Secure Your Async Python Apps with aiohttp oauth2: A Developer's Guide
Dive into the world of asynchronous APIs with our in-depth exploration of aiohttp OAuth2. Learn how this powerful library simplifies authentication and maximizes your API’s efficiency. Perfect for developers looking to streamline their code with async magic!
Welcome to the world of asynchronous programming, where efficiency meets security in the form of aiohttp
and OAuth2. In this article, we’ll dive deep into the seamless integration of these two powerhouses, unlocking the full potential of your APIs with the speed of aiohttp
and the robust security of OAuth2. Whether you’re building a new project or optimizing an existing one, understanding this duo is key to a scalable and secure application.
aiohttp
OAuth2, let’s not forget the role of Apidog in this symphony of code. Apidog is the conductor, ensuring that every note of your API’s performance is documented, tested, and harmonized. With Apidog’s collaborative platform, your API’s lifecycle is managed with precision, from conception to deployment. Downaload Apidog for free.What is aiohttp?
aiohttp is an asynchronous HTTP client/server framework that operates on top of Python’s asyncio
library. It’s designed to support both client and server-side web operations, including handling server websockets and client websockets without the complexity of callback functions.
It’s a powerful tool for developers looking to create high-performance, scalable web applications with modern Python.
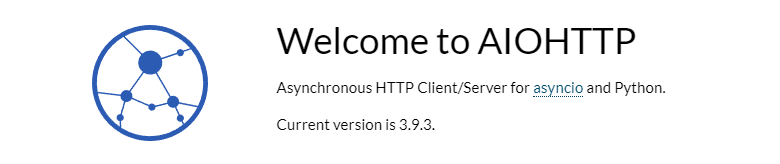
Understanding OAuth2: The Essential Guide to Secure Authorization
OAuth2 is a widely-adopted authorization framework that enables applications to obtain limited access to user accounts on an HTTP service, such as Facebook, GitHub, or Google. It works by delegating user authentication to the service that hosts the user account and authorizing third-party applications to access the user account. OAuth2 provides authorization flows for web and desktop applications, and mobile devices.
The actual implementation of OAuth2 can vary based on the authorization grant type used, but the general flow remains consistent across different scenarios. It’s designed to be flexible and secure, providing a way to standardize the process of secure delegated access.
Combine aiohttp with OAuth2
So, what happens when you combine aiohttp
with OAuth2?
Combining aiohttp
with OAuth2 is like creating a dynamic duo for handling asynchronous web requests with secure authentication. Here’s how they work together:
- Async Requests:
aiohttp
allows you to send asynchronous HTTP requests. This means your application can make multiple requests at the same time without waiting for each one to finish before starting the next. - OAuth2 Authentication: OAuth2 provides a secure way to authenticate these requests. It ensures that only authorized applications can access user data, and it does so without exposing user credentials.
- The Integration: When you integrate OAuth2 with
aiohttp
, you get the best of both worlds. You can make secure, non-blocking requests to services that require OAuth2 authentication. This is particularly useful when you’re dealing with APIs that have rate limits or when you need to handle a large number of requests concurrently. - Token Management:
aiohttp
can handle the entire OAuth2 flow, from obtaining the authorization grant to refreshing access tokens when they expire. This is done in the background, allowing your application to focus on its core functionality. - Error Handling: Since
aiohttp
is asynchronous, it’s important to handle errors properly. OAuth2 errors, such as invalid tokens or expired credentials, need to be caught and managed.aiohttp
provides mechanisms to do this gracefully.
In essence, combiningaiohttp
with OAuth2 allows developers to write cleaner, more efficient code for applications that interact with protected resources. It’s a powerful combination that can greatly enhance the performance and security of your web applications.
What are the benefits of using aiohttp OAuth2
aiohttp OAuth2
rocks for several reasons, especially when it comes to building modern, efficient, and secure web applications. Here’s why developers love using it:
- Asynchronous Efficiency:
aiohttp
is built on Python’sasyncio
library, which means it can handle many requests at once without waiting for each one to complete. This is perfect for high-performance applications that need to make a lot of network requests quickly. - Secure Authentication: OAuth2 is the gold standard for online authentication, and
aiohttp
integrates seamlessly with it. This means you can easily add secure user authentication to your applications without a lot of extra code. - Simplicity and Clean Code: The combination of
aiohttp
and OAuth2 allows for writing code that is both simple and clean. Developers can focus on their application logic rather than the intricacies of network programming and security. - Token Management: Managing tokens is a breeze with
aiohttp OAuth2
. It handles everything from obtaining tokens to refreshing them when they expire, all in an asynchronous manner that doesn’t block your application. - Community and Support: Both
aiohttp
and OAuth2 have strong communities and plenty of documentation, which means it’s easy to find help and resources when you need them.
In short, aiohttp OAuth2
provides a powerful, efficient, and secure way to handle web requests and authentication in modern Python applications. It’s a combination that enables developers to build scalable and robust systems while maintaining readable and maintainable codebases.
Getting Started with aiohttp OAuth2
Getting started with aiohttp
and OAuth2 for asynchronous API development involves a few key steps.
Install aiohttp: Make sure you have aiohttp
installed. You can install it using pip:
pip install aiohttp
Understand OAuth2: Familiarize yourself with the OAuth2 authorization framework and its various grant types. This knowledge will be crucial when implementing OAuth2 with aiohttp
.
Set Up OAuth2: Depending on the OAuth2 grant type you’re using, set up the necessary credentials with the service you’re authenticating against. This typically involves registering your application to get a client ID and client secret.
Implement OAuth2 with aiohttp: Use aiohttp
to handle the OAuth2 flow. This will involve creating an aiohttp.ClientSession
and using it to obtain, refresh, and use access tokens to make authorized requests to the API.
Handle Responses and Errors: Make sure to handle API responses and potential errors properly. This includes checking response statuses and managing exceptions.
Secure Your Tokens: Always keep your tokens secure and manage their lifecycle appropriately. This includes storing them securely and refreshing them before they expire.
Test Your Implementation: Thoroughly test your OAuth2 implementation to ensure it works as expected and handles edge cases and errors gracefully.
Remember, when working with asynchronous code and authentication, it’s important to write robust and secure code that can handle multiple scenarios and potential issues.
How to test your aiohttp OAuth2 API with Apidog?
Integrating aiohttp
with OAuth2 in the context of using Apidog can streamline the process of building and managing APIs. Apidog is an all-in-one collaborative API development platform that supports the entire API lifecycle, including design, documentation, debugging, mocking, and automated testing.
Setting up Apidog for your aiohttp
OAuth2 API involves several steps to ensure that your API is properly documented and tested.
- Open Apidog and create a new request.
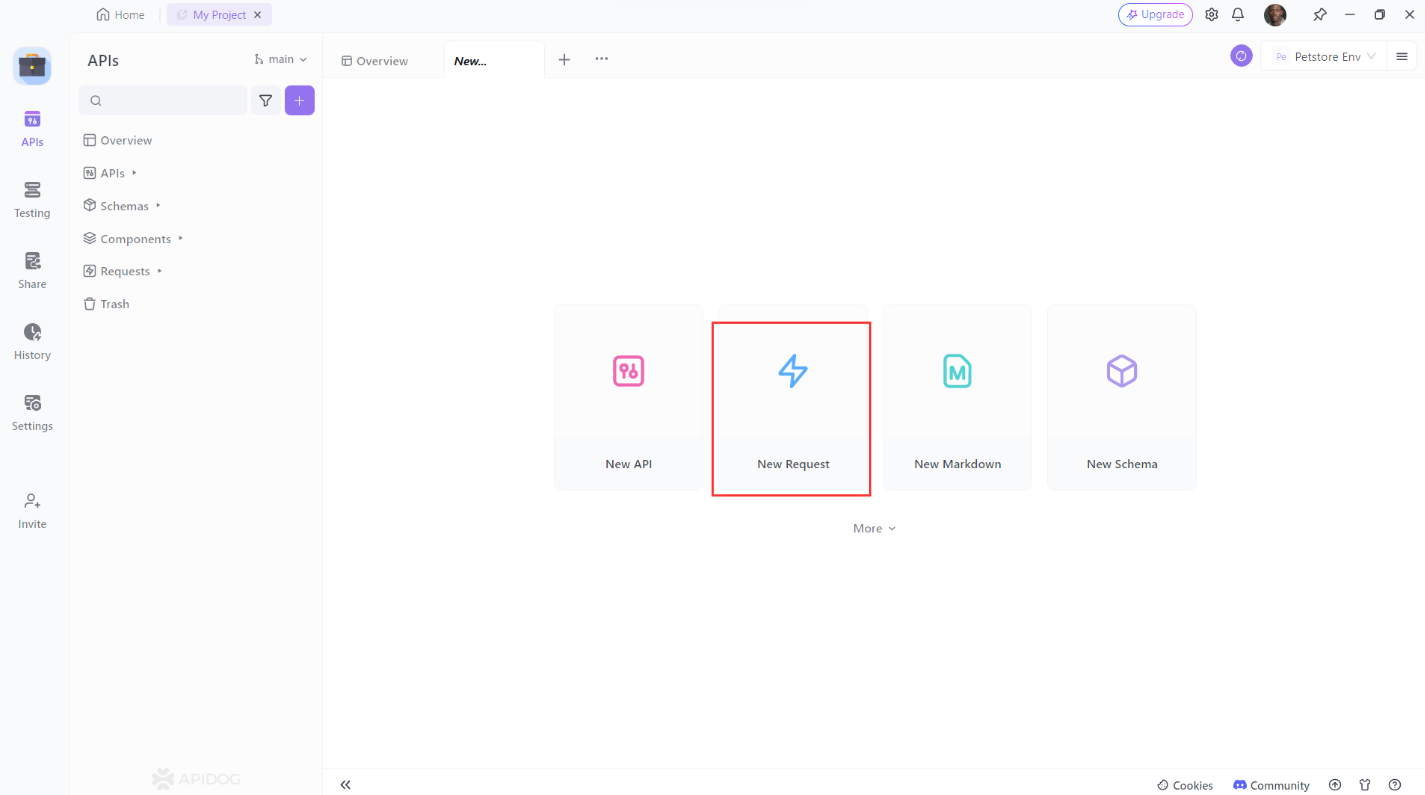
2. Set the request method to POST.
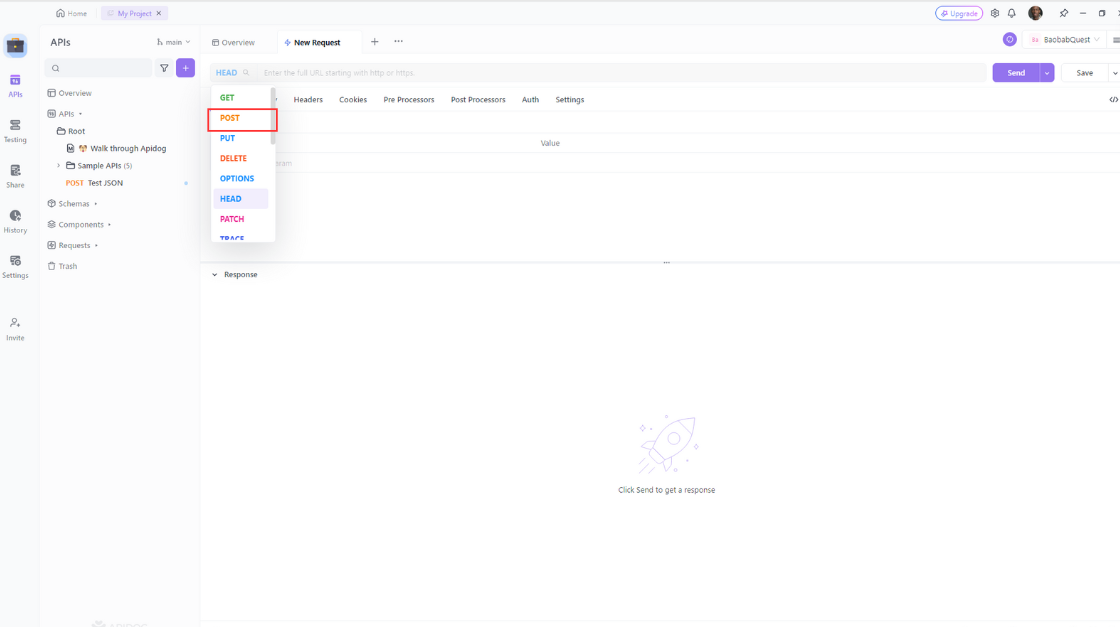
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.
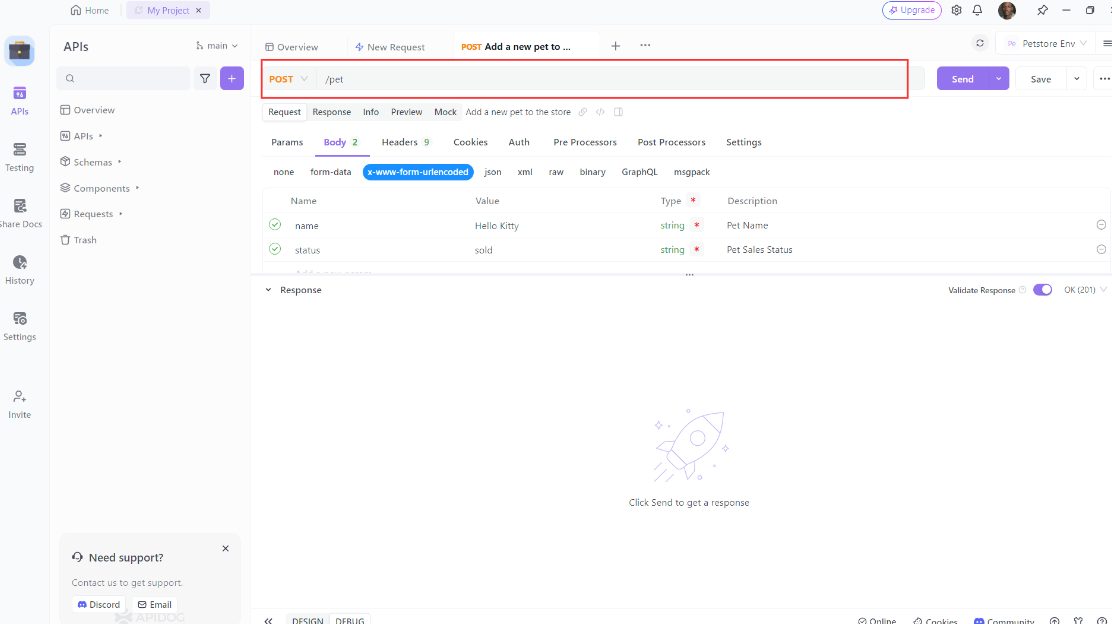
4. Verify that the response is what you expected.
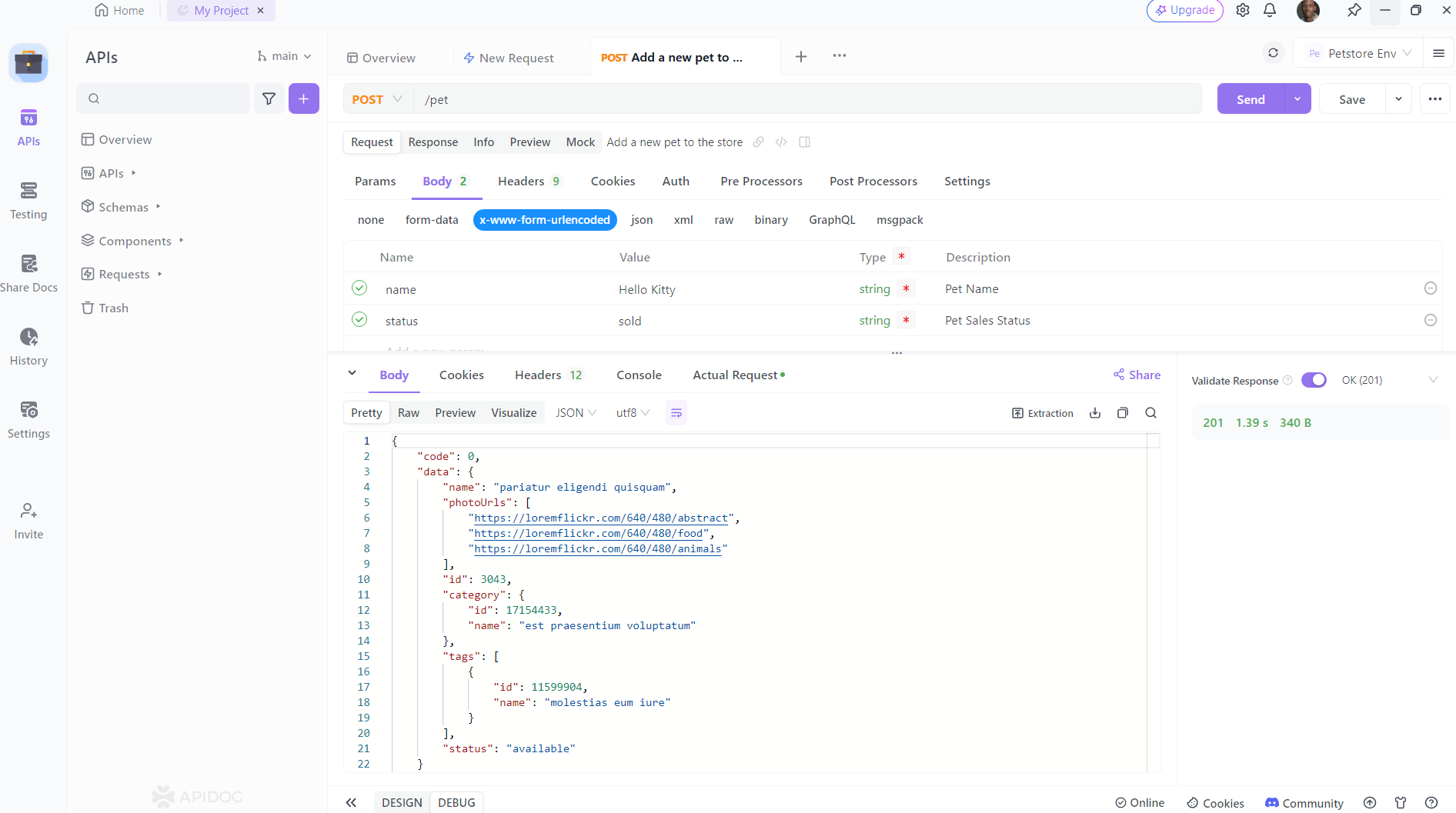
Use Apidog’s built-in tools to send requests to your API and verify the responses. Make sure to test the OAuth2 flow to ensure that it’s working as expected.
Best Practices for aiohttp OAuth2
When working with aiohttp
and OAuth2, following best practices is crucial for creating secure, efficient, and maintainable applications. Here are some key best practices to consider:
- Use Secure Connections: Always use HTTPS to protect the data transmitted between the client and the server.
- Manage Tokens Securely: Store access tokens and sensitive information securely. Avoid hard-coding credentials in your source code.
- Handle Token Lifecycle: Implement proper token expiration and renewal mechanisms. Be prepared to handle token refresh scenarios.
- Error Handling: Implement robust error handling to manage issues like network failures or invalid tokens gracefully.
- Asynchronous Patterns: Understand and correctly implement asynchronous patterns to avoid common pitfalls like deadlocks or race conditions.
- Testing: Write comprehensive tests for your OAuth2 flows to ensure they work correctly under various scenarios.
- Dependency Management: Keep your dependencies, including
aiohttp
and any OAuth2 libraries, up to date to benefit from the latest security patches and features. - Logging and Monitoring: Implement logging and monitoring to keep track of OAuth2 flow successes and failures, which can be crucial for debugging and security auditing.
- Follow OAuth2 Best Practices: Stay informed about the latest OAuth 2.0 Security Best Current Practice and implement the recommended security measures.
By adhering to these best practices, you can ensure that your use of aiohttp
with OAuth2 is as secure and effective as possible.
Conclusion
To wrap up, aiohttp
and OAuth2 together provide a powerful framework for building secure, efficient, and scalable asynchronous web applications. With the added support of Apidog for API management, developers can ensure their APIs are well-documented and thoroughly tested. This trio offers a streamlined path for modern API development, making it an excellent choice for any developer in the Python ecosystem.