In computer science, serialization and deserialization are essential processes that involve converting complex data structures into a format suitable for storage or transmission and then reconstructing them back to their original form.
Introducing to you Apidog, a comprehensive API development platform that provides complete tools for the entire API lifecycle. You can build, test, mock, and document APIs within a single application.
To learn more about Apidog, make sure to check out the button below.
What is Serialization
Serialization is the process of converting complex data structures (such as objects and arrays) into a format suitable for transmission over networks.
This usually refers to text-based representation, such as JSON and XML.
Why is Serialization So Important?
- Data Transfer: APIs exchange data between clients and servers. Serialization transforms complex data into a transportable format.
- Language and Platform Independence: Different systems might use different programming languages or platforms. Serialization ensures data can be understood regardless of the underlying technology.
- Efficiency: Serialized data is often more compact than its original form, improving network transmission speed.
- Readability: Text-based formats like JSON and XML are human-readable, making debugging and troubleshooting easier.
What is Deserialization
Deserialization is the inverse process of serialization. It involves converting data from a serialized format (like JSON, XML, or Protobuf) back into its original data structure, often an object in memory.
Importance of Deserialization in APIs
- Data Processing: Deserialization allows the client to manipulate and use the received data effectively.
- Object Creation: It transforms the raw data into usable objects for further operations.
- Error Handling: Deserialization processes can handle potential errors like data corruption or format mismatches.
Code Examples of Serialization and Deserialization
Example 1 - Python with JSON
Serialization
import json
class Person:
def __init__(self, name, age, city):
self.name = name
self.age = age
self.city = city
person = Person("Alice", 30, "New York")
# Serialize to JSON
json_data = json.dumps(person.__dict__)
print(json_data)
Deserialization
import json
# Assuming json_data is the same as above
# Deserialize from JSON
person_dict = json.loads(json_data)
deserialized_person = Person(**person_dict)
print(deserialized_person.name)
Example 2 - Java with ObjectOutputStream and ObjectInputStream
Serialization
import java.io.*;
class Person implements Serializable {
private String name;
private int age;
// Constructor, getters, and setters
}
public class SerializationExample {
public static void main(String[] args) throws IOException {
Person person = new Person("Bob", 25);
FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(person);
out.close();
fileOut.close();
}
}
Deserialization
import java.io.*;
class DeserializationExample {
public static void main(String[] args) throws IOException, ClassNotFoundException {
FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Person person = (Person) in.readObject();
in.close();
fileIn.close();
System.out.println(person.getName());
}
}
Example 3 - C# with Binary Formatter
Serialization
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
[Serializable]
class Person {
public string Name;
public int Age;
}
class Program {
static void Main(string[] args)
{
Person person = new Person { Name = "Charlie", Age = 35 };
BinaryFormatter formatter = new BinaryFormatter();
using (FileStream stream = new FileStream("person.bin", FileMode.Create, FileAccess.Write))
{
formatter.Serialize(stream, person);
}
}
}
Deserialization
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
class Program {
static void Main(string[] args)
{
BinaryFormatter formatter = new BinaryFormatter();
using (FileStream stream = new FileStream("person.bin", FileMode.Open, FileAccess.Read))
{
Person person = (Person)formatter.Deserialize(stream);
Console.WriteLine(person.Name);
}
}
}
Challenges and Best Practices for Serialization and Deserialization
Serialization and deserialization, while fundamental, present several challenges that developers must address:
Challenges
Performance
- Serialization and deserialization can be computationally expensive, especially for large datasets or complex objects.
- The choice of serialization format significantly impacts performance.
Data Integrity
- Ensuring that data is accurately represented and not corrupted during serialization and deserialization is crucial.
- Data loss or inconsistencies can lead to application errors.
Compatibility
- Maintaining compatibility between different versions of serialized data is essential for evolving systems.
- Schema changes can break deserialization processes.
Security
- Serialized data can be vulnerable to attacks like injection and deserialization vulnerabilities.
- Protecting sensitive information during serialization and deserialization is critical.
Best Practices
Choose the right serialization format
- Consider factors like data size, performance, readability, and compatibility when selecting a format (JSON, XML, Protobuf, etc.).
Optimize performance
- Use efficient algorithms and data structures.
- Consider compression for large datasets.
- Profile your application to identify performance bottlenecks.
Validate data
- Implement strict data validation to prevent invalid or malicious data from being deserialized.
- Use schema validation or data type checking.
Handle errors gracefully
- Implement robust error-handling mechanisms to deal with deserialization failures.
- Provide informative error messages.
Versioning
- Plan for schema evolution and backward compatibility.
- Use versioning mechanisms or compatibility layers.
Security
- Protect sensitive data through encryption or obfuscation.
- Validate input to prevent injection attacks.
- Keep serialization libraries and dependencies up-to-date with security patches.
Testing
- Thoroughly test serialization and deserialization processes.
- Include edge cases and invalid data in test scenarios.
Apidog: One-Stop Solution to All Your API Problems
If you are looking for an API development platform for facilitating your API processes or an alternative to other API tools like Postman and Swagger, then you should check out Apidog.
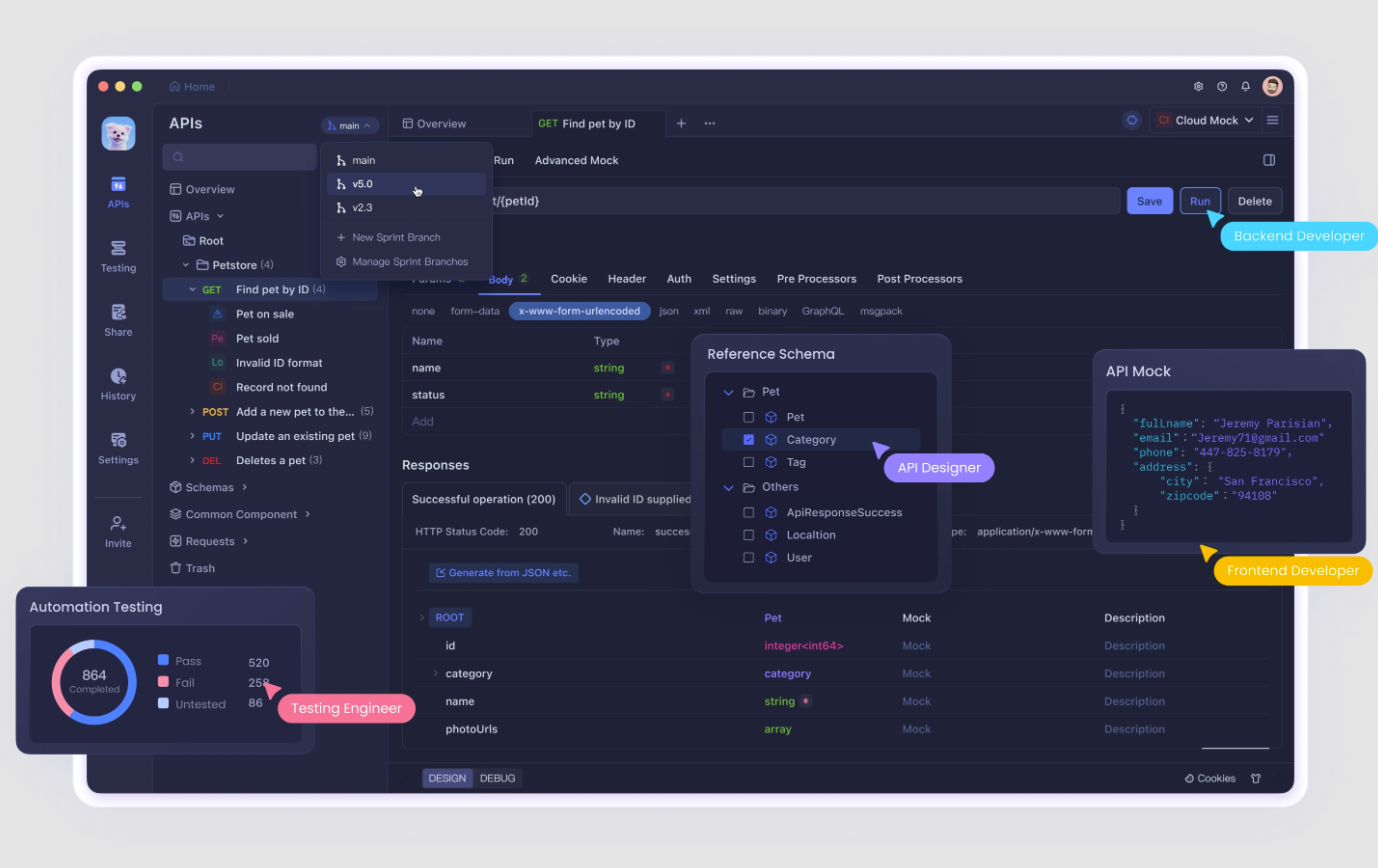
Create APIs with Apidog
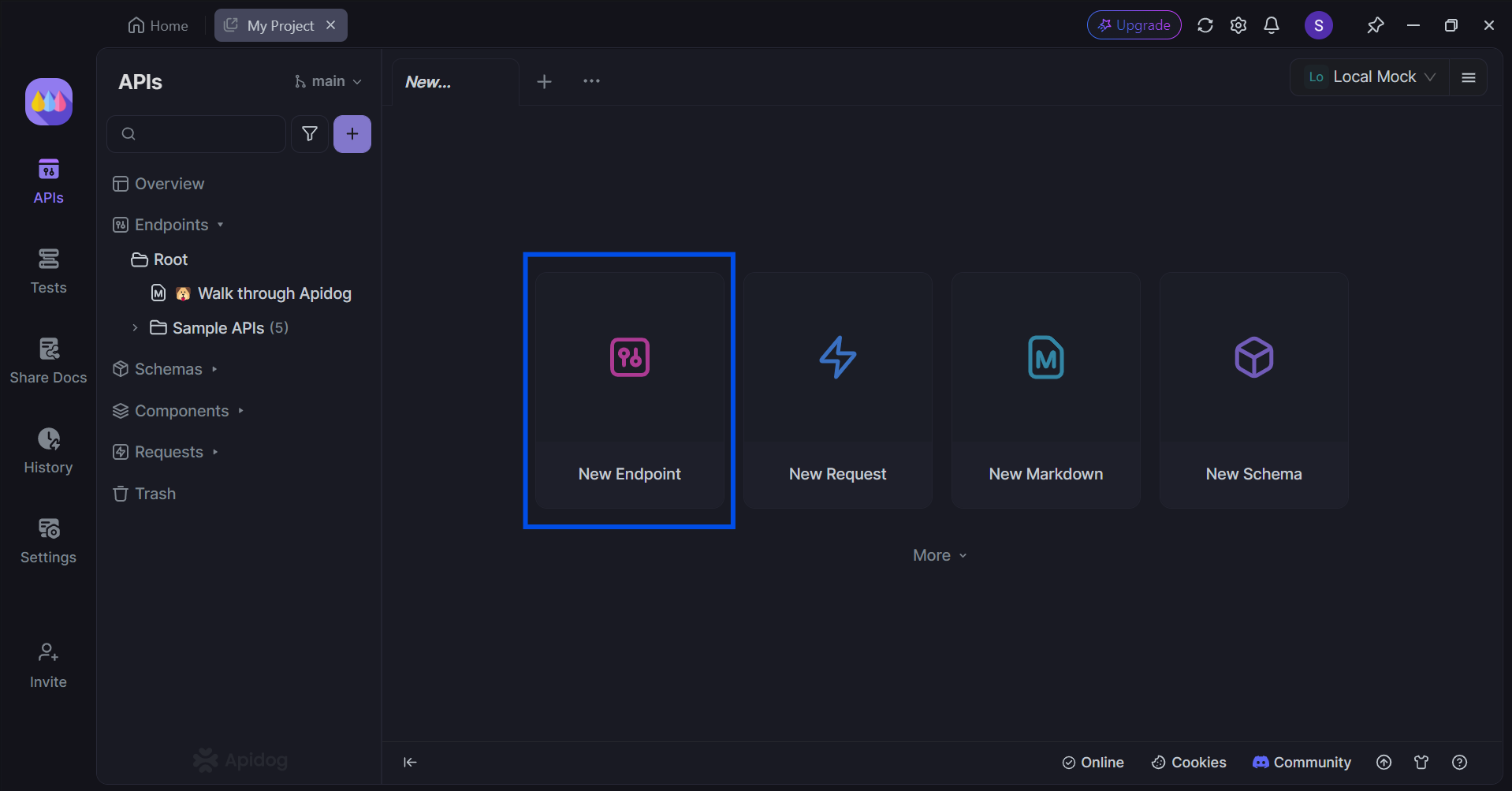
Once you have downloaded Apidog for free, you can initialize a new project. Start by creating a new endpoint for your new application!
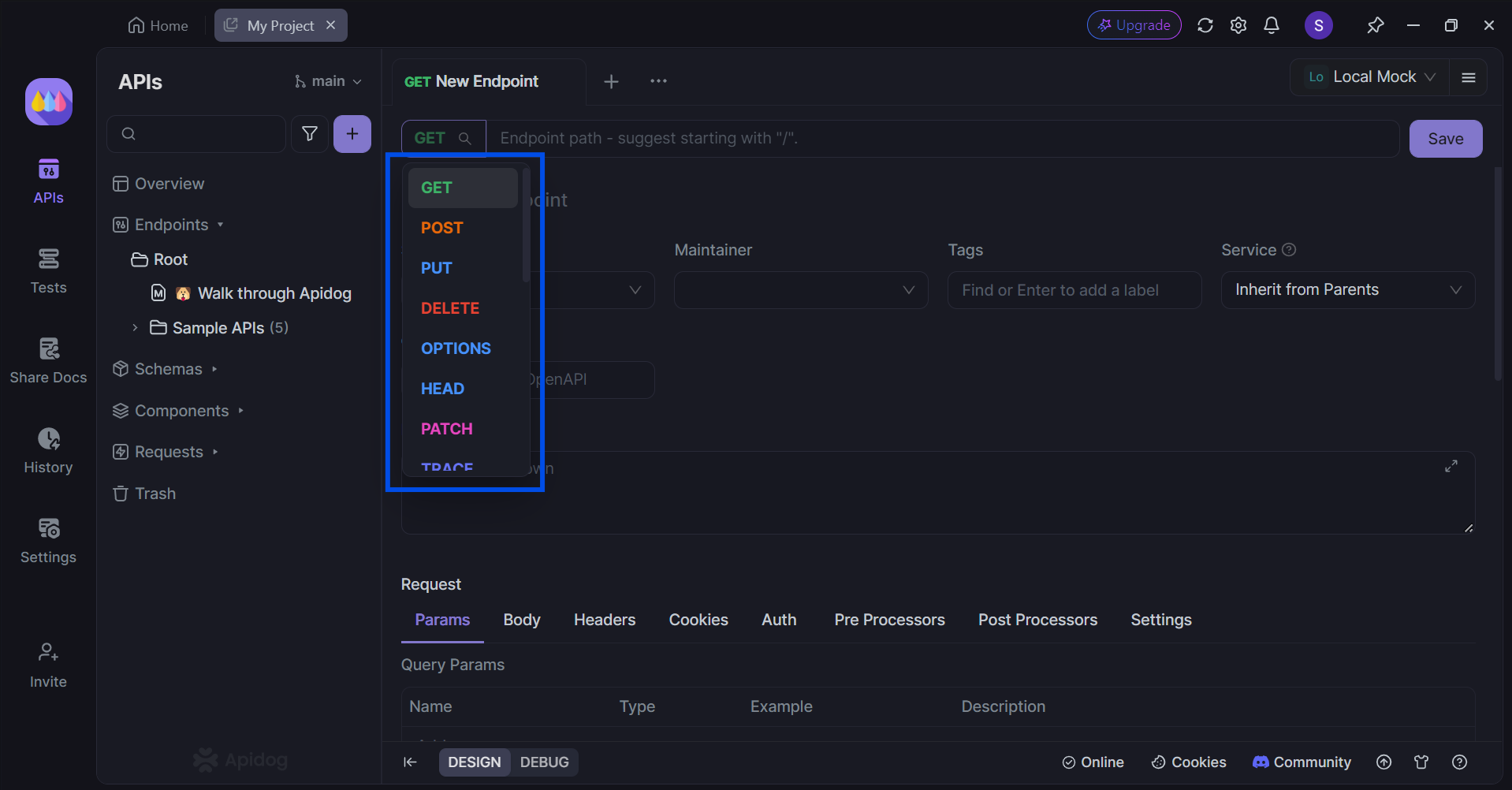
Next, select the HTTP method associated with your API endpoint. During this phase, it is encouraged to include all the necessary details, such as parameters, headers, pre-processor and post-processor scripts.
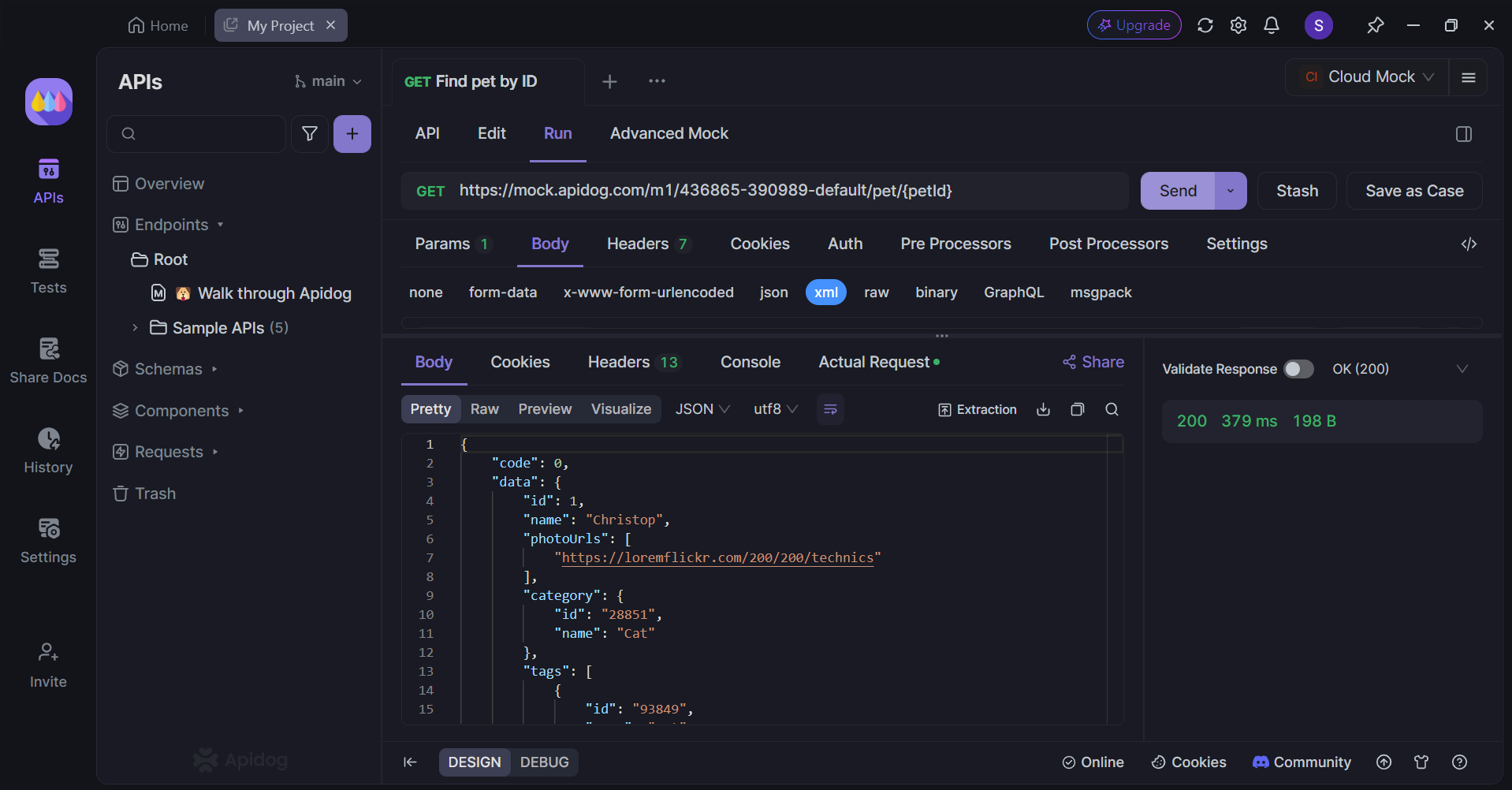
Once you have completed your API, hit the Send
button to check out the API response. The simple yet intuitive user interface makes it effortless to identify problems.
Produce Professional API Documentation
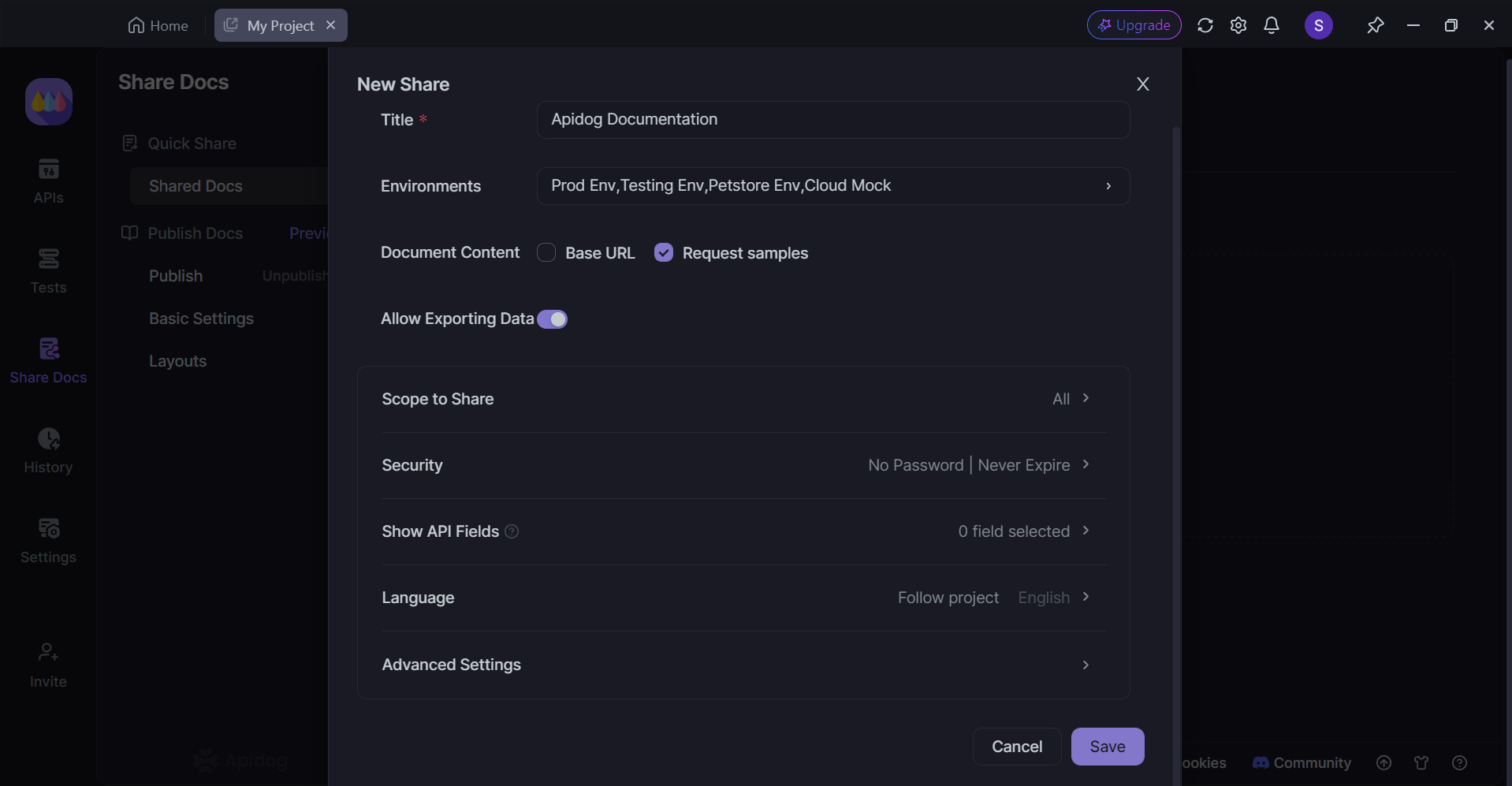
Once you have completed designing your API, you can generate API documentation in a few seconds using the details you have included during the design phase as a template.
You should also consider whether you want a personal domain, and if you wish to keep your API document private. You can set an additional password for extra security!
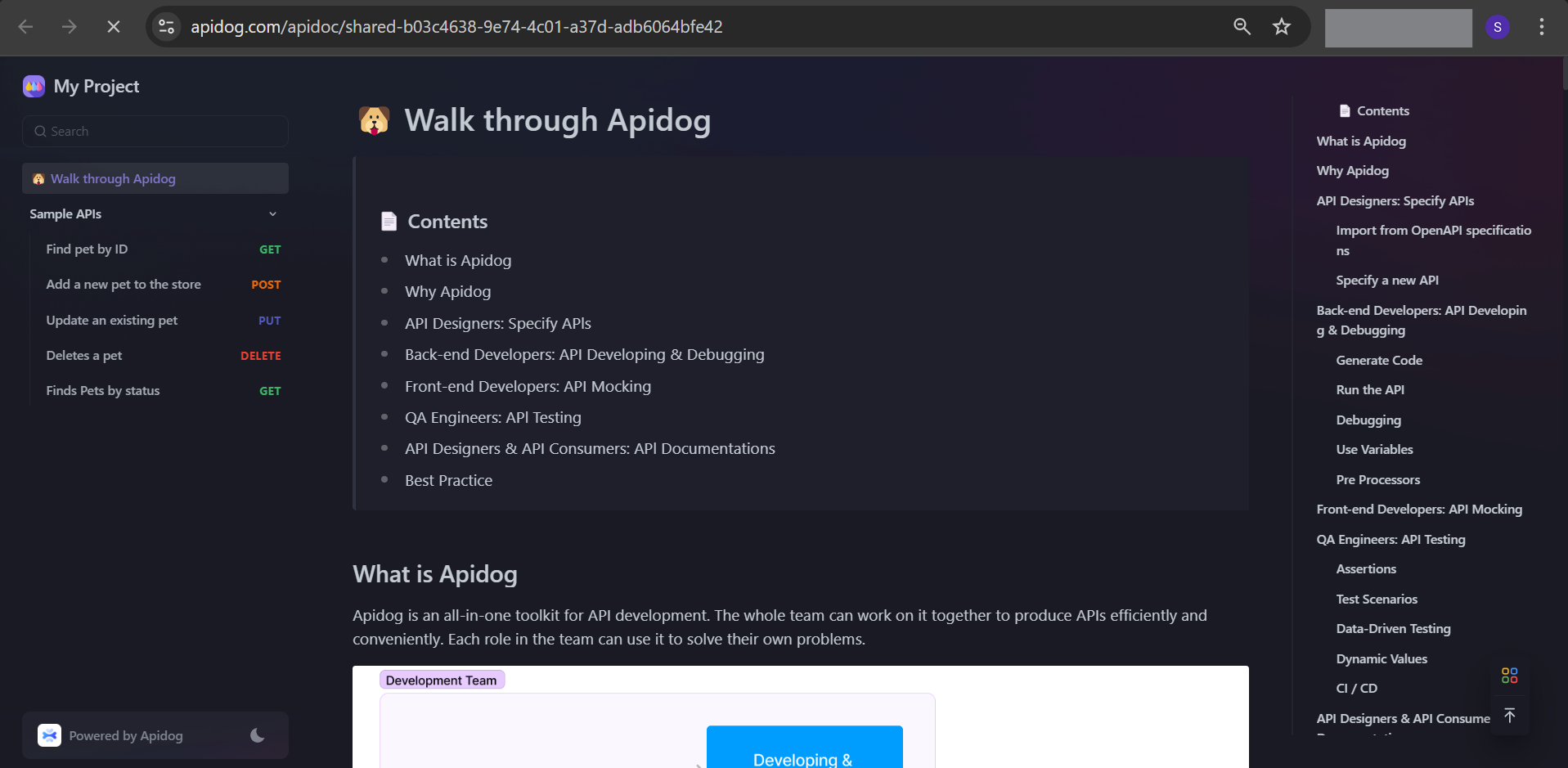
And there you have it - API documentation ready to be shared with a URL! All you have to do now is decide how you wish to share the documentation.
Conclusion
Serialization and deserialization are fundamental to modern computing, serving as the bridge between complex data structures and their transportable, storable representations. While offering immense benefits in data exchange, storage, and processing, these processes also introduce potential challenges such as performance overhead, data integrity issues, and security risks.
As technology continues to evolve, the significance of serialization and deserialization is likely to grow. Advancements in data formats, compression techniques, and security protocols will shape the future of these processes. A deep understanding of serialization and deserialization principles will be increasingly crucial for developers to build efficient, secure, and scalable applications in the digital age.