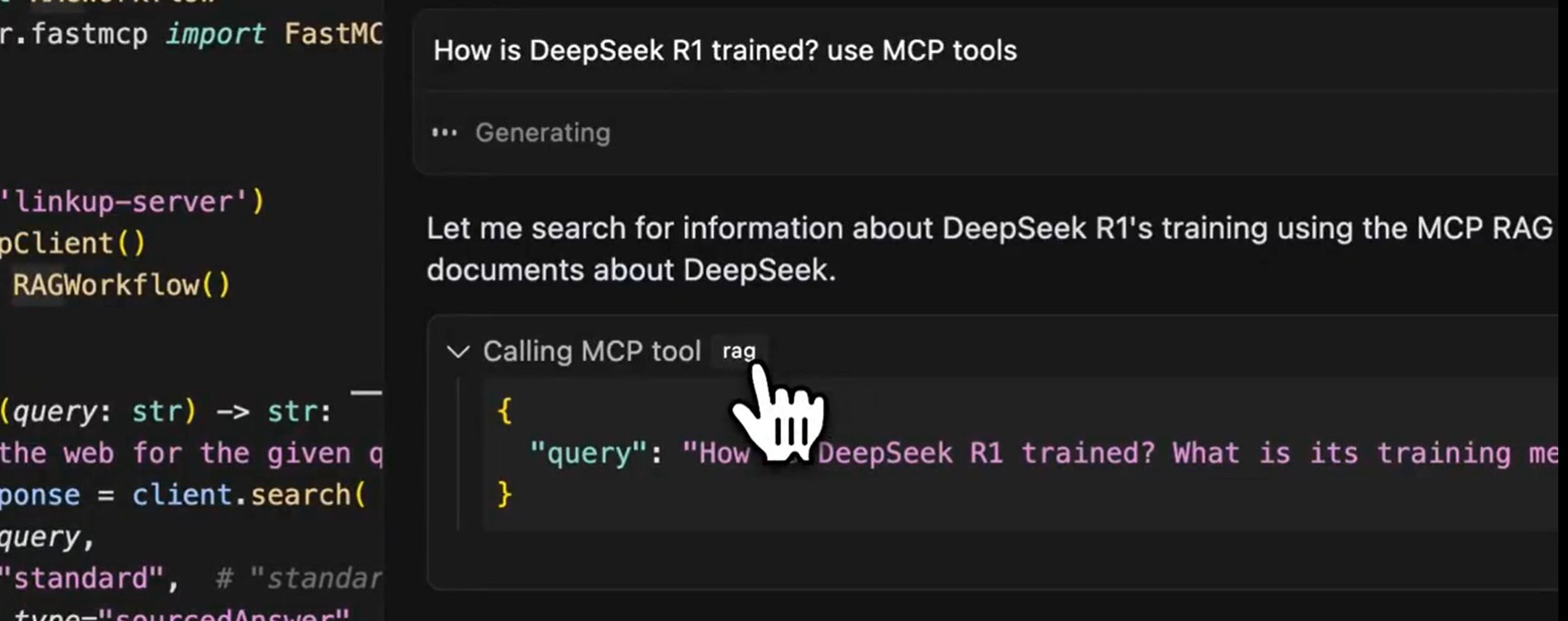
View the results:
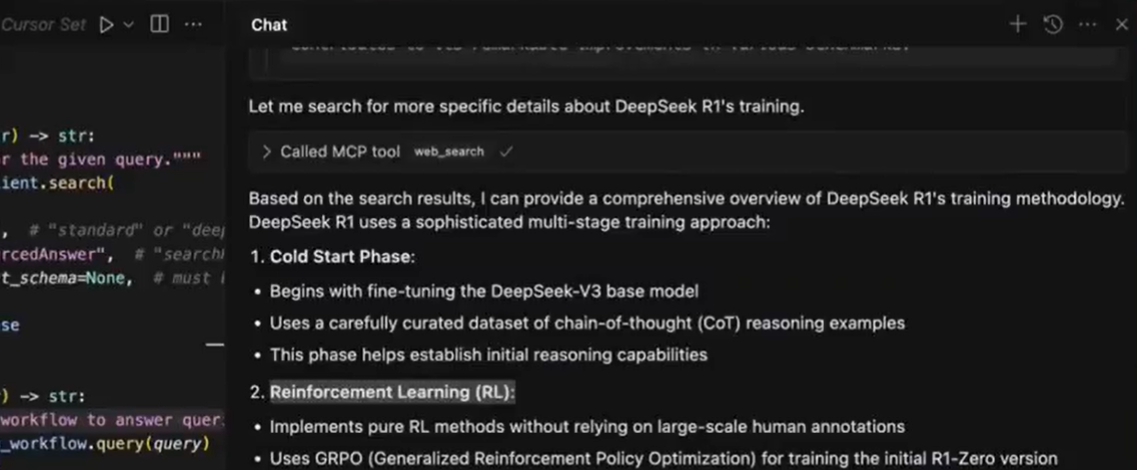
How It Works Under the Hood
Your MCP server acts as a bridge between Cursor and external services:
- When you ask a question, Cursor sends it to your MCP server
- The server processes the request (searching web or analyzing documents)
- Results are sent back to Cursor for display
Understanding the MCP Server Code
This Python script creates a custom MCP (Model Context Protocol) server that adds two powerful AI tools to Cursor: web search and document analysis (RAG). Let's break down what each part does:
1. Importing Dependencies
import asyncio
from dotenv import load_dotenv
from linkup import LinkupClient
from rag import RAGWorkflow
from mcp.server.fastmcp import FastMCP
asyncio
: Enables asynchronous operations (important for handling multiple requests efficiently)dotenv
: Loads environment variables (like API keys) from a.env
fileLinkupClient
: Handles web search functionalityRAGWorkflow
: Manages document analysis (Retrieval-Augmented Generation)FastMCP
: The core MCP server framework
2. Initial Setup
load_dotenv()
mcp = FastMCP('linkup-server')
client = LinkupClient()
rag_workflow = RAGWorkflow()
load_dotenv()
: Loads sensitive configuration (like API keys) from a.env
filemcp
: Creates the main MCP server instance named "linkup-server"client
: Initializes the web search toolrag_workflow
: Prepares the document analysis system
3. Web Search Tool
@mcp.tool()
def web_search(query: str) -> str:
"""Search the web for the given query."""
search_response = client.search(
query=query,
depth="standard", # "standard" or "deep"
output_type="sourcedAnswer", # Options: "searchResults", "sourcedAnswer", or "structured"
structured_output_schema=None, # Required if output_type="structured"
)
return search_response
What it does:
- Adds a tool called
web_search
to your Cursor IDE - Takes a search
query
(like "current weather in Paris") - Can perform different types of searches:
standard
vsdeep
(how thorough the search should be)- Returns either raw results (
searchResults
) or processed answers (sourcedAnswer
) - Returns the search results to Cursor
Example usage in Cursor:
/web_search query="Who won the 2023 Cricket World Cup?"
4. Document Analysis (RAG) Tool
@mcp.tool()
async def rag(query: str) -> str:
"""Use RAG to answer queries using documents from the data directory"""
response = await rag_workflow.query(query)
return str(response)
What it does:
- Creates a tool called
rag
(Retrieval-Augmented Generation) - Analyzes documents you've placed in the
/data
folder - Answers questions based on those documents
- Works asynchronously for better performance with large files
Example usage in Cursor:
/rag query="What are the key safety recommendations in this AI paper?"
5. Server Startup
if __name__ == "__main__":
asyncio.run(rag_workflow.ingest_documents("data"))
mcp.run(transport="stdio")
What happens when you run this:
- First loads all documents from the
data
folder into memory - Starts the MCP server using stdio (standard input/output) communication
- Makes both tools available to Cursor
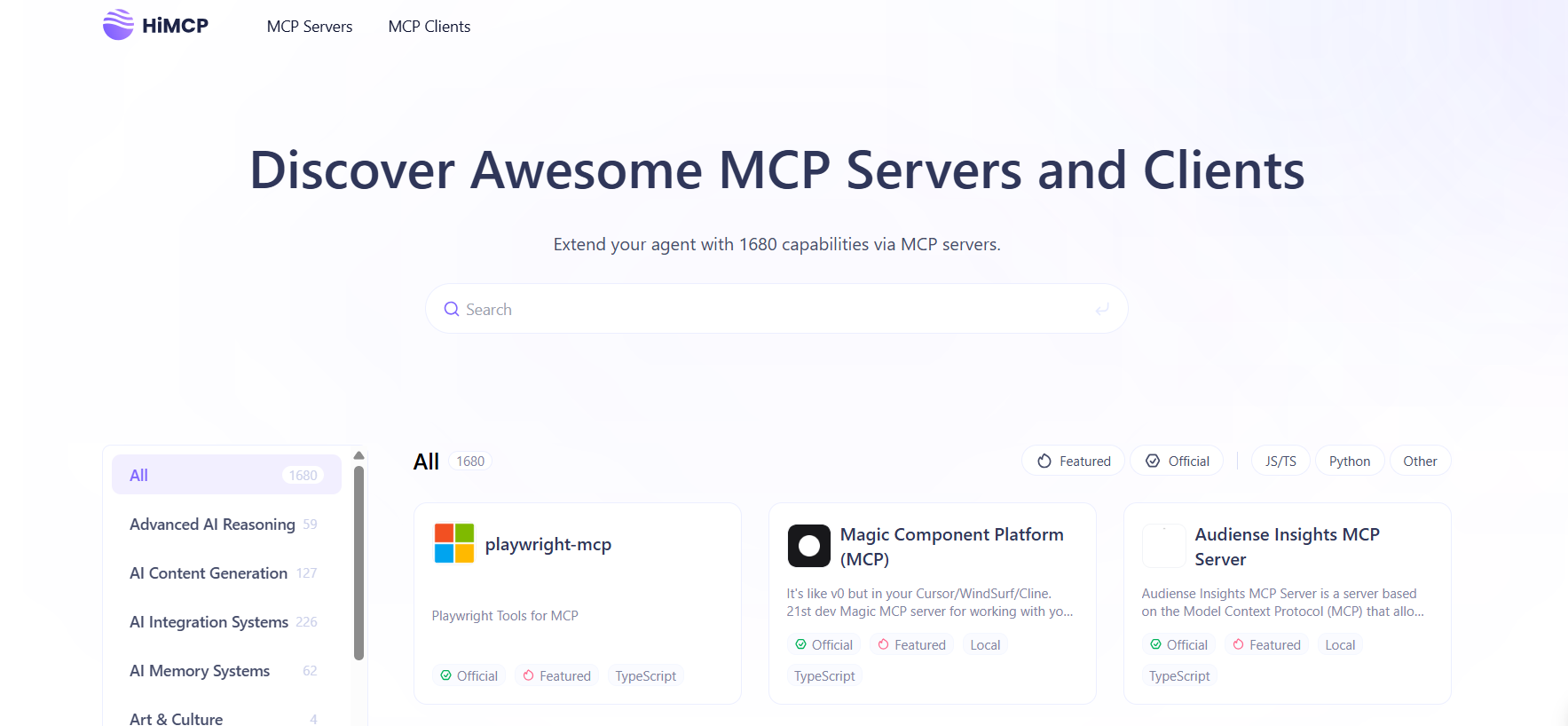
Key Features of The MCP Server Implementation
- Security: Uses
.env
for sensitive data - Flexibility: Offers different search modes (standard/deep)
- Local Processing: Analyzes your private documents without sending them to the cloud
- Performance: Uses async operations for smooth experience
How Cursor Uses This Server
- You type a command in Cursor (like
/web_search
) - Cursor sends your query to this running server
- The server processes it (searching web or analyzing documents)
- Results are returned to Cursor and displayed to you
This turns your Cursor IDE into a powerful research assistant that can both search the web and analyze your personal documents - all through simple chat commands!
Troubleshooting Tips
If something isn't working:
- Check that the UV command points to the correct location
- Make sure all dependencies are installed (run
pip install -r requirements.txt
) - Verify your Python version is 3.8 or higher
- Check Cursor's error logs if the server fails to start
Next Steps:
Now that you have a basic MCP server running, you can:
- Add more tools (like Twitter API integration)
- Customize the web search parameters
- Improve the document analysis with better prompts
- Share your server with teammates
Final Thoughts
Building your first MCP server might seem daunting, but as you've seen, the template makes it straightforward. In less than 30 minutes, you've added powerful new capabilities to Cursor that will save you hours of manual work.
What will you build next? Maybe a tool to:
- Check stock prices
- Search through your codebase
- Generate social media posts
The possibilities are endless! Remember, every expert was once a beginner - you've just taken your first step into the world of MCP server development.
And while you're at it, don't forget to check out Apidog to supercharge you MCP and API development workflow! 🚀