The Bloomberg API (Application Programming Interface) is a powerful tool that provides programmatic access to Bloomberg's extensive financial data services. For financial institutions, hedge funds, asset managers, and software developers, the Bloomberg API offers a way to integrate real-time market data, historical information, and reference data directly into custom applications, trading systems, and analytical tools.
Bloomberg offers several API versions to accommodate different programming languages and use cases:
- BLPAPI (Bloomberg API Core): The foundation C++ API
- Language-specific wrappers for Python, Java, and .NET
- Server API (B-PIPE) for enterprise-level implementations
This tutorial will guide you through the essential steps to set up, connect, and efficiently extract data from Bloomberg's financial ecosystem using their API.
Before diving into the Bloomberg API implementation, consider setting up Apidog as your API testing platform.
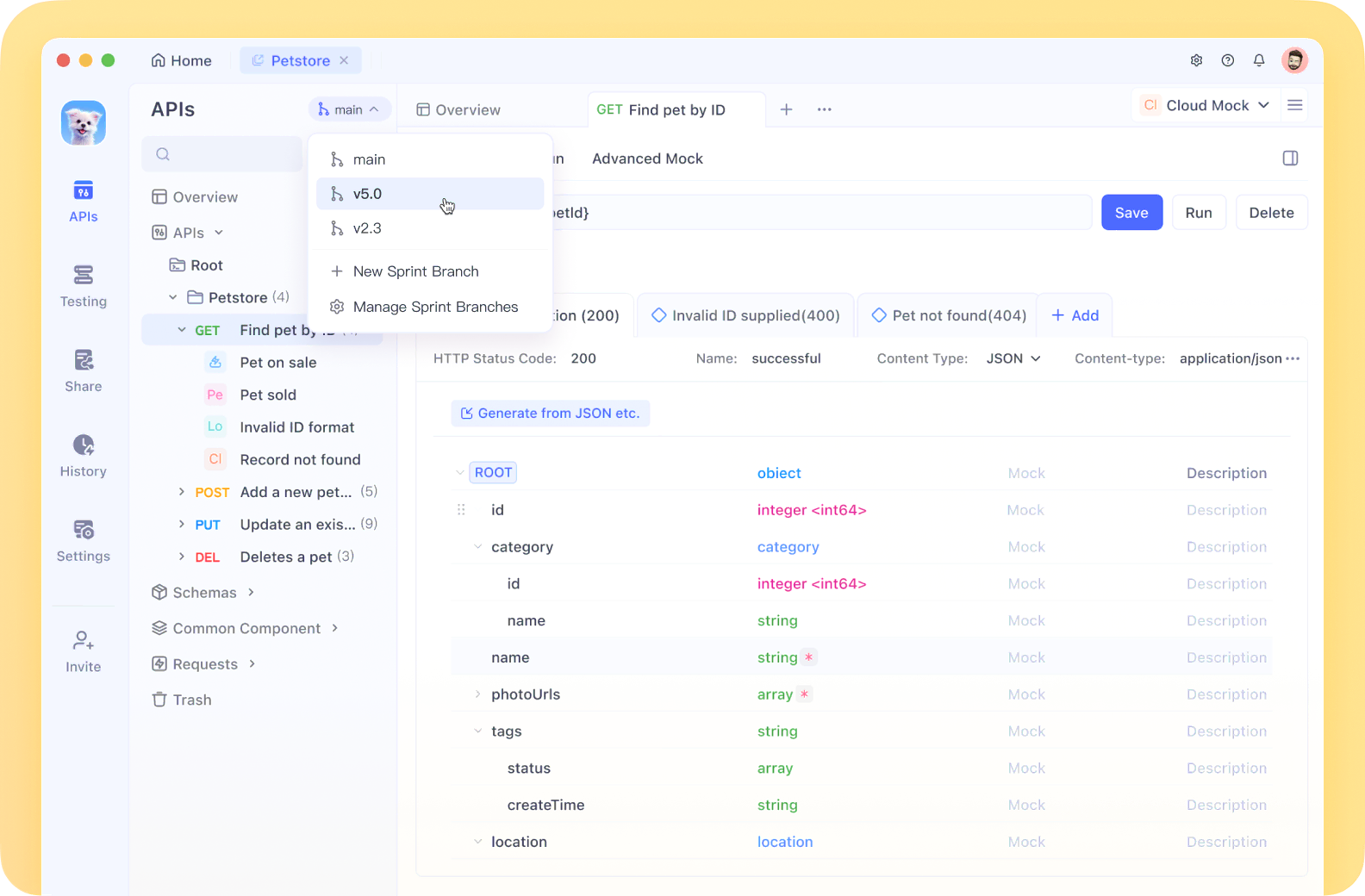
Apidog offers a comprehensive alternative to Postman with enhanced features specifically designed for API development, testing, and documentation. Its intuitive interface and powerful collaboration tools can significantly streamline your Bloomberg API integration workflow.
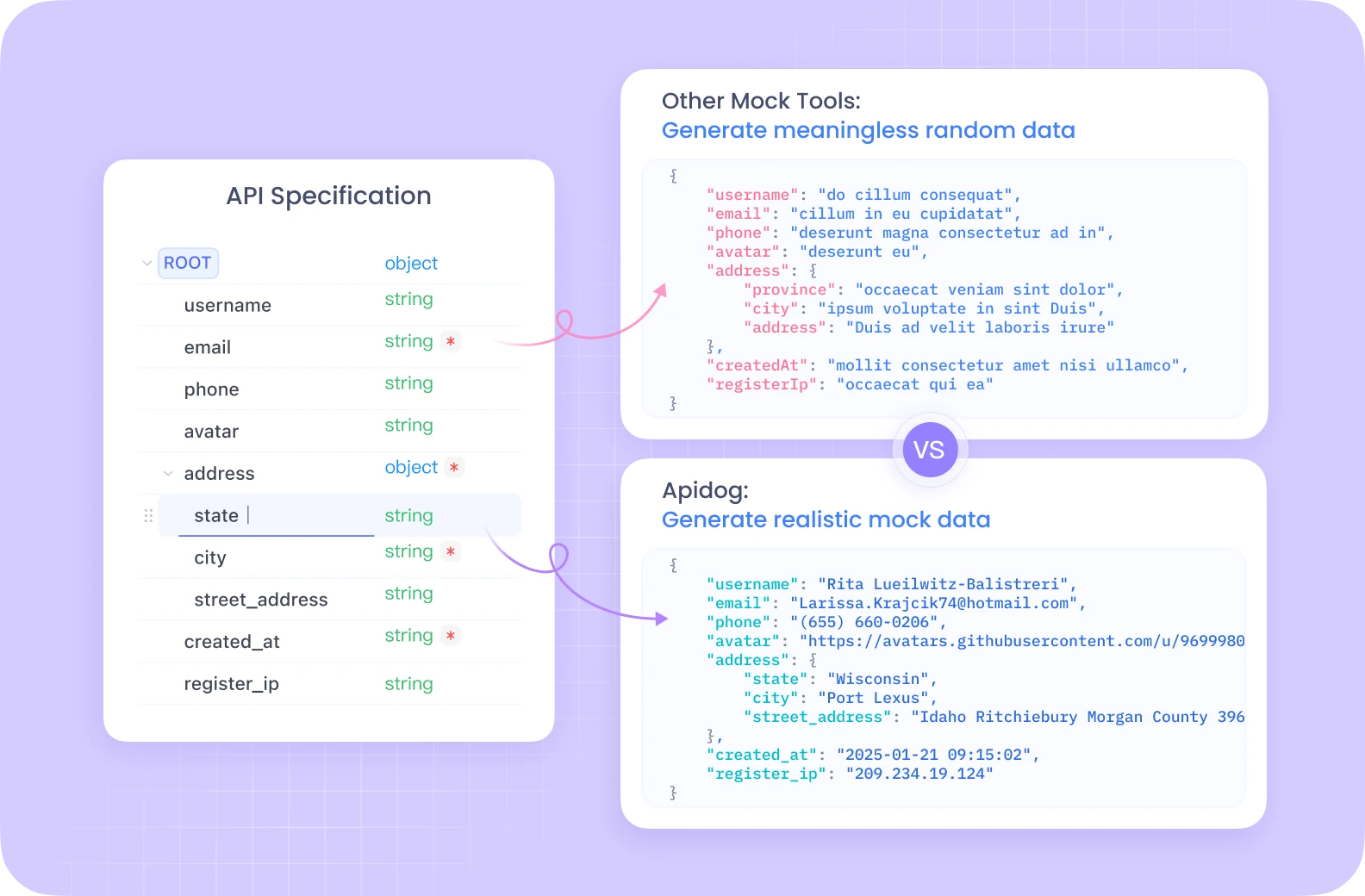
With features like automated testing, mock servers, and better team collaboration capabilities, Apidog is particularly valuable when working with complex financial APIs.
Step 1: Setting Up Bloomberg API for Python
Prerequisites
Before starting with the Bloomberg API in Python, ensure you have:
- A valid Bloomberg Terminal subscription or B-PIPE entitlement
- Bloomberg Desktop API (DAPI) or Server API installed
- Python 3.6 or higher installed on your system
- Basic familiarity with Python programming
- Administrator access to install packages
Installation Process
Install the Bloomberg Python API Package:
The Bloomberg API for Python can be installed using pip:
pip install blpapi
This will install the official Bloomberg API Python wrapper, which communicates with the underlying C++ BLPAPI library.
Verify the Bloomberg Services:
Before proceeding, ensure that Bloomberg services are running on your machine. If you're using the Desktop API, the Bloomberg Terminal must be running and you must be logged in.
Set Environment Variables:
In some configurations, you may need to set specific environment variables to help Python locate the Bloomberg libraries:
import os
os.environ['BLPAPI_ROOT'] = 'C:\\blp\\API' # Adjust path as needed
Verify Installation:
Create a simple test program to ensure the API is correctly installed:
import blpapi
print(f"Bloomberg API version: {blpapi.VERSION_MAJOR}.{blpapi.VERSION_MINOR}.{blpapi.VERSION_PATCH}")
If this runs without errors, your Bloomberg API Python installation is working correctly.
Step 2: Understanding the Bloomberg API Architecture
Before diving into code, it's essential to understand the core components of the Bloomberg API architecture:
Key Components
- Session: The primary interface for communicating with Bloomberg services
- Service: Represents a specific Bloomberg service (e.g., //blp/refdata for reference data)
- Request: A message sent to Bloomberg to retrieve specific data
- Event: Information returned from Bloomberg in response to requests or subscriptions
- Message: The actual data container within events
- Element: Data fields within messages, which can be simple values or complex nested structures
Service Types
Bloomberg API provides access to various services:
- //blp/refdata: Reference data, historical data, and intraday bars
- //blp/mktdata: Real-time market data
- //blp/apiauth: Authentication service
- //blp/instruments: Instrument lookup and search
- //blp/apiflds: Field information service
Step 3: Establishing Connection
The foundation of any Bloomberg API application is establishing a proper connection to Bloomberg services.
Connection Options
Bloomberg API offers several connection methods:
- Desktop API: Connects through the local Bloomberg Terminal
- B-PIPE: Direct connection to Bloomberg data centers (enterprise solution)
- Remote B-PIPE: B-PIPE via remote server with load balancing
Basic Connection Example
import blpapi
def create_session():
"""Establish a connection to Bloomberg API."""
# Initialize session options
session_options = blpapi.SessionOptions()
# Configure connection parameters for Desktop API
session_options.setServerHost("localhost")
session_options.setServerPort(8194) # Standard port for Bloomberg Desktop API
# Optional: Set authentication details for B-PIPE
# session_options.setAuthenticationOptions("AuthenticationMode=APPLICATION_ONLY;ApplicationAuthenticationType=APPNAME_AND_KEY;ApplicationName=YourAppName")
# Create and start the session
session = blpapi.Session(session_options)
if not session.start():
print("Failed to start session.")
return None
print("Successfully connected to Bloomberg API")
return session
# Create the session
session = create_session()
if session is None:
exit()
Connection Security and Authentication
For B-PIPE connections, security is paramount. The authentication process typically involves:
def authenticate_session(session):
"""Authenticate a session for B-PIPE access."""
# Open authentication service
if not session.openService("//blp/apiauth"):
print("Failed to open //blp/apiauth service")
return False
auth_service = session.getService("//blp/apiauth")
# Create authorization request
auth_request = auth_service.createAuthorizationRequest()
auth_request.set("uuid", "YOUR_UUID")
auth_request.set("applicationName", "YOUR_APP_NAME")
# Optional: Add IP addresses for directory service lookup
ip_addresses = auth_request.getElement("ipAddresses")
ip_addresses.appendValue("YOUR_IP_ADDRESS")
# Send request
identity = session.createIdentity()
session.sendAuthorizationRequest(auth_request, identity)
# Process authorization response
while True:
event = session.nextEvent(500)
if event.eventType() == blpapi.Event.RESPONSE or \
event.eventType() == blpapi.Event.PARTIAL_RESPONSE or \
event.eventType() == blpapi.Event.REQUEST_STATUS:
for msg in event:
if msg.messageType() == blpapi.Name("AuthorizationSuccess"):
print("Authorization successful")
return True
elif msg.messageType() == blpapi.Name("AuthorizationFailure"):
print("Authorization failed")
return False
if event.eventType() == blpapi.Event.RESPONSE:
break
return False
Step 4: Making Basic Data Requests
Once connected, you can start requesting data from Bloomberg using various request types.
Opening a Service
Before making requests, you need to open the appropriate service:
def open_service(session, service_name):
"""Open a Bloomberg service."""
if not session.openService(service_name):
print(f"Failed to open {service_name} service")
return None
return session.getService(service_name)
# Open reference data service
refdata_service = open_service(session, "//blp/refdata")
if refdata_service is None:
session.stop()
exit()
Reference Data Request
Reference data requests allow you to retrieve static or calculated fields for securities.
def get_reference_data(refdata_service, securities, fields):
"""Retrieve reference data for specified securities and fields."""
# Create request
request = refdata_service.createRequest("ReferenceDataRequest")
# Add securities to the request
for security in securities:
request.append("securities", security)
# Add fields to the request
for field in fields:
request.append("fields", field)
# Optional: Add overrides
# overrides = request.getElement("overrides")
# override1 = overrides.appendElement()
# override1.setElement("fieldId", "SETTLE_DT")
# override1.setElement("value", "20230630")
print("Sending Reference Data Request:")
print(f" Securities: {securities}")
print(f" Fields: {fields}")
# Send the request
session.sendRequest(request)
# Process the response
results = {}
done = False
while not done:
event = session.nextEvent(500) # Timeout in milliseconds
for msg in event:
if msg.messageType() == blpapi.Name("ReferenceDataResponse"):
security_data_array = msg.getElement("securityData")
for security_data in security_data_array.values():
security = security_data.getElementAsString("security")
# Check for security errors
if security_data.hasElement("securityError"):
error_info = security_data.getElement("securityError")
error_message = error_info.getElementAsString("message")
results[security] = {"error": error_message}
continue
# Process field data
field_data = security_data.getElement("fieldData")
field_values = {}
# Extract all available fields
for field in fields:
if field_data.hasElement(field):
field_value = None
# Handle different data types
field_element = field_data.getElement(field)
if field_element.datatype() == blpapi.DataType.FLOAT64:
field_value = field_data.getElementAsFloat(field)
elif field_element.datatype() == blpapi.DataType.INT32:
field_value = field_data.getElementAsInt(field)
elif field_element.datatype() == blpapi.DataType.STRING:
field_value = field_data.getElementAsString(field)
elif field_element.datatype() == blpapi.DataType.DATE:
field_value = field_data.getElementAsDatetime(field).toString()
else:
field_value = str(field_data.getElement(field))
field_values[field] = field_value
else:
field_values[field] = "N/A"
results[security] = field_values
# Check for field errors
if security_data.hasElement("fieldExceptions"):
field_exceptions = security_data.getElement("fieldExceptions")
for i in range(field_exceptions.numValues()):
field_exception = field_exceptions.getValue(i)
field_id = field_exception.getElementAsString("fieldId")
error_info = field_exception.getElement("errorInfo")
error_message = error_info.getElementAsString("message")
# Add error info to results
if "field_errors" not in results[security]:
results[security]["field_errors"] = {}
results[security]["field_errors"][field_id] = error_message
# Check if we've received the complete response
if event.eventType() == blpapi.Event.RESPONSE:
done = True
return results
# Example usage
securities = ["AAPL US Equity", "MSFT US Equity", "IBM US Equity"]
fields = ["PX_LAST", "NAME", "MARKET_CAP", "PE_RATIO", "DIVIDEND_YIELD"]
reference_data = get_reference_data(refdata_service, securities, fields)
# Print results
for security, data in reference_data.items():
print(f"\nSecurity: {security}")
if "error" in data:
print(f" Error: {data['error']}")
continue
for field, value in data.items():
if field != "field_errors":
print(f" {field}: {value}")
if "field_errors" in data:
print(" Field Errors:")
for field, error in data["field_errors"].items():
print(f" {field}: {error}")
Step 5: Working with Historical Data
Historical data requests allow you to retrieve time series data for one or more securities.
def get_historical_data(refdata_service, security, fields, start_date, end_date, periodicity="DAILY"):
"""Retrieve historical data for the specified security and fields."""
# Create request
request = refdata_service.createRequest("HistoricalDataRequest")
# Set request parameters
request.set("securities", security)
for field in fields:
request.append("fields", field)
request.set("startDate", start_date)
request.set("endDate", end_date)
request.set("periodicitySelection", periodicity)
# Optional parameters
# request.set("maxDataPoints", 100) # Limit number of data points
# request.set("returnEids", True) # Include element identifiers
# request.set("adjustmentNormal", True) # Adjust for normal corporate actions
# request.set("adjustmentAbnormal", True) # Adjust for abnormal corporate actions
# request.set("adjustmentSplit", True) # Adjust for splits
print(f"Requesting historical data for {security} from {start_date} to {end_date}")
# Send the request
session.sendRequest(request)
# Process the response
time_series = []
done = False
while not done:
event = session.nextEvent(500)
for msg in event:
if msg.messageType() == blpapi.Name("HistoricalDataResponse"):
security_data = msg.getElement("securityData")
security_name = security_data.getElementAsString("security")
# Check for security errors
if security_data.hasElement("securityError"):
error_info = security_data.getElement("securityError")
error_message = error_info.getElementAsString("message")
print(f"Error for {security_name}: {error_message}")
return []
# Process field data
field_data = security_data.getElement("fieldData")
for i in range(field_data.numValues()):
field_datum = field_data.getValue(i)
data_point = {"date": field_datum.getElementAsDatetime("date").toString()}
# Extract all requested fields
for field in fields:
if field_datum.hasElement(field):
data_point[field] = field_datum.getElementAsFloat(field)
else:
data_point[field] = None
time_series.append(data_point)
# Check for field errors
if security_data.hasElement("fieldExceptions"):
field_exceptions = security_data.getElement("fieldExceptions")
for i in range(field_exceptions.numValues()):
field_exception = field_exceptions.getValue(i)
field_id = field_exception.getElementAsString("fieldId")
error_info = field_exception.getElement("errorInfo")
error_message = error_info.getElementAsString("message")
print(f"Field error for {field_id}: {error_message}")
# Check if we've received the complete response
if event.eventType() == blpapi.Event.RESPONSE:
done = True
return time_series
# Example usage
security = "IBM US Equity"
fields = ["PX_LAST", "OPEN", "HIGH", "LOW", "VOLUME"]
start_date = "20220101"
end_date = "20221231"
historical_data = get_historical_data(refdata_service, security, fields, start_date, end_date)
# Print the first few data points
print(f"\nHistorical data for {security}:")
for i, data_point in enumerate(historical_data[:5]):
print(f" {data_point['date']}:")
for field in fields:
print(f" {field}: {data_point.get(field)}")
print(f" ... ({len(historical_data)} data points total)")
Step 6: Subscribing to Real-time Market Data
For applications requiring real-time updates, you can subscribe to market data:
def subscribe_market_data(session, securities, fields):
"""Subscribe to real-time market data for specified securities and fields."""
# Open market data service
if not session.openService("//blp/mktdata"):
print("Failed to open //blp/mktdata service")
return False
# Create subscription list
subscriptions = blpapi.SubscriptionList()
# Add securities to subscription
for security in securities:
# Format fields as comma-separated string
fields_str = ",".join(fields)
# Create a unique correlation ID for each security
cid = blpapi.CorrelationId(security)
# Add to subscription list
subscriptions.add(security, fields_str, "", cid)
# Subscribe
session.subscribe(subscriptions)
print(f"Subscribed to market data for {len(securities)} securities")
return subscriptions
def process_market_data(session, max_events=100):
"""Process incoming market data events."""
# Track the latest values
latest_values = {}
try:
counter = 0
while counter < max_events:
event = session.nextEvent(500)
if event.eventType() == blpapi.Event.SUBSCRIPTION_DATA:
for msg in event:
topic = msg.correlationId().value()
if topic not in latest_values:
latest_values[topic] = {}
# Process all fields in the message
for field in msg.asElement().elements():
field_name = field.name()
# Skip administrative fields
if field_name in ["TIMESTAMP", "MESSAGE_TYPE"]:
continue
# Extract value based on data type
if field.datatype() == blpapi.DataType.FLOAT64:
value = field.getValueAsFloat()
elif field.datatype() == blpapi.DataType.INT32:
value = field.getValueAsInt()
elif field.datatype() == blpapi.DataType.STRING:
value = field.getValueAsString()
else:
value = str(field.getValue())
latest_values[topic][field_name] = value
print(f"{topic} {field_name}: {value}")
counter += 1
except KeyboardInterrupt:
print("Subscription processing interrupted")
return latest_values
# Example usage
securities = ["IBM US Equity", "AAPL US Equity", "MSFT US Equity"]
fields = ["LAST_PRICE", "BID", "ASK", "VOLUME"]
subscriptions = subscribe_market_data(session, securities, fields)
if subscriptions:
latest_values = process_market_data(session, max_events=50)
# Print the latest values for each security
print("\nLatest values:")
for security, values in latest_values.items():
print(f" {security}:")
for field, value in values.items():
print(f" {field}: {value}")
# Unsubscribe when done
session.unsubscribe(subscriptions)
Step 7: Working with Complex Data Types and Bulk Data
Bloomberg API can handle complex data structures and large datasets efficiently.
Intraday Bar Data
Intraday bar data provides aggregated price and volume information over specific intervals:
def get_intraday_bars(refdata_service, security, event_type, interval, start_time, end_time):
"""Retrieve intraday bar data."""
# Create request
request = refdata_service.createRequest("IntradayBarRequest")
# Set parameters
request.set("security", security)
request.set("eventType", event_type) # TRADE, BID, ASK, BID_BEST, ASK_BEST, etc.
request.set("interval", interval) # In minutes: 1, 5, 15, 30, 60, etc.
request.set("startDateTime", start_time)
request.set("endDateTime", end_time)
# Send request
session.sendRequest(request)
# Process response
bars = []
done = False
while not done:
event = session.nextEvent(500)
for msg in event:
if msg.messageType() == blpapi.Name("IntradayBarResponse"):
bar_data = msg.getElement("barData")
if bar_data.hasElement("barTickData"):
tick_data = bar_data.getElement("barTickData")
for i in range(tick_data.numValues()):
bar = tick_data.getValue(i)
# Extract bar data
time = bar.getElementAsDatetime("time").toString()
open_price = bar.getElementAsFloat("open")
high = bar.getElementAsFloat("high")
low = bar.getElementAsFloat("low")
close = bar.getElementAsFloat("close")
volume = bar.getElementAsInt("volume")
num_events = bar.getElementAsInt("numEvents")
bars.append({
"time": time,
"open": open_price,
"high": high,
"low": low,
"close": close,
"volume": volume,
"numEvents": num_events
})
if event.eventType() == blpapi.Event.RESPONSE:
done = True
return bars
# Example usage
security = "AAPL US Equity"
event_type = "TRADE"
interval = 5 # 5-minute bars
start_time = "2023-06-01T09:30:00"
end_time = "2023-06-01T16:30:00"
intraday_bars = get_intraday_bars(refdata_service, security, event_type, interval, start_time, end_time)
# Print the first few bars
print(f"\nIntraday {interval}-minute bars for {security}:")
for i, bar in enumerate(intraday_bars[:5]):
print(f" {bar['time']}:")
print(f" OHLC: {bar['open']}/{bar['high']}/{bar['low']}/{bar['close']}")
print(f" Volume: {bar['volume']} ({bar['numEvents']} events)")
print(f" ... ({len(intraday_bars)} bars total)")
Step 8: Advanced Functionality - Bulk Data Requests and Portfolio Analysis
Bloomberg API enables sophisticated analytics and bulk data retrieval:
Portfolio Analysis
def run_portfolio_analysis(refdata_service, portfolio_data, risk_model="BPAM"):
"""Run portfolio analysis using Bloomberg PORT API."""
# Create request
request = refdata_service.createRequest("PortfolioDataRequest")
# Set general parameters
request.set("riskModel", risk_model)
# Add portfolio positions
positions = request.getElement("positions")
for position in portfolio_data:
pos_element = positions.appendElement()
pos_element.setElement("security", position["security"])
pos_element.setElement("weight", position["weight"])
# Add risk factors to analyze
analyses = request.getElement("analyses")
analyses.appendValue("RISK_FACTOR_EXPOSURES")
analyses.appendValue("TRACKING_ERROR_CONTRIBUTION")
# Send request
session.sendRequest(request)
# Process response
# (Note: Actual response handling would be more complex)
results = {}
done = False
while not done:
event = session.nextEvent(500)
# Process event data...
if event.eventType() == blpapi.Event.RESPONSE:
done = True
return results
Step 9: Error Handling and Debugging
Robust Bloomberg API applications require comprehensive error handling:
def handle_bloomberg_exceptions(func):
"""Decorator for handling Bloomberg API exceptions."""
def wrapper(*args, **kwargs):
try:
return func(*args, **kwargs)
except blpapi.InvalidRequestException as e:
print(f"Invalid Request Error: {e}")
except blpapi.InvalidConversionException as e:
print(f"Invalid Type Conversion Error: {e}")
except blpapi.NotFoundException as e:
print(f"Element Not Found Error: {e}")
except blpapi.Exception as e:
print(f"Bloomberg API Error: {e}")
except Exception as e:
print(f"Unexpected error: {e}")
return None
return wrapper
@handle_bloomberg_exceptions
def get_safe_reference_data(refdata_service, securities, fields):
# Implementation with built-in error handling
pass
Step 10: Performance Optimization and Best Practices
For production systems using Bloomberg API:
Request Batching
def batch_security_requests(securities, batch_size=50):
"""Batch a large list of securities into smaller groups."""
for i in range(0, len(securities), batch_size):
yield securities[i:i + batch_size]
# Process a large list of securities in batches
all_securities = ["SECURITY1", "SECURITY2", ..., "SECURITY1000"]
all_results = {}
for batch in batch_security_requests(all_securities):
batch_results = get_reference_data(refdata_service, batch, fields)
all_results.update(batch_results)
Step 11: Clean Up Resources
Always properly close connections when done:
def clean_up(session, subscriptions=None):
"""Properly clean up Bloomberg API resources."""
try:
# Unsubscribe from any active subscriptions
if subscriptions:
session.unsubscribe(subscriptions)
# Stop the session
if session:
session.stop()
print("Bloomberg API session closed")
return True
except Exception as e:
print(f"Error closing Bloomberg session: {e}")
return False
# At the end of your application
clean_up(session, subscriptions)
Conclusion
The Bloomberg API with Python provides powerful access to one of the world's most comprehensive financial data platforms. This tutorial has covered the essential aspects of working with the API, from basic connection and data retrieval to advanced real-time subscriptions and portfolio analysis.
Key points to remember:
- Always properly initialize and close connections
- Batch similar requests for better performance
- Implement comprehensive error handling
- Consider rate limits and data entitlements
- Cache static data when appropriate
- Use appropriate data types and conversion methods
For enterprise-level applications, consider exploring Bloomberg's B-PIPE offering, which provides dedicated connectivity options and higher throughput for mission-critical systems.
As you continue developing with the Bloomberg API, refer to the official Bloomberg BLPAPI documentation for detailed information about available services, fields, and best practices. Bloomberg regularly updates their API offerings, so staying current with the latest developments will ensure you make the most of this powerful financial data access tool.