In the digital age, APIs have become the cornerstone of software development, enabling disparate systems to interact seamlessly. This article delves into the world of APIs, with a special focus on aiohttp
, a potent Python library for managing asynchronous HTTP requests. We’ll explore the intricacies of HTTP POST requests, the nuances of aiohttp
setup, and the best practices for crafting secure, high-performance applications. Whether you’re a seasoned developer or new to the field, this guide will equip you with the knowledge to harness the power of APIs and aiohttp
in your projects.
What is a POST Request?
A POST request is used to send data to a server to create or update a resource. The data is included in the body of the request, which allows for more extensive and complex data to be transmitted compared to a GET request.
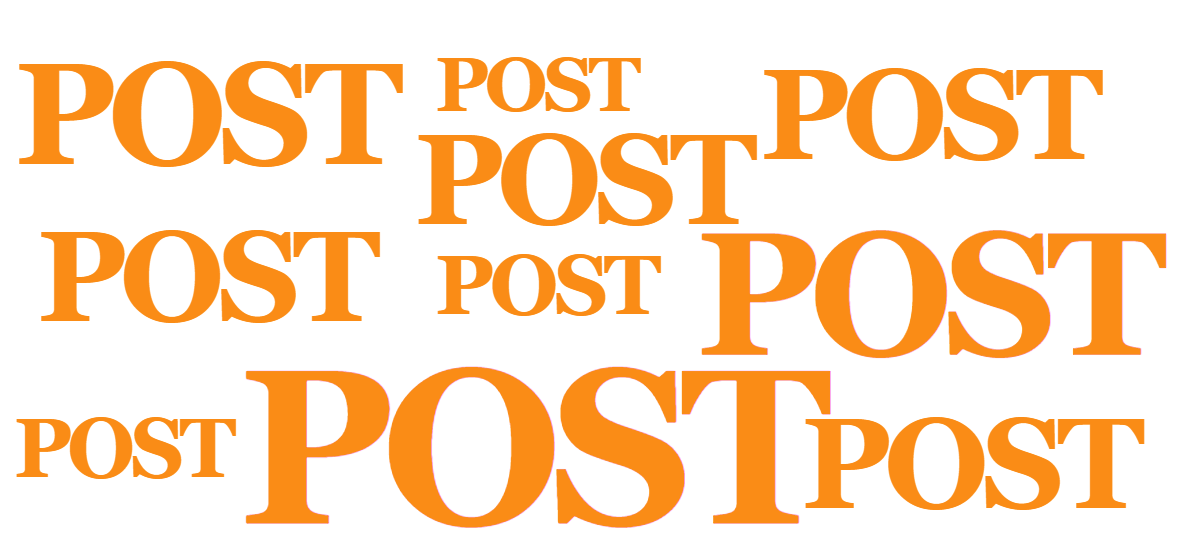
Structure of an HTTP POST Request
An HTTP POST request consists of:
- Start-line: Includes the request method (POST), the request target (URL or path), and the HTTP version.
- Headers: Provide metadata about the request, such as
Content-Type
andContent-Length
. - Empty line: Separates headers from the body.
- Body: Contains the data to be sent to the server.
Here’s a basic example:
POST /path/resource HTTP/1.1
Host: example.com
Content-Type: application/x-www-form-urlencoded
Content-Length: length
field1=value1&field2=value2
When to Use POST Over GET
- Data Submission: Use POST when submitting data to be processed to a server, like in forms.
- Sensitive Data: POST does not expose data in the URL, making it a better choice for sensitive information.
- Non-idempotent Actions: POST is used for operations that change the server state, such as creating or updating resources, where the same operation may have different results if repeated.
- Large or Complex Data: POST has no size limitations and can handle complex data structures, unlike GET.
Remember, while POST requests are more secure than GET requests because the data is not exposed in the URL, they should still be sent over HTTPS to ensure encryption of the data during transmission.
Setting Up Your Environment
To install aiohttp , you can use pip
, the Python package installer. Here’s the command you would typically run in your terminal or command prompt:
pip install aiohttp
If you’re using a specific version of Python or need to ensure that pip
is targeting the right installation, you might use:
python -m pip install aiohttp
or for Python 3 specifically:
pip3 install aiohttp
Here’s a simple example of setting up an aiohttp
session for making HTTP requests:
import aiohttp
import asyncio
async def main():
# Creating a client session
async with aiohttp.ClientSession() as session:
# Making a GET request
async with session.get('http://example.com') as response:
# Printing the response status code
print("Status:", response.status)
# Printing the content of the response
print("Content:", await response.text())
# Running the main coroutine
asyncio.run(main())
This code creates an aiohttp.ClientSession
, which is used to make a GET request to ‘http://example.com’. The response is then printed out, showing the status code and the content of the response. Remember to replace ‘http://example.com’ with the actual URL you wish to request.
Creating the Payload for an HTTP POST Request
To create a payload for an HTTP POST request, you can use a dictionary to represent your data and then convert it to JSON format if necessary. Here’s an example in Python using the json
library:
import json
# Data to be sent
data = {
'key1': 'value1',
'key2': 'value2'
}
# Convert to JSON
json_payload = json.dumps(data)
Sending the Request and Handling the Response using aiohtptp
Using aiohttp
, you can send the POST request with the payload and handle the response as follows:
import aiohttp
import asyncio
import json
async def send_post_request(url, data):
async with aiohttp.ClientSession() as session:
# Sending the POST request
async with session.post(url, data=json.dumps(data)) as response:
# Handling the response
response_data = await response.text()
return response.status, response_data
# URL and data
url = 'http://example.com/api'
data = {'key1': 'value1', 'key2': 'value2'}
# Run the coroutine
asyncio.run(send_post_request(url, data))
Error Handling and Troubleshooting Common Issues
When working with aiohttp
, it’s important to handle exceptions that may occur during the request process. Here’s how you can add error handling:
async def send_post_request(url, data):
try:
async with aiohttp.ClientSession() as session:
async with session.post(url, data=json.dumps(data)) as response:
response.raise_for_status() # Raises an exception for 400 and 500 codes
return await response.text()
except aiohttp.ClientError as e:
print(f'HTTP Client Error: {e}')
except asyncio.TimeoutError as e:
print(f'Request timeout: {e}')
except Exception as e:
print(f'Unexpected error: {e}')
This code snippet includes a try-except block to catch various exceptions, such as ClientError
for client-side errors, TimeoutError
for timeouts, and a general Exception
for any other unexpected errors. It’s also a good practice to log these exceptions for further debugging. Remember to replace 'http://example.com/api'
with the actual endpoint you’re targeting.
How to test aiohttp post request with apidog
Testing an aiohttp
POST request with Apidog involves a few steps to ensure that your API is functioning correctly.
Here is how you can use Apidog to test your aiohttp POST request:
- Open Apidog and create a new request.
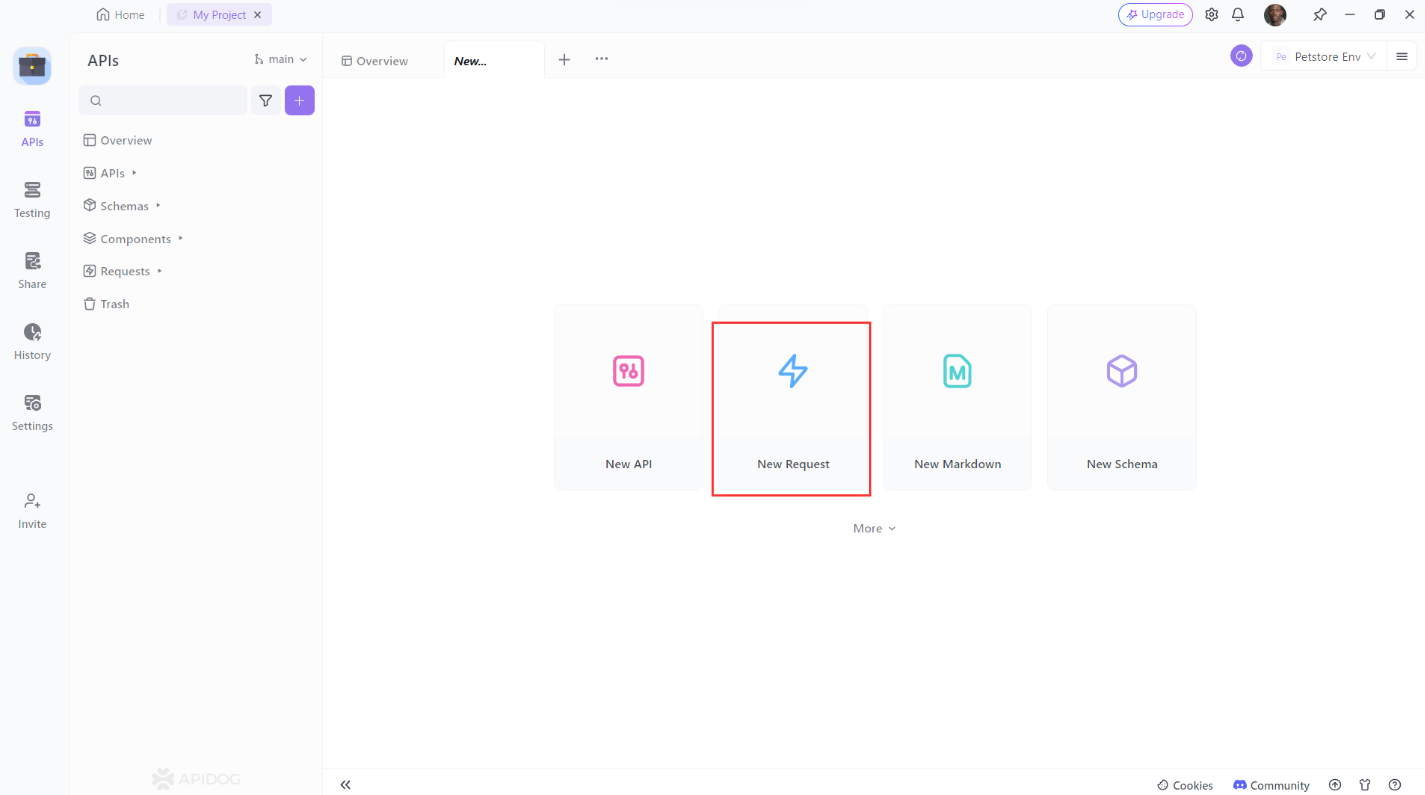
2. Set the request method to POST.
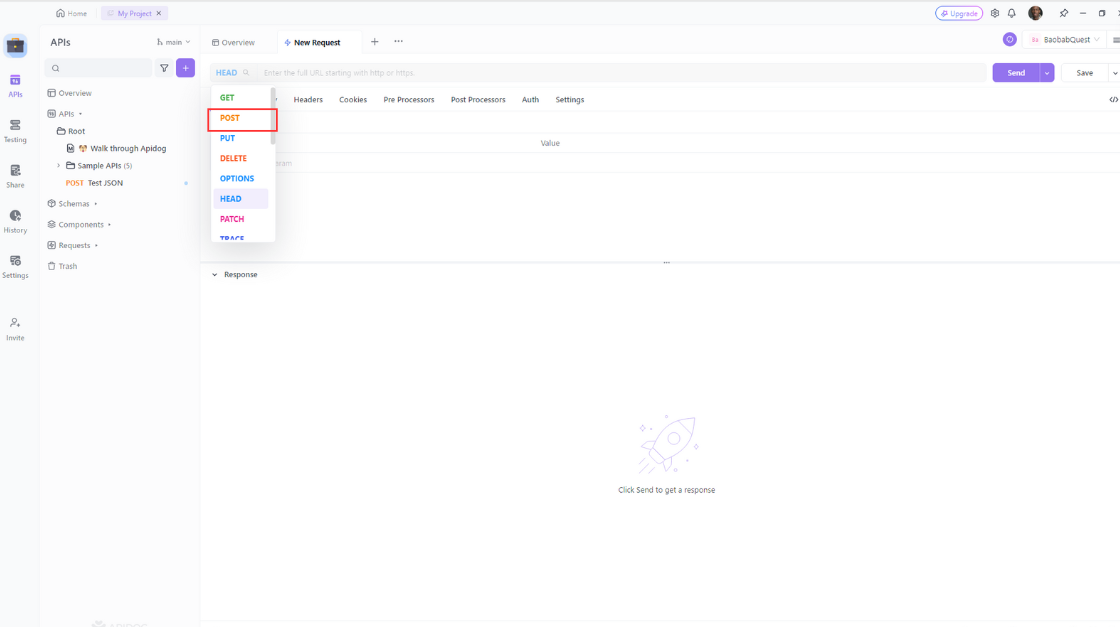
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.
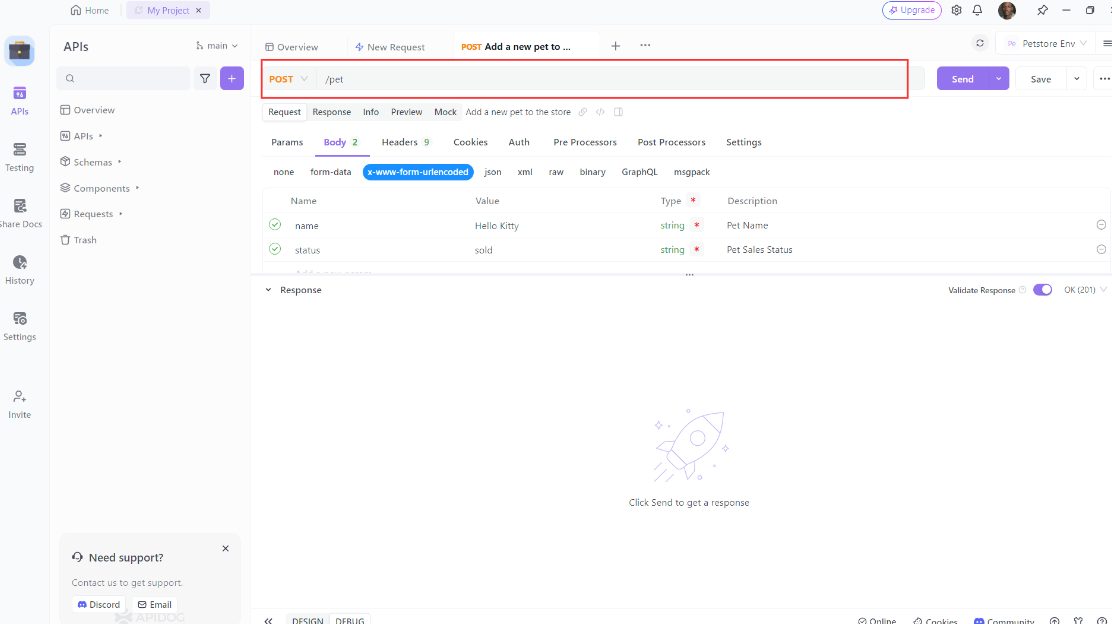
4. Verify that the response is what you expected.
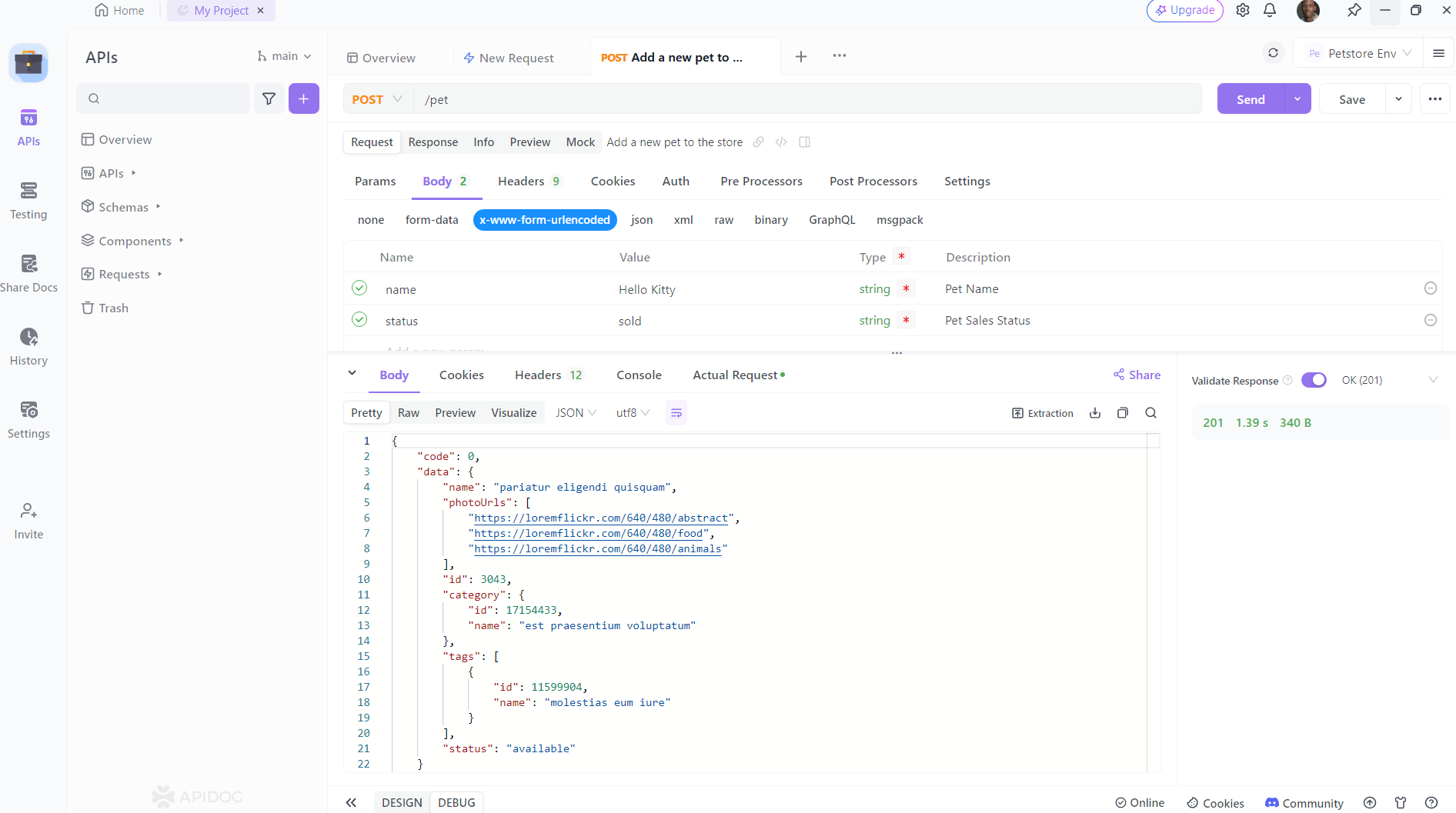
Best Practices for aiohttp POST Requests
When working with aiohttp
and POST requests, it’s important to adhere to best practices to ensure security, optimize performance, and maintain clean code.
Ensuring Security and Privacy
- SSL/TLS: Always use
https
to encrypt data in transit. - Session Management: Use
ClientSession
to manage cookies and headers securely. - Input Validation: Validate and sanitize input to prevent injection attacks.
- Error Handling: Implement proper error handling to avoid exposing sensitive information.
Optimizing Performance
- Connection Pooling: Reuse connections with
ClientSession
to reduce latency. - Asynchronous Operations: Leverage
async
andawait
to handle I/O operations without blocking. - Concurrent Requests: Use
asyncio.gather
to make concurrent requests and improve throughput. - Resource Management: Use context managers to ensure resources like sessions are properly closed.
Writing Clean and Maintainable Code
- Code Structure: Organize code with functions and classes to improve readability.
- Comments and Documentation: Comment your code and maintain up-to-date documentation.
- Consistent Style: Follow PEP 8 or other style guides for consistent code formatting.
- Version Control: Use version control systems like Git to track changes and collaborate.
By following these practices, you can create aiohttp
POST requests that are secure, efficient, and easy to maintain. Remember, the key to successful implementation is continuous learning and adapting to new patterns and practices as they emerge in the community.
Conclusion
APIs are crucial for modern software development, enabling diverse systems to communicate and collaborate. The aiohttp
library is a key player in Python for handling asynchronous HTTP requests, offering a way to build efficient and scalable web applications. Understanding and implementing HTTP POST requests, following best practices, and testing with tools like Apidog are essential for robust API development. As technology evolves, mastering these elements is vital for creating innovative solutions and staying ahead in the field.