Loops are essential for effectively carrying out repetitive operations in the vast programming field. One such loop recognized for its adaptability and simplicity is the 'foreach' loop.
Widely used in many programming languages, the foreach loop provides an advanced method for iterating across collections of elements. This article will examine the syntax of the foreach loop, its various implementations in programming languages, and its application in Apidog.
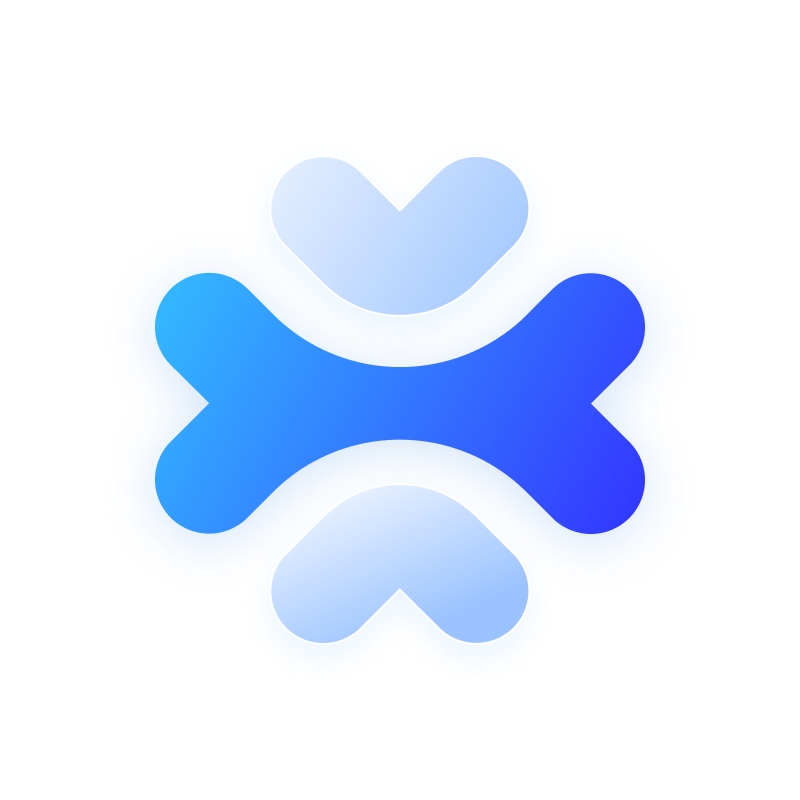
Understanding the ForEach Loop:
Programmers frequently utilize the ForEach loop construct to iterate through components inside a collection, providing a simple and expressive way to handle data sequences. The ForEach loop eliminates the need to manage indices or counters, allowing it to focus on the components instead of typical loops.
Syntax of Foreach Loop in Different Programming Languages
Programming languages might differ significantly in the syntax of a foreach loop, but the basic structure is always the same. Here is a generic representation:
for element in iterable:
foreach ($iterable as $element) {
}
for (dataType element : iterable) {
}
iterable.forEach(function(element) {
});
Explanation of key components in the code:
Element: Depicts the iteration's active element.
Iterable: Describes the set of items being accessed.
Code block: The series of instructions carried out for every iterable element.
Let's use PHP to explore various applications and demonstrate the usefulness and adaptability of the 'foreach' loop. You might be wondering why I chose PHP over other languages. The explanation is simple: PHP is an excellent programming language for processing data. It is widely used, has a concise syntax, and its 'foreach' loop makes it easier to iterate over arrays.
Applications of Foreach Loop PHP
1. List iteration
$programming_languages = ["Python", "Java", "JavaScript"];
foreach ($programming_languages as $language) {
echo $language . "\n";
}
Explanation:
List iteration utilizing a foreach loop to traverse a variety of programming languages is demonstrated in the provided PHP code. The array $programming_languages, which contains three different programming languages, is defined in the first line. Then, the foreach loop assigns the current language to the loop variable $language for each iteration as it works efficiently through all of the elements in the array.
The foreach loop's usefulness for efficient array iteration and output is then illustrated by the echo $language. "\n"; instruction, which outputs each programming language on a novel line. As demonstrated by this example, the foreach loop makes processing array items easier to understand and simpler.
2. Dictionary iteration
$programming_language = ["name" => "Python", "release_year" => 1991, "creator" => "Guido van Rossum"];
foreach ($programming_language as $key => $value) {
echo "$key: $value\n";
}
Explanation:
This PHP code demonstrates how to efficiently iterate through the key-value pairs in an associative array using a `foreach` loop, providing an understanding of programming language properties. The first line introduces the associative array $programming_language, which contains the essential attributes such as language name, release year, and creator.
The `foreach` loop then iteratively goes over every key-value pair in the array. The loop variables ‘$key’ and `$value’ automatically assume each iteration's current key and value. The output of each key-value pair is organized and formatted elegantly in the following `echo "$key: $value\n";` command, producing an organized representation.
The output echoes each attribute after execution, highlighting how readable and efficient the `foreach` loop is. This code exemplifies how such a loop construct facilitates fast data handling in programming, making traversing and showing data in associative arrays easier.
3. File iteration
$filename = "example.txt";
$file = fopen($filename, "r");
if ($file) {
foreach (file($filename) as $line) {
echo trim($line) . "\n";
}
fclose($file);
} else {
echo "Unable to open file: $filename";
}
Explanation:
The above PHP code uses a `foreach` loop to illustrate a basic file reading procedure. The code opens a file called "example.txt" for reading in the first few lines, and if that succeeds, it uses a `foreach` loop to run over each line in the file. The current line's content is sent to the loop variable $line for each iteration, and the ‘echo trim($line)’ is executed. The "\n" statement ensures a tidy representation by outputting the line without leading or trailing whitespaces.
A check is included in the code to ensure that the file is opened successfully. An error message detailing the problem is printed if the file cannot be opened. This short yet effective PHP script illustrates how to read and display a file's contents using a `foreach` loop, illustrating a typical file handling scenario.
ForEach Loop in Apidog: Enhancing API Testing
Apidog is an all-in-one platform that helps streamline the API development lifecycle, from designing to testing. Recently, Apidog introduced the ForEach Loop feature, which simplifies the process of iterating through lists during automated testing.
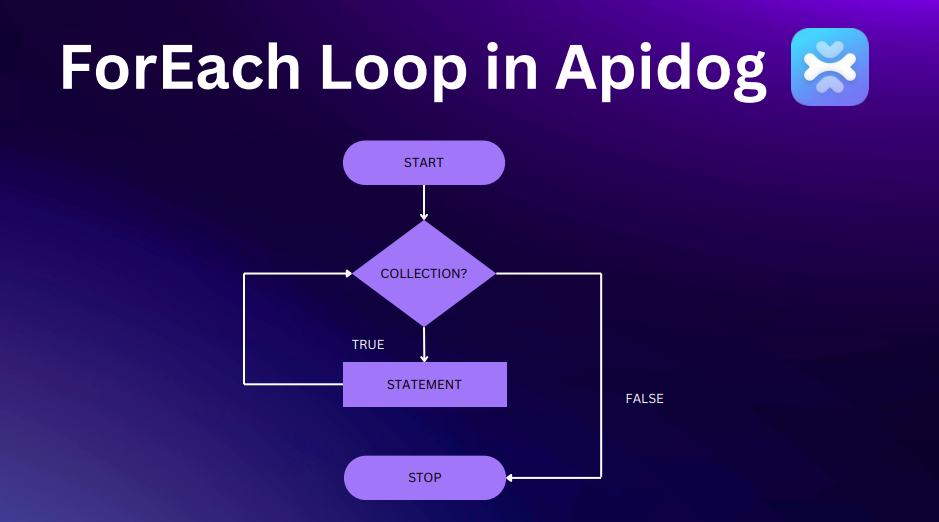
Apidog’s New API Testing Feature - ForEach Loop
Apidog’s ForEach Loop feature allows users to iterate over multiple items within test scenarios, providing a smoother and more flexible testing process. This feature can significantly reduce the complexity of running tests that involve large datasets or repeated actions.
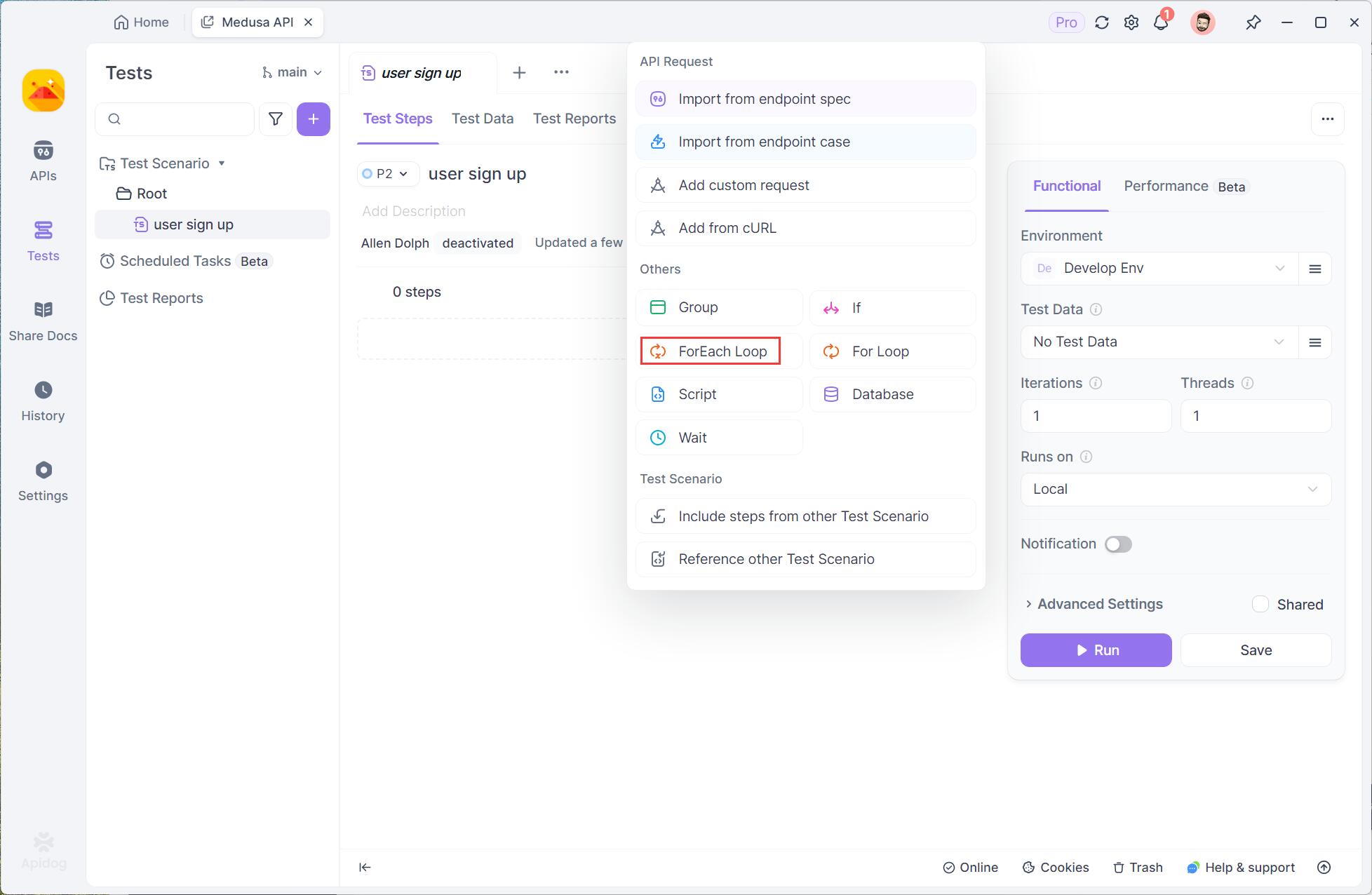
Enhancements in Apidog’s Automated Testing
Apidog has made several improvements to its automated testing capabilities, and the introduction of the ForEach Loop is one of the most notable features. Here's how it enhances the testing process:
Redesigned Automated Testing Module: A substantial modification was made to the automated testing module. Most notably, test cases
are now called test scenarios
, and test suites
were eliminated. This reorganization intends to improve and streamline the testing procedure.
Improved UI for Folder Trees: Test scenarios are now neatly organized in a folder tree structure with tabbed pages, making it easier to navigate and manage your tests.
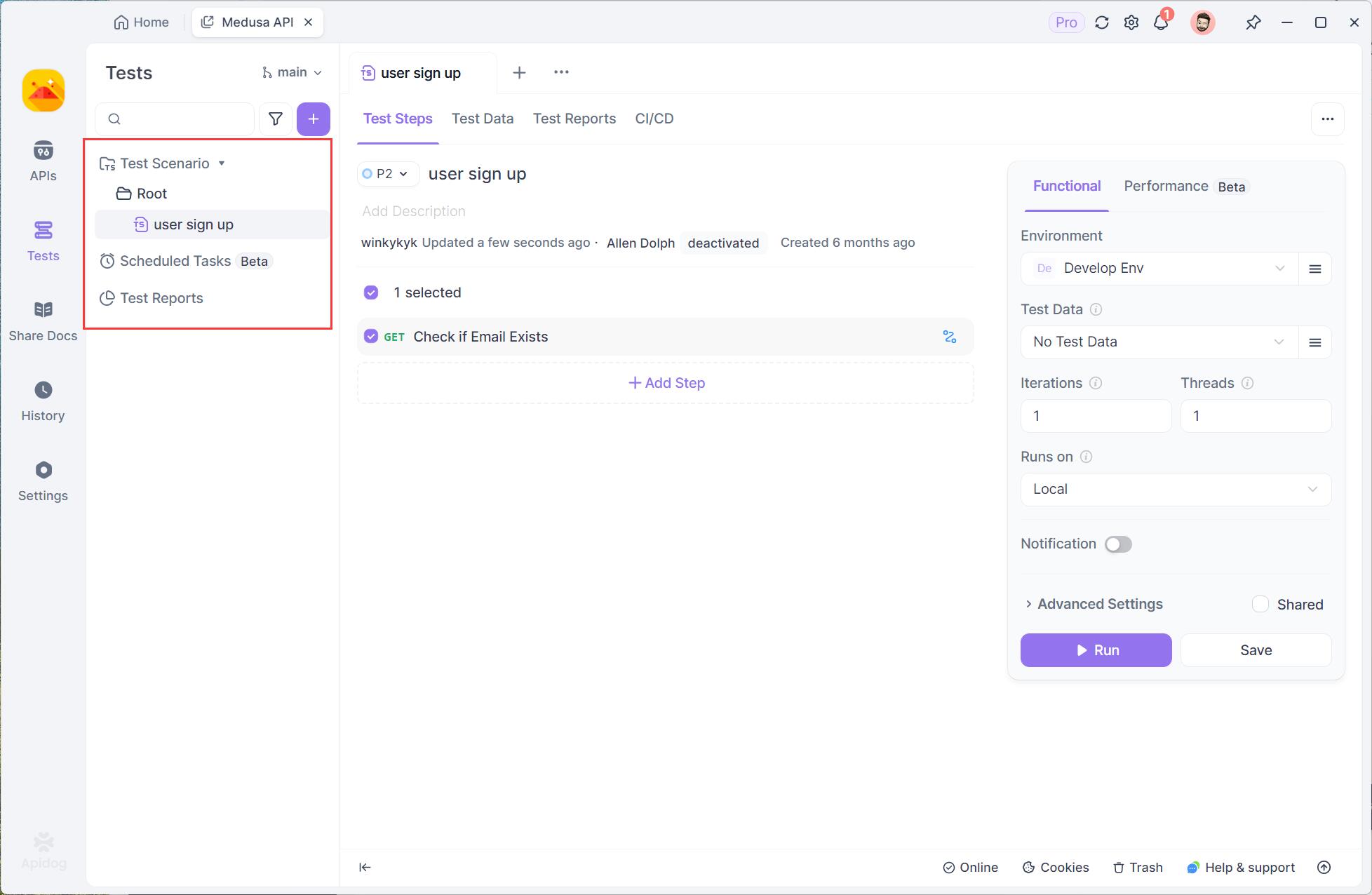
Support for Multiple Test Data Sets: You can now configure multiple test data sets within a single scenario, offering more flexibility when running tests.
Enhanced Integration of CI/CD: Apidog now automatically generates code for configuration files for popular CI/CD tools like Jenkins and GitHub Actions, making it easier to integrate with your pipelines.
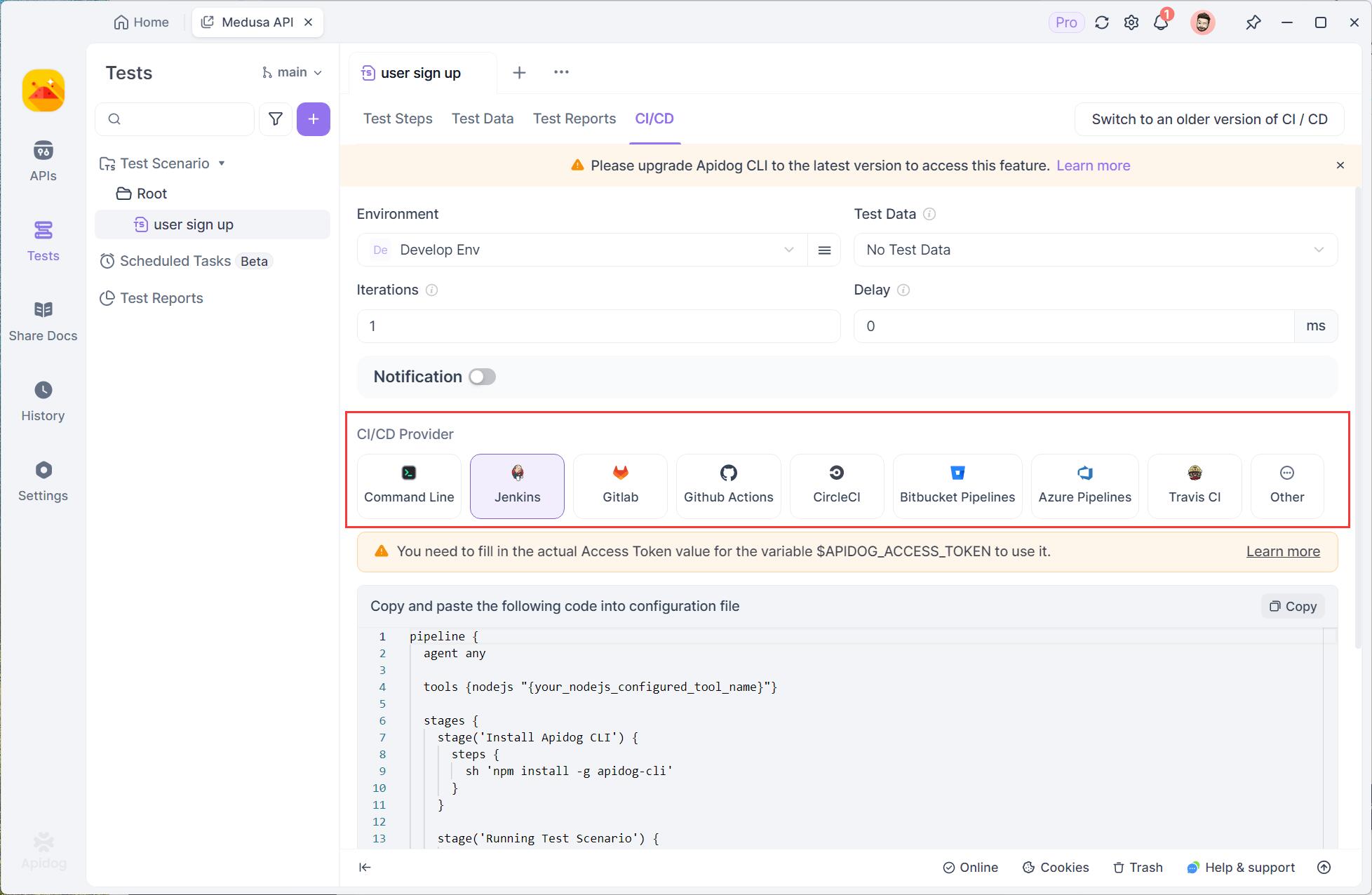
How to Create Test Scenarios in Apidog?
To use the ForEach Loop in Apidog, follow these steps:
Prerequisite:
Apidog is installed on your system.
Steps to Create a Test Scenario in Apidog:
Step 1: Log in to your Apidog account and navigate to the Tests
page. Click the '+' button to create a new test scenario.
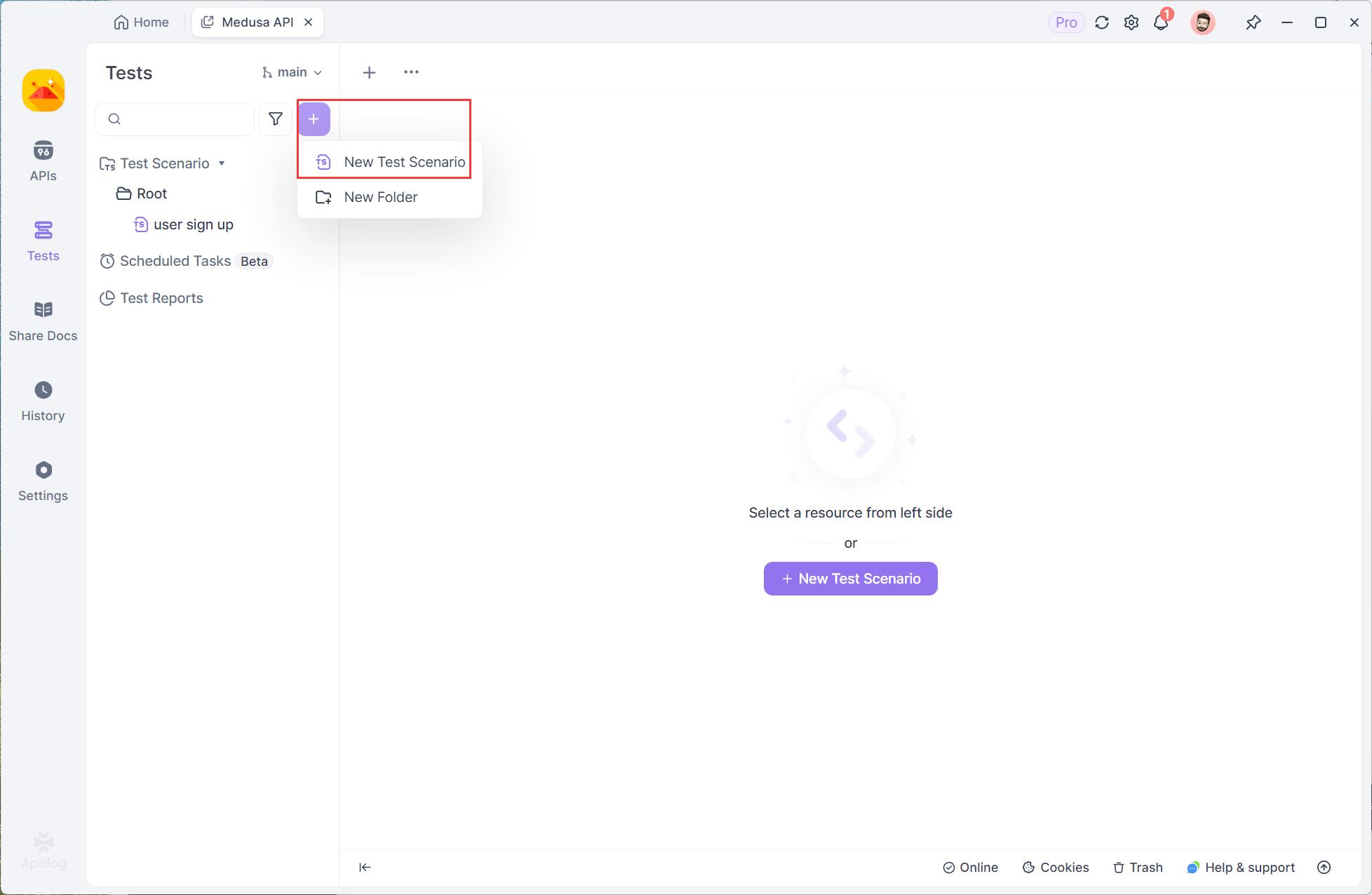
Step 2: Configure the test scenario’s parameters, such as name, priority, and folder.
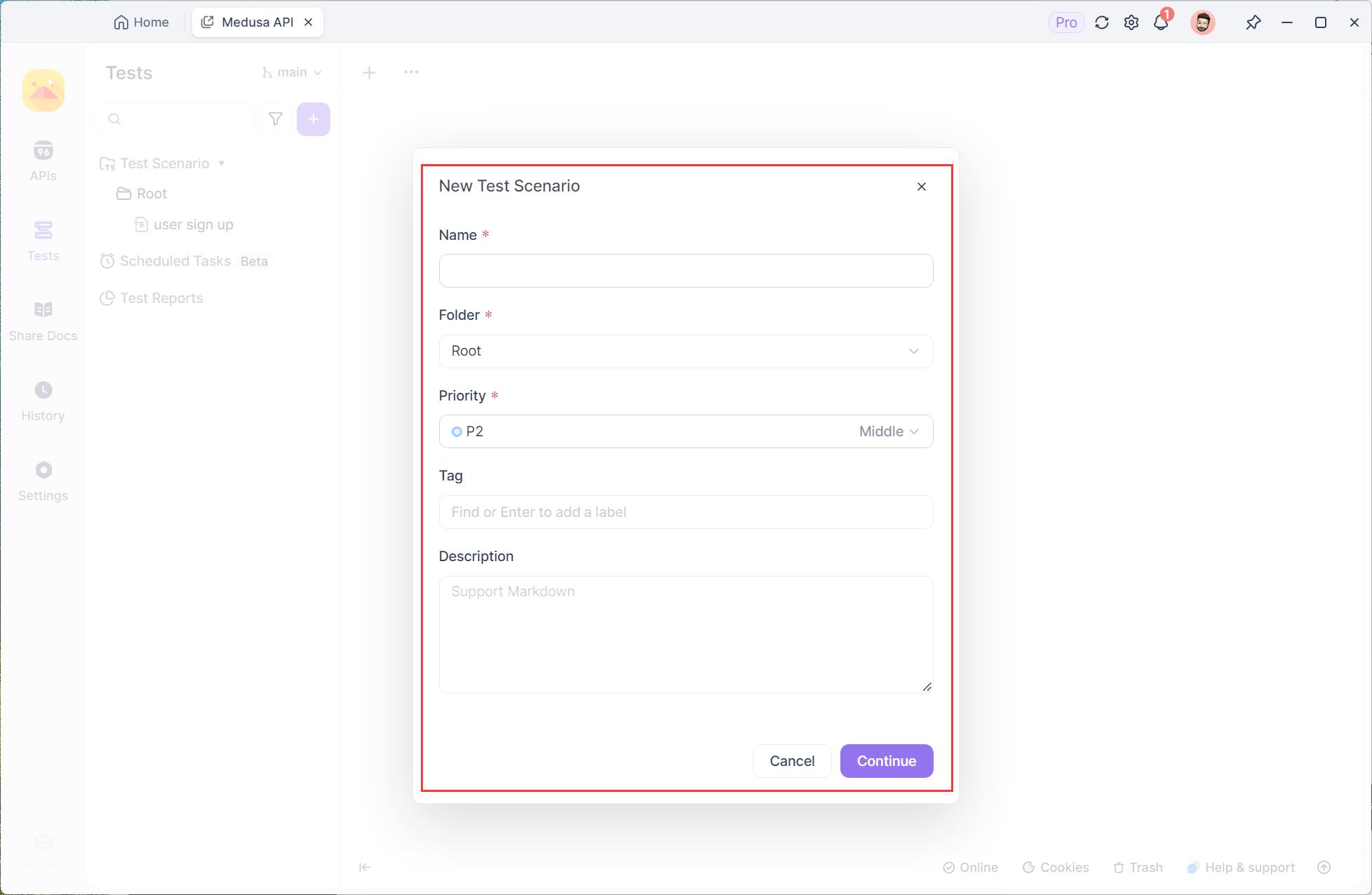
Step 3: Add test steps into the test scenario.
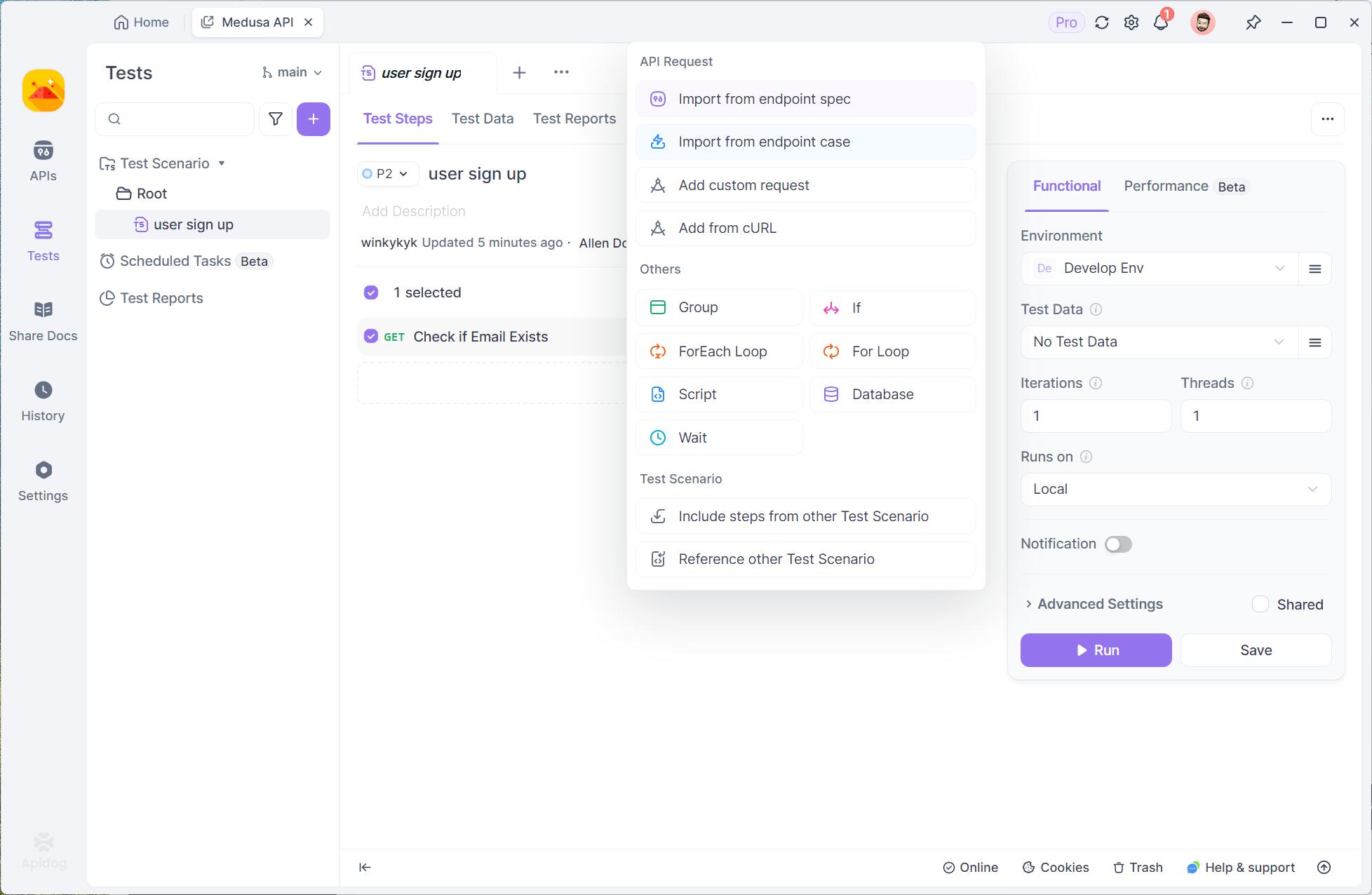
Let's select Import from endpoint spec
.
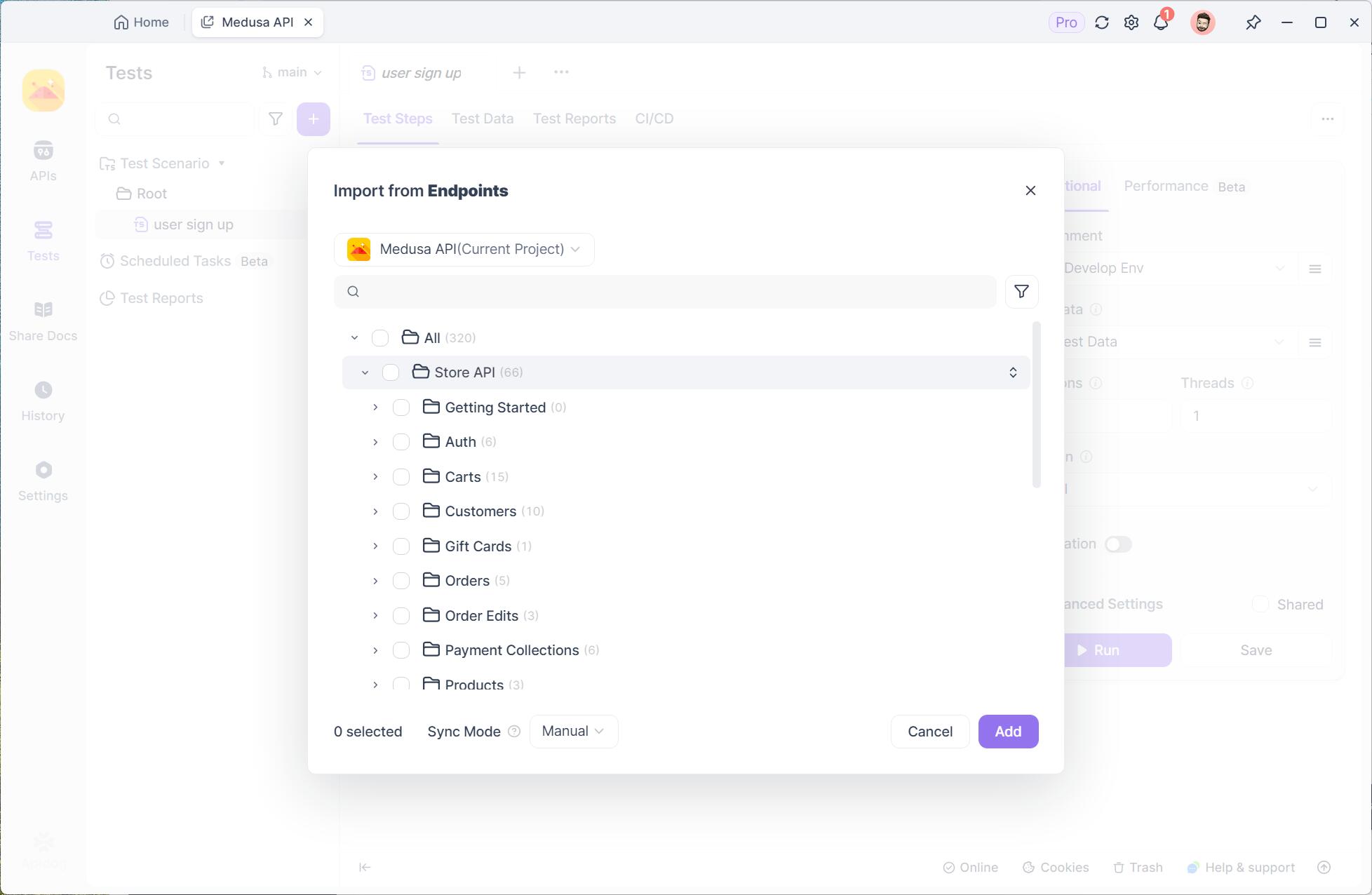
Step 4: Define a ForEach Loop for a specific step
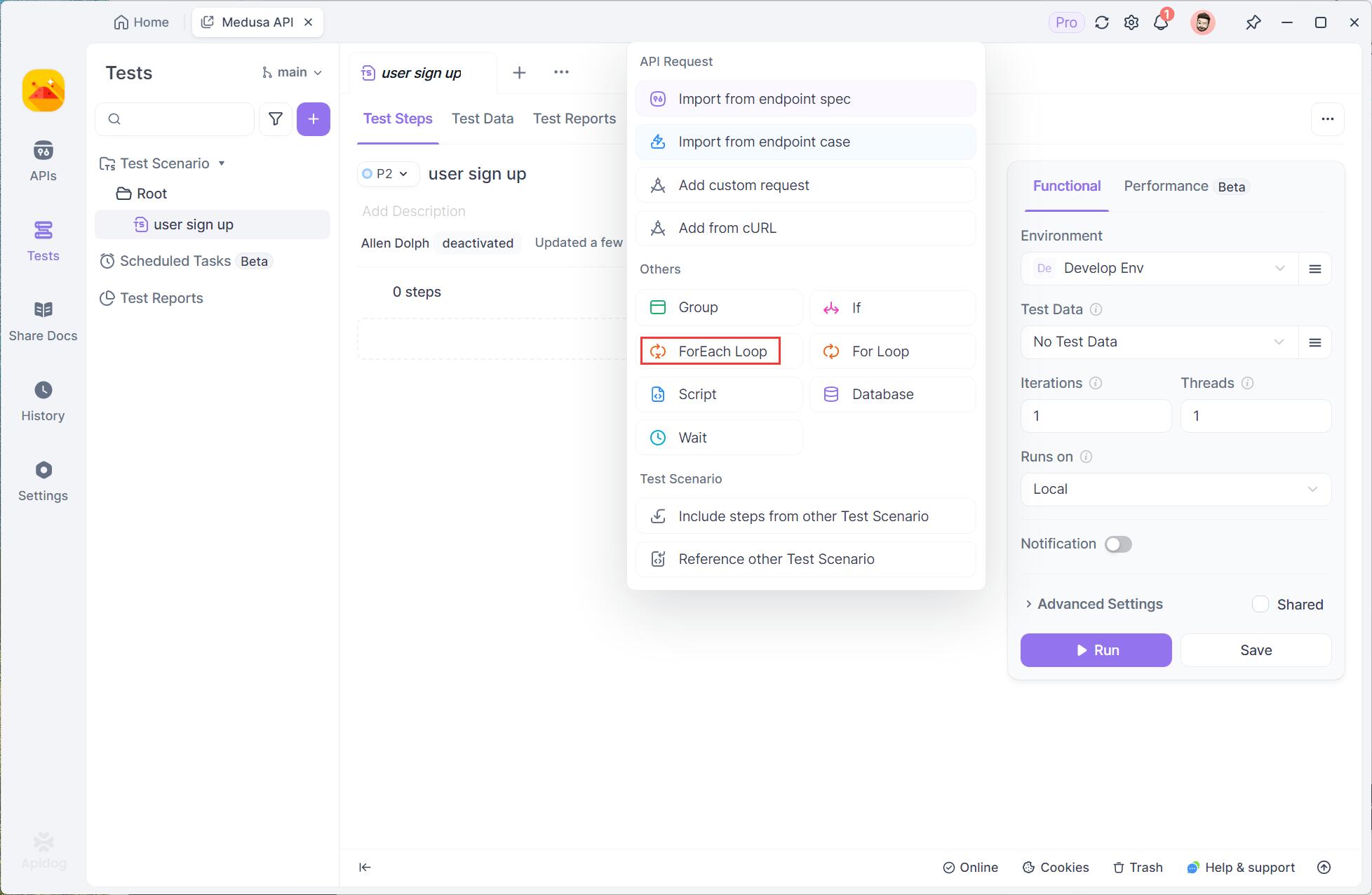
Step 5: Review the test scenario to ensure it covers all necessary aspects.
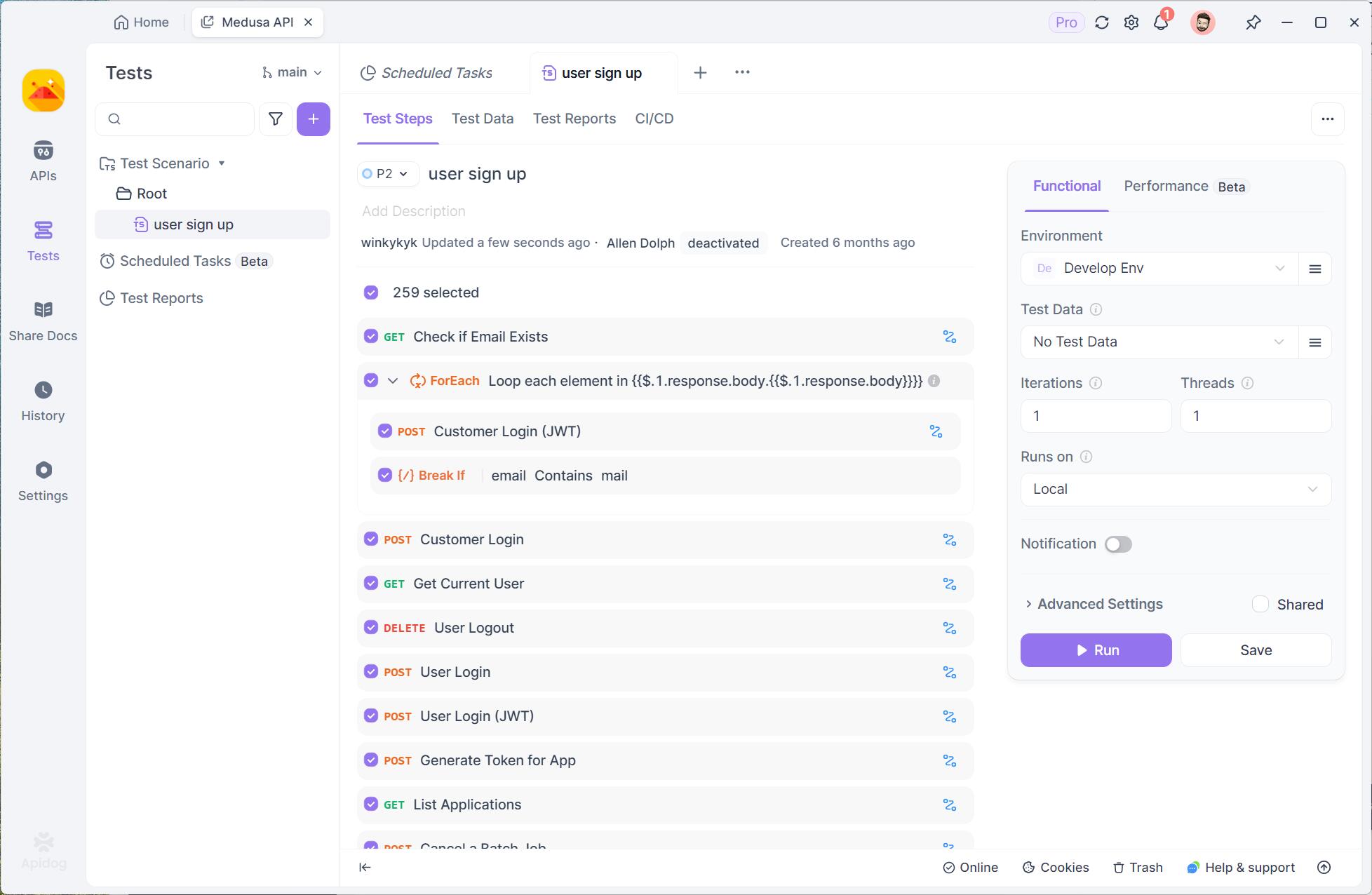
Step 6: Run the test scenario and track any defects or issues that arise.
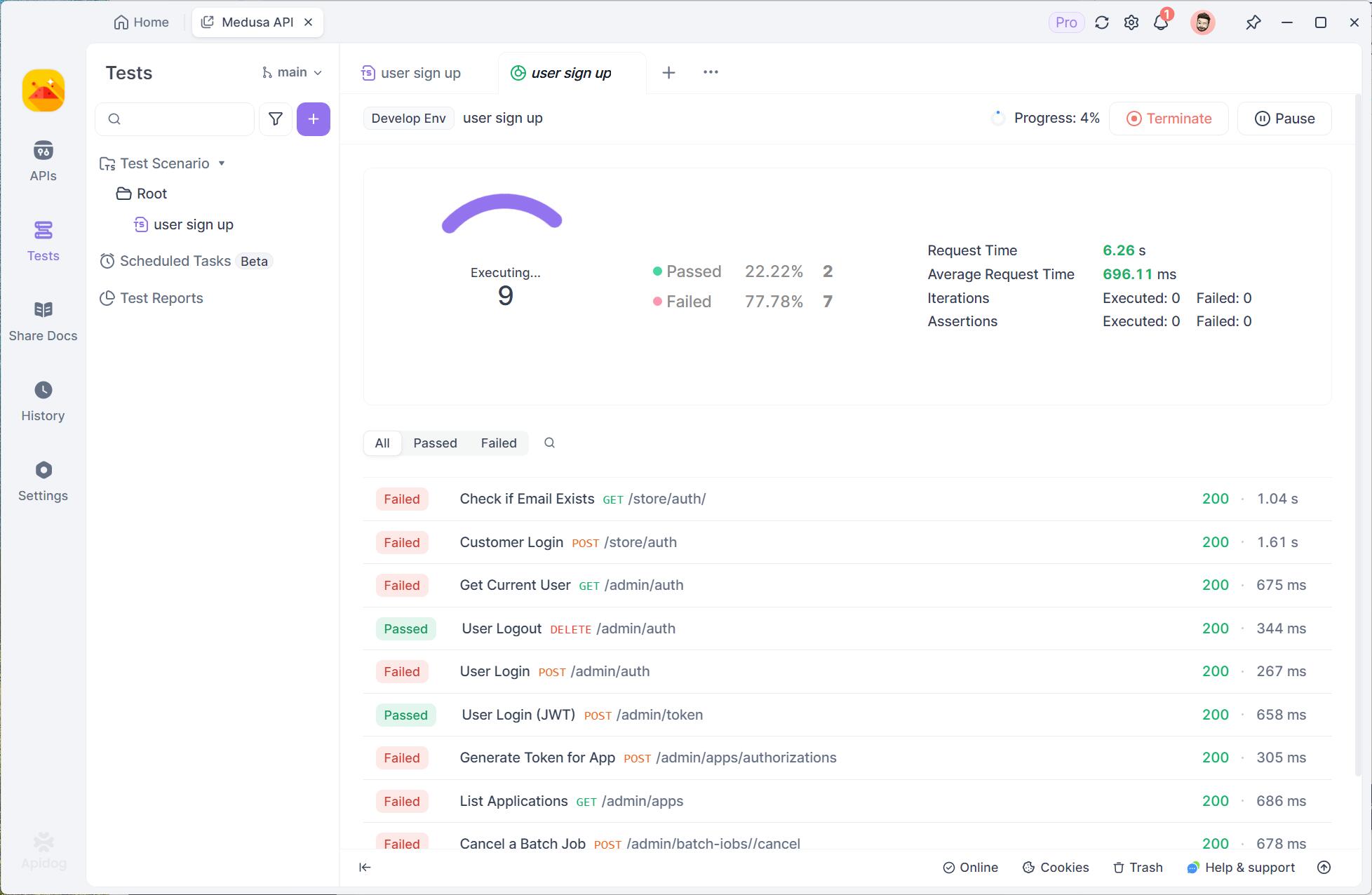
Benefits of ForEach Loop:
The ForEach Loop offers several key benefits for developers and testers:
Readability: The syntax is simple and clear, making the code more accessible, even to those with less experience.
Simplicity: Unlike other loops, foreach doesn't require manually managing indices or counters, reducing the chance for errors.
Efficiency: The loop is optimized for iterating over collections, reducing boilerplate code and making the codebase cleaner.
Versatility: It can be used across various data structures, including arrays, lists, and maps.
Reduced Errors: The foreach loop automatically handles iteration and termination conditions, minimizing common errors like off-by-one mistakes.
In Apidog, the ForEach Loop feature simplifies testing by allowing users to efficiently iterate through multiple test data sets, ensuring comprehensive and reliable results.
Conclusion:
In conclusion, testing experts must fully understand the complexities of creating test scenarios, including the innovative use of the ForEach Loop in Apidog. This tutorial has covered basic concepts, including well-defined goals and a thorough comprehension of user behavior. Adopting the ForEach Loop feature allows testers to traverse through lists of objects more quickly and effectively, improving the overall testing experience on the Apidog platform.