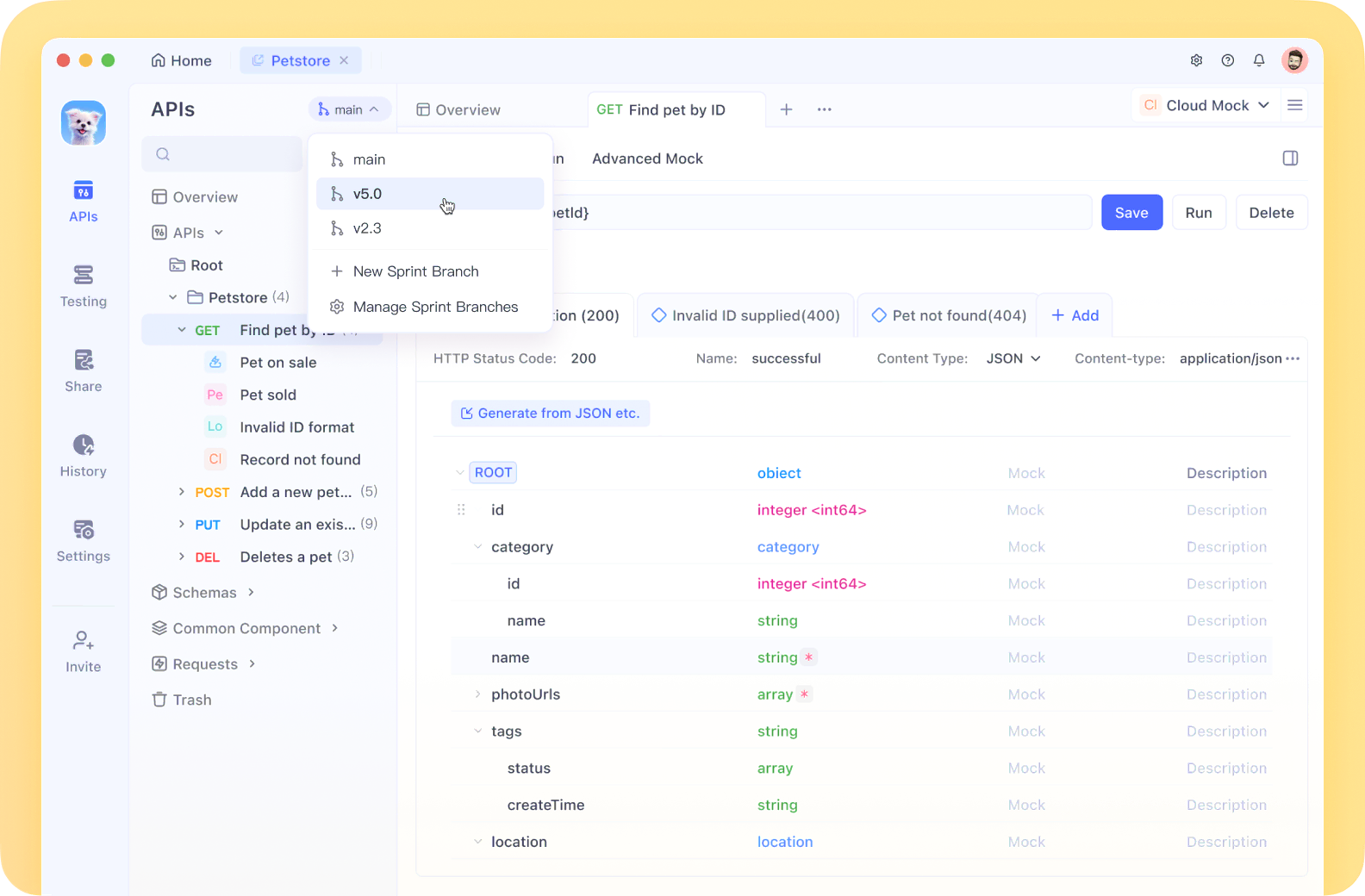
Khi chúng ta nghĩ về Selenium WebDriver, chúng ta thường liên tưởng nó với tự động hóa trình duyệt và kiểm tra giao diện người dùng. Tuy nhiên, Selenium cũng có thể là một công cụ mạnh mẽ cho việc kiểm tra API khi được sử dụng đúng cách. Hướng dẫn này sẽ hướng dẫn bạn qua quy trình tận dụng các khả năng của Selenium WebDriver để thực hiện kiểm tra API, cung cấp cho bạn các ví dụ thực tế và các thực tiễn tốt nhất.
Kiểm tra API (Giao diện lập trình ứng dụng) liên quan đến việc kiểm tra các API của ứng dụng trực tiếp, xác minh chức năng, độ tin cậy, hiệu suất và bảo mật của chúng. Trong khi có những công cụ chuyên dụng cho việc kiểm tra API như Postman, REST Assured hoặc SoapUI, Selenium WebDriver có thể là một bổ sung quý giá cho bộ công cụ kiểm tra API của bạn, đặc biệt khi bạn muốn kết hợp kiểm tra giao diện người dùng và API trong cùng một khuôn khổ.
Yêu cầu trước khi bắt đầu
Trước khi bắt đầu kiểm tra API với Selenium WebDriver, hãy đảm bảo rằng bạn đã có:
- Kiến thức cơ bản về Selenium WebDriver
- Một ngôn ngữ lập trình mà bạn chọn (Java, Python, C#, JavaScript)
- Selenium WebDriver được thiết lập trong môi trường phát triển của bạn
- Hiểu biết về các API REST/SOAP
- Kiến thức cơ bản về các phương thức HTTP (GET, POST, PUT, DELETE)
Hiểu về Kiến trúc
Kiến trúc của Selenium WebDriver khiến nó phù hợp cho kiểm tra API thông qua khả năng:
- Gửi yêu cầu HTTP trực tiếp bằng cách sử dụng các thư viện tích hợp hoặc bên ngoài
- Xử lý phản hồi và xác minh dữ liệu
- Được tích hợp với các khuôn khổ thử nghiệm hiện có
- Quản lý dữ liệu thử nghiệm và môi trường
Thiết lập Môi trường của bạn
Hãy thiết lập một môi trường cơ bản cho việc kiểm tra API với Selenium WebDriver:
Ví dụ Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class SeleniumApiTesting {
private WebDriver driver;
public void setUp() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless"); // Chế độ đầu không để kiểm tra API
driver = new ChromeDriver(options);
}
public void tearDown() {
if (driver != null) {
driver.quit();
}
}
}
Ví dụ Python
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
import requests
import json
def setup_driver():
chrome_options = Options()
chrome_options.add_argument("--headless") # Chế độ đầu không để kiểm tra API
driver = webdriver.Chrome(options=chrome_options)
return driver
def teardown_driver(driver):
if driver is not None:
driver.quit()
Các phương pháp kiểm tra API với Selenium WebDriver
1. Sử dụng Thư viện HTTP Bản địa
Cách tiếp cận đơn giản nhất là sử dụng các thư viện HTTP bản địa của ngôn ngữ lập trình của bạn cùng với Selenium WebDriver.
Ví dụ Java - Yêu cầu GET
public String performGetRequest(String endpoint) throws IOException {
URL url = new URL(endpoint);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
System.out.println("Mã phản hồi GET: " + responseCode);
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
} else {
return "Yêu cầu GET thất bại với mã phản hồi: " + responseCode;
}
}
Ví dụ Python - Yêu cầu POST
def perform_post_request(endpoint, payload):
headers = {'Content-Type': 'application/json'}
response = requests.post(endpoint, data=json.dumps(payload), headers=headers)
print(f"Mã phản hồi POST: {response.status_code}")
if response.status_code == 200:
return response.json()
else:
return f"Yêu cầu POST thất bại với mã phản hồi: {response.status_code}"
2. Sử dụng JavaScriptExecutor cho các Cuộc gọi AJAX
Cách tiếp cận khác là sử dụng JavaScriptExecutor của Selenium để thực hiện các cuộc gọi AJAX trực tiếp từ trình duyệt.
Ví dụ Java
import org.openqa.selenium.JavascriptExecutor;
public String performApiCallWithJsExecutor(String endpoint, String method, String payload) {
String script = String.format(
"var xhr = new XMLHttpRequest();" +
"xhr.open('%s', '%s', false);" +
"xhr.setRequestHeader('Content-Type', 'application/json');" +
"xhr.send('%s');" +
"return xhr.responseText;",
method, endpoint, payload
);
return (String) ((JavascriptExecutor) driver).executeScript(script);
}
Ví dụ Python
def perform_api_call_with_js_executor(driver, endpoint, method, payload):
script = f"""
var xhr = new XMLHttpRequest();
xhr.open('{method}', '{endpoint}', false);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.send('{payload}');
return xhr.responseText;
"""
return driver.execute_script(script)
3. Chặn và Chỉnh sửa các Cuộc gọi API
Selenium WebDriver có thể được sử dụng để chặn và chỉnh sửa các cuộc gọi API được thực hiện bởi ứng dụng, đặc biệt khi kết hợp với các công cụ proxy như BrowserMob Proxy.
Ví dụ Java với BrowserMob Proxy
import net.lightbody.bmp.BrowserMobProxy;
import net.lightbody.bmp.BrowserMobProxyServer;
import net.lightbody.bmp.client.ClientUtil;
import org.openqa.selenium.Proxy;
public void setupProxyForApiInterception() {
// Bắt đầu proxy
BrowserMobProxy proxy = new BrowserMobProxyServer();
proxy.start(0);
// Lấy đối tượng proxy Selenium
Proxy seleniumProxy = ClientUtil.createSeleniumProxy(proxy);
// Cấu hình Chrome để sử dụng proxy
ChromeOptions options = new ChromeOptions();
options.setCapability("proxy", seleniumProxy);
// Tạo phiên bản WebDriver
driver = new ChromeDriver(options);
// Thêm bộ lọc yêu cầu để chặn các cuộc gọi API
proxy.addRequestFilter((request, contents, messageInfo) -> {
if (request.getUri().contains("/api/")) {
System.out.println("Chặn cuộc gọi API: " + request.getUri());
// Chỉnh sửa tiêu đề, tham số, hoặc thân yêu cầu nếu cần
}
return null;
});
// Thêm bộ lọc phản hồi để kiểm tra và chỉnh sửa phản hồi API
proxy.addResponseFilter((response, contents, messageInfo) -> {
if (messageInfo.getOriginalRequest().getUri().contains("/api/")) {
String responseBody = contents.getTextContents();
System.out.println("Phản hồi API: " + responseBody);
// Chỉnh sửa phản hồi nếu cần
}
});
}
Xây dựng một Khung Kiểm Tra API Hoàn Chỉnh
Hãy tạo một khung kiểm tra API có cấu trúc hơn bằng Selenium WebDriver:
Ví dụ Java
import org.json.JSONObject;
import org.testng.Assert;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
import java.io.IOException;
public class ApiTestFramework {
private WebDriver driver;
private final String BASE_URL = "<https://api.example.com>";
@BeforeClass
public void setUp() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless");
driver = new ChromeDriver(options);
}
@Test
public void testGetUserEndpoint() throws IOException {
String endpoint = BASE_URL + "/api/users/1";
String response = performGetRequest(endpoint);
JSONObject jsonResponse = new JSONObject(response);
Assert.assertEquals(jsonResponse.getString("name"), "John Doe");
Assert.assertEquals(jsonResponse.getInt("id"), 1);
Assert.assertTrue(jsonResponse.has("email"));
}
@Test
public void testCreateUserEndpoint() throws IOException {
String endpoint = BASE_URL + "/api/users";
JSONObject payload = new JSONObject();
payload.put("name", "Jane Smith");
payload.put("email", "jane.smith@example.com");
String response = performPostRequest(endpoint, payload.toString());
JSONObject jsonResponse = new JSONObject(response);
Assert.assertTrue(jsonResponse.has("id"));
Assert.assertEquals(jsonResponse.getString("name"), "Jane Smith");
}
private String performGetRequest(String endpoint) throws IOException {
// Triển khai từ ví dụ trước
}
private String performPostRequest(String endpoint, String payload) throws IOException {
URL url = new URL(endpoint);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
connection.setDoOutput(true);
// Ghi payload vào kết nối
try (java.io.OutputStream os = connection.getOutputStream()) {
byte[] input = payload.getBytes("utf-8");
os.write(input, 0, input.length);
}
int responseCode = connection.getResponseCode();
System.out.println("Mã phản hồi POST: " + responseCode);
try (BufferedReader br = new BufferedReader(
new InputStreamReader(connection.getInputStream(), "utf-8"))) {
StringBuilder response = new StringBuilder();
String responseLine;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
return response.toString();
}
}
@AfterClass
public void tearDown() {
if (driver != null) {
driver.quit();
}
}
}
Ví dụ Python
import unittest
import json
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
import requests
class ApiTestFramework(unittest.TestCase):
BASE_URL = "<https://api.example.com>"
def setUp(self):
chrome_options = Options()
chrome_options.add_argument("--headless")
self.driver = webdriver.Chrome(options=chrome_options)
def test_get_user_endpoint(self):
endpoint = self.BASE_URL + "/api/users/1"
response = self.perform_get_request(endpoint)
self.assertEqual(response['name'], "John Doe")
self.assertEqual(response['id'], 1)
self.assertIn('email', response)
def test_create_user_endpoint(self):
endpoint = self.BASE_URL + "/api/users"
payload = {
"name": "Jane Smith",
"email": "jane.smith@example.com"
}
response = self.perform_post_request(endpoint, payload)
self.assertIn('id', response)
self.assertEqual(response['name'], "Jane Smith")
def perform_get_request(self, endpoint):
response = requests.get(endpoint)
return response.json() if response.status_code == 200 else None
def perform_post_request(self, endpoint, payload):
headers = {'Content-Type': 'application/json'}
response = requests.post(endpoint, data=json.dumps(payload), headers=headers)
return response.json() if response.status_code in [200, 201] else None
def tearDown(self):
if self.driver:
self.driver.quit()
if __name__ == '__main__':
unittest.main()
Kết hợp Kiểm Tra Giao Diện và API
Một trong những lợi thế lớn nhất của việc sử dụng Selenium WebDriver cho việc kiểm tra API là khả năng kết hợp kiểm tra giao diện và kiểm tra API trong cùng một khuôn khổ:
@Test
public void testLoginAndVerifyUserDataAPI() throws IOException {
// Phần giao diện: Đăng nhập qua giao diện
driver.get("<https://example.com/login>");
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("password");
driver.findElement(By.id("loginButton")).click();
// Chờ cho việc đăng nhập hoàn tất
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.urlContains("/dashboard"));
// Trích xuất mã thông báo xác thực từ cookie hoặc bộ nhớ cục bộ
String authToken = (String) ((JavascriptExecutor) driver)
.executeScript("return localStorage.getItem('authToken');");
// Phần API: Sử dụng mã thông báo để thực hiện cuộc gọi API đã xác thực
String endpoint = BASE_URL + "/api/user/profile";
URL url = new URL(endpoint);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setRequestProperty("Authorization", "Bearer " + authToken);
// Xử lý và xác minh phản hồi API
int responseCode = connection.getResponseCode();
Assert.assertEquals(responseCode, 200);
// Đọc và phân tích phản hồi
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String response = in.lines().collect(Collectors.joining());
in.close();
JSONObject jsonResponse = new JSONObject(response);
// Xác minh rằng dữ liệu API khớp với những gì hiển thị trong giao diện
String uiUsername = driver.findElement(By.id("profileUsername")).getText();
Assert.assertEquals(jsonResponse.getString("username"), uiUsername);
}
Các Thực Tiễn Tốt Nhất cho Kiểm Tra API với Selenium WebDriver
- Sử dụng Chế độ Đầu không: Khi thực hiện các bài kiểm tra API thuần túy, hãy chạy Selenium ở chế độ đầu không để tiết kiệm tài nguyên.
- Phân tách Kiểm Tra API và Giao Diện: Mặc dù bạn có thể kết hợp chúng, hãy giữ các bài kiểm tra API và giao diện tách biệt về mặt logic.
- Tận dụng Thư viện Giả định: Sử dụng các thư viện giả định toàn diện để xác thực các phản hồi API.
- Triển khai Xử lý Lỗi: Thêm xử lý lỗi phù hợp cho các lỗi API.
- Ghi lại các Yêu cầu và Phản hồi API: Để mục đích gỡ lỗi, hãy ghi lại tất cả các tương tác API.
- Các Bài kiểm tra Tham số hóa: Sử dụng các nhà cung cấp dữ liệu hoặc kiểm tra tham số hóa để thử nghiệm các kịch bản khác nhau.
- Triển khai Xử lý Xác thực: Tạo các phương pháp có thể tái sử dụng để xử lý xác thực.
- Xem xét Hiệu suất: Hãy nhớ rằng Selenium có thể thêm tải, so với các công cụ kiểm tra API chuyên dụng.
Hạn chế của việc Sử dụng Selenium WebDriver cho Kiểm tra API
- Tải Hiệu suất: Selenium chủ yếu được thiết kế cho tự động hóa trình duyệt, vì vậy nó thêm tải cho việc kiểm tra API thuần túy.
- Các Tính năng Cụ thể về API bị Hạn chế: Các công cụ kiểm tra API chuyên biệt cung cấp nhiều tính năng cụ thể hơn cho việc kiểm tra API.
- Phụ thuộc vào Trình duyệt: Ngay cả trong chế độ đầu không, Selenium yêu cầu có một trình duyệt, điều này có thể không cần thiết cho kiểm tra API.
- Độ Phức tạp: Thiết lập Selenium cho kiểm tra API có thể phức tạp hơn so với việc sử dụng các công cụ kiểm tra API chuyên dụng.
Khi nào Nên Sử dụng Selenium WebDriver cho Kiểm tra API
Selenium WebDriver phù hợp nhất cho kiểm tra API trong các tình huống sau:
- Khi bạn đã có một khung kiểm tra giao diện dựa trên Selenium và muốn mở rộng nó.
- Khi bạn cần kiểm tra những kịch bản liên quan đến cả giao diện và tương tác API.
- Khi bạn cần kiểm tra API trong bối cảnh trình duyệt (như kiểm tra các cuộc gọi API JavaScript).
- Khi bạn muốn chặn và chỉnh sửa các cuộc gọi API được thực hiện bởi ứng dụng.
Kết luận
Selenium WebDriver có thể là một công cụ đa năng cho việc kiểm tra API, đặc biệt là khi được tích hợp với các khung kiểm tra giao diện hiện có. Mặc dù nó có thể không thay thế các công cụ kiểm tra API chuyên dụng cho những nhu cầu kiểm tra API phức tạp, nhưng nó cung cấp một cách linh hoạt để kết hợp kiểm tra giao diện và API trong cùng một khung.
Bằng cách tận dụng các phương pháp và ví dụ được cung cấp trong hướng dẫn này, bạn có thể sử dụng hiệu quả Selenium WebDriver cho các yêu cầu kiểm tra API của mình. Hãy nhớ xem xét các thỏa hiệp và chọn công cụ phù hợp cho nhu cầu kiểm tra cụ thể của bạn.
Dù bạn đang kiểm tra REST API, dịch vụ SOAP, hay các điểm cuối GraphQL, Selenium WebDriver có thể giúp bạn tạo ra các bài kiểm tra toàn diện xác thực cả giao diện người dùng của ứng dụng và chức năng API cơ bản của nó.
Đối với những nhu cầu kiểm tra API nâng cao hơn, bạn có thể muốn xem xét việc kết hợp Selenium WebDriver với các thư viện hoặc khung kiểm tra API chuyên dụng như REST Assured (cho Java) hoặc Requests (cho Python) để đạt được lợi ích tốt nhất từ cả hai thế giới.
Chúc bạn kiểm tra vui vẻ!