10 API Design Best Practices for Building Effective and Efficient APIs
Welcome to a deep dive into the art of API design! In our interconnected digital world, APIs (Application Programming Interfaces) are crucial for facilitating seamless interactions between different software applications. But creating an effective API involves much more than just programming skills; it requires strategic planning, a focus on security, and a user-centric approach. So, let's embark on a journey to explore how you can craft APIs that are not only functional but also robust and intuitive.
Click to Download button below to enjoy Apidog's capabilities in API development.
What is an API?
First things first, what's an API? Imagine you're at a restaurant. The API is like the waiter who takes your order (the request) to the kitchen (the system) and brings back your food (the response). In tech terms, it's a set of rules and protocols for building and interacting with software applications. It's the middleman that lets different software talk to each other seamlessly.
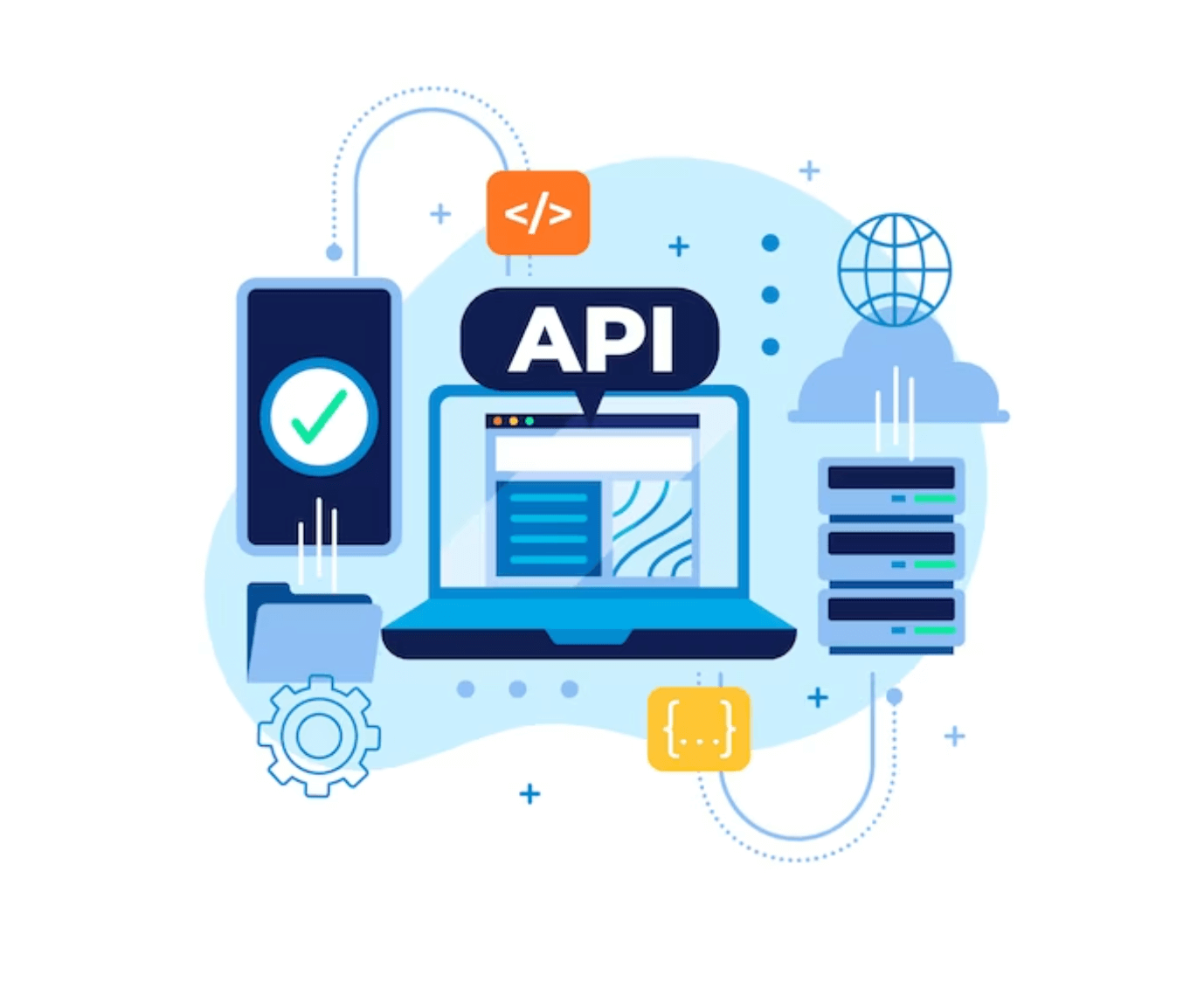
Why Quality API Design Matters
Now, you might wonder, "Why all this fuss about API design?" Here's the thing: a well-designed API can make your software a joy to use, while a poorly designed one can lead to a world of headaches. It's like having a great conversation versus one where you can't get a word in edgewise. Good API design ensures efficient communication between software components, making developers' lives easier and ultimately leading to better user experiences.
The Top 10 Best Practices for API Design
Start with a Strategic Plan
Before you start coding, you need to understand what your API is supposed to do. This involves identifying your target audience, understanding their needs, and defining the problems your API will solve.
api_scope = "E-commerce Data Management"
api_objectives = ["Streamline product data access", "Secure transaction processing", "Real-time inventory management"]
Implement Robust Security Measures
API security is paramount. You need to protect data and user privacy by implementing strong authentication, authorization, and encryption methods.
# Using Flask and Flask-HTTPAuth for Basic Authentication
from flask import Flask
from flask_httpauth import HTTPBasicAuth
app = Flask(__name__)
auth = HTTPBasicAuth()
@auth.verify_password
def verify(username, password):
# Add logic to authenticate users
return username == 'user' and password == 'password'
@app.route('/secure-data')
@auth.login_required
def get_secure_data():
return "Secure Data Access"
Embrace Simplicity and Intuition
Your API should be straightforward to use. Complex APIs can be difficult to integrate and lead to higher learning curves.
# Creating a simple API endpoint in Flask
@app.route('/product/<int:id>', methods=['GET'])
def get_product(id):
product = find
Maintain Consistency
Consistency in API design is like having a uniform language across your platform. It ensures that users don't have to relearn new patterns for different parts of your API. It covers aspects like naming conventions, error messages, and URI structures.
# Consistent naming conventions in Flask API
@app.route('/products/<int:product_id>', methods=['GET'])
def get_product(product_id):
# Logic to retrieve a product
@app.route('/products/<int:product_id>', methods=['PUT'])
def update_product(product_id):
# Logic to update a product
Implement RESTful Principles
RESTful APIs are designed around resources and use HTTP methods explicitly. They are client-server, stateless, cacheable, and layered systems. Embracing RESTful principles means making your API predictable and in line with web standards.
# RESTful API endpoints in Flask
@app.route('/orders', methods=['POST'])
def create_order():
# Logic to create an order
@app.route('/orders/<int:order_id>', methods=['GET'])
def get_order(order_id):
# Logic to retrieve an order
Prioritize Performance
Performance optimization might involve techniques like using faster data access methods, optimizing algorithms, or implementing asynchronous processing. The goal is to make your API respond as quickly as possible while using minimal resources.
Provide Comprehensive Documentation
Your documentation should be clear, concise, and updated regularly. It should cover all aspects of your API, including endpoints, parameters, data formats, and error codes. Tools like Swagger or Redoc can be used to create interactive documentation.
Plan for Evolution with Versioning
Versioning helps in managing changes to your API without breaking compatibility with existing clients. Common strategies include URL versioning, header versioning, or using media types.
Encourage and Utilize User Feedback
User feedback is essential for understanding how your API is being used and what improvements are needed. This can be gathered through surveys, user interviews, or monitoring community forums.
Rigorous and Comprehensive Testing
Your testing strategy should include unit tests for individual components, integration tests for workflows, and end-to-end tests for the entire API. Automated testing frameworks can be beneficial in this regard.
How to Design Effective API with Apidog
API development can be a complex task, but with Apidog, it becomes accessible and manageable. This guide outlines five key steps: defining your API's goals, structuring the API, implementing security protocols, building and testing your API, and documenting and refining the final product for optimal performance and user experience.
Define Your API’s Goals: Establish what you want your API to achieve, who the end-users are, and the functionalities it should offer.
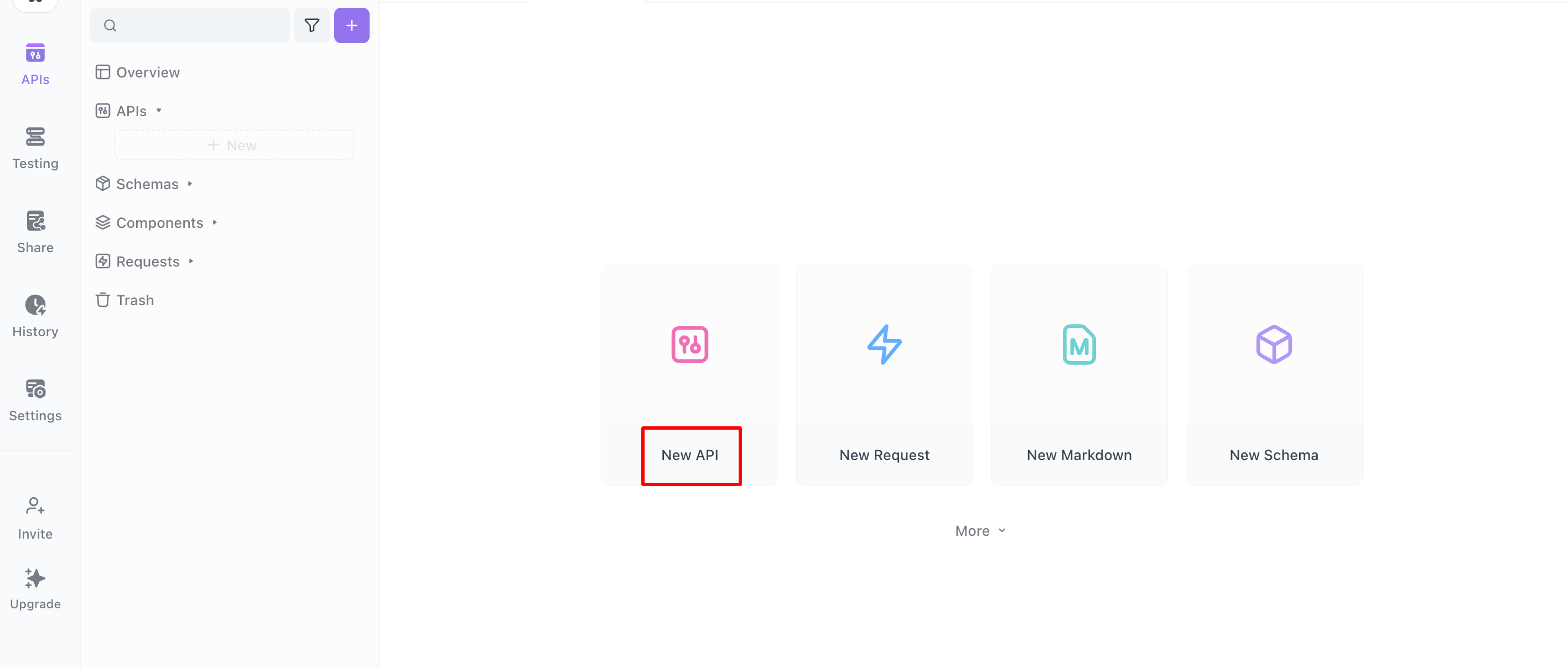
Sketch the API Structure: Outline your API’s endpoints, resources it will manage, and the types of requests it will handle (like GET, POST, PUT, DELETE).
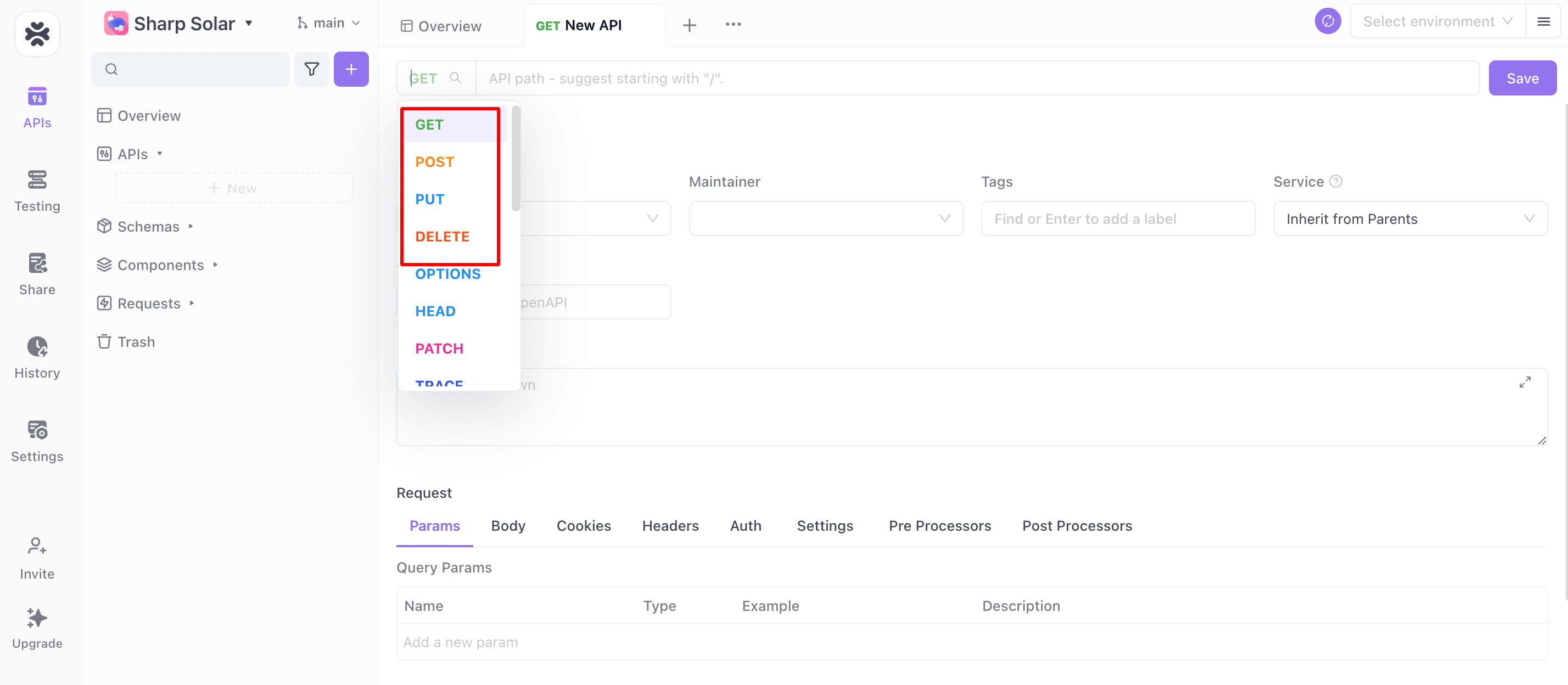
Implement Security Protocols: Ensure to include authentication and authorization mechanisms for data security.
Build and Test Your API: Use Apidog to construct the API, focusing on creating clean, efficient code. Conduct thorough testing to ensure functionality and reliability.
Document and Refine: Provide clear documentation for ease of use and maintainability. After initial deployment, gather feedback to refine and improve the API.
Conclusion
In the realm of software development, APIs are like the connective tissue linking different systems and applications. The art of API design is intricate, requiring a careful balance of technical acumen, foresight, and user-centric design principles. By adhering to these 10 best practices, you are not just building an API; you are crafting an experience, a gateway through which users interact with your application. Remember, a well-designed API is more than a set of functions; it’s a reflection of your commitment to quality, security, and usability. So, as you embark on this journey of API design, keep these principles in mind and strive to create APIs that are not just good, but exceptional. Happy coding, and here's to creating APIs that empower and inspire!