REST API URLs (REST API URLs V.S. RESTful APIs) are significant in web service communication. Without further ado, let us take a closer look at what they are - and see the best practices and examples they are to learn from.
What are REST API URLs?
REST (Representational State Transfer) API URLs, or Uniform Resource Locators, provide unique addresses to interact with resources within a RESTful API, enabling targeted access to data and functionality.
Basic Structure of REST API URLs
A standardized REST API URL structure typically includes:
- Protocol: Protocols are usually in the form of HTTP or HTTPS, which specify how to communicate with the API.
- Host: The host defines the server address where the API resides (e.g.,
api.example.com
). - Path: A path defines the specific resource within the API, starting with a forward slash (e.g.,
/users
). - Query String (optional): Query strings, which are optional, allow developers to add additional parameters that can filter or refine the resource, using key-value pairs after a question mark (e.g.,
/users?name=John
).
Why Understanding REST API URLs Matters
There are a variety of reasons why web developers are required to understand the core concept of REST API URLs. These are a few of the main reasons:
- Clarity and Precision: By understanding a REST API URL, you can identify specific resources, ensuring accurate interactions.
- Usability and Consistency: Well-structured REST API URLs promote ease of understanding and prediction.
- Interoperability and Standards: Following best practices for REST API URLs enables smooth communication with various tools and applications, making it easier for other developers to use your API
- Versioning and Evolution: Clear versioning schemes aid in managing REST API URL updates and maintaining compatibility.
- Security and Control: REST API URLs can be designed to limit access to sensitive data from the public or malicious users.
Examples of REST API URLs
If you are wondering what REST API URLs look like, here are a few real-world samples of REST API URLs that you may have come across before reading this post!
- GitHub:
https://api.github.com/users/Bard
retrieves information about the user "Bard". - OpenWeatherMap:
https://api.openweathermap.org/data/2.5/weather?q=London
gets weather data for London. - Unsplash:
https://api.unsplash.com/photos/random?count=1
retrieves one random photo.
These REST API URLs are commonly seen as the website address, which changes whenever a relay of data is required, or when you change web pages.
Basics of REST API URLs
When deciding on your REST API's URL, you have to consider a few variables and characteristics, such as:
- Plural Nouns Over Verbs: When you are crafting your REST API URL, use plural nouns, and not verbs to represent resources in HTTP methods.
- Be Consistency: Advocate for consistent naming conventions and structures within the REST API URL scheme. For example, you should consistently use HTTP response status codes to represent operation outcomes on resources, and your REST API URLs should only include plural nouns and no verbs.
- Plurals for collections: Explain the convention of using plurals for resources representing collections.
- Versioning considerations: Discuss different approaches to versioning and their impact on the REST API URLs. You may also consider a version number for more consistency.
Best Practices for Structuring REST API URLs
REST API URLs have a certain theoretical method for structuring. These theories are standardized among web developers to reduce the time needed to recall or fix web services whenever such situations occur.
- Resource Hierarchy: Explain how to represent nested resources in the URL structure. Like navigating files on your device, you may choose to name your REST API URL based on the resources (files) you have.
- Filtering & Pagination: Discuss using query parameters for filtering and pagination.
- Error handling: Explain how to use standard HTTP status codes and provide meaningful error messages that developers may face when using your REST API.
- Security considerations: Briefly mention URL security best practices, such as avoiding sensitive data in URLs
- Documentation & Examples: Promote the importance of clear documentation and provide practical URL examples.
Comparison of Optimal and Bad REST API URL Examples
Practice Nesting and Naming Resources
- Good:
https://api.example.com/orders/456/items/789
- Bad:
https://api.example.com/order_456_item_789
From the good URL example, it can be easily seen that the item displayed 789 can be found within the order 456 resource. However, the bad URL example combines these resource identifiers, making it harder to understand and read.
Clarity and Precision
- Good:
https://api.example.com/users/123
- Bad:
https://api.example.com/get_user?id=123
The good URL does not consist of any verbs and is very straightforward with what it is currently identifying. However, the bad URL has a generic verb. This clouds developers with an unclear operation.
Consistency
- Good:
https://api.example.com/products/{product_id}
- Bad:
https://api.example.com/product_detail/abc
andhttps://api.example.com/get_item/xyz
The good URL example uses a placeholder, which helps developers with a predictable URL structure, whereas the bad URL examples have inconsistent naming conventions.
Designing REST APIs with Apidog
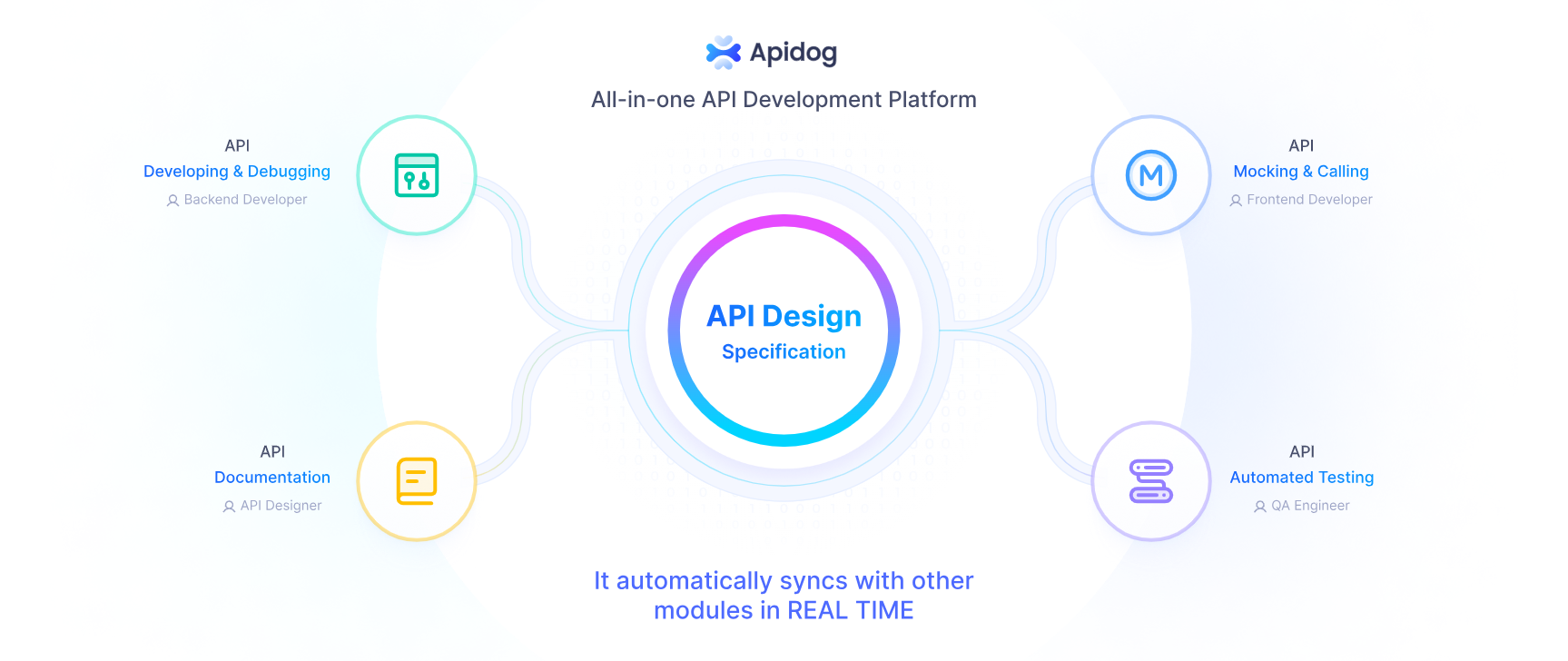
Apidog is a design-first API development platform that allows you to design, test, and document REST APIs efficiently:
1. Designing REST API
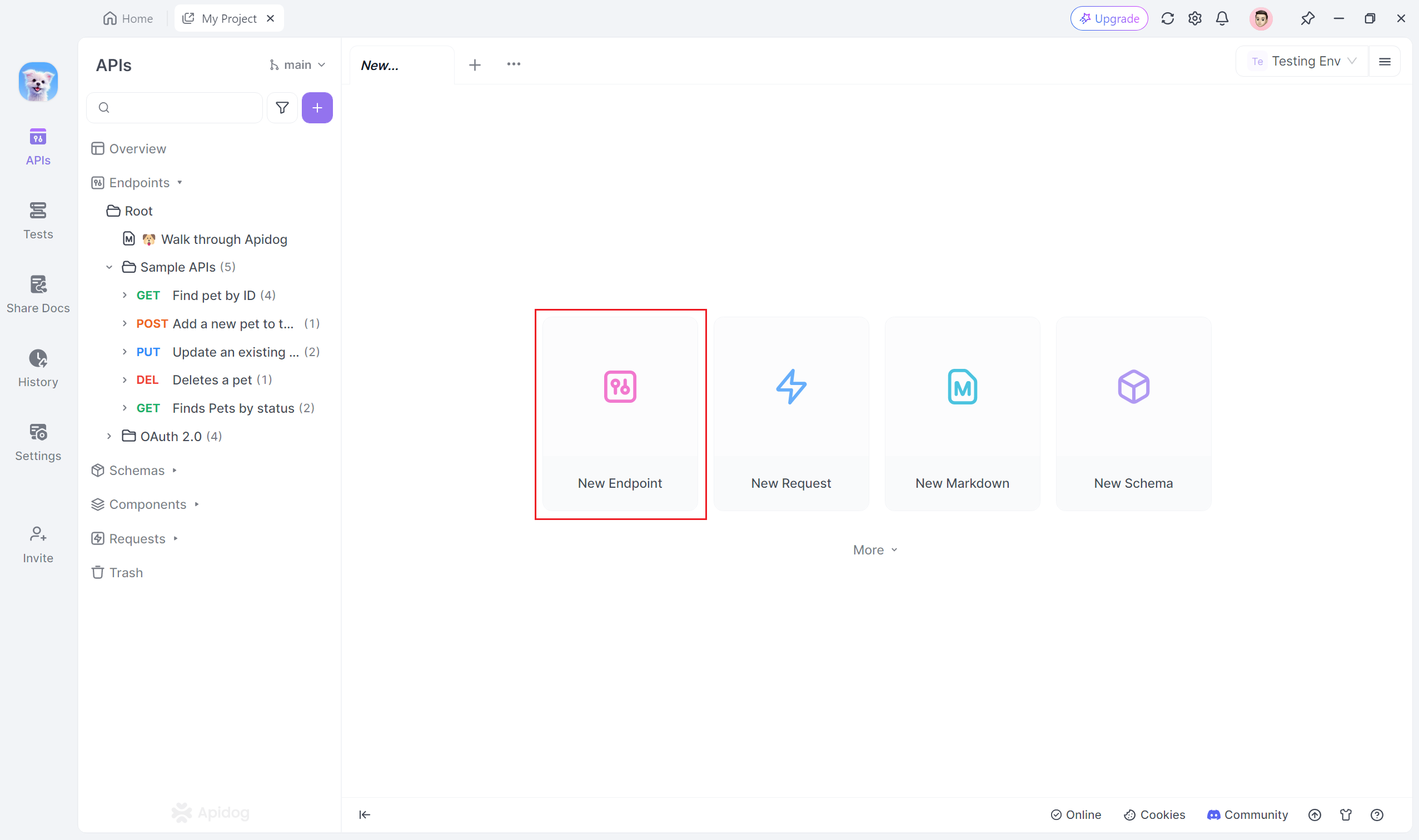
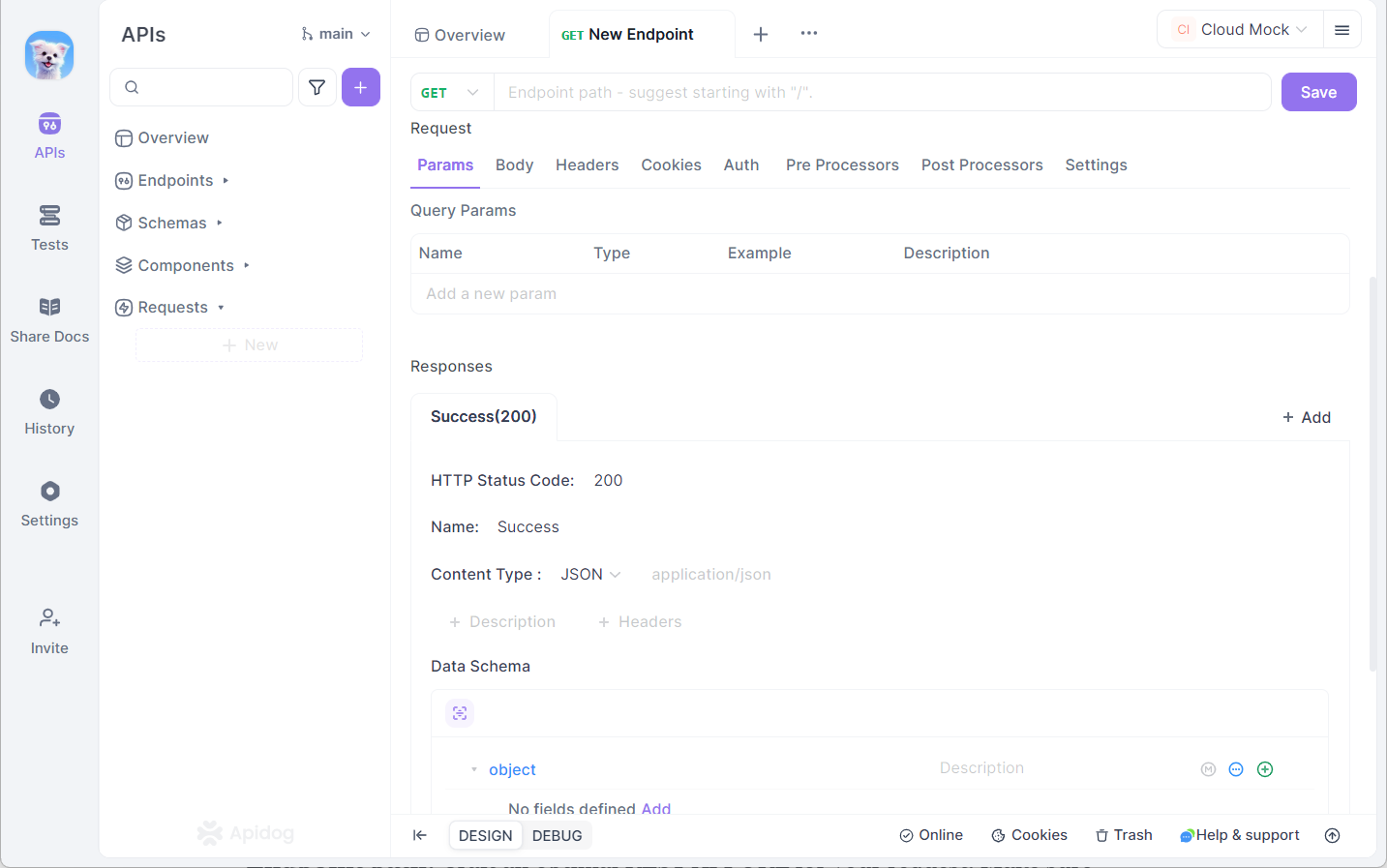
- Create a New Endpoint: On the project, create a new endpoint by clicking
+
at the left top of the page. - HTTP Methods: Decide what REST API method you want. The most common methods are specifically GET, POST, PUT, and DELETE. Nonetheless, Apidog provides the options to choose OPTIONS, HEAD, and PATCH.
- Define Request and Response Params: On the API design dashboard, fill in the request parameters, response parameters, example responses, and any necessary information.
- Add Security and Authentication: Specify authentication methods (e.g., API key, OAuth). And configure any necessary security settings for your API.
2. Generating REST API Documentation Automatically
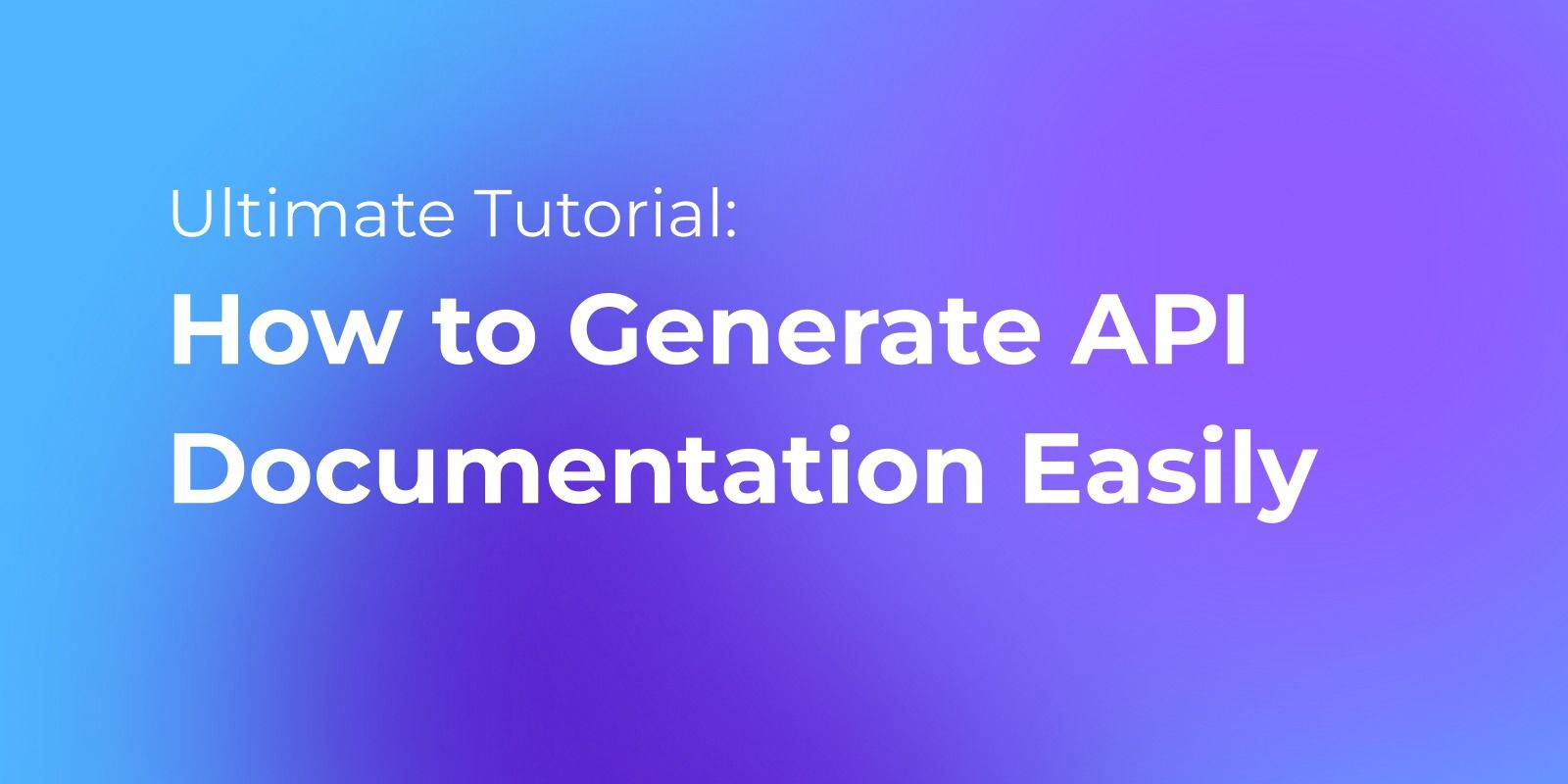
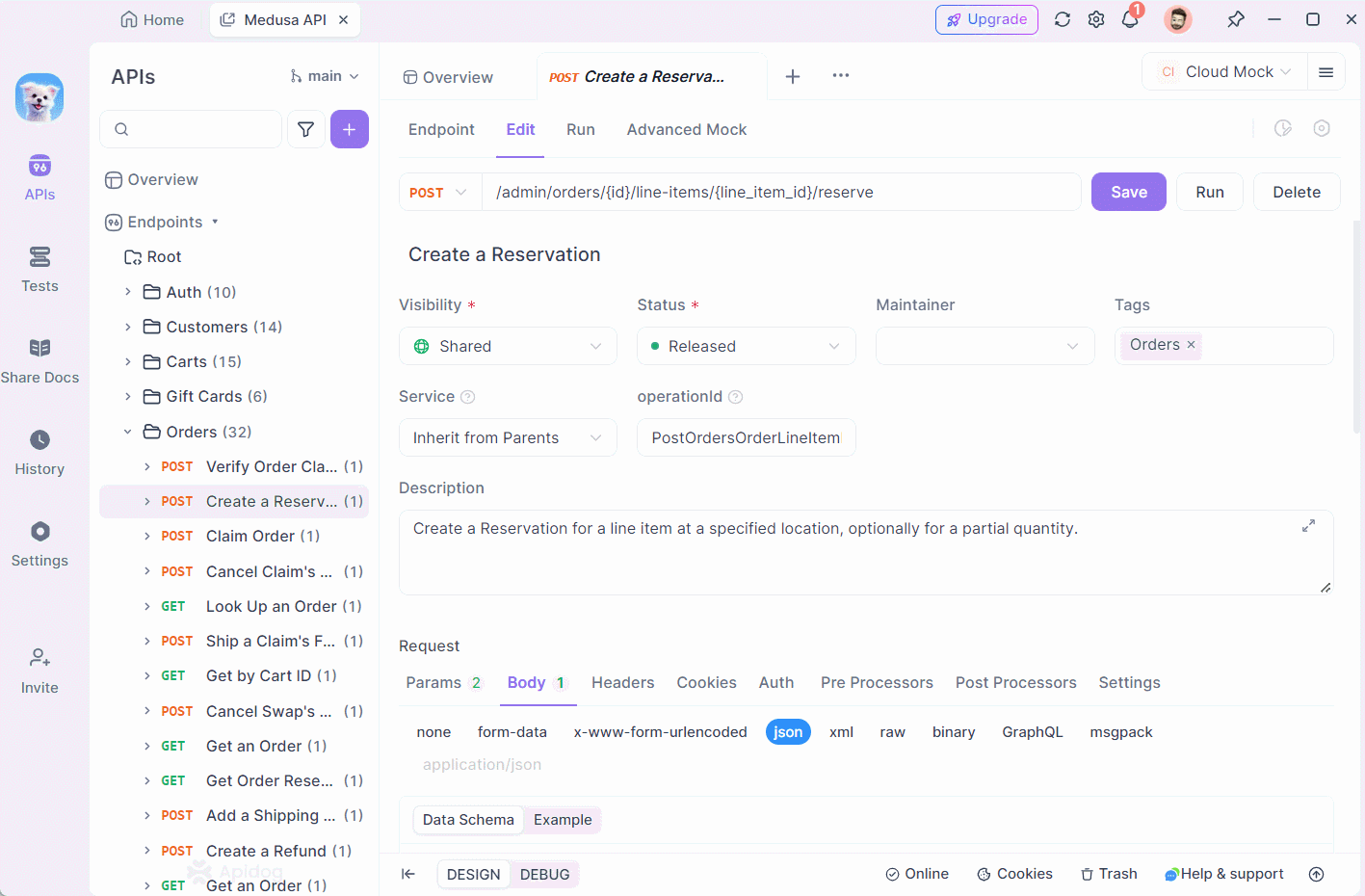
After completing the REST API specifications using the built-in visual dashboard, generate your REST API documentation effortlessly by clicking the Save
button in the top right corner. Apidog will automatically create comprehensive documentation based on your API design.
3. Testing REST API
Apidog offers robust capabilities for both manual and automated testing of REST APIs, ensuring a comprehensive testing process throughout the API lifecycle.
Manual Testing
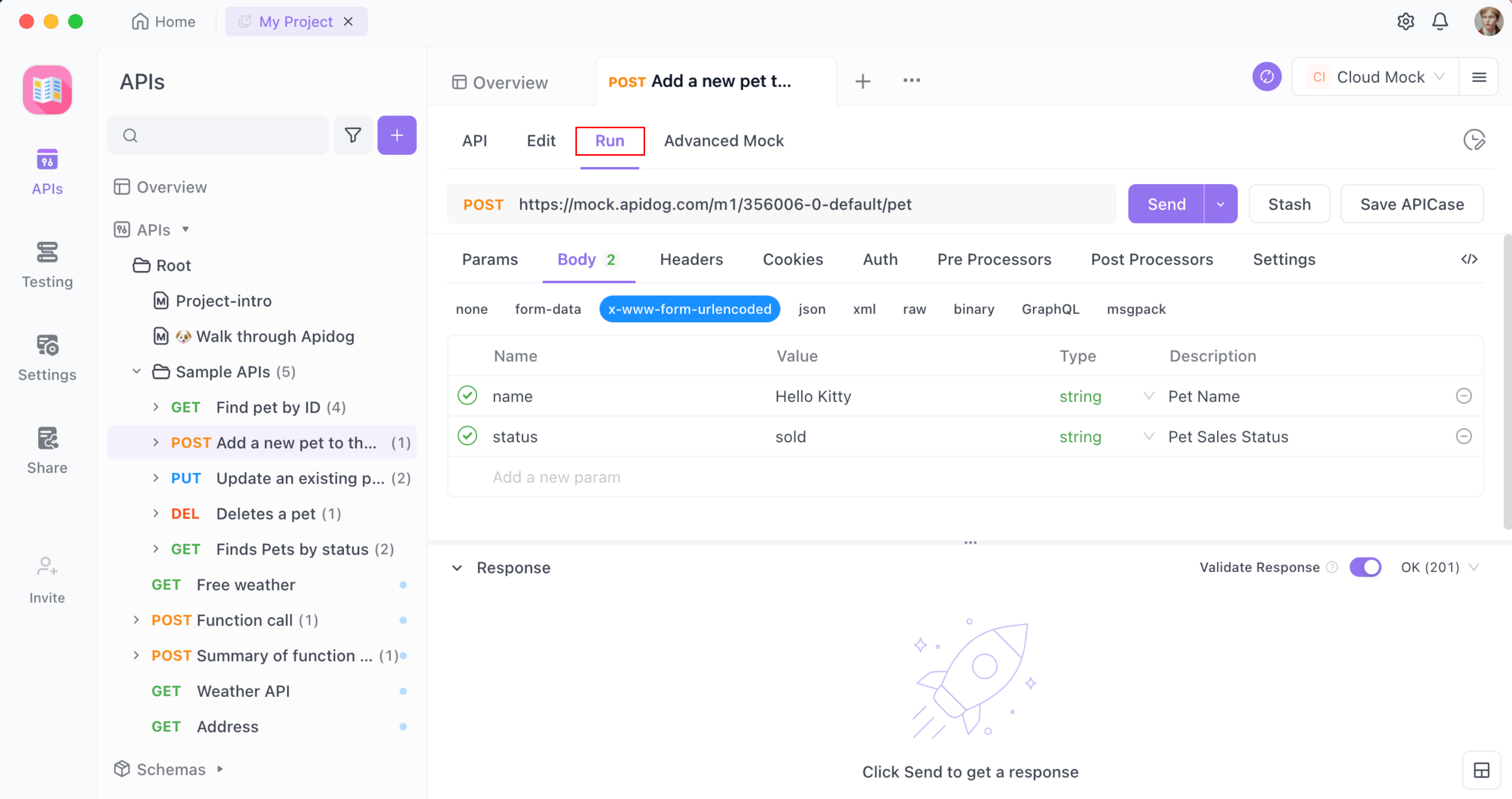
With Apidog's intuitive interface, developers can easily perform manual testing of REST APIs. Here's how it works:
- Send API Request in One Click: You can click
Send
at the top right corner of the REST API documentation to test the endpointmanually. Â - Get Real-Time Feedback: As you send requests, Apidog provides immediate feedback on responses, enabling you to analyze results quickly with actionable tips and make adjustments as needed.
- Testing Scenarios: You can create various testing scenarios to ensure your API behaves as expected under different conditions, making it easier to identify and fix issues.
Automated Testing
In addition to manual testing, Apidog supports automated testing, which streamlines the process and enhances efficiency:
- Visual Test Scenario Designer: The test scenario designer features a user-friendly drag-and-drop user interface that simplifies the creation of test scenarios. You can easily add and arrange components, such as requests, cases, and assertions, without writing complex code.
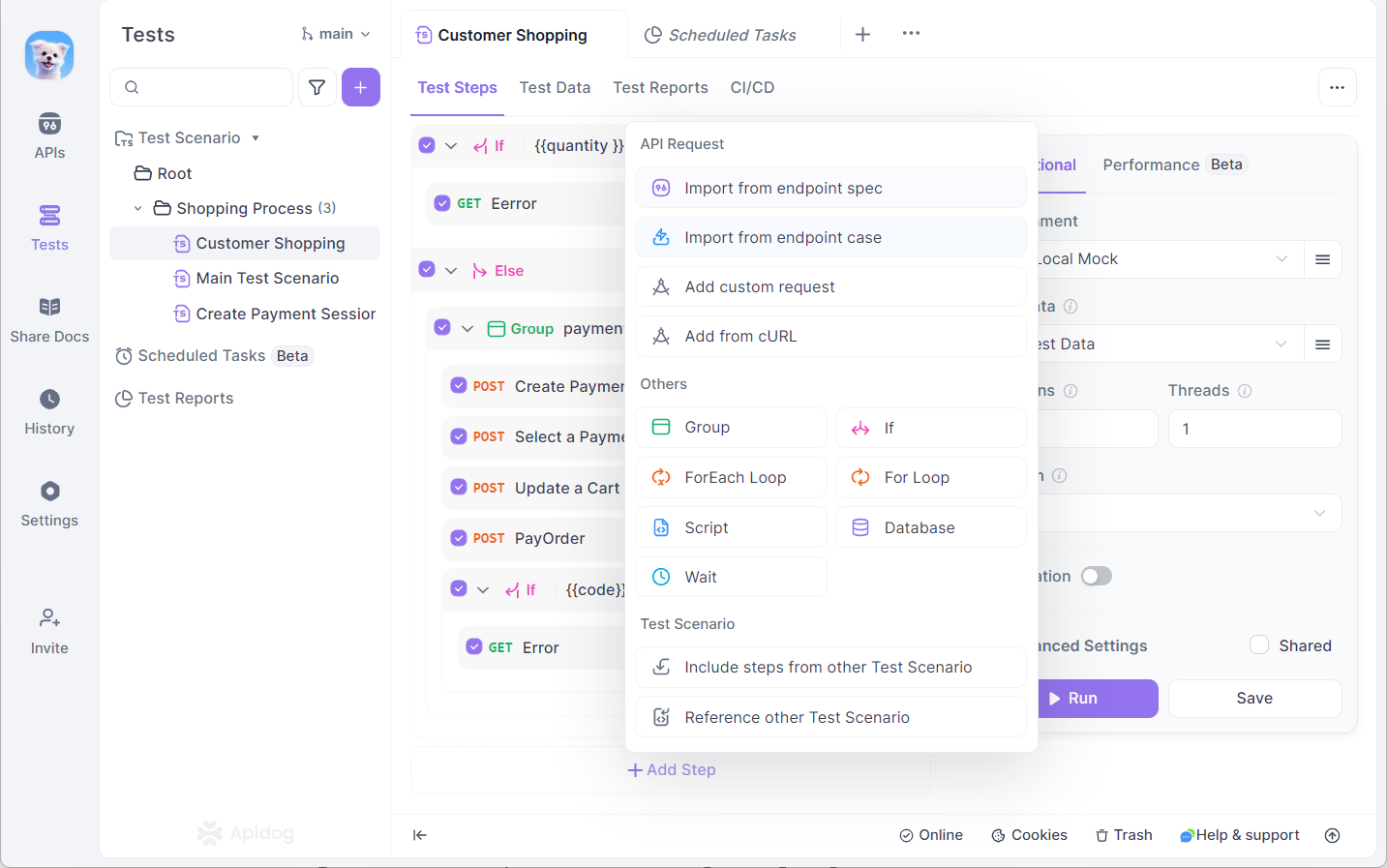
- Comprehensive Reporting: Automated tests in Apidog generate detailed reports that outline the outcomes of each test case. This makes it easy to track performance over time and identify areas for improvement.
- Sharing Test Report with Teammates via Links: Apart from generate detailed API test reports, Apidog makes it easier to share the report with your teammates via links, where your teammates can directly perform testing to reverify the issues.
- Integration with CI/CD: You can integrate Apidog with Continuous Integration/Continuous Deployment (CI/CD) pipelines, enabling automated testing to run as part of your development workflow. This ensures that any changes made to the API are tested immediately.
4. Mocking REST API without Backend Support
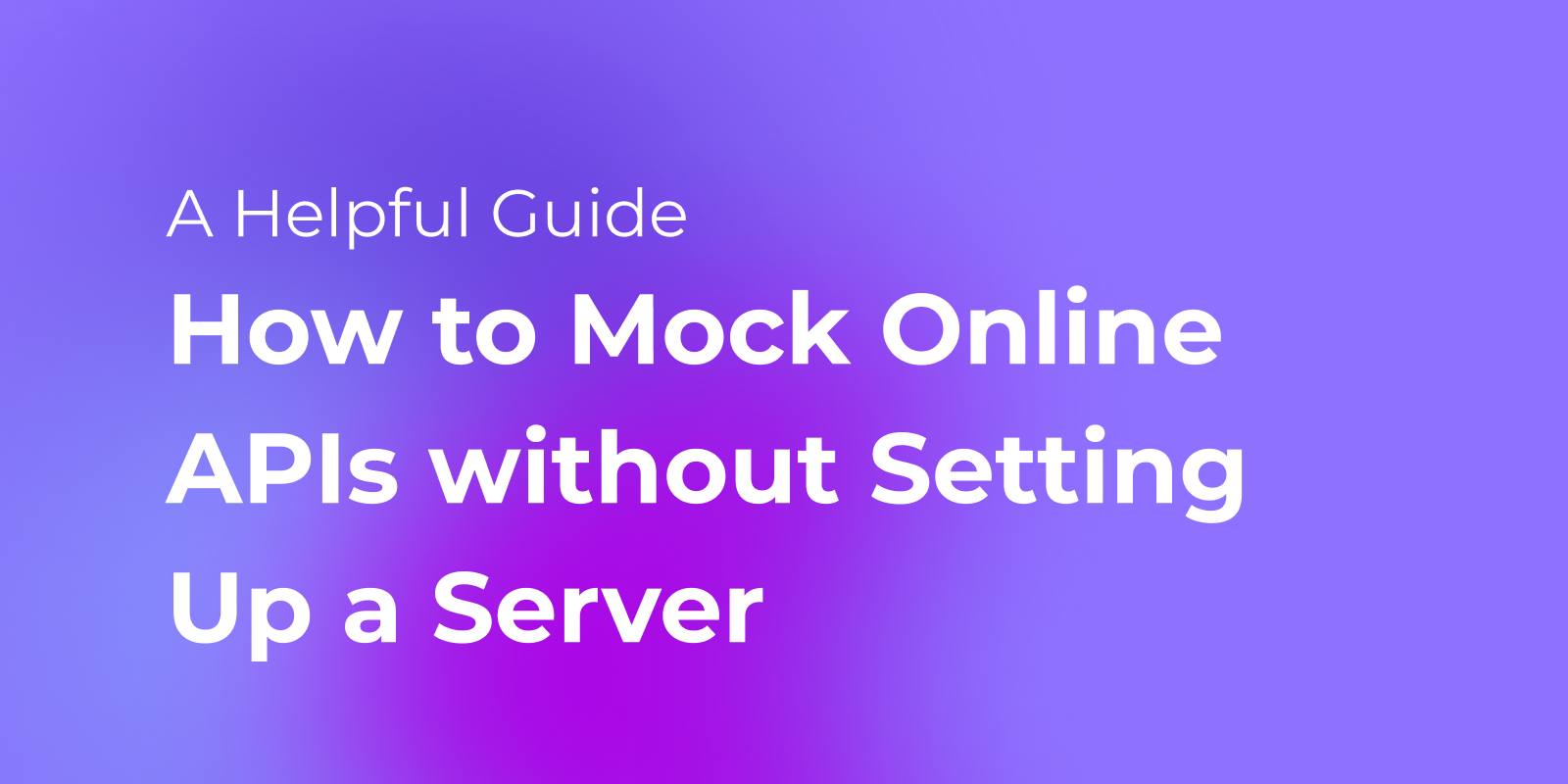
Apidog’s feature for mocking REST APIs allows developers to simulate API endpoints without requiring a fully developed backend. This capability is essential for front-end developers, QA teams, and product managers who need to test applications and workflows while the backend is still under development.
Once your REST API documentation is created, Apidog automatically generates the Mock server to facilitate the mocking process without additional configurations.
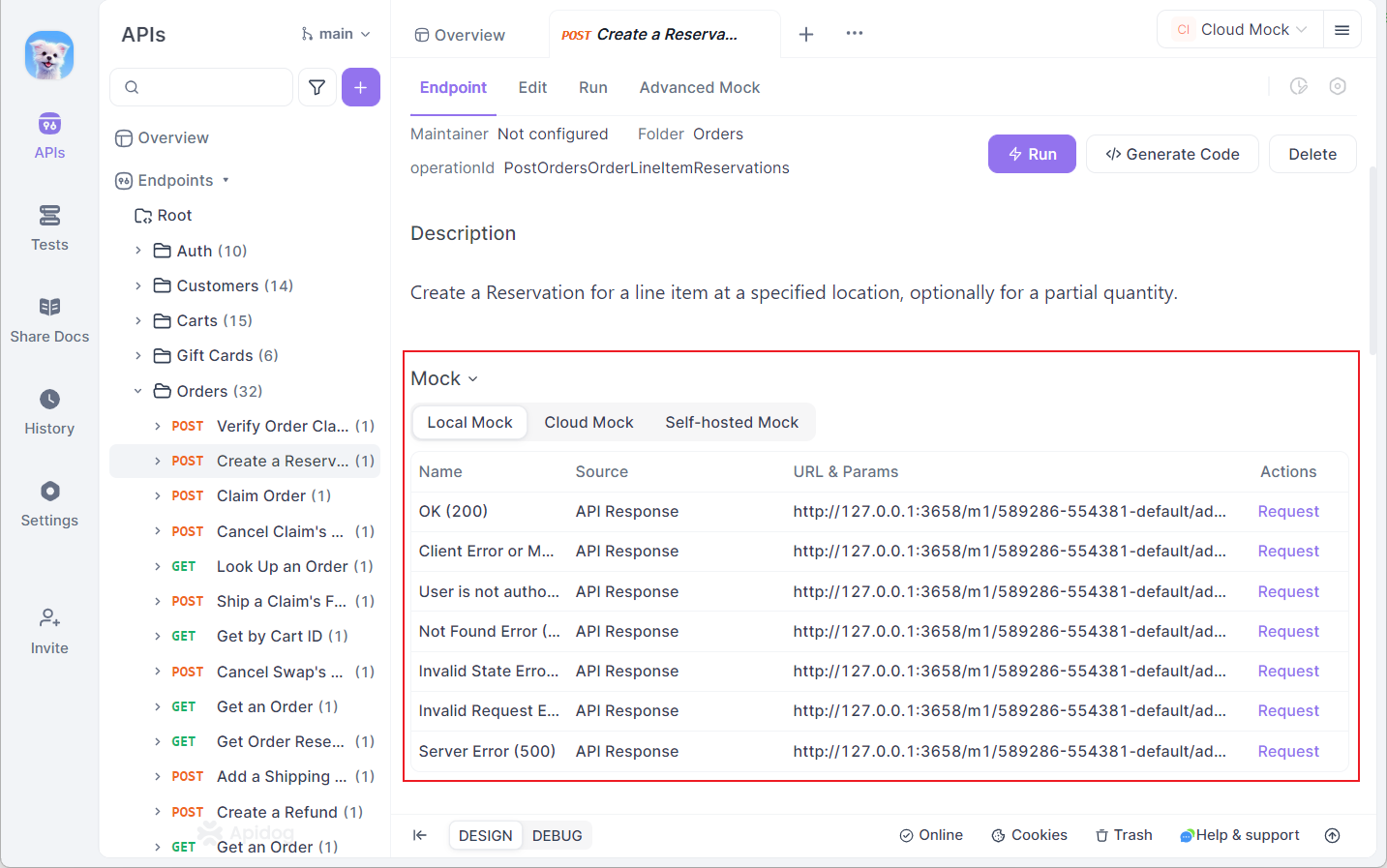
5. Sharing and Publishing REST API Documentation
Apidog offers robust sharing and publishing options for API documentation, ensuring that stakeholders or teammates can easily access and collaborate on API projects. Users can share documentation through a unique URL, enabling real-time access for team members, clients, or external partners.
Additionally, Apidog allows for customizable privacy settings, ensuring that sensitive information is protected while still facilitating collaboration. Users can also generate comprehensive documentation automatically, making it simple to keep everyone updated on changes and enhancements to the API. This streamlined sharing process enhances transparency and fosters effective communication among all project participants.
You can also create different API versions of the REST API documentation.
Conclusion
Understanding and implementing best practices for REST API URLs is crucial for building efficient and user-friendly web services. By adhering to structured naming conventions, ensuring clarity, and leveraging tools like Apidog for design, testing, mocking and documentation, developers can create robust APIs that enhance usability and facilitate seamless integration.