Basic Authentication: A Comprehensive Guide for Developers
Learn about Basic Authentication, Bearer Token, OAuth, and JWT in Apidog's comprehensive guide on API Authentication and Authorization.
In today's digital landscape, APIs are the backbone of modern applications, driving communication and data exchange between software components. As such, securing them is a top priority.
Imagine building a phenomenal application with a powerful API, only to overlook its security. Before you know it, unauthorized access, data breaches, and misuse of your application occur. Proper security measures, including authentication and authorization, must be implemented to prevent these undesirable outcomes.
When combined, authentication and authorization form a robust security framework that safeguards your APIs and their valuable resources, ensuring only legitimate users access the appropriate data and services. By focusing on API security from the outset, you can prevent unauthorized access and maintain the trust of your customers and partners.
Diving Into Authorization Types
Basic Authentication:
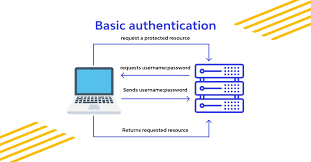
This classic lock-and-key method is simple and widely used, requiring a username and password combination that's Base64 encoded and sent in the HTTP request header. Although easy to implement, it has security drawbacks. Always pair Basic Authentication with HTTPS encryption to keep your data safe, which prevents eavesdropping and man-in-the-middle attacks.
A coded Example of Basic Authentication is below:
# Make a GET request to an API endpoint with authentication
import requests
api_url = 'https://api.example.com/endpoint'
credentials = ('your_username', 'your_password')
response = requests.get(api_url, auth=credentials)
print(response.content)
Bearer Token:
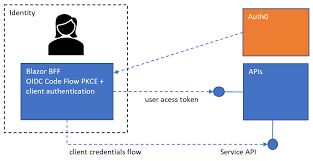
This VIP pass grants access to exclusive resources only after successful authentication. To access exclusive resources, users must successfully authenticate and include the token as a "Bearer" token in the request header. While this method provides more robust security than Basic Authentication, it is essential to exercise caution as tokens are susceptible to theft. To ensure the protection of your application, utilize HTTPS and implement mechanisms to refresh and expire tokens.
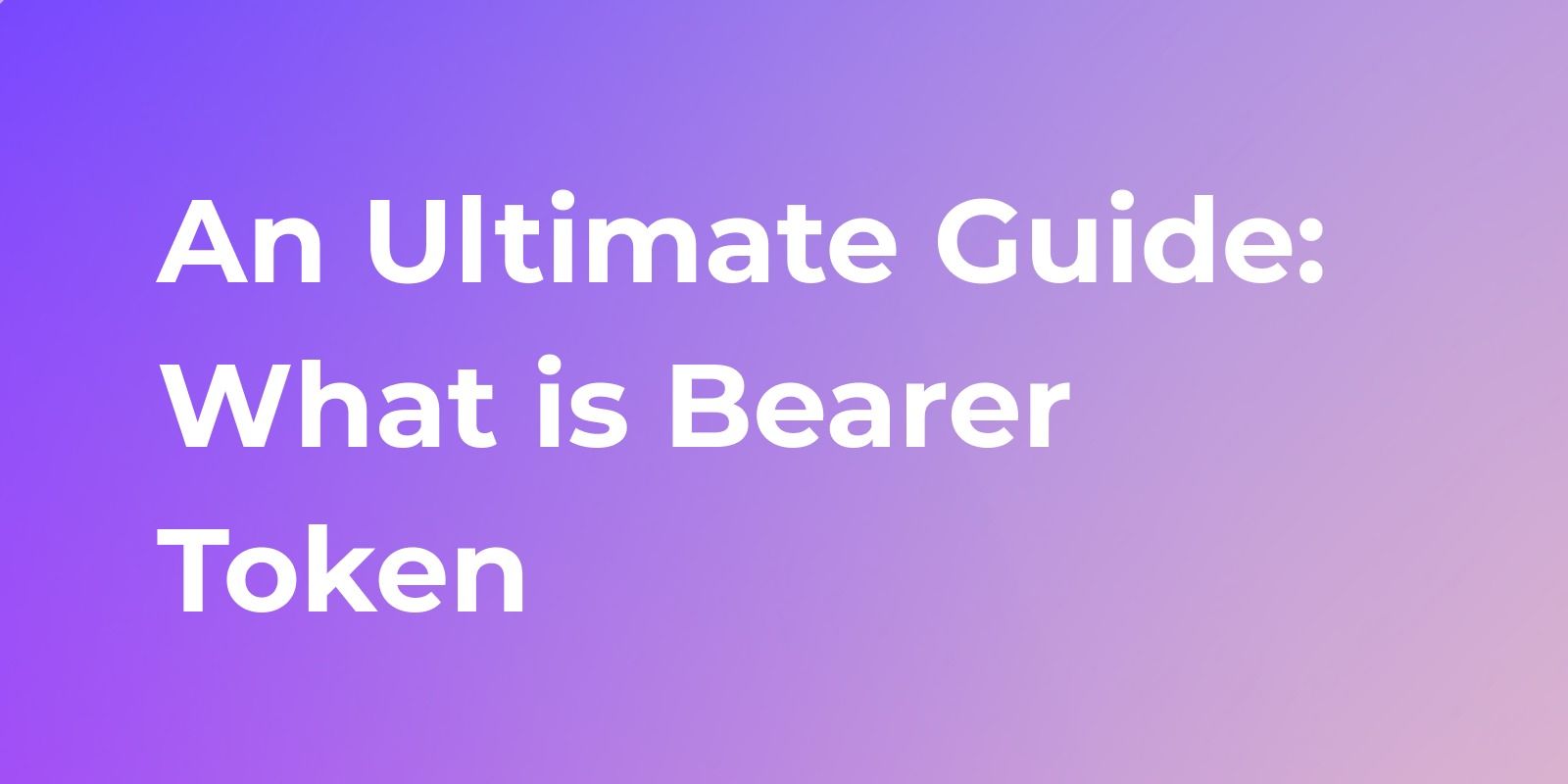
An Example of a Bearer Token is below:
# Make a GET request to an API endpoint with authentication
import requests
api_url = 'https://api.example.com/endpoint'
credentials = ('your_username', 'your_password')
response = requests.get(api_url, auth=credentials)
OAuth:
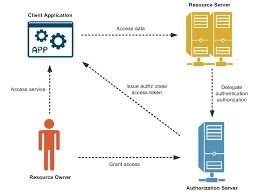
OAuth is the ultimate red carpet experience, providing granular access control and supporting third-party application integration. OAuth trades user credentials for access tokens, which are then used to access protected resources. This additional layer of security and flexibility is great, but setting up OAuth can be complex. Be prepared to navigate multiple authorization flows and token management strategies. Here is the related article on OAuth 2.0.
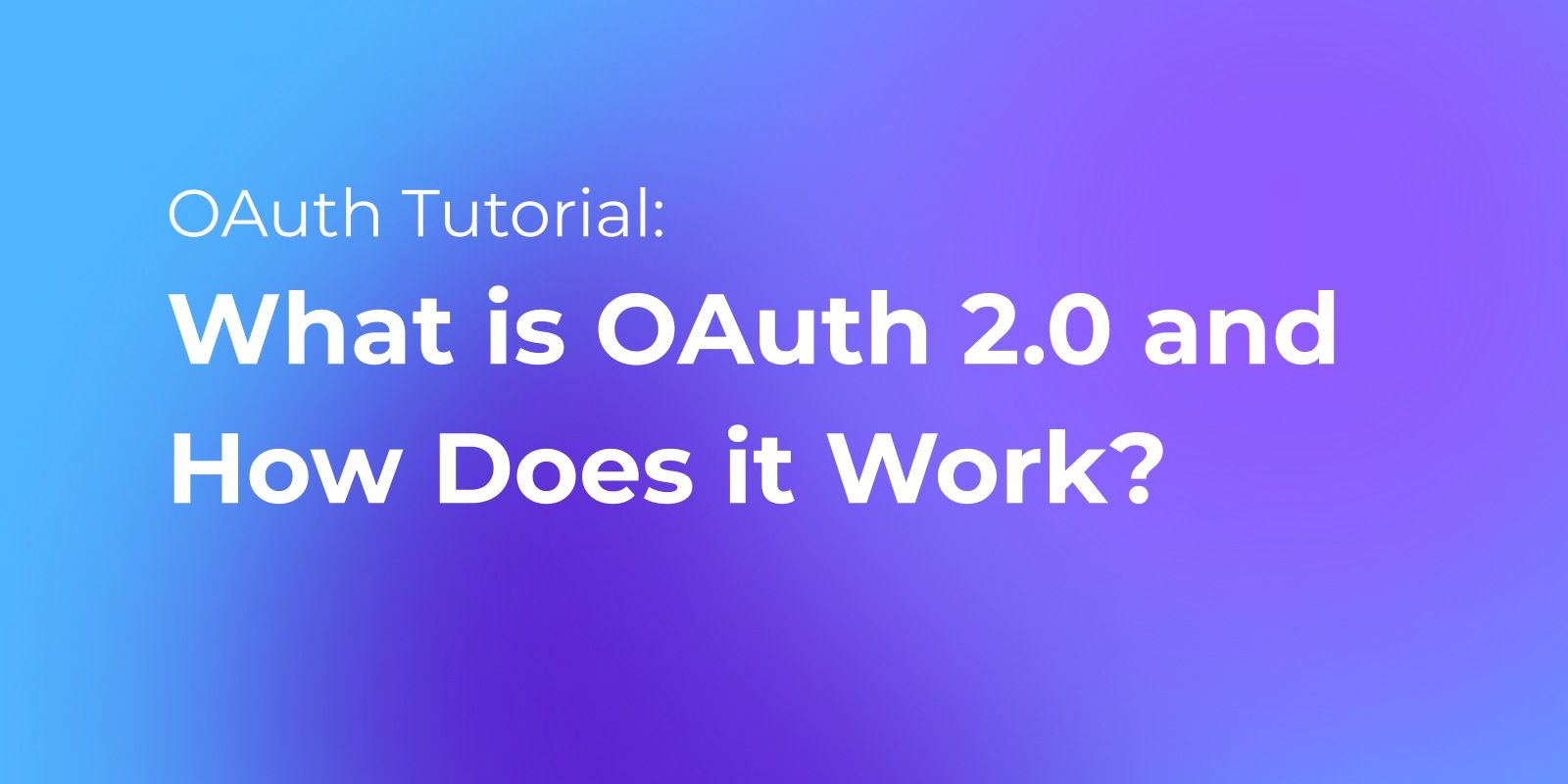
An example of OAuth is below:
# Make an authenticated GET request to an API endpoint using OAuth2.0
import requests
# Authenticate with OAuth2.0 client credentials
auth_response = requests.post('https://example.com/oauth/token', data={
'grant_type': 'client_credentials',
'client_id': 'your_client_id',
'client_secret': 'your_client_secret'
})
access_token = auth_response.json()['access_token']
# Make a GET request to the API endpoint with the access token in the Authorization header
api_url = 'https://example.com/api/endpoint'
api_headers = {'Authorization': f'Bearer {access_token}'}
api_response = requests.get(api_url, headers=api_headers)
# Print the response JSON data
print(api_response.json())
JWT (JSON Web Token):
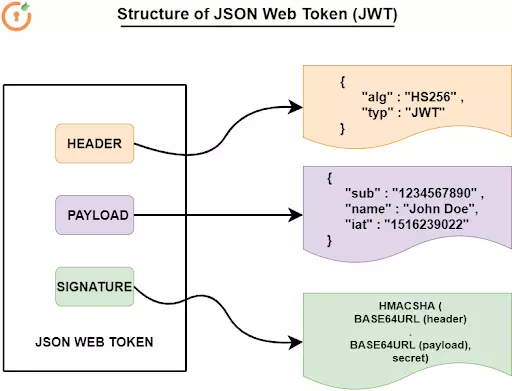
The Swiss Army knife of authorization methods, JWT, carries all the necessary information within the token. These self-contained tokens are compact and secure and support various signing algorithms, making JWT a popular choice for modern applications. To maximize JWT, familiarize yourself with token structure, signature verification, and the best secure token storage and handling practices.
A coded Example of JWT is below:
# Make an authenticated GET request to an API endpoint using JWT
import requests
import jwt
# Encode the payload as a JWT token
payload = {'user_id': 123}
secret_key = 'your_secret_key'
jwt_token = jwt.encode(payload, secret_key, algorithm='HS256').decode()
# Make a GET request to the API endpoint with the JWT token in the Authorization header
api_url = 'https://example.com/api/endpoint'
api_headers = {'Authorization': f'Bearer {jwt_token}'}
api_response = requests.get(api_url, headers=api_headers)
# Print the response JSON data
print(api_response.json())
Each of these authentication methods has its advantages and drawbacks. When choosing an approach, consider factors like ease of implementation, required security levels, and compatibility with your application's architecture. And always remember to follow best practices to keep your users and data safe.
The Great Authentication vs. Authorization Debate
It's easy to mix up authentication and authorization but remember:
- Authentication is all about identity – who are you?
- Authorization is about permissions – what can you do?
Authentication proves you can enter the club, while authorization ensures you only sneak into the VIP section with permission. Together, they form the backbone of API security.
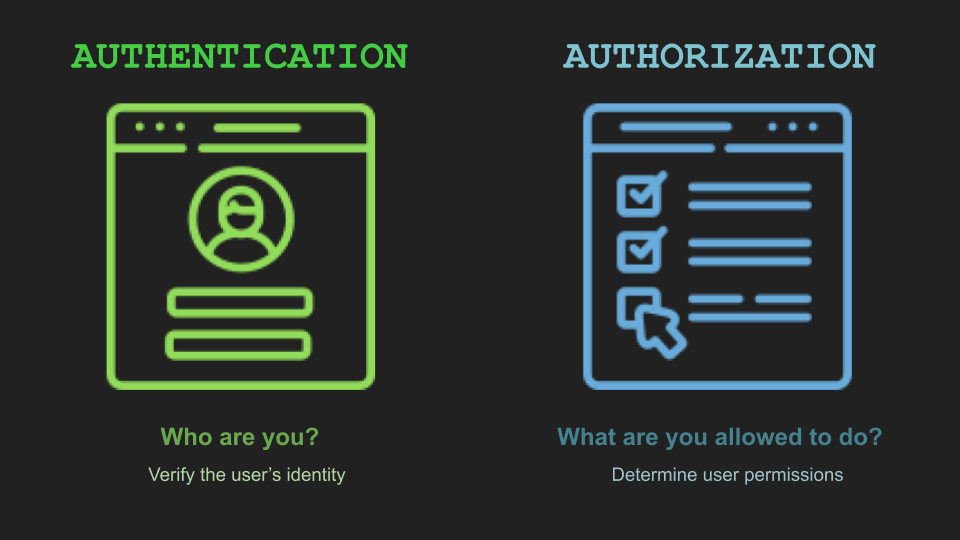
Why API Security Needs Both Authentication and Authorization?
Imagine a world where authentication or authorization protects your APIs. With authentication, you can know if that guest at your exclusive club is an imposter. Without authorization, your authenticated guests could wander into restricted areas, creating chaos. Combining these two security layers creates a powerful defense mechanism, keeping your APIs and their resources safe and sound.
Apidog: Your Reliable Partner in API Protection
Apidog is an innovative platform designed to make managing and securing your APIs a seamless experience. Offering a variety of authentication methods to suit your unique requirements, Apidog has your back, whether you prefer the simplicity of Basic Authentication, the flexibility of Bearer tokens, or the advanced features of OAuth and JWT.
Why Choose Apidog?
From its versatility to its ease of use, Apidog is designed to cater to the diverse needs of developers and organizations. Here are some key reasons to choose Apidog for your API security and management needs:
Versatility:
Apidog supports many authentication methods, allowing you to select the best option for your application. From the user-friendly Basic Authentication to the more complex OAuth and JWT, Apidog has you covered.
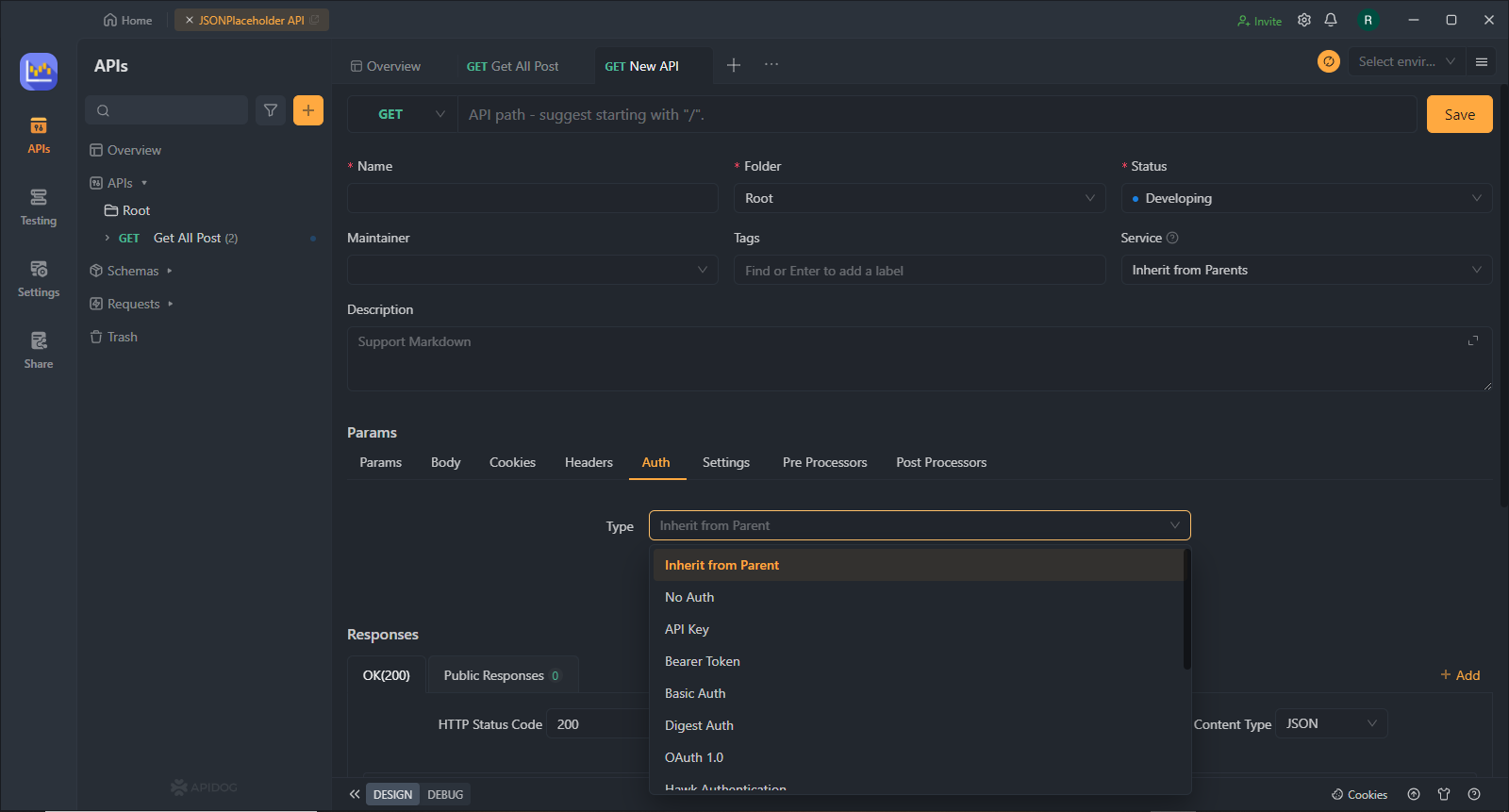
Ease of Use:
With its intuitive user interface and straightforward implementation, Apidog allows developers of all skill levels to quickly and easily manage their APIs. The platform is designed to make API security accessible and efficient without overwhelming users with technical jargon or complex configurations.
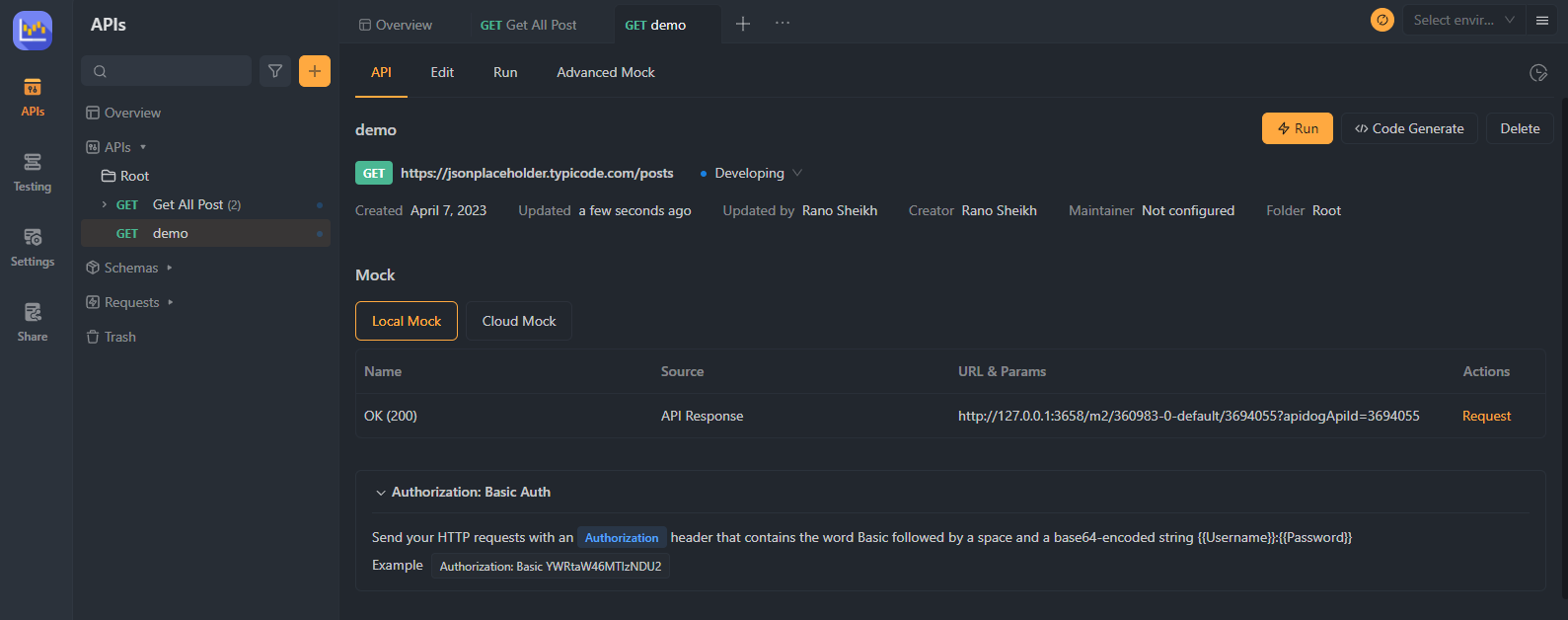
Authentication and Authorization Management:
Apidog simplifies implementing and managing various authentication and authorization methods. You can easily configure your desired method, monitor its performance, and make updates as needed – all within the Apidog platform.
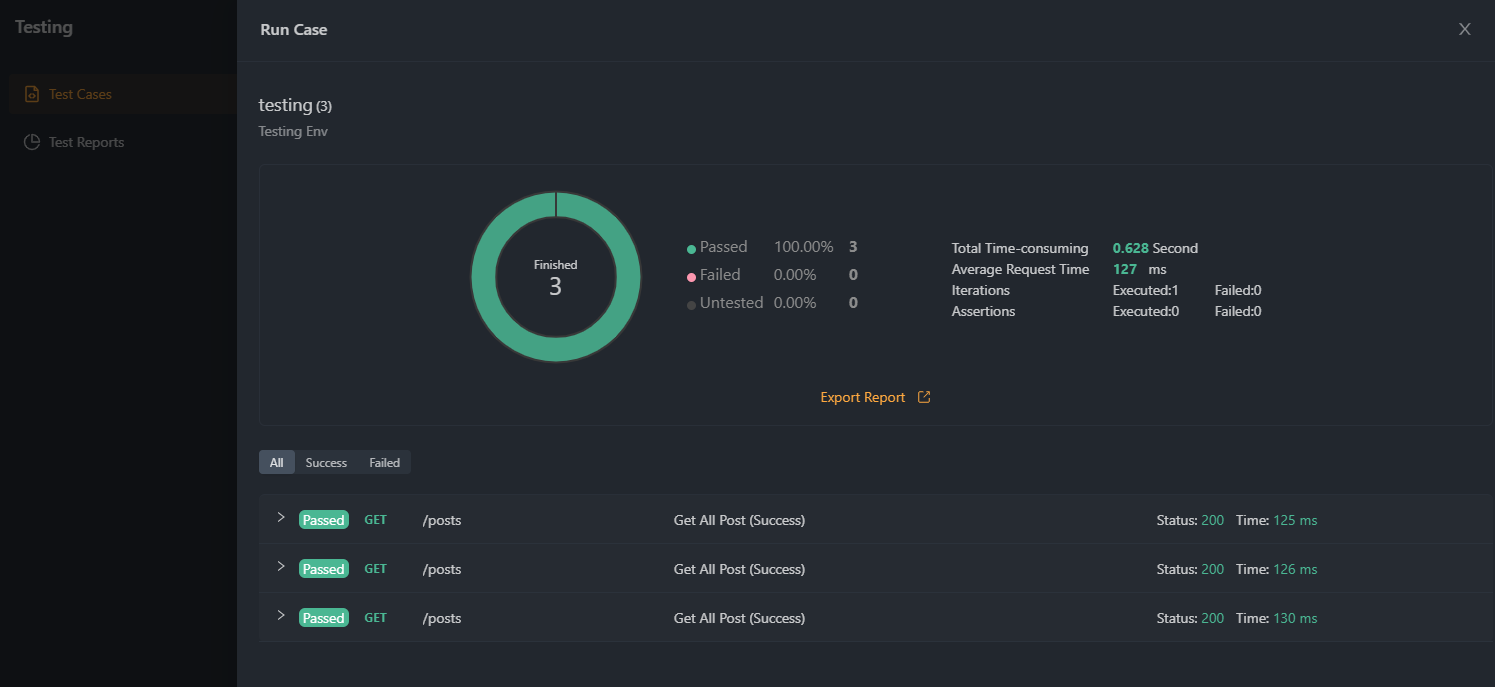
Scalability:
As your application grows and evolves, so too does Apidog. The platform is built to scale with your needs, providing robust support for expanding APIs and ensuring your security measures grow alongside your application.
Dedicated Support:
Apidog's team of experts is always available to provide guidance and support, ensuring your API security implementation is seamless and successful. Their dedication to helping you protect your APIs is another reason Apidog is the ideal choice for API security and management.
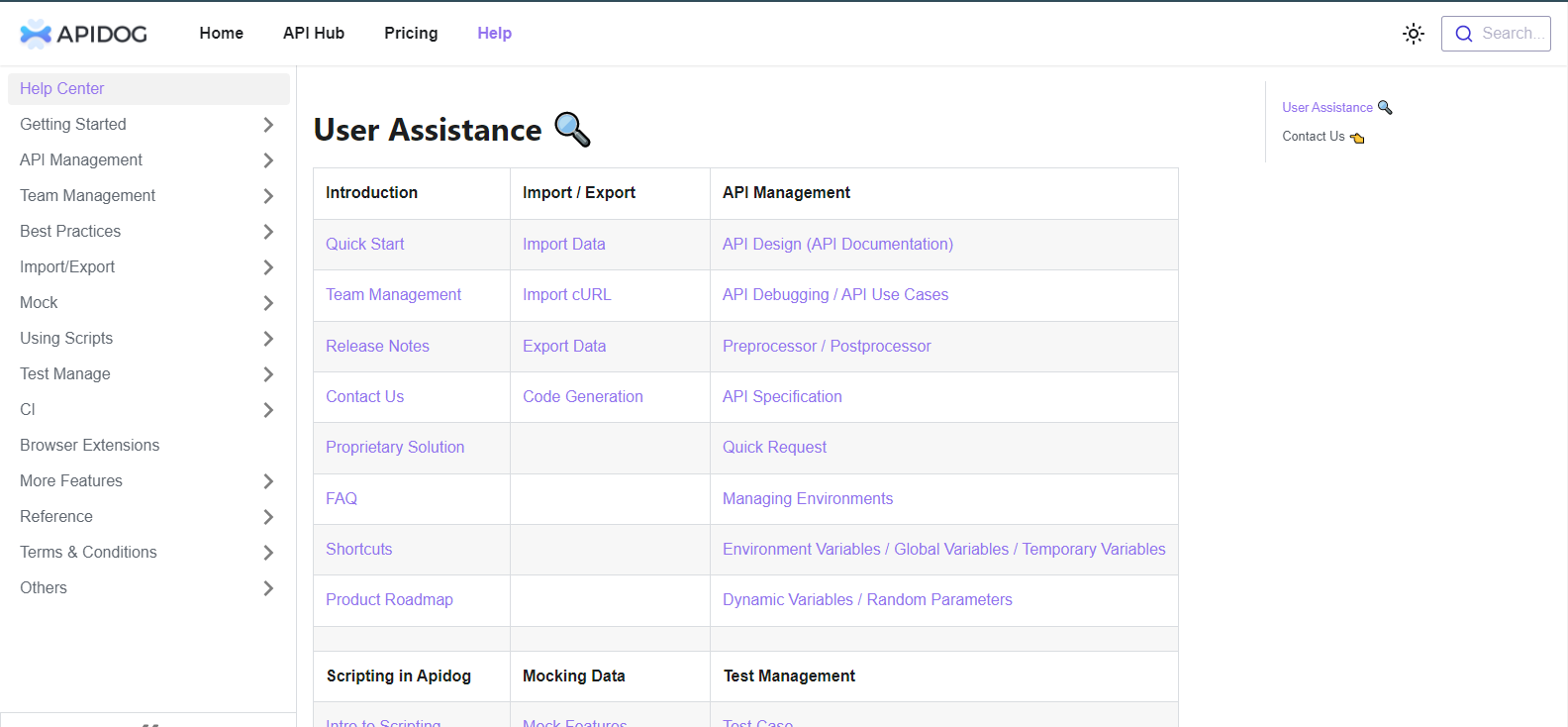
Access Control:
Apidog allows you to establish fine-grained access control, defining specific permissions for different users or clients. It ensures that your API resources are only accessible to those with the appropriate authorization level.
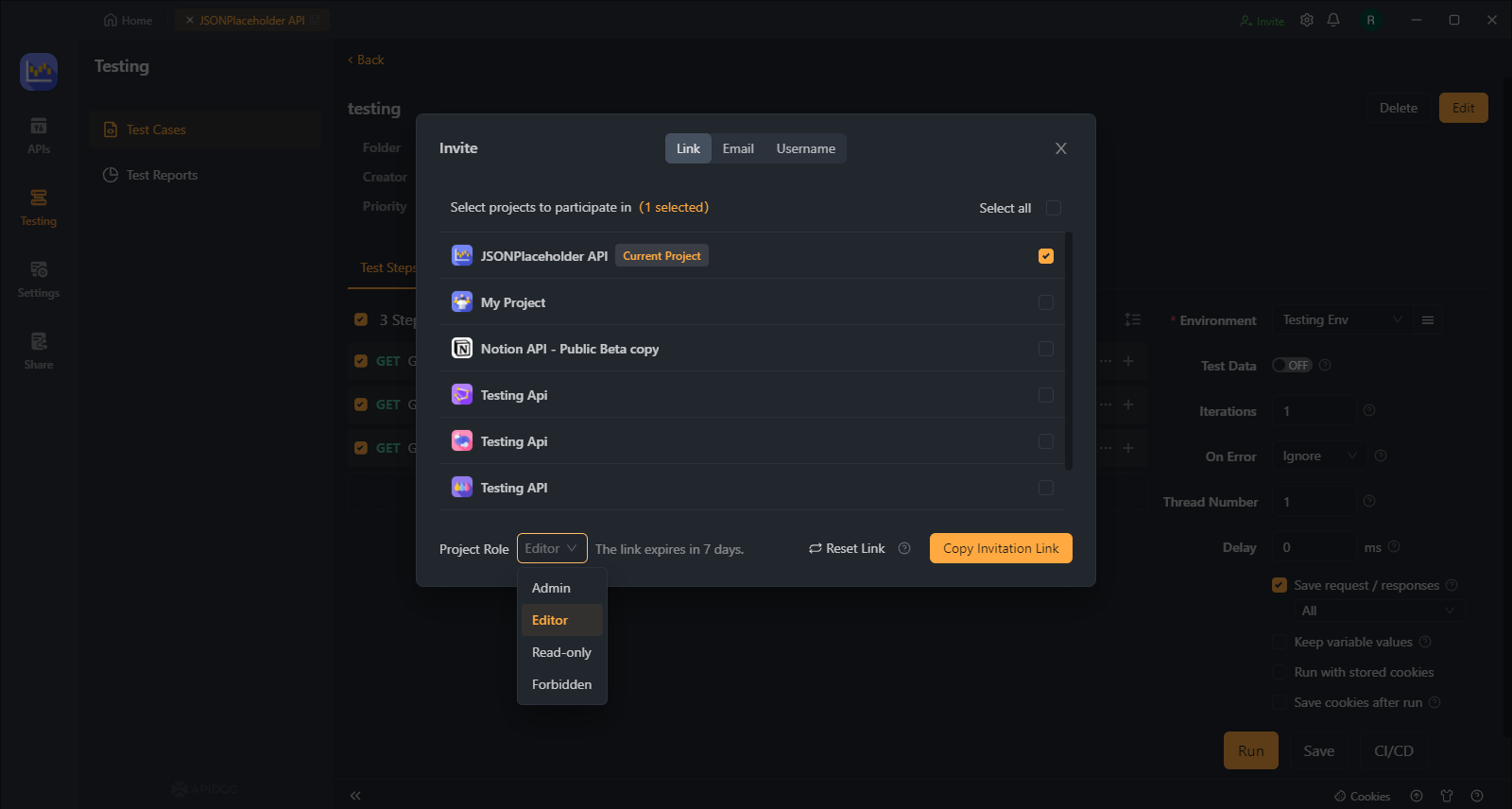
Integration Support:
Apidog is designed to integrate seamlessly with your existing development and deployment workflows. With support for popular tools and platforms, Apidog ensures a smooth and efficient API security implementation process.
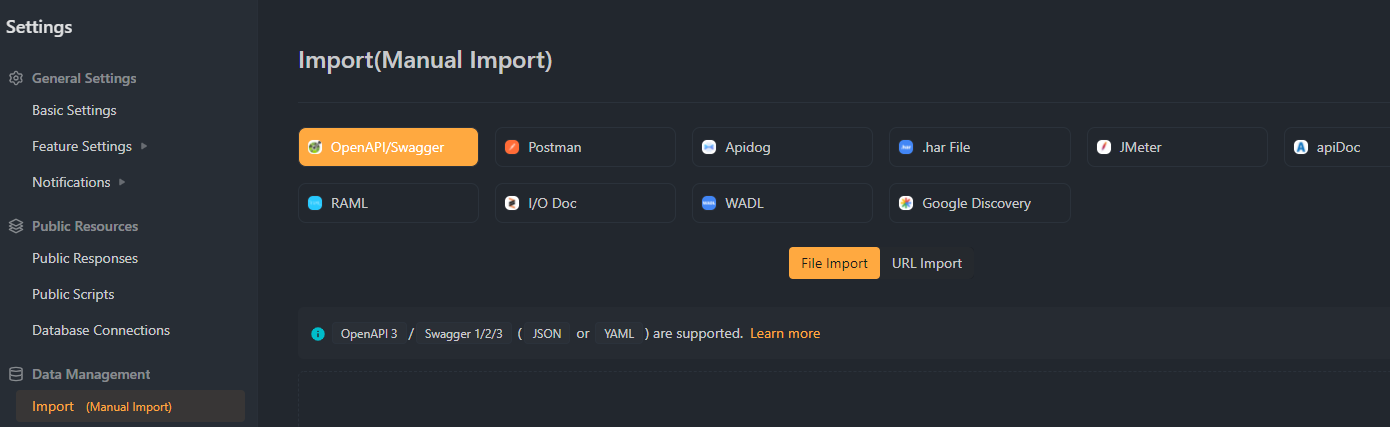
Step-by-Step Basic Authentication Setup in Apidog
Create an Apidog Account
Sign up for an Apidog account if you haven't already. After completing the registration process, log in to access the user dashboard, where you'll manage your API security.
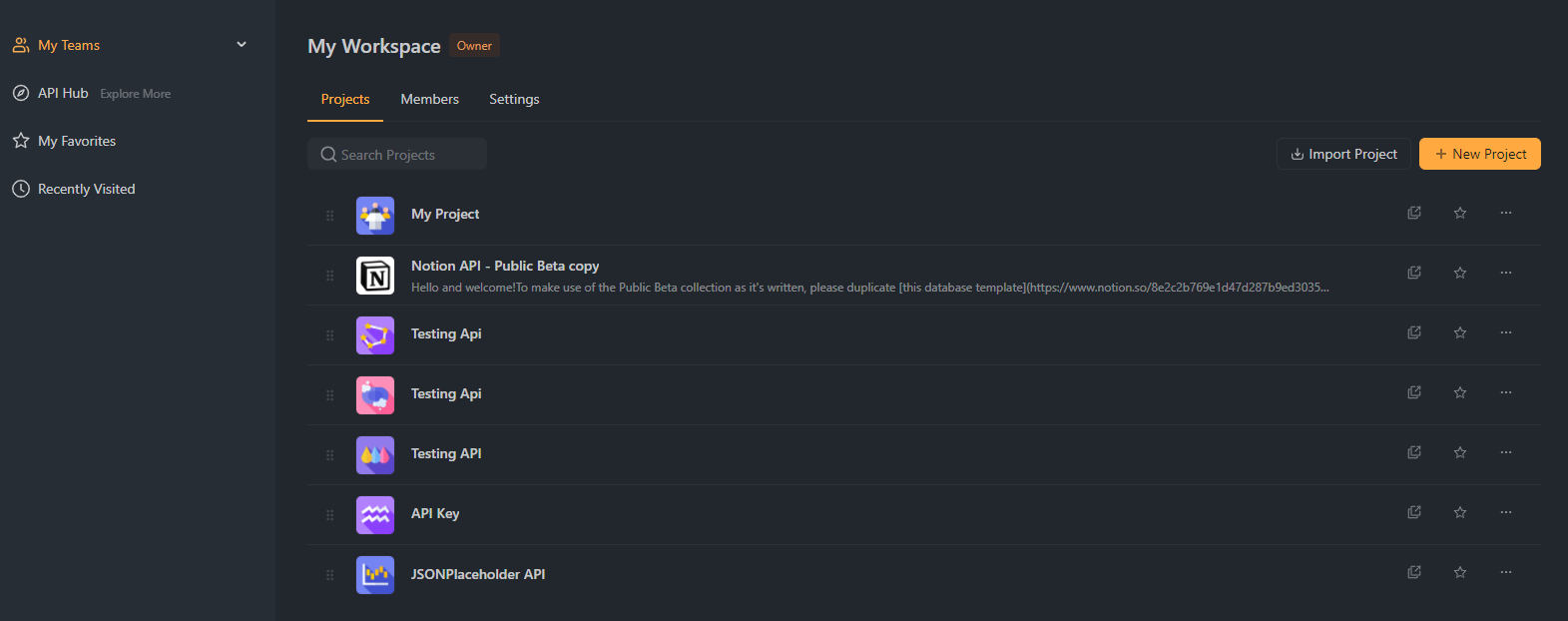
Step 1. Add Your API
In the Apidog dashboard, find the "Add API" or "Create New API" button and click it to start configuring your API. You'll need to provide basic information about your API, such as its name, base URL, and a brief description.
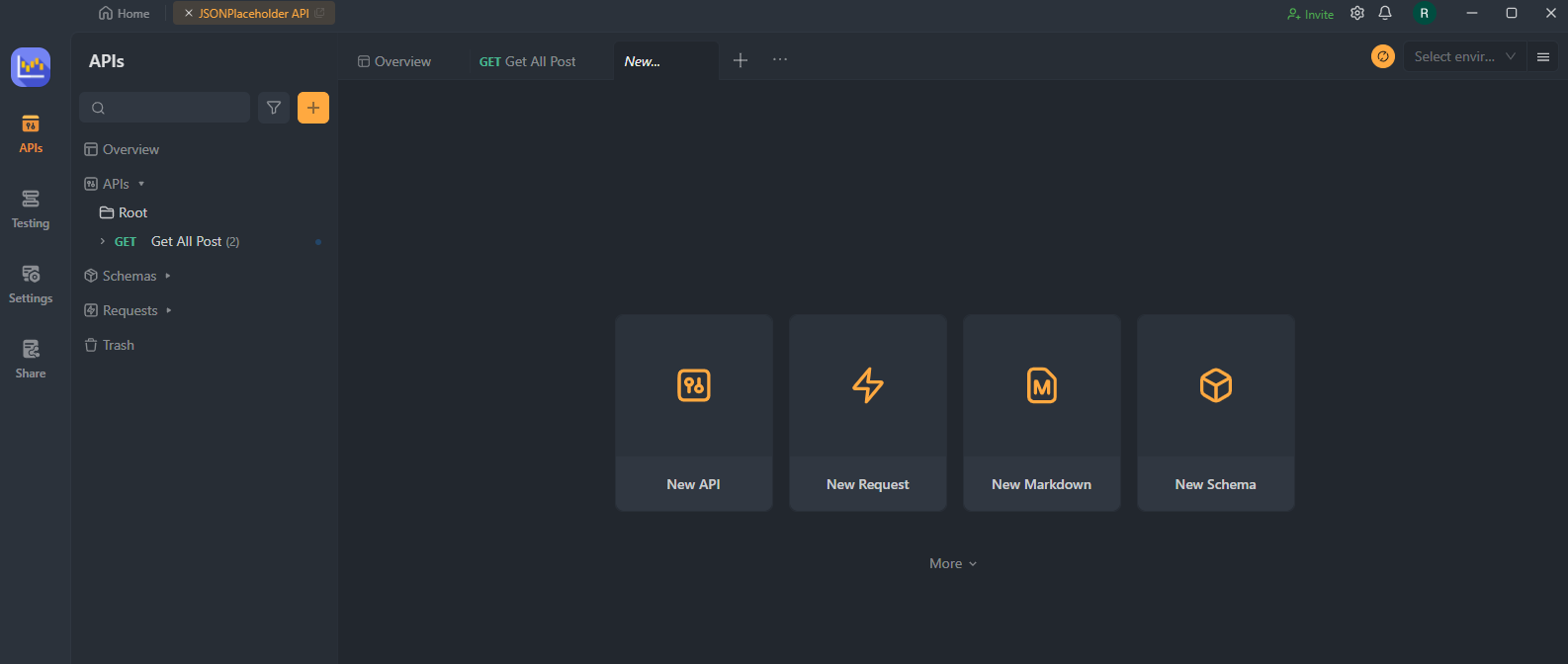
Step 2. Choose Basic Authentication
Navigate to the "Auth" section of your API settings. Here, you'll find various authentication options supported by Apidog. Select "Basic Auth" to use a simple username and password combination to secure your API.
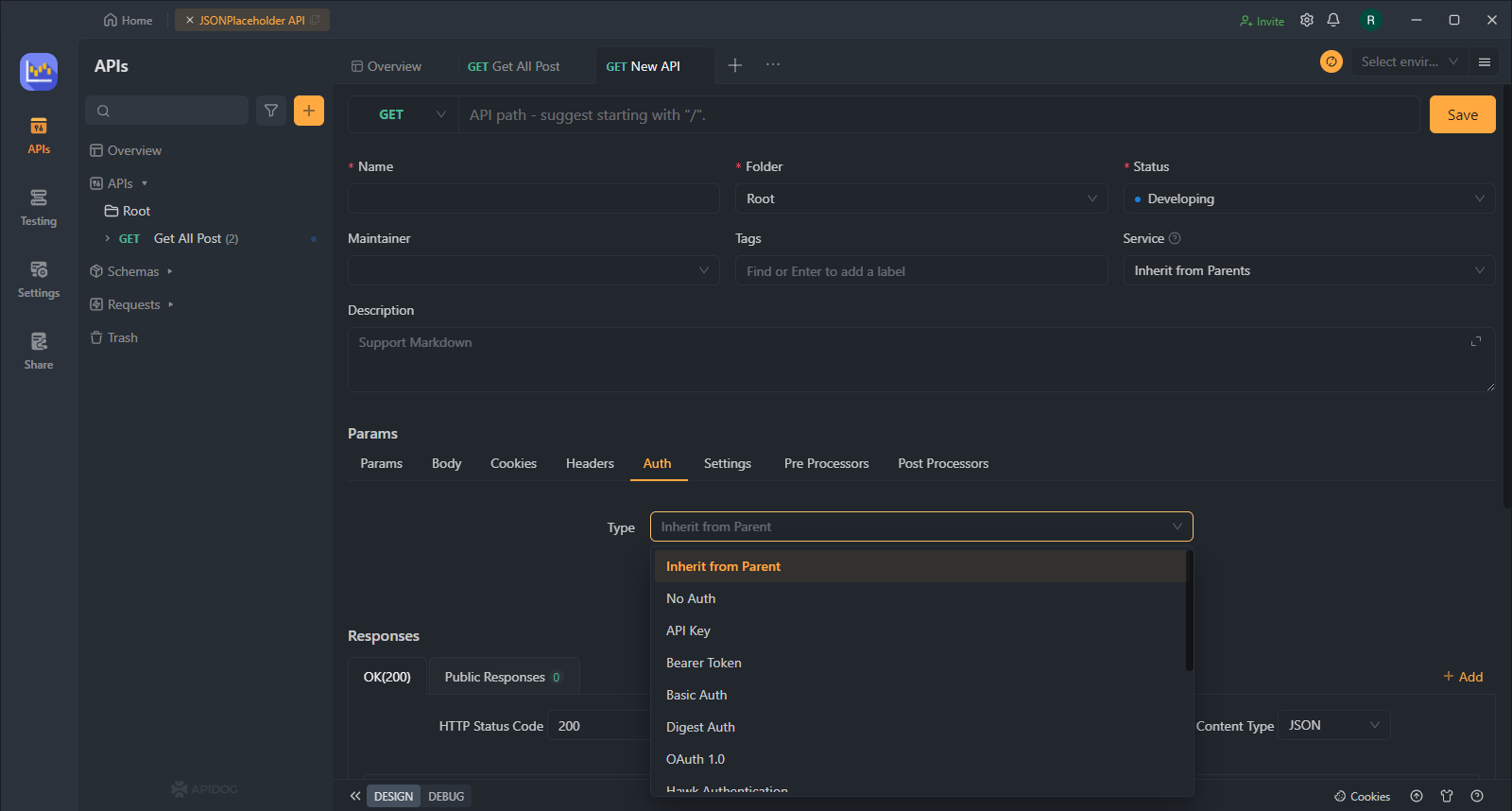
Step 3. Configure Authentication Settings
Provide a unique username and password for each user who requires access to your API. You can create multiple users accounts with different access permissions by defining user roles or specific access levels. Make sure to use strong passwords and avoid sharing credentials among multiple users.
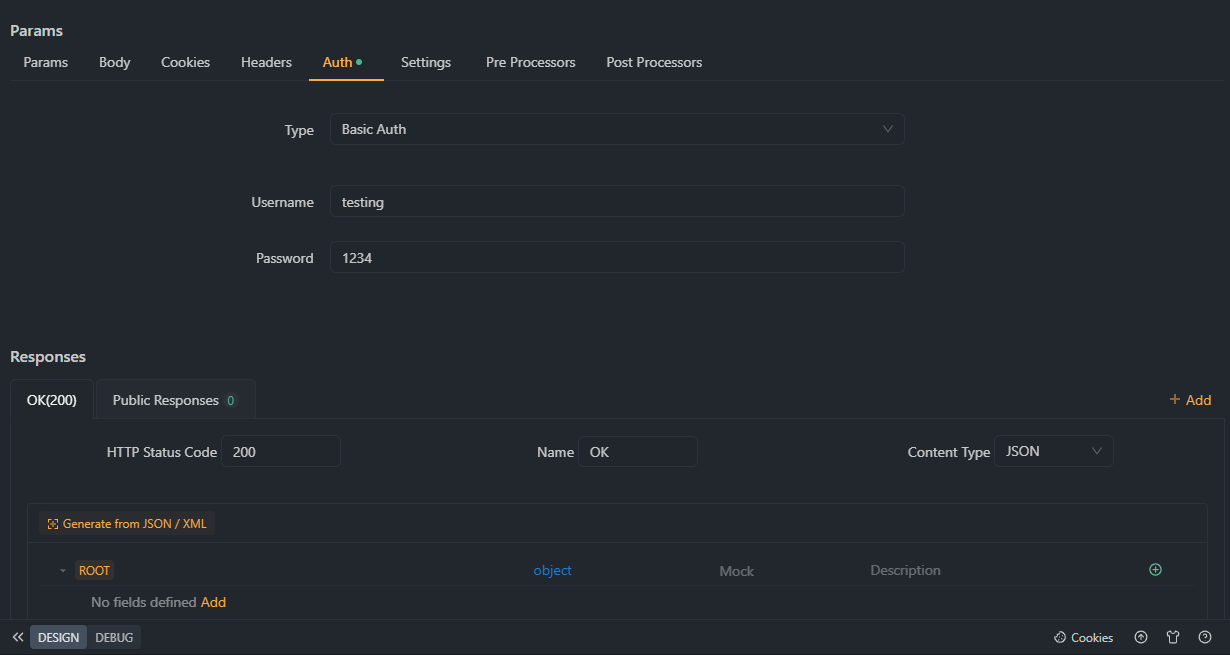
Step 4. Apply Authentication Settings
Once you've configured your user accounts and their corresponding credentials, save your settings and apply them to your API. will generate the necessary code or configuration settings to implement Basic Authentication for your API.
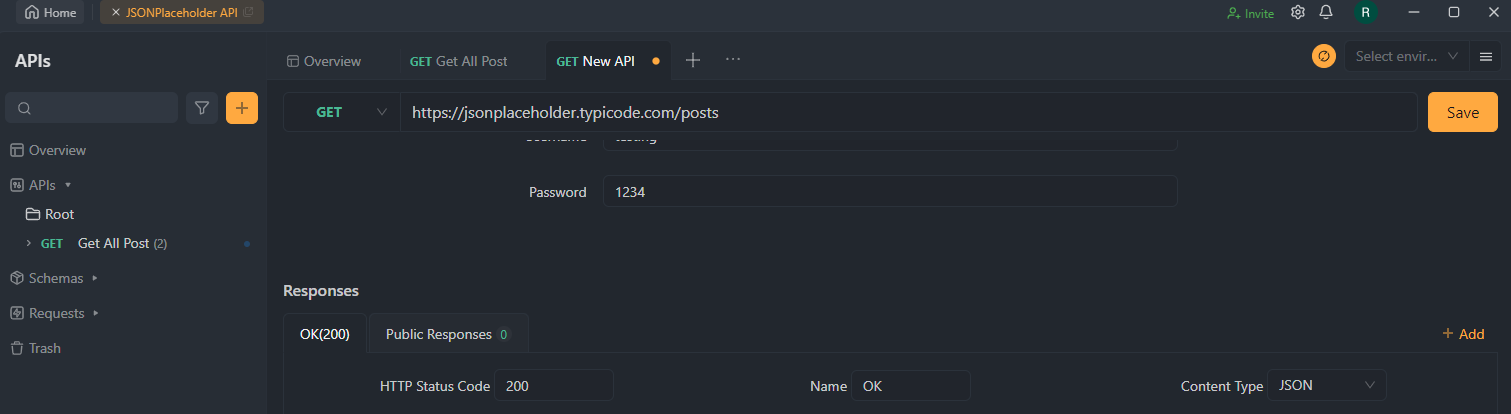
Step 5. Update Your API Code
Integrate the generated code or configuration settings from into your existing API codebase. It will ensure that Basic Authentication is applied to all incoming API requests, requiring valid credentials for access.
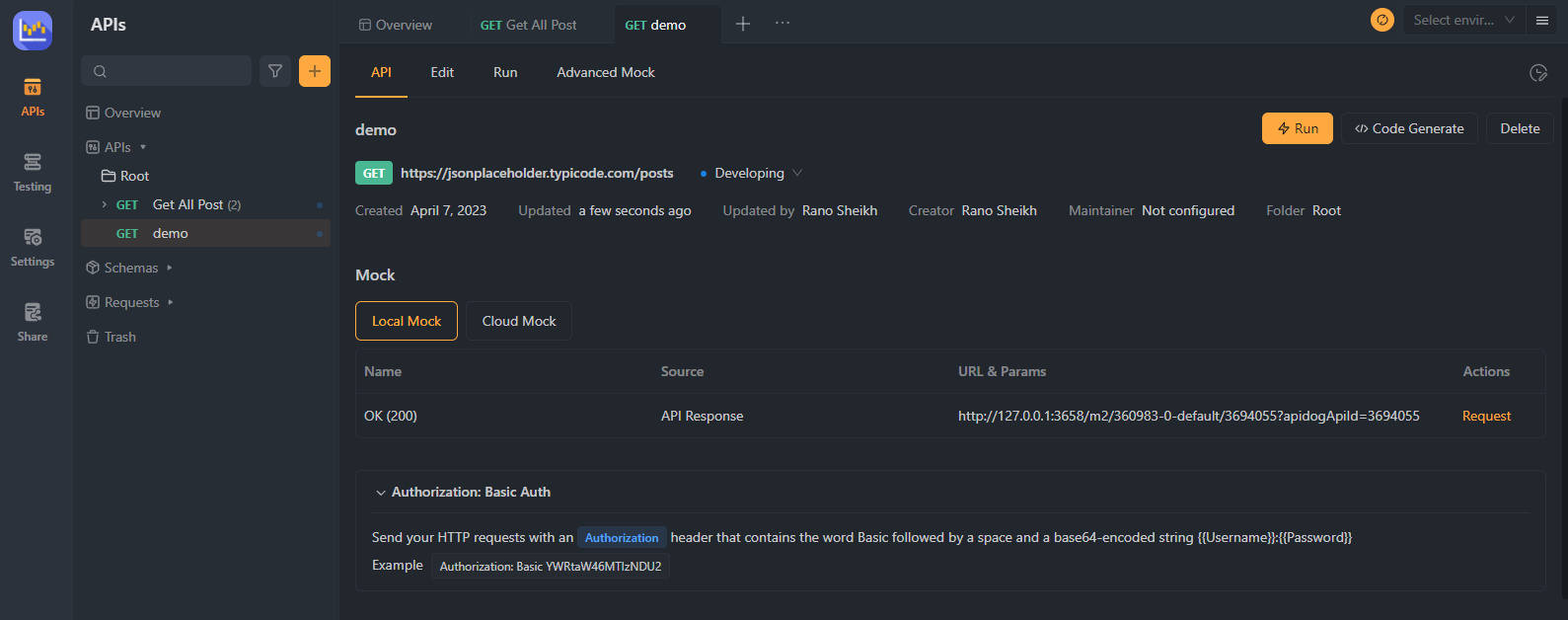
Step 6. Test Your Authentication
Verify that Basic Authentication now protects your API by sending requests using various tools. Ensure that only authorized users with valid credentials can access your API resources and that unauthorized requests are denied.
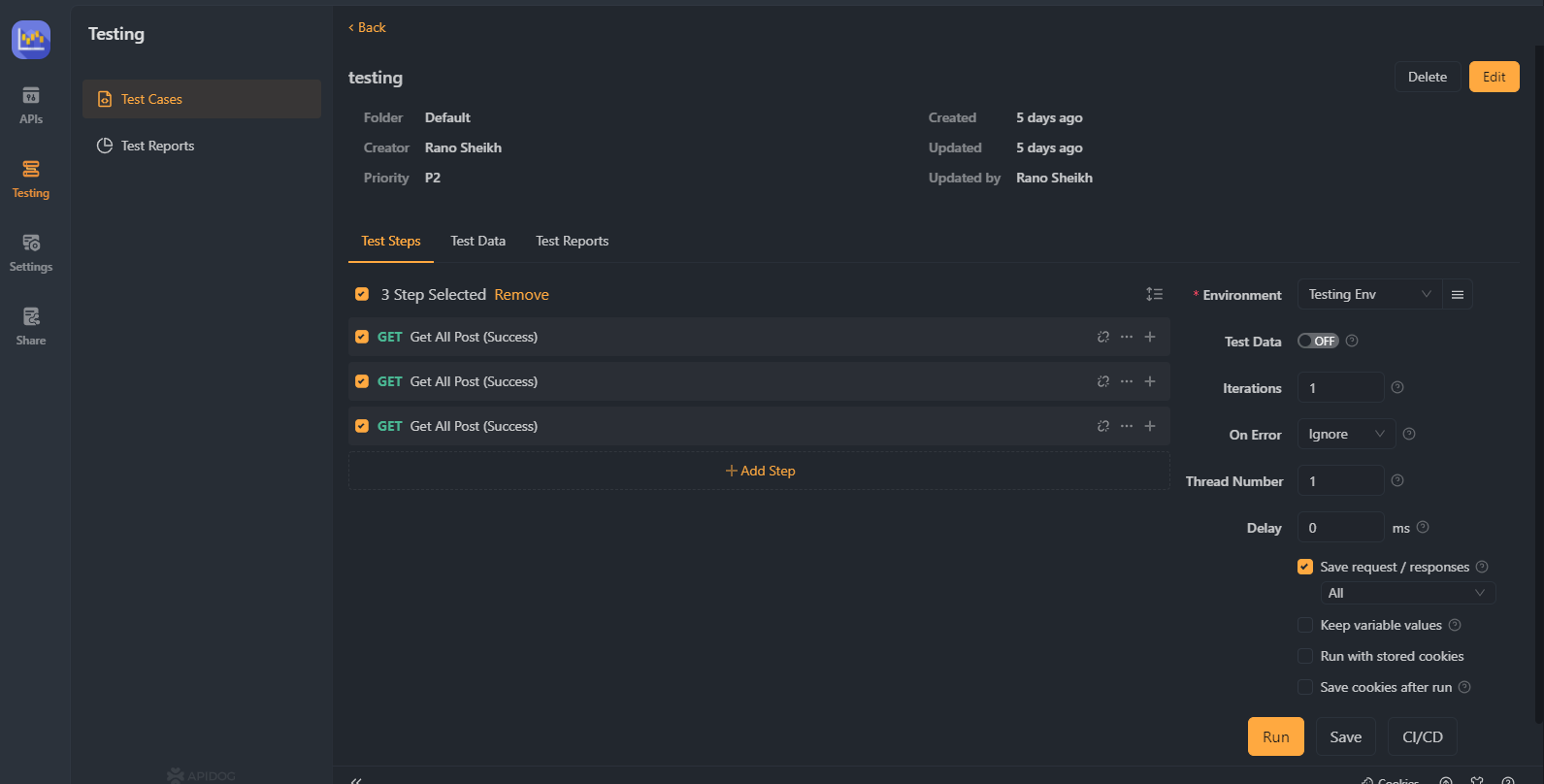
Step 7. Monitor and Analyze API Usage
Utilize Apidog's built-in analytics tools to monitor and analyze your API usage. Track key metrics, such as the number of authenticated requests, response times, and error rates, to assess the effectiveness of your Basic Authentication implementation and make any necessary adjustments.
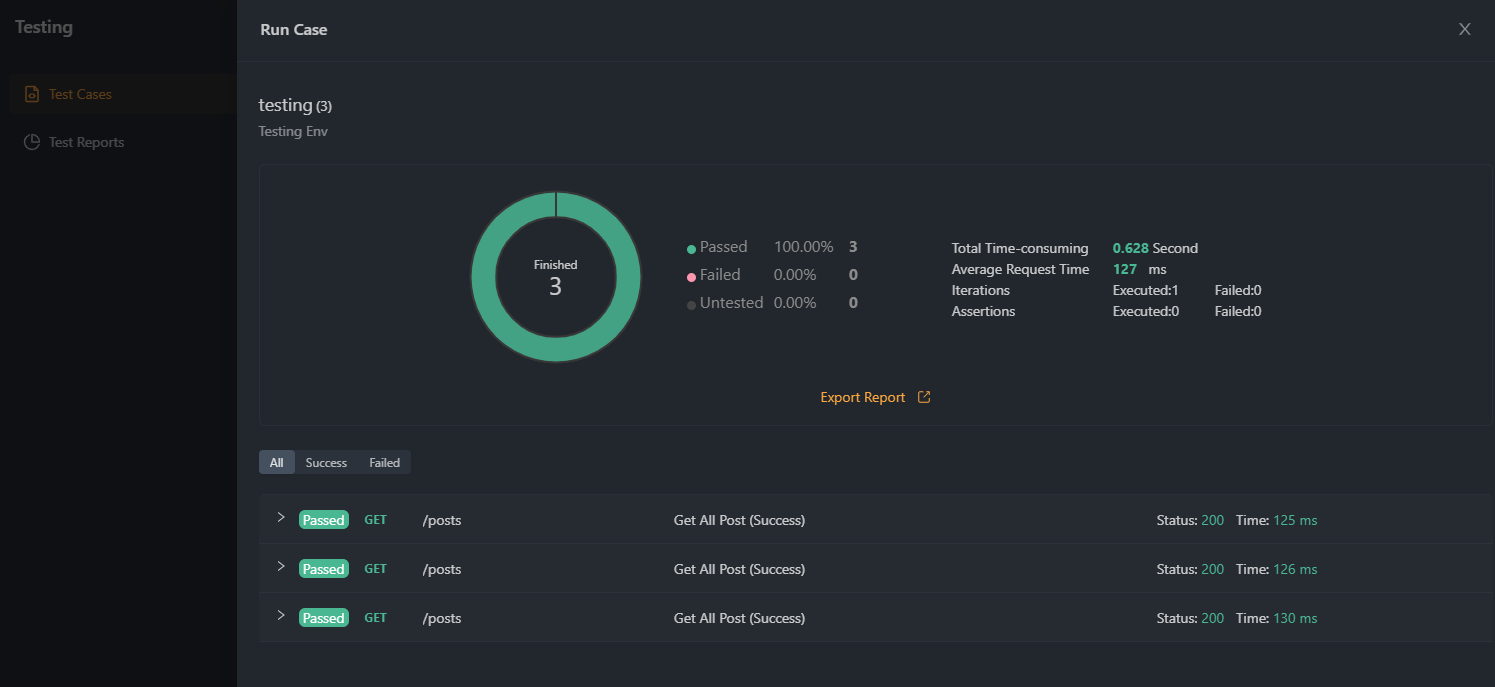
Remember that it supports other authentication methods like Bearer tokens, OAuth, and JWT, which may be more suitable depending on your use case. The process for implementing these methods will be similar, but you'll need to configure the appropriate settings based on the chosen method.
Best Practices of Authentication and Authorization
Best practices are essential for maintaining the security and stability of your applications and systems. Here is a list of recommended practices for authentication and authorization:
Authentication Best Practices:
- Encourage unique passwords: Prompt users to create passwords that combine upper and lower case letters, numbers, and special characters to enhance security.
- Adopt multi-factor authentication (MFA): Strengthen security by requiring users to provide at least two forms of identification, such as a password, a hardware token, or biometric data.
- Safeguard password storage: Use secure hashing and salting techniques when storing passwords, and avoid using plaintext or weak hashing algorithms.
- Set up a secure password reset process: Confirm the user's identity before allowing password resets, like by sending a temporary code to their email or phone number.
- Control login attempts: Prevent brute-force attacks by limiting consecutive login attempts and implementing lockout mechanisms or time delays after failed tries.
- Utilize encrypted communication: Transmit sensitive information via HTTPS and other security protocols, including authentication credentials.
- Keep software updated: Regularly apply the latest security patches to software, libraries, and frameworks to guard against known vulnerabilities.
Authorization Best Practices:
- Principle of least privilege: Allow users and applications only the minimum access required to perform tasks, reducing the risk of data loss or security breaches.
- Implement role-based access control (RBAC): Manage and maintain access controls more easily by assigning permissions to roles instead of individual users.
- Opt for short-lived tokens and sessions: Reduce the risk of unauthorized access by using access tokens or sessions that expire after a certain period of inactivity.
- Use secure communication protocols: Transmit sensitive authorization-related information through HTTPS and other secure communication methods.
By following these best practices for authentication and authorization, you can improve the security of your applications and systems, protect sensitive data, and mitigate the risk of unauthorized access.
Apidog in Action: Real-World Use Cases
Apidog's versatile features and capabilities make it an ideal solution for various applications across various industries. Here are some real-world use cases that demonstrate the power and effectiveness of Apidog:
E-commerce Applications
Protecting client information and payment information is essential in e-commerce. Apidog's strong authentication and access control features make sure that only authorized individuals can access sensitive data, and its real-time security alerts assist in spotting possible risks before they become serious.
Healthcare Solutions
Healthcare applications often handle sensitive patient data, making robust API security essential. Apidog's support for advanced authentication methods and granular access control enables healthcare providers to protect patient information while complying with industry regulations such as HIPAA.
Financial Services
Financial services APIs must safeguard confidential financial data and comply with strict industry regulations. Apidog's versatile authentication options and real-time security monitoring enable financial institutions to maintain robust API security while meeting regulatory requirements.
IoT Devices and Platforms
As IoT devices and platforms proliferate, securing the APIs that enable communication between them is critical. Apidog's scalable platform allows IoT developers to implement strong API security measures that can adapt as their networks expand and evolve.
Social Media and Content Platforms
APIs play a central role in social media and content platforms, enabling users to share and access various types of content. Apidog's comprehensive API security features ensure that user data and content are protected from unauthorized access, helping maintain user trust and platform integrity.
Conclusion
In a world where API security is paramount, stands out as a reliable and comprehensive solution for managing and protecting your APIs. With its versatile authentication options, user-friendly interface, robust security monitoring, and dedicated support, is trustworthy.
Experience the ultimate API management solution with Apidog! Unlock robust security, versatile authentication options, and an intuitive interface. Don't wait – try today and elevate your API security and efficiency to new heights!